export const revisePath = (path) => {
if (!path) {
return "";
}
if (path[0] == "/") {
return path;
} else {
return `/${path}`;
}
};
export function getUrlParam(name) {
var reg = new RegExp("(^|&)" + name + "=([^&]*)(&|$)");
var r = window.location.search.substr(1).match(reg);
if (r != null) return decodeURIComponent(r[2]);
return null;
}
export function firstMenu(list) {
let path = "";
const fn = (arr) => {
arr.forEach((e) => {
if (e.type == 1) {
if (!path) {
path = e.path;
}
} else {
fn(e.children);
}
});
};
fn(list);
return path || "/404";
}
export function orderBy(list, key) {
return list.sort((a, b) => a[key] - b[key]);
}
export function deepTree(list) {
let newList = [];
let map = {};
list.forEach((e) => (map[e.id] = e));
list.forEach((e) => {
let parent = map[e.parentId];
if (parent) {
(parent.children || (parent.children = [])).push(e);
} else {
if (!e.parentId) {
newList.push(e);
}
}
});
const fn = (list) => {
list.map((e) => {
if (e.children instanceof Array) {
e.children = orderBy(e.children, "orderNum");
fn(e.children);
}
});
};
fn(newList);
return orderBy(newList, "orderNum");
}
export function revDeepTree(list = []) {
let d = [];
const deep = (list) => {
list.forEach((e) => {
d.push(e);
if (e.children && isArray(e.children)) {
deep(e.children);
}
});
};
deep(list || []);
return d;
}
export function debounce(fn, delay) {
let timer = null;
return function () {
let args = arguments;
let context = this;
if (timer) {
clearTimeout(timer);
timer = setTimeout(function () {
fn.apply(context, args);
}, delay);
} else {
timer = setTimeout(function () {
fn.apply(context, args);
}, delay);
}
};
}
export function isArray(value) {
if (typeof Array.isArray === "function") {
return Array.isArray(value);
} else {
return Object.prototype.toString.call(value) === "[object Array]";
}
}
export function isObject(value) {
return Object.prototype.toString.call(value) === "[object Object]";
}
export function isNumber(value) {
return !isNaN(Number(value));
}
export function isFunction(value) {
return typeof value == "function";
}
export function isString(value) {
return typeof value == "string";
}
export function isEmpty(value) {
if (isArray(value)) {
return value.length === 0;
}
if (isObject(value)) {
return Object.keys(value).length === 0;
}
return value === "" || value === undefined || value === null;
}
export function cloneDeep(obj) {
let d = isArray(obj) ? obj : {};
if (isObject(obj)) {
for (let key in obj) {
if (obj.hasOwnProperty && obj.hasOwnProperty(key)) {
if (obj[key] && typeof obj[key] === "object") {
d[key] = cloneDeep(obj[key]);
} else {
d[key] = obj[key];
}
}
}
}
return d;
}
export function clone(obj) {
return Object.create(Object.getPrototypeOf(obj), Object.getOwnPropertyDescriptors(obj));
}
export function certainProperty(obj, keys) {
return keys.reduce((result, key) => {
if (obj.hasOwnProperty(key)) {
result[key] = obj[key];
}
return result;
}, {});
}
export function deepMerge(a, b) {
let k;
for (k in b) {
a[k] =
a[k] && a[k].toString() === "[object Object]" ? deepMerge(a[k], b[k]) : (a[k] = b[k]);
}
return a;
}
export function contains(parent, node) {
if (document.documentElement.contains) {
return parent !== node && parent.contains(node);
} else {
while (node && (node = node.parentNode)) if (node === parent) return true;
return false;
}
}
export function moreList(res, { list, pagination }) {
const { page, size } = res.pagination;
const len = res.list.length;
const max = list.length;
if (page == 1) {
list.splice(0, max, ...res.list);
} else {
let start = max - (max % size);
let end = start + len;
list.splice(start, end, ...res.list);
}
if (len == size) {
res.pagination.page += 1;
}
Object.assign(pagination, res.pagination);
return page != 1;
}
export function isPc() {
const userAgentInfo = navigator.userAgent;
const Agents = ["Android", "iPhone", "SymbianOS", "Windows Phone", "iPad", "iPod"];
let flag = true;
for (let v = 0; v < Agents.length; v++) {
if (userAgentInfo.indexOf(Agents[v]) > 0) {
flag = false;
break;
}
}
return flag;
}
export const isIOS = !!navigator.userAgent.match(/\(i[^;]+;( U;)? CPU.+Mac OS X/);
export function getBrowser() {
let ua = navigator.userAgent.toLowerCase();
let btypeInfo = (ua.match(/firefox|chrome|safari|opera/g) || "other")[0];
if ((ua.match(/msie|trident/g) || [])[0]) {
btypeInfo = "msie";
}
let pc = "";
let prefix = "";
let plat = "";
let isTocuh =
"ontouchstart" in window || ua.indexOf("touch") !== -1 || ua.indexOf("mobile") !== -1;
if (isTocuh) {
if (ua.indexOf("ipad") !== -1) {
pc = "pad";
} else if (ua.indexOf("mobile") !== -1) {
pc = "mobile";
} else if (ua.indexOf("android") !== -1) {
pc = "androidPad";
} else {
pc = "pc";
}
} else {
pc = "pc";
}
switch (btypeInfo) {
case "chrome":
case "safari":
case "mobile":
prefix = "webkit";
break;
case "msie":
prefix = "ms";
break;
case "firefox":
prefix = "Moz";
break;
case "opera":
prefix = "O";
break;
default:
prefix = "webkit";
break;
}
plat = ua.indexOf("android") > 0 ? "android" : navigator.platform.toLowerCase();
return {
version: (ua.match(/[\s\S]+(?:rv|it|ra|ie)[\/: ]([\d.]+)/) || [])[1],
plat: plat,
type: btypeInfo,
pc: pc,
prefix: prefix,
isMobile: pc == "pc" ? false : true
};
}
没有合适的资源?快使用搜索试试~ 我知道了~
COOL-ADMIN 基于uniCloud后台通用管理系统
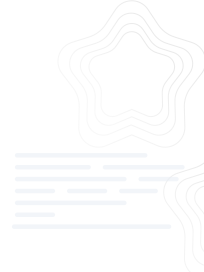
共266个文件
svg:112个
js:69个
vue:50个

需积分: 5 1 下载量 29 浏览量
2023-03-22
00:42:11
上传
评论 1
收藏 263KB ZIP 举报
温馨提示
0.0.9(2021-03-17) 适配手机端; 调整前端目录结构; 0.0.8(2020-09-02) 精简优化云函数依赖包 更新通用crud到最新版 修复分页显示问题 0.0.6(2020-09-02) 精简优化云函数依赖包 更新通用crud到最新版 修复分页显示问题 0.0.5(2020-08-08) 新增视频上传 新增优化图片空间 优化上传逻辑 0.0.4(2020-08-07) 优化cl-upload组件 修复sys_data索引过长导致的报错 0.0.2(2020-08-06) 修改文案 v0.0.1(2020-08-06) 新增用户、角色、菜单、部门管理 新增多种组件如:图片空间、邀请码、右键菜单等 新增登录和权限校验 新增通用的云函数框架 新增权限控制 同步COOL-ADMIN-PRO新特性
资源推荐
资源详情
资源评论
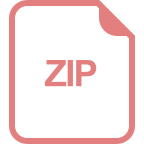
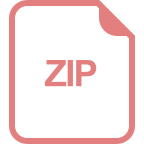
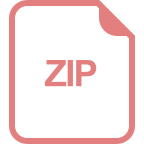
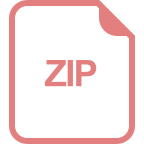
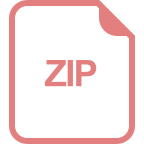
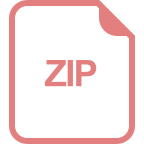
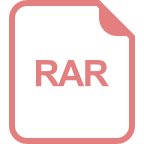
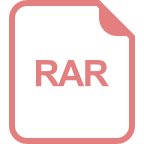
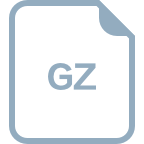
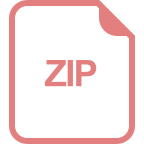
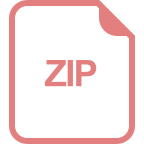
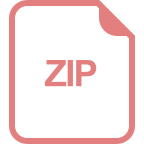
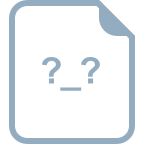
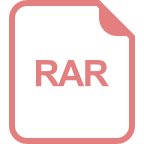
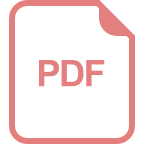
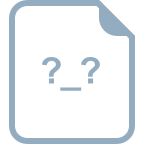
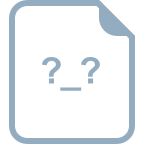
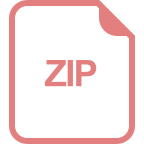
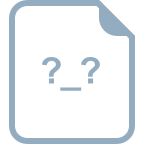
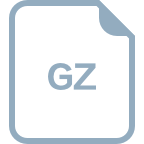
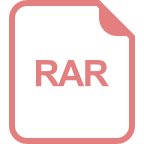
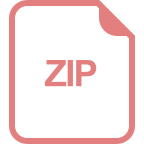
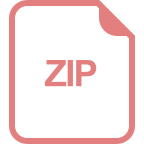
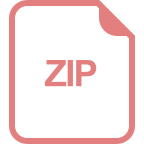
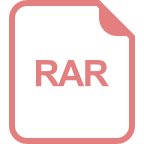
收起资源包目录

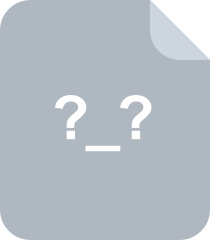
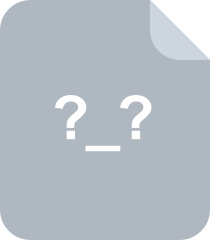
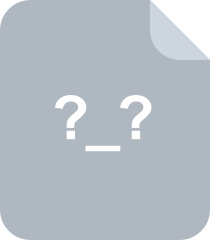
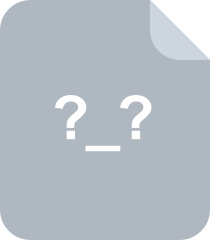
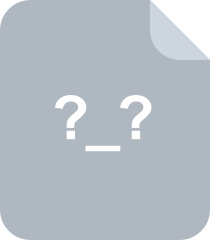
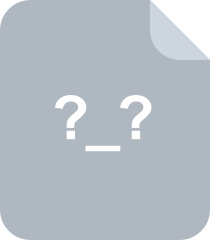
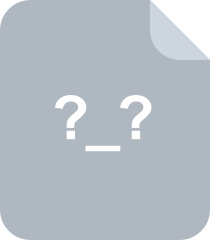
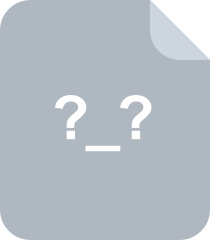
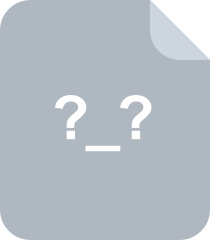
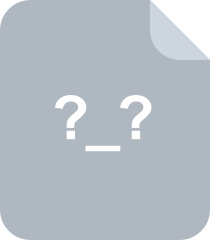
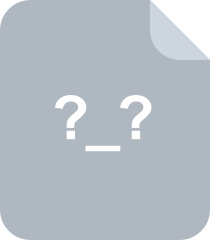
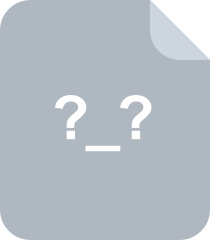
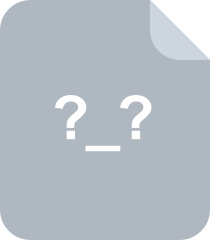
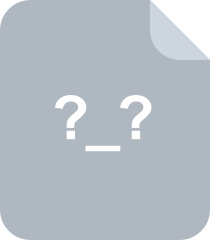
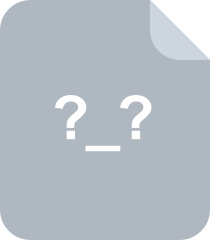
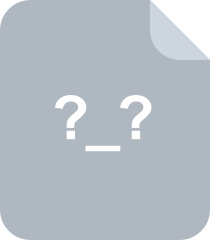
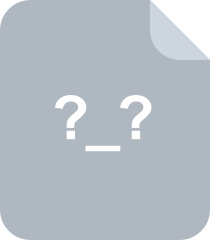
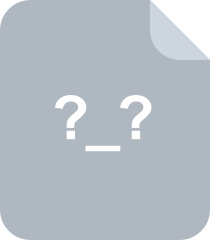
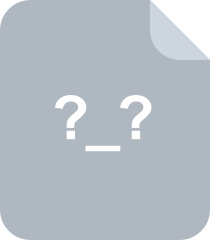
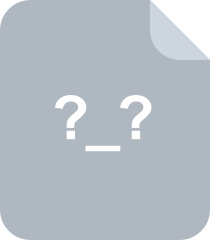
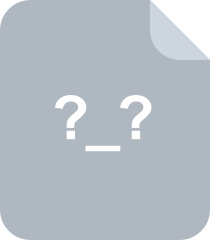
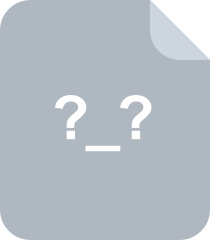
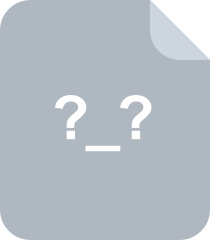
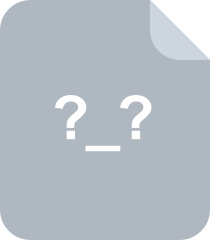
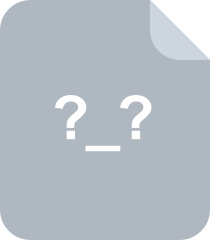
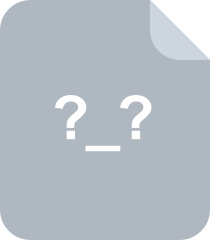
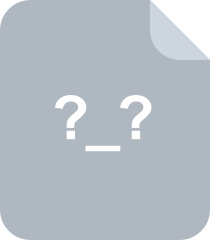
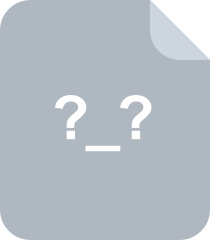
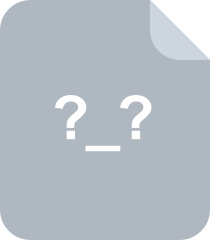
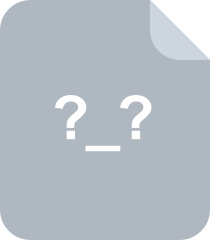
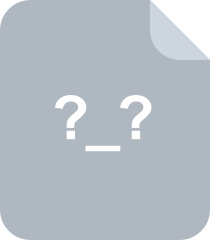
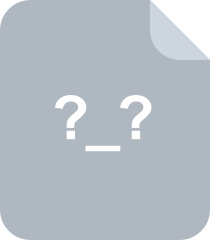
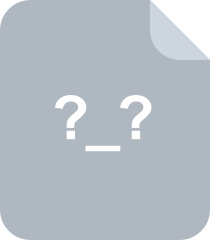
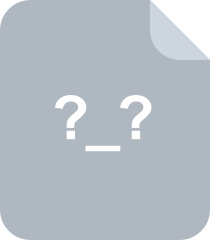
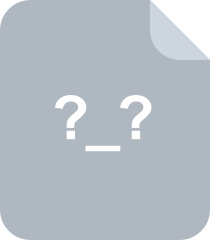
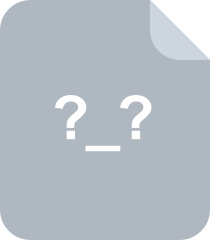
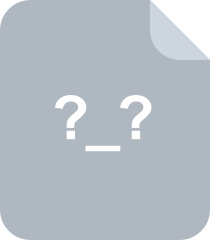
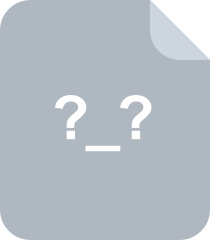
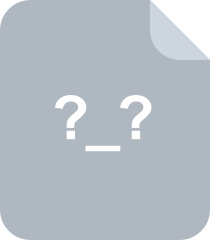
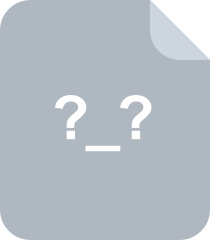
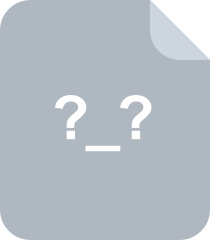
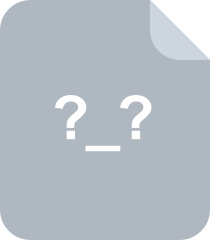
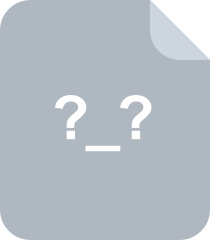
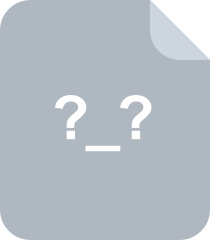
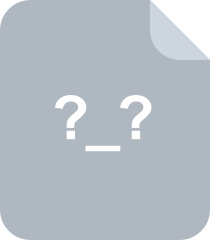
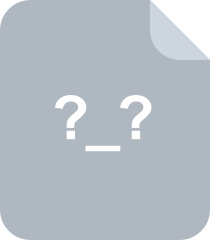
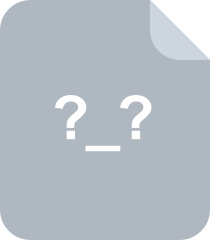
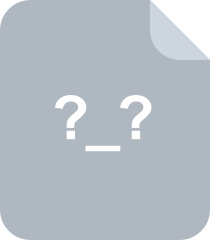
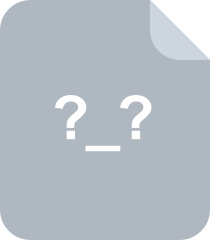
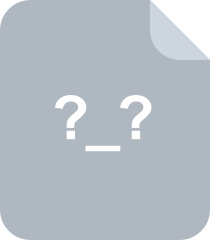
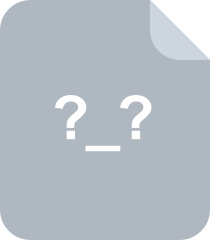
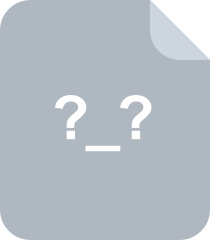
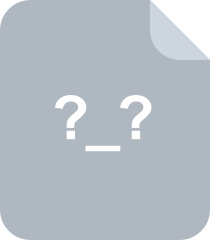
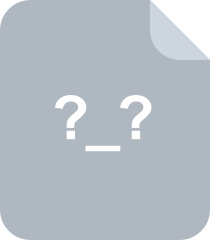
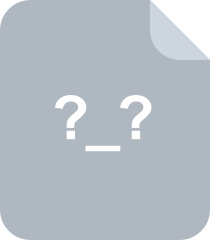
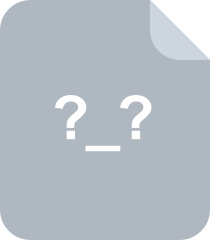
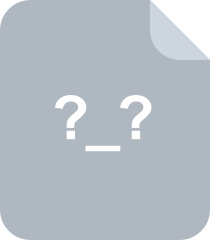
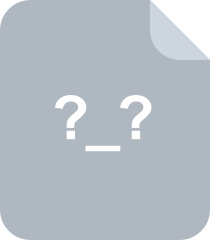
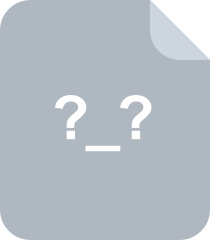
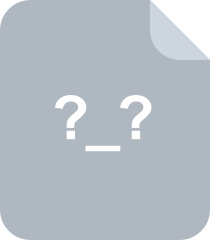
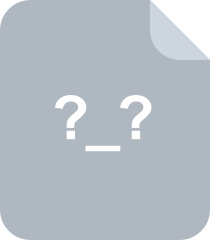
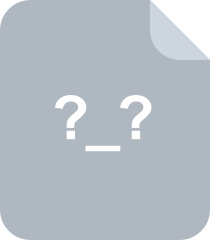
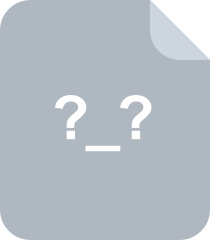
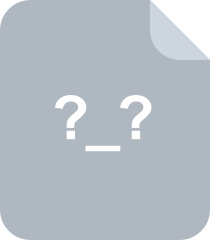
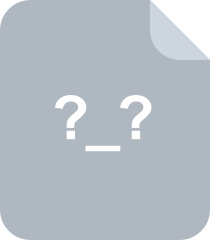
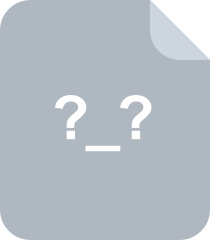
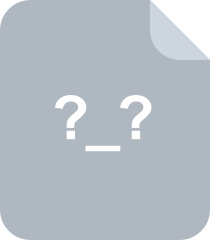
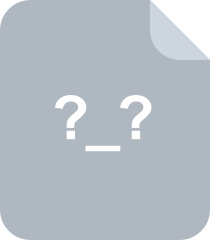
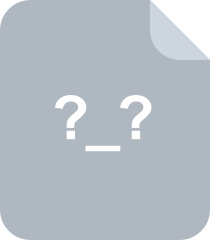
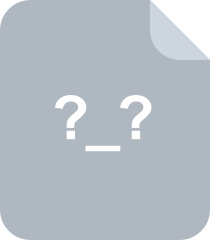
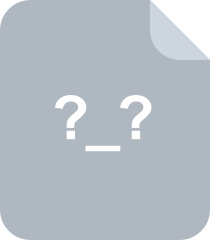
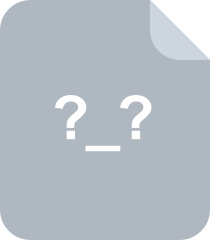
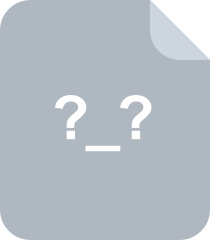
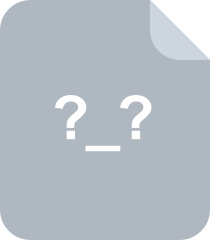
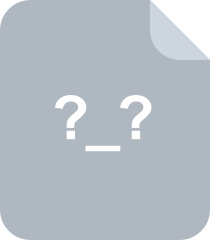
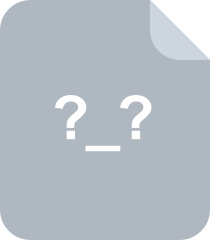
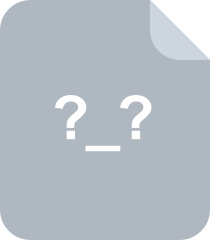
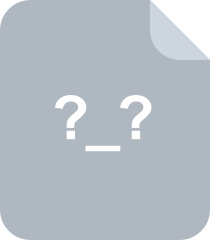
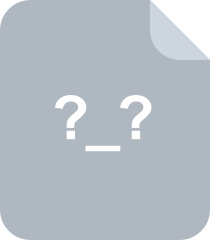
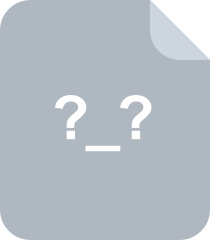
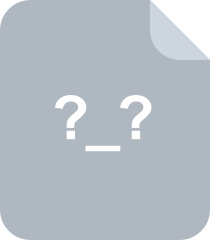
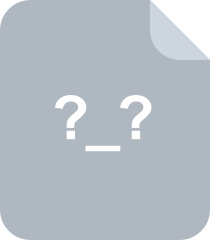
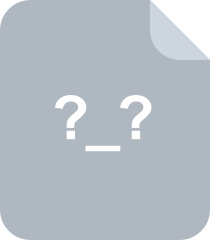
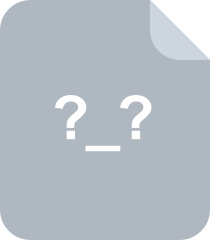
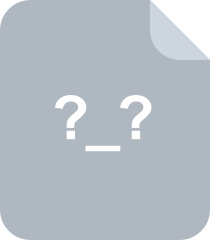
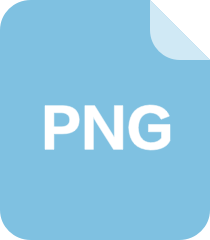
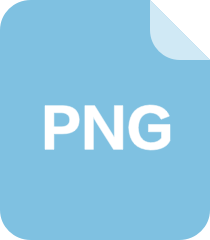
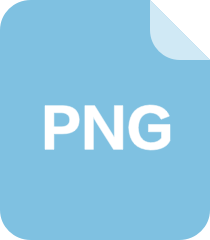
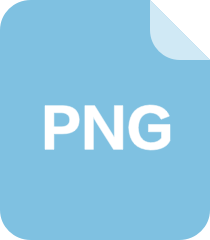
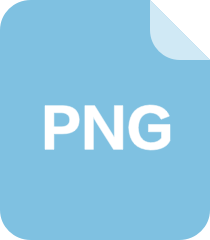
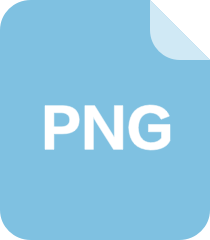
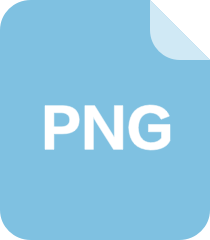
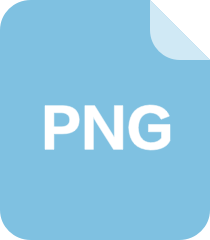
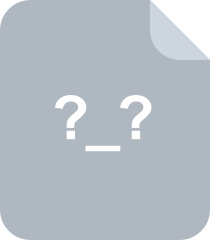
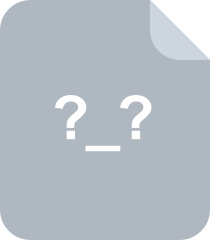
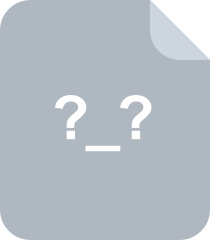
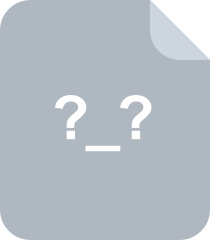
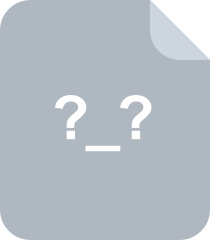
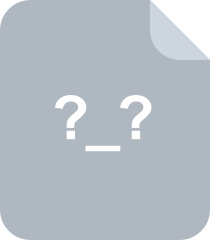
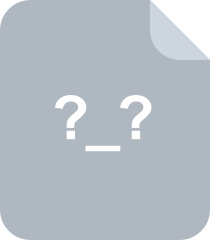
共 266 条
- 1
- 2
- 3
资源评论
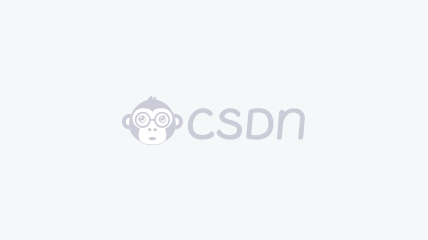

$encoding
- 粉丝: 98
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

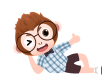
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


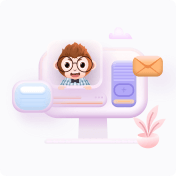
安全验证
文档复制为VIP权益,开通VIP直接复制
