angular8 实现动态 加载组件
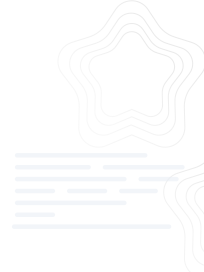

在Angular框架中,动态加载组件是一项高级特性,它允许我们在运行时根据需求创建和插入新的组件实例。这对于构建可扩展的、模块化的应用或者实现插件系统非常有用。本篇文章将深入探讨如何在Angular 8中实现动态加载组件。 我们需要理解Angular组件的基本概念。组件是Angular应用的基本构建块,它们负责渲染UI并处理用户交互。通常,组件在应用启动时就被定义和编译。但是,动态加载组件意味着我们可以在运行时决定要加载哪个组件,何时加载,甚至可以多次加载不同的组件。 要实现动态加载组件,我们主要需要以下步骤: 1. **创建动态组件**: 你需要有一个或多个组件类,这些组件将会被动态加载。确保这些组件已经正确地导入到你的项目中,并且已经通过`@Component`装饰器进行了配置。 2. **元数据注册**: 在`ngModule`中,使用` declarations`属性声明这些组件,并在`entryComponents`数组中添加,这是为了让Angular知道这些组件可以被动态创建。 ```typescript @NgModule({ declarations: [DynamicComponent, ...], entryComponents: [DynamicComponent, ...] }) export class AppModule { } ``` 3. **动态组件工厂**: 使用`ComponentFactoryResolver`服务来获取目标组件的`ComponentFactory`。`ComponentFactory`是Angular用于创建组件实例的对象。 ```typescript import { ComponentFactoryResolver } from '@angular/core'; @Component({ ... }) export class MyComponent { ... } constructor(private componentFactoryResolver: ComponentFactoryResolver) { } getComponentFactory() { const componentFactory = this.componentFactoryResolver.resolveComponentFactory(MyComponent); return componentFactory; } ``` 4. **插入组件**: 获取到`ComponentFactory`后,我们需要一个位置来插入这个新创建的组件。这通常是一个名为`ViewContainerRef`的元素,它可以是一个已存在的DOM元素或者一个自定义的`<ng-template>`元素。 ```typescript @Component({ template: '<div #dynamic></div>' }) export class HostComponent { @ViewChild('dynamic', { read: ViewContainerRef }) container: ViewContainerRef; createComponent(componentFactory) { this.container.clear(); const componentRef = this.container.createComponent(componentFactory); // 可以访问组件实例 const instance = componentRef.instance; // ... } } ``` 5. **销毁组件**: 当不再需要动态加载的组件时,可以通过`ComponentRef`对象的`destroy()`方法来销毁它。 ```typescript componentRef.destroy(); ``` 在Angular 8中,动态加载组件的应用场景广泛,比如用于创建自定义表单控件、弹出框、可扩展的导航菜单等。这个过程涉及到Angular的核心机制,理解和掌握动态加载组件能帮助开发者更灵活地构建复杂的应用。 动态加载组件是Angular 8的一个强大特性,它提供了在运行时创建和插入组件的能力,极大地提高了应用的灵活性和可扩展性。通过正确地创建组件工厂、获取`ViewContainerRef`以及调用`ComponentFactoryResolver`,开发者可以轻松地在应用中实现这一功能。



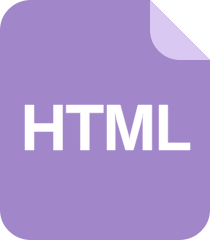
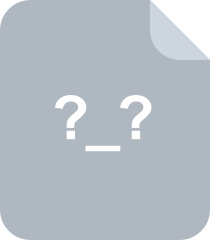
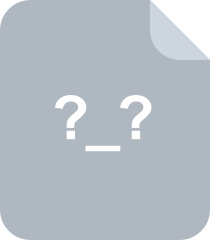
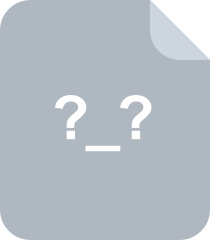

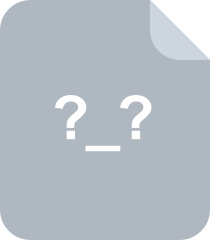
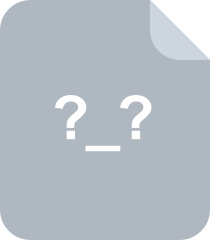
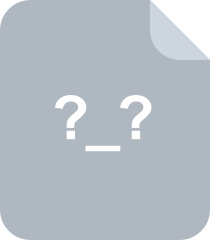
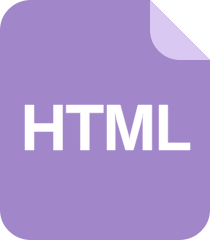
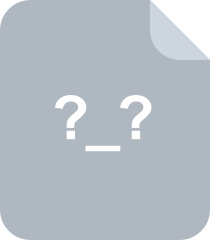
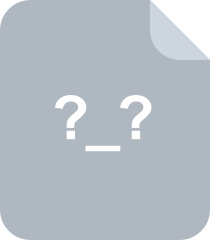
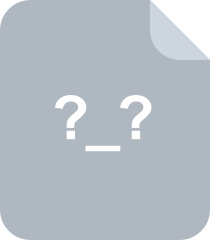
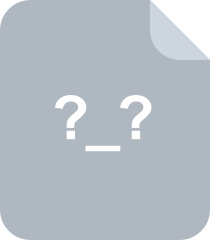

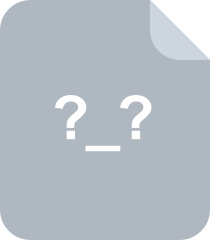
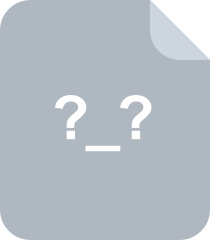
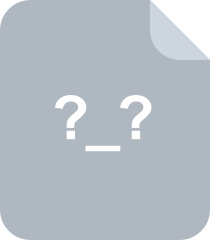
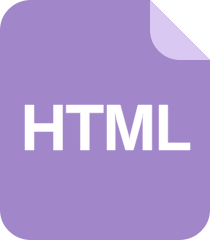
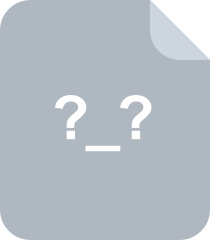
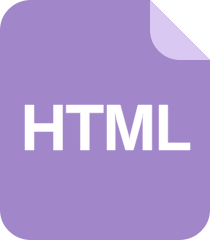
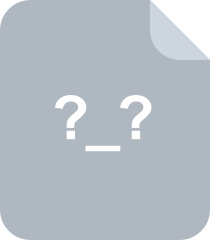
- 1
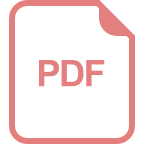
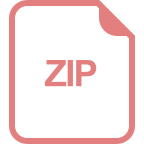
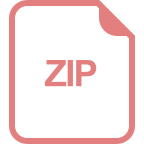
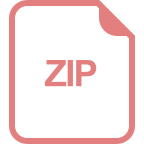
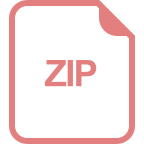
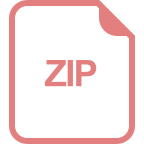
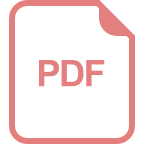
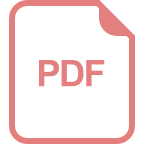
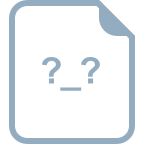
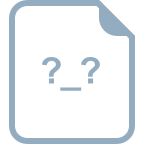
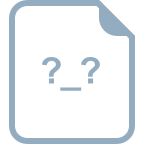
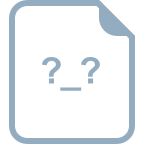
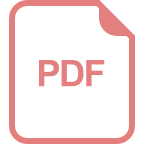
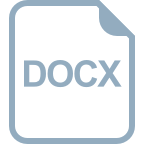
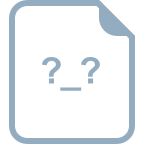
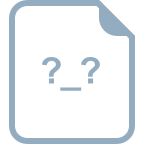
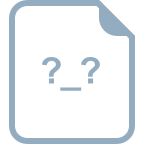
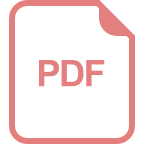
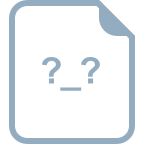
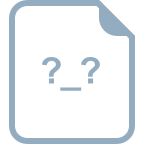
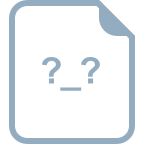
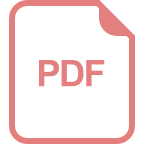
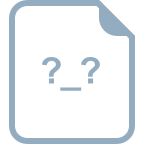
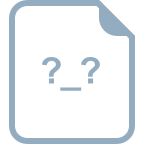
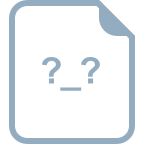

- 粉丝: 404
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

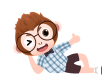
最新资源
- 面向初学者的 Java 教程(包含 500 个代码示例).zip
- 阿里云OSS Java版SDK.zip
- 阿里云api网关请求签名示例(java实现).zip
- 通过示例学习 Android 的 RxJava.zip
- 通过多线程编程在 Java 中发现并发模式和特性 线程、锁、原子等等 .zip
- 通过在终端中进行探索来学习 JavaScript .zip
- 通过不仅针对初学者而且针对 JavaScript 爱好者(无论他们的专业水平如何)设计的编码挑战,自然而自信地拥抱 JavaScript .zip
- 适用于 Kotlin 和 Java 的现代 JSON 库 .zip
- yolo5实战-yolo资源
- english-chinese-dictionary-数据结构课程设计

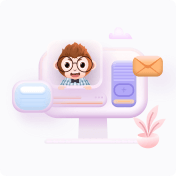

评论0