package com.controller;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.entity.Article;
import com.entity.Asks;
import com.entity.Cart;
import com.entity.Cate;
import com.entity.Complains;
import com.entity.Fav;
import com.entity.Goods;
import com.entity.Hist;
import com.entity.Items;
import com.entity.Orders;
import com.entity.Seller;
import com.entity.Topic;
import com.entity.Users;
import com.github.pagehelper.Page;
import com.service.ArticleService;
import com.service.AsksService;
import com.service.CartService;
import com.service.CateService;
import com.service.ComplainsService;
import com.service.FavService;
import com.service.GoodsService;
import com.service.HistService;
import com.service.OrdersService;
import com.service.RecommendService;
import com.service.SellerService;
import com.service.TopicService;
import com.service.UsersService;
import com.util.VeDate;
@RestController // 定义为控制器 返回JSON类型数据
@RequestMapping(value = "/index", produces = "application/json; charset=utf-8") // 设置请求路径
@CrossOrigin // 允许跨域访问其资源
//作者联系 QQ 549710689 毕设成品下载网址 wisdomdd.cn
public class IndexController extends BaseController {
// @Autowired的作用是自动注入依赖的ServiceBean
@Autowired
private AsksService asksService;
@Autowired
private SellerService sellerService;
@Autowired
private UsersService usersService;
@Autowired
private ArticleService articleService;
@Autowired
private CateService cateService;
@Autowired
private GoodsService goodsService;
@Autowired
private CartService cartService;
@Autowired
private HistService histService;
@Autowired
private OrdersService ordersService;
@Autowired
private TopicService topicService;
@Autowired
private ComplainsService complainsService;
@Autowired
private FavService favService;
@Autowired
private RecommendService recommendService;
// TODO Auto-generated method stub
// 预处理 获取基础参数
@GetMapping(value = "front.action")
public Map<String, Object> front() {
Map<String, Object> map = new HashMap<String, Object>();
List<Cate> cateList = this.cateService.getAllCate();
map.put("cateList", cateList);
List<Goods> hotList = this.goodsService.getGoodsByHot();
//视图调用
//List<Goods> hotList = this.goodsService.getGoodsByHotView();
map.put("hotList", hotList);
return map;
}
// 前台首页
@GetMapping(value = "index.action")
public Map<String, Object> index() {
Map<String, Object> map = new HashMap<String, Object>();
List<Cate> cateList = this.cateService.getCateFront();
List<Cate> frontList = new ArrayList<Cate>();
for (Cate cate : cateList) {
List<Goods> goodsList = this.goodsService.getGoodsByCate(cate.getCateid());
cate.setGoodsList(goodsList);
frontList.add(cate);
}
map.put("frontList", frontList);
return map;
}
// 新闻公告
@GetMapping(value = "article.action")
public Map<String, Object> article(@RequestParam(defaultValue = "1") Integer page, @RequestParam(defaultValue = "20") Integer limit) {
Map<String, Object> map = new HashMap<String, Object>();
Page<Article> pager = com.github.pagehelper.PageHelper.startPage(page, limit);// 定义当前页和分页条数
List<Article> list = this.articleService.getAllArticle();
// 返回的map中定义数据格式
map.put("count", pager.getTotal());
map.put("total", list.size());
map.put("data", list);
map.put("code", 0);
map.put("msg", "");
map.put("page", page);
map.put("limit", limit);
return map;
}
// 公告详情
@GetMapping(value = "read.action")
public Map<String, Object> read(String id) {
Map<String, Object> map = new HashMap<String, Object>();
Article article = this.articleService.getArticleById(id);
article.setHits("" + (Integer.parseInt(article.getHits()) + 1));
this.articleService.updateArticle(article);
map.put("article", article);
return map;
}
// 用户登录
@PostMapping(value = "login.action")
public Map<String, Object> login(@RequestBody String jsonStr) {
Map<String, Object> map = new HashMap<String, Object>();
JSONObject obj = JSONObject.parseObject(jsonStr);
String username = obj.getString("username");
String password = obj.getString("password");
Users usersEntity = new Users();
usersEntity.setUsername(username);
List<Users> userslist = this.usersService.getUsersByCond(usersEntity);
if (userslist.size() == 0) {
map.put("success", false);
map.put("message", "用户名不存在");
} else {
Users users = userslist.get(0);
if (password.equals(users.getPassword())) {
map.put("success", true);
map.put("message", "登录成功");
map.put("userid", users.getUsersid());
map.put("username", users.getUsername());
map.put("realname", users.getRealname());
} else {
map.put("success", false);
map.put("message", "密码错误");
}
}
return map;
}
@PostMapping(value = "slogin.action")
public Map<String, Object> slogin(@RequestBody String jsonStr) {
Map<String, Object> map = new HashMap<String, Object>();
JSONObject obj = JSONObject.parseObject(jsonStr);
String username = obj.getString("username");
String password = obj.getString("password");
Seller sellerEntity = new Seller();
sellerEntity.setUsername(username);
List<Seller> sellerlist = this.sellerService.getSellerByCond(sellerEntity);
if (sellerlist.size() == 0) {
map.put("success", false);
map.put("message", "商户不存在");
} else {
Seller seller = sellerlist.get(0);
if ("待审核".equals(seller.getStatus())) {
map.put("success", false);
map.put("message", "商户待审核");
} else if (password.equals(seller.getPassword())) {
map.put("success", true);
map.put("message", "登录成功");
map.put("sellerid", seller.getSellerid());
map.put("username", seller.getUsername());
map.put("sellername", seller.getSellername());
} else {
map.put("success", false);
map.put("message", "密码错误");
}
}
return map;
}
// 获取提示问题
@GetMapping(value = "asks.action")
public List<Asks> asks() {
return this.asksService.getAllAsks();
}
// 用户注册
@PostMapping(value = "register.action")
public Map<String, Object> register(@RequestBody String jsonStr) {
Map<String, Object> map = new HashMap<String, Object>();
JSONObject obj = JSONObject.parseObject(jsonStr); // 将JSON字符串转换成object
Users users = new Users();
users.setUsername(obj.getString("username"));
users.setPassword(obj.getString("password"));
users.setSex(obj.getString("sex"));
users.setBirthday(obj.getString("birthday"));
users.setContact(obj.getString("contact"));
users.setRealname(obj.getString("realname"));
users.setAsksid(obj.getString("asksid"));
users.setAnswer(obj.getString("answer"));
users.setRegdate(VeDate.getStringDateShort());
int num = this.usersService.insertUsers(users);
if (num > 0) {
map.put("success", true);
map.put("code", num);
map.put("message", "注册�
没有合适的资源?快使用搜索试试~ 我知道了~
基于Springboot和Vue的化妆品协同过滤推荐系统前后端分离源码
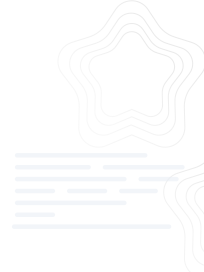
共1467个文件
gif:634个
js:168个
png:153个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 199 浏览量
2024-03-25
22:25:48
上传
评论 1
收藏 79.9MB ZIP 举报
温馨提示
项目简介:本源码为基于Springboot和Vue构建的化妆品协同过滤推荐系统,采用前后端分离的设计模式。该项目主要以Java为开发语言,辅以JavaScript、CSS和HTML等技术。整个项目包含1467个文件,其中包括634个gif动画、168个JavaScript文件、153个PNG图像、111个XML配置文件、81个Java源代码文件、78个CSS样式表、73个HTML页面、56张JPG图片、20个SVG图形以及16个EOT字体文件。系统功能全面,支持用户、多商户及管理员协同工作,致力于提供高效、个性化的化妆品推荐服务。
资源推荐
资源详情
资源评论
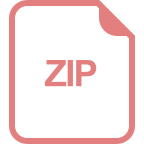
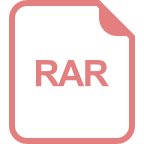
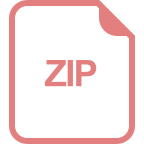
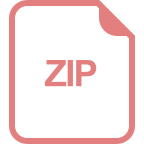
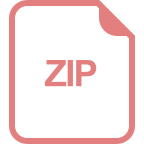
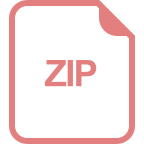
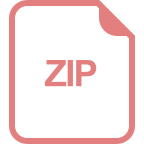
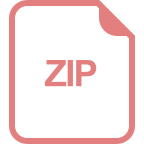
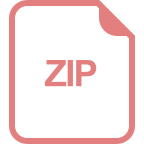
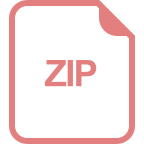
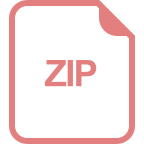
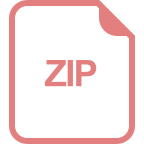
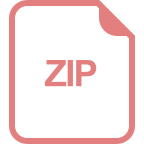
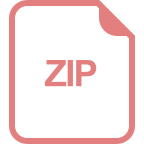
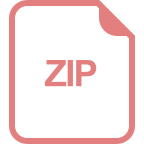
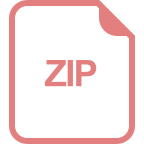
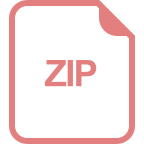
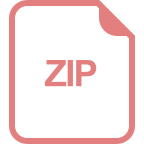
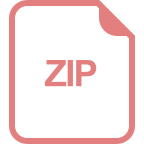
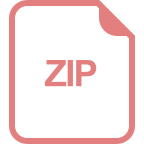
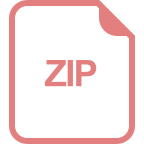
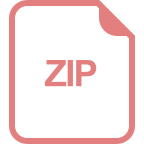
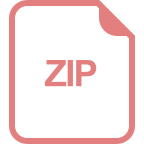
收起资源包目录

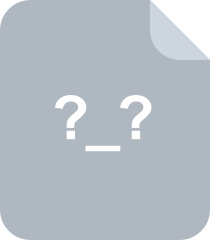
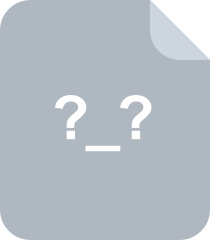
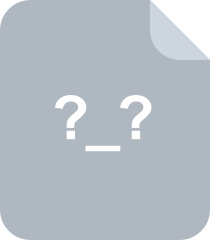
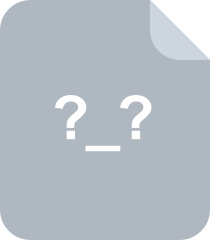
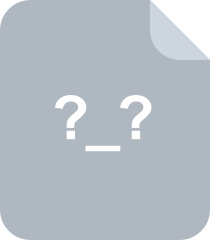
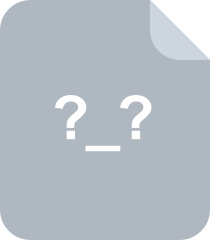
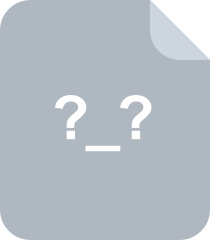
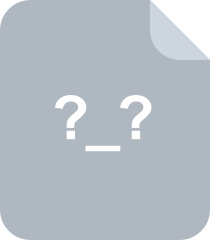
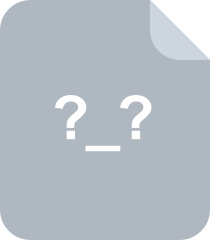
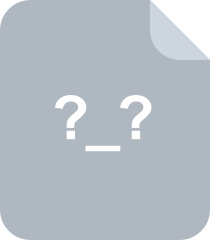
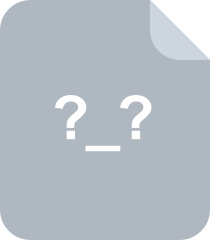
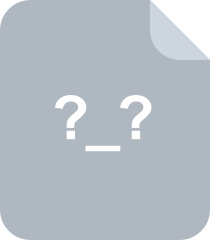
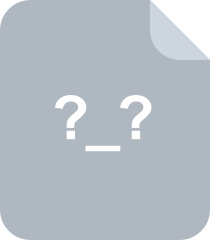
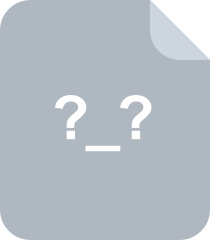
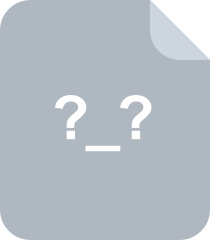
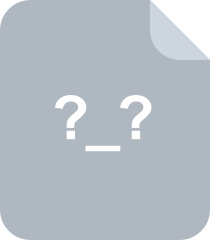
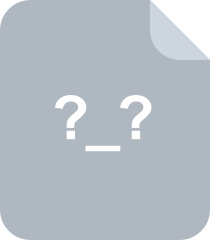
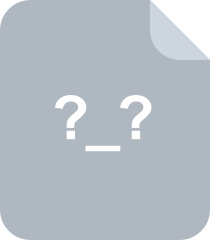
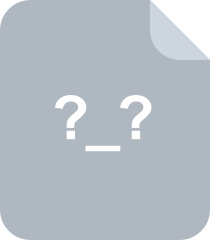
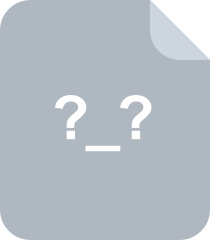
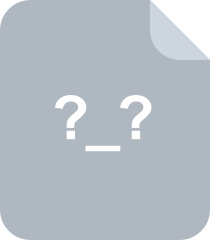
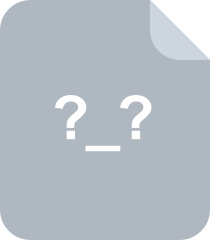
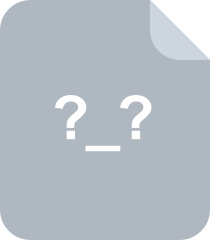
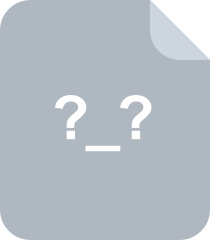
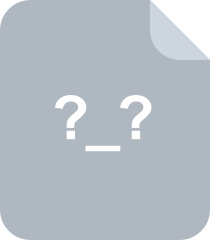
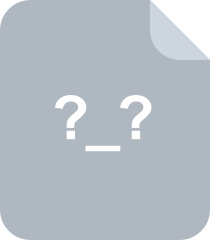
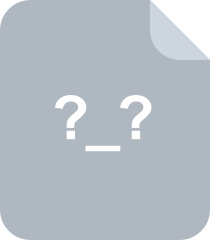
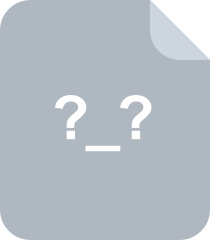
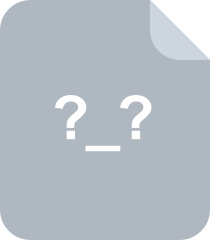
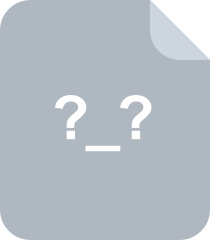
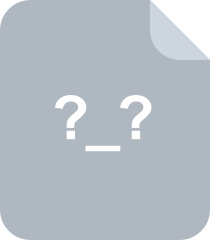
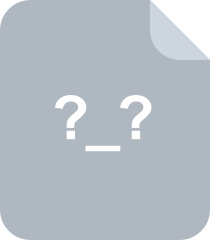
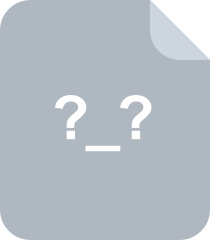
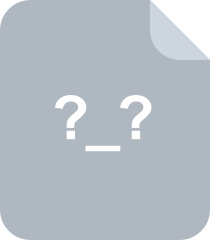
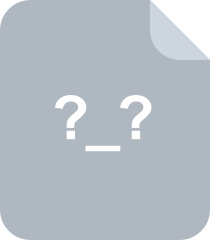
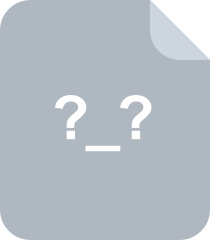
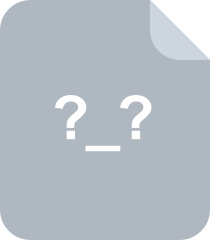
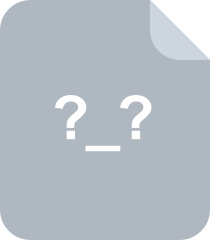
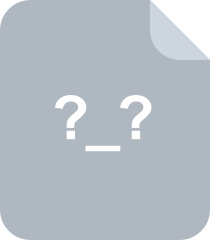
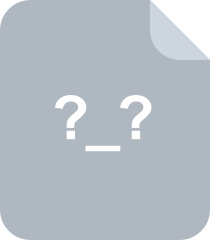
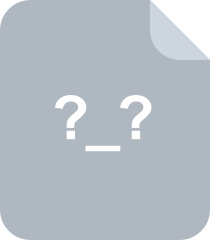
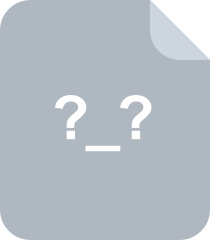
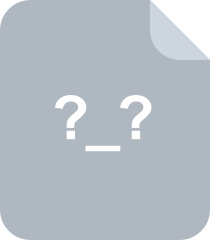
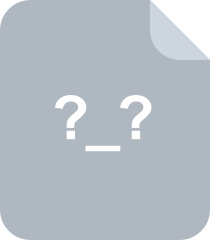
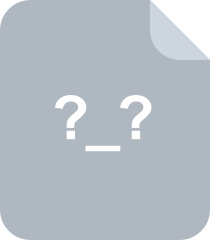
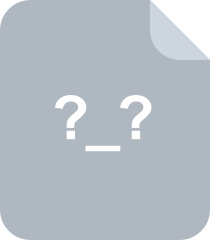
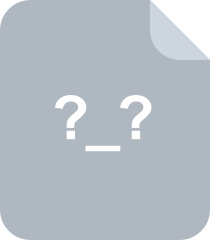
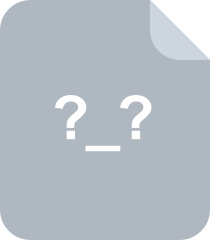
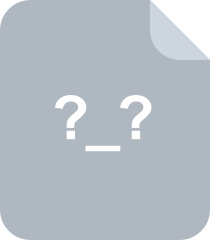
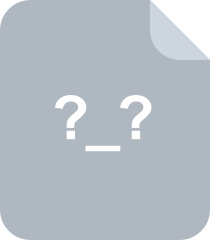
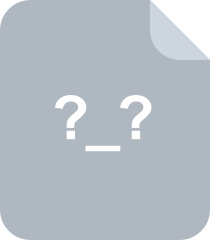
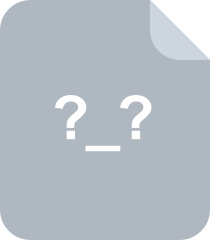
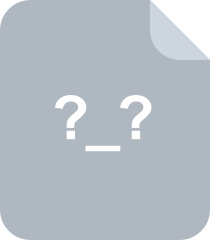
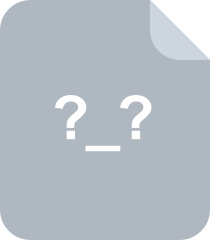
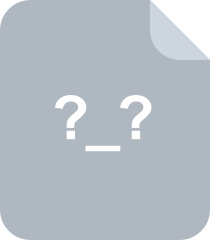
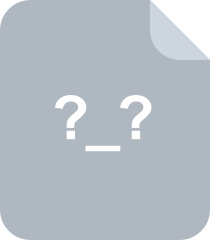
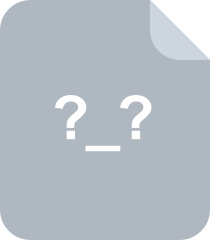
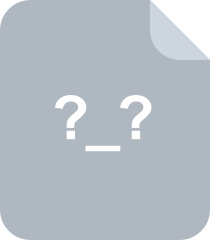
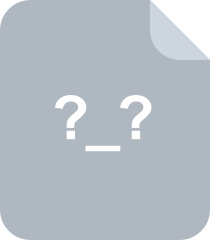
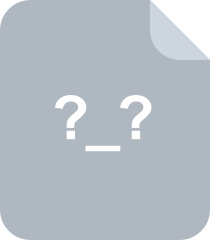
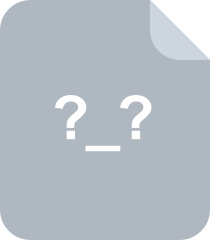
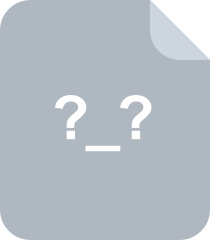
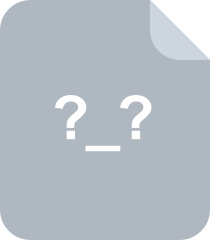
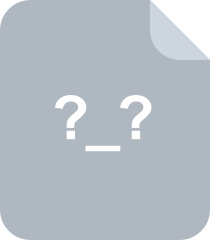
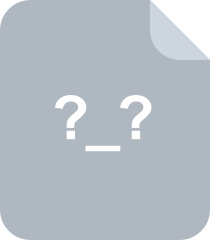
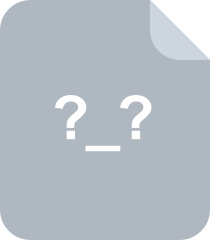
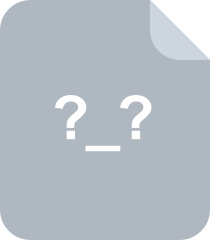
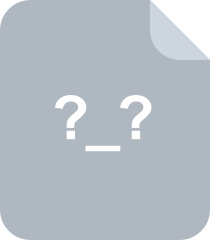
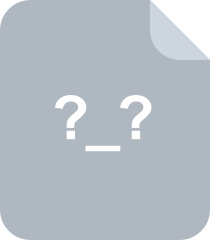
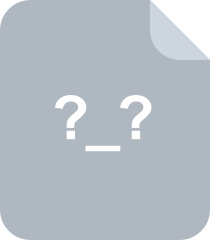
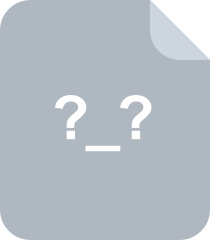
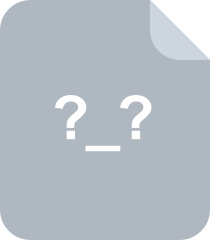
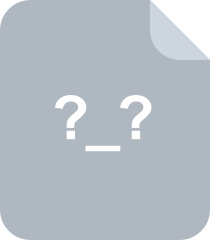
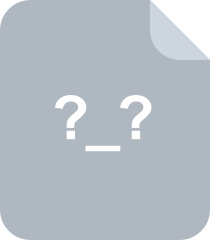
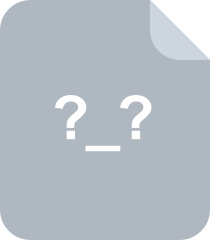
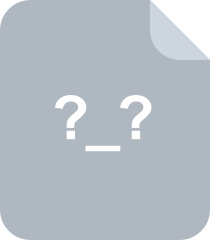
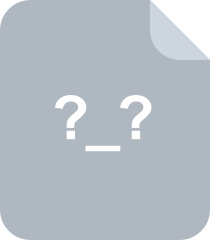
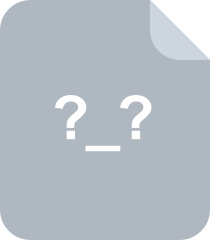
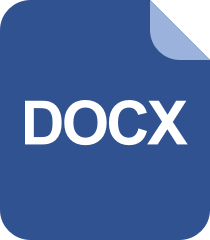
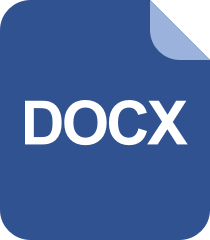
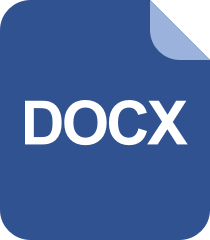
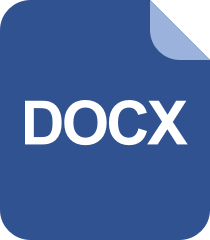
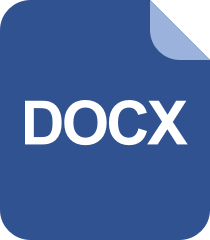
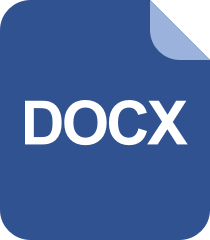
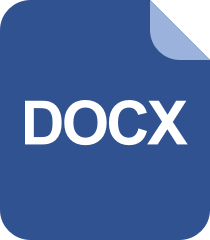
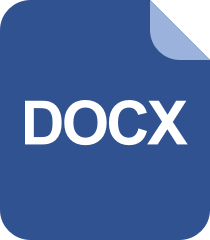
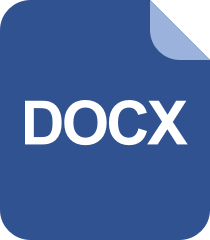
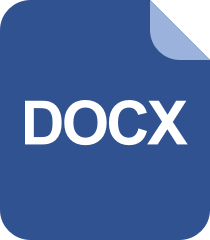
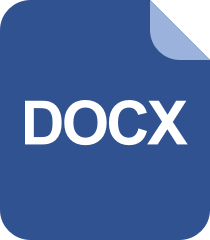
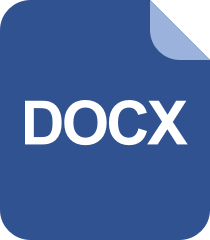
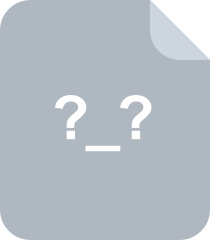
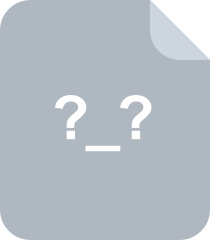
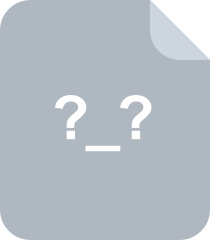
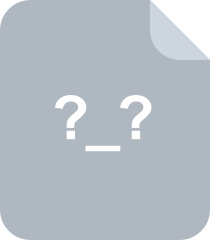
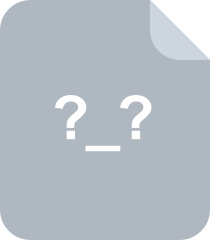
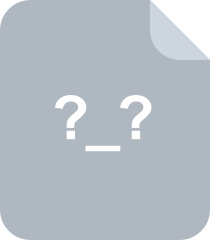
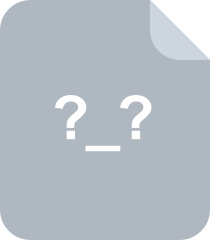
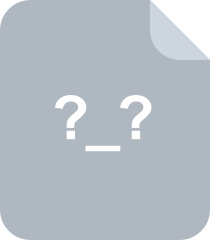
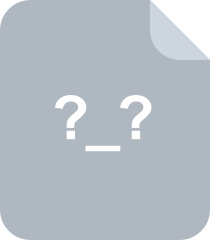
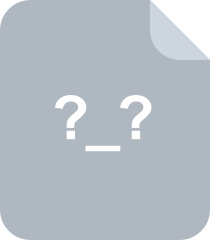
共 1467 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
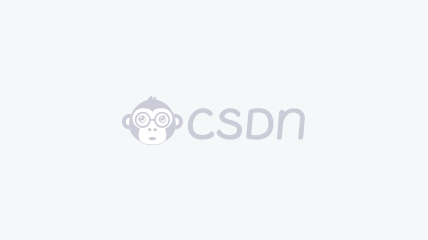

沐知全栈开发
- 粉丝: 5703
- 资源: 5219

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

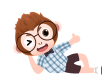
最新资源
- exp4_2.c.sln
- [雷军]美妙的爱情......福的味道。.mp3
- 2023-04-06-项目笔记 - 第三百二十阶段 - 4.4.2.318全局变量的作用域-318 -2025.11.17
- 2023-04-06-项目笔记 - 第三百二十阶段 - 4.4.2.318全局变量的作用域-318 -2025.11.17
- java资源异步IO框架 Cindy
- java资源业务流程管理(BPM)和工作流系统 Activiti
- java资源高性能内存消息和事件驱动库 Chronicle
- 哋它亢技术应用2慕课自动化学习
- java资源高性能的JSON处理 Jackson
- java资源高性能的Java 3D引擎 Xith3D
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


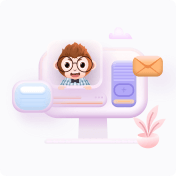
安全验证
文档复制为VIP权益,开通VIP直接复制
