/*
* Datart
* <p>
* Copyright 2021
* <p>
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package datart.server.service.impl;
import datart.core.base.consts.Const;
import datart.core.base.consts.FileOwner;
import datart.core.base.exception.Exceptions;
import datart.core.base.exception.NotFoundException;
import datart.core.base.exception.ParamException;
import datart.core.common.DateUtils;
import datart.core.common.FileUtils;
import datart.core.common.UUIDGenerator;
import datart.core.entity.*;
import datart.core.mappers.ext.*;
import datart.security.base.ResourceType;
import datart.security.util.PermissionHelper;
import datart.server.base.dto.DashboardBaseInfo;
import datart.server.base.dto.DashboardDetail;
import datart.server.base.dto.WidgetDetail;
import datart.server.base.params.*;
import datart.server.base.transfer.ImportStrategy;
import datart.server.base.transfer.TransferConfig;
import datart.server.base.transfer.model.DashboardResourceModel;
import datart.server.base.transfer.model.DashboardTemplateModel;
import datart.server.service.*;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.BeanUtils;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.FileSystemUtils;
import java.io.File;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
@Slf4j
@Service
public class DashboardServiceImpl extends BaseService implements DashboardService {
private final DashboardMapperExt dashboardMapper;
private final WidgetMapperExt widgetMapper;
private final RelWidgetElementMapperExt rweMapper;
private final RelWidgetWidgetMapperExt rwwMapper;
private final RelRoleResourceMapperExt rrrMapper;
private final RoleService roleService;
private final FileService fileService;
private final FolderMapperExt folderMapper;
private final ViewMapperExt viewMapper;
private final DatachartMapperExt datachartMapper;
private final WidgetService widgetService;
private final FolderService folderService;
private final VariableService variableService;
private final ViewService viewService;
private final DatachartService datachartService;
public DashboardServiceImpl(DashboardMapperExt dashboardMapper,
WidgetMapperExt widgetMapper,
RelWidgetElementMapperExt rweMapper,
RelWidgetWidgetMapperExt rwwMapper,
RelRoleResourceMapperExt rrrMapper, RoleService roleService,
FileService fileService,
FolderMapperExt folderMapper,
ViewMapperExt viewMapper,
DatachartMapperExt datachartMapper,
WidgetService widgetService,
FolderService folderService,
VariableService variableService,
ViewService viewService,
DatachartService datachartService) {
this.dashboardMapper = dashboardMapper;
this.widgetMapper = widgetMapper;
this.rweMapper = rweMapper;
this.rwwMapper = rwwMapper;
this.rrrMapper = rrrMapper;
this.roleService = roleService;
this.fileService = fileService;
this.folderMapper = folderMapper;
this.viewMapper = viewMapper;
this.datachartMapper = datachartMapper;
this.widgetService = widgetService;
this.folderService = folderService;
this.variableService = variableService;
this.viewService = viewService;
this.datachartService = datachartService;
}
@Override
public List<DashboardBaseInfo> listDashboard(String orgId) {
List<Dashboard> dashboards = dashboardMapper.listByOrgId(orgId);
return dashboards.stream().filter(dashboard -> securityManager
.hasPermission(PermissionHelper.vizPermission(dashboard.getOrgId(), "*", dashboard.getId(), Const.READ)))
.map(DashboardBaseInfo::new)
.collect(Collectors.toList());
}
@Override
@Transactional
public boolean delete(String dashboardId) {
//remove from folder
DashboardService.super.delete(dashboardId);
return 1 == folderMapper.deleteByRelTypeAndId(ResourceType.DASHBOARD.name(), dashboardId);
}
@Override
public void deleteReference(Dashboard dashboard) {
dashboardMapper.deleteDashboard(dashboard.getId());
}
@Override
@Transactional
public boolean archive(String id) {
DashboardService.super.archive(id);
//remove from folder
return folderMapper.deleteByRelTypeAndId(ResourceType.DASHBOARD.name(), id) == 1;
}
@Override
public DashboardDetail getDashboardDetail(String dashboardId) {
Dashboard dashboard = retrieve(dashboardId);
DashboardDetail dashboardDetail = new DashboardDetail();
BeanUtils.copyProperties(dashboard, dashboardDetail);
//folder index
Folder folder = folderMapper.selectByRelTypeAndId(ResourceType.DASHBOARD.name(), dashboardId);
if (folder != null) {
dashboardDetail.setParentId(folder.getParentId());
dashboardDetail.setIndex(folder.getIndex());
}
Set<String> viewIds = new HashSet<>();
Set<String> datachartIds = new HashSet<>();
// get all widgets details
List<WidgetDetail> widgetDetails = getWidgets(dashboardId, datachartIds, viewIds);
dashboardDetail.setWidgets(widgetDetails);
// charts
if (!CollectionUtils.isEmpty(datachartIds)) {
dashboardDetail.setDatacharts(datachartMapper.listByIds(datachartIds));
} else {
dashboardDetail.setDatacharts(Collections.emptyList());
}
//views
List<String> chartViews = dashboardDetail.getDatacharts().stream().map(Datachart::getViewId).collect(Collectors.toList());
viewIds.addAll(chartViews);
if (!CollectionUtils.isEmpty(viewIds)) {
dashboardDetail.setViews(viewMapper.listByIds(viewIds));
} else {
dashboardDetail.setViews(Collections.emptyList());
}
//variables
LinkedList<Variable> variables = new LinkedList<>(variableService.listOrgQueryVariables(dashboard.getOrgId()));
if (!CollectionUtils.isEmpty(viewIds)) {
for (String viewId : viewIds) {
variables.addAll(variableService.listViewQueryVariables(viewId));
}
}
dashboardDetail.setQueryVariables(variables);
// download permission
dashboardDetail.setDownload(securityManager.hasPermission(PermissionHelper.vizPermission(dashboard.getOrgId(), "*", dashboardId, Const.DOWNLOAD)));
return dashboardDetail;
}
@Override
public Folder copyDashboard(DashboardCreateParam dashboard) throws IOException {
Folder folder = createWithFolder(dashboard);
DashboardDetail copy = getDashboardDetail(dashboard.getId());
BeanUtils.copyProperties(dashboard, copy);
// copy database records
if (CollectionUtils.isNotEmpty(copy.getWidgets())) {

沐知全栈开发
- 粉丝: 5817
- 资源: 5226
最新资源
- 基于协同过滤算法的东北特产销售系统的实现--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于SpringBoot的私房菜定制上门服务系统的设计与实现pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于协同过滤算法的私人诊所管理系统_6t4o8--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于springboot的实习管理系统-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于协同过滤算法的体育商品推荐系统_t81xg--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于协同过滤算法的黔醉酒业白酒销售系统_p091v--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于springboot的网购平台管理系统_0q1i3--论文-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于疫情防控管理系统的数据可视化分析与实现_3mkgh-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于SpringBoot的网络海鲜市场系统的设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 基于springboot的乡村政务办公系统的设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于协同过滤算法商品推荐系统pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于SpringBoot的小学生身体素质测评管理系统设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 基于Springboot的小区疫情购物系统录pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 抽水蓄能电站的最佳调度方案研究 参考文献:抽水蓄能电站的最佳调度方案研究 非完全复献 matlab?粒子群算法 主要内容:研究抽水蓄能机组调峰填谷的功能,目标是从电网的利益出发,结合抽水蓄能电站的运行
- 基于springboot的校园失物招领系统--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于SpringBoot的学生网上选课系统--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


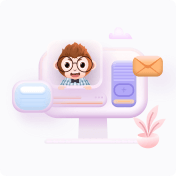