"""
This module contains definitions to build schemas which `pydantic_core` can
validate and serialize.
"""
from __future__ import annotations as _annotations
import sys
import warnings
from collections.abc import Mapping
from datetime import date, datetime, time, timedelta
from decimal import Decimal
from typing import TYPE_CHECKING, Any, Callable, Dict, Hashable, List, Set, Tuple, Type, Union
from typing_extensions import deprecated
if sys.version_info < (3, 12):
from typing_extensions import TypedDict
else:
from typing import TypedDict
if sys.version_info < (3, 11):
from typing_extensions import Protocol, Required, TypeAlias
else:
from typing import Protocol, Required, TypeAlias
if sys.version_info < (3, 9):
from typing_extensions import Literal
else:
from typing import Literal
if TYPE_CHECKING:
from pydantic_core import PydanticUndefined
else:
# The initial build of pydantic_core requires PydanticUndefined to generate
# the core schema; so we need to conditionally skip it. mypy doesn't like
# this at all, hence the TYPE_CHECKING branch above.
try:
from pydantic_core import PydanticUndefined
except ImportError:
PydanticUndefined = object()
ExtraBehavior = Literal['allow', 'forbid', 'ignore']
class CoreConfig(TypedDict, total=False):
"""
Base class for schema configuration options.
Attributes:
title: The name of the configuration.
strict: Whether the configuration should strictly adhere to specified rules.
extra_fields_behavior: The behavior for handling extra fields.
typed_dict_total: Whether the TypedDict should be considered total. Default is `True`.
from_attributes: Whether to use attributes for models, dataclasses, and tagged union keys.
loc_by_alias: Whether to use the used alias (or first alias for "field required" errors) instead of
`field_names` to construct error `loc`s. Default is `True`.
revalidate_instances: Whether instances of models and dataclasses should re-validate. Default is 'never'.
validate_default: Whether to validate default values during validation. Default is `False`.
populate_by_name: Whether an aliased field may be populated by its name as given by the model attribute,
as well as the alias. (Replaces 'allow_population_by_field_name' in Pydantic v1.) Default is `False`.
str_max_length: The maximum length for string fields.
str_min_length: The minimum length for string fields.
str_strip_whitespace: Whether to strip whitespace from string fields.
str_to_lower: Whether to convert string fields to lowercase.
str_to_upper: Whether to convert string fields to uppercase.
allow_inf_nan: Whether to allow infinity and NaN values for float fields. Default is `True`.
ser_json_timedelta: The serialization option for `timedelta` values. Default is 'iso8601'.
ser_json_bytes: The serialization option for `bytes` values. Default is 'utf8'.
ser_json_inf_nan: The serialization option for infinity and NaN values
in float fields. Default is 'null'.
hide_input_in_errors: Whether to hide input data from `ValidationError` representation.
validation_error_cause: Whether to add user-python excs to the __cause__ of a ValidationError.
Requires exceptiongroup backport pre Python 3.11.
coerce_numbers_to_str: Whether to enable coercion of any `Number` type to `str` (not applicable in `strict` mode).
regex_engine: The regex engine to use for regex pattern validation. Default is 'rust-regex'. See `StringSchema`.
cache_strings: Whether to cache strings. Default is `True`, `True` or `'all'` is required to cache strings
during general validation since validators don't know if they're in a key or a value.
"""
title: str
strict: bool
# settings related to typed dicts, model fields, dataclass fields
extra_fields_behavior: ExtraBehavior
typed_dict_total: bool # default: True
# used for models, dataclasses, and tagged union keys
from_attributes: bool
# whether to use the used alias (or first alias for "field required" errors) instead of field_names
# to construct error `loc`s, default True
loc_by_alias: bool
# whether instances of models and dataclasses (including subclass instances) should re-validate, default 'never'
revalidate_instances: Literal['always', 'never', 'subclass-instances']
# whether to validate default values during validation, default False
validate_default: bool
# used on typed-dicts and arguments
populate_by_name: bool # replaces `allow_population_by_field_name` in pydantic v1
# fields related to string fields only
str_max_length: int
str_min_length: int
str_strip_whitespace: bool
str_to_lower: bool
str_to_upper: bool
# fields related to float fields only
allow_inf_nan: bool # default: True
# the config options are used to customise serialization to JSON
ser_json_timedelta: Literal['iso8601', 'float'] # default: 'iso8601'
ser_json_bytes: Literal['utf8', 'base64', 'hex'] # default: 'utf8'
ser_json_inf_nan: Literal['null', 'constants'] # default: 'null'
# used to hide input data from ValidationError repr
hide_input_in_errors: bool
validation_error_cause: bool # default: False
coerce_numbers_to_str: bool # default: False
regex_engine: Literal['rust-regex', 'python-re'] # default: 'rust-regex'
cache_strings: Union[bool, Literal['all', 'keys', 'none']] # default: 'True'
IncExCall: TypeAlias = 'set[int | str] | dict[int | str, IncExCall] | None'
class SerializationInfo(Protocol):
@property
def include(self) -> IncExCall: ...
@property
def exclude(self) -> IncExCall: ...
@property
def context(self) -> Any | None:
"""Current serialization context."""
@property
def mode(self) -> str: ...
@property
def by_alias(self) -> bool: ...
@property
def exclude_unset(self) -> bool: ...
@property
def exclude_defaults(self) -> bool: ...
@property
def exclude_none(self) -> bool: ...
@property
def serialize_as_any(self) -> bool: ...
def round_trip(self) -> bool: ...
def mode_is_json(self) -> bool: ...
def __str__(self) -> str: ...
def __repr__(self) -> str: ...
class FieldSerializationInfo(SerializationInfo, Protocol):
@property
def field_name(self) -> str: ...
class ValidationInfo(Protocol):
"""
Argument passed to validation functions.
"""
@property
def context(self) -> Any | None:
"""Current validation context."""
...
@property
def config(self) -> CoreConfig | None:
"""The CoreConfig that applies to this validation."""
...
@property
def mode(self) -> Literal['python', 'json']:
"""The type of input data we are currently validating"""
...
@property
def data(self) -> Dict[str, Any]:
"""The data being validated for this model."""
...
@property
def field_name(self) -> str | None:
"""
The name of the current field being validated if this validator is
attached to a model field.
"""
...
ExpectedSerializationTypes = Literal[
'none',
'int',
'bool',
'float',
'str',
'bytes',
'bytearray',
'list',
'tuple',
'set',
'frozenset',
'generator',
'dict',
'datetime',
'date',
'time',
'timedelta',
'url',
'multi-host-url',
'json',
'uuid',
]
class SimpleSerSchema(TypedDict, total=False):
type: Required[ExpectedSerializationTypes]
def simple_ser_schema(type: ExpectedSerializationTypes) -> SimpleSerSchema:
"""
Returns a schema for serialization with a custom type.
Args:
type: The type to use for serialization
"""
retur
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目是一款基于Python开发的野生鸟类数据存储与识别系统源码,包含3634个文件,涵盖1210个JavaScript文件、380个TypeScript文件、362个Markdown文件、309个JSON文件、273个地图文件、242个Python字节码文件、232个Python源代码文件、89个MJS文件、49个YAML文件、31个JPG图片文件。系统界面采用HTML和CSS构建,后端逻辑以Python实现,并涉及Shell脚本调用。该系统旨在为鸟类研究者提供高效的数据存储和识别解决方案。
资源推荐
资源详情
资源评论
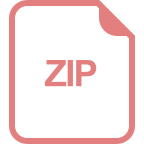
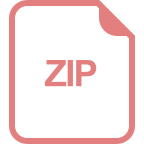
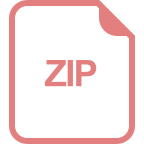
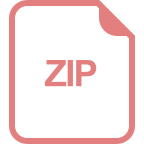
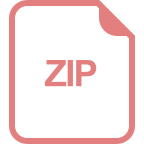
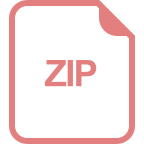
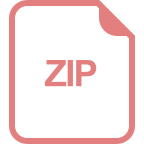
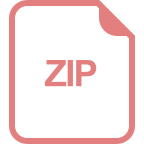
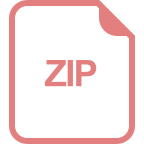
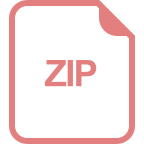
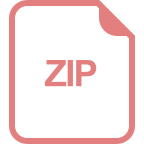
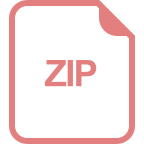
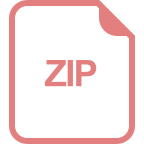
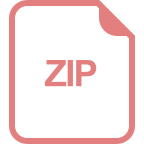
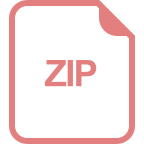
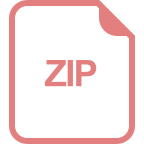
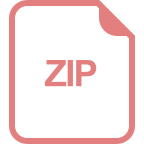
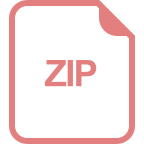
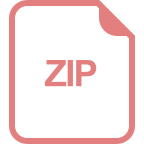
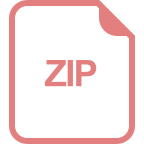
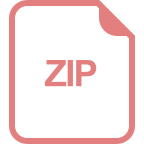
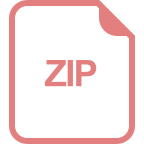
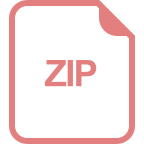
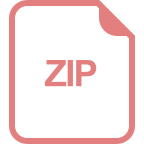
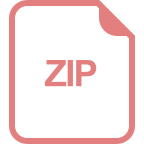
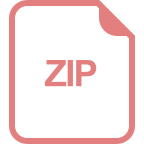
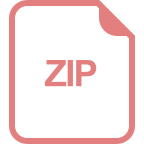
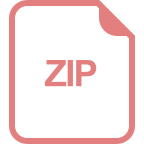
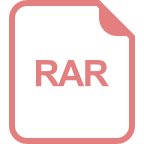
收起资源包目录

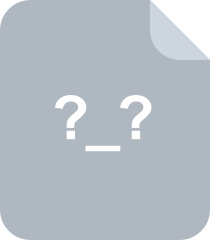
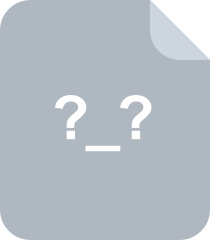
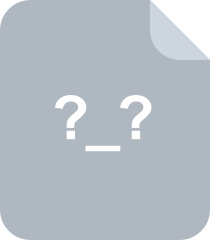
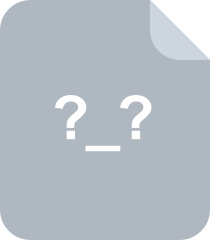
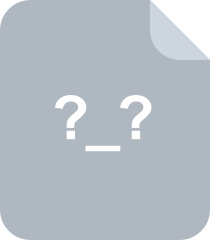
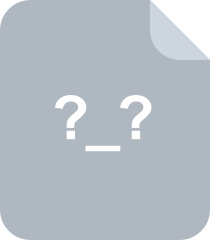
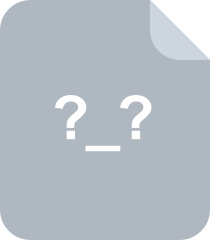
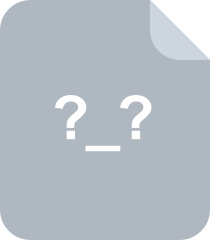
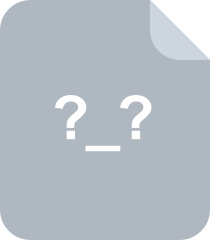
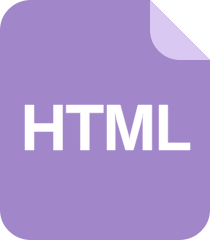
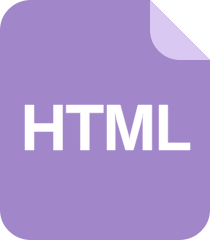
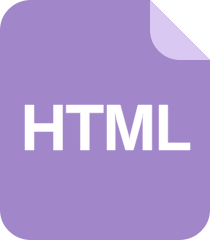
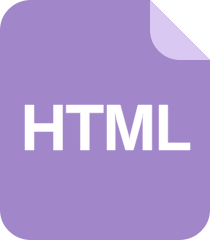
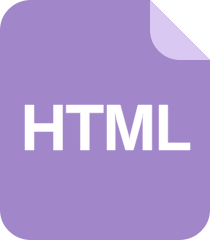
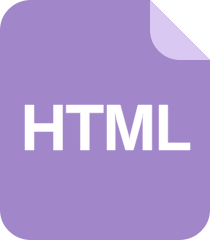
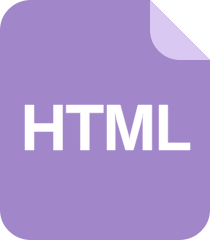
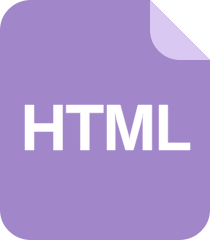
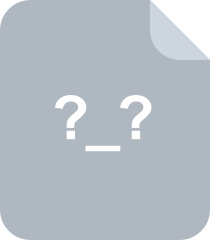
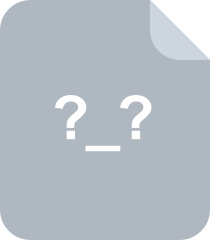
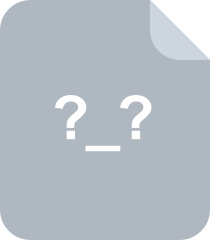
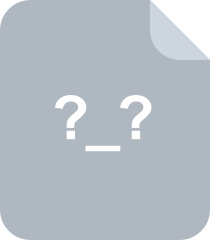
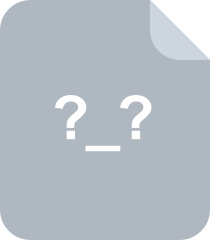
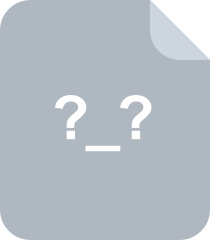
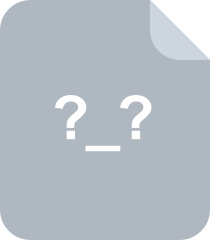
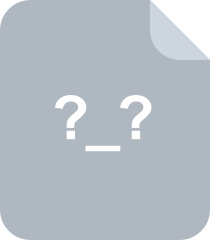
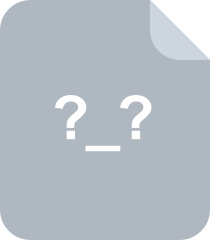
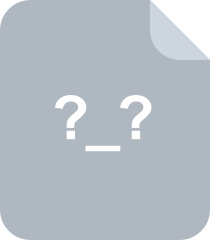
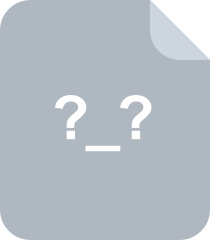
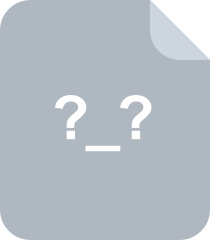
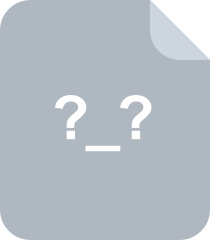
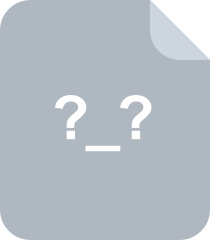
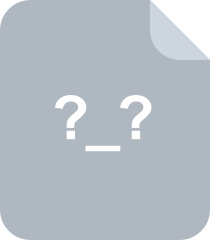
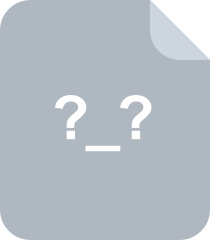
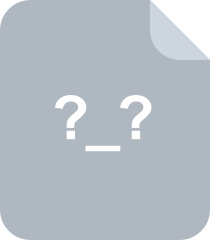
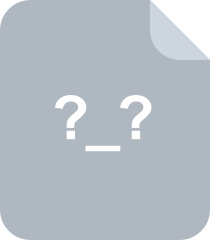
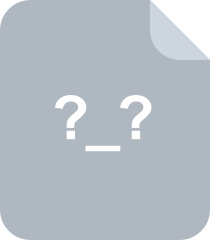
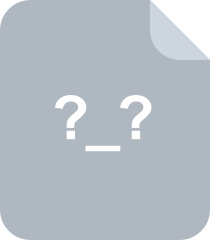
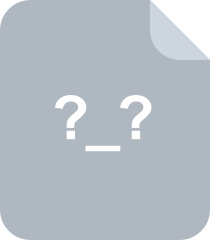
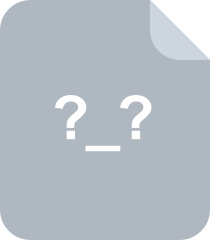
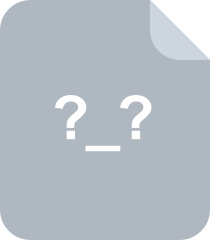
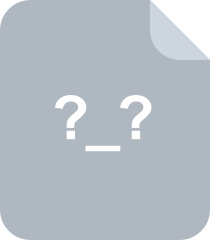
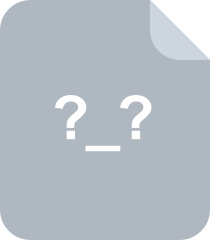
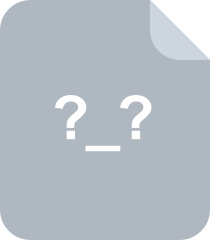
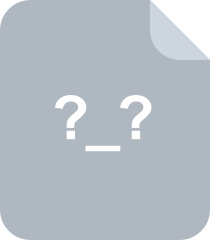
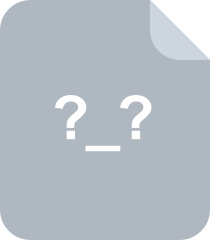
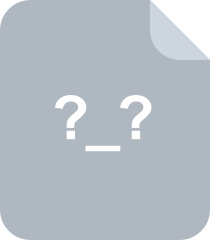
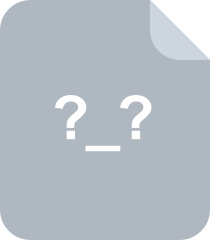
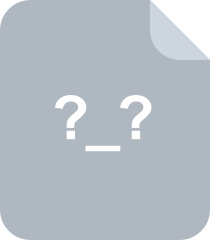
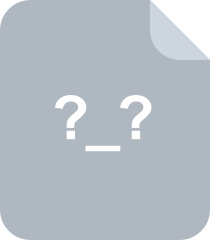
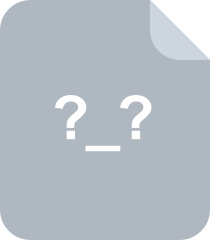
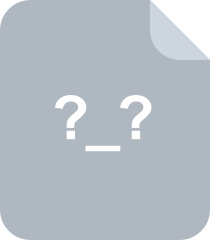
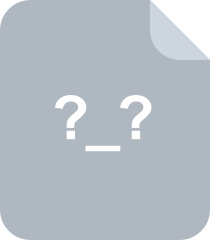
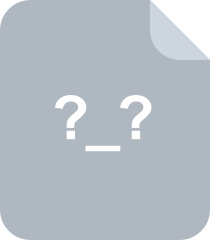
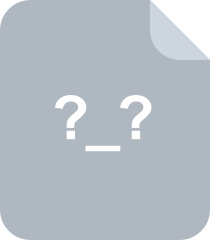
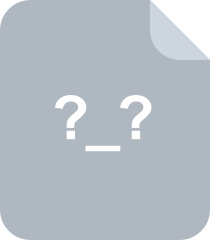
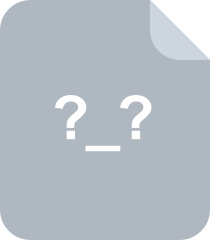
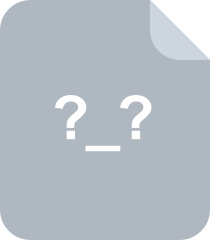
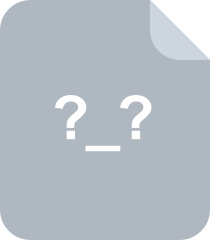
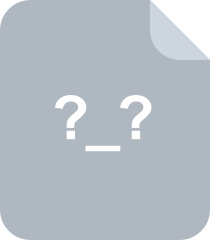
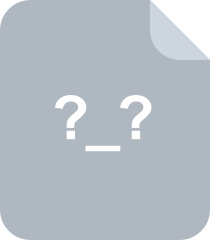
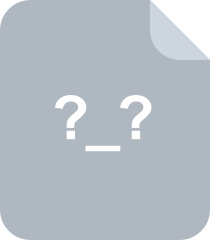
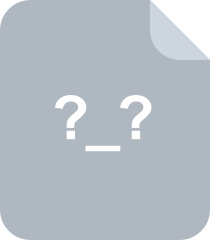
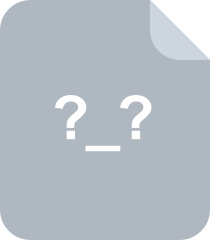
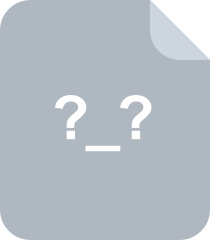
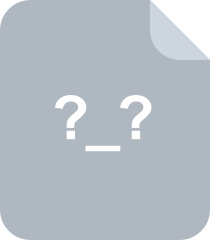
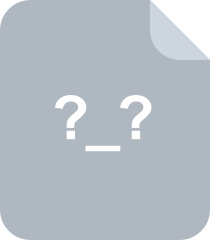
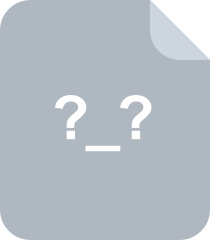
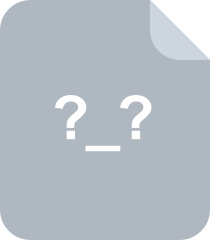
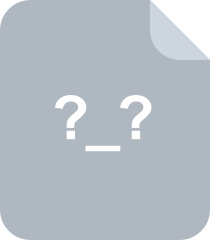
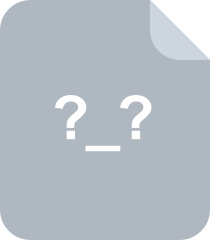
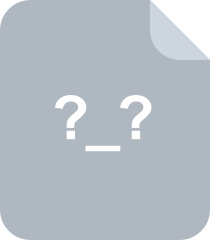
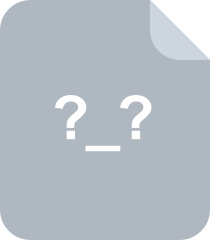
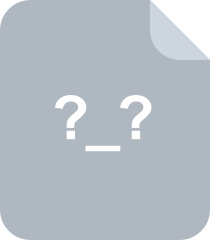
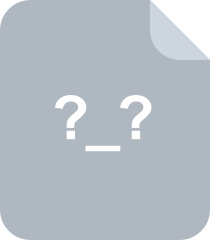
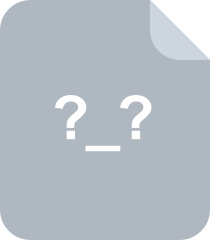
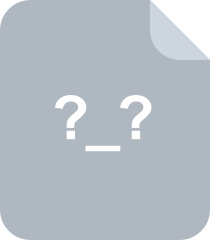
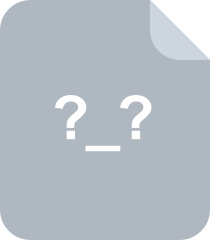
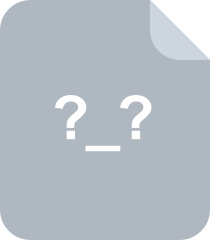
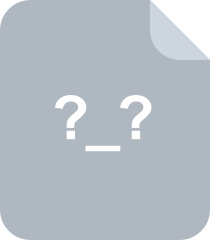
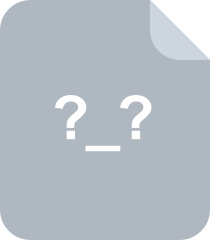
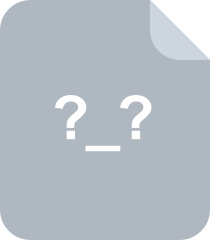
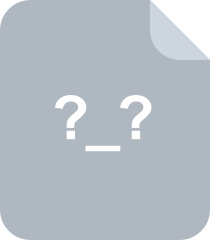
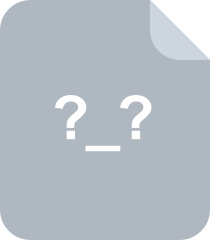
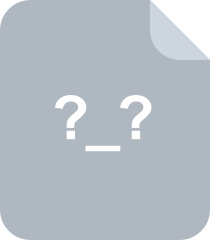
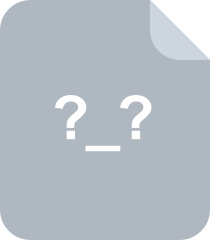
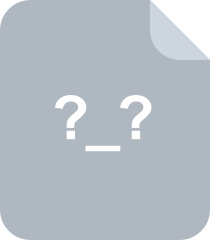
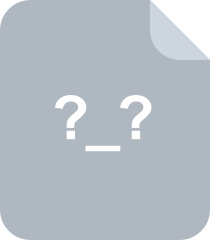
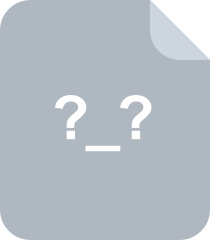
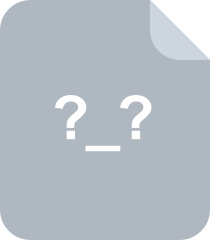
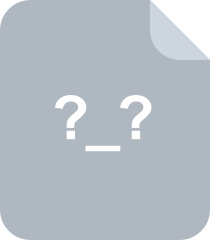
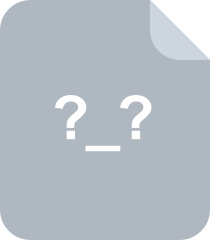
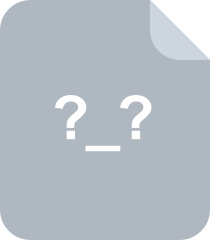
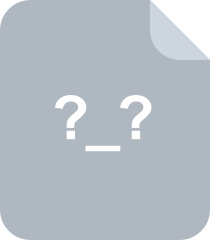
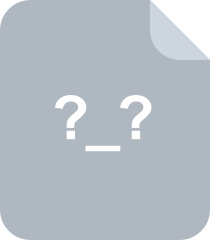
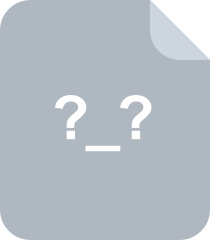
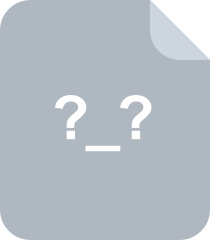
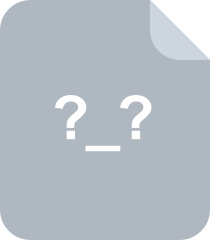
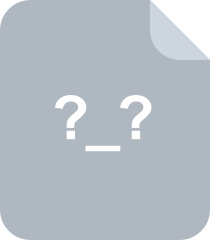
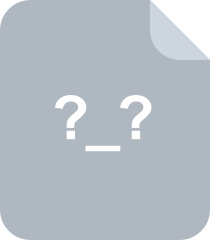
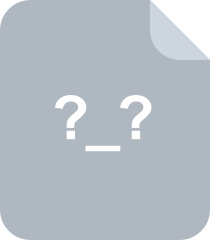
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
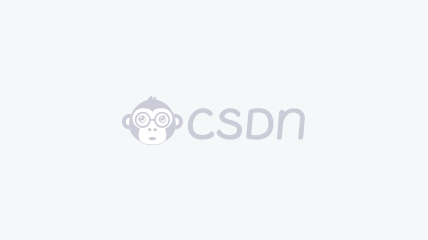

沐知全栈开发
- 粉丝: 5752
- 资源: 5215
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

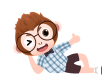
最新资源
- 基于Kotlin语言的Android开发工具类集合源码
- 零延迟 DirectX 11 扩展实用程序.zip
- 基于Java的语音识别系统设计源码
- 基于Java和HTML的yang_home766个人主页设计源码
- 基于Java与前端技术的全国实时疫情信息网站设计源码
- 基于鸿蒙系统的HarmonyHttpClient设计源码,纯Java实现类似OkHttp的HttpNet框架与优雅的Retrofit注解解析
- 基于HTML和JavaScript的廖振宇图书馆前端设计源码
- 基于Java的Android开发工具集合源码
- 通过 DirectX 12 Hook (kiero) 实现通用 ImGui.zip
- 基于Java开发的YY网盘个人网盘设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


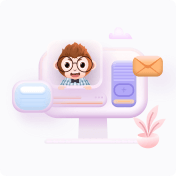
安全验证
文档复制为VIP权益,开通VIP直接复制
