package com.utils;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.HashOperations;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.stereotype.Component;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* @description:
* @author: huangan
* @date: 2024/10/29 13:22
*/
@Slf4j
@Component
public class RedisUtils {
@Autowired
private StringRedisTemplate redisTemplate;
/**
* 写入redis缓存(不设置expire存活时间)
* @param key
* @param value
* @return
*/
public boolean set(final String key, String value) {
boolean result = false;
try {
ValueOperations<String, String> operations = redisTemplate.opsForValue();
operations.set(key, value);
result = true;
} catch (Exception e) {
log.error("写入redis缓存失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* 写入redis缓存(设置expire存活时间)
* @param key
* @param value
* @param expire
* @return
*/
public boolean set(final String key, String value, Long expire) {
boolean result = false;
try {
ValueOperations<String, String> operations = redisTemplate.opsForValue();
operations.set(key, value);
redisTemplate.expire(key, expire, TimeUnit.SECONDS);
result = true;
} catch (Exception e) {
log.error("写入redis缓存(设置expire存活时间)失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* map格式写入redis缓存(不设置expire存活时间)
* @param key
* @param modelMap
* @return
*/
public boolean setMap(String key, Map<String, Object> modelMap) {
boolean result = false;
try {
HashOperations<String, Object, Object> hps = redisTemplate.opsForHash();
hps.putAll(key, modelMap);
result = true;
} catch (Exception e) {
log.error("写入redis缓存失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* map格式写入redis缓存(设置expire存活时间)
* @param key
* @param modelMap
* @param expire
* @return
*/
public boolean setMap(final String key, Map<String, Object> modelMap, Long expire) {
boolean result = false;
try {
HashOperations<String, Object, Object> hps = redisTemplate.opsForHash();
hps.putAll(key, modelMap);
redisTemplate.expire(key, expire, TimeUnit.SECONDS);
result = true;
} catch (Exception e) {
log.error("写入redis缓存(设置expire存活时间)失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* 读取redis缓存
*
* @param key
* @return
*/
public Object get(final String key) {
Object result = null;
try {
ValueOperations<String, String> operations = redisTemplate.opsForValue();
result = operations.get(key);
} catch (Exception e) {
log.error("读取redis缓存失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* 读取map类型redis缓存
* @param mapName
* @return
*/
public Map<String, Object> getMapValue(String mapName) {
Map<String, Object> result = null;
try {
HashOperations<String, String, Object> hps = redisTemplate.opsForHash();
result = hps.entries(mapName);
} catch (Exception e) {
log.error("读取redis缓存失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* 判断redis缓存中是否有对应的key
* @param key
* @return
*/
public boolean exists(final String key) {
boolean result = false;
try {
result = redisTemplate.hasKey(key);
} catch (Exception e) {
log.error("判断redis缓存中是否有对应的key失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* redis根据key删除对应的value
* @param key
* @return
*/
public boolean remove(final String key) {
boolean result = false;
try {
if (exists(key)) {
redisTemplate.delete(key);
}
result = true;
} catch (Exception e) {
log.error("redis根据key删除对应的value失败!错误信息为:" + e.getMessage());
}
return result;
}
/**
* redis根据keys批量删除对应的value
* @param keys
* @return
*/
public void remove(final String... keys) {
for (String key : keys) {
remove(key);
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
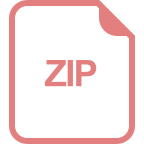
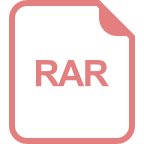
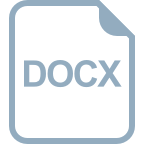
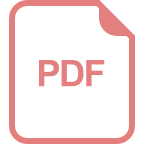
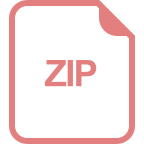
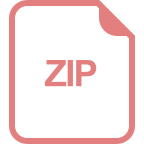
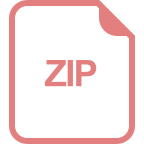
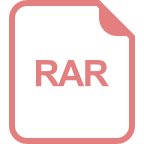
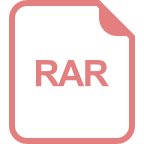
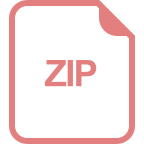
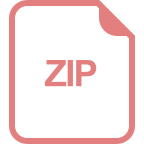
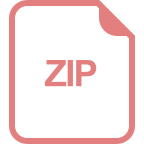
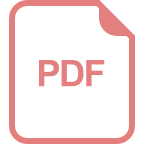
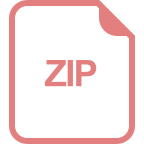
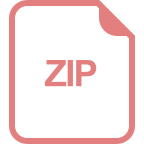
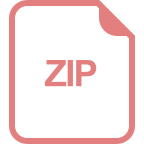
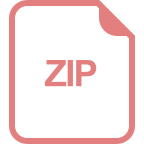
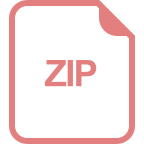
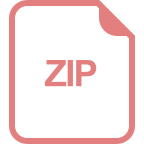
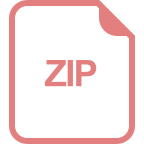
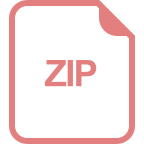
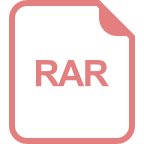
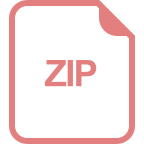
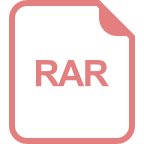
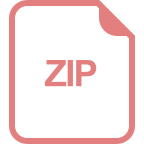
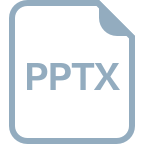
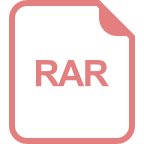
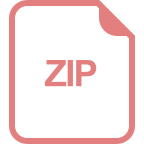
收起资源包目录


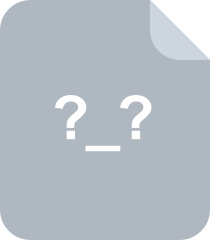





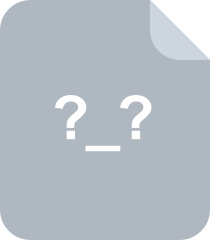



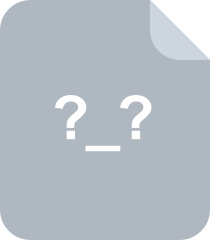

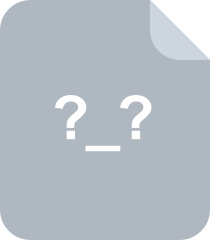

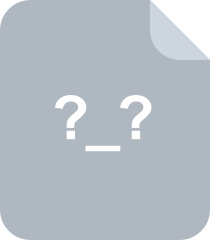
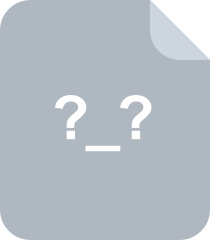
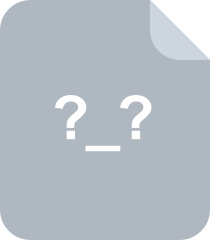
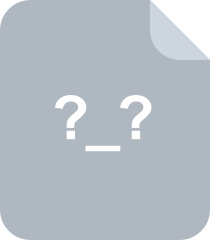

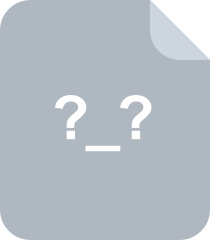

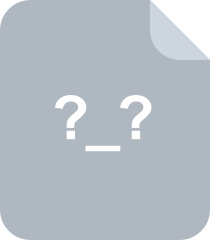
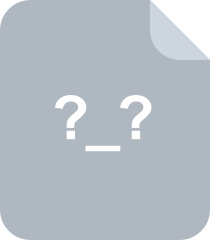


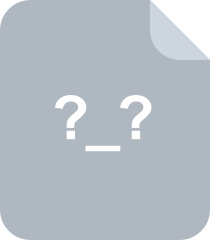

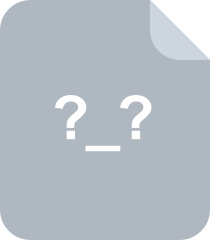
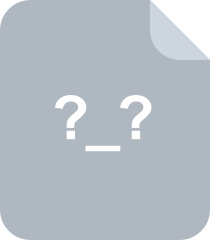

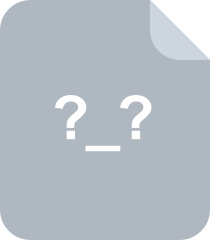
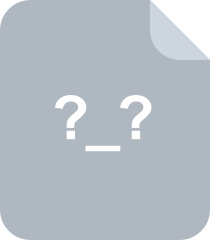
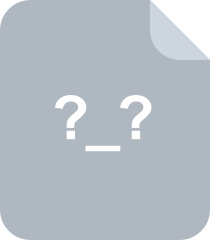
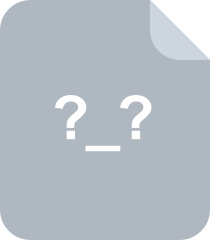

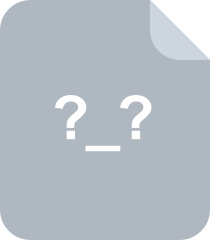
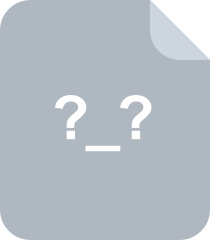
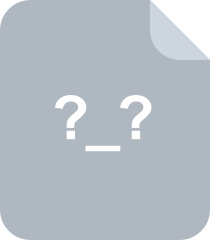
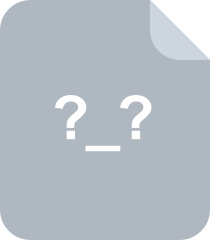
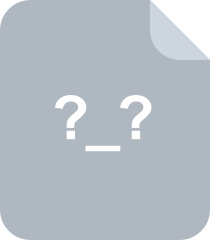


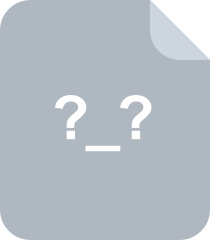


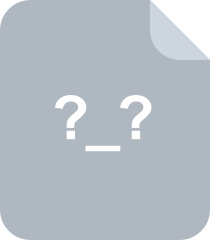

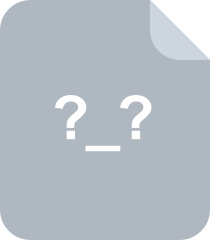

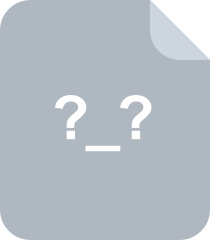
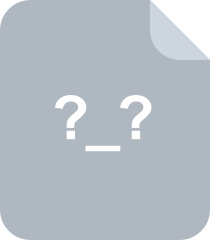
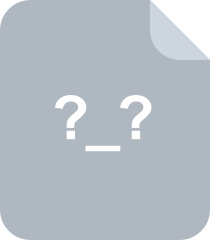

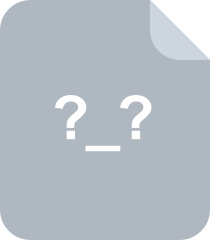

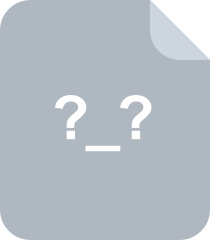
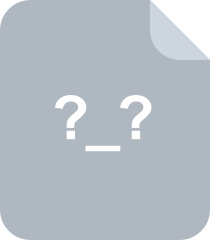


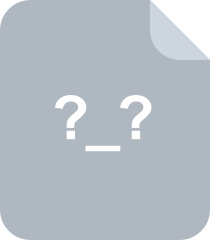
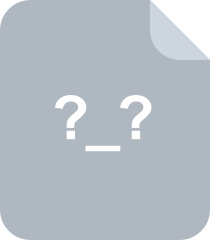

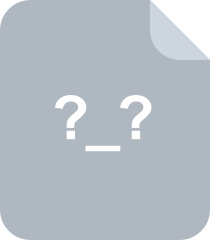
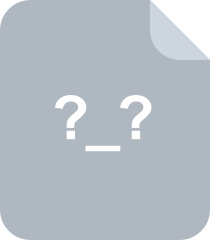

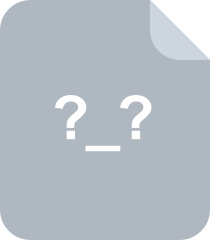
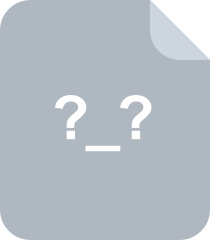
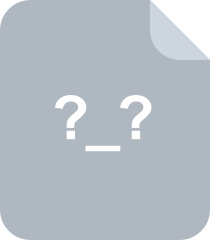
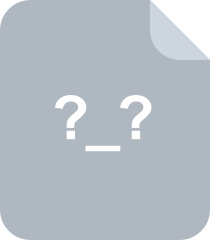


共 40 条
- 1
资源评论
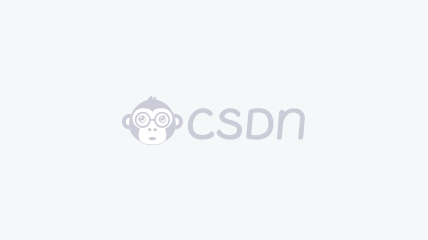

weixin_45863786
- 粉丝: 67
- 资源: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

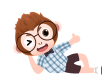
最新资源
- GTK 的 Go 绑定.zip
- GraphQL 的 Go,Golang 实现.zip
- Go(golang)游戏服务器框架.zip
- ASP.NET C#+JS多文件上传源码
- Go(golang)中的 JavaScript 解释器.zip
- goth 包提供了一种简单、干净且惯用的方式来为 Go Web 应用程序编写身份验证包 .zip
- PHP 中 Cookie 和 Session 的使用简易教程(学习笔记)
- SoftEther VPN Client + VPN Gate Client 插件
- GoRequest-简化的HTTP客户端(受nodejs SuperAgent启发).zip
- 主要物体检测15-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


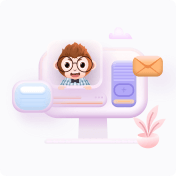
安全验证
文档复制为VIP权益,开通VIP直接复制
