#define _CRT_SECURE_NO_WARNINGS 1
//牛客网C语言入门刷题
//https://www.nowcoder.com/ta/beginner-programmers
//#include <stdio.h>
//bc001
//开始练习!2021.12.07
//int main()
//{
// printf("Practice makes perfect!\n");
// return 0;
//}
//#include <stdio.h>
////bc002
//int main()
//{
// printf("v v\n");
// printf(" v v \n");
// printf(" v \n");
// return 0;
//}
//#include <stdio.h>
////bc003
//int main()
//{
//
// printf("The size of short is %d bytes.\n", sizeof(short));
// printf("The size of int is %d bytes.\n", sizeof(int));
// printf("The size of long is %d bytes.\n", sizeof(long));
// printf("The size of long long is %d bytes.\n", sizeof(long long));
//
// return 0;
//}
//#include<stdio.h>
////新bc03
//int main()
//{
// int a;
// scanf("%d", &a);
// printf("%d", a);
// return 0;
//}
//#include<stdio.h>
////新bc04
//int main()
//{
// float a;
// scanf("%f", &a);
// printf("%.3f", a);
// return 0;
//}
//
//#include <stdio.h>
////bc006-小飞机
//int main()
//{
// printf(" ** \n");
// printf(" ** \n");
// printf("************\n");
// printf("************\n");
// printf(" * * \n");
// printf(" * * \n");
// return 0;
//}
//#include<stdio.h>
////新bc07
//int main()
//{
// char a;
// scanf("%c", &a);
// printf("%c%c%c\n", a, a, a);
// printf("%c%c%c\n", a, a, a);
// printf("%c%c%c\n", a, a, a);
// return 0;
//}
//#include <stdio.h>
////bc007
//int main()
//{
// int a = 1234;
// printf("%#o %#X", a, a);
// return 0;
//}
//#include<stdio.h>
////新bc08
//int main()
//{
// char a;
// scanf("%c", &a);
//
// printf(" ");
// printf("%c\n", a);
// printf(" ");
// printf("%c%c%c\n", a, a, a);
// printf("%c%c%c%c%c\n", a, a, a, a, a);
// printf(" ");
// printf("%c%c%c\n", a, a, a);
// printf(" ");
// printf("%c\n", a);
// return 0;
//}
//#include <stdio.h>
////bc-008
//int main()
//{
// int a = 0xABCDEF;
// printf("%15d", a);
// return 0;
//}
//#include<stdio.h>
//
//int main()
//{
// float a = 0;
// scanf("%f", &a);
// //输入四舍五入后的整数部分
// printf("%.0f", a);
//
// return 0;
//}
//#include <stdio.h>
////bc09
//int main()
//{
//
// int a = printf("Hello world!");
// printf("\n");
// printf("%d", a);
//
// return 0;
//}
//#include <stdio.h>
////bc10
//int main()
//{
// int a, b, c;
// scanf("%d %d %d", &a, &b, &c);
// printf("score1=%d,score2=%d,score3=%d", a, b, c);
// return 0;
//}
//#include <stdio.h>
////bc11
//int main()
//{
// int a;
// float b, c, d;
// scanf("%d;%f,%f,%f", &a, &b, &c, &d);
//
// printf("The each subject score of No. %d is %.2f, %.2f, %.2f.", a, b, c, d);
// return 0;
//}//double类型不会四舍五入,但是float可以
//#include <stdio.h>
//bc12-打印圣诞树
//int main()
//{
// char n;
// scanf("%c", &n);//输入字符
//
// int m = 4;//空格个数,第一行4个
//
// for (int i = 1; i <= 5; i++)
// {
// int j = 0;
// for ( j = 0; j < m; j++)
// {
// printf(" ");
// }
// m--;//下一行少一个
// for (int k = 0; k < i; k++)
// {
// printf("%c ", n);
// }
// printf("\n");
//
// }
// return 0;
//}
//#include <stdio.h>
////bc13-ASCII
//int main()
//{
// int arr[] = { 73, 32, 99, 97, 110, 32, 100, 111, 32, 105, 116 , 33 };
// int sz = sizeof(arr) / sizeof(arr[0]);
// for (int i = 0; i < sz; i++)
// {
// printf("%c", arr[i]);
// }
//
// return 0;
//}
//#include <stdio.h>
////bc14.输入一个人的出生日期(包括年月日),将该生日中的年、月、日分别输出
//
//int main()
//{
// int year,month,date;
// scanf("%4d%2d%2d", &year,&month,&date);
// //通过scanf函数的% m格式控制可以指定输入域宽,输入数据域宽(列数),按此宽度截取所需数据
// //%4d格式控制符,限制scanf截取4位放入指定变量
// printf("year=%d\n", year);
// printf("month=%02d\n", month);//%02d,两位,不够左补0
// printf("date=%02d\n", date);
//
// return 0;
//}
//#include <stdio.h>
////bc15-交换两个数并输出
//int main()
//{
// //a=1,b=2
// int a, b;
// scanf("a=%d,b=%d", &a, &b);
// int tmp=a;//交换
// a = b;
// b = tmp;
// printf("a=%d,b=%d",a,b);
// return 0;
//}
//#include<stdio.h>
////新bc15,交换大小写
//int main()
//{
// char a;
// while (scanf("%c", &a) != EOF)
// {
// getchar();//吞掉回车
// char b = a + 32;
// printf("%c\n", b);
// }
//
//
// return 0;
//}
//#include <stdio.h>
////bc16输出字符对应的ASCII码
//int main()
//{
// char a = 0;
// scanf("%c", &a);
// printf("%d\n",a);
//
// return 0;
//}
//#include<stdio.h>
////新BC16 十六进制转十进制
//int main()
//{
// int a = 0xABCDEF;
// printf("%15d\n", a);
//
// return 0;
//}
//#include<stdio.h>
//
////bc17:计算(-8 + 22)×a - 10 + c÷2,其中,a = 40,c = 212。
//int main()
//{
// int sum = 0;
// int a = 40, c = 212;
// sum = (22-8) * a-10+c/2;
// printf("%d", sum);
// return 0;
//}
//#include<stdio.h>
////bc18,输入a和b,计算a和b的商+余数
//int main()
//{
// int a, b;
// scanf("%d %d", &a, &b);
//
// printf("%d %d", a / b, a % b);
//
// return 0;
//}
//#include <stdio.h>
////新BC17:10进制转8和16
//int main()
//{
// int a = 1234;
// printf("%#o %#X", a, a);
// return 0;
//}
//
//#include<stdio.h>
//BC18 牛牛的空格分隔
//int main()
//{
// char a;
// int b;
// float c;
// scanf("%c %d %f", &a, &b, &c);
// printf("%c %d %f\n", a, b, c);
//
//
// return 0;
//}
//
//#include<stdio.h>
////bc19反向输出一个4位数
//int main()
//{
// int n;
// scanf("%d", &n);
// while (n)
// {
// printf("%d", n % 10);
// n /= 10;
// }
//
// return 0;
//}
//#include<stdio.h>
////新BC19 牛牛的对齐
//int main()
//{
// int a, b, c;
// scanf("%d %d %d", &a, &b, &c);
// printf("%-8d%-8d%-8d\n", a, b, c);//靠右对齐需要加-
//
// return 0;
//}
//
//#include<stdio.h>
////bc20
////计算规则45+80=25,1234+10=34+10
//int main()
//{
// int a, b;
// int sum;
// scanf("%d%d", &a, &b);
//
// int a1, b1;
// a1 = a % 10;
// a /= 10;
// a1 += a % 10 * 10;
// b1 = b % 10;
// b /= 10;
// b1 += b % 10 * 10;
//
// //printf("%d %d", a1, b1);
// sum = a1 + b1;
//
// int sum1 = 0;
// sum1 = sum % 10;
// sum /= 10;
// sum1 += sum % 10 * 10;
//
// //if (a <= 100 && b <= 100)
// //{
// // sum = a + b;
// // if (sum >= 100&&sum!=200)
// // {
// // sum -= 100;
// // }
// // else if (sum == 200)
// // {
// // sum = 0;
// // }
// //}
//
// printf("%d", sum1);
// return 0;
//}
//#include<stdio.h>
////新BC20 进制A+B
//int main()
//{
// int a, b;
// scanf("%x", &a);//输入十六进制,0x小写
// scanf("%o", &b);//输入八进制数
// int c = a + b;
//
// printf("%d\n", c);
//
// return 0;
//}
//#include<stdio.h>
////bc21浮点数的个位数字
//int main()
//{
// float a = 0;
// scanf("%f", &a);
//
// int b= (int)a % 10;
// printf("%d", b);
// return 0;
//}
//#include<stdio.h>
////新BC21 牛牛学加法
//int main()
//{
// int a, b;
// scanf("%d %d", &a, &b);
// printf("%d\n", a + b);
//
// return 0;
//}
//#include<stdio.h>
////BC22:一年约有 3.156×10^7 s,要求输入您的年龄,显示该年龄合多少秒
//int main()
//{
// double f = 3.156E7;
// double old = 0;
// scanf("%lf", &old);
// double s = old * f;
// printf("%.0lf", s);
//
// return 0;
//}
//#include<stdio.h>
////新BC22 牛牛学除法
//int main()
//{
// int a, b;
// scanf("%d %d", &a, &b);
// printf("%d\n", a / b);
//
// return 0;
//}
//#include<stdio.h>
////BC23 给定秒数seconds,把秒转化成小时、分钟和秒
//int main()
//{
// int s = 0;
// scanf("%d", & s);
//
// //s/60得到小时
// //s%60得到余数,再÷60得到分钟
//
// //3661: 1h 1m 1s
// int seconds = s % 3600;
// if (seconds >= 60)
// {
// seconds %= 60;
// }
// s -= seconds;//3660
//
// int min = s/ 60;//61
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
多人OJ打卡系统项目源码,共507个文件,采用C++和C等语言开发,涉及多种文件类型如C++源代码、C源代码、Markdown文档、PNG图片、文本文件、Git忽略文件、LICENSE文件、C源代码、SUO文件和SLN文件等。该项目是一个基于C++和C的多人OJ打卡系统设计,旨在提供一个高效、稳定的多人OJ打卡解决方案。欢迎加入这个多人OJ打卡仓库。
资源推荐
资源详情
资源评论
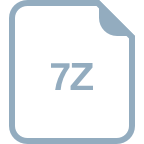
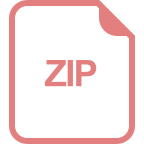
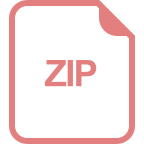
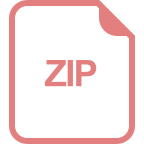
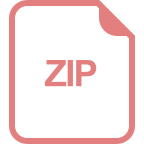
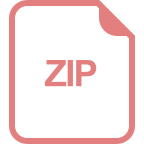
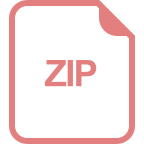
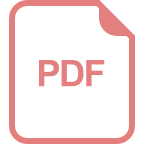
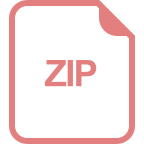
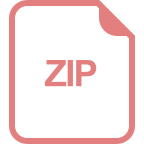
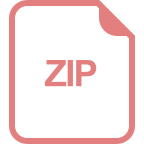
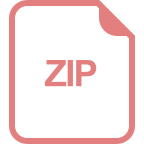
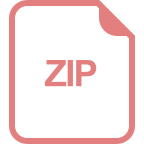
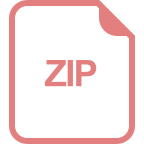
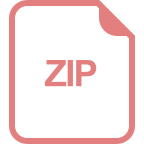
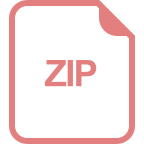
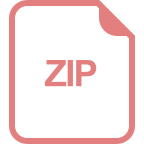
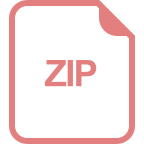
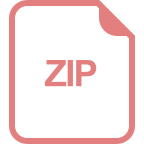
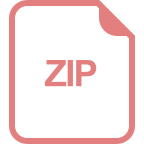
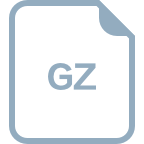
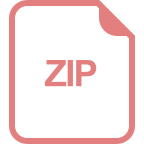
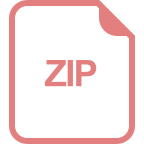
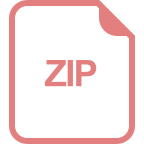
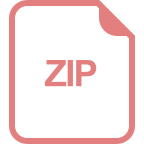
收起资源包目录

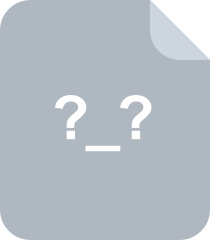
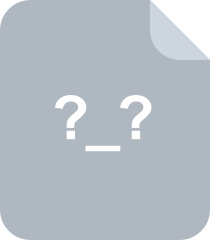
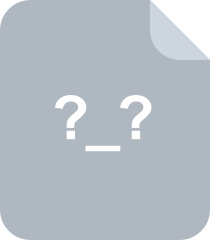
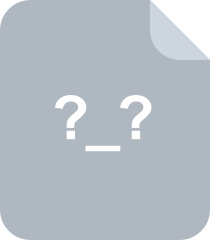
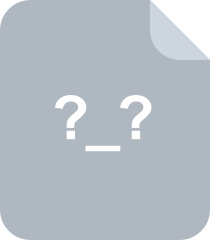
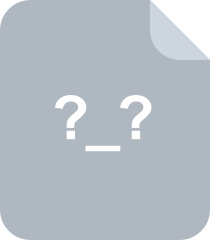
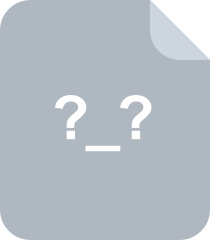
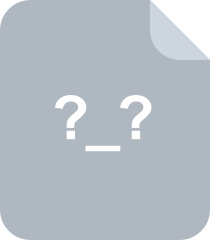
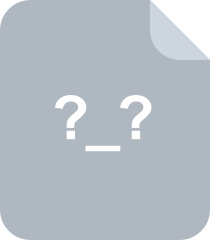
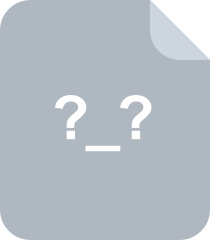
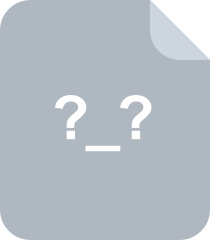
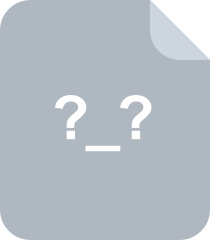
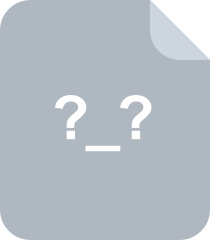
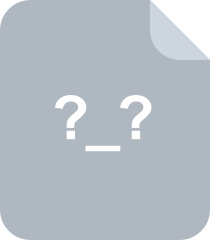
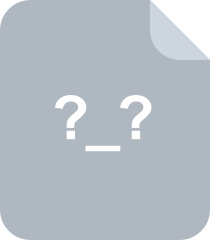
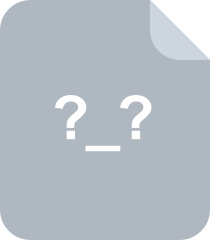
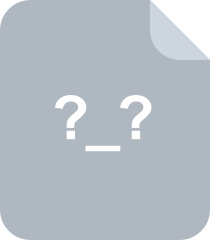
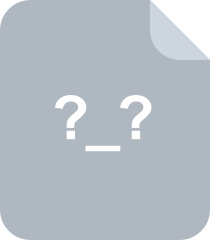
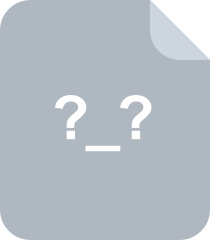
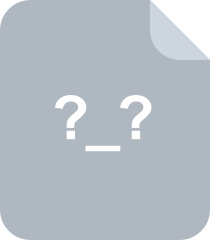
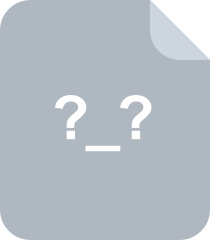
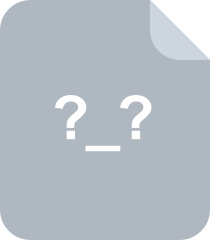
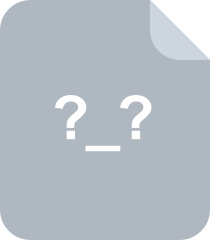
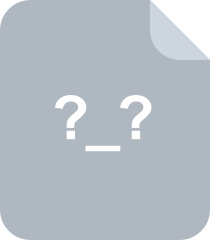
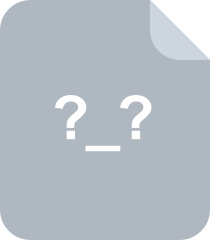
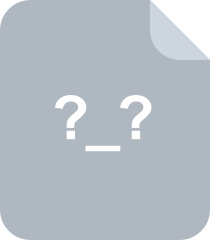
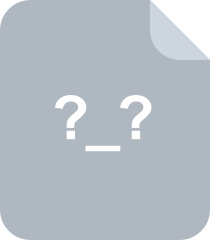
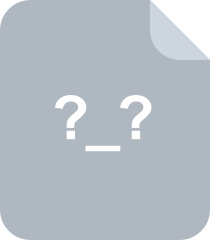
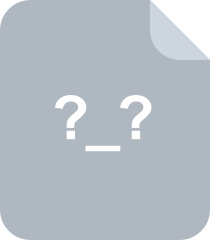
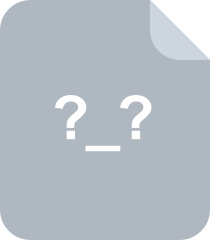
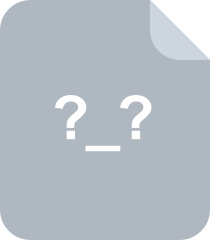
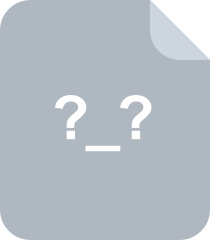
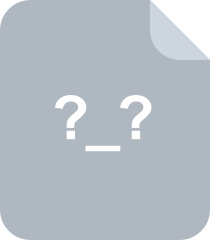
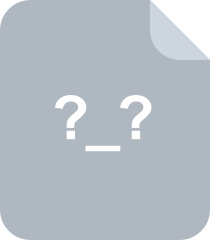
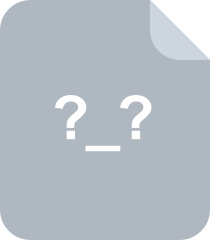
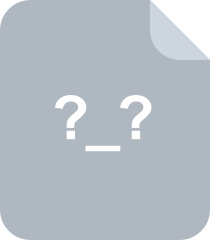
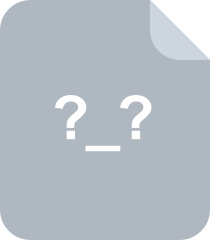
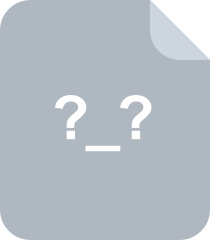
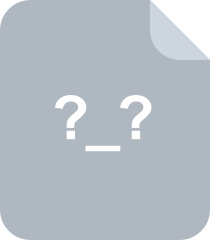
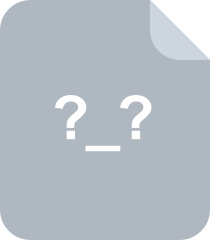
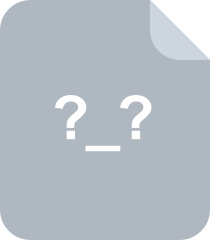
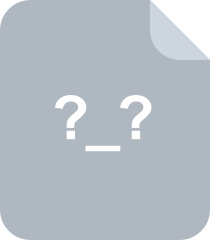
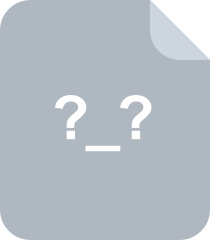
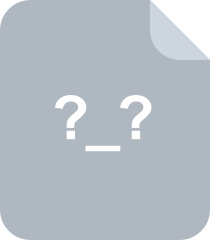
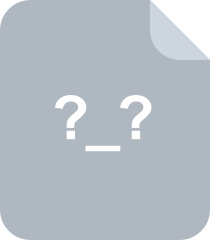
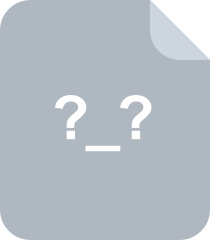
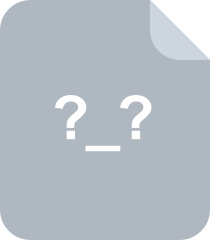
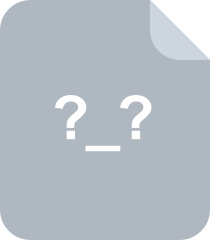
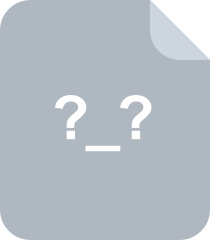
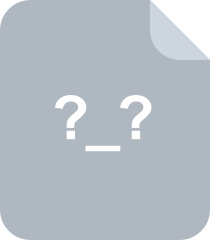
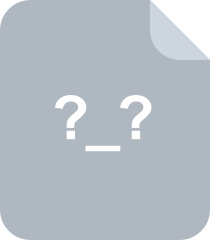
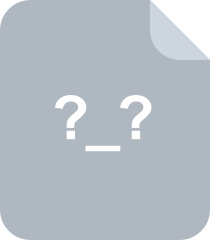
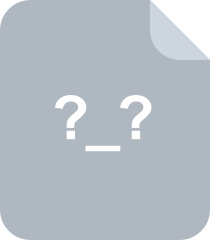
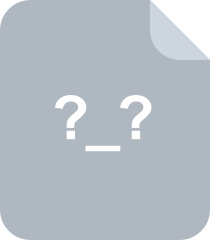
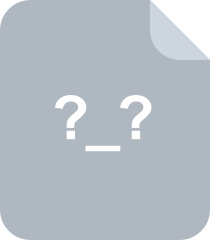
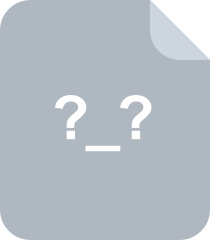
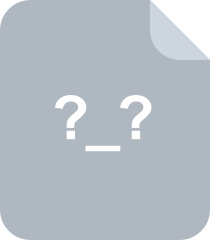
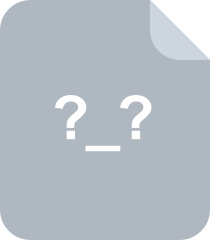
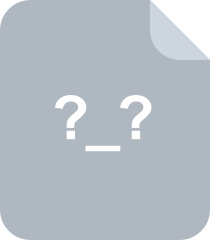
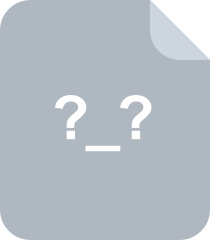
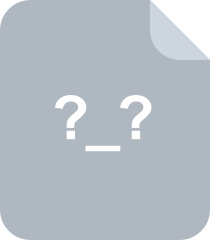
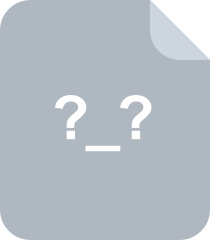
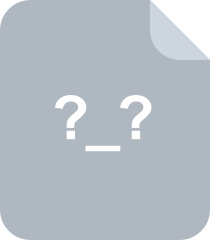
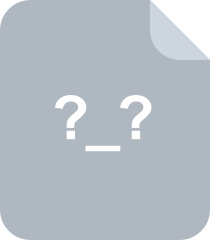
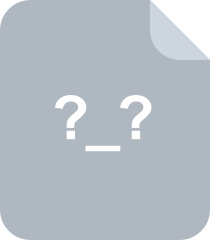
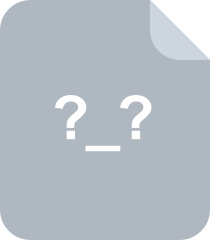
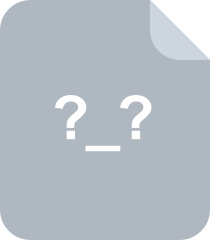
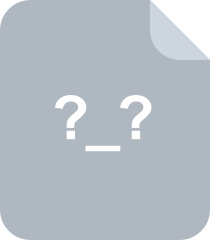
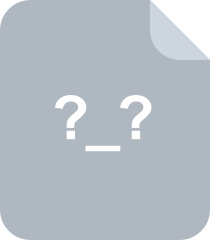
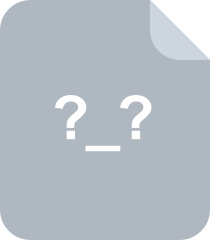
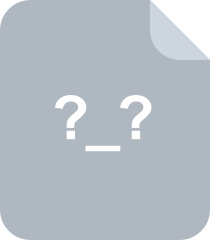
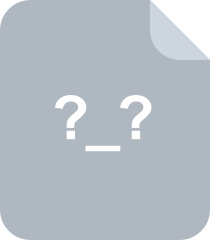
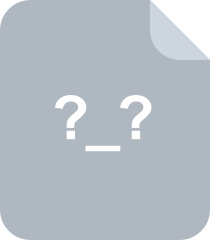
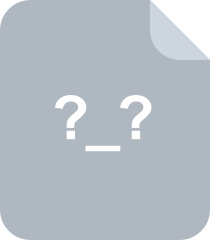
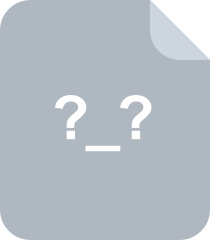
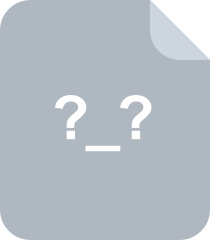
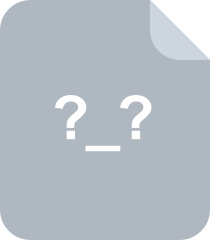
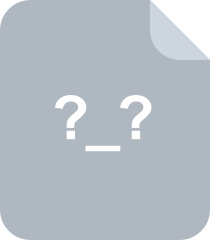
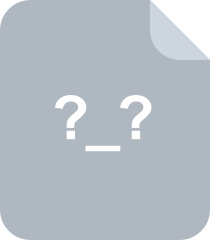
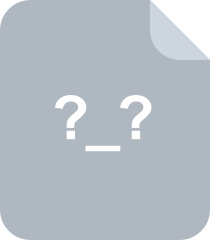
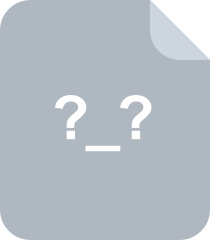
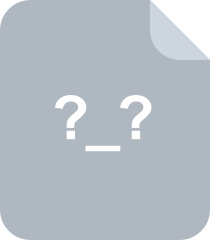
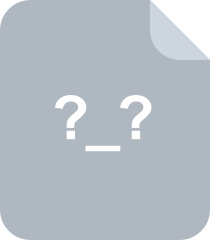
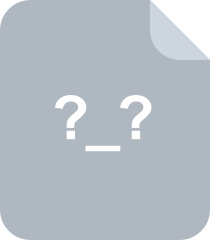
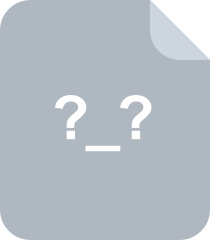
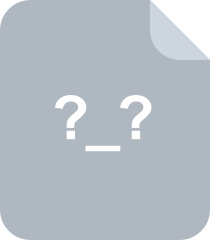
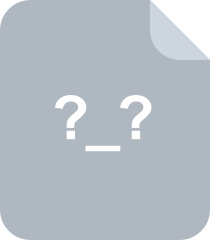
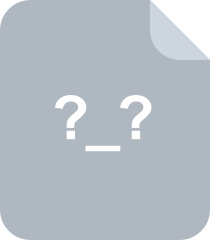
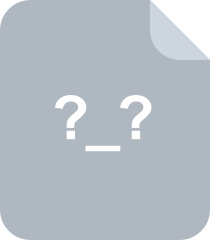
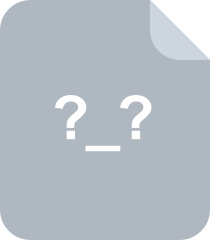
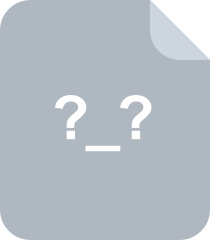
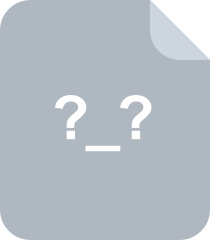
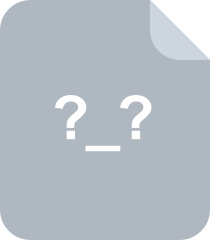
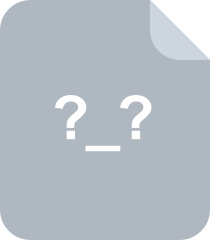
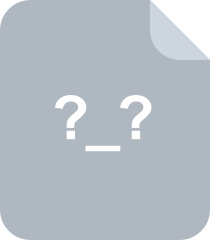
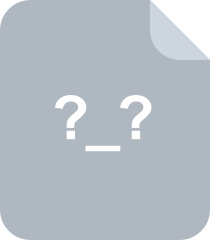
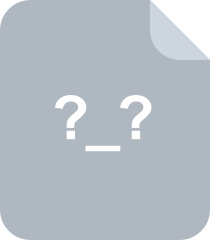
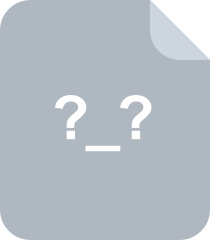
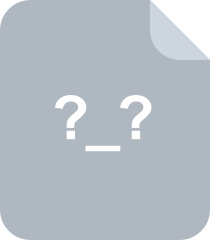
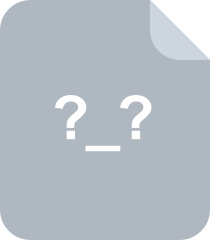
共 515 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
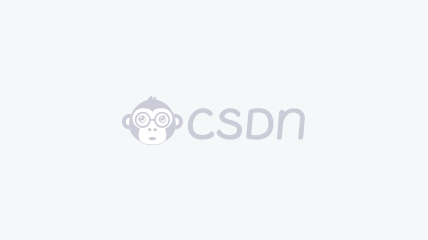

沐知全栈开发
- 粉丝: 5814
- 资源: 5226
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

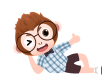
最新资源
- 基于php+mysql+微信小程序的家校联系小程序 源码+数据库(高分毕业设计).zip
- 带有平面定位系统的机器人模型sw2018可编辑全套技术资料100%好用.zip
- 通过html创建一个基本的圣诞树形状并添加飘雪花效果.zip
- HiSPi Interface Protocol V1.50.00 - Rev. B
- 最新更新!!!全国及各城市POI数据2012-2023年
- (24562814)Simulink永磁同步电机控制仿真系列2模型
- (2632060)奇异值分解(svd)的delphi代码
- (3625040)k-means聚类算法
- (43006034)AP3010DN-V2-FAT-V200R019C00SPC905.zip
- (4680440)Java坦克大战源码
- 某安全大厂重要参数被曝
- (66389424)MFC实现多边形裁剪 计算机图形学
- (8637652)java 坦克大战
- (9517836)网络编程UDP
- (102127232)【电力负荷预测】遗传算法优化BP神经网络电力负荷预测【含Matlab源码 1524期】.zip
- (11551018)数控直流稳压电源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


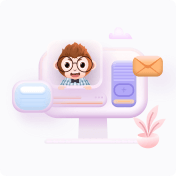
安全验证
文档复制为VIP权益,开通VIP直接复制
