package com.kdx.springai.controller;
import org.springframework.ai.chat.client.ChatClient;
import org.springframework.ai.chat.model.ChatModel;
import org.springframework.ai.chat.model.ChatResponse;
import org.springframework.ai.chat.prompt.Prompt;
import org.springframework.ai.image.ImagePrompt;
import org.springframework.ai.image.ImageResponse;
import org.springframework.ai.openai.*;
import org.springframework.ai.openai.api.OpenAiApi;
import org.springframework.ai.openai.api.OpenAiAudioApi;
import org.springframework.ai.openai.audio.speech.SpeechPrompt;
import org.springframework.ai.openai.audio.speech.SpeechResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Flux;
import java.io.FileOutputStream;
import java.io.IOException;
@RestController
@RequestMapping("/ai")
public class HelloController {
@Autowired
private ChatClient chatClient;
@Autowired(required = false)
private ChatModel chatModel;
@Autowired(required = false)
private OpenAiImageModel openaiImageModel;
@Autowired(required = false)
private OpenAiAudioSpeechModel openAiAudioSpeechModel;
@RequestMapping("/hello")
public String hello() {
return "hello";
}
//交流
@RequestMapping("/chat")
public String generation(@RequestParam(value = "message", defaultValue = "讲个笑话") String message) {
//prompt:提示词
return this.chatClient.prompt()
//用户信息
.user(message)
//请求大模型
.call()
//返回文本
.content();
}
//流式响应
@RequestMapping(value = "/stream", produces = "text/html;charset=UTF-8")
public Flux<String> stream(@RequestParam(value = "message", defaultValue = "讲个笑话") String message) {
Flux<String> output = chatClient.prompt()
.user(message)
//流式调用
.stream()
.content();
return output;
}
//chatModel api
@RequestMapping(value = "/ChatResponse", produces = "text/html;charset=UTF-8")
public String ChatResponse(@RequestParam(value = "message") String message) {
ChatResponse response = chatModel.call(new Prompt(
message,
OpenAiChatOptions.builder()
//选择gpt版本
.withModel("gpt-4-32k")
.withTemperature(0.4f)
.build()
));
return response.getResult().getOutput().getContent();
}
//文生图
@RequestMapping(value = "/openaiImageModel", produces = "text/html;charset=UTF-8")
public String openaiImageModel(@RequestParam(value = "message") String message) {
ImageResponse response = openaiImageModel.call(
new ImagePrompt(message,
OpenAiImageOptions.builder()
//图片质量
.withQuality("hd")
//生成几张
.withN(1)
//尺寸
.withHeight(1024)
.withWidth(1024).build())
);
return response.getResult().getOutput().getUrl();
}
//文生语音
@RequestMapping(value = "/writeByte", produces = "text/html;charset=UTF-8")
public String writeByte(@RequestParam(value = "message") String message) {
OpenAiAudioSpeechOptions speechOptions = OpenAiAudioSpeechOptions.builder()
.withModel(OpenAiAudioApi.TtsModel.TTS_1.value)
.withVoice(OpenAiAudioApi.SpeechRequest.Voice.ALLOY)
.withResponseFormat(OpenAiAudioApi.SpeechRequest.AudioResponseFormat.MP3)
.withSpeed(1.0f)
.build();
SpeechPrompt speechPrompt = new SpeechPrompt(message, speechOptions);
SpeechResponse response = openAiAudioSpeechModel.call(speechPrompt);
byte[] body = response.getResult().getOutput();
try {
writeByte(body,"D:\\Project");
} catch (Exception e) {
System.out.println(e);
}
return "ok";
}
public static void writeByte(byte[] audioBytes, String outputFilePath) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream(outputFilePath + "111.mp3");
fileOutputStream.write(audioBytes);
fileOutputStream.close();
}
//functionCall
@RequestMapping(value = "/functionCall", produces = "text/html;charset=UTF-8")
public String functionCall(@RequestParam(value = "message") String message) {
OpenAiChatOptions aiChatOptions = OpenAiChatOptions.builder()
//设置实现了function接口的bean名称
.withFunction("LocationNameFunction")
.withModel(OpenAiApi.ChatModel.GPT_3_5_TURBO)
.build();
ChatResponse response = chatModel.call(new Prompt(message, aiChatOptions));
return response.getResult().getOutput().getContent();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
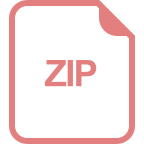
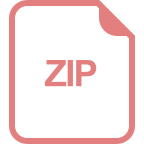
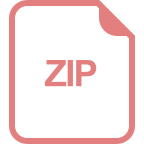
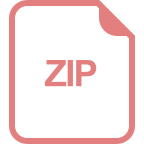
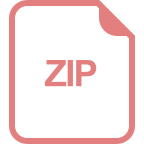
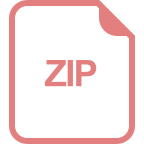
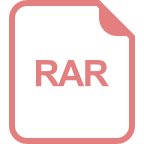
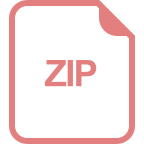
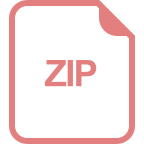
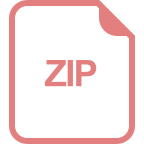
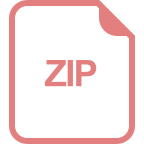
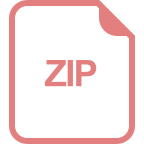
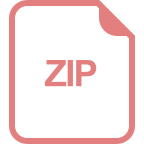
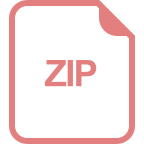
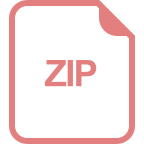
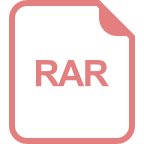
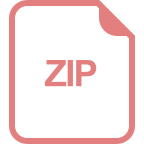
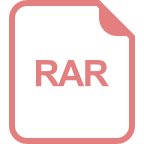
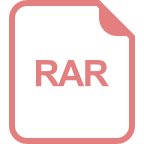
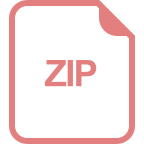
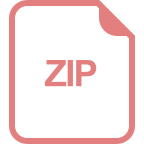
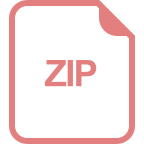
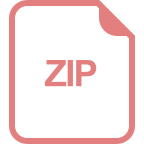
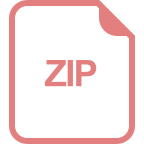
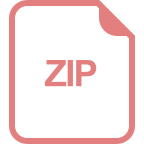
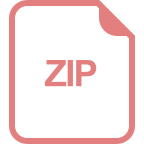
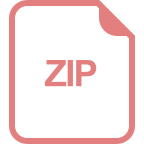
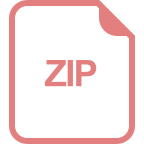
收起资源包目录


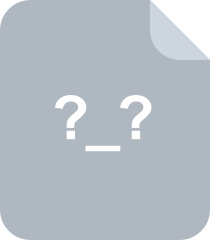






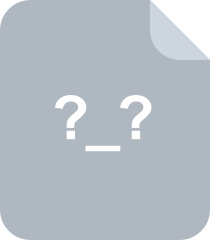




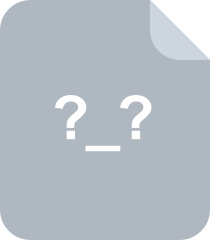





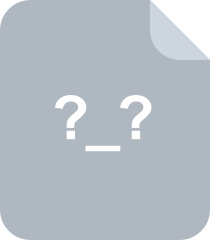
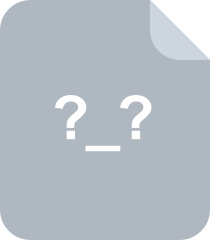

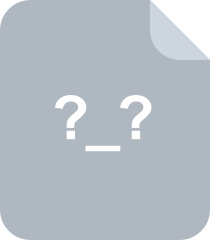

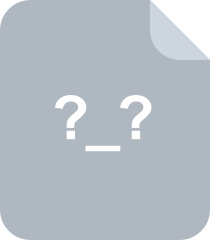


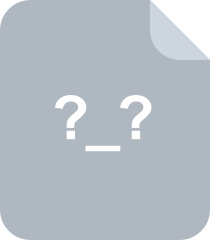

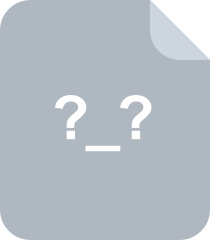
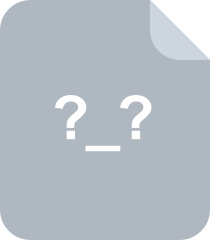
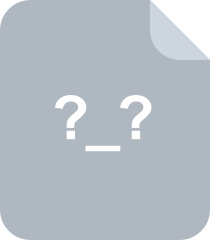
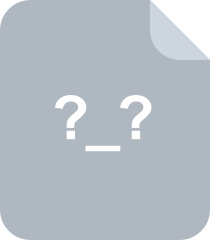
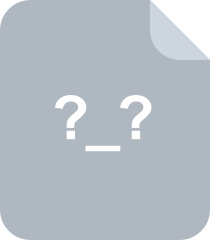
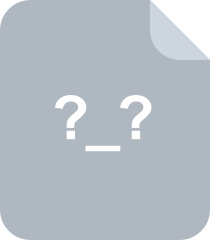
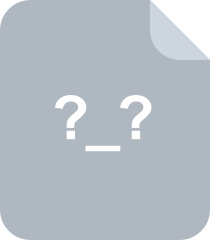
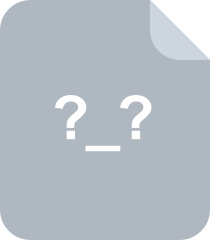
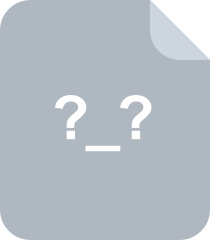


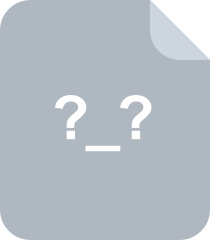




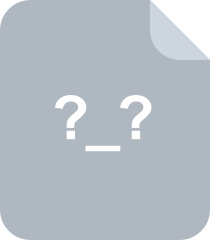
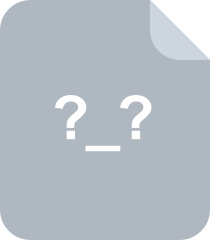

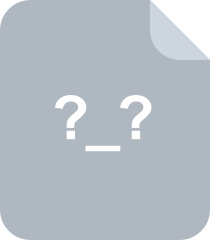
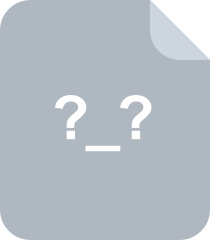
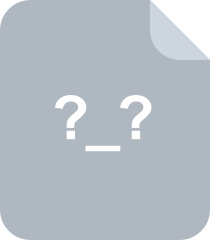

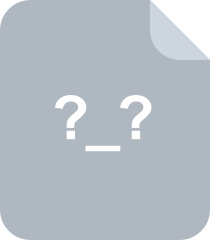




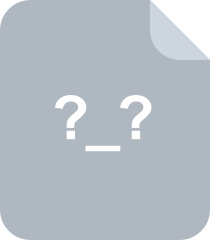




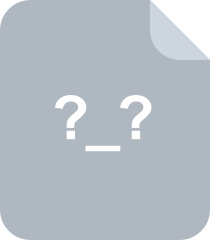
共 26 条
- 1
资源评论
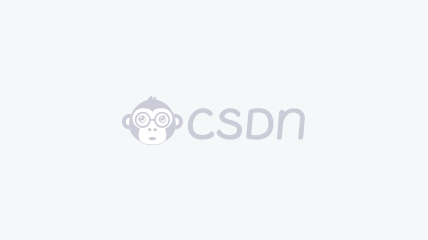

西敏寺的乐章
- 粉丝: 4062
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

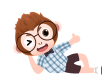
最新资源
- 使用 GSD (DirectX Hook Library) 绘制十字线.zip
- 使用 Graphic, DirectX, OpenGL 进行全屏拍摄.zip
- jd-gui-windows-1.6.6 java反编译工具
- 经典分子模拟教程 《The art of molucular dynamics simulation》作者: D.C. Rapaport 出版社:Cambridge Universi
- InputTip - 根据输入法中英文状态切换鼠标样式的小工具
- 使用 Dx3D9 Sprite 对象的 DirectX 2D 引擎.zip
- C code for "The art of molecular dynamics simulation"
- 国外版剪映 特效无限用,无需登录
- 使用 DX12 编写的基于物理的渲染器,具有基于图像的照明、经典的延迟和平铺照明方法.zip
- windows命令行curl命令工具
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


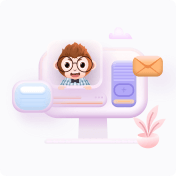
安全验证
文档复制为VIP权益,开通VIP直接复制
