/*! *****************************************************************************
Copyright (c) Microsoft Corporation. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License"); you may not use
this file except in compliance with the License. You may obtain a copy of the
License at http://www.apache.org/licenses/LICENSE-2.0
THIS CODE IS PROVIDED ON AN *AS IS* BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, EITHER EXPRESS OR IMPLIED, INCLUDING WITHOUT LIMITATION ANY IMPLIED
WARRANTIES OR CONDITIONS OF TITLE, FITNESS FOR A PARTICULAR PURPOSE,
MERCHANTABLITY OR NON-INFRINGEMENT.
See the Apache Version 2.0 License for specific language governing permissions
and limitations under the License.
***************************************************************************** */
"use strict";
var ts = (() => {
var __defProp = Object.defineProperty;
var __getOwnPropNames = Object.getOwnPropertyNames;
var __esm = (fn, res) => function __init() {
return fn && (res = (0, fn[__getOwnPropNames(fn)[0]])(fn = 0)), res;
};
var __commonJS = (cb, mod) => function __require() {
return mod || (0, cb[__getOwnPropNames(cb)[0]])((mod = { exports: {} }).exports, mod), mod.exports;
};
var __export = (target, all) => {
for (var name in all)
__defProp(target, name, { get: all[name], enumerable: true });
};
// src/compiler/corePublic.ts
var versionMajorMinor, version, Comparison;
var init_corePublic = __esm({
"src/compiler/corePublic.ts"() {
"use strict";
versionMajorMinor = "5.2";
version = "5.2.2";
Comparison = /* @__PURE__ */ ((Comparison3) => {
Comparison3[Comparison3["LessThan"] = -1] = "LessThan";
Comparison3[Comparison3["EqualTo"] = 0] = "EqualTo";
Comparison3[Comparison3["GreaterThan"] = 1] = "GreaterThan";
return Comparison3;
})(Comparison || {});
}
});
// src/compiler/core.ts
function length(array) {
return array ? array.length : 0;
}
function forEach(array, callback) {
if (array) {
for (let i = 0; i < array.length; i++) {
const result = callback(array[i], i);
if (result) {
return result;
}
}
}
return void 0;
}
function forEachRight(array, callback) {
if (array) {
for (let i = array.length - 1; i >= 0; i--) {
const result = callback(array[i], i);
if (result) {
return result;
}
}
}
return void 0;
}
function firstDefined(array, callback) {
if (array === void 0) {
return void 0;
}
for (let i = 0; i < array.length; i++) {
const result = callback(array[i], i);
if (result !== void 0) {
return result;
}
}
return void 0;
}
function firstDefinedIterator(iter, callback) {
for (const value of iter) {
const result = callback(value);
if (result !== void 0) {
return result;
}
}
return void 0;
}
function reduceLeftIterator(iterator, f, initial) {
let result = initial;
if (iterator) {
let pos = 0;
for (const value of iterator) {
result = f(result, value, pos);
pos++;
}
}
return result;
}
function zipWith(arrayA, arrayB, callback) {
const result = [];
Debug.assertEqual(arrayA.length, arrayB.length);
for (let i = 0; i < arrayA.length; i++) {
result.push(callback(arrayA[i], arrayB[i], i));
}
return result;
}
function intersperse(input, element) {
if (input.length <= 1) {
return input;
}
const result = [];
for (let i = 0, n = input.length; i < n; i++) {
if (i)
result.push(element);
result.push(input[i]);
}
return result;
}
function every(array, callback) {
if (array) {
for (let i = 0; i < array.length; i++) {
if (!callback(array[i], i)) {
return false;
}
}
}
return true;
}
function find(array, predicate, startIndex) {
if (array === void 0)
return void 0;
for (let i = startIndex ?? 0; i < array.length; i++) {
const value = array[i];
if (predicate(value, i)) {
return value;
}
}
return void 0;
}
function findLast(array, predicate, startIndex) {
if (array === void 0)
return void 0;
for (let i = startIndex ?? array.length - 1; i >= 0; i--) {
const value = array[i];
if (predicate(value, i)) {
return value;
}
}
return void 0;
}
function findIndex(array, predicate, startIndex) {
if (array === void 0)
return -1;
for (let i = startIndex ?? 0; i < array.length; i++) {
if (predicate(array[i], i)) {
return i;
}
}
return -1;
}
function findLastIndex(array, predicate, startIndex) {
if (array === void 0)
return -1;
for (let i = startIndex ?? array.length - 1; i >= 0; i--) {
if (predicate(array[i], i)) {
return i;
}
}
return -1;
}
function findMap(array, callback) {
for (let i = 0; i < array.length; i++) {
const result = callback(array[i], i);
if (result) {
return result;
}
}
return Debug.fail();
}
function contains(array, value, equalityComparer = equateValues) {
if (array) {
for (const v of array) {
if (equalityComparer(v, value)) {
return true;
}
}
}
return false;
}
function arraysEqual(a, b, equalityComparer = equateValues) {
return a.length === b.length && a.every((x, i) => equalityComparer(x, b[i]));
}
function indexOfAnyCharCode(text, charCodes, start) {
for (let i = start || 0; i < text.length; i++) {
if (contains(charCodes, text.charCodeAt(i))) {
return i;
}
}
return -1;
}
function countWhere(array, predicate) {
let count = 0;
if (array) {
for (let i = 0; i < array.length; i++) {
const v = array[i];
if (predicate(v, i)) {
count++;
}
}
}
return count;
}
function filter(array, f) {
if (array) {
const len = array.length;
let i = 0;
while (i < len && f(array[i]))
i++;
if (i < len) {
const result = array.slice(0, i);
i++;
while (i < len) {
const item = array[i];
if (f(item)) {
result.push(item);
}
i++;
}
return result;
}
}
return array;
}
function filterMutate(array, f) {
let outIndex = 0;
for (let i = 0; i < array.length; i++) {
if (f(array[i], i, array)) {
array[outIndex] = array[i];
outIndex++;
}
}
array.length = outIndex;
}
function clear(array) {
array.length = 0;
}
function map(array, f) {
let result;
if (array) {
result = [];
for (let i = 0; i < array.length; i++) {
result.push(f(array[i], i));
}
}
return result;
}
function* mapIterator(iter, mapFn) {
for (const x of iter) {
yield mapFn(x);
}
}
function sameMap(array, f) {
if (array) {
for (let i = 0; i < array.length; i++) {
const item = array[i];
const mapped = f(item, i);
if (item !== mapped) {
const result = array.slice(0, i);
result.push(mapped);
for (i++; i < array.length; i++) {
result.push(f(array[i], i));
}
return result;
}
}
}
return array;
}
function flatten(array) {
const result = [];
for (const v of array) {
if (v) {
if (isArray(v)) {
addRange(result, v);
} else {
result.push(v);
}
}
}
return result;
}
function flatMap(array, mapfn) {
let result;
if (array) {
for (let i = 0; i < array.length; i++) {
const v = mapfn(array[i], i);
if (v) {
if (isArray(v)) {
result = addRan
没有合适的资源?快使用搜索试试~ 我知道了~
基于Vue.js的uni-app跨平台应用前端框架设计源码
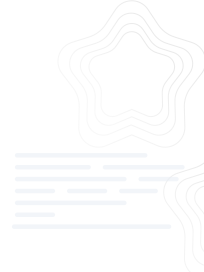
共2000个文件
ts:1216个
js:227个
json:198个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 122 浏览量
2024-04-19
15:16:27
上传
评论
收藏 138.16MB ZIP 举报
温馨提示
本源码为基于Vue.js的uni-app跨平台应用前端框架设计,共包含1967个文件,其中js文件1405个,json文件127个,vue文件107个,ts文件102个,md文件74个,png文件25个,wxss文件11个,html文件11个,css文件10个,wxml文件7个。该项目是一个使用Vue.js开发跨平台应用的前端框架,适合用于开发多种平台的应用。
资源推荐
资源详情
资源评论
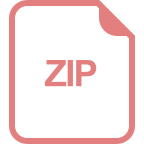
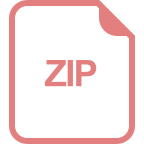
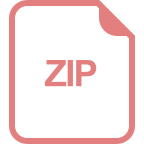
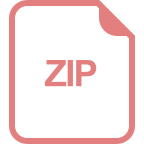
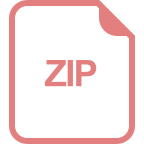
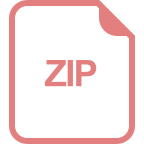
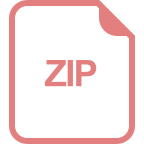
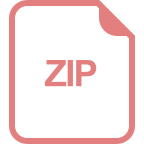
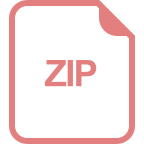
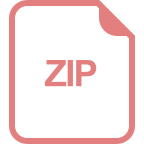
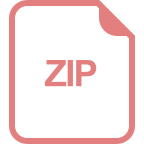
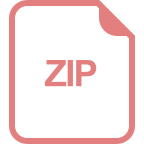
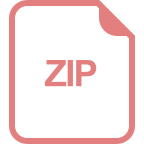
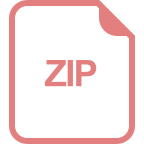
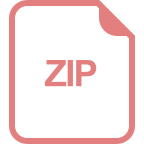
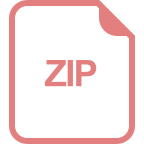
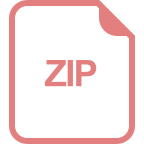
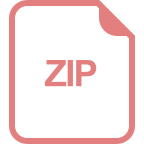
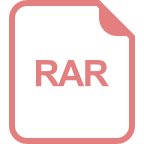
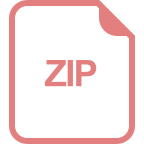
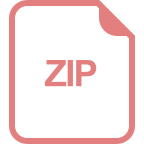
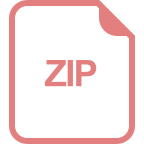
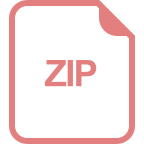
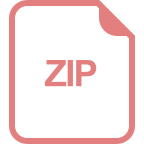
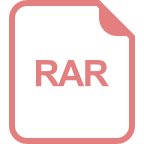
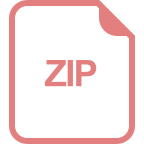
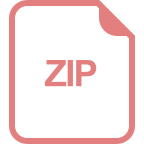
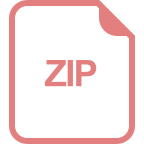
收起资源包目录

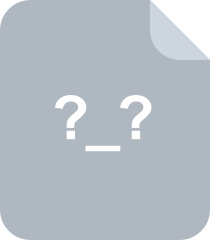
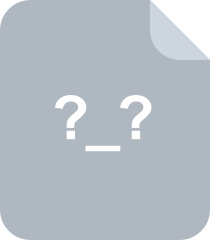
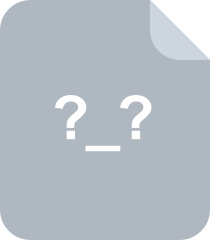
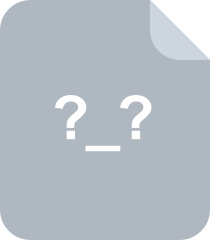
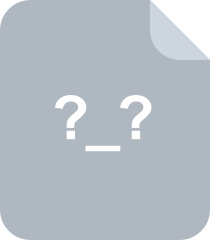
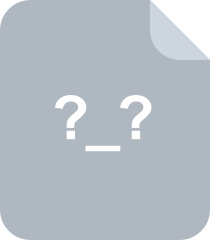
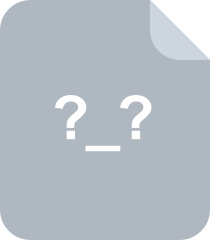
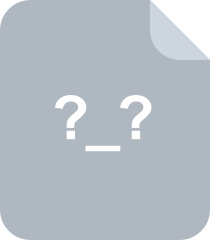
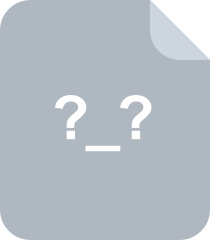
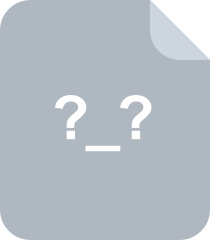
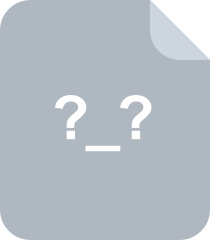
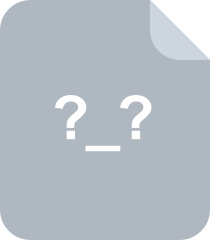
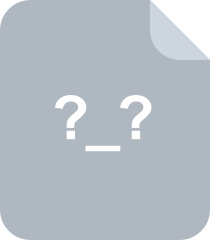
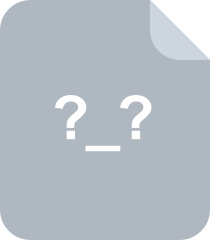
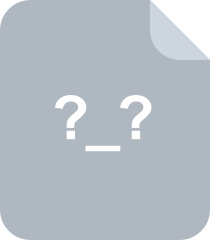
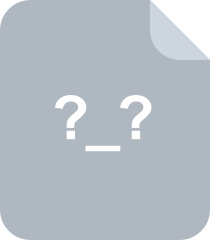
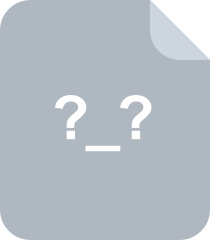
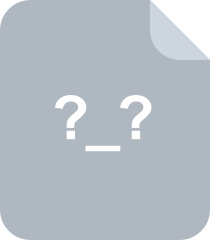
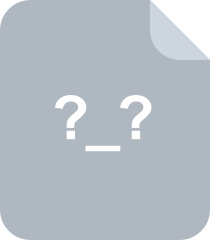
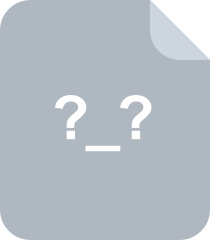
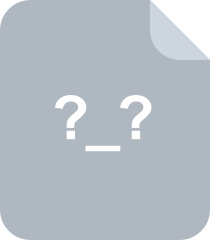
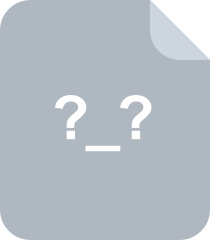
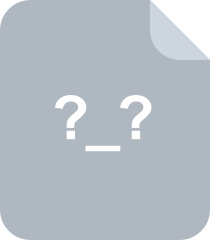
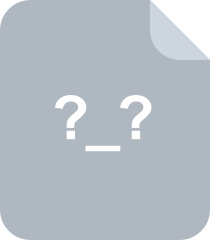
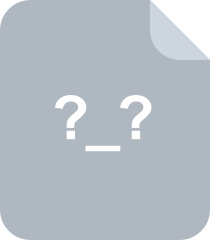
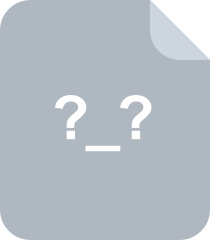
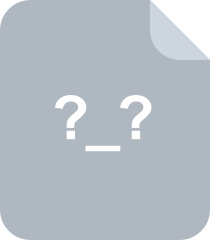
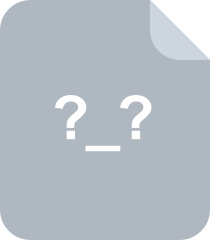
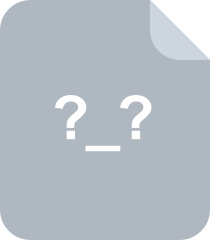
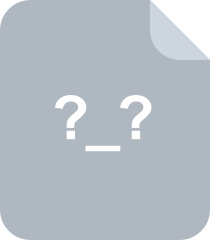
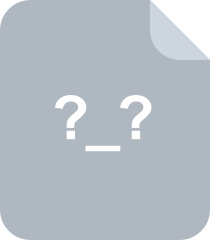
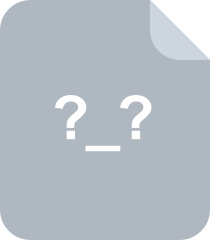
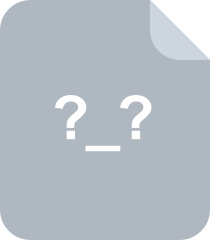
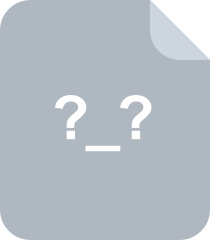
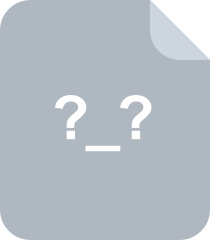
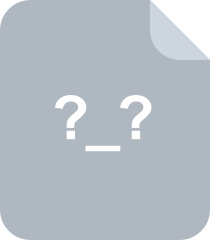
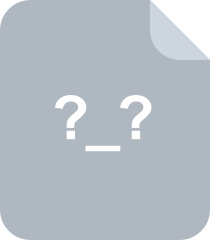
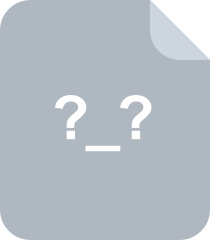
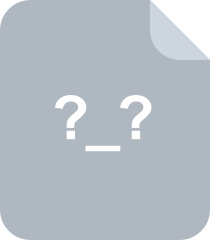
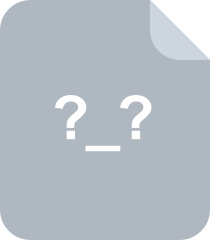
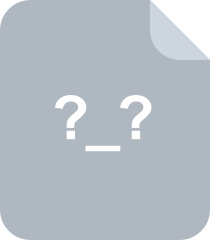
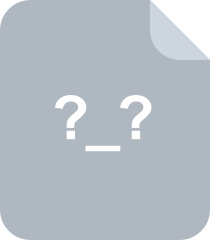
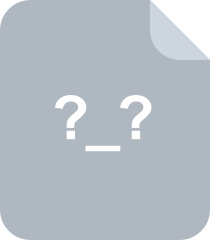
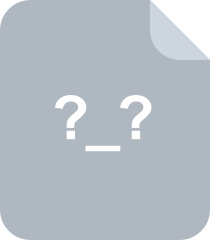
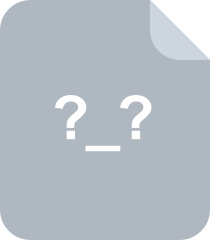
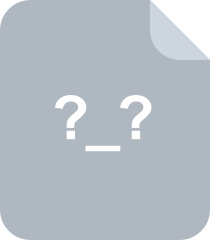
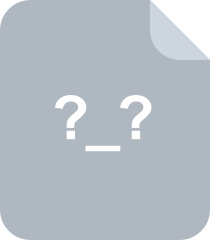
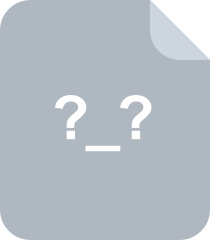
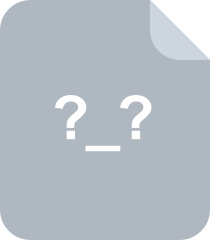
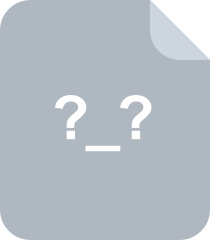
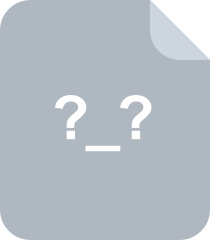
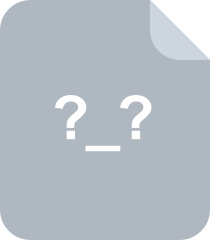
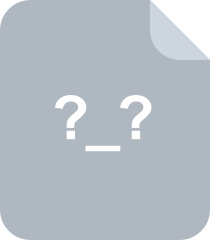
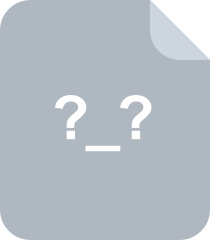
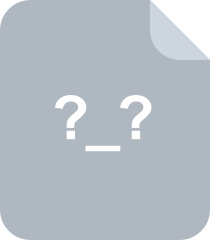
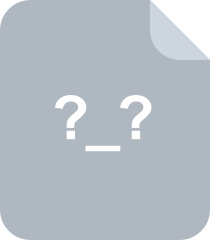
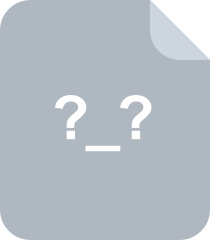
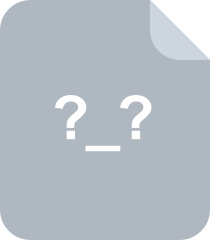
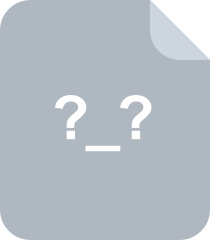
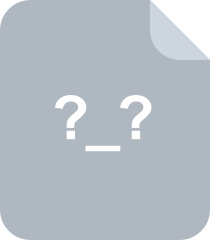
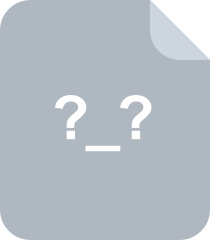
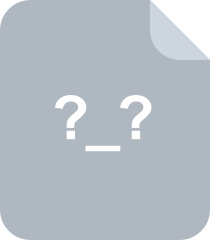
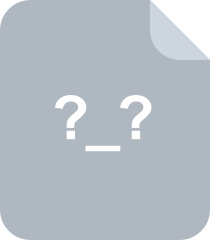
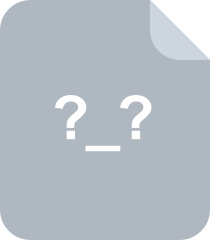
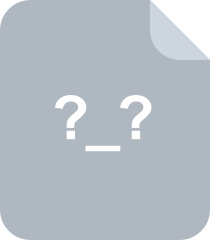
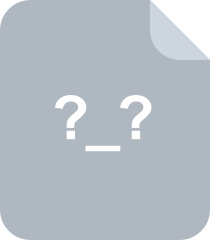
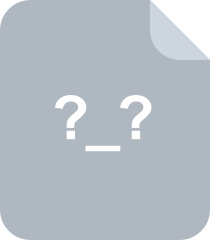
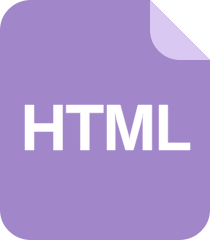
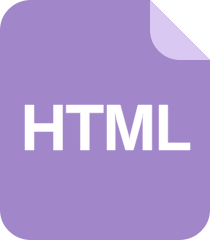
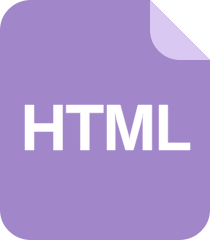
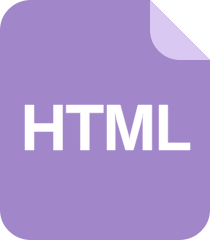
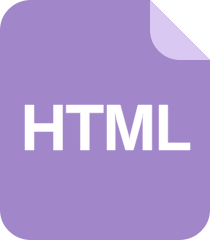
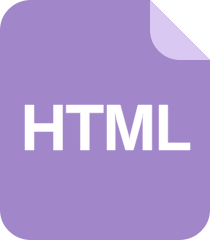
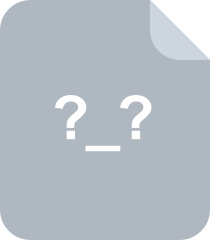
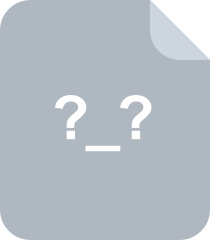
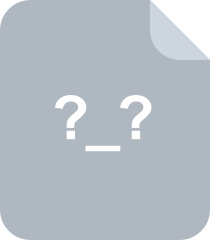
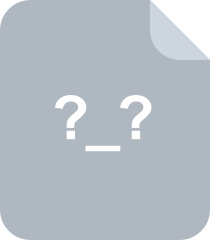
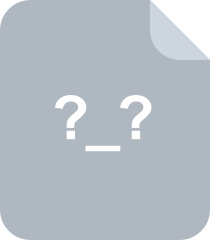
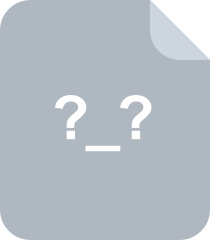
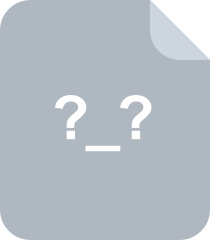
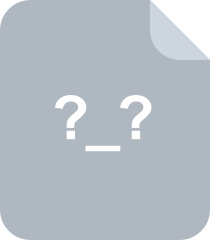
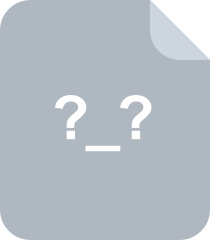
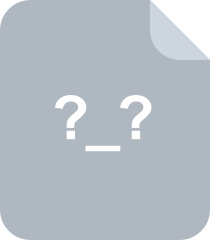
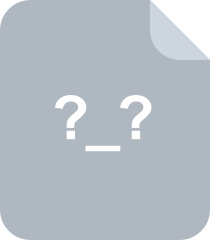
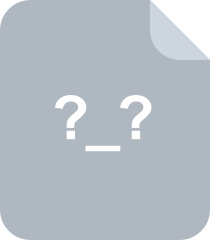
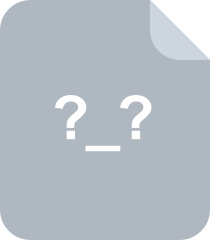
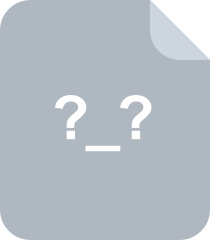
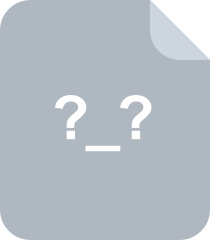
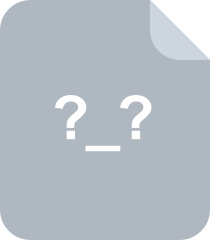
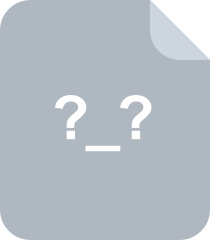
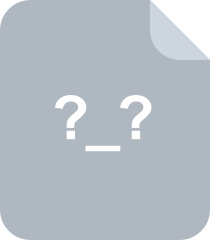
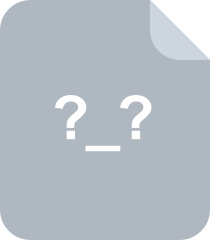
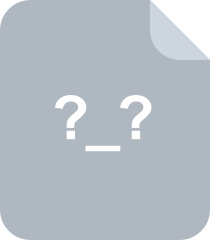
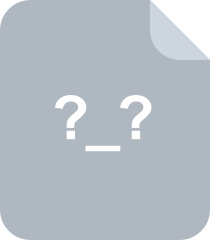
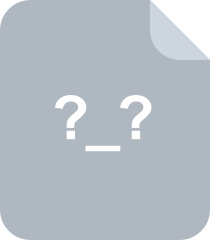
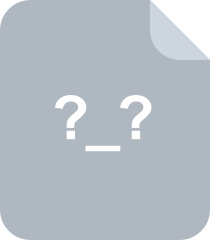
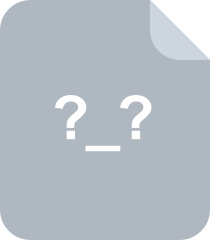
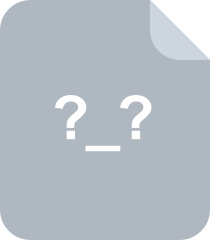
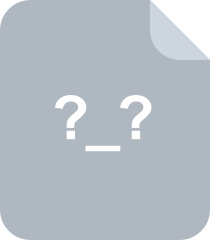
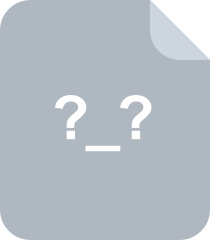
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
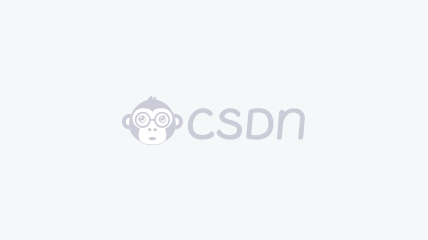

沐知全栈开发
- 粉丝: 5810
- 资源: 5218
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

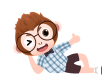
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


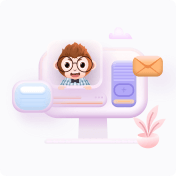
安全验证
文档复制为VIP权益,开通VIP直接复制
