第一个是蓝牙设配器,对蓝牙的操作都需要用到它,很重要,BluetoothGatt作为中央来使用和处理数据,使用时有一个回调方法BluetoothGattCallback返回中央的状态和周边提供的数据,BluetoothCattService作为周边来提供数据;BluetoothGattServerCallback返回周边的状态。BluetoothDevice是蓝牙设备,BluetoothCattCharacteristic是蓝牙设备的特征。 ### 手机蓝牙连接通信的实现技术 #### 一、引言 随着移动通信技术的发展,蓝牙技术作为一种短距离无线通信技术,在智能手机等移动设备上得到了广泛应用。本文将详细介绍Android平台下蓝牙连接通信的实现机制,包括核心组件、工作流程以及关键API的使用。 #### 二、蓝牙连接通信的核心组件 在Android蓝牙通信开发中,有几个核心组件至关重要: 1. **`BluetoothAdapter`**: - **作用**:蓝牙适配器是进行蓝牙操作的基础,用于管理本地蓝牙设备。 - **重要性**:所有的蓝牙操作都需要通过`BluetoothAdapter`实例来进行。 2. **`BluetoothGatt`**: - **作用**:通用属性配置文件(Generic Attribute Profile)的客户端,用于连接到远程设备并与其交互。 - **使用方式**:通过`connectGatt()`方法建立与远程设备的连接,并通过回调`BluetoothGattCallback`来接收状态变化。 3. **`BluetoothGattCallback`**: - **作用**:定义了一系列回调方法,用于接收来自`BluetoothGatt`的状态更新和数据传输结果。 - **关键方法**: - `onConnectionStateChange(BluetoothGatt gatt, int status, int newState)`:连接状态改变时调用。 - `onServicesDiscovered(BluetoothGatt gatt, int status)`:服务发现完成时调用。 - `onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status)`:读取特征值完成后调用。 - `onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic)`:当特征值发生变化时调用。 4. **`BluetoothGattService`**: - **作用**:表示一个服务,通常包含一系列相关的特征。 - **关联**:通过`BluetoothGatt.getService(UUID serviceUuid)`方法获取特定的服务。 5. **`BluetoothGattServerCallback`**: - **作用**:定义了一系列回调方法,用于接收来自`BluetoothGattServer`的状态更新和数据传输结果。 6. **`BluetoothDevice`**: - **作用**:表示一个远程蓝牙设备。 - **属性**:每个设备都有一个唯一的MAC地址。 7. **`BluetoothGattCharacteristic`**: - **作用**:表示服务中的一个特征,可以包含可读或可写的数据。 - **属性**:每个特征都有一个唯一的UUID标识。 #### 三、蓝牙连接通信的工作流程 1. **权限声明**: - 在AndroidManifest.xml中添加必要的权限,例如`<uses-permission android:name="android.permission.BLUETOOTH"/>`。 2. **打开蓝牙**: - 使用`BluetoothAdapter.getDefaultAdapter().enable()`方法打开蓝牙。 - 可以通过系统设置对话框让用户手动开启蓝牙。 3. **扫描蓝牙设备**: - 通过`BluetoothAdapter.getDefaultAdapter().startDiscovery()`启动设备扫描。 - 使用`BroadcastReceiver`监听扫描结果广播。 4. **连接设备**: - 获取目标设备的MAC地址。 - 关闭蓝牙扫描`BluetoothAdapter.getDefaultAdapter().cancelDiscovery()`。 - 使用`BluetoothDevice.connectGatt()`建立连接。 5. **服务发现和服务交互**: - 通过`BluetoothGatt.discoverServices()`发现远程设备上的服务。 - 对特定的服务和特征进行读写操作。 6. **数据通信**: - 通过`BluetoothGatt.writeCharacteristic()`写入数据。 - 通过`BluetoothGatt.readCharacteristic()`读取数据。 #### 四、示例代码分析 在实际开发中,开发者需要根据具体的业务需求选择合适的方法来实现蓝牙通信。例如: ```java // 打开蓝牙 BluetoothAdapter.getDefaultAdapter().enable(); // 开始扫描 BluetoothAdapter.getDefaultAdapter().startDiscovery(); // 创建回调类 private final BluetoothGattCallback mGattCallback = new BluetoothGattCallback() { @Override public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) { super.onConnectionStateChange(gatt, status, newState); if (newState == BluetoothProfile.STATE_CONNECTED) { gatt.discoverServices(); // 发现服务 } } @Override public void onServicesDiscovered(BluetoothGatt gatt, int status) { super.onServicesDiscovered(gatt, status); if (status == BluetoothGatt.GATT_SUCCESS) { List<BluetoothGattService> services = gatt.getServices(); for (BluetoothGattService service : services) { // 遍历服务 List<BluetoothGattCharacteristic> characteristics = service.getCharacteristics(); for (BluetoothGattCharacteristic characteristic : characteristics) { // 遍历特征 gatt.readCharacteristic(characteristic); // 读取特征值 } } } } @Override public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) { super.onCharacteristicRead(gatt, characteristic, status); if (status == BluetoothGatt.GATT_SUCCESS) { // 处理读取到的数据 } } @Override public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) { super.onCharacteristicChanged(gatt, characteristic); // 处理特征值变化事件 } }; // 连接设备 BluetoothGatt gatt = device.connectGatt(context, false, mGattCallback); ``` #### 五、总结 本文详细介绍了Android平台下的蓝牙连接通信实现技术,包括关键组件的作用、工作流程以及示例代码。蓝牙通信是移动设备间数据交换的重要手段之一,了解其工作机制对于开发支持蓝牙功能的应用程序至关重要。希望本文能为相关领域的开发者提供有益的帮助和参考。
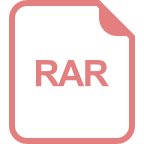
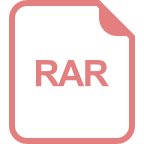
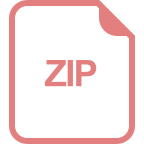
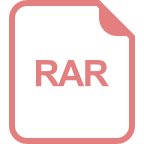
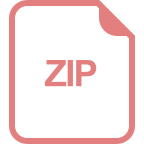
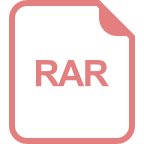
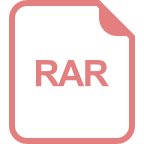
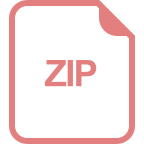
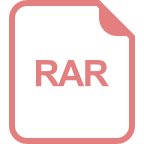
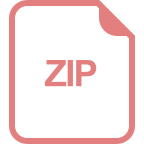
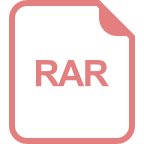
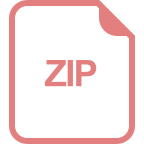
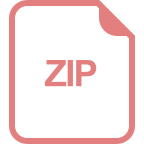
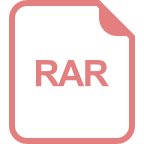
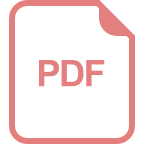
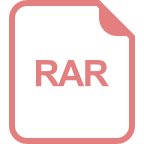
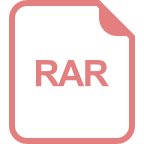
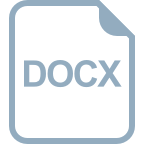
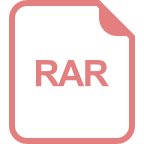
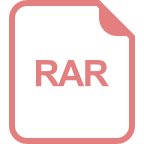
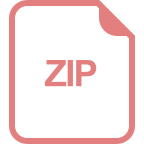
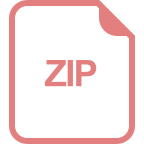
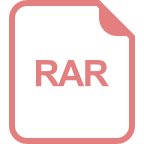
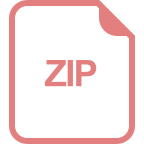
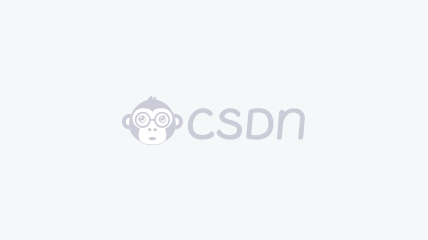

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

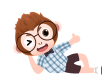
最新资源
- 数据结构--实验报告2.docx
- 本软件包是用于Windows下往云端上传代码的工具
- MySQL-server-5.6.22-1.linux_glibc2.5.x86_64.rpm
- 基于Vue开发的碳排放数据可视化+源码+项目文档(毕业设计&课程设计&项目开发)
- 20241125-国外立体仓库丹巴赫堆垛机博图PLC项目&电气图纸PDF
- 信息化在线教学平台-毕业设计,基于Python+Django+Vue+MySql开发,源码+数据库+毕业论文+视频演示
- 基于PyTorch和Transformers框架的双语和跨语言嵌入模型(含项目源码、使用教程).zip
- CUMT自然语言处理报告-Qwen2大模型实现命名实体识别
- 毕业设计,基于Python+Django+Vue+MySql开发的信息化在线教学平台,内含完整源代码,数据库,毕业论文,视频教程
- 通信原理-AMI编译码(归零码)实验报告

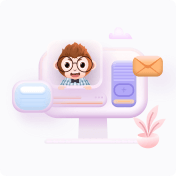
