// ProfileDialog.java 设置邮件服务器、POP/SMTP参数设置
package javamailsystem;
import java.awt.*;
import javax.swing.*;
import com.borland.jbcl.layout.*;
import javax.swing.border.*;
import java.util.Properties;
import java.io.*;
import java.awt.event.*;
public class ProfileDialog extends JDialog {
private static final long serialVersionUID = 1L;
Border border1;
Border border2;
BorderLayout borderLayout1 = new BorderLayout();
JLabel jLabel1 = new JLabel();
JLabel jLabel2 = new JLabel();
JLabel jLabel3 = new JLabel();
JLabel jLabel4 = new JLabel();
JLabel jLabel5 = new JLabel();
JLabel jLabel6 = new JLabel();
JLabel jLabel7 = new JLabel();
JLabel jLabel8 = new JLabel();
JPanel jPanel1 = new JPanel();
JPanel jPanel2 = new JPanel();
JPanel jPanel3 = new JPanel();
JPanel jPanel4 = new JPanel();
JTabbedPane jTabbedPane1 = new JTabbedPane();
JPanel panel1 = new JPanel();
JTextField popAddressField = new JTextField();
JButton popCancelButton = new JButton();
JTextField popHostField = new JTextField();
JButton popOkButton = new JButton();
JPasswordField popPassword = new JPasswordField();
JTextField popUserNameField = new JTextField();
JTextField smtpAddressField = new JTextField();
JButton smtpCancelCancel = new JButton();
JTextField smtpHostField = new JTextField();
JButton smtpOkButton = new JButton();
JPasswordField smtpPassword = new JPasswordField();
JTextField smtpUserNameField = new JTextField();
TitledBorder titledBorder1;
XYLayout xYLayout1 = new XYLayout();
XYLayout xYLayout2 = new XYLayout();
XYLayout xYLayout3 = new XYLayout();
XYLayout xYLayout4 = new XYLayout();
public ProfileDialog() {
this(null, "", false);
}
public ProfileDialog(Frame frame, String title, boolean modal) {
super(frame, title, modal);
try {
jbInit();
pack();
}
catch(Exception ex) {
ex.printStackTrace();
}
}
private void jbInit() throws Exception {
border1 = BorderFactory.createLineBorder(SystemColor.controlText,1);
titledBorder1 = new TitledBorder(new EtchedBorder(EtchedBorder.RAISED,Color.white,new Color(148, 145, 140)),"POP3属性");
border2 = BorderFactory.createCompoundBorder(new TitledBorder(new EtchedBorder(EtchedBorder.RAISED,Color.white,new Color(148, 145, 140)),""),BorderFactory.createEmptyBorder(6,6,6,6));
panel1.setLayout(borderLayout1);
panel1.setPreferredSize(new Dimension(400, 300));
jPanel1.setLayout(xYLayout1);
jPanel3.setBorder(border2);
jPanel3.setLayout(xYLayout2);
popOkButton.setFont(new java.awt.Font("Dialog", 0, 13));
popOkButton.setText("确 定");
popOkButton.addActionListener(new ProfileDialog_popOkButton_actionAdapter(this));
popCancelButton.setText("取 消");
popCancelButton.addActionListener(new ProfileDialog_popOkButton_actionAdapter(this));
popCancelButton.setFont(new java.awt.Font("Dialog", 0, 13));
jLabel1.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel1.setText("POP3服务器:");
popHostField.setFont(new java.awt.Font("Dialog", 0, 12));
popHostField.setText("");
jLabel3.setText("帐 户 名:");
jLabel3.setFont(new java.awt.Font("Dialog", 0, 12));
popUserNameField.setFont(new java.awt.Font("Dialog", 0, 12));
popUserNameField.setText("");
jLabel4.setText("密 码:");
jLabel4.setFont(new java.awt.Font("Dialog", 0, 12));
jPanel2.setLayout(xYLayout3);
jLabel5.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel5.setText("密 码:");
jLabel7.setText("SMTP服务器:");
jLabel7.setFont(new java.awt.Font("Dialog", 0, 12));
smtpHostField.setText("");
smtpHostField.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel8.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel8.setText("帐 户 名:");
smtpUserNameField.setText("");
smtpUserNameField.setFont(new java.awt.Font("Dialog", 0, 12));
jPanel4.setLayout(xYLayout4);
jPanel4.setBorder(border2);
smtpCancelCancel.setFont(new java.awt.Font("Dialog", 0, 13));
smtpCancelCancel.setText("取 消");
smtpCancelCancel.addActionListener(new ProfileDialog_smtpOkButton_actionAdapter(this));
smtpOkButton.setText("确 定");
smtpOkButton.addActionListener(new ProfileDialog_smtpOkButton_actionAdapter(this));
smtpOkButton.setFont(new java.awt.Font("Dialog", 0, 13));
popPassword.setText("");
smtpPassword.setText("");
jLabel2.setText("Email址址:");
jLabel2.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel6.setFont(new java.awt.Font("Dialog", 0, 12));
jLabel6.setText("Email址址:");
getContentPane().add(panel1);
panel1.add(jTabbedPane1, BorderLayout.CENTER);
jTabbedPane1.add(jPanel1, "POP3设置");
jPanel3.add(popPassword, new XYConstraints(108, 126, 235, 30));
jPanel3.add(jLabel4, new XYConstraints(17, 125, 84, 29));
jPanel3.add(popUserNameField, new XYConstraints(108, 84, 233, 28));
jPanel3.add(jLabel3, new XYConstraints(17, 83, 84, 29));
jPanel3.add(popAddressField, new XYConstraints(108, 42, 233, 28));
jPanel3.add(jLabel2, new XYConstraints(17, 42, 84, 29));
jPanel3.add(popHostField, new XYConstraints(108, 0, 233, 28));
jPanel3.add(jLabel1, new XYConstraints(17, 0, 84, 29));
jPanel1.add(popOkButton, new XYConstraints(91, 229, 84, 28));
jPanel1.add(popCancelButton, new XYConstraints(224, 229, 84, 28));
jPanel1.add(jPanel3, new XYConstraints(13, 21, 374, 199));
jTabbedPane1.add(jPanel2, "SMTP设置");
jPanel4.add(smtpHostField, new XYConstraints(110, 0, 233, 28));
jPanel4.add(jLabel7, new XYConstraints(19, 0, 84, 29));
jPanel2.add(jPanel4, new XYConstraints(9, 22, 377, 197));
jPanel2.add(smtpOkButton, new XYConstraints(90, 232, 84, 28));
jPanel2.add(smtpCancelCancel, new XYConstraints(220, 232, 84, 28));
jPanel4.add(smtpPassword, new XYConstraints(110, 126, 234, 29));
jPanel4.add(jLabel5, new XYConstraints(19, 126, 84, 29));
jPanel4.add(jLabel8, new XYConstraints(19, 84, 84, 29));
jPanel4.add(smtpUserNameField, new XYConstraints(110, 84, 233, 28));
jPanel4.add(smtpAddressField, new XYConstraints(110, 42, 233, 28));
jPanel4.add(jLabel6, new XYConstraints(19, 42, 84, 29));
loadPopProperties();
loadSmtpProperties();
}
//
void loadPopProperties(){
Properties p=new Properties();
try{
//装载pop3属性
FileInputStream in = new FileInputStream("pop3.properties");
p.load(in);
popHostField.setText(p.getProperty("pop3.host"));
popAddressField.setText(p.getProperty("pop3.address"));
popUserNameField.setText(p.getProperty("pop3.username"));
popPassword.setText(p.getProperty("pop3.password"));
in.close();
}catch(IOException e){
e.printStackTrace();
}
}
void loadSmtpProperties(){
Properties p=new Properties();
try{
//装载smtp属性
FileInputStream in=new FileInputStream("smtp.properties");
p.load(in);
smtpHostField.setText(p.getProperty("smtp.host"));
smtpAddressField.setText(p.getProperty("smtp.address"));
smtpUserNameField.setText(p.getProperty("smtp.username"));
smtpPassword.setText(p.getProperty("smtp.password"));
in.close();
}catch(IOException e){
e.printStackTrace();
}
}
void popCancelButton_actionPerformed(ActionEvent e) {
loadPopProperties();
}
void popOkButton_actionPerformed(ActionEvent e) {
savePopProperties();
}
void savePopProperties(){
Properties p=new Properties();
try{
//保存pop3设置
FileOutputStream out=new FileOutputStream("pop3.properties");
p.setProperty("pop3.host",popHostField.getText());
p.setProperty("pop3.address",popAddressFiel
没有合适的资源?快使用搜索试试~ 我知道了~
基于Java的mail的邮件收发系统
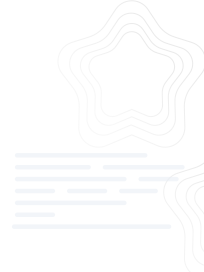
共76个文件
class:44个
java:16个
jar:8个

需积分: 0 17 下载量 144 浏览量
2023-05-18
09:51:51
上传
评论 2
收藏 36.94MB RAR 举报
温馨提示
电子邮件的研究也存在着以下的问题: (1) Unix式的平台 在Unix平台下以Sendmail的资格最老,但是由于Unix系统是开发源代码的,所以导致Sendmail有不少代码缺陷和漏洞,如有些代码缺陷可以让攻击者远程地利用红帽子或SlackwareLinux软件的计算机,APP漏洞,即用sendmail系统的APP漏洞能够取得root权限。 (2) Winodws式的平台 在windows领域种类繁多的邮件服务器中,微软的Exchange排在首位,但是它存在着以下问题: a 该系统只能运行在Windows NT上,而WinNT本身的不可靠、不稳定决定了Exchange Server的不稳定、不可靠。 b Exchange Server具有严重的内存泄漏问题,随着系统运行时间的增加会越来越慢,平均至少要一周重启一次。 c Windows NT系统面临着巨大的病毒感染隐患,一旦Exchange Server感染病毒,会造成Exchange Server本身的瘫痪,感染速度惊人。杀毒软件只会“亡羊补牢”。 d Exchange Server对邮件账户的支持是非常有限的,一般超过200
资源推荐
资源详情
资源评论
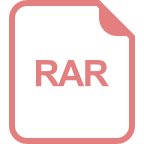
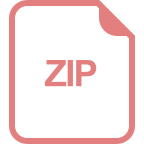
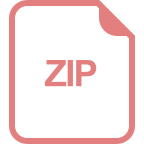
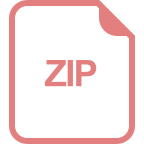
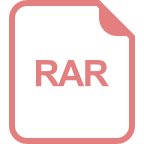
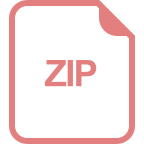
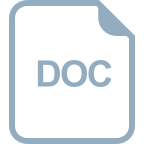
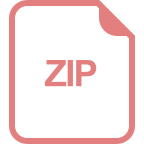
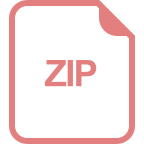
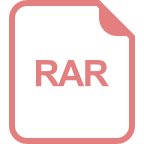
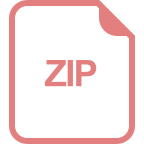
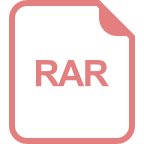
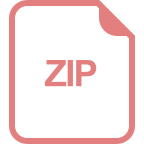
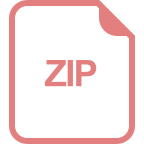
收起资源包目录




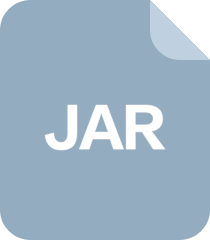
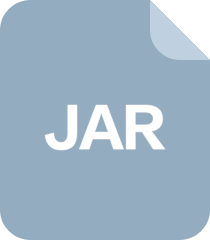
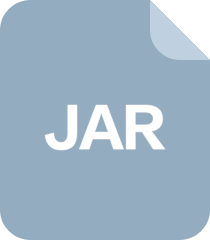
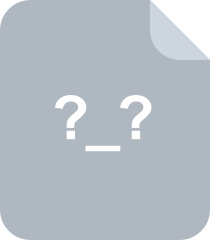
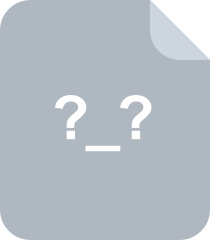


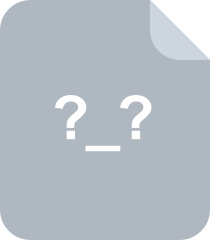
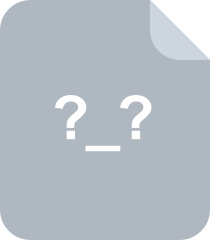
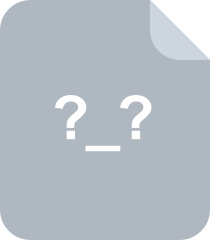
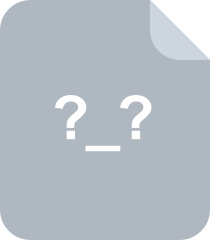
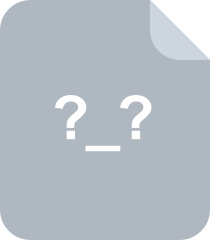
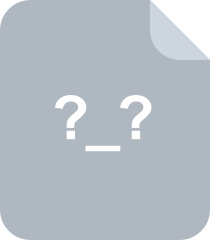
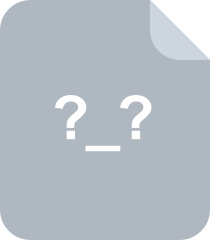
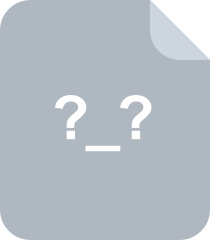


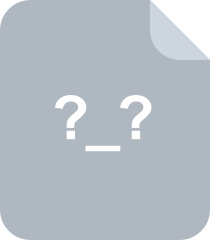
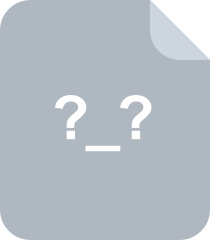
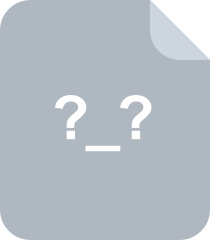
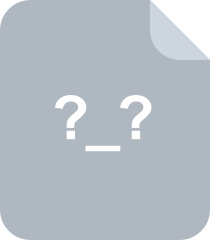
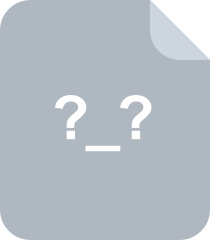
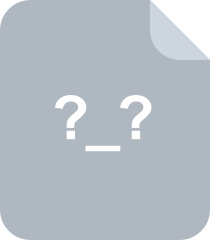
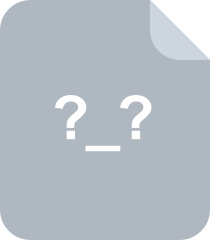
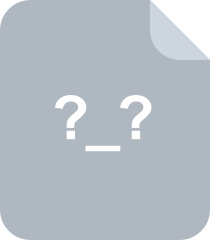
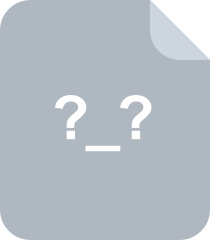
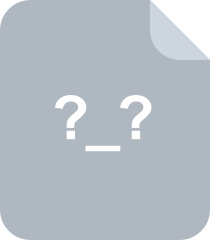
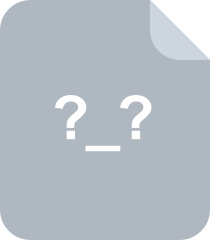
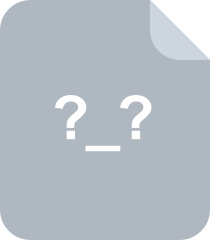
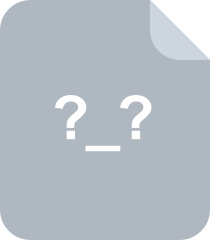
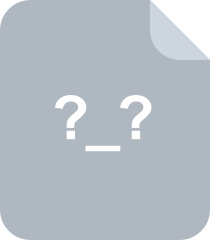
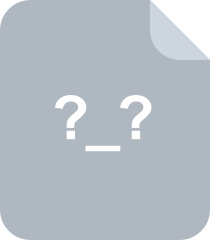
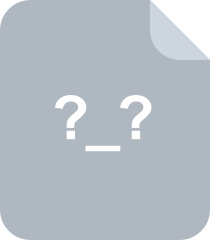
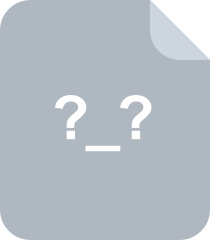
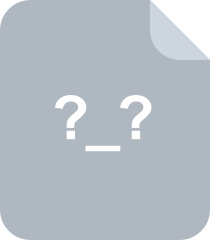
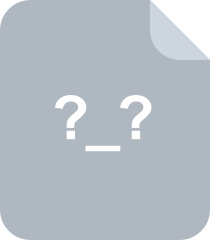
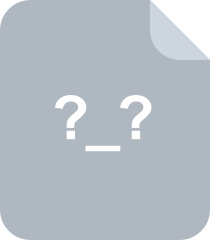
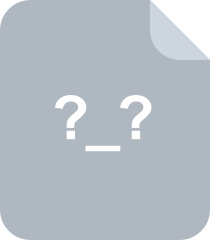
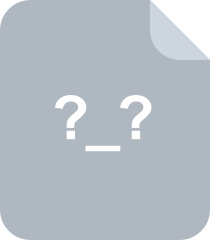
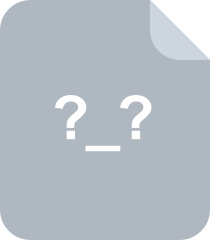
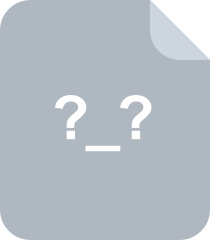
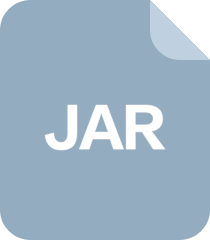


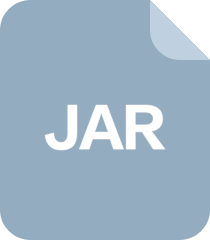
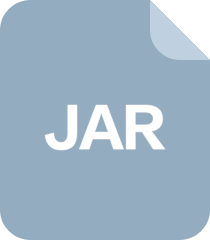
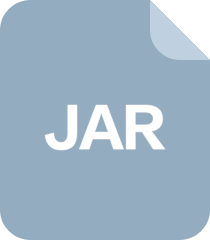
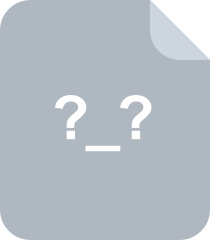
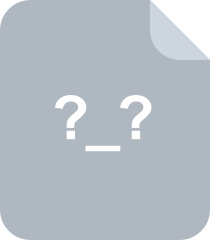


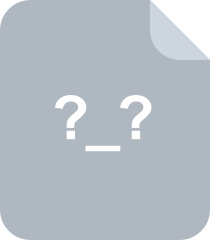
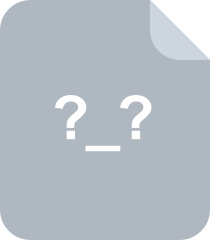
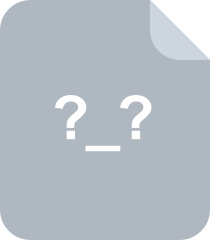
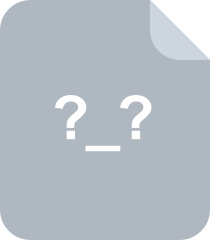
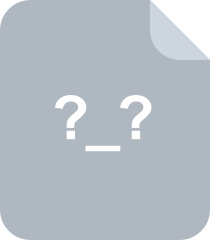
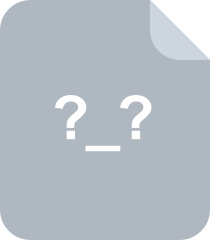
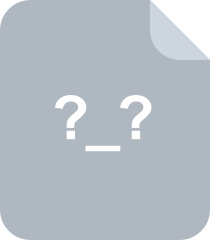
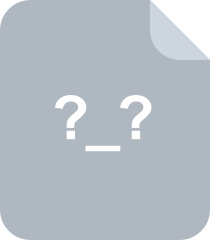


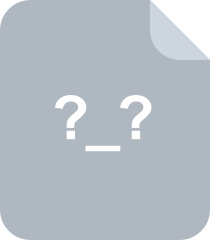
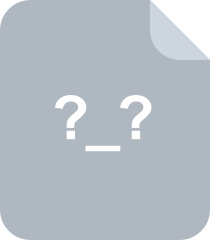
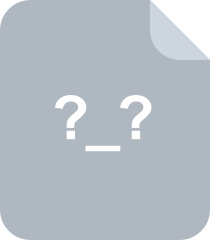
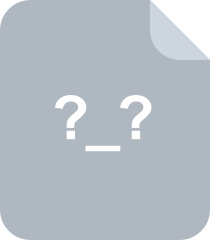
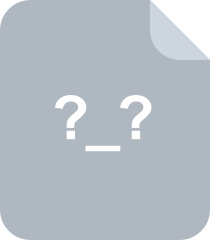
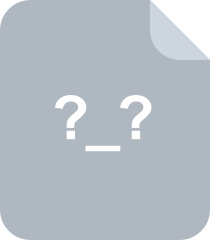
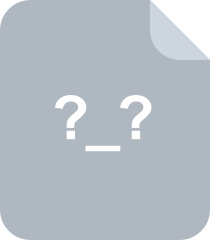
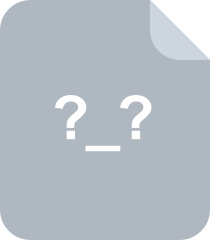
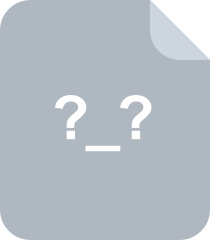
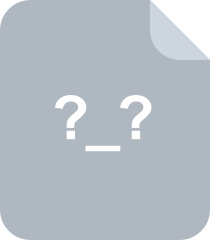
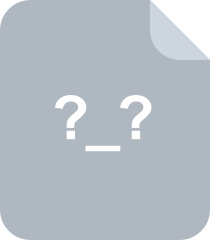
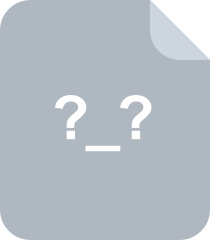
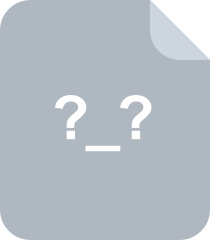
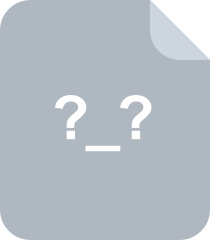
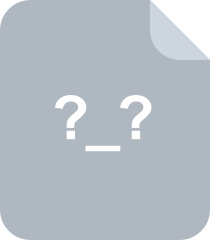
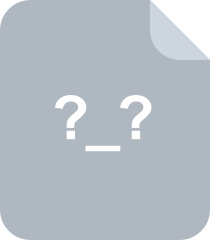
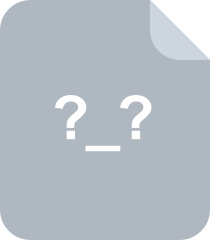
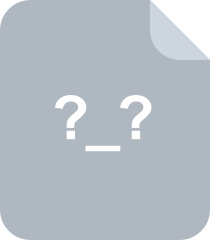
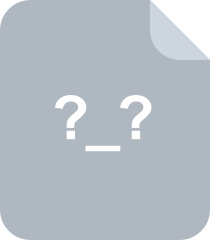
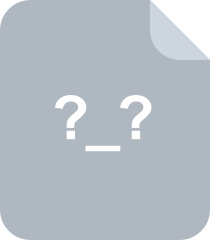
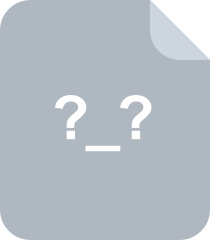
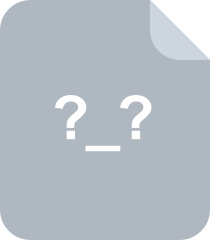
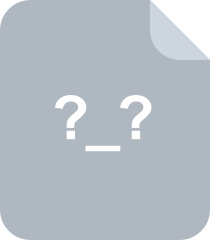
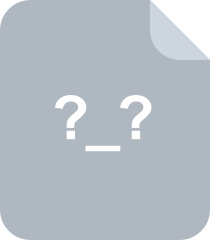
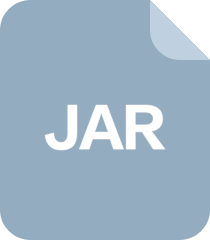
共 76 条
- 1
资源评论
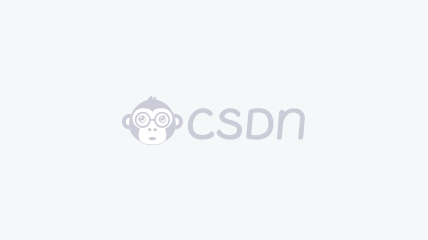

一叶再见知秋
- 粉丝: 3
- 资源: 172
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

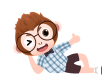
最新资源
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
- (源码)基于Spring Boot和Vue的房屋租赁管理系统.zip
- (源码)基于Android的饭店点菜系统.zip
- (源码)基于Android平台的权限管理系统.zip
- (源码)基于CC++和wxWidgets框架的LEGO模型火车控制系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


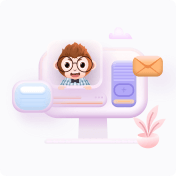
安全验证
文档复制为VIP权益,开通VIP直接复制
