# Gin Web Framework
<img align="right" width="159px" src="https://raw.githubusercontent.com/gin-gonic/logo/master/color.png">
[](https://travis-ci.org/gin-gonic/gin)
[](https://codecov.io/gh/gin-gonic/gin)
[](https://goreportcard.com/report/github.com/gin-gonic/gin)
[](https://godoc.org/github.com/gin-gonic/gin)
[](https://gitter.im/gin-gonic/gin?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://sourcegraph.com/github.com/gin-gonic/gin?badge)
[](https://www.codetriage.com/gin-gonic/gin)
[](https://github.com/gin-gonic/gin/releases)
Gin is a web framework written in Go (Golang). It features a martini-like API with much better performance, up to 40 times faster thanks to [httprouter](https://github.com/julienschmidt/httprouter). If you need performance and good productivity, you will love Gin.
## Contents
- [Installation](#installation)
- [Prerequisite](#prerequisite)
- [Quick start](#quick-start)
- [Benchmarks](#benchmarks)
- [Gin v1.stable](#gin-v1-stable)
- [Build with jsoniter](#build-with-jsoniter)
- [API Examples](#api-examples)
- [Using GET,POST,PUT,PATCH,DELETE and OPTIONS](#using-get-post-put-patch-delete-and-options)
- [Parameters in path](#parameters-in-path)
- [Querystring parameters](#querystring-parameters)
- [Multipart/Urlencoded Form](#multiparturlencoded-form)
- [Another example: query + post form](#another-example-query--post-form)
- [Map as querystring or postform parameters](#map-as-querystring-or-postform-parameters)
- [Upload files](#upload-files)
- [Grouping routes](#grouping-routes)
- [Blank Gin without middleware by default](#blank-gin-without-middleware-by-default)
- [Using middleware](#using-middleware)
- [How to write log file](#how-to-write-log-file)
- [Custom Log Format](#custom-log-format)
- [Model binding and validation](#model-binding-and-validation)
- [Custom Validators](#custom-validators)
- [Only Bind Query String](#only-bind-query-string)
- [Bind Query String or Post Data](#bind-query-string-or-post-data)
- [Bind Uri](#bind-uri)
- [Bind HTML checkboxes](#bind-html-checkboxes)
- [Multipart/Urlencoded binding](#multiparturlencoded-binding)
- [XML, JSON, YAML and ProtoBuf rendering](#xml-json-yaml-and-protobuf-rendering)
- [JSONP rendering](#jsonp)
- [Serving static files](#serving-static-files)
- [Serving data from reader](#serving-data-from-reader)
- [HTML rendering](#html-rendering)
- [Multitemplate](#multitemplate)
- [Redirects](#redirects)
- [Custom Middleware](#custom-middleware)
- [Using BasicAuth() middleware](#using-basicauth-middleware)
- [Goroutines inside a middleware](#goroutines-inside-a-middleware)
- [Custom HTTP configuration](#custom-http-configuration)
- [Support Let's Encrypt](#support-lets-encrypt)
- [Run multiple service using Gin](#run-multiple-service-using-gin)
- [Graceful restart or stop](#graceful-restart-or-stop)
- [Build a single binary with templates](#build-a-single-binary-with-templates)
- [Bind form-data request with custom struct](#bind-form-data-request-with-custom-struct)
- [Try to bind body into different structs](#try-to-bind-body-into-different-structs)
- [http2 server push](#http2-server-push)
- [Define format for the log of routes](#define-format-for-the-log-of-routes)
- [Set and get a cookie](#set-and-get-a-cookie)
- [Testing](#testing)
- [Users](#users)
## Installation
To install Gin package, you need to install Go and set your Go workspace first.
1. Download and install it:
```sh
$ go get -u github.com/gin-gonic/gin
```
2. Import it in your code:
```go
import "github.com/gin-gonic/gin"
```
3. (Optional) Import `net/http`. This is required for example if using constants such as `http.StatusOK`.
```go
import "net/http"
```
### Use a vendor tool like [Govendor](https://github.com/kardianos/govendor)
1. `go get` govendor
```sh
$ go get github.com/kardianos/govendor
```
2. Create your project folder and `cd` inside
```sh
$ mkdir -p $GOPATH/src/github.com/myusername/project && cd "$_"
```
3. Vendor init your project and add gin
```sh
$ govendor init
$ govendor fetch github.com/gin-gonic/gin@v1.3
```
4. Copy a starting template inside your project
```sh
$ curl https://raw.githubusercontent.com/gin-gonic/examples/master/basic/main.go > main.go
```
5. Run your project
```sh
$ go run main.go
```
## Prerequisite
Now Gin requires Go 1.6 or later and Go 1.7 will be required soon.
## Quick start
```sh
# assume the following codes in example.go file
$ cat example.go
```
```go
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
```
```
# run example.go and visit 0.0.0.0:8080/ping on browser
$ go run example.go
```
## Benchmarks
Gin uses a custom version of [HttpRouter](https://github.com/julienschmidt/httprouter)
[See all benchmarks](/BENCHMARKS.md)
Benchmark name | (1) | (2) | (3) | (4)
--------------------------------------------|-----------:|------------:|-----------:|---------:
**BenchmarkGin_GithubAll** | **30000** | **48375** | **0** | **0**
BenchmarkAce_GithubAll | 10000 | 134059 | 13792 | 167
BenchmarkBear_GithubAll | 5000 | 534445 | 86448 | 943
BenchmarkBeego_GithubAll | 3000 | 592444 | 74705 | 812
BenchmarkBone_GithubAll | 200 | 6957308 | 698784 | 8453
BenchmarkDenco_GithubAll | 10000 | 158819 | 20224 | 167
BenchmarkEcho_GithubAll | 10000 | 154700 | 6496 | 203
BenchmarkGocraftWeb_GithubAll | 3000 | 570806 | 131656 | 1686
BenchmarkGoji_GithubAll | 2000 | 818034 | 56112 | 334
BenchmarkGojiv2_GithubAll | 2000 | 1213973 | 274768 | 3712
BenchmarkGoJsonRest_GithubAll | 2000 | 785796 | 134371 | 2737
BenchmarkGoRestful_GithubAll | 300 | 5238188 | 689672 | 4519
BenchmarkGorillaMux_GithubAll | 100 | 10257726 | 211840 | 2272
BenchmarkHttpRouter_GithubAll | 20000 | 105414 | 13792 | 167
BenchmarkHttpTreeMux_GithubAll | 10000 | 319934 | 65856 | 671
BenchmarkKocha_GithubAll | 10000 | 209442 | 23304 | 843
BenchmarkLARS_GithubAll | 20000 | 62565 | 0 | 0
BenchmarkMacaron_GithubAll | 2000 | 1161270 | 204194 | 2000
BenchmarkMartini_GithubAll | 200 | 9991713 | 226549 | 2325
BenchmarkPat_GithubAll | 200 | 5590793 | 1499568 | 27435
BenchmarkPossum_GithubAll | 10000 | 319768 | 84448 | 609
BenchmarkR2router_GithubAll | 10000 | 305134 | 77328 | 979
BenchmarkRivet_GithubAll | 10000 | 132134 | 16272 | 167
Be
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【作品名称】:基于Arduino+Android的无线智能小车系统的设计与实现【毕业设计】(论文+源码) 【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。 基于Arduino+Android的无线智能小车的设计与实现 简述 1.硬件部分使用基于Arduino平台的ESP8266 2.Android实现控制小车运动,Android使用kotlin编写 3.数据查看使用web端 4.服务器程序使用Golang编写,可轻松部署到云服务器 在采集数据的需求下,还要求它能运动,因此无线智能小车将是不二之选。无线智能小车在无线和小车结合下,既能运动又能采集数据,而且在无线网络下,更加的便利,不需要拖着长长的通信线同时还能及时地回传数据可谓是一举多得。 本文将基于Arduino平台和Android设计并实现能够无线远程控制运动并采集温湿度的智能小车系统。 关键词:Arduino,Android,无线,智能小车
资源推荐
资源详情
资源评论
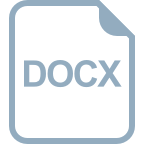
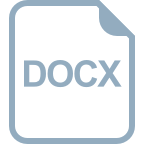
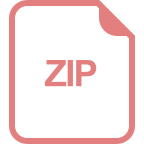
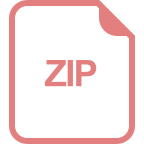
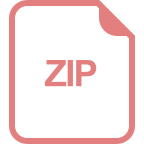
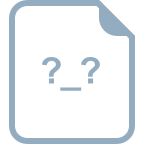
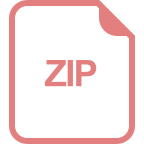
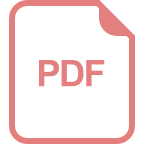
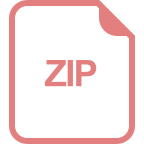
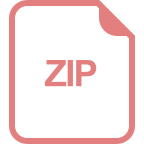
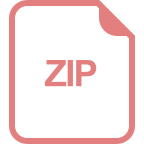
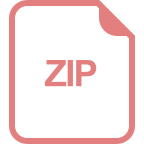
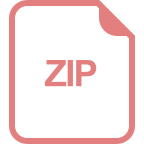
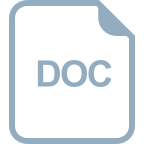
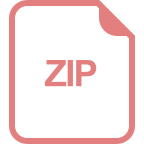
收起资源包目录

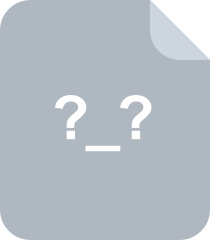
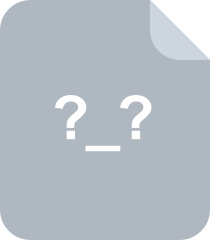
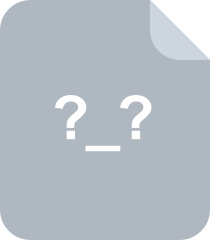
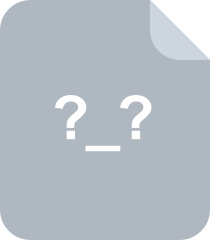
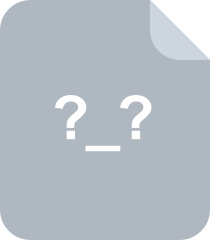
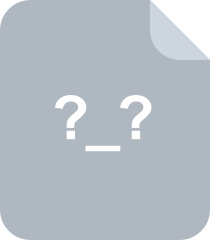
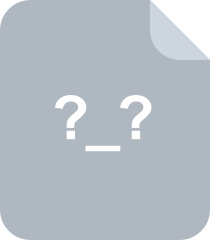
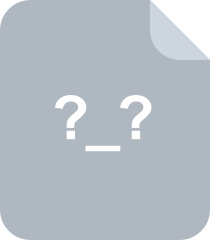
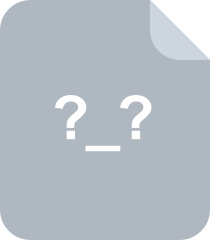
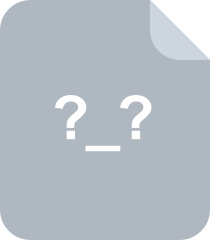
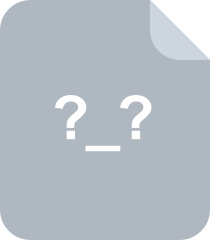
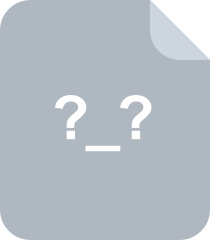
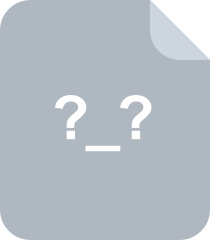
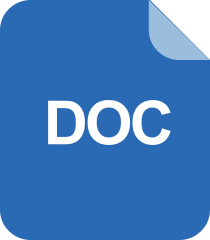
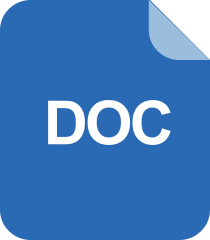
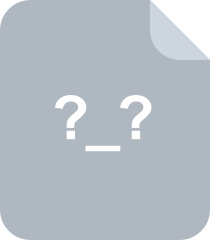
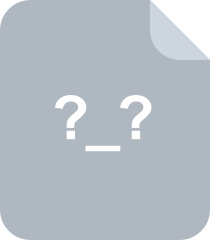
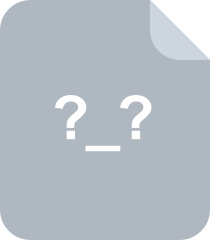
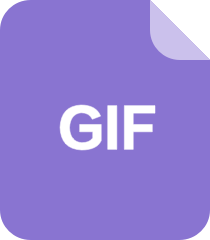
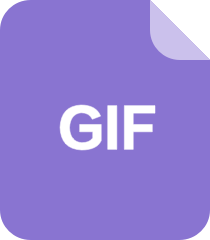
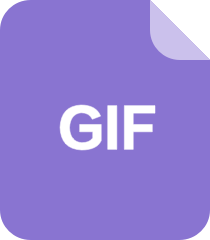
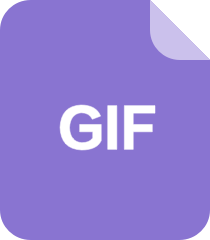
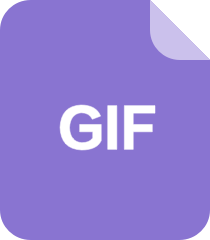
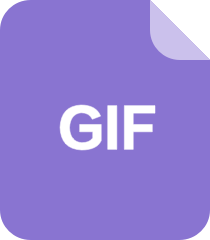
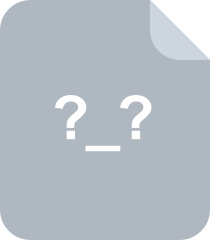
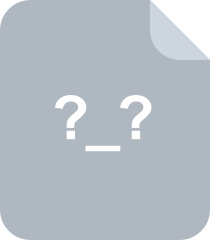
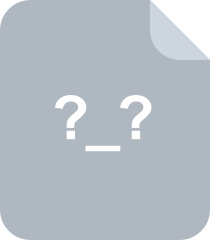
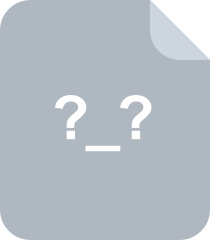
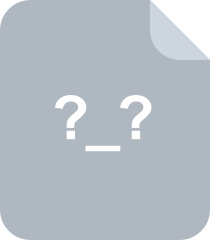
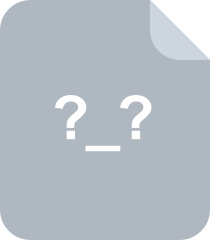
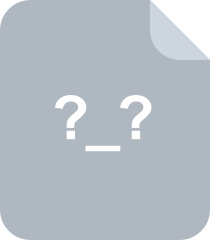
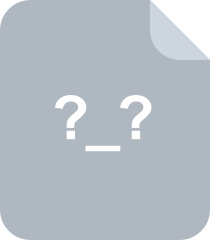
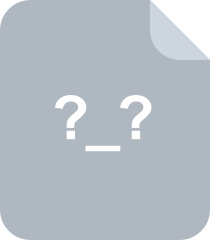
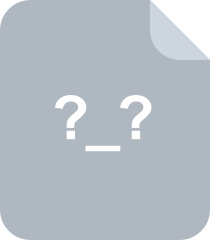
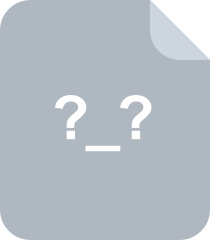
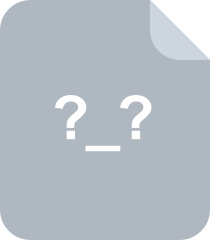
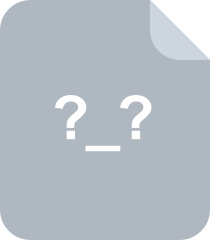
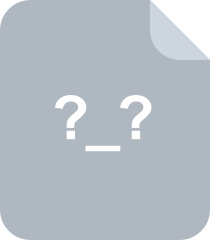
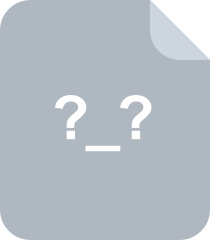
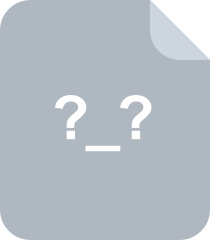
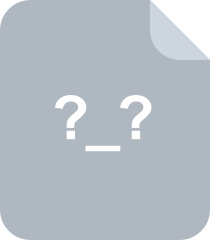
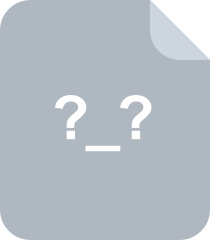
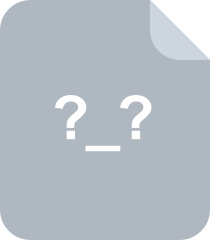
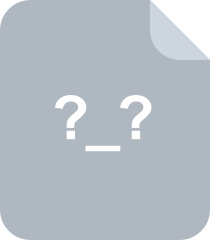
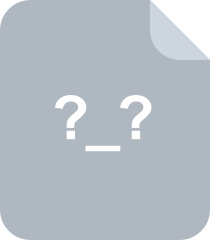
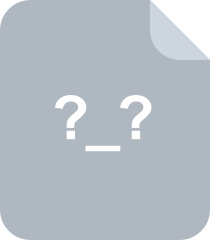
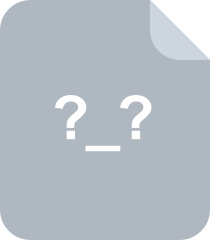
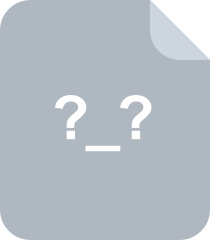
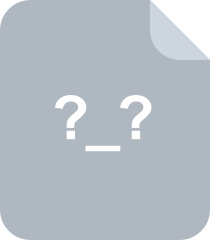
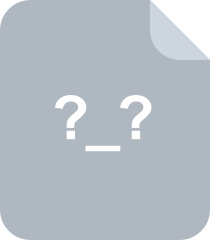
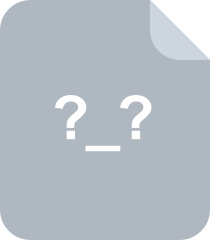
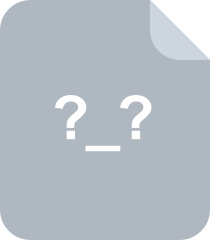
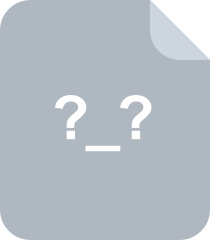
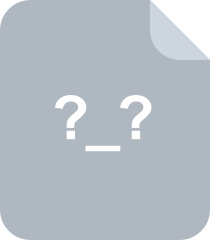
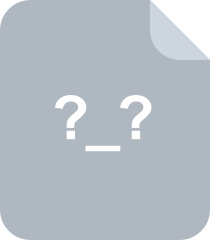
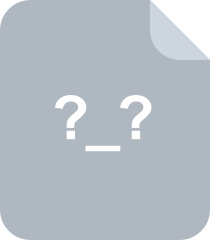
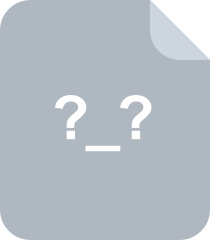
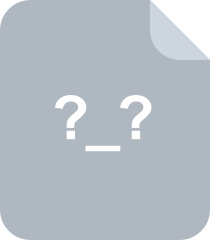
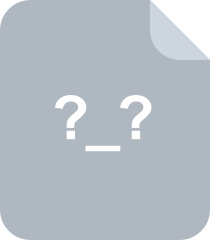
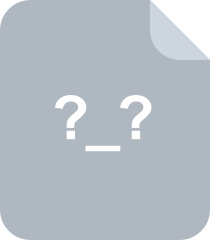
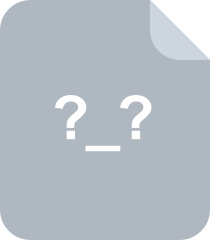
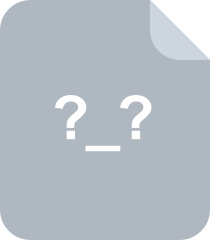
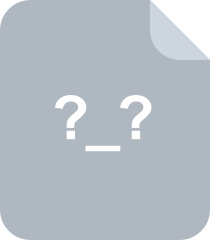
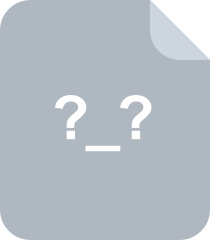
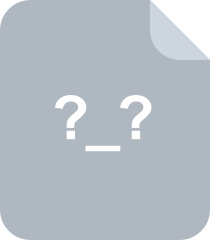
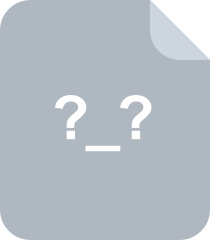
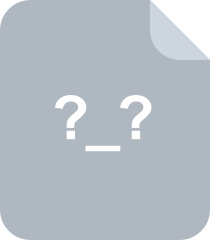
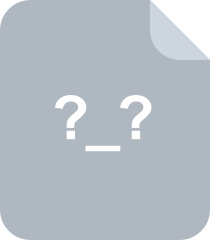
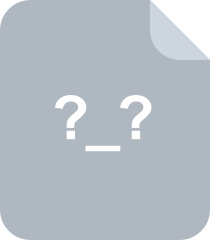
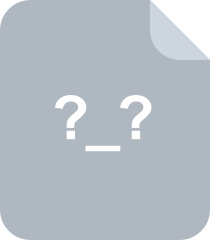
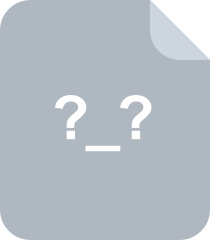
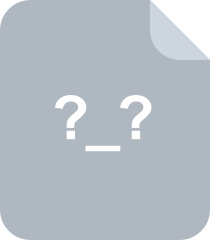
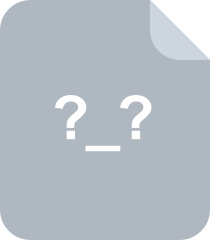
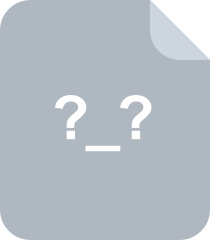
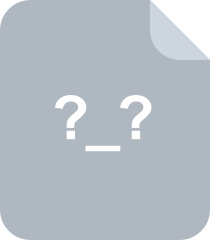
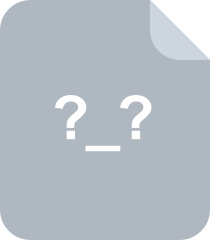
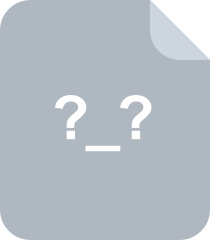
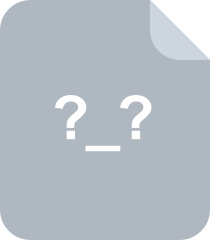
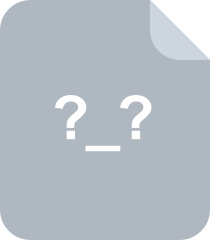
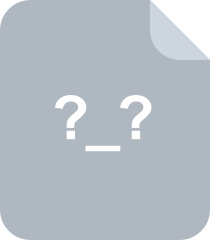
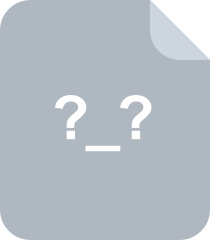
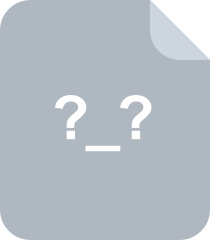
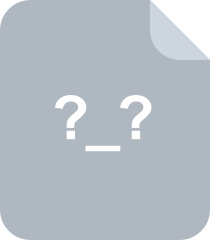
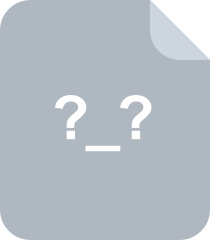
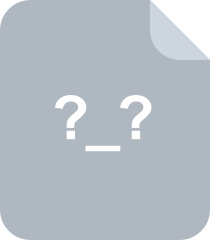
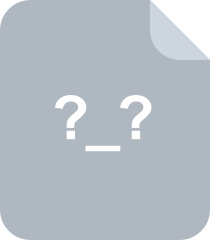
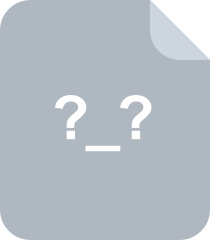
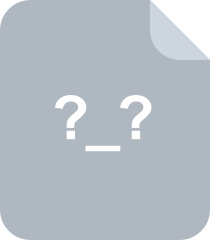
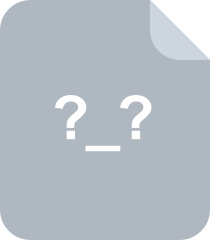
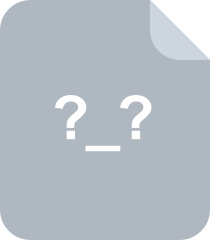
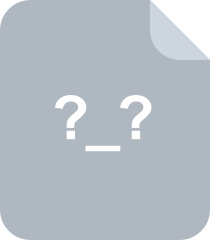
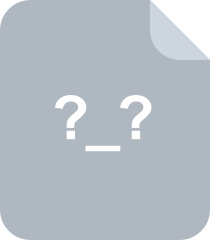
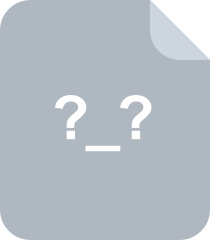
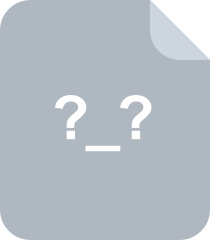
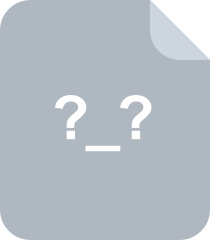
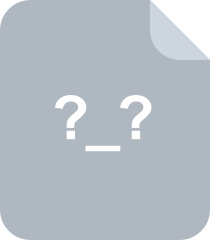
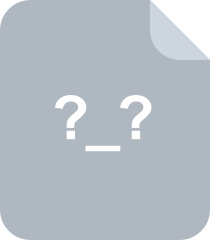
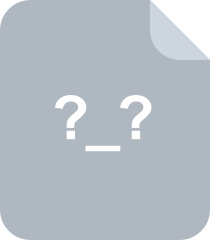
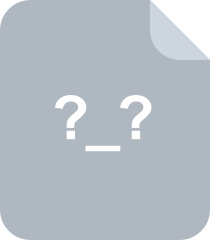
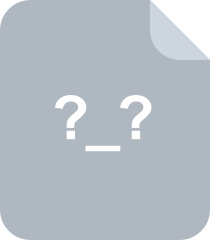
共 367 条
- 1
- 2
- 3
- 4
资源评论
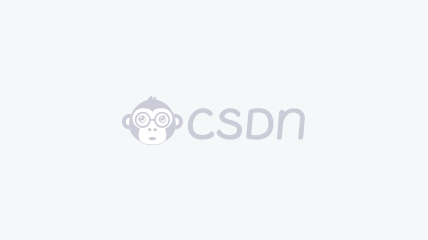

MarcoPage
- 粉丝: 4302
- 资源: 8839
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

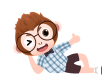
最新资源
- (源码)基于C语言的系统服务框架.zip
- (源码)基于Spring MVC和MyBatis的选课管理系统.zip
- (源码)基于ArcEngine的GIS数据处理系统.zip
- (源码)基于JavaFX和MySQL的医院挂号管理系统.zip
- (源码)基于IdentityServer4和Finbuckle.MultiTenant的多租户身份认证系统.zip
- (源码)基于Spring Boot和Vue3+ElementPlus的后台管理系统.zip
- (源码)基于C++和Qt框架的dearoot配置管理系统.zip
- (源码)基于 .NET 和 EasyHook 的虚拟文件系统.zip
- (源码)基于Python的金融文档智能分析系统.zip
- (源码)基于Java的医药管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


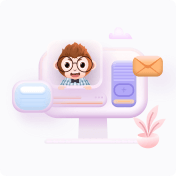
安全验证
文档复制为VIP权益,开通VIP直接复制
