/**
*
*/
package com.chen.action;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import com.chen.bean.Comments;
import com.chen.bean.News;
import com.chen.bean.Pages;
import com.chen.bean.Topics;
import com.chen.bean.Types;
import com.chen.bean.Users;
import com.chen.service.TopicService;
/**
* @author chenguoji
* @email chenguo_ji@163.com
*/
public class TopicAction {
private TopicService topicService;
private Topics topic;
private Types ttype;
private News tnew;
private Pages pageBean;
private int nowPage = 1;
private int listFloor[];
private int comFlag = 0;
private String content;
private List<Topics> listTopic;
private List<Comments> listComment;
HttpSession session = ServletActionContext.getRequest().getSession();
HttpServletResponse response = ServletActionContext.getResponse();
HttpServletRequest request = ServletActionContext.getRequest();
public String add() throws Exception {
Users user = (Users) session.getAttribute("tu");
boolean flag = this.topicService.add(topic, user, response, request,
ttype);
if (flag != true) {
return "topic_add_no";
}
return "topic_add_ok";
}
/**
* 去往指定的帖子,默认只显示未被删除的评论
*
* @return
* @throws Exception
*/
public String goTopic() throws Exception {
Topics top = this.topicService.find(topic.getId());
if(top == null){
return "error";
}
List<Comments> list = new ArrayList<Comments>();
List<Comments> listTemp = new ArrayList<Comments>();
listTemp.addAll(top.getTopicComments());
if (this.comFlag == 0) {
for (int i = 0; i < listTemp.size(); i++) {
if (listTemp.get(i).getStatus() == 0) {
list.add(listTemp.get(i));
}
}
} else if (this.comFlag == 2) {
for (int i = 0; i < listTemp.size(); i++) {
if (listTemp.get(i).getCommentsUser().getId() == top
.getTopicsUser().getId()) {
list.add(listTemp.get(i));
}
}
} else if (this.comFlag == 3) {
for (int i = 0; i < listTemp.size(); i++) {
if (listTemp.get(i).getIntegral() > 0) {
list.add(listTemp.get(i));
}
}
} else {
list = listTemp;
}
/* 先将list按楼层排序 */
if (list.size() > 1) {
this.QuickSort(list, 0, list.size() - 1);
}
this.nowPage = (this.nowPage == 0) ? 1 : this.nowPage;
/* 再将list按要求分页 */
this.pageBean = this.QueryCommentsForPage(10, nowPage, list);
this.listComment = this.pageBean.getListComments();
this.topic = top;
return "topic_go_ok";
}
/**
* 将从指定的topic里面获取到的comment进行按floor快速排序
*
* @param list
* @param first
* @param end
*/
public void QuickSort(List<Comments> list, int first, int end) {
/* 初始化各变量 */
int i = first;
int j = end;
Comments temp = list.get(i); // 用子表的第一个记录作为枢轴记录
while (i < j) { // 从表的两端交替向中间扫描
while (i < j && temp.getFloor() <= list.get(j).getFloor())
j--;
list.set(i, list.get(j)); // 采用替换而不是交换的方式进行操作
while (i < j && list.get(i).getFloor() <= temp.getFloor())
i++;
list.set(j, list.get(i)); // 采用替换而不是交换的方式进行操作
}
list.set(i, temp); // 将枢轴数值替换回L->R[i]
if (first < i - 1)
QuickSort(list, first, i - 1); // 对低子表进行递归
if (i + 1 < end)
QuickSort(list, i + 1, end); // 对高子表进行递归
}
/**
* 将从指定的topic里面获取到的comment进行分页处理
*
* @return
*/
public Pages QueryCommentsForPage(int pageSize, int nowPage,
List<Comments> listComment) {
int allRecords = listComment.size();
int totalPage = Pages.calculateTotalPage(pageSize, allRecords);// 总页数
final int currentoffset = Pages.currentPage_startRecord(pageSize,
nowPage);// 当前页的开始记录
final int length = pageSize;
final int currentPage = Pages.judgeCurrentPage(nowPage);
int toIndex = 0;
if (allRecords >= length + currentoffset) {
toIndex = currentoffset + length;
} else {
toIndex = allRecords;
}
List<Comments> subListComment = listComment.subList(currentoffset,
toIndex);
Pages pagebean = new Pages();
pagebean.setPageSize(pageSize);
pagebean.setAllRecords(allRecords);
pagebean.setCurrentPage(currentPage);
pagebean.setTotalPages(totalPage);
pagebean.setListComments(subListComment);
pagebean.init();
return pagebean;
}
public String GoEnd() throws Exception {
this.topic = this.topicService.find(topic.getId());
List<Comments> list = new ArrayList<Comments>();
list.addAll(this.topic.getTopicComments());
/* 先将list按楼层排序 */
if (list.size() > 1) {
this.QuickSort(list, 0, list.size() - 1);
}
this.listComment = list;
return "topic_goEnd_ok";
}
public String EndTopic() throws Exception {
//Users user = (Users) session.getAttribute("tu");
String listfloor = request.getParameter("listFloor");
String[] str = listfloor.split(",");
int[] listFloor = new int[str.length];
for (int i = 0; i < listFloor.length; i++) {
listFloor[i] = Integer.parseInt(str[i]);
}
Topics top = this.topicService.find(topic.getId());
Users user = top.getTopicsUser();
List<Comments> list = new ArrayList<Comments>();
List<Comments> listTemp = new ArrayList<Comments>();
listTemp.addAll(top.getTopicComments());
for (int i = 0; i < listTemp.size(); i++) {
int m = listTemp.get(i).getCommentsUser().getId();
int n = top.getTopicsUser().getId();
if (m != n) {
list.add(listTemp.get(i));
}
}
/* 先将list按楼层排序 */
if (list.size() > 1) {
this.QuickSort(list, 0, list.size() - 1);
}
this.listComment = list;
this.topicService.endTopic(listFloor, listComment);
user.setIntegral(user.getIntegral()+top.getIntegral()*20/100);
top.setTopicsUser(user);
top.setStatus(1);
this.topicService.update(top);
return "topic_end_ok";
}
public String getIndexHotTopic() throws Exception {
this.listTopic = this.topicService.getIndexHotTopic(10);
return "topic_getIndexHot_ok";
}
public String getIndexNiceTopic() throws Exception {
this.listTopic = this.topicService.getIndexNiceTopic(10);
return "topic_getIndexNice_ok";
}
public String getIndexFreshTopic() throws Exception {
this.listTopic = this.topicService.getIndexFreshTopic(10);
return "topic_getIndexFresh_ok";
}
public String getAllTopic() throws Exception {
this.pageBean = this.topicService.getAllForPages(10, nowPage);
this.listTopic = pageBean.getListTopics();
return "topic_getALL_ok";
}
public String GetHotTopic() throws Exception {
this.pageBean = this.topicService.getHotForPages(10, nowPage);
this.listTopic = pageBean.getListTopics();
return "topic_getHot_ok";
}
public String GetNiceTopic() throws Exception {
this.pageBean = this.topicService.getNiceForPages(10, nowPage);
this.listTopic = pageBean.getListTopics();
return "topic_getNice_ok";
}
public String Search() throws Exception {
this.pageBean = this.topicService.search(content, 5, nowPage);
this.listTopic = pageBean.getListTopics();
System.out.println(listTopic);
return "topic_search_ok";
}
public String goTopicByNews() throws Exception {
Users user = (Users) session.getAttribute("tu");
this.topicService.updateNews(this.tnew, user);
Topics top = this.topicService.find(topic.getId());
List<Comments> list = new ArrayList<Comments>();
List<Comments> listTemp = new ArrayList<Comments>();
listTemp.addAll(top.getTopicComments());
if (this.comFlag == 0) {
for (int i = 0; i < listTemp.size(); i++) {
if (listTemp.get(i).getStatus() == 0) {
list.add(listTemp.get(i));
}
}
} else if (this.comFlag == 2) {
for (int i = 0; i < listTemp.size(); i++) {
if (listTemp.get(i).getCommentsUser().getId() == top
.getT
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、项目简介 本项目是一套基于spring+jsp+mysql实现的Java web论坛系统,主要针对计算机相关专业的正在做毕设的学生与需要项目实战练习的Java学习者。 包含:项目源码、数据库脚本等,该项目附带全部源码可作为毕设使用。 项目都经过严格调试,eclipse 确保可以运行! 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值 二、技术实现 后端:spring,mysql,面向对象 前端采用:jsp,jquery,css 运行环境及开发工具:jdk8,idea或者eclipse,Navicat 三、系统功能 论坛系统分为前后台功能 前台管理功能:包括 用户的登录和注册功能,最热门帖子排行榜列表,可以发布帖子和回复帖子,消息通知,修改资料,修改密码等功能 后台管理功能:包括用户管理,帖子功能,版块管理,公告管理,帮助功能等 四、文件里面含有运行指导视频 https://blog.csdn.net/weixin_43860634/article/details/125534777
资源推荐
资源详情
资源评论
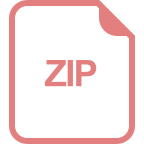
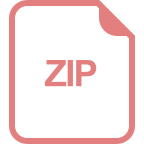
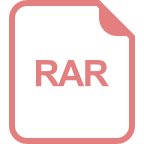
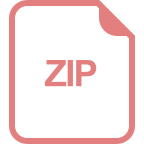
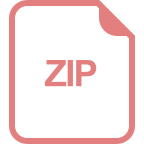
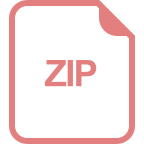
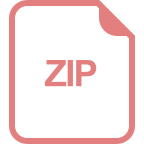
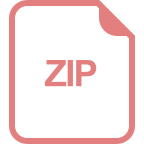
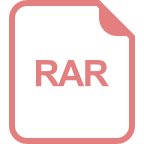
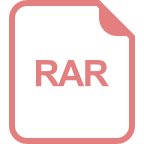
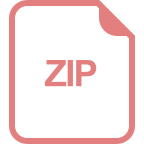
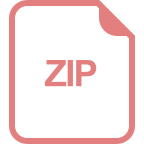
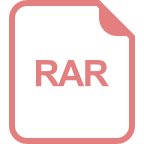
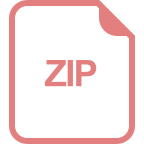
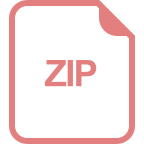
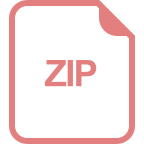
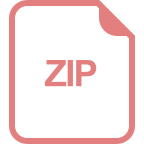
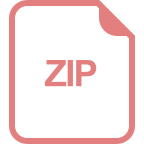
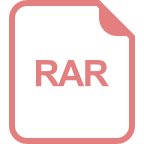
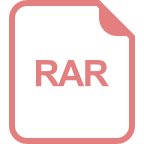
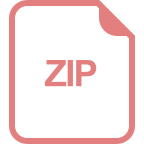
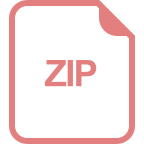
收起资源包目录

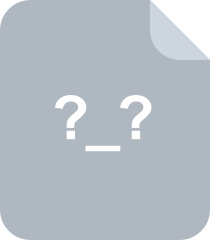
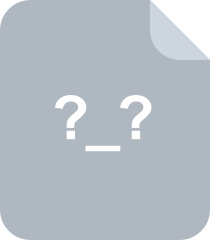
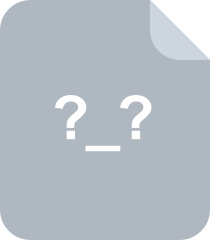
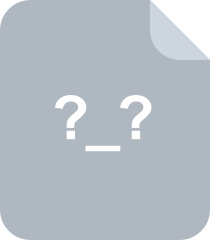
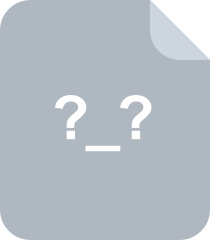
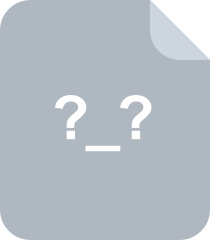
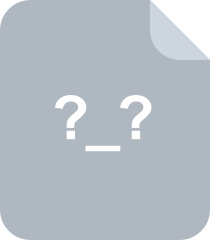
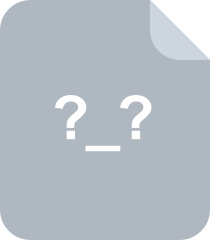
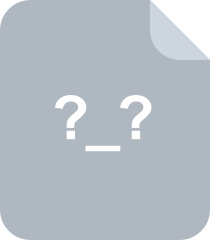
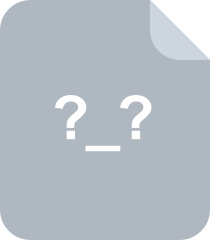
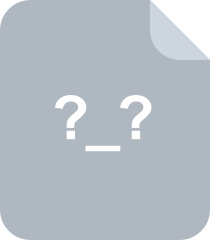
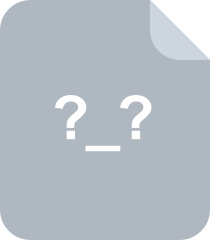
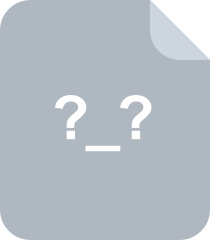
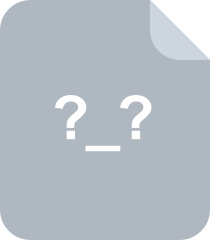
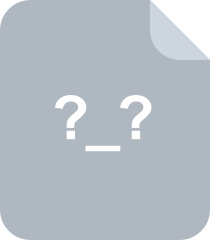
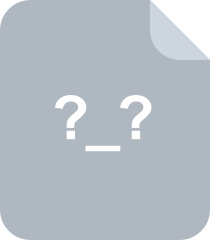
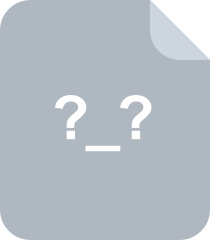
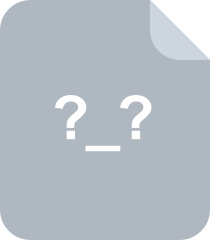
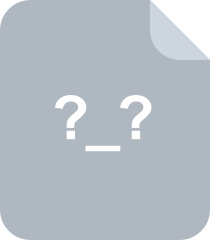
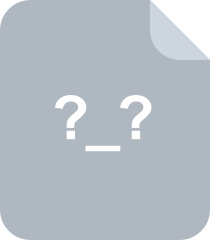
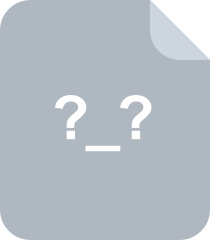
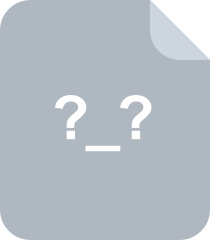
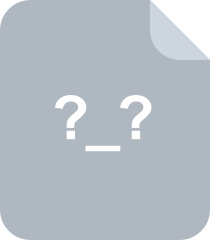
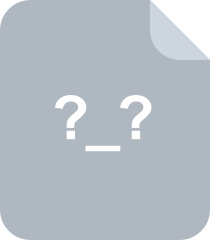
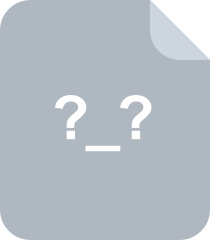
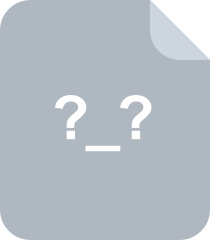
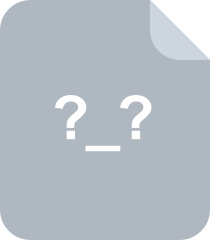
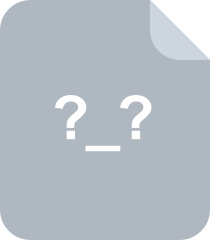
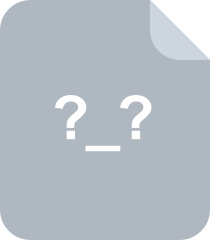
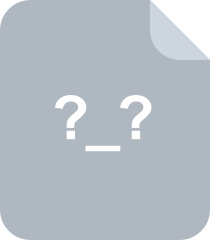
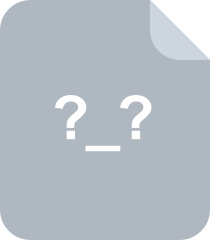
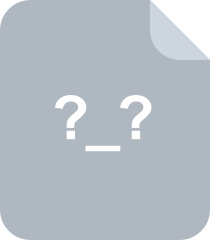
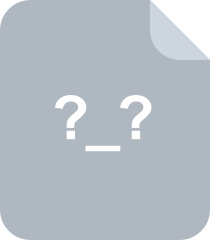
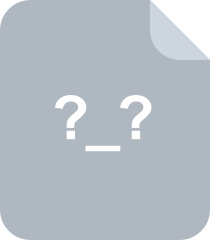
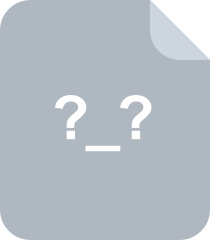
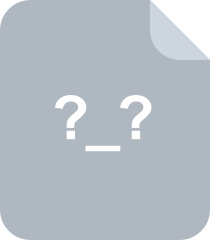
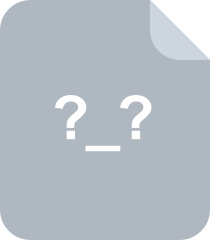
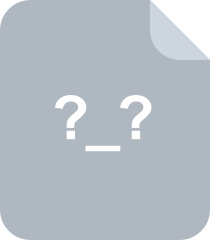
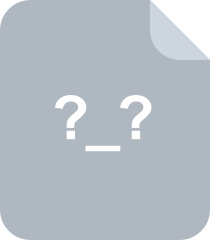
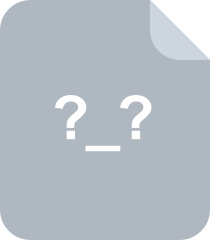
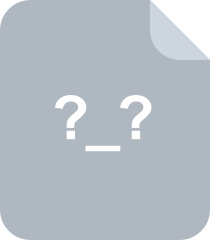
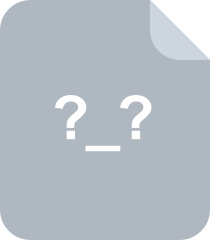
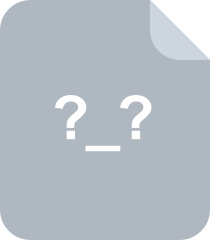
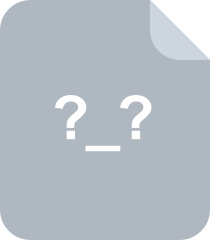
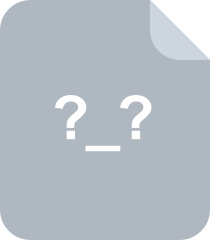
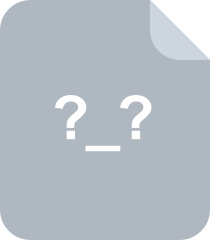
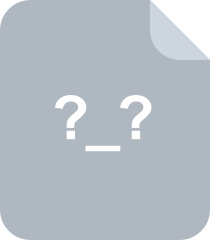
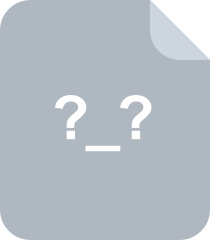
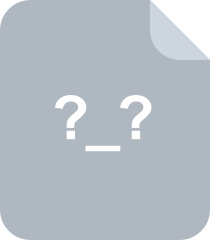
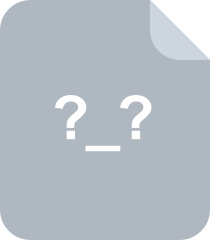
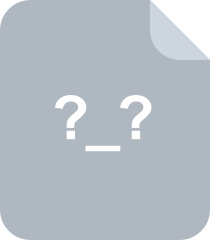
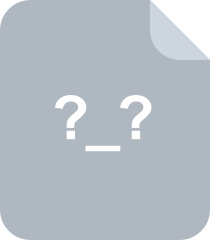
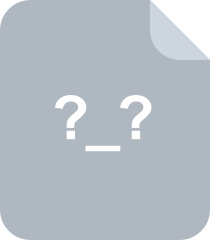
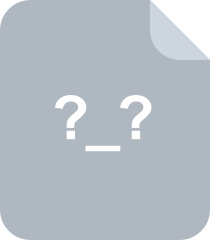
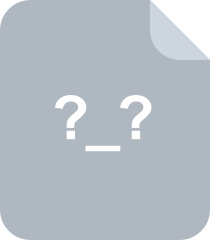
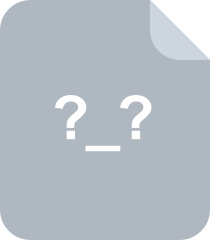
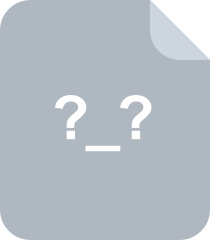
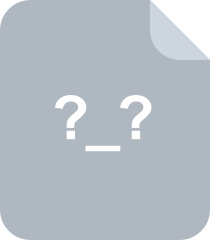
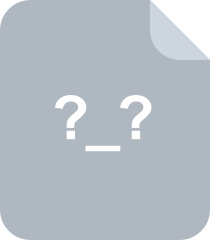
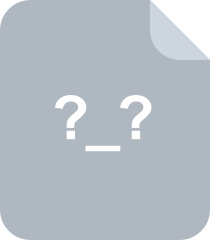
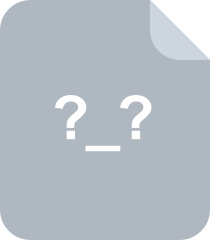
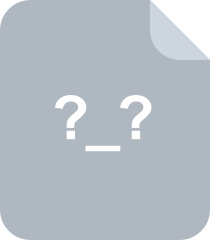
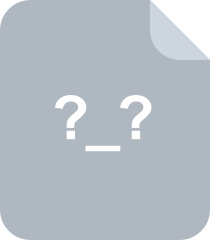
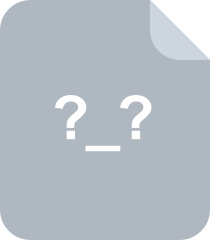
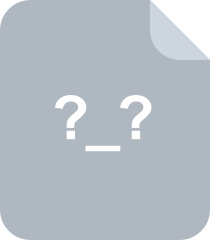
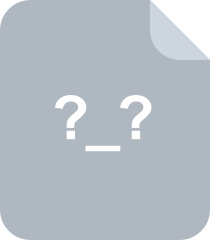
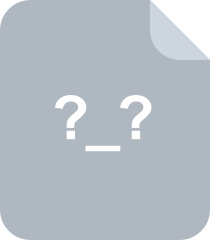
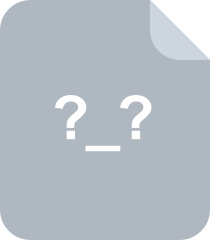
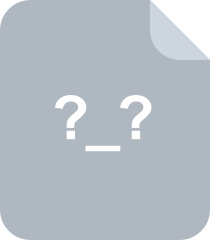
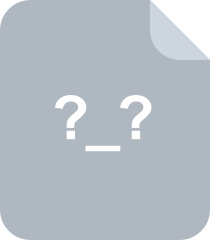
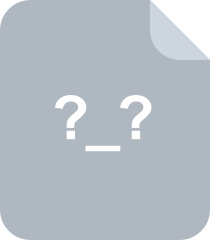
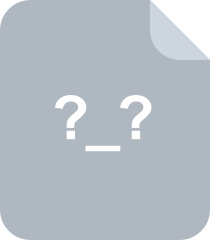
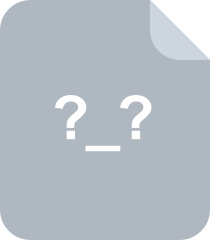
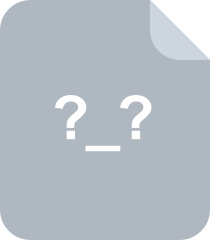
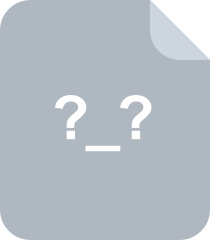
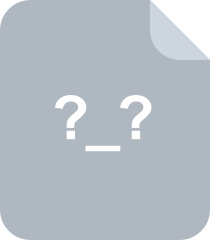
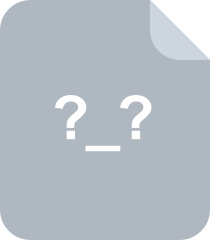
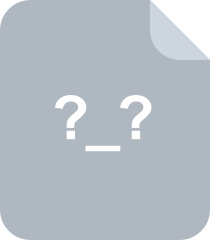
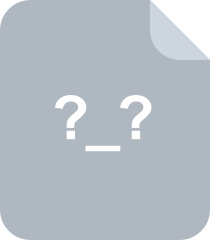
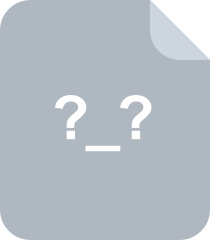
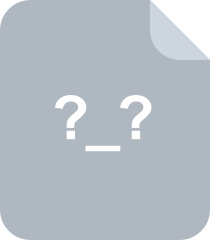
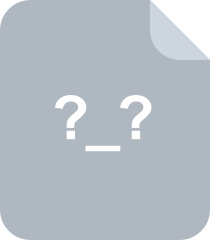
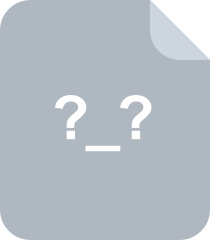
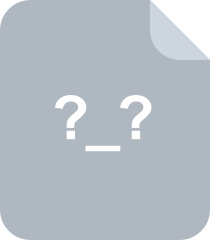
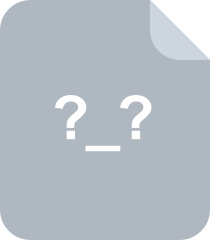
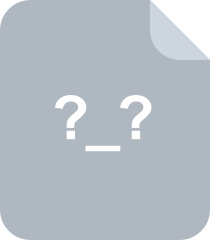
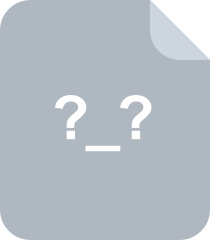
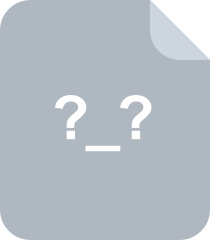
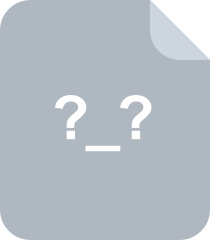
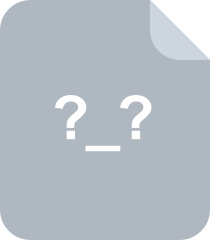
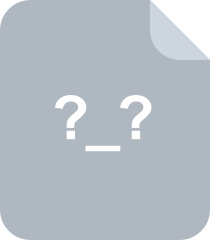
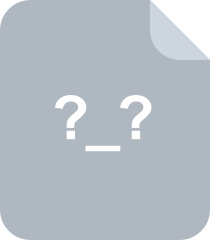
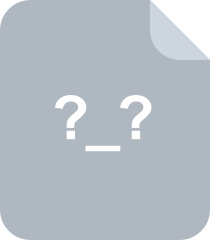
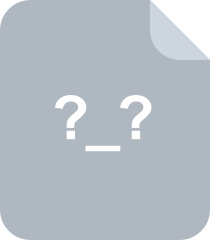
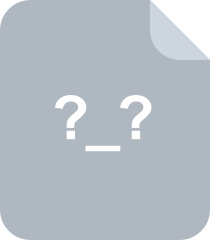
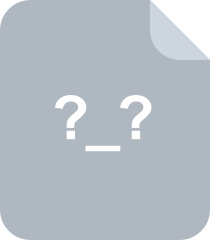
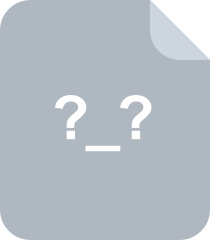
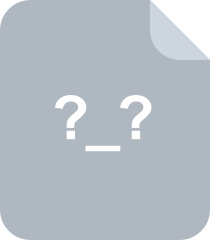
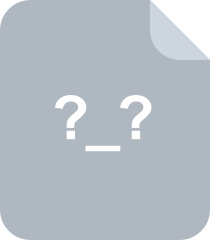
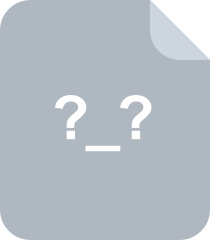
共 595 条
- 1
- 2
- 3
- 4
- 5
- 6
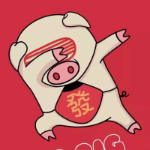
飞翔的佩奇
- 粉丝: 6103
- 资源: 1603
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

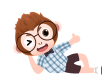
最新资源
- (源码)基于Redis和Elasticsearch的日志与指标处理系统.zip
- 学习记录111111111111111111111111
- (源码)基于Python和Selenium的jksb系统健康申报助手.zip
- (源码)基于HiEasyX库的学习工具系统.zip
- (源码)基于JSP+Servlet+JDBC的学生宿舍管理系统.zip
- (源码)基于Arduino和Raspberry Pi的自动化花园系统.zip
- (源码)基于JSP和Servlet的数据库管理系统.zip
- (源码)基于Python的文本相似度计算系统.zip
- (源码)基于Spring Boot和Redis的高并发秒杀系统.zip
- (源码)基于Java的Web汽车销售管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


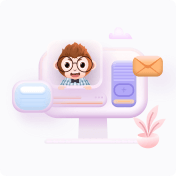
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页