<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006~2021 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: liu21st <liu21st@gmail.com>
// +----------------------------------------------------------------------
declare (strict_types = 1);
namespace think;
use ArrayAccess;
use think\file\UploadedFile;
use think\route\Rule;
/**
* 请求管理类
* @package think
*/
class Request implements ArrayAccess
{
/**
* 兼容PATH_INFO获取
* @var array
*/
protected $pathinfoFetch = ['ORIG_PATH_INFO', 'REDIRECT_PATH_INFO', 'REDIRECT_URL'];
/**
* PATHINFO变量名 用于兼容模式
* @var string
*/
protected $varPathinfo = 's';
/**
* 请求类型
* @var string
*/
protected $varMethod = '_method';
/**
* 表单ajax伪装变量
* @var string
*/
protected $varAjax = '_ajax';
/**
* 表单pjax伪装变量
* @var string
*/
protected $varPjax = '_pjax';
/**
* 域名根
* @var string
*/
protected $rootDomain = '';
/**
* HTTPS代理标识
* @var string
*/
protected $httpsAgentName = '';
/**
* 前端代理服务器IP
* @var array
*/
protected $proxyServerIp = [];
/**
* 前端代理服务器真实IP头
* @var array
*/
protected $proxyServerIpHeader = ['HTTP_X_REAL_IP', 'HTTP_X_FORWARDED_FOR', 'HTTP_CLIENT_IP', 'HTTP_X_CLIENT_IP', 'HTTP_X_CLUSTER_CLIENT_IP'];
/**
* 请求类型
* @var string
*/
protected $method;
/**
* 域名(含协议及端口)
* @var string
*/
protected $domain;
/**
* HOST(含端口)
* @var string
*/
protected $host;
/**
* 子域名
* @var string
*/
protected $subDomain;
/**
* 泛域名
* @var string
*/
protected $panDomain;
/**
* 当前URL地址
* @var string
*/
protected $url;
/**
* 基础URL
* @var string
*/
protected $baseUrl;
/**
* 当前执行的文件
* @var string
*/
protected $baseFile;
/**
* 访问的ROOT地址
* @var string
*/
protected $root;
/**
* pathinfo
* @var string
*/
protected $pathinfo;
/**
* pathinfo(不含后缀)
* @var string
*/
protected $path;
/**
* 当前请求的IP地址
* @var string
*/
protected $realIP;
/**
* 当前控制器名
* @var string
*/
protected $controller;
/**
* 当前操作名
* @var string
*/
protected $action;
/**
* 当前请求参数
* @var array
*/
protected $param = [];
/**
* 当前GET参数
* @var array
*/
protected $get = [];
/**
* 当前POST参数
* @var array
*/
protected $post = [];
/**
* 当前REQUEST参数
* @var array
*/
protected $request = [];
/**
* 当前路由对象
* @var Rule
*/
protected $rule;
/**
* 当前ROUTE参数
* @var array
*/
protected $route = [];
/**
* 中间件传递的参数
* @var array
*/
protected $middleware = [];
/**
* 当前PUT参数
* @var array
*/
protected $put;
/**
* SESSION对象
* @var Session
*/
protected $session;
/**
* COOKIE数据
* @var array
*/
protected $cookie = [];
/**
* ENV对象
* @var Env
*/
protected $env;
/**
* 当前SERVER参数
* @var array
*/
protected $server = [];
/**
* 当前FILE参数
* @var array
*/
protected $file = [];
/**
* 当前HEADER参数
* @var array
*/
protected $header = [];
/**
* 资源类型定义
* @var array
*/
protected $mimeType = [
'xml' => 'application/xml,text/xml,application/x-xml',
'json' => 'application/json,text/x-json,application/jsonrequest,text/json',
'js' => 'text/javascript,application/javascript,application/x-javascript',
'css' => 'text/css',
'rss' => 'application/rss+xml',
'yaml' => 'application/x-yaml,text/yaml',
'atom' => 'application/atom+xml',
'pdf' => 'application/pdf',
'text' => 'text/plain',
'image' => 'image/png,image/jpg,image/jpeg,image/pjpeg,image/gif,image/webp,image/*',
'csv' => 'text/csv',
'html' => 'text/html,application/xhtml+xml,*/*',
];
/**
* 当前请求内容
* @var string
*/
protected $content;
/**
* 全局过滤规则
* @var array
*/
protected $filter;
/**
* php://input内容
* @var string
*/
// php://input
protected $input;
/**
* 请求安全Key
* @var string
*/
protected $secureKey;
/**
* 是否合并Param
* @var bool
*/
protected $mergeParam = false;
/**
* 架构函数
* @access public
*/
public function __construct()
{
// 保存 php://input
$this->input = file_get_contents('php://input');
}
public static function __make(App $app)
{
$request = new static();
if (function_exists('apache_request_headers') && $result = apache_request_headers()) {
$header = $result;
} else {
$header = [];
$server = $_SERVER;
foreach ($server as $key => $val) {
if (0 === strpos($key, 'HTTP_')) {
$key = str_replace('_', '-', strtolower(substr($key, 5)));
$header[$key] = $val;
}
}
if (isset($server['CONTENT_TYPE'])) {
$header['content-type'] = $server['CONTENT_TYPE'];
}
if (isset($server['CONTENT_LENGTH'])) {
$header['content-length'] = $server['CONTENT_LENGTH'];
}
}
$request->header = array_change_key_case($header);
$request->server = $_SERVER;
$request->env = $app->env;
$inputData = $request->getInputData($request->input);
$request->get = $_GET;
$request->post = $_POST ?: $inputData;
$request->put = $inputData;
$request->request = $_REQUEST;
$request->cookie = $_COOKIE;
$request->file = $_FILES ?? [];
return $request;
}
/**
* 设置当前包含协议的域名
* @access public
* @param string $domain 域名
* @return $this
*/
public function setDomain(string $domain)
{
$this->domain = $domain;
return $this;
}
/**
* 获取当前包含协议的域名
* @access public
* @param bool $port 是否需要去除端口号
* @return string
*/
public function domain(bool $port = false): string
{
return $this->scheme() . '://' . $this->host($port);
}
/**
* 获取当前根域名
* @access public
* @return string
*/
public function rootDomain(): string
{
$root = $this->rootDomain;
if (!$root) {
$item = explode('.', $this->host());
$count = count($item);
$root = $count > 1 ? $item[$count - 2] . '.' . $item[$count - 1] : $item[0];
}
return $root;
}
/**
* 设置当前泛域名的值
* @access public
* @param string $domain 域名
* @return $this
*/
没有合适的资源?快使用搜索试试~ 我知道了~
基于ThinkPHP6.0的博客网站设计源码
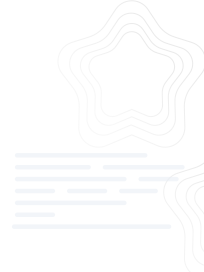
共1201个文件
php:499个
gif:174个
js:144个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 23 浏览量
2024-04-07
10:54:53
上传
评论
收藏 24.54MB ZIP 举报
温馨提示
本项目是一个基于ThinkPHP6.0语言开发的博客网站,包含1196个文件,主要文件类型包括PHP源代码、图片、JavaScript脚本、CSS样式表、HTML页面、JSON配置文件、Markdown文档和Git忽略文件。系统设计旨在为用户提供一个高效、便捷的博客发布和浏览平台,支持多种文件类型的上传和展示,以满足用户在内容发布和阅读方面的需求。
资源推荐
资源详情
资源评论
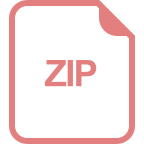
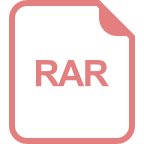
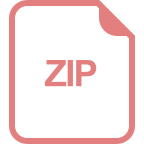
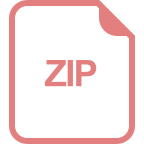
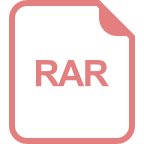
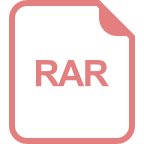
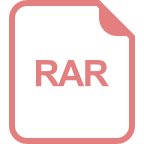
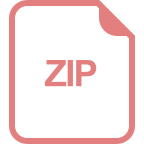
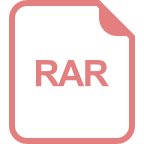
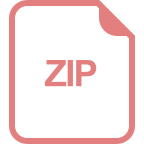
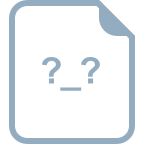
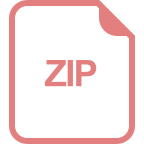
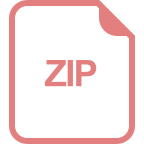
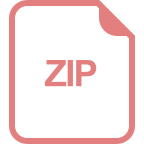
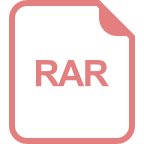
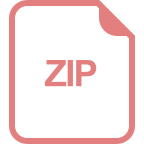
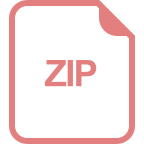
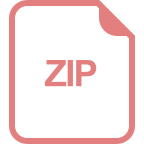
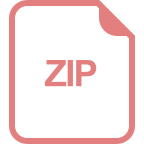
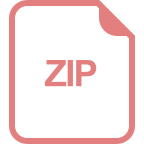
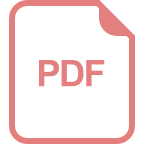
收起资源包目录

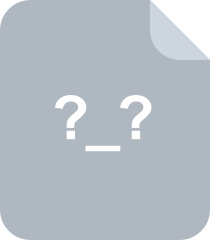
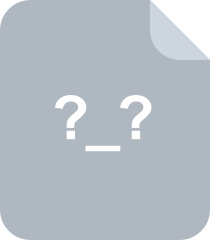
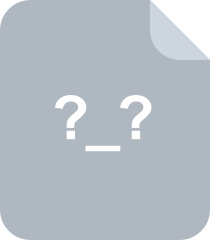
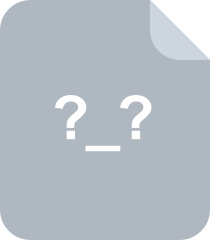
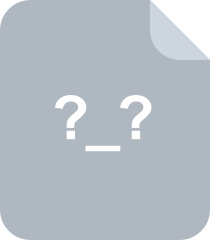
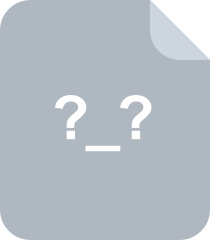
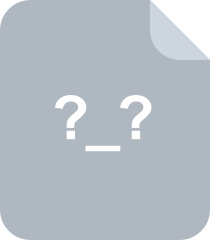
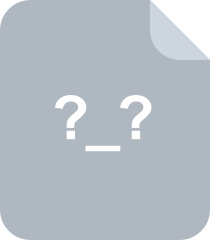
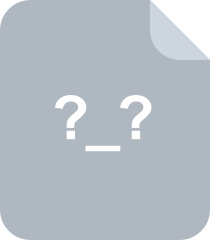
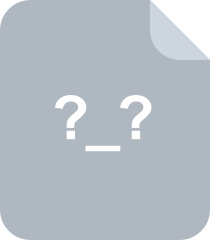
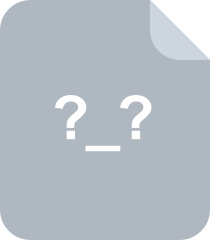
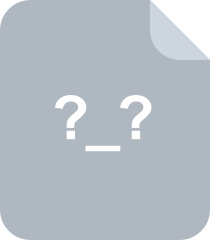
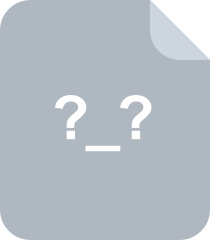
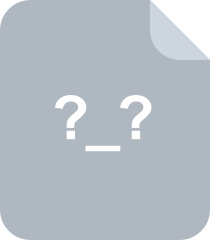
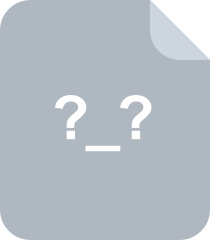
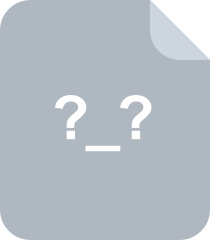
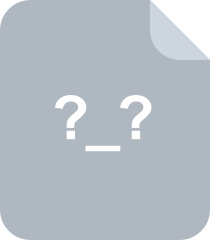
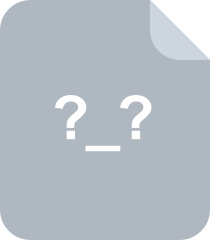
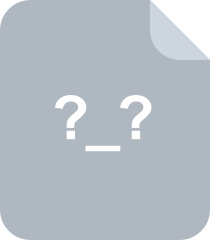
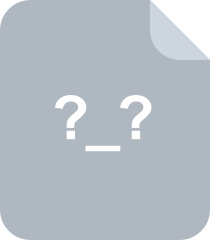
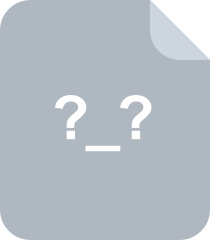
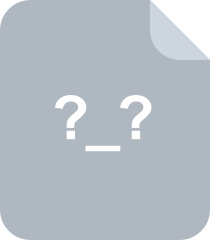
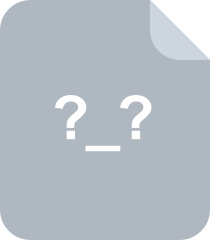
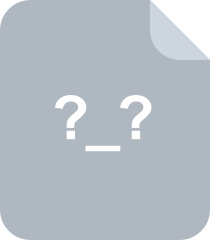
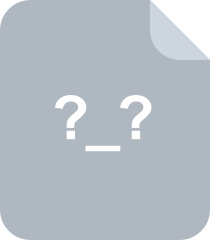
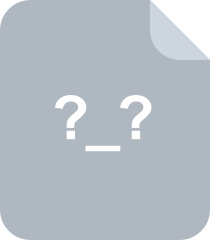
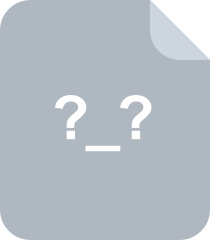
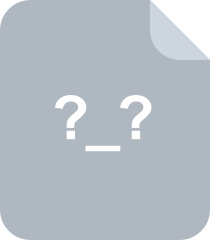
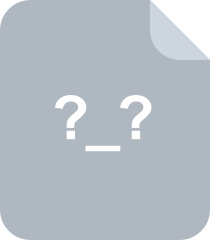
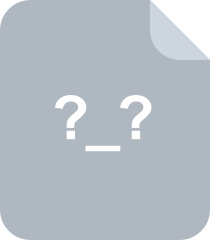
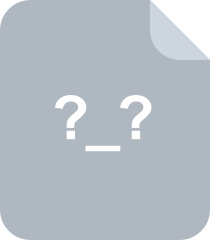
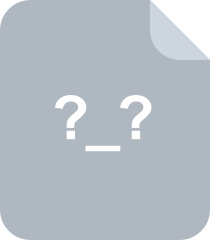
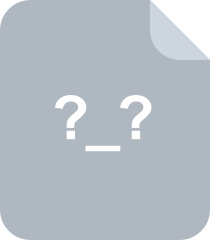
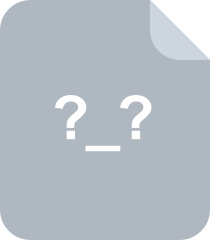
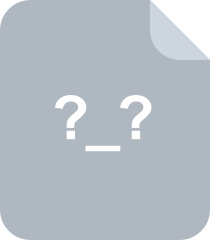
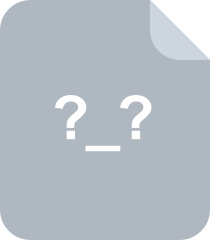
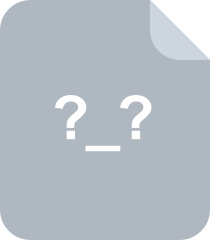
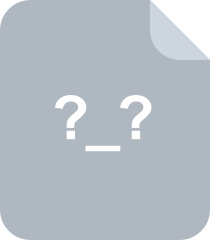
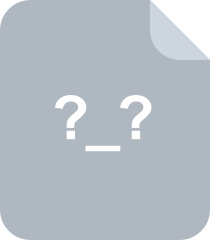
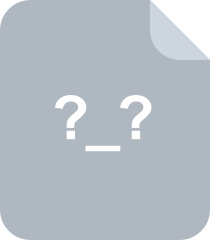
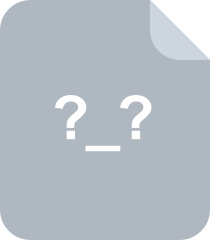
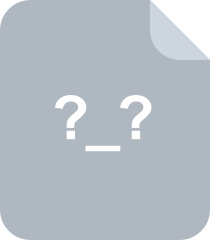
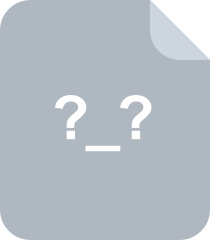
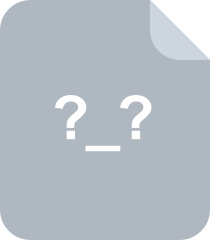
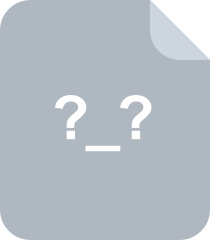
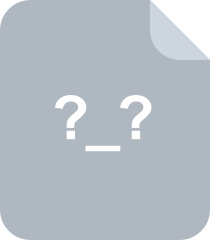
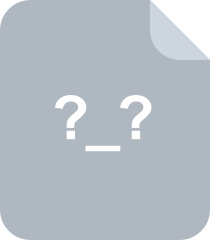
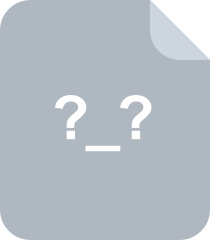
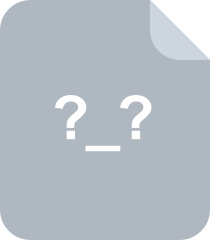
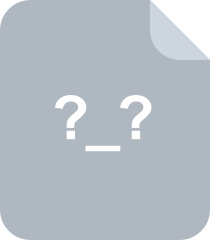
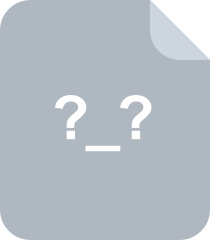
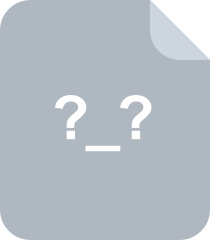
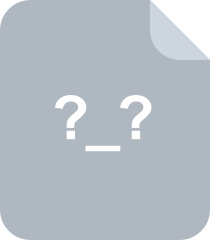
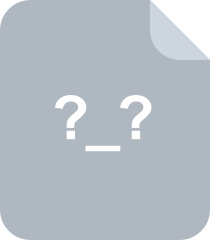
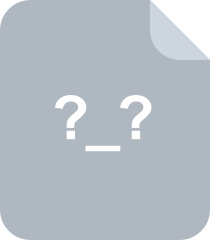
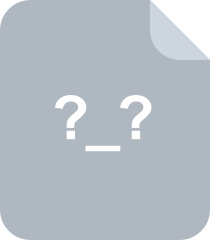
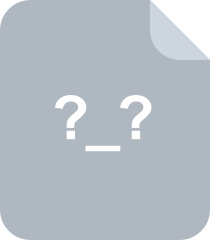
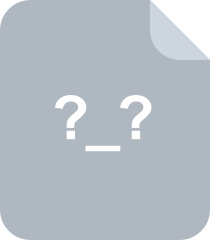
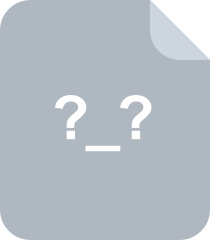
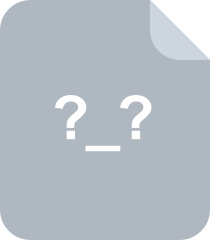
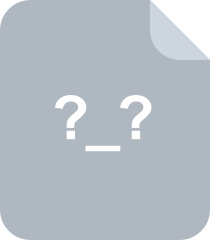
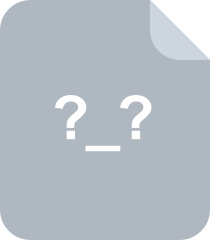
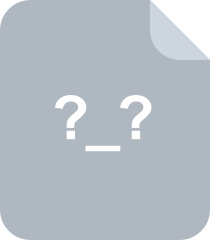
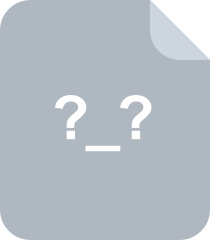
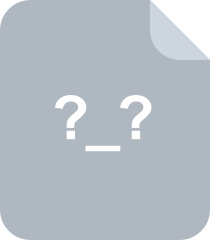
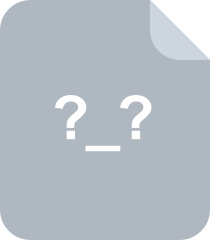
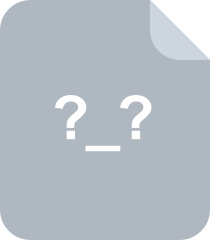
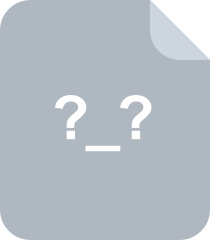
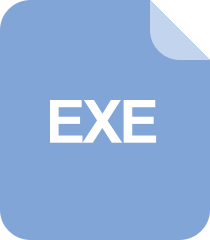
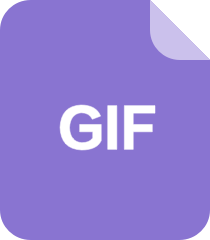
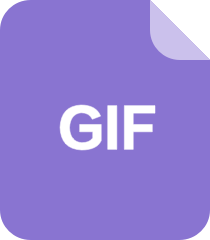
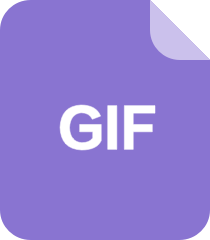
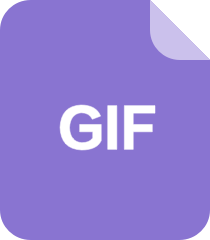
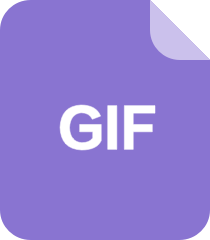
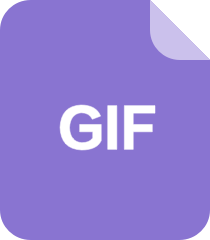
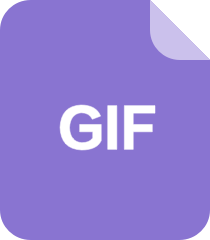
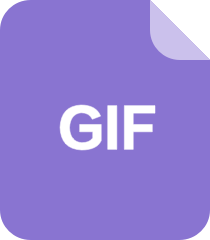
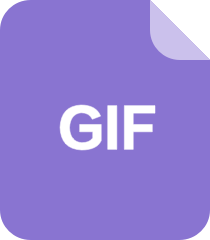
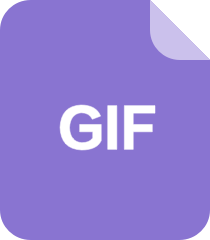
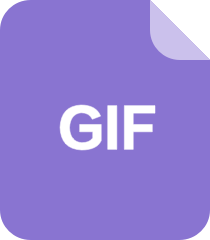
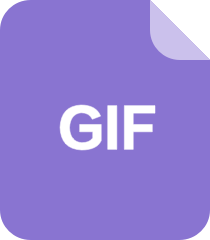
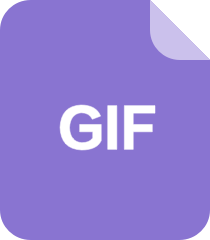
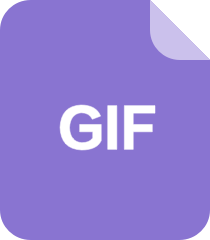
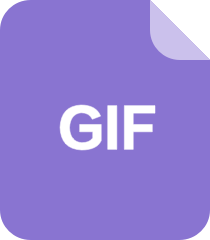
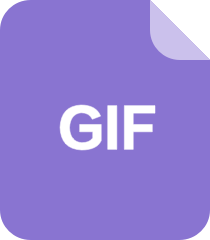
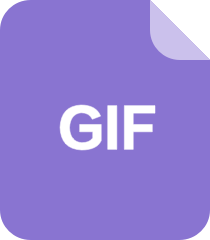
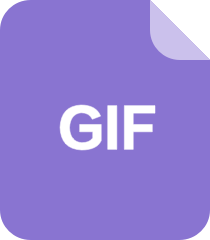
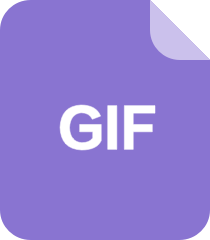
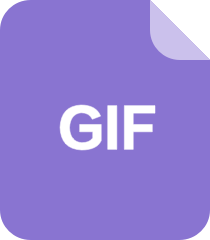
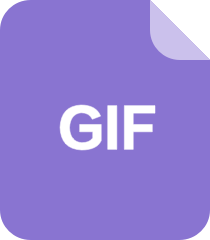
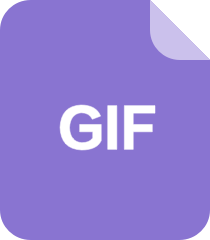
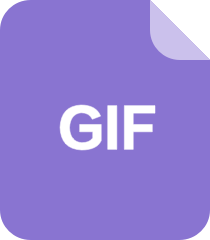
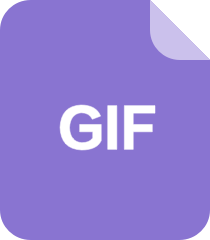
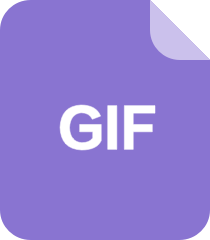
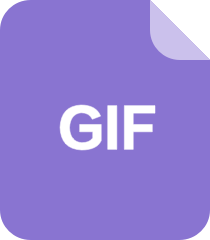
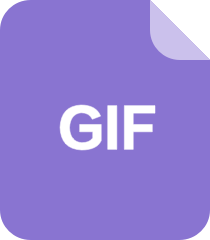
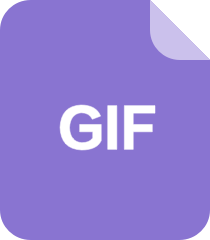
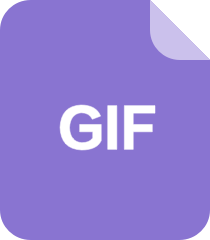
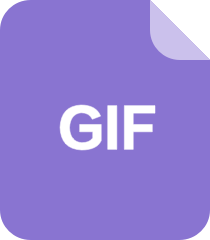
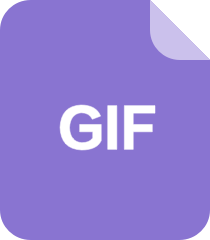
共 1201 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
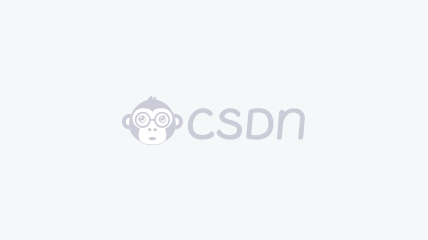

沐知全栈开发
- 粉丝: 5701
- 资源: 5216
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

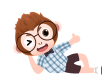
最新资源
- vue3和ue5.3进行通信
- java银行帐目管理系统(源代码+论文).zip
- 2003-2020年中国31省对外直接投资流量数据全集:各省OFDI流量详录-最新出炉.zip
- javaweb-shanyu01项目web文件夹
- 中国品牌日研究特辑-数字经济时代下中国品牌高质量发展之用户趋势.pdf
- im即时通讯app软件开发语音海外社交聊天视频交友app群聊搭建源码
- 2024-2025年全球客户体验卓越报告:超越喧嚣借力AI打造卓越客户体验.pdf
- minio arm64 docker镜像包
- 中文大模型基准测评2024年10月报告-2024年度中文大模型阶段性进展评估.pdf
- 使用 AWR 进行 Exadata 性能诊断
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


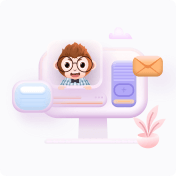
安全验证
文档复制为VIP权益,开通VIP直接复制
