# Google-AAD
考纲:https://developers.google.com/training/certification/associate-android-developer/study-guide/
# Table of Contents:
- [Google-AAD](#google-aad)
- [Android Core:](#android-core)
- [Understand the architecture of the Android system](#understand-the-architecture-of-the-android-system)
- [Android Basics](#android-basics)
- [Four app components:](#four-app-components)
- [Be able to describe the basic building blocks of an Android app](#be-able-to-describe-the-basic-building-blocks-of-an-android-app)
- [Know how to build and run an Android app](#know-how-to-build-and-run-an-android-app)
- [Display simple messages in a popup using a Toast or a Snackbar](#display-simple-messages-in-a-popup-using-a-toast-or-a-snackbar)
- [Toast:](#toast)
- [Snackbar:](#snackbar)
- [Be able to display a message outside your app's UI using Notifications](#be-able-to-display-a-message-outside-your-apps-ui-using-notifications)
- [Create Channel:](#create-channel)
- [Builder:](#builder)
- [Show:](#show)
- [Understand how to localize an app](#understand-how-to-localize-an-app)
- [Doc](#doc)
- [Codelab](#codelab)
- [Be able to schedule a background task using JobScheduler](#be-able-to-schedule-a-background-task-using-jobscheduler)
- [CodeLab](#codelab-1)
- [User interface:](#user-interface)
- [Understand the Android activity lifecycle](#understand-the-android-activity-lifecycle)
- [Be able to create an Activity that displays a Layout](#be-able-to-create-an-activity-that-displays-a-layout)
- [Be able to construct a UI with ConstraintLayout](#be-able-to-construct-a-ui-with-constraintlayout)
- [Understand how to create a custom View class and add it to a Layout](#understand-how-to-create-a-custom-view-class-and-add-it-to-a-layout)
- [Know how to implement a custom app theme](#know-how-to-implement-a-custom-app-theme)
- [Be able to add accessibility hooks to a custom View](#be-able-to-add-accessibility-hooks-to-a-custom-view)
- [Know how to apply content descriptions to views for accessibility](#know-how-to-apply-content-descriptions-to-views-for-accessibility)
- [Understand how to display items in a RecyclerView](#understand-how-to-display-items-in-a-recyclerview)
- [Be able to bind local data to a RecyclerView list using the Paging library](#be-able-to-bind-local-data-to-a-recyclerview-list-using-the-paging-library)
- [Know how to implement menu-based navigation](#know-how-to-implement-menu-based-navigation)
- [Understand how to implement drawer navigation](#understand-how-to-implement-drawer-navigation)
- [DataManagement:](#datamanagement)
- [Understand how to define data using Room entities](#understand-how-to-define-data-using-room-entities)
- [Be able to access Room database with data access object (DAO)](#be-able-to-access-room-database-with-data-access-object-dao)
- [Know how to observe and respond to changing data using LiveData](#know-how-to-observe-and-respond-to-changing-data-using-livedata)
- [Understand how to use a Repository to mediate data operations](#understand-how-to-use-a-repository-to-mediate-data-operations)
- [Be able to read and parse raw resources or asset files](#be-able-to-read-and-parse-raw-resources-or-asset-files)
- [Be able to create persistent Preference data from user input](#be-able-to-create-persistent-preference-data-from-user-input)
- [Understand how to change the behavior of the app based on user preferences](#understand-how-to-change-the-behavior-of-the-app-based-on-user-preferences)
- [Debugging:](#debugging)
- [Testing:](#testing)
- [Espresso:](#espresso)
- [UI Automator:](#ui-automator)
- [JUnit:](#junit)
## Android Core:
### Understand the architecture of the Android system
#### [Android Basics](https://developer.android.com/guide/components/fundamentals)
#### Four app components:
* [Activities](https://developer.android.com/guide/components/activities/intro-activities)
Activity life cycle:
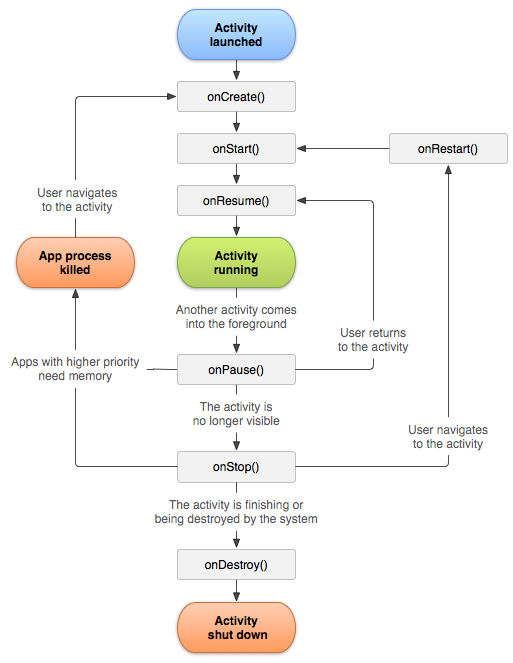
Fragment life cycle:

* [Services](https://developer.android.com/reference/android/app/Service)
A Service itself is actually very simple, providing two main features:
A facility for the application to tell the system about something it wants to be doing in the background (even when the user is not directly interacting with the application). This corresponds to calls to `Context.startService()`, which ask the system to schedule work for the service, to be run until the service or someone else explicitly stop it.
A facility for an application to expose some of its functionality to other applications. This corresponds to calls to` Context.bindService()`, which allows a long-standing connection to be made to the service in order to interact with it.
* [BroadcastReveivers](https://developer.android.com/guide/components/broadcasts#system-broadcasts)
* [ContentProviders](https://developer.android.com/guide/topics/providers/content-providers)
[Room with content provider example](https://github.com/googlesamples/android-architecture-components/tree/master/PersistenceContentProviderSample)
```xml
<provider
android:name=".provider.SampleContentProvider"
android:authorities="com.example.android.contentprovidersample.provider"
android:exported="true"
android:permission="com.example.android.contentprovidersample.provider.READ_WRITE"/>
```
```java
/**
* A {@link ContentProvider} based on a Room database.
*
* <p>Note that you don't need to implement a ContentProvider unless you want to expose the data
* outside your process or your application already uses a ContentProvider.</p>
*/
public class SampleContentProvider extends ContentProvider {
/** The authority of this content provider. */
public static final String AUTHORITY = "com.example.android.contentprovidersample.provider";
/** The URI for the Cheese table. */
public static final Uri URI_CHEESE = Uri.parse(
"content://" + AUTHORITY + "/" + Cheese.TABLE_NAME);
/** The match code for some items in the Cheese table. */
private static final int CODE_CHEESE_DIR = 1;
/** The match code for an item in the Cheese table. */
private static final int CODE_CHEESE_ITEM = 2;
/** The URI matcher. */
private static final UriMatcher MATCHER = new UriMatcher(UriMatcher.NO_MATCH);
static {
MATCHER.addURI(AUTHORITY, Cheese.TABLE_NAME, CODE_CHEESE_DIR);
MATCHER.addURI(AUTHORITY, Cheese.TABLE_NAME + "/*", CODE_CHEESE_ITEM);
}
@Override
public boolean onCreate() {
return true;
}
@Nullable
@Override
public Cursor query(@NonNull Uri uri, @Nullable String[] projection, @Nullable String selection,
@Nullable String[] selectionArgs, @Nullable String sortOrder) {
final int code = MATCHER.match(uri);
if (code == CODE_CHEESE_DIR || code == CODE_CHEESE_ITEM) {
final Context context = getContext();
if (context == null) {
return null;
}
CheeseDao cheese = SampleDatabase.getInstance(context).cheese();
final Cursor cursor;
if (code == CODE_CHEESE_DIR) {
cursor = cheese.selectAll();
} else {
cursor = cheese.selectById(ContentUris.parseId(uri));
}
cursor.setNotificationUri(context.getContentResolver(), uri);
return cursor;
} else {
throw new IllegalArgumentException("Unknown URI: " + uri);
}
}
@Nullable
@Override
public String getType(@NonNull Uri uri) {
switch (MATCHER.match(uri)) {
case CODE_CHEESE_DIR:
return "vnd.android.cursor.dir/" + AUTHORITY + "." + Cheese.TABLE_NAME;
case CODE_CHEESE_ITEM:
return "vnd.android.cursor.item/" + AUT