import java.io.*;
import java.util.LinkedList;
public class File类 {
public File类() {
}
//===============1===============
public void writer(String list) throws IOException {
String[] strings = list.split(",");
//在几班,从String中获取数字
String str = strings[4].trim();
String string1 = "";
if (str != null && !"".equals(str)) {
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) >= 48 && str.charAt(i) <= 57) {
string1 += str.charAt(i);
}
}
}
int x = Integer.parseInt(string1);
//学号,从String中获取数字
String string2 = strings[0].trim();
String string3 = "";
if (string2 != null && !"".equals(string2)) {
for (int i = 0; i < string2.length(); i++) {
if (string2.charAt(i) >= 48 && string2.charAt(i) <= 57) {
string3 += string2.charAt(i);
}
}
}
int a = Integer.parseInt(string3);
//目录
File file = new File("E:\\student");
File file1 = new File("E:\\student\\classNum" + x);
String string = "";
String[] strings3 = file.list();
for (String s : strings3) {
string += s;
}
//获取目录下的所有文件名称
if (string.contains("E:\\student\\classNum" + x)) {
//文件.txt
String str1 = "E:\\student\\classNum" + x + "\\student" + a + ".txt";
BufferedWriter bw = new BufferedWriter(new FileWriter(str1, true));
bw.write(list);
bw.newLine();
bw.flush();
bw.close();
} else {
//不存在file1
String str2 = "E:\\student\\classNum" + x;
File file2 = new File(str2);
//创建新班级
file2.mkdir();
String str1 = str2 + "\\student" + a + ".txt";
BufferedWriter bw = new BufferedWriter(new FileWriter(str1, true));
bw.write(list);
bw.newLine();
bw.flush();
bw.close();
}
}
//===============2===============
public void delStudent(int stuNum) throws IOException {
String s1 = "stuNum=" + stuNum;
String s2 = getAll();
String[] strings1 = s2.split("\n");
//从全部信息中获得哪一个是学号是stuNum的学生信息
int x = 0;
for (int i = 0; i < strings1.length; i++) {
if (strings1[i].contains(s1)) {
x = i;
break;
}
}
//获得班号
String[] strings = strings1[x].split(",");
try {
String string2 = strings[4].trim();
String string3 = "";
if (string2 != null && !"".equals(string2)) {
for (int i = 0; i < string2.length(); i++) {
if (string2.charAt(i) >= 48 && string2.charAt(i) <= 57) {
string3 += string2.charAt(i);
}
}
}
//班号
int a = Integer.parseInt(string3);
String s3 = "E:\\student\\classNum" + a + "\\student" + stuNum + ".txt";
File file = new File(s3);
//判断是否删除成功
if (file.delete()) {
System.out.println("删除成功");
} else if (!file.exists()) {
System.out.println("删除失败,该学生不存在");
}
} catch (ArrayIndexOutOfBoundsException e) {
}
}
//获取全部学生信息,并返回学生信息的字符串;
public String getAll() throws IOException {
String s1 = "";
String s3 = "";
String s5 = "";
LinkedList<String> lii = new LinkedList<>();
File file = new File("E:\\student");
File[] files = file.listFiles();
for (File F : files) {
s1 += F.getAbsolutePath();
}
String[] strings = s1.split("E");
for (int i = 1; i < strings.length; i++) {
String s2 = "E" + strings[i];
File file1 = new File(s2);
File[] files1 = file1.listFiles();
for (File F : files1) {
s3 += F.getAbsolutePath();
}
}
String[] strings1 = s3.split("E");
for (int i = 1; i < strings1.length; i++) {
String s4 = "E" + strings1[i];
File file1 = new File(s4);
BufferedReader br = new BufferedReader(new FileReader(file1));
lii.add(br.readLine());
br.close();
}
for (int i = 0; i < lii.size(); i++) {
s5 += lii.get(i) + "\n";
}
return s5;
}
//===============3===============
public void age加() throws IOException {
String[] strings = getAll().split("\n");
for (int i = 0; i < strings.length; i++) {
String[] strings1 = strings[i].split(",");
//stuNum是几
String string2 = strings1[0].trim();
String string3 = "";
if (string2 != null && !"".equals(string2)) {
for (int j = 0; j < string2.length(); j++) {
if (string2.charAt(j) >= 48 && string2.charAt(j) <= 57) {
string3 += string2.charAt(j);
}
}
}
//学号
int a = Integer.parseInt(string3);
//classNum是几
String string4 = strings1[4].trim();
String string5 = "";
if (string4 != null && !"".equals(string4)) {
for (int k = 0; k < string4.length(); k++) {
if (string4.charAt(k) >= 48 && string4.charAt(k) <= 57) {
string5 += string4.charAt(k);
}
}
}
//班号
int b = Integer.parseInt(string5);
//age是几
String string6 = strings1[3].trim();
String string7 = "";
if (string6 != null && !"".equals(string6)) {
for (int l = 0; l < string6.length(); l++) {
if (string6.charAt(l) >= 48 && string6.charAt(l) <= 57) {
string7 += string6.charAt(l);
}
}
}
//年龄
int c = Integer.parseInt(string7) + 1;
String s = "E:\\student\\classNum" + b + "\\student" + a + ".txt";
File file1 = new File(s);
file1.delete();
File file = new File(s);
String s1 = strings1[0] + "," + strings1[1] + "," + strings1[2] + ", " + "age=" + c + "," + strings1[4];
try {
BufferedWriter bw = new BufferedWriter(new FileWriter(file));
bw.write(s1);
bw.close();
} catch (FileNotFoundException e) {
}
}
System.out.println("操作成功");
}
//===============4===============
public void shift(int stuNum, int classNum, String classNum1) throws IOException {
//转移前的路径
String s1 = "E:\\student\\classNum" + classNum + "\\student" + stuNum + ".txt";
File file1 = new File(s1);
//转移后的路径
String s2 = "E:\\student\\classNum" + classNum1 + "\\student" + stuNum + ".txt";
File file = new File(s2);
try {
BufferedReader br = new BufferedReader(new FileReader(s1));
BufferedWriter bw = new BufferedWriter(new FileWriter(s2));
String line;
while ((line = br.readLine()) != null) {
String[] strings = line.split(",");
String s3 = "classNum=" + classNum1 + "}";
String s4 = strings[0] + "," + strings[1] + "," + strings[2] + "," + strings[3] + ", " + s3;
bw.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是基于Java的JavaEE企业级应用设计源码,包含357个文件,其中包括346个Java源文件、7个TXT文本文件、2个PNG图像文件、1个Properties配置文件和1个PDF文档。系统专注于提供企业级应用功能,支持用户管理、权限控制等功能,为用户提供了一个稳定、高效的JavaEE应用开发平台。
资源推荐
资源详情
资源评论
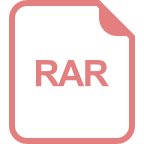
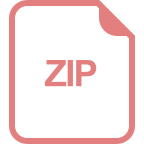
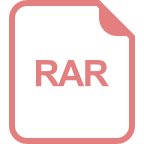
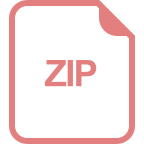
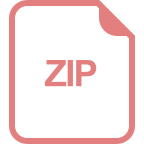
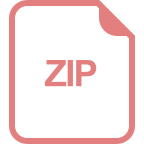
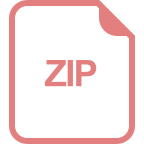
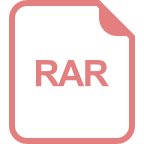
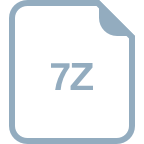
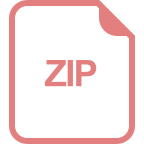
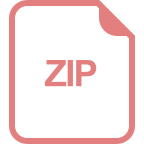
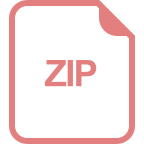
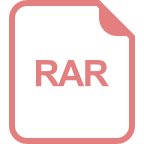
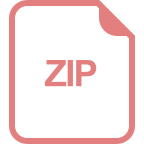
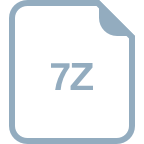
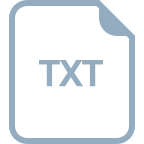
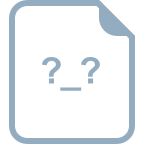
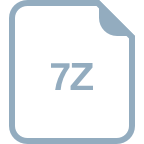
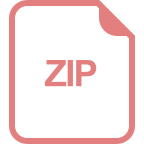
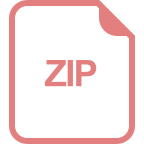
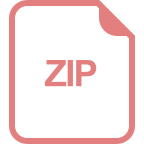
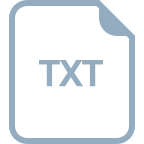
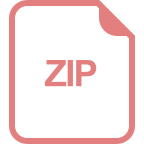
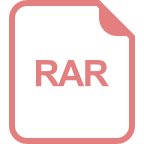
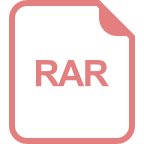
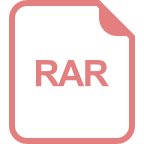
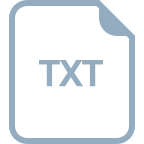
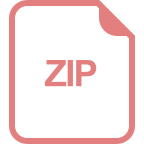
收起资源包目录

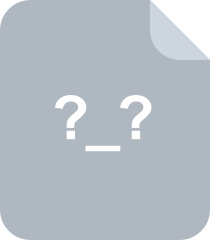
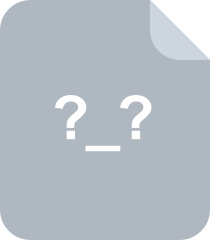
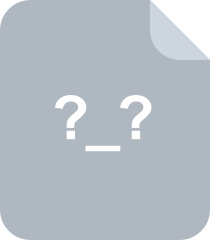
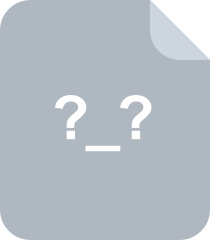
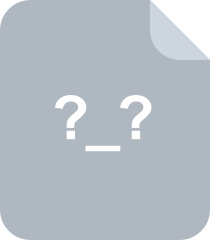
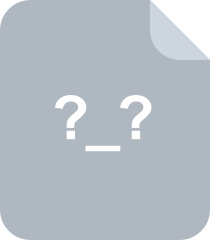
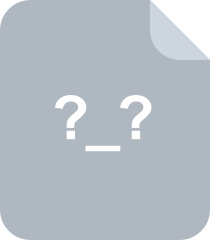
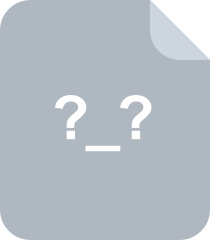
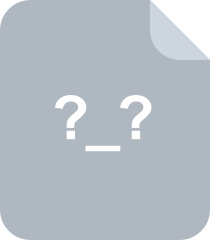
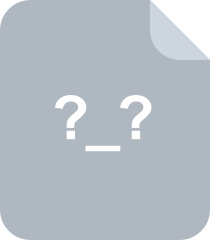
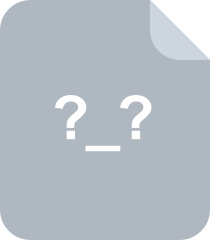
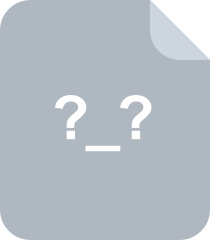
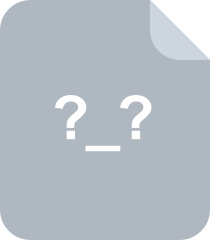
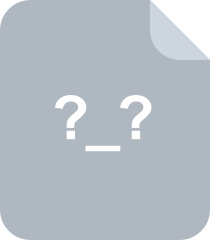
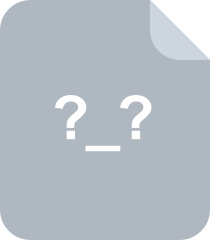
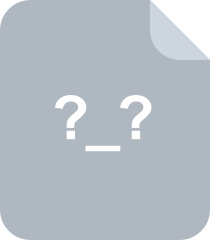
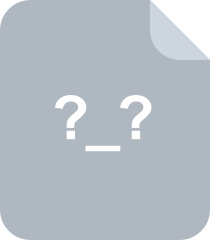
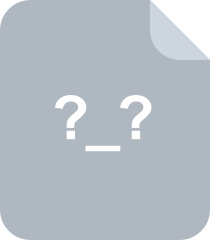
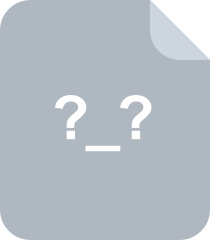
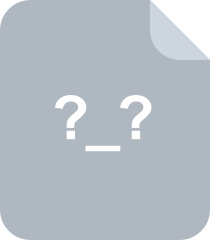
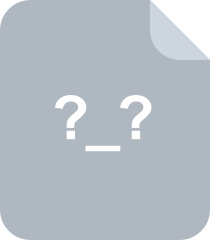
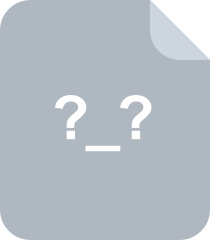
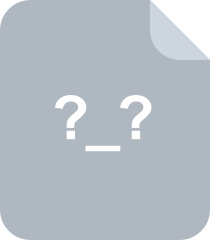
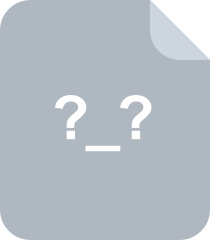
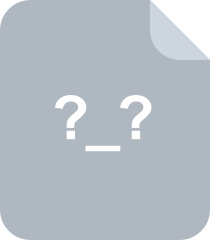
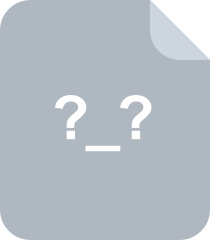
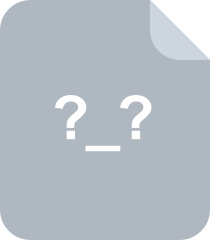
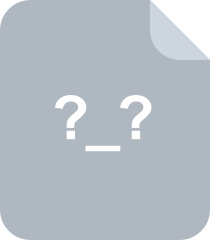
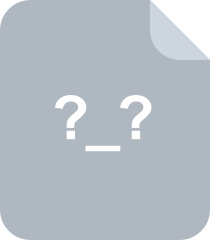
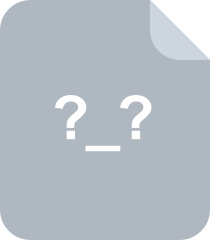
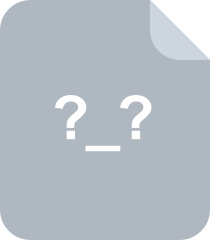
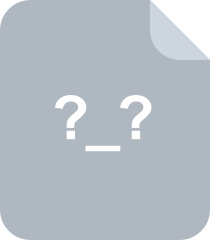
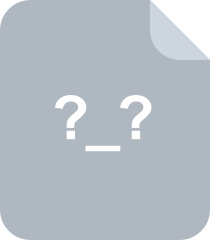
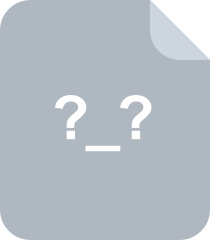
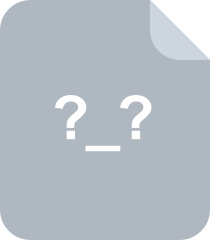
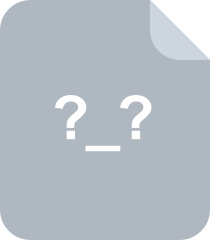
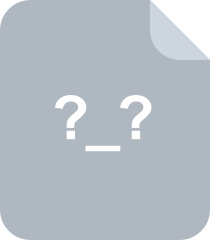
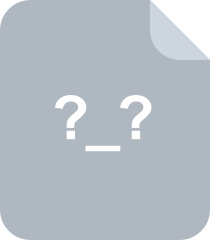
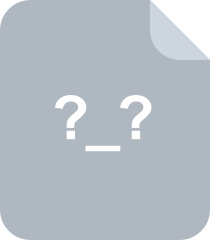
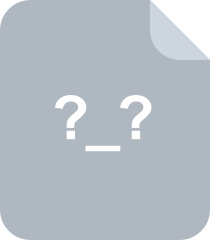
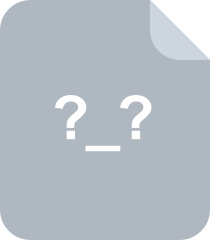
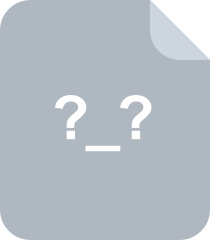
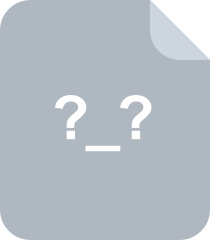
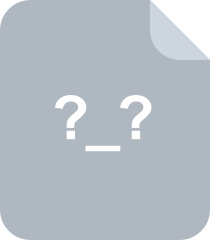
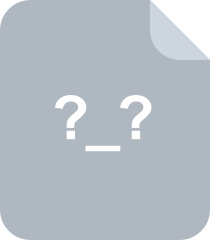
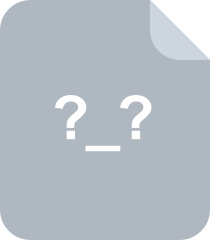
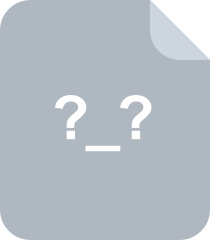
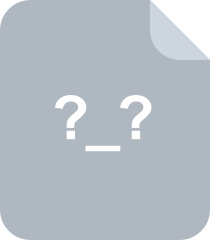
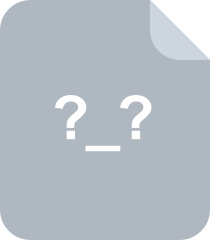
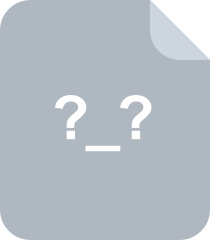
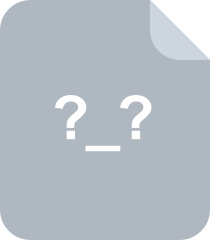
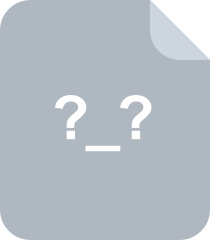
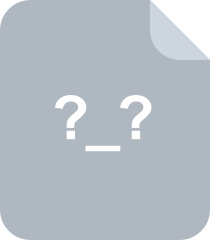
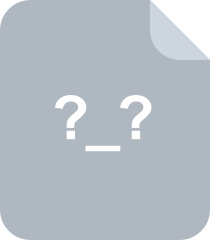
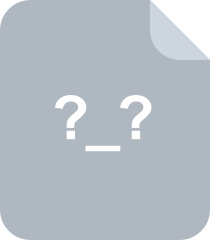
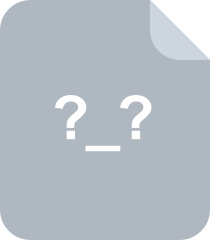
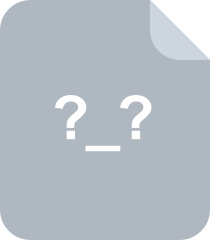
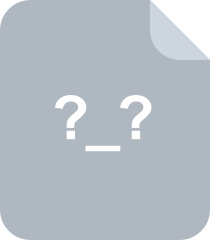
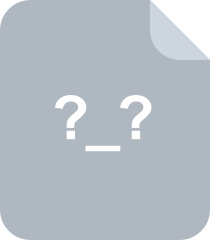
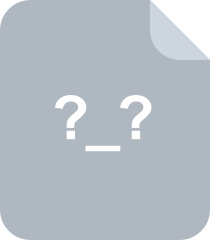
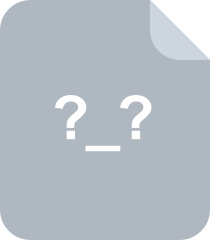
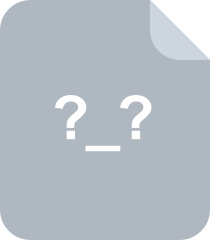
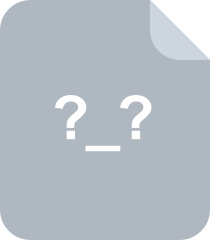
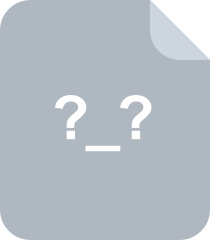
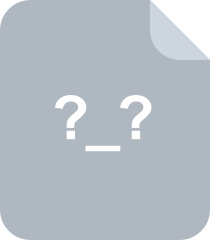
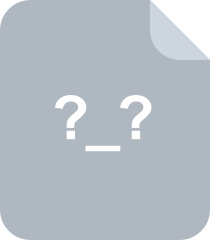
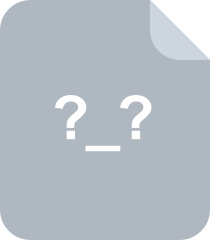
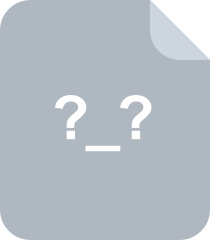
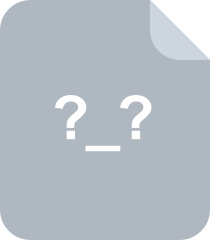
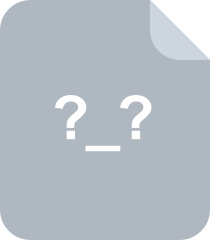
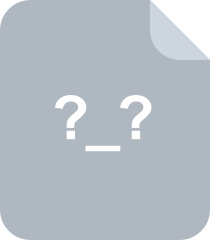
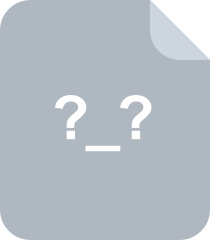
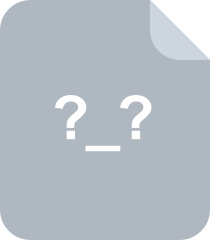
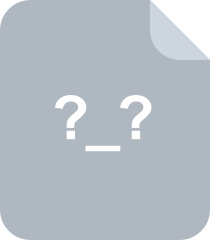
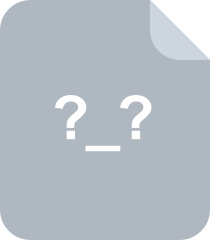
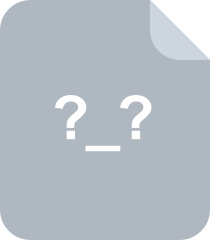
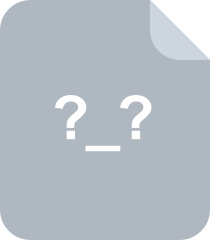
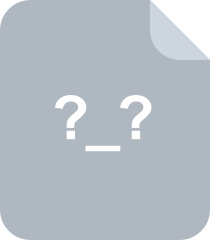
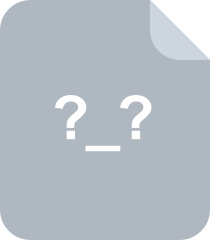
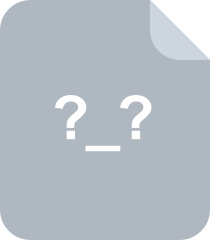
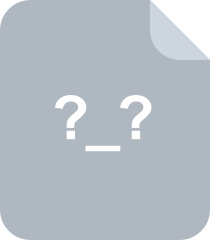
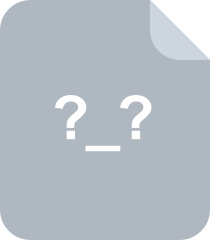
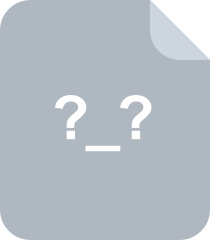
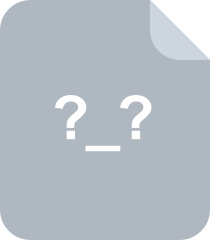
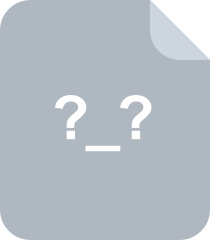
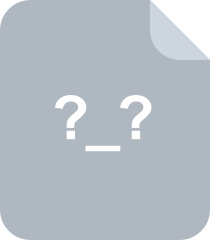
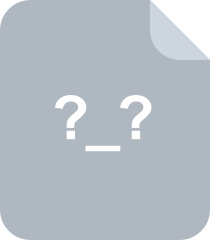
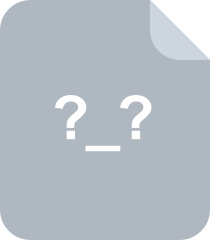
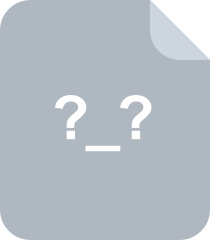
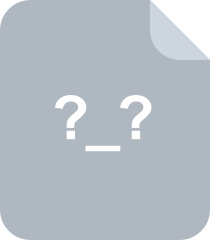
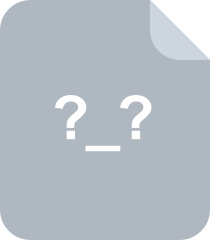
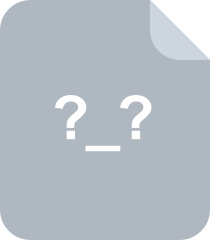
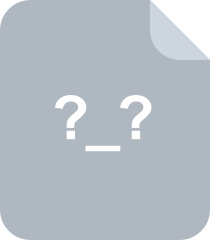
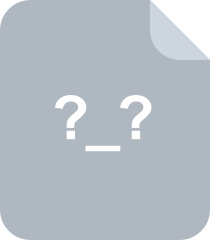
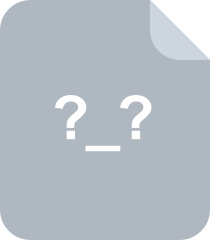
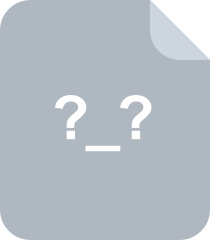
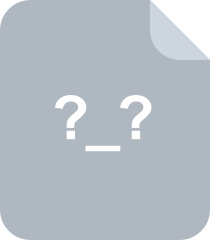
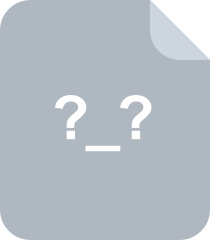
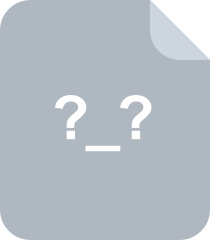
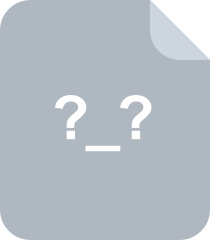
共 360 条
- 1
- 2
- 3
- 4
资源评论
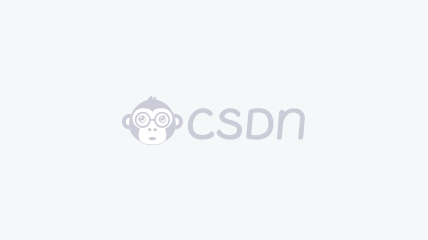

沐知全栈开发
- 粉丝: 5706
- 资源: 5224
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

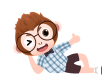
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


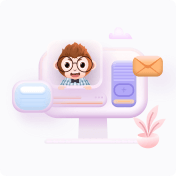
安全验证
文档复制为VIP权益,开通VIP直接复制
