"no use strict";
!(function(window) {
if (typeof window.window != "undefined" && window.document)
return;
if (window.require && window.define)
return;
if (!window.console) {
window.console = function() {
var msgs = Array.prototype.slice.call(arguments, 0);
postMessage({type: "log", data: msgs});
};
window.console.error =
window.console.warn =
window.console.log =
window.console.trace = window.console;
}
window.window = window;
window.ace = window;
window.onerror = function(message, file, line, col, err) {
postMessage({type: "error", data: {
message: message,
data: err.data,
file: file,
line: line,
col: col,
stack: err.stack
}});
};
window.normalizeModule = function(parentId, moduleName) {
// normalize plugin requires
if (moduleName.indexOf("!") !== -1) {
var chunks = moduleName.split("!");
return window.normalizeModule(parentId, chunks[0]) + "!" + window.normalizeModule(parentId, chunks[1]);
}
// normalize relative requires
if (moduleName.charAt(0) == ".") {
var base = parentId.split("/").slice(0, -1).join("/");
moduleName = (base ? base + "/" : "") + moduleName;
while (moduleName.indexOf(".") !== -1 && previous != moduleName) {
var previous = moduleName;
moduleName = moduleName.replace(/^\.\//, "").replace(/\/\.\//, "/").replace(/[^\/]+\/\.\.\//, "");
}
}
return moduleName;
};
window.require = function require(parentId, id) {
if (!id) {
id = parentId;
parentId = null;
}
if (!id.charAt)
throw new Error("worker.js require() accepts only (parentId, id) as arguments");
id = window.normalizeModule(parentId, id);
var module = window.require.modules[id];
if (module) {
if (!module.initialized) {
module.initialized = true;
module.exports = module.factory().exports;
}
return module.exports;
}
if (!window.require.tlns)
return console.log("unable to load " + id);
var path = resolveModuleId(id, window.require.tlns);
if (path.slice(-3) != ".js") path += ".js";
window.require.id = id;
window.require.modules[id] = {}; // prevent infinite loop on broken modules
importScripts(path);
return window.require(parentId, id);
};
function resolveModuleId(id, paths) {
var testPath = id, tail = "";
while (testPath) {
var alias = paths[testPath];
if (typeof alias == "string") {
return alias + tail;
} else if (alias) {
return alias.location.replace(/\/*$/, "/") + (tail || alias.main || alias.name);
} else if (alias === false) {
return "";
}
var i = testPath.lastIndexOf("/");
if (i === -1) break;
tail = testPath.substr(i) + tail;
testPath = testPath.slice(0, i);
}
return id;
}
window.require.modules = {};
window.require.tlns = {};
window.define = function(id, deps, factory) {
if (arguments.length == 2) {
factory = deps;
if (typeof id != "string") {
deps = id;
id = window.require.id;
}
} else if (arguments.length == 1) {
factory = id;
deps = [];
id = window.require.id;
}
if (typeof factory != "function") {
window.require.modules[id] = {
exports: factory,
initialized: true
};
return;
}
if (!deps.length)
// If there is no dependencies, we inject "require", "exports" and
// "module" as dependencies, to provide CommonJS compatibility.
deps = ["require", "exports", "module"];
var req = function(childId) {
return window.require(id, childId);
};
window.require.modules[id] = {
exports: {},
factory: function() {
var module = this;
var returnExports = factory.apply(this, deps.map(function(dep) {
switch (dep) {
// Because "require", "exports" and "module" aren't actual
// dependencies, we must handle them seperately.
case "require": return req;
case "exports": return module.exports;
case "module": return module;
// But for all other dependencies, we can just go ahead and
// require them.
default: return req(dep);
}
}));
if (returnExports)
module.exports = returnExports;
return module;
}
};
};
window.define.amd = {};
require.tlns = {};
window.initBaseUrls = function initBaseUrls(topLevelNamespaces) {
for (var i in topLevelNamespaces)
require.tlns[i] = topLevelNamespaces[i];
};
window.initSender = function initSender() {
var EventEmitter = window.require("ace/lib/event_emitter").EventEmitter;
var oop = window.require("ace/lib/oop");
var Sender = function() {};
(function() {
oop.implement(this, EventEmitter);
this.callback = function(data, callbackId) {
postMessage({
type: "call",
id: callbackId,
data: data
});
};
this.emit = function(name, data) {
postMessage({
type: "event",
name: name,
data: data
});
};
}).call(Sender.prototype);
return new Sender();
};
var main = window.main = null;
var sender = window.sender = null;
window.onmessage = function(e) {
var msg = e.data;
if (msg.event && sender) {
sender._signal(msg.event, msg.data);
}
else if (msg.command) {
if (main[msg.command])
main[msg.command].apply(main, msg.args);
else if (window[msg.command])
window[msg.command].apply(window, msg.args);
else
throw new Error("Unknown command:" + msg.command);
}
else if (msg.init) {
window.initBaseUrls(msg.tlns);
require("ace/lib/es5-shim");
sender = window.sender = window.initSender();
var clazz = require(msg.module)[msg.classname];
main = window.main = new clazz(sender);
}
};
})(this);
define("ace/lib/oop",["require","exports","module"], function(require, exports, module) {
"use strict";
exports.inherits = function(ctor, superCtor) {
ctor.super_ = superCtor;
ctor.prototype = Object.create(superCtor.prototype, {
constructor: {
value: ctor,
enumerable: false,
writable: true,
configurable: true
}
});
};
exports.mixin = function(obj, mixin) {
for (var key in mixin) {
obj[key] = mixin[key];
}
return obj;
};
exports.implement = function(proto, mixin) {
exports.mixin(proto, mixin);
};
});
define("ace/range",["require","exports","module"], function(require, exports, module) {
"use strict";
var comparePoints = function(p1, p2) {
return p1.row - p2.row || p1.column - p2.column;
};
var Range = function(startRow, startColumn, endRow, endColumn) {
this.start = {
row: startRow,
column: startColumn
};
this.end = {
row: endRow,
column: endColumn
};
};
(function() {
this.isEqual = function(range) {
return this.start.row === range.start.row &&
this.end.row === range.end.row &&
this.start.column === range.start.column &&
this.end.column === range.end.column;
};
this.toString = function() {
return ("Range: [" + this.start.row + "/" + this.start.column +
"] -> [" + this.end.row + "/" + this.end.column + "]");
};
this.contains = function(row, column) {
return this.compare(row, column)
没有合适的资源?快使用搜索试试~ 我知道了~
传智健康-项目源码+项目总结
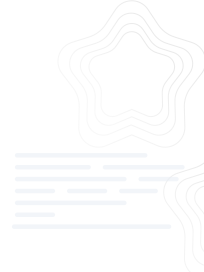
共3511个文件
js:1916个
jar:385个
png:296个

需积分: 44 40 下载量 193 浏览量
2022-03-27
14:38:35
上传
评论 6
收藏 626.86MB ZIP 举报
温馨提示
传智健康管理系统是一款应用于健康管理机构的业务系统,实现健康管理机构工作内容可视化、会员管理专业化、健康评估数字化、健康干预流程化、知识库集成化,从而提高健康管理师的工作效率,加强与会员间的互动,增强管理者对健康管理机构运营情况的了解 主要后端技术栈: Spring,SpringMVC,Mybatis (SSM框架) zookeeper,dubbo,SpringSecrity (分布式与权限) Git,Apache POI,Echarts (版本控制与报表) 腾讯云短信服务,七牛云存储服务,微信开发平台 (第三方服务) ———————————————— 版权声明:本文为CSDN博主「Alkaid..」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。 原文链接:https://blog.csdn.net/weixin_45907602/article/details/123533046
资源详情
资源评论
资源推荐
收起资源包目录

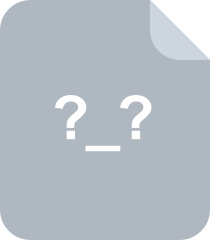
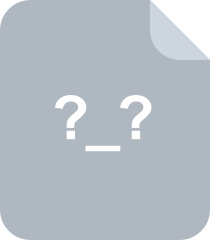
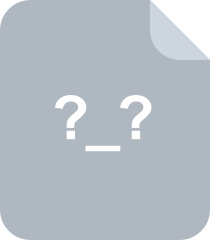
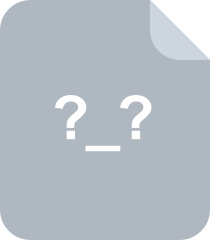
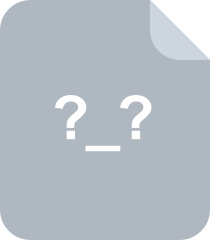
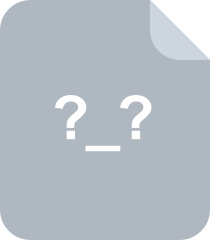
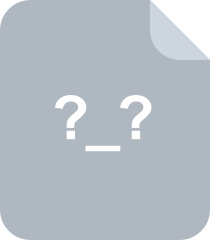
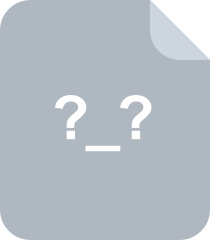
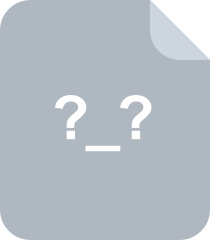
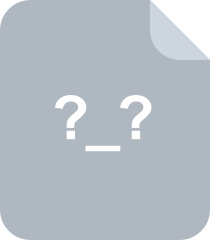
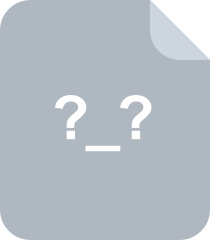
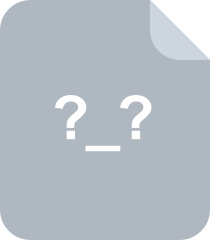
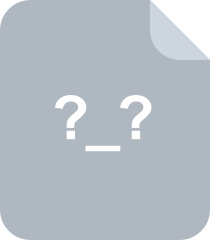
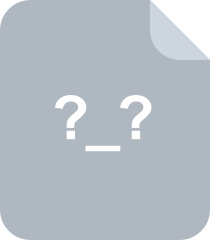
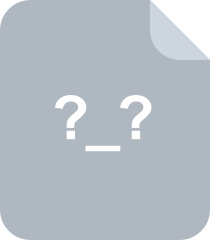
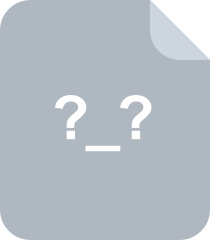
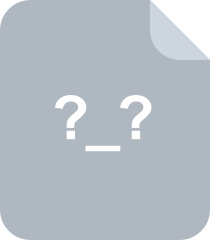
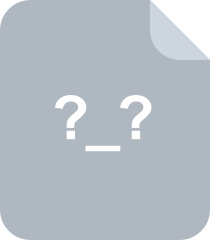
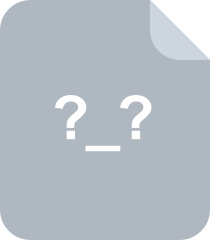
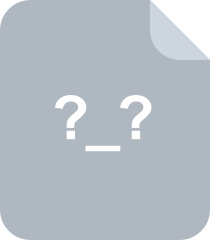
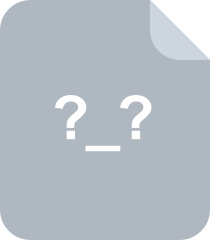
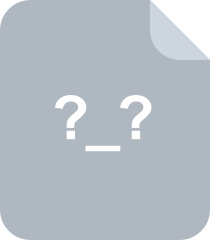
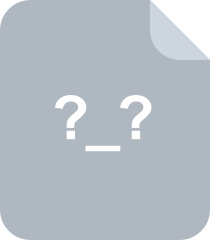
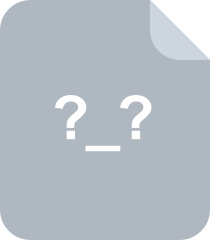
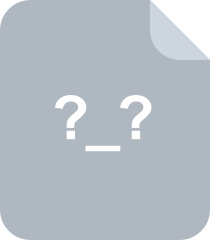
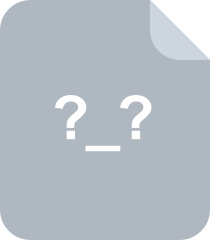
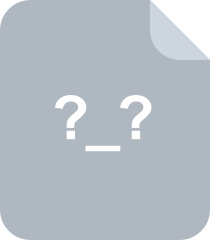
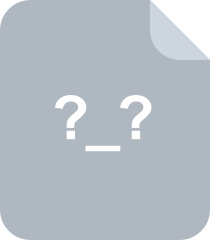
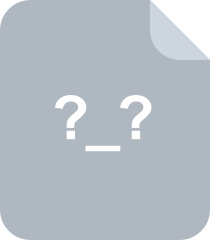
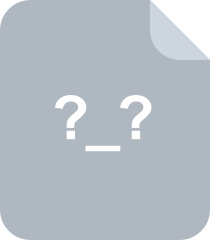
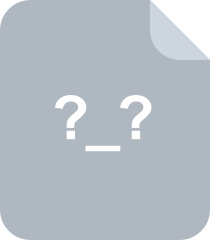
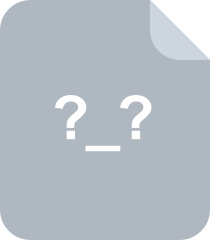
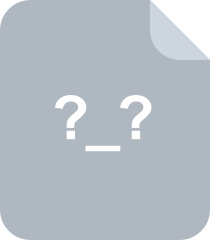
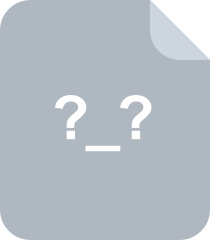
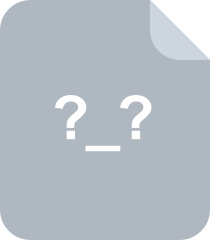
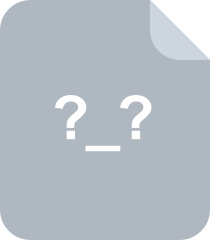
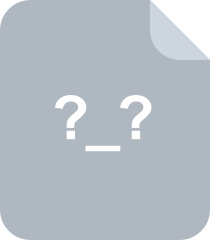
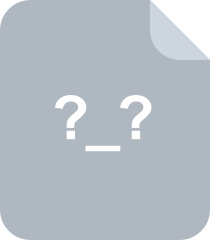
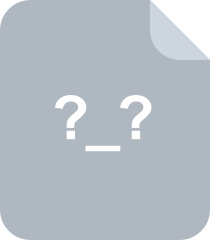
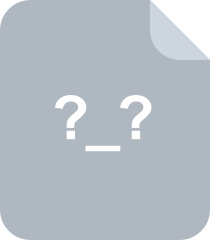
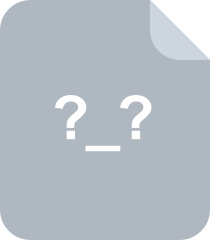
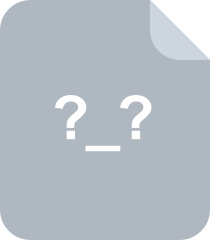
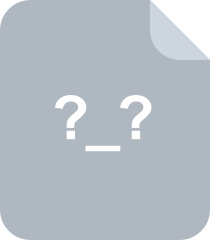
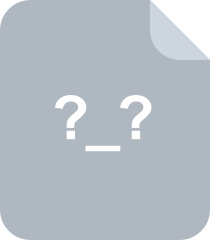
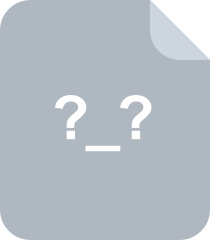
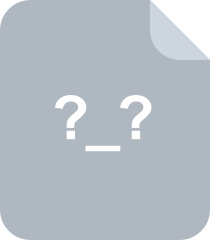
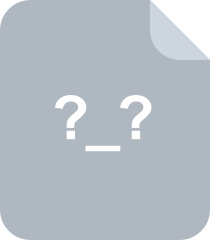
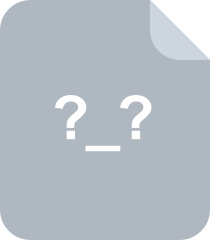
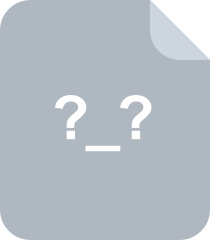
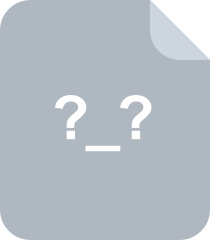
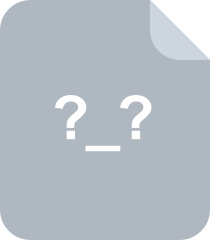
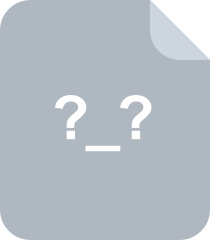
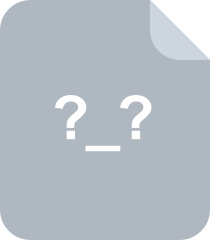
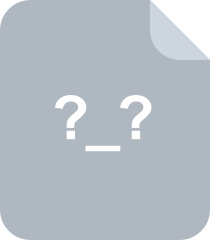
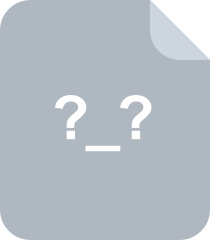
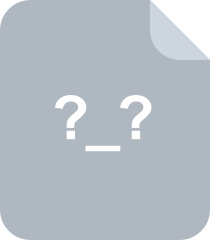
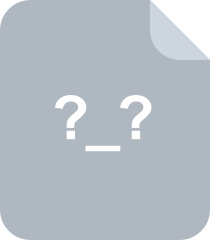
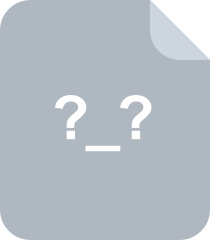
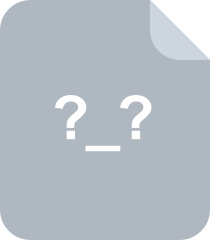
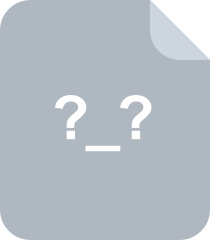
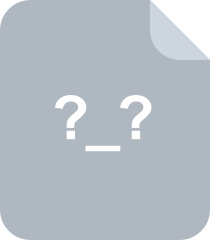
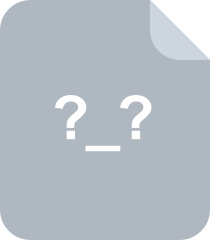
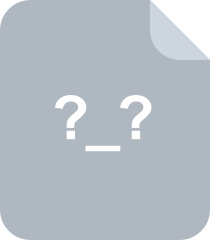
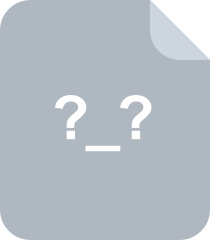
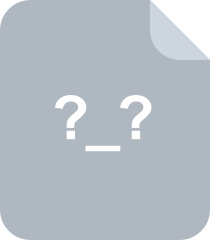
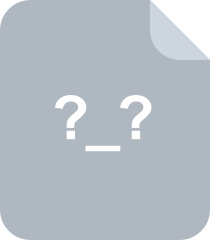
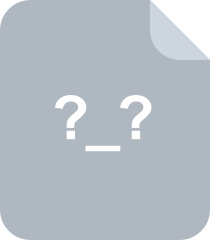
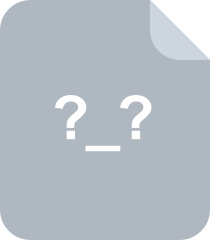
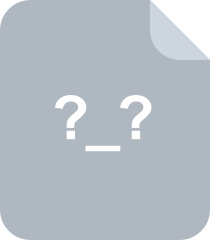
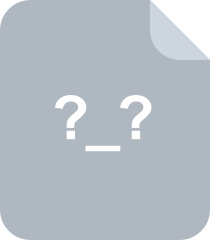
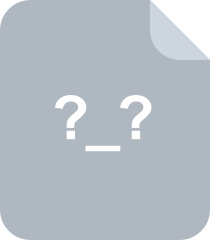
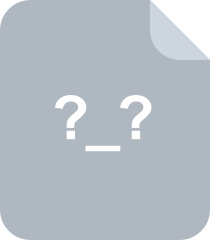
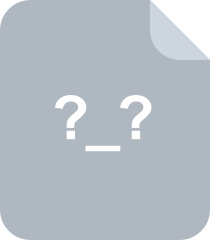
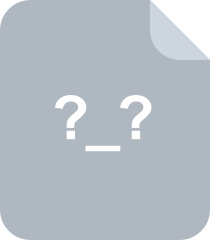
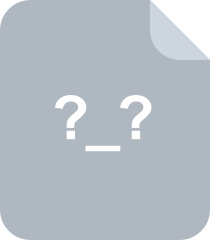
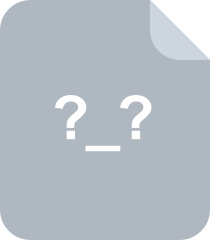
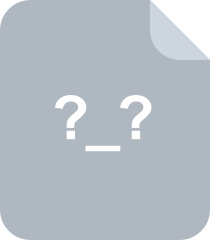
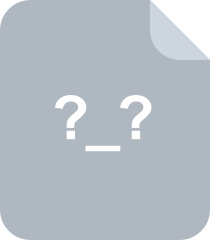
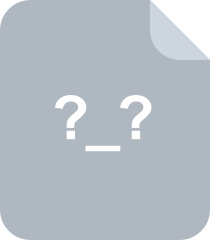
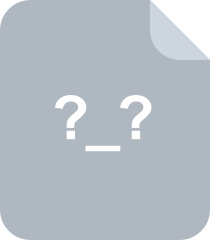
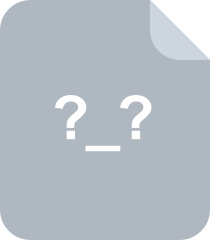
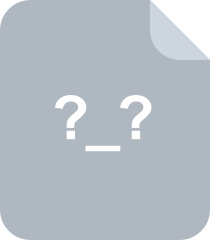
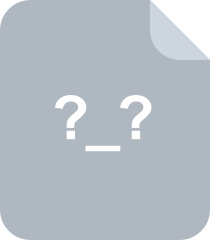
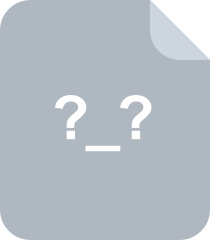
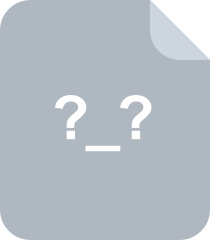
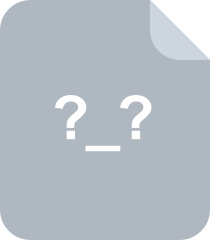
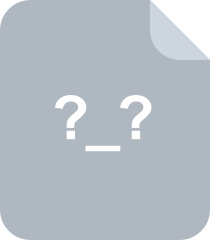
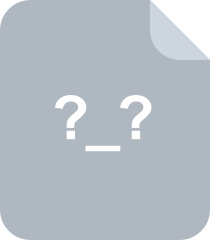
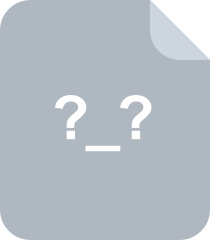
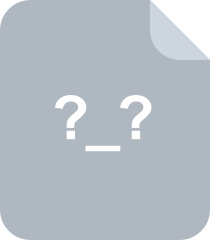
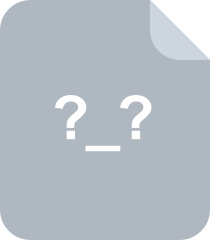
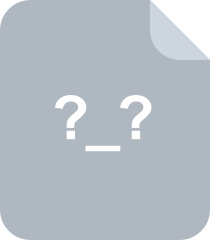
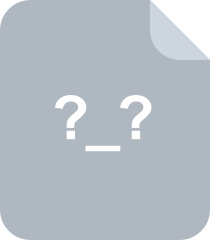
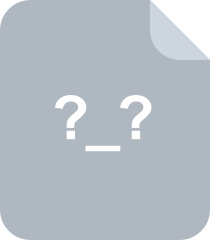
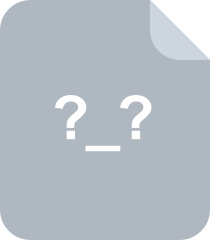
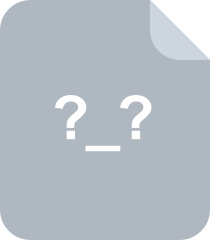
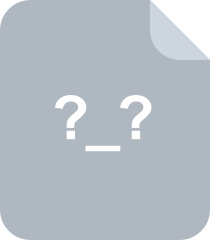
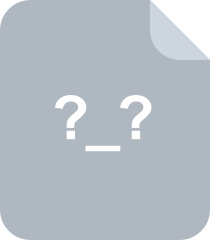
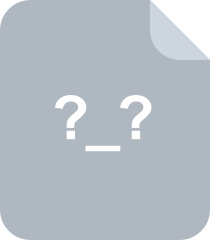
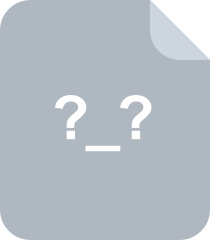
共 3511 条
- 1
- 2
- 3
- 4
- 5
- 6
- 36
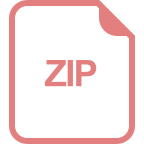
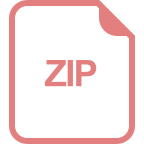
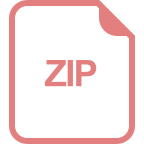
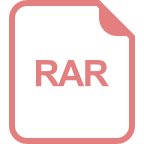
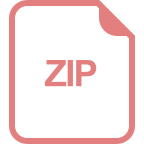
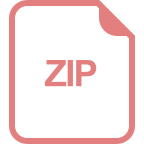
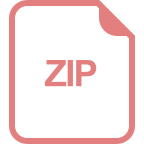
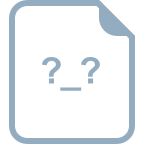
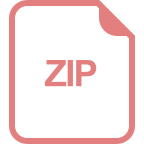
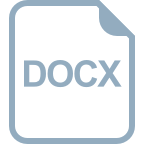
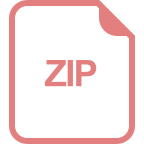
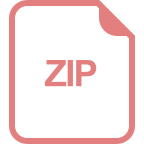
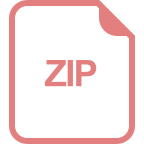
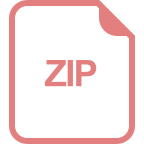

Hash..
- 粉丝: 8
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

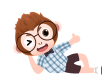
最新资源
- 散装物料卸船机step全套技术开发资料100%好用.zip
- MSS市场专项考试题库
- (174756810)跨年烟花代码python
- (175424836)JSP企业电子投票系统(源代码+论文+开题报告+外文翻译+文献综述).rar
- (175470002)JSP企业电子投票系统(源代码+论文+开题报告+外文翻译+文献综述)
- (175759628)贪吃蛇.zip
- (175833246)JSP企业电子投票系统(源代码+论文+开题报告+外文翻译+文献综述).rar.tar.gz
- 自行车、汽车、猫、狗、人类、入侵者检测39-YOLO(v5至v11)、COCO数据集合集.rar
- (175860660)基于51单片机直流电压电流表设计LCD1602液晶实训仿真
- (175931624)基于jsp的投票管理系统源码数据库论文.doc
- 在ARM9核心板KNM1001上实现uIP FTP及TFTP客户端
- (176056440)zotero 插件分享 茉莉花压缩包
- Overview of the Scalable Video Coding Extension of the H.264/AVC Standard
- 汽车之家计量学分析.zip
- (176074624)EPLAN P8部件库:包含低压电气控制系统设计常用品Pai型号 导入单个文件很小几十M,简单易用
- (176333852)《数据库原理及应用教程(微课版)》关系数据库思维导图源文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


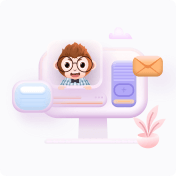
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0