C51入门小项目-双向路口交通信号灯(蜂鸣器提醒行人+数码管倒计时)博客对应源码
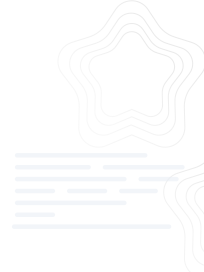

本项目是针对C51单片机初学者设计的一个实用案例——双向路口交通信号灯系统。这个系统结合了蜂鸣器提醒功能和数码管倒计时显示,旨在帮助学习者理解C51单片机的基本原理及其在实际应用中的操作。 C51是Atmel公司开发的一种针对8051系列微控制器的高级语言,它扩展了标准的ANSI C,添加了一些针对微控制器的特定功能。在这个项目中,我们将使用C51编写控制交通信号灯的程序,包括设置红绿灯交替的时间周期,以及在特定时间通过蜂鸣器发出声音提醒行人,并在数码管上显示剩余时间。 我们需要了解8051单片机的基本结构和工作原理。8051单片机具有中央处理器(CPU)、内部RAM、ROM、定时/计数器、并行I/O口等核心部件。在这个项目中,我们将利用I/O口来控制交通灯的状态,定时/计数器则用于实现红绿灯的自动切换。 接着,交通信号灯的控制策略通常包括红绿黄三色灯的交替显示。在C51程序中,我们将定义相应的变量来存储当前灯的状态,并使用循环和条件判断语句来实现不同颜色灯的切换。例如,我们可以设定一个计数器变量,每过一定时间(如30秒)就切换到下一个灯状态。 蜂鸣器提醒功能的实现,需要通过控制单片机的I/O口来驱动蜂鸣器。当红灯亮起,蜂鸣器会持续发出声音,提醒行人不要过马路。这可以通过在程序中添加对蜂鸣器控制端口的电平翻转来实现,比如使用P0或P2口的某个引脚输出高电平使蜂鸣器发声,低电平时关闭声音。 数码管倒计时显示则需要用到数码管驱动技术。数码管可以是7段数码管或共阴极或共阳极的8段数码管,根据实际情况选择合适的驱动方式。在C51程序中,我们需要定义相应的数码管段码,然后通过位操作来驱动数码管显示倒计时的数字。倒计时的计算同样基于定时/计数器,每隔一定时间更新数码管上的数值。 至于“微信图片_20200904115052.jpg”和“仿真截图.png”,这些可能是电路图或系统运行的仿真画面,它们可以帮助我们直观地理解电路布局和系统工作流程。而“仿真”文件可能包含了电路的仿真配置,这对于验证和调试程序至关重要。“程序”文件应该是C51的源代码,里面包含了实现上述功能的具体指令。 这个项目涵盖了C51单片机编程的基础知识,包括I/O口控制、定时器/计数器应用、蜂鸣器驱动和数码管显示等。通过这个项目,学习者不仅可以加深对C51编程的理解,还能提升动手实践能力,为后续更复杂的单片机项目打下坚实基础。
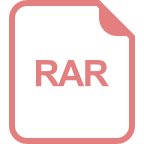
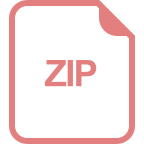
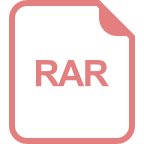
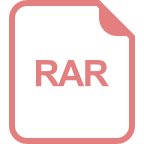
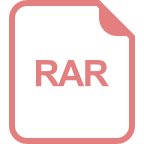
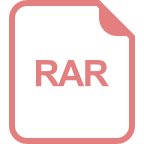
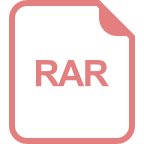
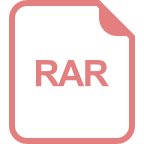
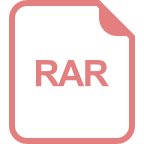
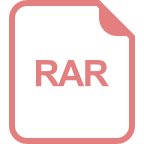
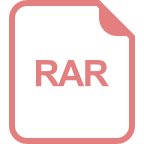
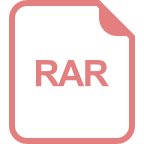
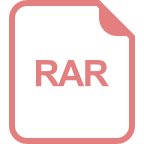
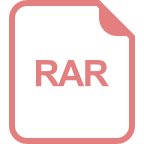
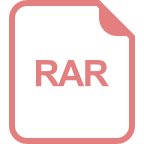
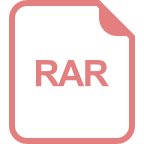
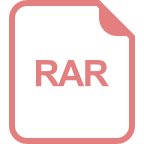
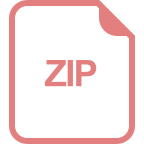
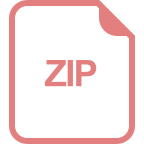
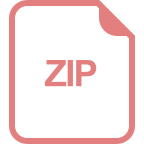


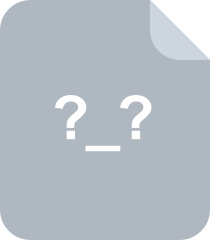
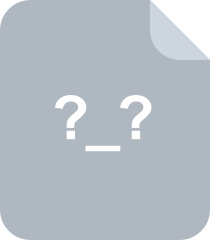
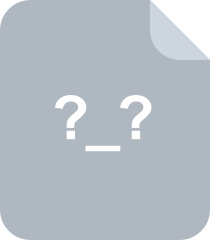
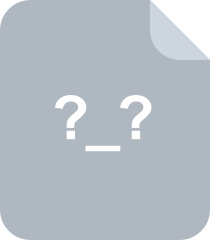
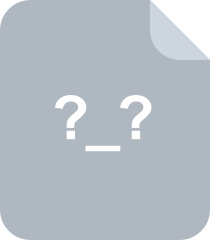
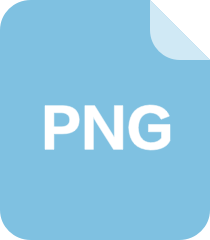


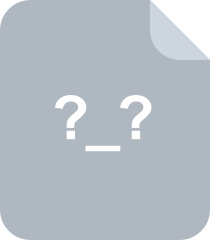
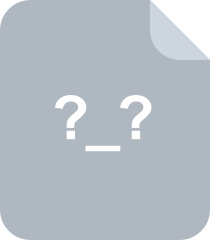
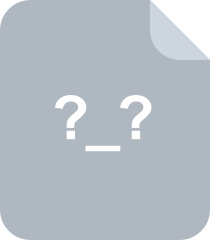
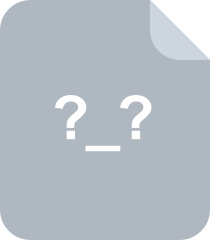
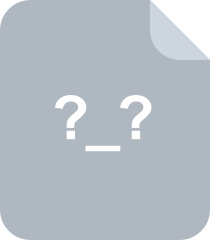
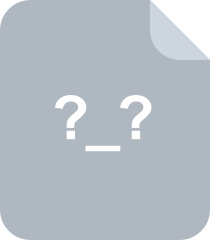
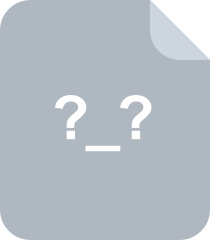
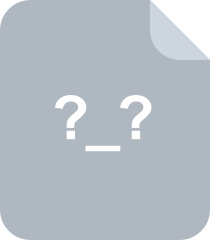
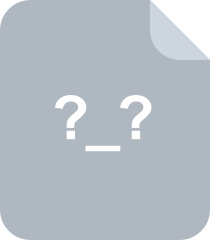
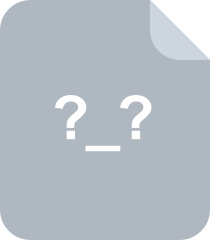
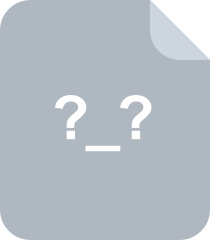
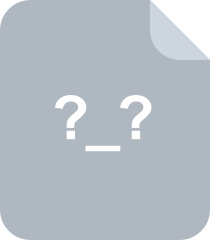
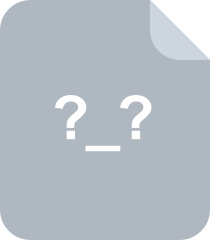
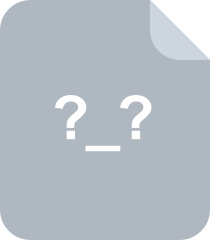
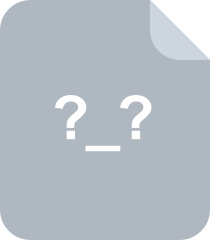
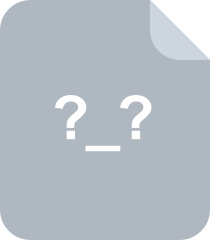

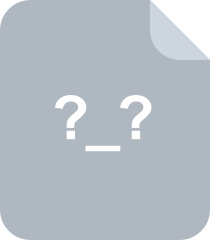
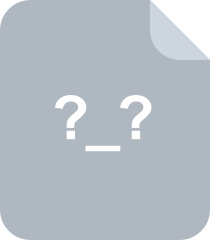
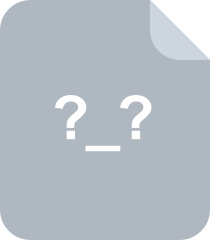
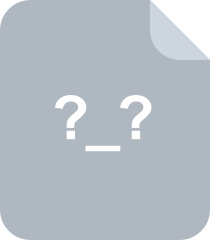
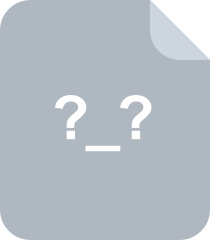
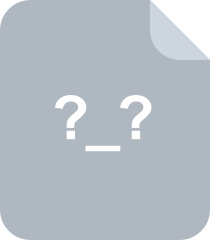
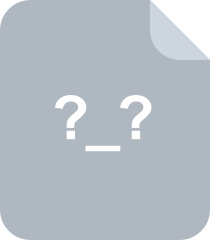
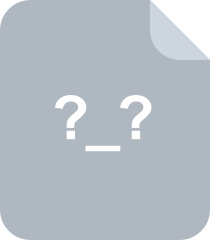
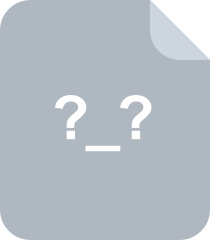
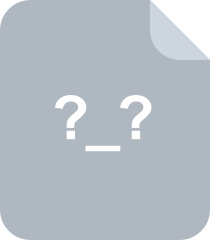
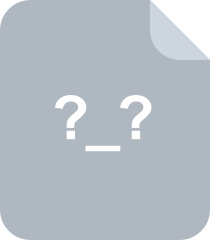
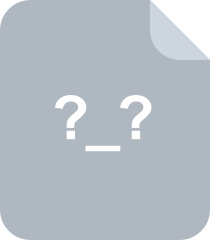
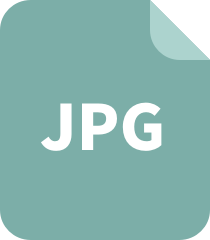
- 1
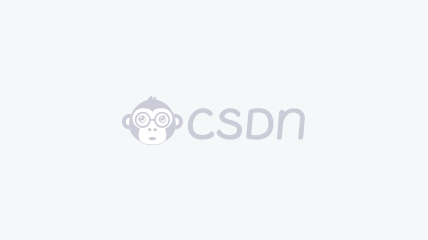

- 粉丝: 517
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

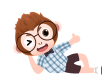
最新资源
- 基于springboot+vue的实践性教学系统源码(java毕业设计完整源码+LW).zip
- 基于SpringBoot的食物营养分析与推荐网站的设计与实现源码(java毕业设计完整源码+LW).zip
- AvalonDock-master WPF
- 基于BS架构社区快递上门服务系统设计与实现源码(java毕业设计完整源码).zip
- (177631206)大麦抢票+源代码+辅助工具+详细文档教程
- 基于javaweb宿舍管理系统源码(java毕业设计完整源码).zip
- 英飞凌79AMOSFET
- 基于javaweb的在线购物平台源码(java毕业设计完整源码).zip
- ETOPO2022一秒钟冰面高程数据集.zip
- 基于全阶滑模观测器的IPMSM无位置传感器控制策略研究
- 基于JavaWeb的宠物救助及领养平台的设计与实现源码(java毕业设计完整源码+LW).zip
- 设计模式 DesignPatterns C#源码
- 基于Java技术的救灾物资调动系统源码(java毕业设计完整源码).zip
- 机械设计无纺布检测机(sw可编辑+工程图+bom)全套设计资料100%好用.zip
- MATLAB用yalmip+cplex解决电动汽车有序充放电问题,目标函数为总负荷峰谷差最小,代码可运行且有注释
- 基于java的城市公交查询系统源码(java毕业设计完整源码+LW).zip

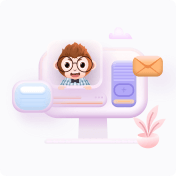
