package com.yyl.mapper.impl;
import com.yyl.bean.Student;
import com.yyl.mapper.StudentMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
/*
持久层实现类
*/
public class StudentMapperImpl implements StudentMapper {
/*
查询全部
*/
@Override
public List<Student> selectAll() {
List<Student> list = null;
SqlSession sqlSession = null;
InputStream is = null;
try{
//1.加载核心配置文件
is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2.获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3.通过工厂对象获取SqlSession对象
sqlSession = sqlSessionFactory.openSession(true);
//4.执行映射配置文件中的sql语句,并接收结果
list = sqlSession.selectList("StudentMapper.selectAll");
} catch (Exception e) {
e.printStackTrace();
} finally {
//5.释放资源
if(sqlSession != null) {
sqlSession.close();
}
if(is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//6.返回结果
return list;
}
/*
根据id查询
*/
@Override
public Student selectById(Integer id) {
Student stu = null;
SqlSession sqlSession = null;
InputStream is = null;
try{
//1.加载核心配置文件
is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2.获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3.通过工厂对象获取SqlSession对象
sqlSession = sqlSessionFactory.openSession(true);
//4.执行映射配置文件中的sql语句,并接收结果
stu = sqlSession.selectOne("StudentMapper.selectById",id);
} catch (Exception e) {
e.printStackTrace();
} finally {
//5.释放资源
if(sqlSession != null) {
sqlSession.close();
}
if(is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//6.返回结果
return stu;
}
/*
新增功能
*/
@Override
public Integer insert(Student stu) {
Integer result = null;
SqlSession sqlSession = null;
InputStream is = null;
try{
//1.加载核心配置文件
is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2.获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3.通过工厂对象获取SqlSession对象
sqlSession = sqlSessionFactory.openSession(true);
//4.执行映射配置文件中的sql语句,并接收结果
result = sqlSession.insert("StudentMapper.insert",stu);
} catch (Exception e) {
e.printStackTrace();
} finally {
//5.释放资源
if(sqlSession != null) {
sqlSession.close();
}
if(is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//6.返回结果
return result;
}
/*
修改功能
*/
@Override
public Integer update(Student stu) {
Integer result = null;
SqlSession sqlSession = null;
InputStream is = null;
try{
//1.加载核心配置文件
is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2.获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3.通过工厂对象获取SqlSession对象
sqlSession = sqlSessionFactory.openSession(true);
//4.执行映射配置文件中的sql语句,并接收结果
result = sqlSession.update("StudentMapper.update",stu);
} catch (Exception e) {
e.printStackTrace();
} finally {
//5.释放资源
if(sqlSession != null) {
sqlSession.close();
}
if(is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//6.返回结果
return result;
}
/*
删除功能
*/
@Override
public Integer delete(Integer id) {
Integer result = null;
SqlSession sqlSession = null;
InputStream is = null;
try{
//1.加载核心配置文件
is = Resources.getResourceAsStream("MyBatisConfig.xml");
//2.获取SqlSession工厂对象
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(is);
//3.通过工厂对象获取SqlSession对象
sqlSession = sqlSessionFactory.openSession(true);
//4.执行映射配置文件中的sql语句,并接收结果
result = sqlSession.delete("StudentMapper.delete",id);
} catch (Exception e) {
e.printStackTrace();
} finally {
//5.释放资源
if(sqlSession != null) {
sqlSession.close();
}
if(is != null) {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//6.返回结果
return result;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Mybatis 框架理解与快速入门详解代码与数据库
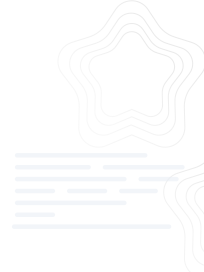
共33个文件
xml:8个
class:7个
java:7个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 72 浏览量
2022-06-21
10:49:45
上传
评论
收藏 2.83MB ZIP 举报
温馨提示
软件框架(software framework),通常指的是为了实现某个业界标准或完成特定基本任务的软件组件规范,也指为了实现某个软件组件规范时,提供规范所要求之基础功能的软件产品。框架的功能类似于基础设施,与具体的软件应用无关,但是提供并实现最为基础的软件架构和体系。总的来说:框架是一款半成品软件,我们可以基于这个半成品软件继续开发,来完成我们个性化的需求!如图下图所示:我们可以拿不同的框架来搭建我们自己的成品。 1. 为什么需要框架技术:软件系统随着业务的发展,变得越来越复杂,不同领域的业务所涉及到的知;软件框架(software framework),通常指的是为了实现某个业界标准或完成特定基本任务的软件组件规范,也指为了实现某个软件组件规范时,提供规范所要求之基础功能的软件产品。框架的功能类似于基础设施,与具体的软件应用无关,但是提供并实现最为基础的软件架构和体系。总的来说:框架是一款半成品软件,我们可以基于这个半成品软件继续开发,来完成我们个性化的需求!如图下图所示:我们可以拿不同的框架来搭建我们自己的成品。 1. 为什么需要框架技术:软件系统随着业务的发展,变得越来越复杂,
资源推荐
资源详情
资源评论
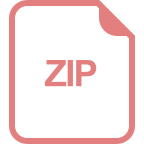
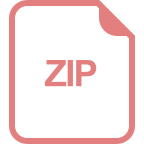
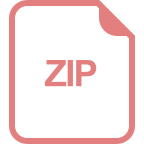
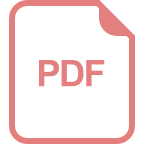
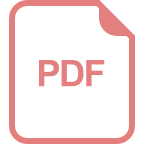
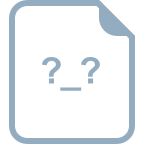
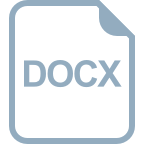
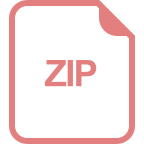
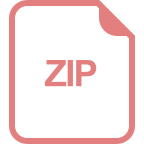
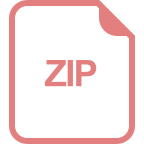
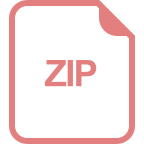
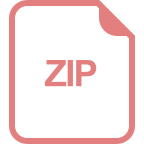
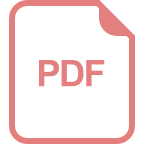
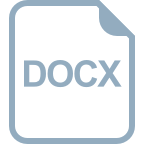
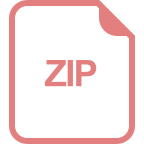
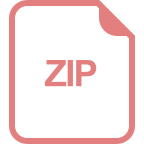
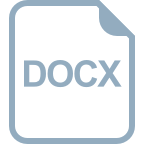
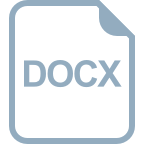
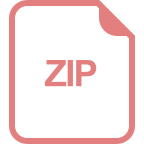
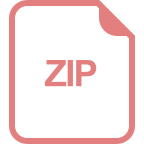
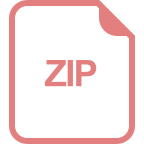
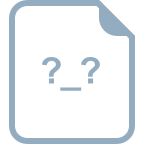
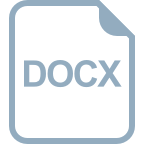
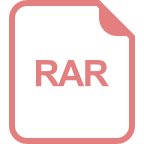
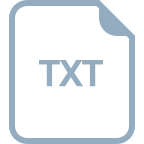
收起资源包目录







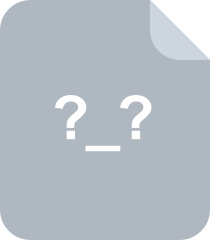
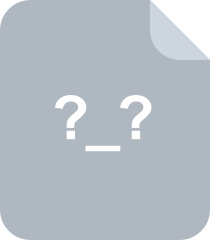
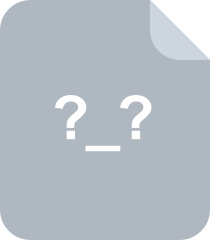
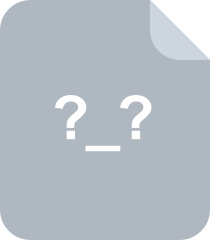



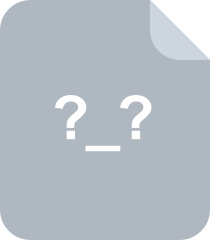

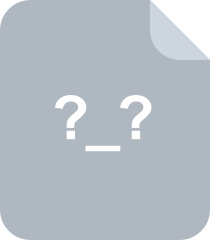


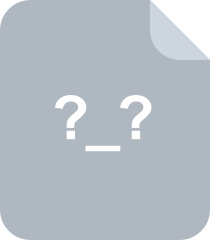
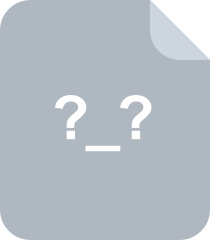

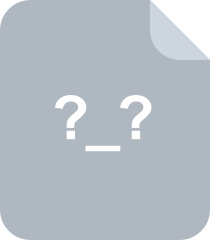

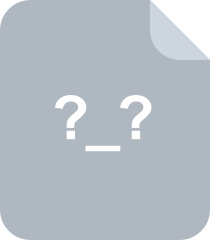

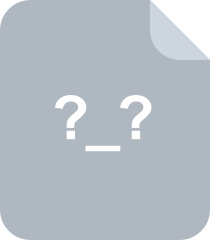
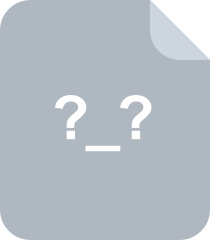
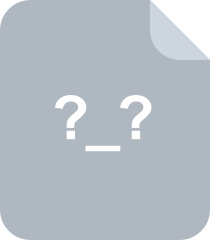

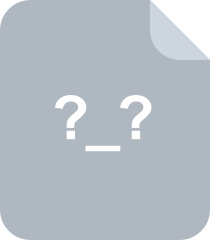
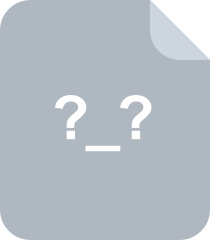
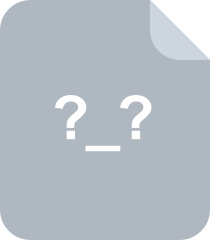



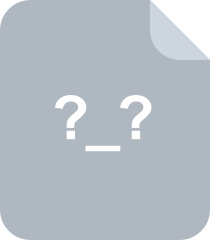

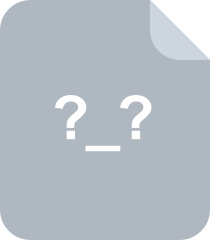

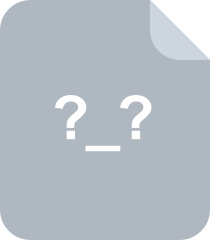

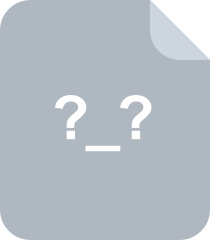

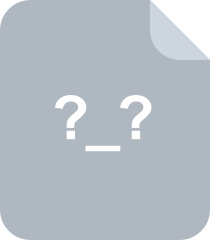


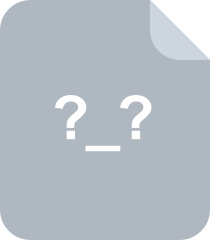
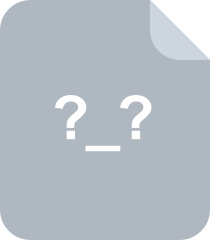
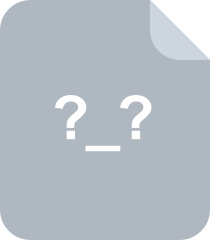


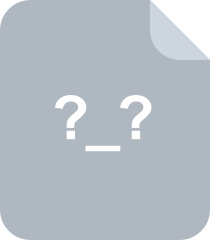
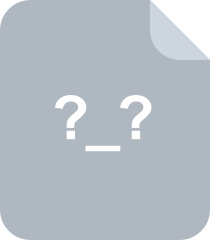
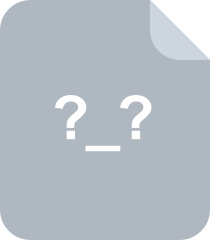
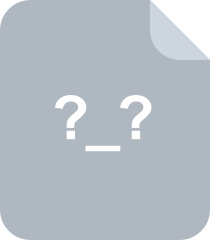
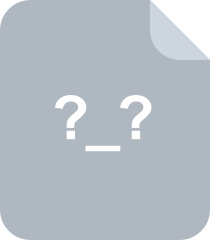

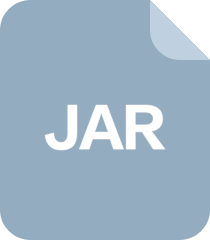
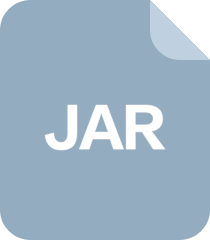
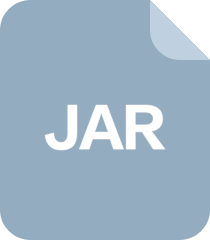

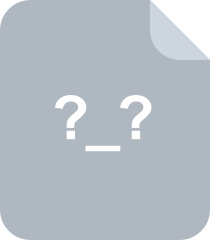
共 33 条
- 1
资源评论
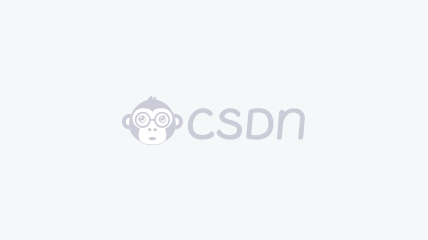

我心向阳iu
- 粉丝: 11w+
- 资源: 155
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

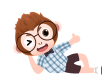
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


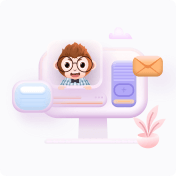
安全验证
文档复制为VIP权益,开通VIP直接复制
