# The `test` npm package
[](https://github.com/nodejs/node-core-test/actions/workflows/ci.yml)
This is a user-land port of [`node:test`](https://nodejs.org/api/test.html),
the experimental test runner introduced in Node.js 18. This module makes it
available in Node.js 14 and later.
Minimal dependencies, with full test suite.
Differences from the core implementation:
- Doesn't hide its own stack frames.
- Some features require the use of `--experimental-abortcontroller` CLI flag to
work on Node.js v14.x. It's recommended to pass
`NODE_OPTIONS='--experimental-abortcontroller --no-warnings'` in your env if
you are testing on v14.x.
## Docs
### Test runner
> Stability: 1 - Experimental
<!-- source_link=lib/test.js -->
The `node:test` module facilitates the creation of JavaScript tests.
To access it:
```mjs
import test from 'test'
```
```cjs
const test = require('test')
```
Tests created via the `test` module consist of a single function that is
processed in one of three ways:
1. A synchronous function that is considered failing if it throws an exception,
and is considered passing otherwise.
2. A function that returns a `Promise` that is considered failing if the
`Promise` rejects, and is considered passing if the `Promise` resolves.
3. A function that receives a callback function. If the callback receives any
truthy value as its first argument, the test is considered failing. If a
falsy value is passed as the first argument to the callback, the test is
considered passing. If the test function receives a callback function and
also returns a `Promise`, the test will fail.
The following example illustrates how tests are written using the
`test` module.
```js
test('synchronous passing test', t => {
// This test passes because it does not throw an exception.
assert.strictEqual(1, 1)
})
test('synchronous failing test', t => {
// This test fails because it throws an exception.
assert.strictEqual(1, 2)
})
test('asynchronous passing test', async t => {
// This test passes because the Promise returned by the async
// function is not rejected.
assert.strictEqual(1, 1)
})
test('asynchronous failing test', async t => {
// This test fails because the Promise returned by the async
// function is rejected.
assert.strictEqual(1, 2)
})
test('failing test using Promises', t => {
// Promises can be used directly as well.
return new Promise((resolve, reject) => {
setImmediate(() => {
reject(new Error('this will cause the test to fail'))
})
})
})
test('callback passing test', (t, done) => {
// done() is the callback function. When the setImmediate() runs, it invokes
// done() with no arguments.
setImmediate(done)
})
test('callback failing test', (t, done) => {
// When the setImmediate() runs, done() is invoked with an Error object and
// the test fails.
setImmediate(() => {
done(new Error('callback failure'))
})
})
```
If any tests fail, the process exit code is set to `1`.
#### Subtests
The test context's `test()` method allows subtests to be created. This method
behaves identically to the top level `test()` function. The following example
demonstrates the creation of a top level test with two subtests.
```js
test('top level test', async t => {
await t.test('subtest 1', t => {
assert.strictEqual(1, 1)
})
await t.test('subtest 2', t => {
assert.strictEqual(2, 2)
})
})
```
In this example, `await` is used to ensure that both subtests have completed.
This is necessary because parent tests do not wait for their subtests to
complete. Any subtests that are still outstanding when their parent finishes
are cancelled and treated as failures. Any subtest failures cause the parent
test to fail.
## Skipping tests
Individual tests can be skipped by passing the `skip` option to the test, or by
calling the test context's `skip()` method as shown in the
following example.
```js
// The skip option is used, but no message is provided.
test('skip option', { skip: true }, t => {
// This code is never executed.
})
// The skip option is used, and a message is provided.
test('skip option with message', { skip: 'this is skipped' }, t => {
// This code is never executed.
})
test('skip() method', t => {
// Make sure to return here as well if the test contains additional logic.
t.skip()
})
test('skip() method with message', t => {
// Make sure to return here as well if the test contains additional logic.
t.skip('this is skipped')
})
```
## `describe`/`it` syntax
Running tests can also be done using `describe` to declare a suite
and `it` to declare a test.
A suite is used to organize and group related tests together.
`it` is an alias for `test`, except there is no test context passed,
since nesting is done using suites.
```js
describe('A thing', () => {
it('should work', () => {
assert.strictEqual(1, 1);
});
it('should be ok', () => {
assert.strictEqual(2, 2);
});
describe('a nested thing', () => {
it('should work', () => {
assert.strictEqual(3, 3);
});
});
});
```
`describe` and `it` are imported from the `test` module.
```mjs
import { describe, it } from 'test';
```
```cjs
const { describe, it } = require('test');
```
### `only` tests
If `node--test` is started with the `--test-only` command-line option, it is
possible to skip all top level tests except for a selected subset by passing
the `only` option to the tests that should be run. When a test with the `only`
option set is run, all subtests are also run. The test context's `runOnly()`
method can be used to implement the same behavior at the subtest level.
```js
// Assume node--test is run with the --test-only command-line option.
// The 'only' option is set, so this test is run.
test('this test is run', { only: true }, async t => {
// Within this test, all subtests are run by default.
await t.test('running subtest')
// The test context can be updated to run subtests with the 'only' option.
t.runOnly(true)
await t.test('this subtest is now skipped')
await t.test('this subtest is run', { only: true })
// Switch the context back to execute all tests.
t.runOnly(false)
await t.test('this subtest is now run')
// Explicitly do not run these tests.
await t.test('skipped subtest 3', { only: false })
await t.test('skipped subtest 4', { skip: true })
})
// The 'only' option is not set, so this test is skipped.
test('this test is not run', () => {
// This code is not run.
throw new Error('fail')
})
```
## Filtering tests by name
The [`--test-name-pattern`][] command-line option can be used to only run tests
whose name matches the provided pattern. Test name patterns are interpreted as
JavaScript regular expressions. The `--test-name-pattern` option can be
specified multiple times in order to run nested tests. For each test that is
executed, any corresponding test hooks, such as `beforeEach()`, are also
run.
Given the following test file, starting Node.js with the
`--test-name-pattern="test [1-3]"` option would cause the test runner to execute
`test 1`, `test 2`, and `test 3`. If `test 1` did not match the test name
pattern, then its subtests would not execute, despite matching the pattern. The
same set of tests could also be executed by passing `--test-name-pattern`
multiple times (e.g. `--test-name-pattern="test 1"`,
`--test-name-pattern="test 2"`, etc.).
```js
test('test 1', async (t) => {
await t.test('test 2');
await t.test('test 3');
});
test('Test 4', async (t) => {
await t.test('Test 5');
await t.test('test 6');
});
```
Test name patterns can also be specified using regular expression literals. This
allows regular expression flags to be used. In the previous example, starting
Node.js with `--test-name-pattern="/test [4-5]/i"` would match `Test 4` and
`Test 5` because the pattern is case-insensitive.
Test name patterns do not change the set
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
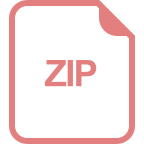
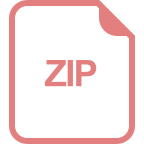
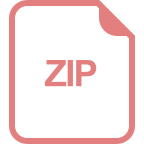
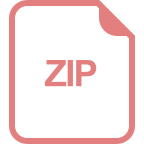
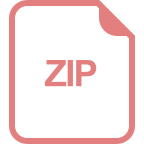
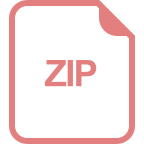
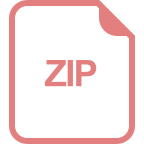
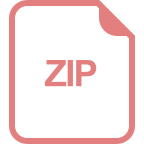
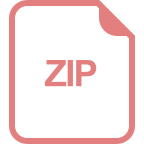
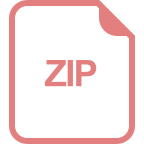
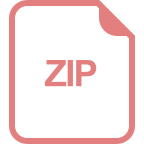
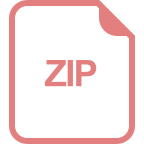
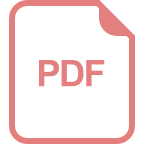
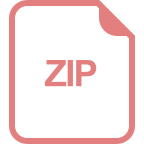
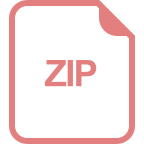
收起资源包目录

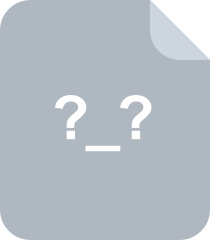
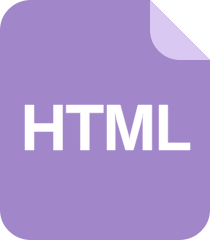
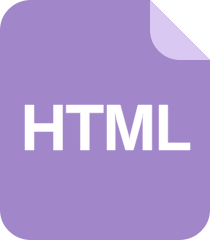
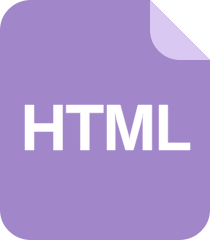
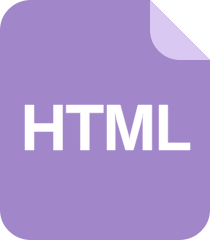
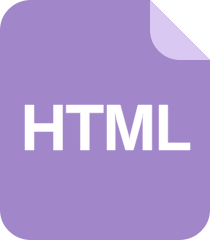
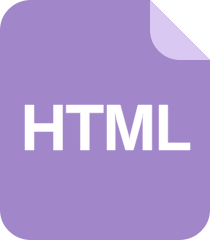
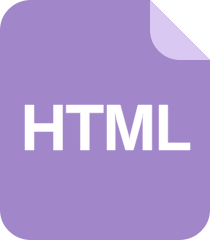
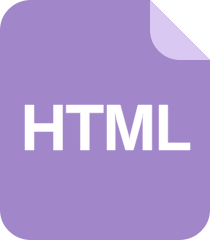
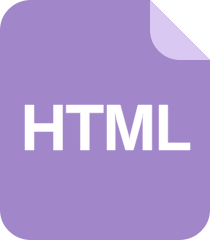
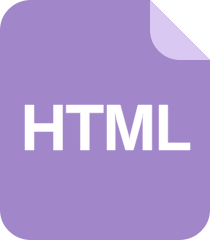
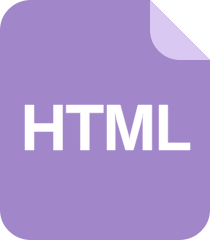
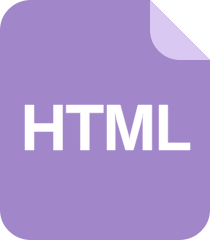
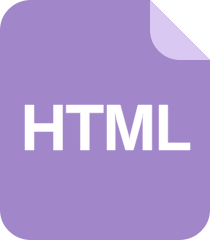
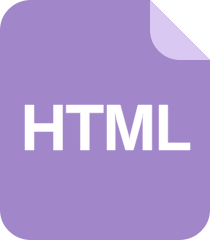
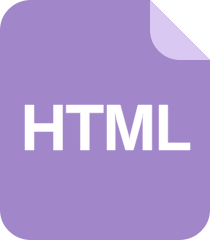
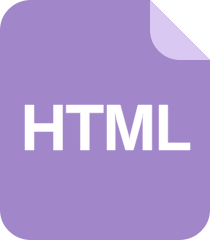
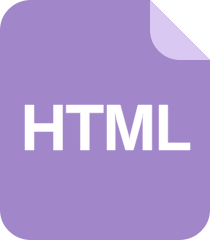
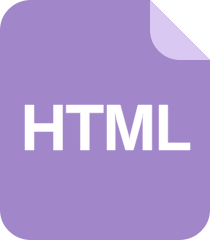
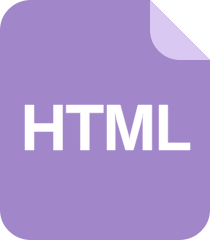
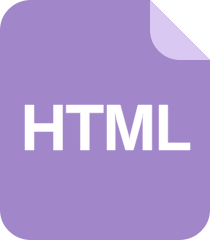
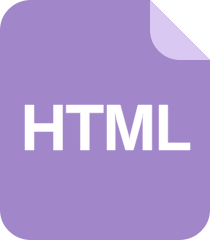
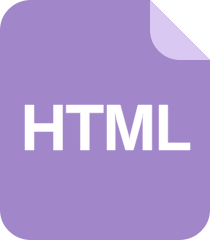
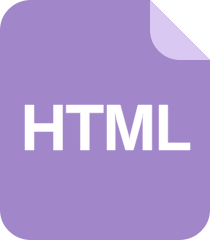
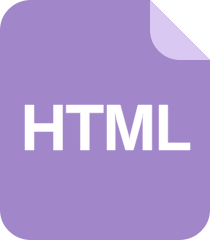
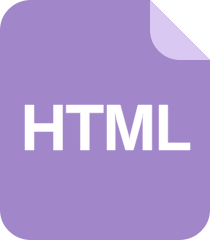
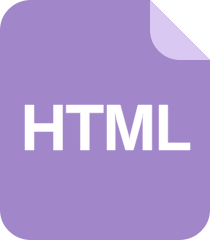
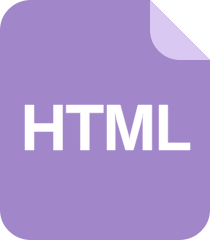
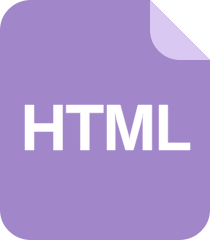
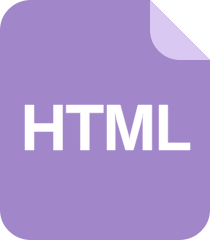
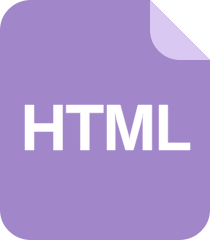
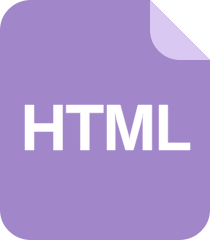
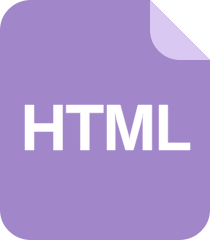
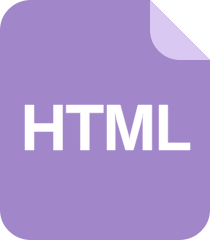
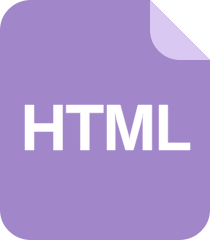
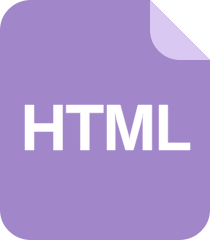
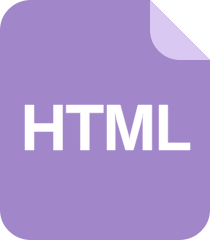
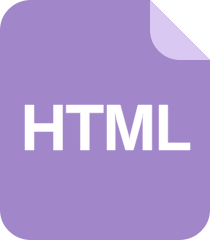
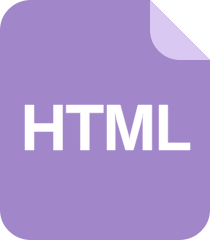
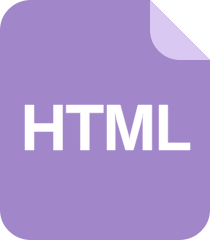
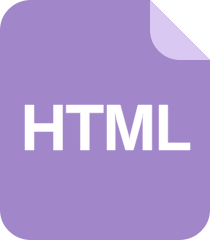
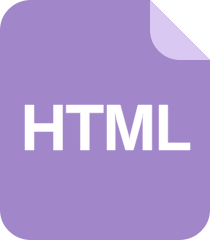
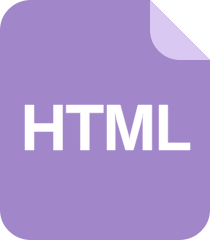
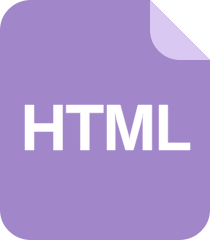
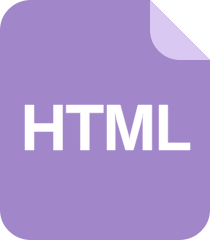
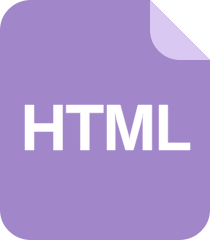
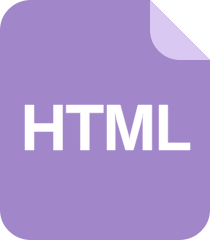
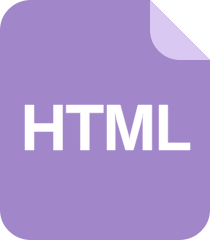
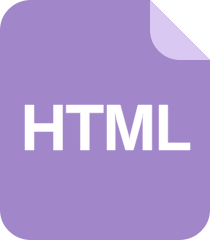
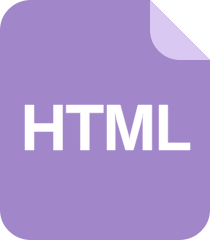
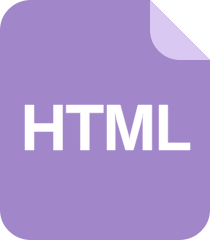
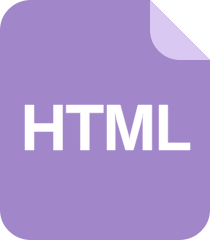
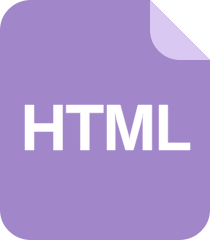
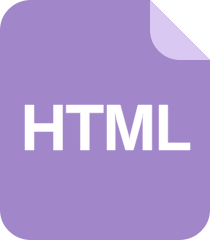
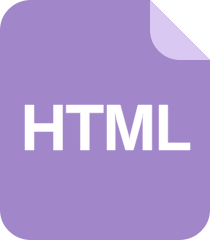
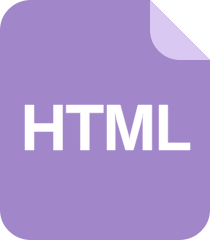
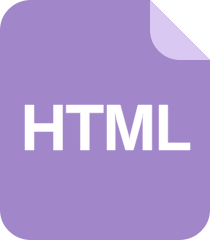
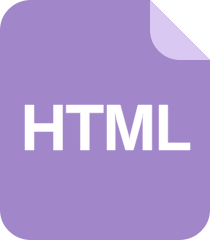
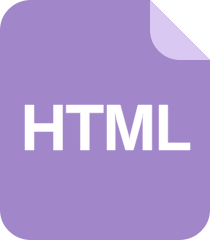
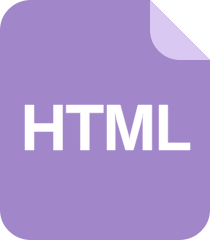
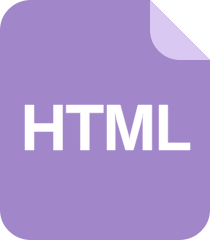
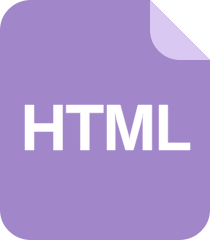
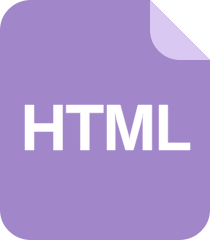
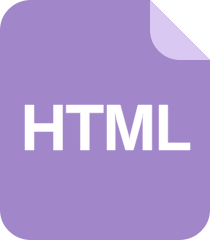
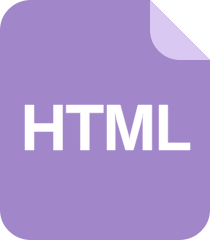
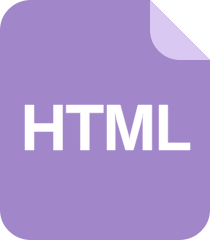
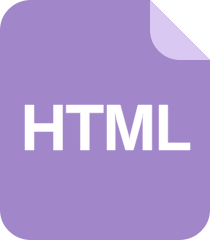
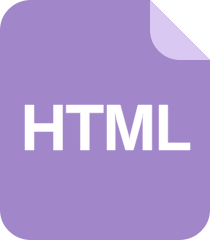
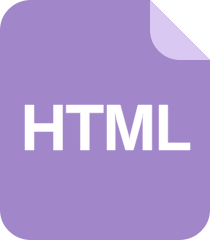
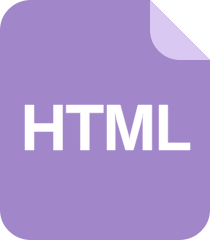
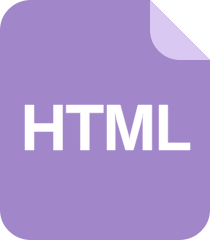
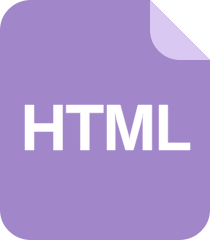
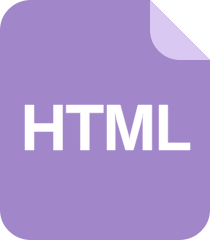
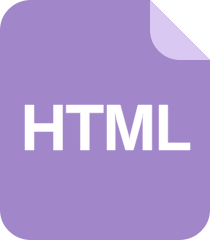
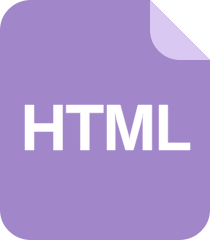
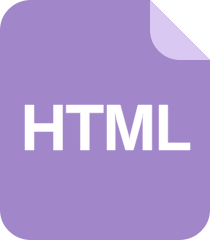
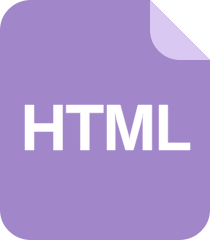
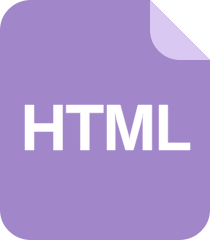
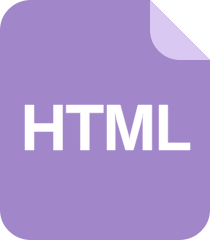
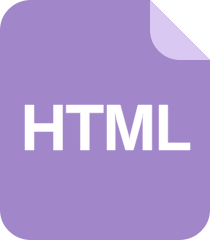
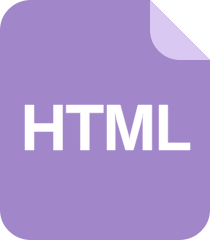
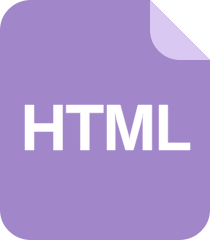
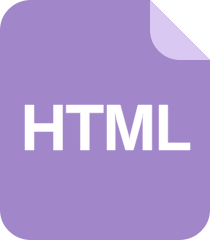
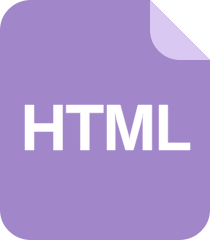
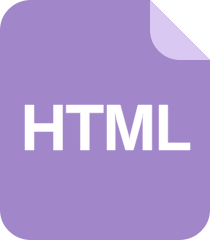
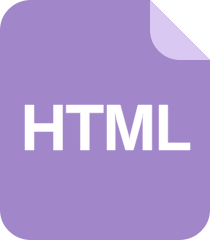
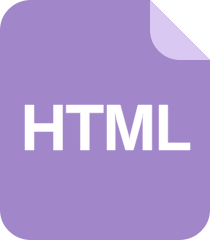
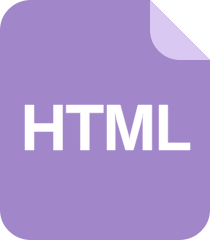
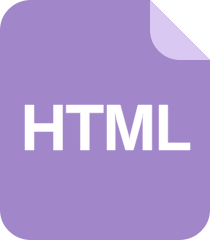
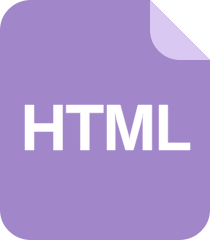
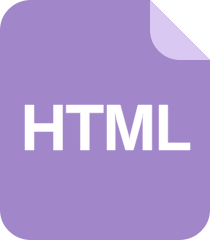
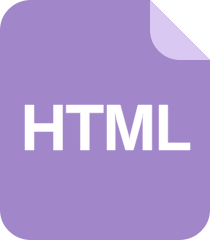
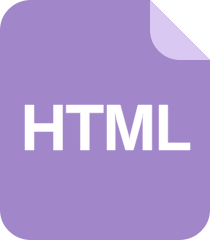
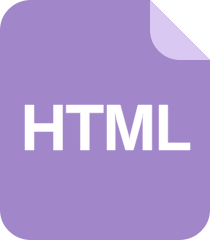
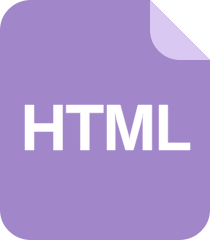
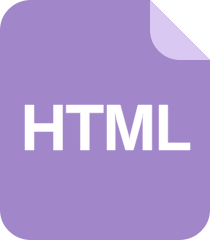
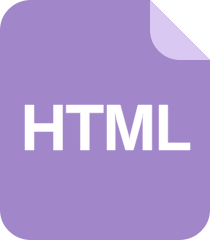
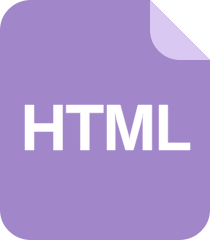
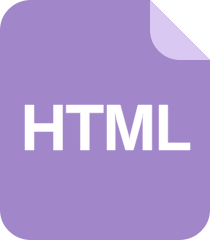
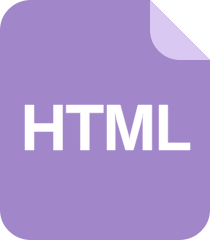
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
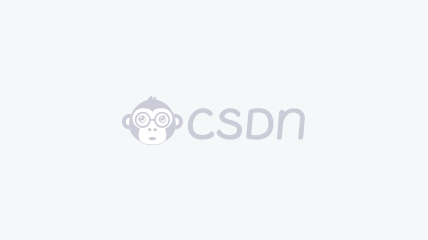

YunFeiDong
- 粉丝: 152
- 资源: 3930
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

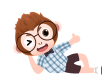
最新资源
- (完整)数据库课程设计餐厅点餐说明书-21ab6d3c8beb172ded630b1c59eef8c75ebf952c.doc
- 2023-04-06-项目笔记 - 第一百五十四阶段 - 4.4.2.152全局变量的作用域-152 -2024.06.04
- 松哥解协议松哥解协议松哥解协议松哥解协议松哥解协议
- 618节日618节日618节日
- tensorflow-gpu-2.9.1-cp37-cp37m-win-amd64.whl
- tensorflow-gpu-2.9.0-cp37-cp37m-win-amd64.whl
- tensorflow-gpu-2.9.0-cp39-cp39-win-amd64.whl
- lcd daimalcd daima
- 电影领域-推荐算法-个性化内容-观影决策-电影推荐小程序.zip
- 电气控制PLC考试题库
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


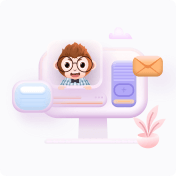
安全验证
文档复制为VIP权益,开通VIP直接复制
