/**
******************************************************************************
* @file stm32f4xx_rcc.c
* @author MCD Application Team
* @version V1.8.0
* @date 04-November-2016
* @brief This file provides firmware functions to manage the following
* functionalities of the Reset and clock control (RCC) peripheral:
* + Internal/external clocks, PLL, CSS and MCO configuration
* + System, AHB and APB busses clocks configuration
* + Peripheral clocks configuration
* + Interrupts and flags management
*
@verbatim
===============================================================================
##### RCC specific features #####
===============================================================================
[..]
After reset the device is running from Internal High Speed oscillator
(HSI 16MHz) with Flash 0 wait state, Flash prefetch buffer, D-Cache
and I-Cache are disabled, and all peripherals are off except internal
SRAM, Flash and JTAG.
(+) There is no prescaler on High speed (AHB) and Low speed (APB) busses;
all peripherals mapped on these busses are running at HSI speed.
(+) The clock for all peripherals is switched off, except the SRAM and FLASH.
(+) All GPIOs are in input floating state, except the JTAG pins which
are assigned to be used for debug purpose.
[..]
Once the device started from reset, the user application has to:
(+) Configure the clock source to be used to drive the System clock
(if the application needs higher frequency/performance)
(+) Configure the System clock frequency and Flash settings
(+) Configure the AHB and APB busses prescalers
(+) Enable the clock for the peripheral(s) to be used
(+) Configure the clock source(s) for peripherals which clocks are not
derived from the System clock (I2S, RTC, ADC, USB OTG FS/SDIO/RNG)
@endverbatim
******************************************************************************
* @attention
*
* <h2><center>© COPYRIGHT 2016 STMicroelectronics</center></h2>
*
* Licensed under MCD-ST Liberty SW License Agreement V2, (the "License");
* You may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.st.com/software_license_agreement_liberty_v2
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f4xx_rcc.h"
/** @addtogroup STM32F4xx_StdPeriph_Driver
* @{
*/
/** @defgroup RCC
* @brief RCC driver modules
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* ------------ RCC registers bit address in the alias region ----------- */
#define RCC_OFFSET (RCC_BASE - PERIPH_BASE)
/* --- CR Register ---*/
/* Alias word address of HSION bit */
#define CR_OFFSET (RCC_OFFSET + 0x00)
#define HSION_BitNumber 0x00
#define CR_HSION_BB (PERIPH_BB_BASE + (CR_OFFSET * 32) + (HSION_BitNumber * 4))
/* Alias word address of CSSON bit */
#define CSSON_BitNumber 0x13
#define CR_CSSON_BB (PERIPH_BB_BASE + (CR_OFFSET * 32) + (CSSON_BitNumber * 4))
/* Alias word address of PLLON bit */
#define PLLON_BitNumber 0x18
#define CR_PLLON_BB (PERIPH_BB_BASE + (CR_OFFSET * 32) + (PLLON_BitNumber * 4))
/* Alias word address of PLLI2SON bit */
#define PLLI2SON_BitNumber 0x1A
#define CR_PLLI2SON_BB (PERIPH_BB_BASE + (CR_OFFSET * 32) + (PLLI2SON_BitNumber * 4))
/* Alias word address of PLLSAION bit */
#define PLLSAION_BitNumber 0x1C
#define CR_PLLSAION_BB (PERIPH_BB_BASE + (CR_OFFSET * 32) + (PLLSAION_BitNumber * 4))
/* --- CFGR Register ---*/
/* Alias word address of I2SSRC bit */
#define CFGR_OFFSET (RCC_OFFSET + 0x08)
#define I2SSRC_BitNumber 0x17
#define CFGR_I2SSRC_BB (PERIPH_BB_BASE + (CFGR_OFFSET * 32) + (I2SSRC_BitNumber * 4))
/* --- BDCR Register ---*/
/* Alias word address of RTCEN bit */
#define BDCR_OFFSET (RCC_OFFSET + 0x70)
#define RTCEN_BitNumber 0x0F
#define BDCR_RTCEN_BB (PERIPH_BB_BASE + (BDCR_OFFSET * 32) + (RTCEN_BitNumber * 4))
/* Alias word address of BDRST bit */
#define BDRST_BitNumber 0x10
#define BDCR_BDRST_BB (PERIPH_BB_BASE + (BDCR_OFFSET * 32) + (BDRST_BitNumber * 4))
/* --- CSR Register ---*/
/* Alias word address of LSION bit */
#define CSR_OFFSET (RCC_OFFSET + 0x74)
#define LSION_BitNumber 0x00
#define CSR_LSION_BB (PERIPH_BB_BASE + (CSR_OFFSET * 32) + (LSION_BitNumber * 4))
/* --- DCKCFGR Register ---*/
/* Alias word address of TIMPRE bit */
#define DCKCFGR_OFFSET (RCC_OFFSET + 0x8C)
#define TIMPRE_BitNumber 0x18
#define DCKCFGR_TIMPRE_BB (PERIPH_BB_BASE + (DCKCFGR_OFFSET * 32) + (TIMPRE_BitNumber * 4))
/* --- CFGR Register ---*/
#define RCC_CFGR_OFFSET (RCC_OFFSET + 0x08)
#if defined(STM32F410xx)
/* Alias word address of MCO1EN bit */
#define RCC_MCO1EN_BIT_NUMBER 0x8
#define RCC_CFGR_MCO1EN_BB (PERIPH_BB_BASE + (RCC_CFGR_OFFSET * 32) + (RCC_MCO1EN_BIT_NUMBER * 4))
/* Alias word address of MCO2EN bit */
#define RCC_MCO2EN_BIT_NUMBER 0x9
#define RCC_CFGR_MCO2EN_BB (PERIPH_BB_BASE + (RCC_CFGR_OFFSET * 32) + (RCC_MCO2EN_BIT_NUMBER * 4))
#endif /* STM32F410xx */
/* ---------------------- RCC registers bit mask ------------------------ */
/* CFGR register bit mask */
#define CFGR_MCO2_RESET_MASK ((uint32_t)0x07FFFFFF)
#define CFGR_MCO1_RESET_MASK ((uint32_t)0xF89FFFFF)
/* RCC Flag Mask */
#define FLAG_MASK ((uint8_t)0x1F)
/* CR register byte 3 (Bits[23:16]) base address */
#define CR_BYTE3_ADDRESS ((uint32_t)0x40023802)
/* CIR register byte 2 (Bits[15:8]) base address */
#define CIR_BYTE2_ADDRESS ((uint32_t)(RCC_BASE + 0x0C + 0x01))
/* CIR register byte 3 (Bits[23:16]) base address */
#define CIR_BYTE3_ADDRESS ((uint32_t)(RCC_BASE + 0x0C + 0x02))
/* BDCR register base address */
#define BDCR_ADDRESS (PERIPH_BASE + BDCR_OFFSET)
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
static __I uint8_t APBAHBPrescTable[16] = {0, 0, 0, 0, 1, 2, 3, 4, 1, 2, 3, 4, 6, 7, 8, 9};
/* Private function prototypes -----------------------------------------------*/
/* Private functions ---------------------------------------------------------*/
/** @defgroup RCC_Private_Functions
* @{
*/
/** @defgroup RCC_Group1 Internal and external clocks, PLL, CSS and MCO configuration functions
* @brief Internal and external clocks, PLL, CSS and MCO configuration functions
*
@verbatim
===================================================================================
##### Internal and external clocks, PLL, CSS and MCO configuration functions #####
===================================================================================
[..]
This section provide functions allowing to configure the internal/exter
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
内容概括:STM32F407智能遥控小车完整代码 材料内容:使用STM32F407VGT6芯片 2.4G无线模块使用两块NRF24L01 电脑上使用串口转NRF24L01 电机驱动使用TB6612FNG 电源模块是充电宝加上DC电源升压模块 能学习到什么:SPI通信 2.4G无线通信使用 红外模块使用 电机驱动的使用 适合人群:想要做关于单片机方面的毕业设计 对遥控小车有兴趣的人群
资源详情
资源评论
资源推荐
收起资源包目录

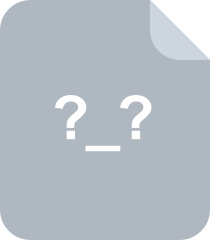
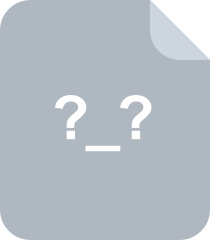
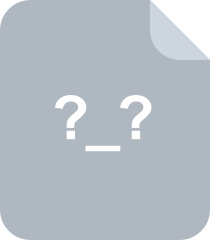
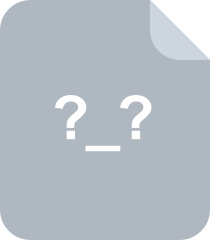
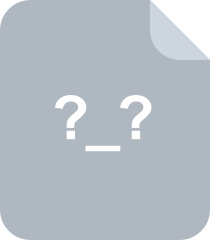
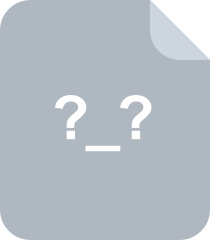
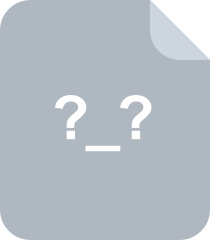
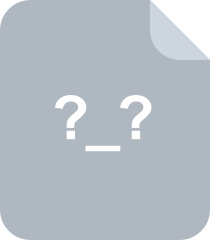
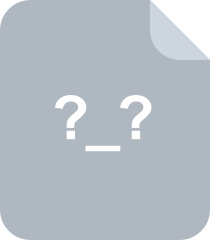
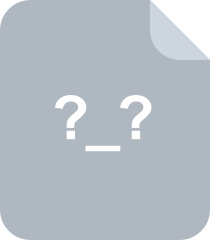
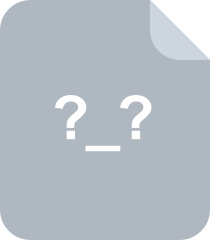
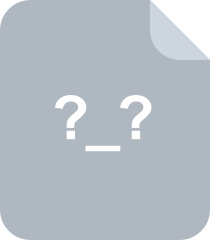
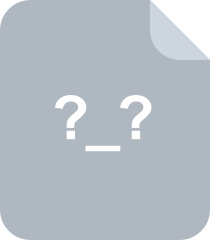
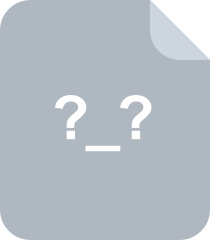
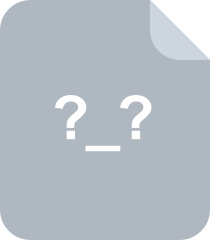
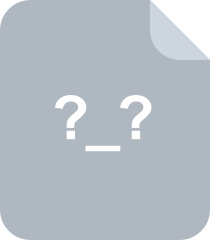
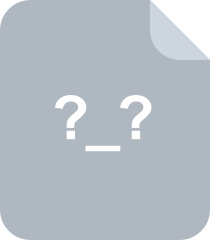
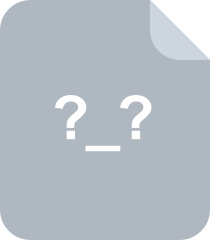
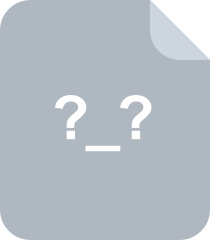
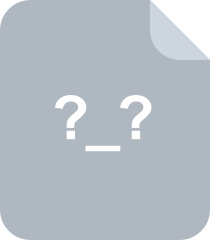
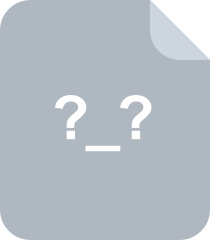
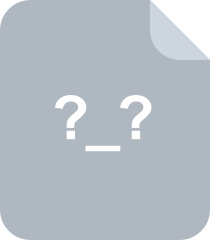
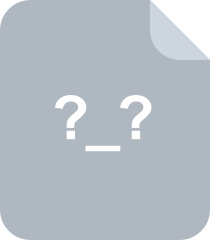
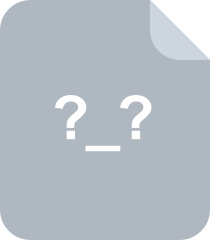
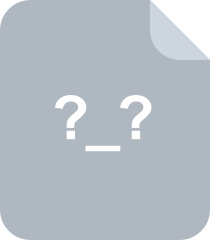
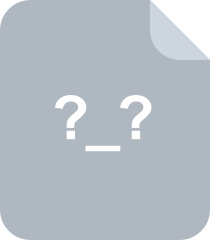
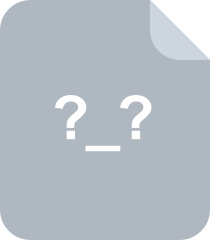
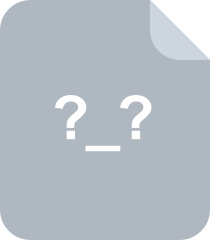
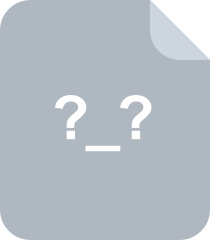
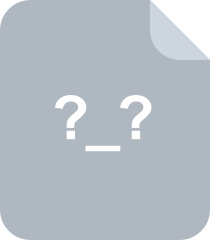
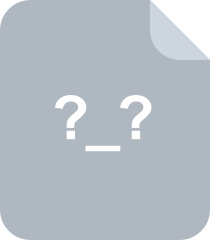
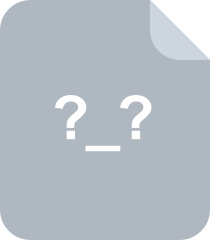
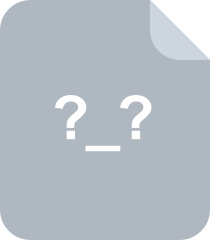
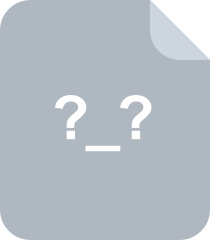
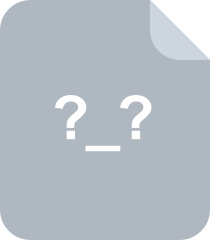
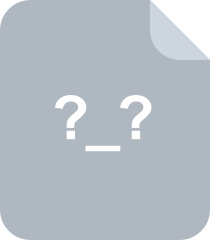
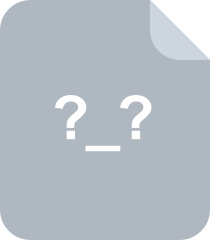
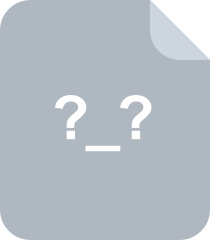
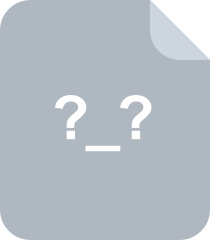
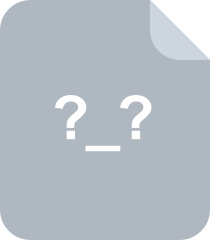
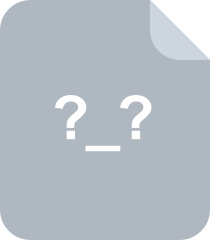
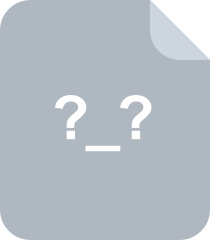
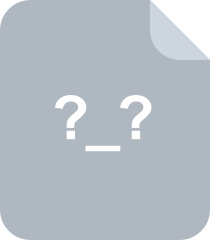
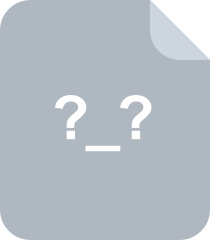
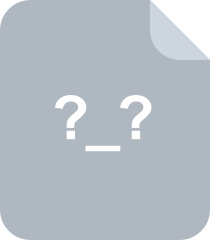
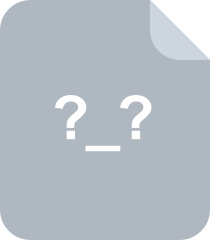
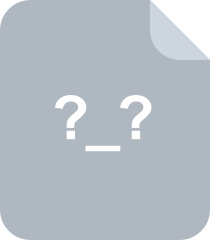
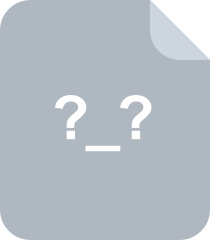
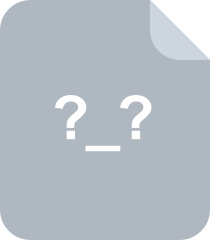
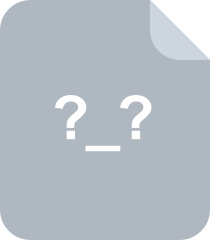
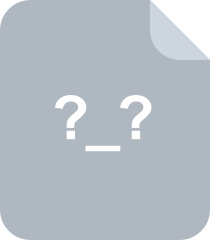
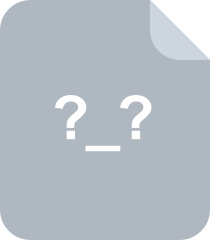
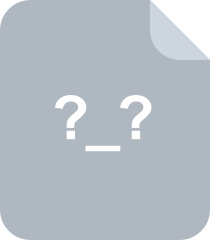
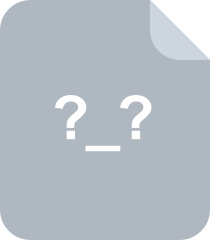
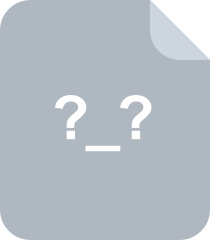
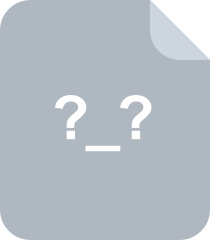
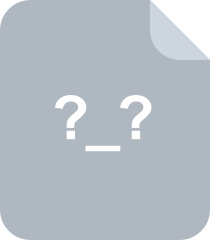
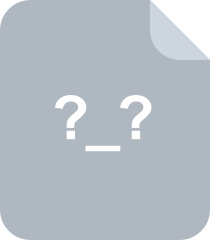
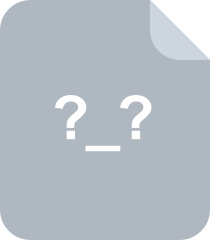
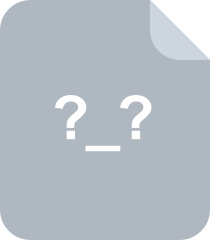
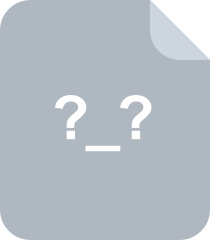
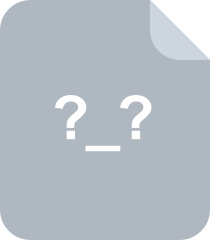
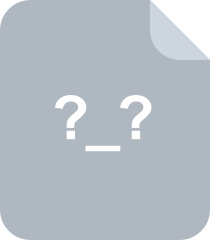
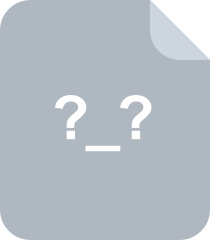
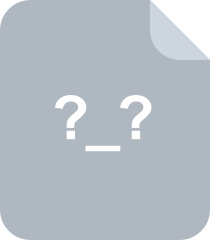
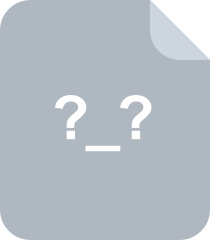
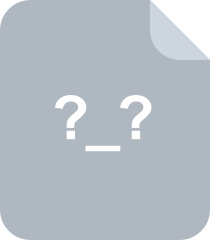
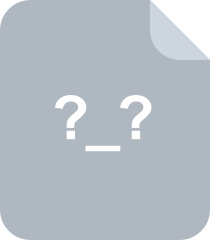
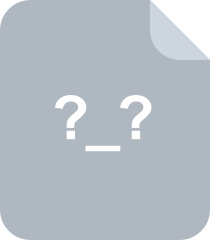
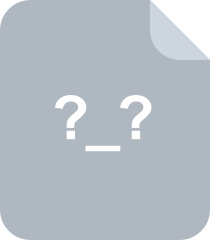
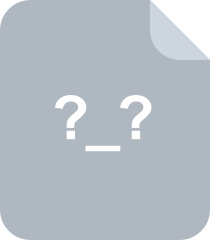
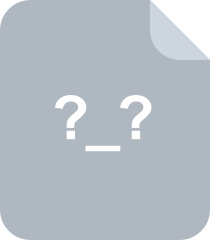
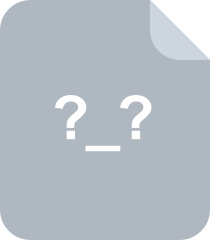
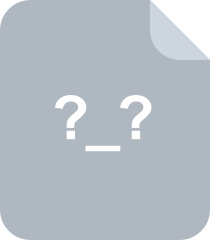
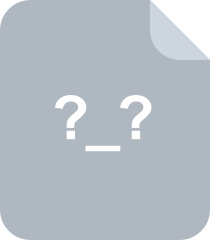
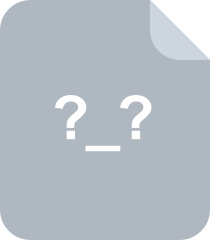
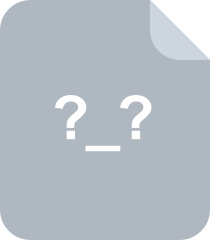
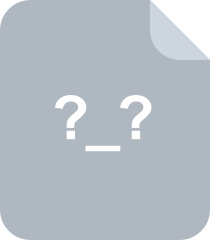
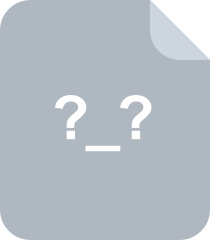
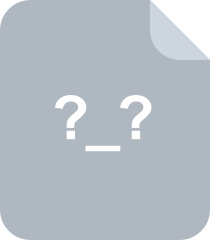
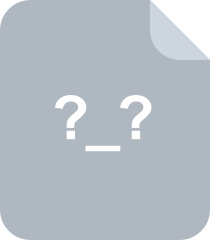
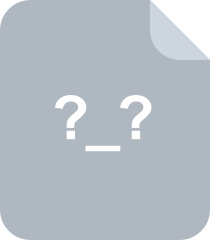
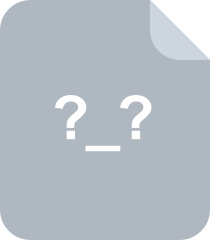
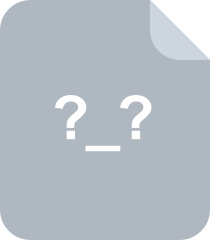
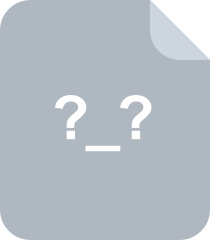
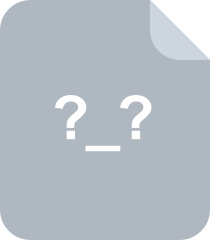
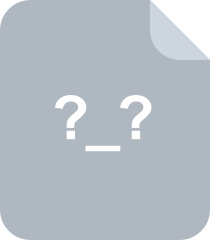
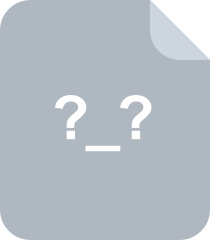
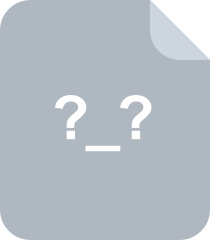
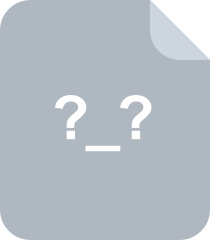
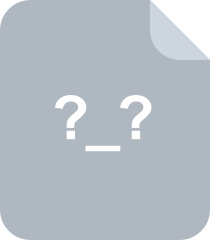
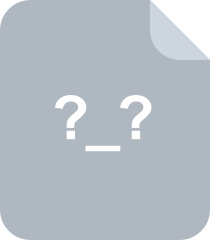
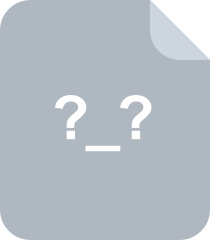
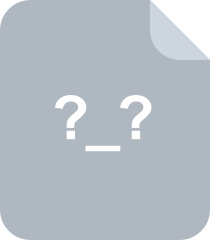
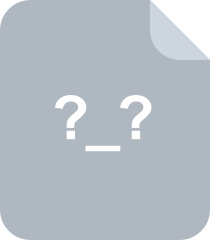
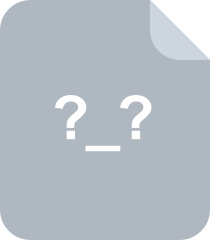
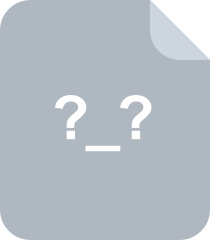
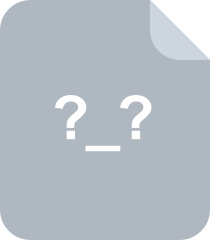
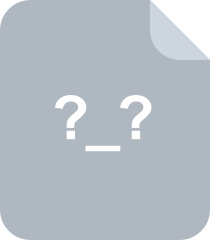
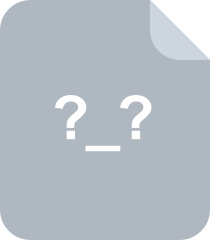
共 257 条
- 1
- 2
- 3
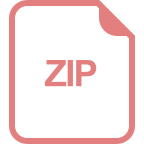
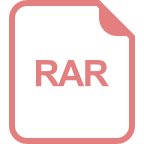
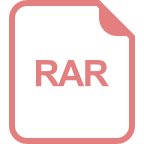
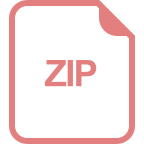
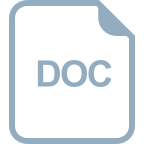
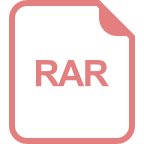
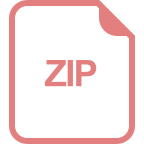
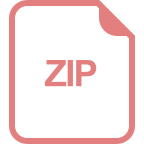
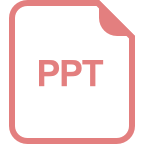
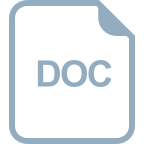
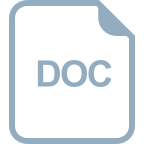
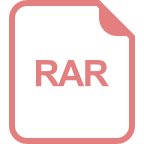
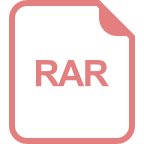
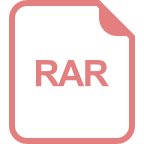
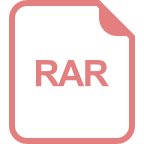
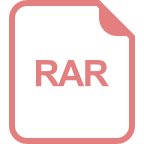
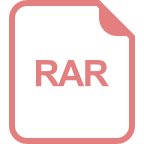
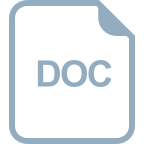

我们是冠军
- 粉丝: 2
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

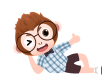
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


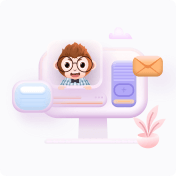
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0