<?php
class TP_yyToken implements ArrayAccess
{
public $string = '';
public $metadata = array();
public function __construct($s, $m = array())
{
if ($s instanceof TP_yyToken) {
$this->string = $s->string;
$this->metadata = $s->metadata;
} else {
$this->string = (string) $s;
if ($m instanceof TP_yyToken) {
$this->metadata = $m->metadata;
} elseif (is_array($m)) {
$this->metadata = $m;
}
}
}
public function __toString()
{
return $this->string;
}
public function offsetExists($offset)
{
return isset($this->metadata[ $offset ]);
}
public function offsetGet($offset)
{
return $this->metadata[ $offset ];
}
public function offsetSet($offset, $value)
{
if ($offset === null) {
if (isset($value[ 0 ])) {
$x = ($value instanceof TP_yyToken) ? $value->metadata : $value;
$this->metadata = array_merge($this->metadata, $x);
return;
}
$offset = count($this->metadata);
}
if ($value === null) {
return;
}
if ($value instanceof TP_yyToken) {
if ($value->metadata) {
$this->metadata[ $offset ] = $value->metadata;
}
} elseif ($value) {
$this->metadata[ $offset ] = $value;
}
}
public function offsetUnset($offset)
{
unset($this->metadata[ $offset ]);
}
}
class TP_yyStackEntry
{
public $stateno; /* The state-number */
public $major; /* The major token value. This is the code
** number for the token at this stack level */
public $minor; /* The user-supplied minor token value. This
** is the value of the token */
}
;
#line 11 "../smarty/lexer/smarty_internal_templateparser.y"
/**
* Smarty Template Parser Class
*
* This is the template parser.
* It is generated from the smarty_internal_templateparser.y file
*
* @author Uwe Tews <uwe.tews@googlemail.com>
*/
class Smarty_Internal_Templateparser
{
#line 23 "../smarty/lexer/smarty_internal_templateparser.y"
const Err1 = "Security error: Call to private object member not allowed";
const Err2 = "Security error: Call to dynamic object member not allowed";
const Err3 = "PHP in template not allowed. Use SmartyBC to enable it";
/**
* result status
*
* @var bool
*/
public $successful = true;
/**
* return value
*
* @var mixed
*/
public $retvalue = 0;
/**
* @var
*/
public $yymajor;
/**
* last index of array variable
*
* @var mixed
*/
public $last_index;
/**
* last variable name
*
* @var string
*/
public $last_variable;
/**
* root parse tree buffer
*
* @var Smarty_Internal_ParseTree
*/
public $root_buffer;
/**
* current parse tree object
*
* @var Smarty_Internal_ParseTree
*/
public $current_buffer;
/**
* lexer object
*
* @var Smarty_Internal_Templatelexer
*/
public $lex;
/**
* internal error flag
*
* @var bool
*/
private $internalError = false;
/**
* {strip} status
*
* @var bool
*/
public $strip = false;
/**
* compiler object
*
* @var Smarty_Internal_TemplateCompilerBase
*/
public $compiler = null;
/**
* smarty object
*
* @var Smarty
*/
public $smarty = null;
/**
* template object
*
* @var Smarty_Internal_Template
*/
public $template = null;
/**
* block nesting level
*
* @var int
*/
public $block_nesting_level = 0;
/**
* security object
*
* @var Smarty_Security
*/
public $security = null;
/**
* template prefix array
*
* @var \Smarty_Internal_ParseTree[]
*/
public $template_prefix = array();
/**
* security object
*
* @var \Smarty_Internal_ParseTree[]
*/
public $template_postfix = array();
/**
* constructor
*
* @param Smarty_Internal_Templatelexer $lex
* @param Smarty_Internal_TemplateCompilerBase $compiler
*/
function __construct(Smarty_Internal_Templatelexer $lex, Smarty_Internal_TemplateCompilerBase $compiler)
{
$this->lex = $lex;
$this->compiler = $compiler;
$this->template = $this->compiler->template;
$this->smarty = $this->template->smarty;
$this->security = isset($this->smarty->security_policy) ? $this->smarty->security_policy : false;
$this->current_buffer = $this->root_buffer = new Smarty_Internal_ParseTree_Template();
}
/**
* insert PHP code in current buffer
*
* @param string $code
*/
public function insertPhpCode($code)
{
$this->current_buffer->append_subtree($this, new Smarty_Internal_ParseTree_Tag($this, $code));
}
/**
* merge PHP code with prefix code and return parse tree tag object
*
* @param string $code
*
* @return Smarty_Internal_ParseTree_Tag
*/
public function mergePrefixCode($code)
{
$tmp = '';
foreach ($this->compiler->prefix_code as $preCode) {
$tmp .= $preCode;
}
$this->compiler->prefix_code = array();
$tmp .= $code;
return new Smarty_Internal_ParseTree_Tag($this, $this->compiler->processNocacheCode($tmp, true));
}
const TP_VERT = 1;
const TP_COLON = 2;
const TP_PHP = 3;
const TP_NOCACHE = 4;
const TP_TEXT = 5;
const TP_STRIPON = 6;
const TP_STRIPOFF = 7;
const TP_LITERALSTART = 8;
const TP_LITERALEND = 9;
const TP_LITERAL = 10;
const TP_RDEL = 11;
const TP_SIMPELOUTPUT = 12;
const TP_LDEL = 13;
const TP_DOLLARID = 14;
const TP_EQUAL = 15;
const TP_SIMPLETAG = 16;
const TP_ID = 17;
const TP_PTR = 18;
const TP_LDELMAKENOCACHE = 19;
const TP_LDELIF = 20;
const TP_LDELFOR = 21;
const TP_SEMICOLON = 22;
const TP_INCDEC = 23;
const TP_TO = 24;
const TP_STEP = 25;
const TP_LDELFOREACH = 26;
const TP_SPACE = 27;
const TP_AS = 28;
const TP_APTR = 29;
const TP_LDELSETFILTER = 30;
const TP_SMARTYBLOCKCHILDPARENT = 31;
const TP_CLOSETAG = 32;
const TP_LDELSLASH = 33;
const TP_ATTR = 34;
const TP_INTEGER = 35;
const TP_COMMA = 36;
const TP_OPENP = 37;
const TP_CLOSEP = 38;
const TP_MATH = 39;
const TP_UNIMATH = 40;
const TP_ISIN = 41;
const TP_QMARK = 42;
const TP_NOT = 43;
const TP_TYPECAST = 44;
const TP_HEX = 45;
const TP_DOT = 46;
const TP_INSTANCEOF = 47;
const TP_SINGLEQUOTESTRING = 48;
const TP_DOUBLECOLON = 49;
const TP_NAMESPACE = 50;
const TP_AT = 51;
const TP_HATCH = 52;
const TP_OPENB = 53;
const TP_CLOSEB = 54;
const TP_DOLLAR = 55;
const TP_LOGOP = 56;
const TP_SLOGOP = 57;
const TP_TLOGOP = 58;
const TP_SINGLECOND = 59;
const TP_QUOTE = 60;
const TP_BACKTICK = 61;
const YY_NO_ACTION = 534;
const YY_ACCEPT_ACTION = 533;
const YY_ERROR_ACTION = 532;
const YY_SZ_ACTTAB = 2017;
static public $yy_action = array(269, 8, 133, 295, 335, 80, 282, 219, 7, 84, 128, 178, 255, 276, 113, 102, 13, 83,
227, 286, 305, 220, 36, 223, 283, 21, 32, 297, 41, 14, 90, 40, 44, 260, 213, 231,
250, 235, 210, 128, 81, 1, 298, 296, 102, 269, 8, 132, 79, 335, 196, 184, 219, 7,
84, 26, 297, 461, 101, 113, 39, 24, 278, 227, 461, 305, 220, 171, 206, 222, 2
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本设计源码提供了一个基于PHP的IDEMaker开源订餐系统。项目包含2216个文件,主要使用PHP、HTML、JavaScript、CSS和Shell编程语言。文件类型包括1383个PHP源代码文件、136个PHPT配置文件、129个PNG图片文件、92个HTML页面文件、89个JPG图片文件、51个JavaScript脚本文件、46个CSS样式文件、45个YAML配置文件、44个Markdown文档、44个XML配置文件。该系统是一个B2B2C商业化的开源项目平台,适合用于学习和实践PHP技术,以及开发订餐相关的网站应用。
资源推荐
资源详情
资源评论
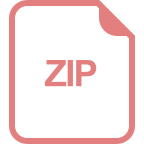
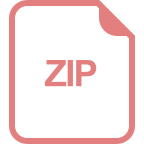
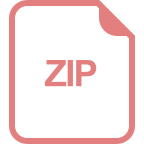
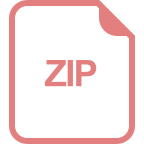
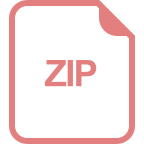
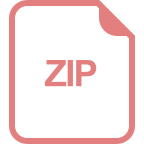
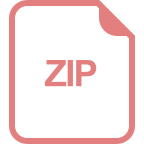
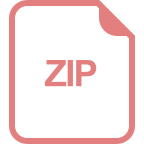
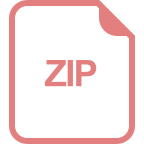
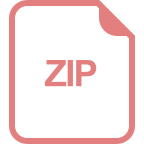
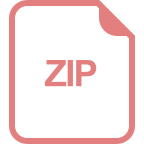
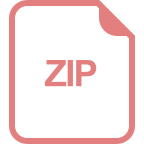
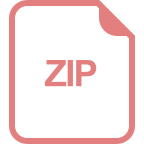
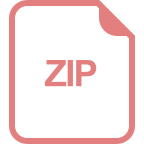
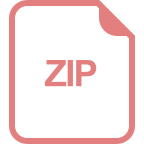
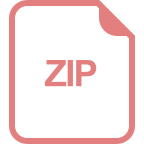
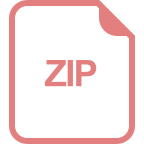
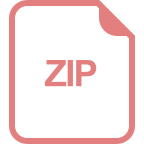
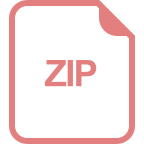
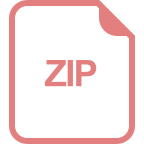
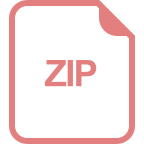
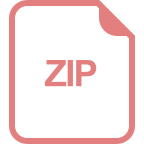
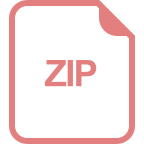
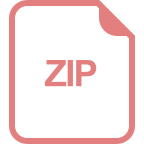
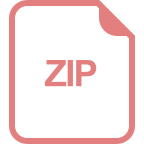
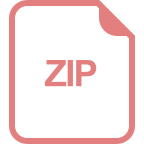
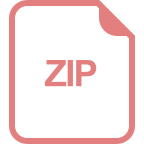
收起资源包目录

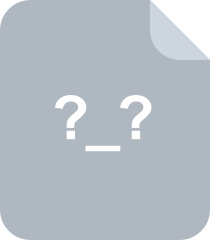
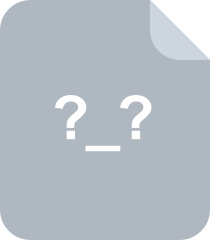
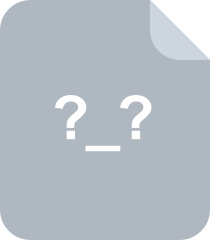
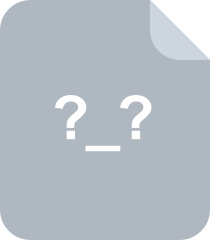
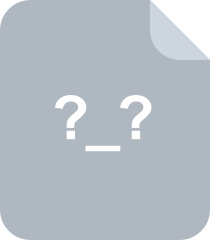
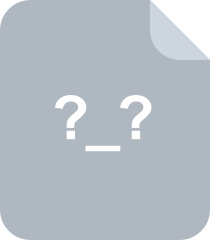
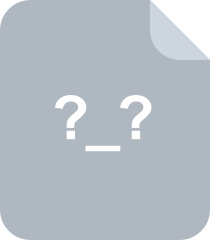
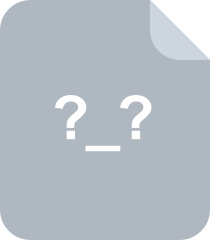
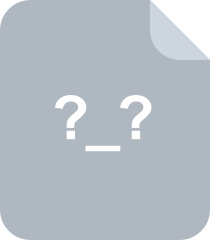
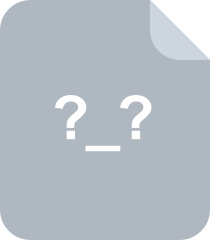
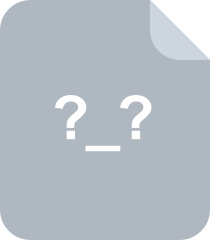
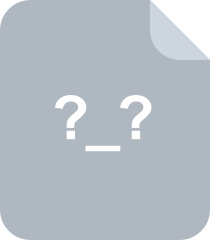
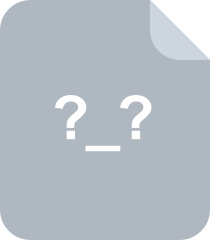
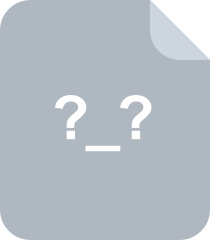
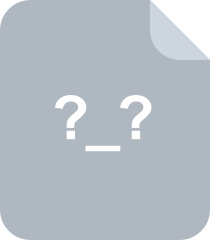
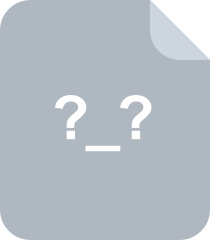
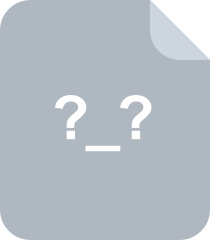
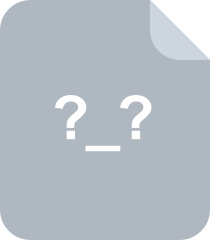
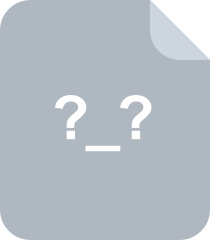
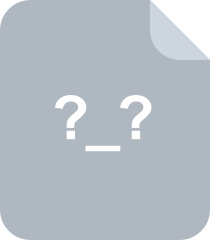
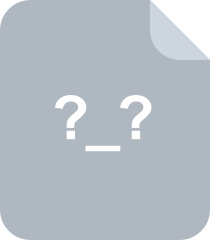
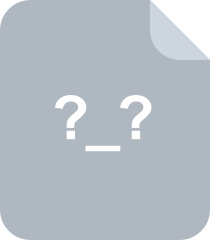
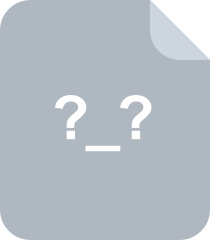
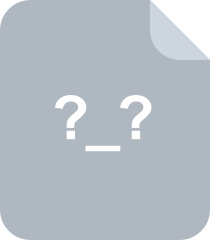
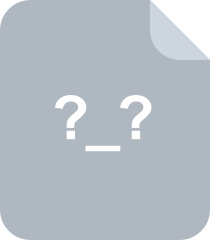
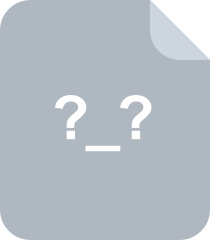
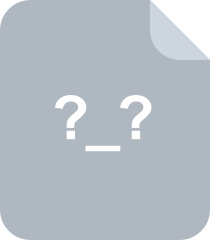
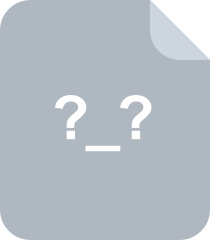
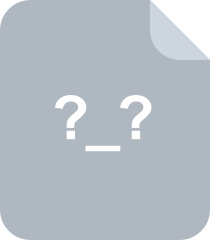
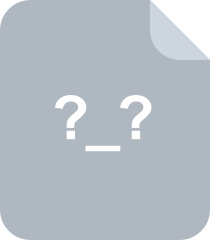
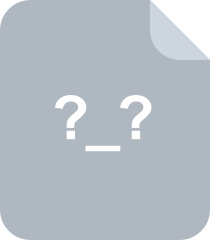
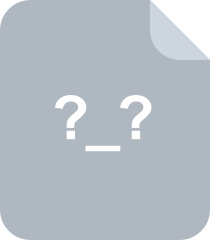
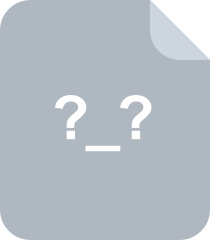
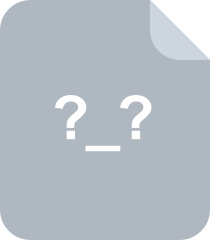
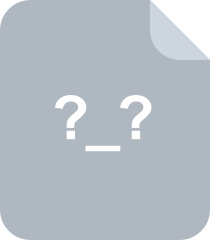
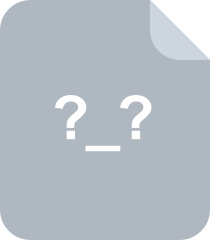
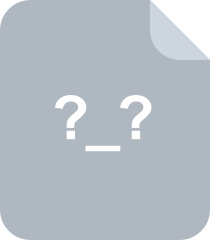
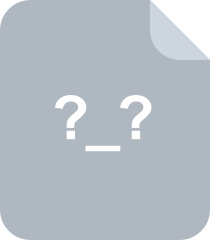
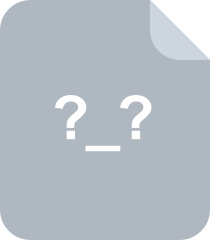
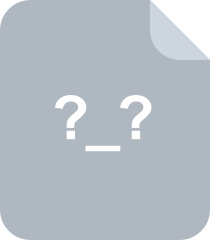
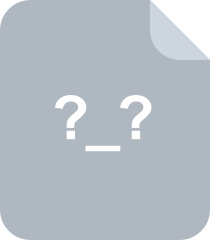
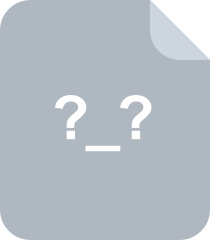
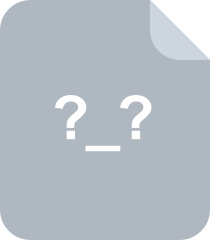
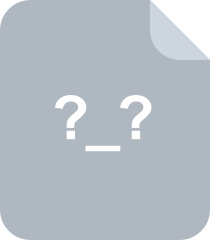
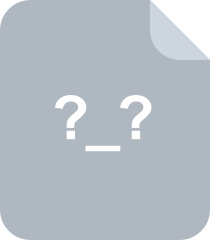
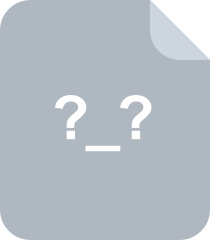
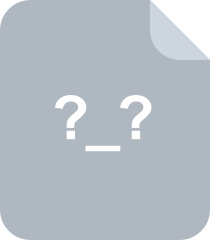
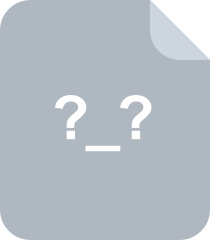
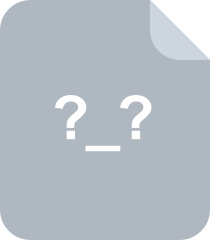
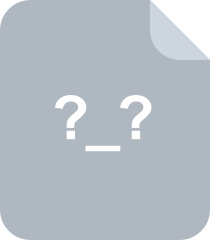
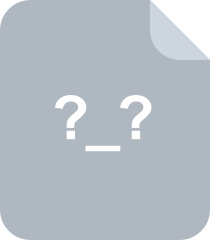
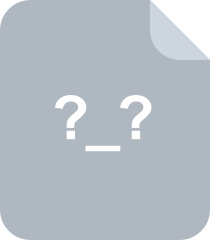
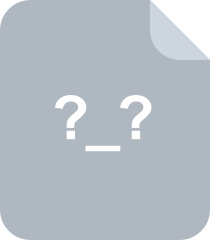
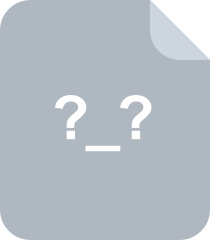
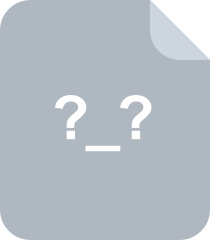
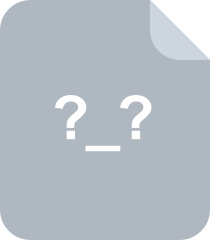
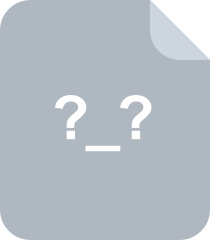
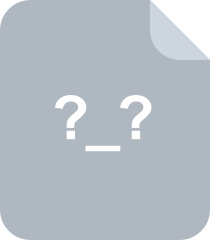
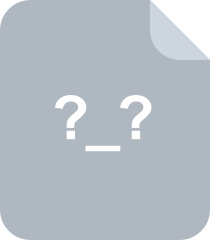
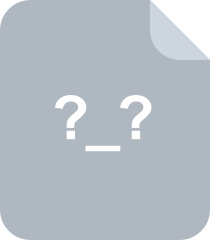
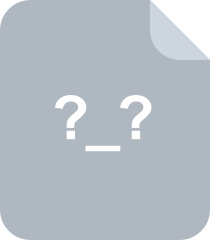
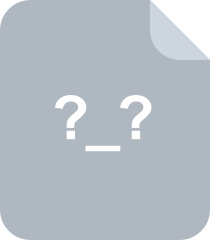
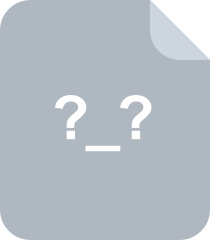
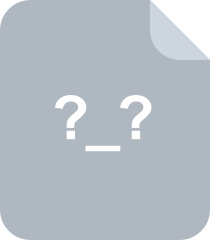
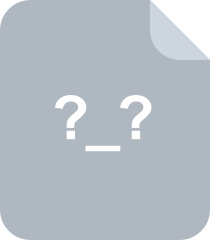
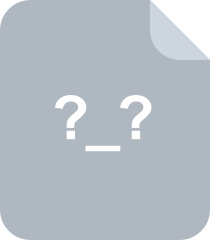
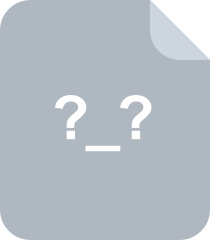
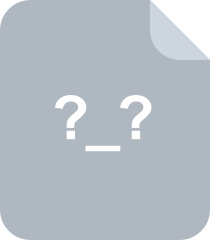
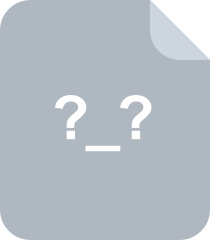
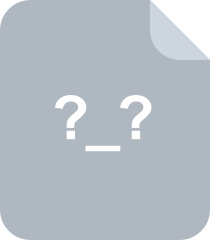
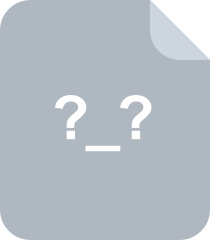
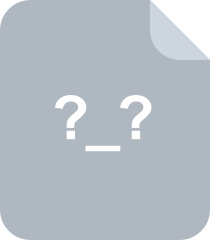
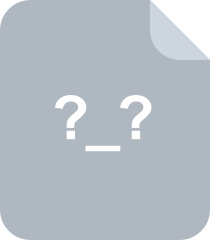
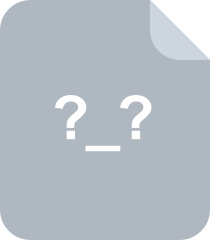
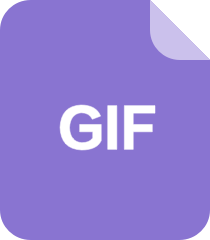
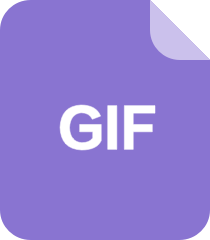
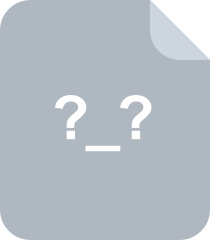
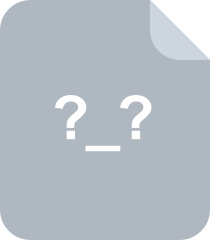
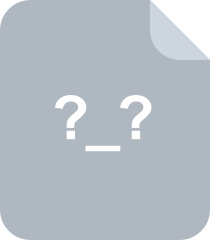
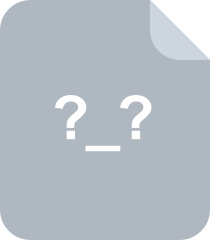
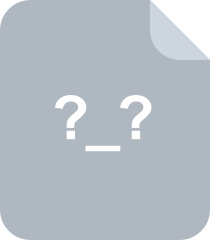
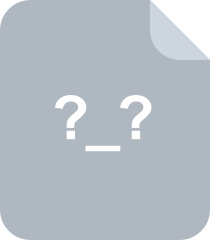
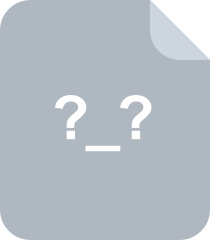
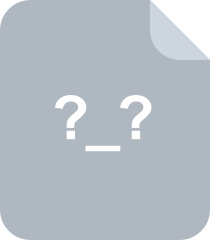
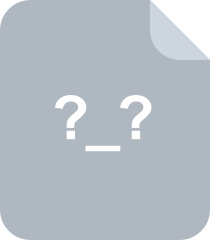
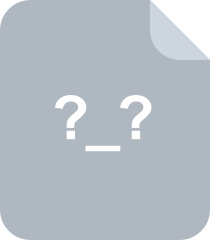
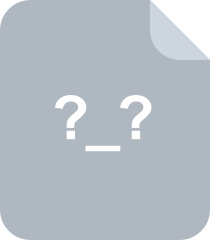
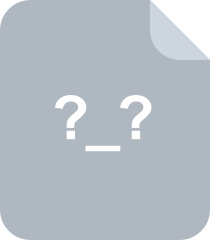
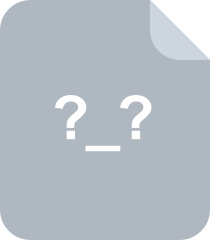
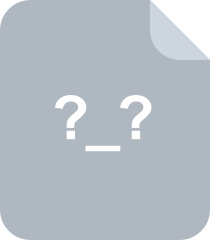
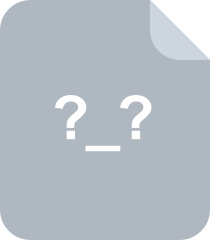
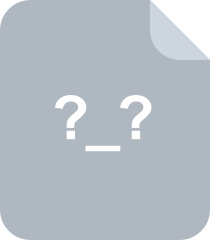
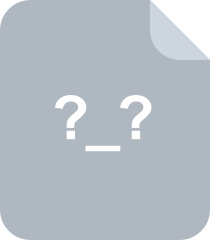
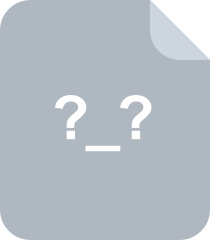
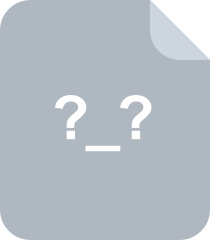
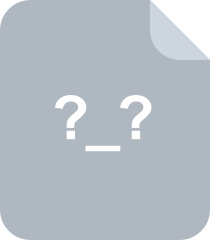
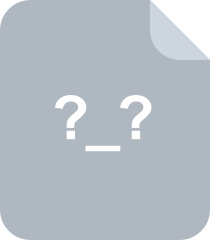
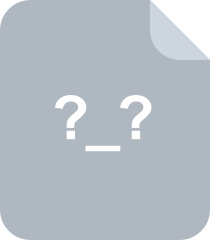
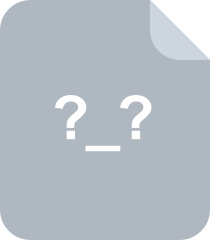
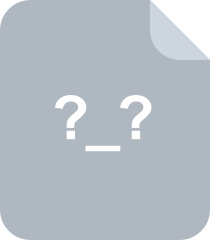
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
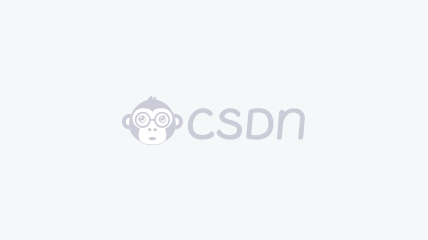

沐知全栈开发
- 粉丝: 5703
- 资源: 5215
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

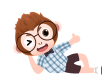
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


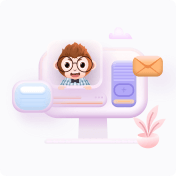
安全验证
文档复制为VIP权益,开通VIP直接复制
