===========
NumPy C-API
===========
::
unsigned int
PyArray_GetNDArrayCVersion(void )
Included at the very first so not auto-grabbed and thus not labeled.
::
int
PyArray_SetNumericOps(PyObject *dict)
Set internal structure with number functions that all arrays will use
::
PyObject *
PyArray_GetNumericOps(void )
Get dictionary showing number functions that all arrays will use
::
int
PyArray_INCREF(PyArrayObject *mp)
For object arrays, increment all internal references.
::
int
PyArray_XDECREF(PyArrayObject *mp)
Decrement all internal references for object arrays.
(or arrays with object fields)
::
void
PyArray_SetStringFunction(PyObject *op, int repr)
Set the array print function to be a Python function.
::
PyArray_Descr *
PyArray_DescrFromType(int type)
Get the PyArray_Descr structure for a type.
::
PyObject *
PyArray_TypeObjectFromType(int type)
Get a typeobject from a type-number -- can return NULL.
New reference
::
char *
PyArray_Zero(PyArrayObject *arr)
Get pointer to zero of correct type for array.
::
char *
PyArray_One(PyArrayObject *arr)
Get pointer to one of correct type for array
::
PyObject *
PyArray_CastToType(PyArrayObject *arr, PyArray_Descr *dtype, int
is_f_order)
For backward compatibility
Cast an array using typecode structure.
steals reference to dtype --- cannot be NULL
This function always makes a copy of arr, even if the dtype
doesn't change.
::
int
PyArray_CastTo(PyArrayObject *out, PyArrayObject *mp)
Cast to an already created array.
::
int
PyArray_CastAnyTo(PyArrayObject *out, PyArrayObject *mp)
Cast to an already created array. Arrays don't have to be "broadcastable"
Only requirement is they have the same number of elements.
::
int
PyArray_CanCastSafely(int fromtype, int totype)
Check the type coercion rules.
::
npy_bool
PyArray_CanCastTo(PyArray_Descr *from, PyArray_Descr *to)
leaves reference count alone --- cannot be NULL
PyArray_CanCastTypeTo is equivalent to this, but adds a 'casting'
parameter.
::
int
PyArray_ObjectType(PyObject *op, int minimum_type)
Return the typecode of the array a Python object would be converted to
Returns the type number the result should have, or NPY_NOTYPE on error.
::
PyArray_Descr *
PyArray_DescrFromObject(PyObject *op, PyArray_Descr *mintype)
new reference -- accepts NULL for mintype
::
PyArrayObject **
PyArray_ConvertToCommonType(PyObject *op, int *retn)
This function is only used in one place within NumPy and should
generally be avoided. It is provided mainly for backward compatibility.
The user of the function has to free the returned array.
::
PyArray_Descr *
PyArray_DescrFromScalar(PyObject *sc)
Return descr object from array scalar.
New reference
::
PyArray_Descr *
PyArray_DescrFromTypeObject(PyObject *type)
::
npy_intp
PyArray_Size(PyObject *op)
Compute the size of an array (in number of items)
::
PyObject *
PyArray_Scalar(void *data, PyArray_Descr *descr, PyObject *base)
Get scalar-equivalent to a region of memory described by a descriptor.
::
PyObject *
PyArray_FromScalar(PyObject *scalar, PyArray_Descr *outcode)
Get 0-dim array from scalar
0-dim array from array-scalar object
always contains a copy of the data
unless outcode is NULL, it is of void type and the referrer does
not own it either.
steals reference to outcode
::
void
PyArray_ScalarAsCtype(PyObject *scalar, void *ctypeptr)
Convert to c-type
no error checking is performed -- ctypeptr must be same type as scalar
in case of flexible type, the data is not copied
into ctypeptr which is expected to be a pointer to pointer
::
int
PyArray_CastScalarToCtype(PyObject *scalar, void
*ctypeptr, PyArray_Descr *outcode)
Cast Scalar to c-type
The output buffer must be large-enough to receive the value
Even for flexible types which is different from ScalarAsCtype
where only a reference for flexible types is returned
This may not work right on narrow builds for NumPy unicode scalars.
::
int
PyArray_CastScalarDirect(PyObject *scalar, PyArray_Descr
*indescr, void *ctypeptr, int outtype)
Cast Scalar to c-type
::
PyObject *
PyArray_ScalarFromObject(PyObject *object)
Get an Array Scalar From a Python Object
Returns NULL if unsuccessful but error is only set if another error occurred.
Currently only Numeric-like object supported.
::
PyArray_VectorUnaryFunc *
PyArray_GetCastFunc(PyArray_Descr *descr, int type_num)
Get a cast function to cast from the input descriptor to the
output type_number (must be a registered data-type).
Returns NULL if un-successful.
::
PyObject *
PyArray_FromDims(int NPY_UNUSED(nd) , int *NPY_UNUSED(d) , int
NPY_UNUSED(type) )
Deprecated, use PyArray_SimpleNew instead.
::
PyObject *
PyArray_FromDimsAndDataAndDescr(int NPY_UNUSED(nd) , int
*NPY_UNUSED(d) , PyArray_Descr
*descr, char *NPY_UNUSED(data) )
Deprecated, use PyArray_NewFromDescr instead.
::
PyObject *
PyArray_FromAny(PyObject *op, PyArray_Descr *newtype, int
min_depth, int max_depth, int flags, PyObject
*context)
Does not check for NPY_ARRAY_ENSURECOPY and NPY_ARRAY_NOTSWAPPED in flags
Steals a reference to newtype --- which can be NULL
::
PyObject *
PyArray_EnsureArray(PyObject *op)
This is a quick wrapper around
PyArray_FromAny(op, NULL, 0, 0, NPY_ARRAY_ENSUREARRAY, NULL)
that special cases Arrays and PyArray_Scalars up front
It *steals a reference* to the object
It also guarantees that the result is PyArray_Type
Because it decrefs op if any conversion needs to take place
so it can be used like PyArray_EnsureArray(some_function(...))
::
PyObject *
PyArray_EnsureAnyArray(PyObject *op)
::
PyObject *
PyArray_FromFile(FILE *fp, PyArray_Descr *dtype, npy_intp num, char
*sep)
Given a ``FILE *`` pointer ``fp``, and a ``PyArray_Descr``, return an
array corresponding to the data encoded in that file.
The reference to `dtype` is stolen (it is possible that the passed in
dtype is not held on to).
The number of elements to read is given as ``num``; if it is < 0, then
then as many as possible are read.
If ``sep`` is NULL or empty, then binary data is assumed, else
text data, with ``sep`` as the separator between elements. Whitespace in
the separator matches any length of whitespace in the text, and a match
for whitespace around the separator is added.
For memory-mapped files, use the buffer interface. No more data than
necessary is read by this routine.
::
PyObject *
PyArray_FromString(char *data, npy_intp slen, PyArray_Descr
*dtype, npy_intp num, char *sep)
Given a pointer to a string ``data``, a string length ``slen``, and
a ``PyArray_Descr``, return an array corresponding to the data
encoded in that string.
If the dtype is NULL, the default array type is used (double).
If non-null, the reference is stolen.
If ``slen`` is < 0, then the end of string is used for text data.
It is an error for ``slen`` to be < 0 for binary data (since embedded NULLs
would be the norm).
The number of elements to read is given as ``num``; if it is < 0, then
then as many as possible are read.
If ``sep`` is NULL or empty, then binary data is assumed, else
text data, with ``sep`` as the separator between elements. Whitespace in
the separator matches any length of whitespace in the text, and a match
for whitespace around the separator is added.
::
PyObject *
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
redis desktop manager是一款功能强大的redis数据库管理软件,可以帮助用户轻松快速的查看与操控整个数据库。redis desktop manager不仅拥有十分简洁直观的操作界面,而且所有功能信息一目了然,是广大用户必备的数据库管理神器。 redis desktop manager具有操作简单、方便快捷、功能完善、性能稳定等优点,支持用户采用可视化操作界面对数据库进行各方面工作,不管是新手用户还是专业的开发人员,该软件都是你管理数据库的最佳帮手。
资源详情
资源评论
资源推荐
收起资源包目录

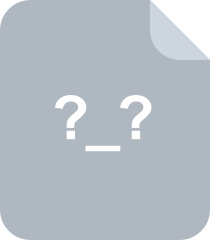
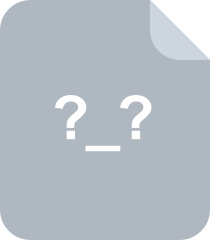
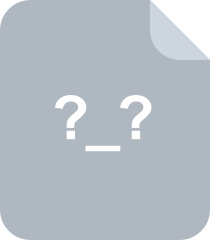
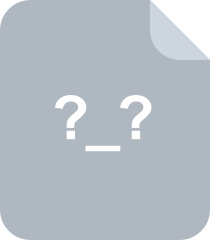
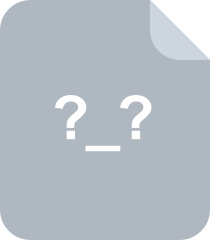
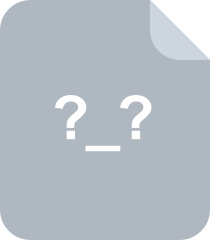
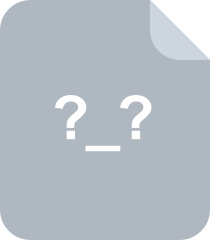
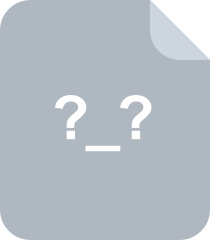
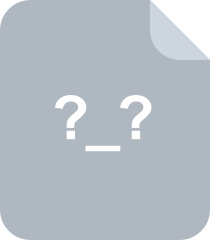
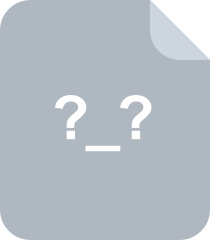
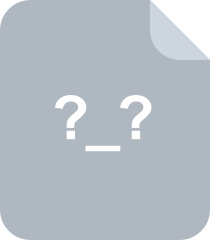
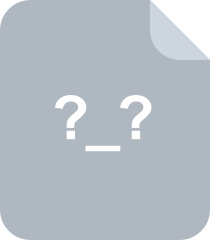
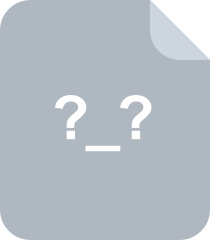
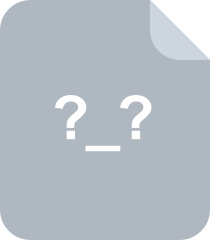
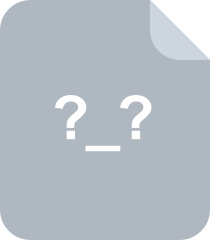
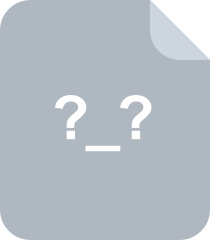
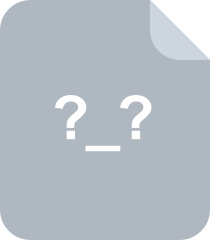
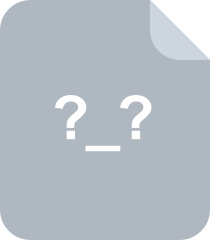
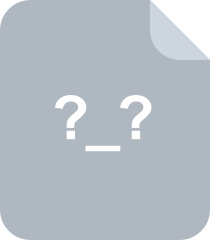
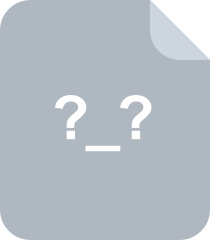
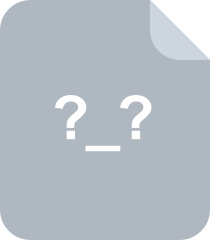
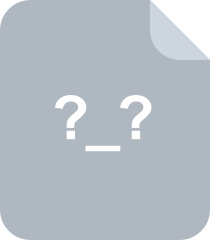
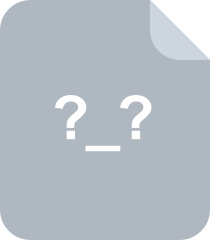
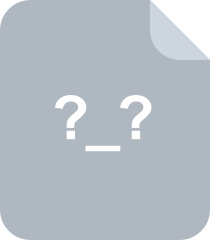
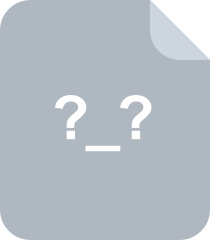
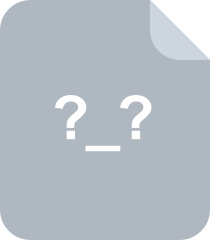
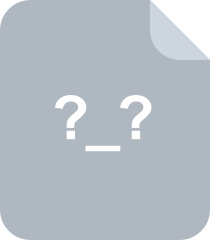
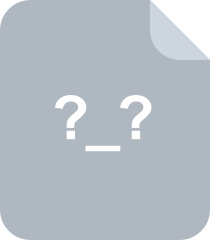
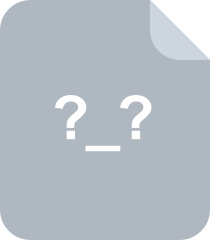
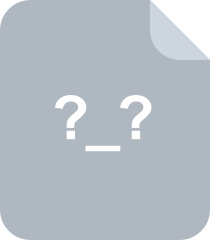
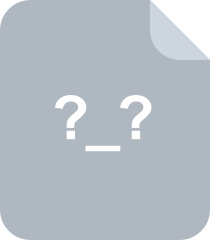
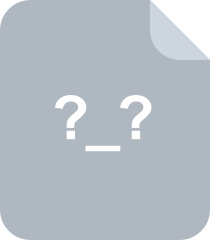
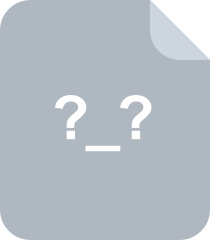
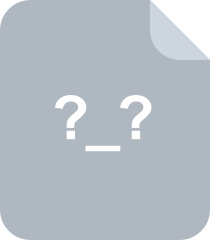
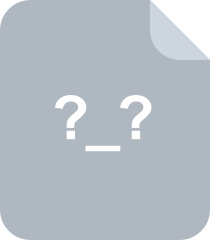
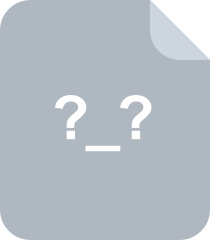
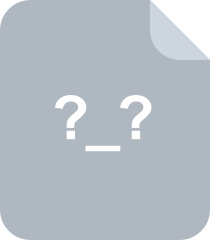
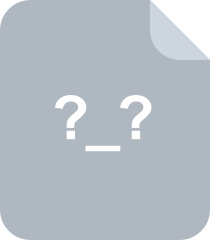
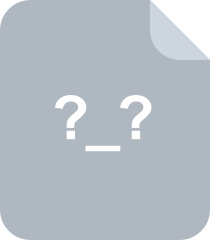
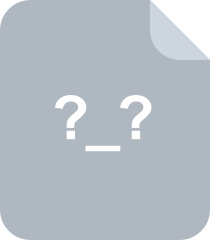
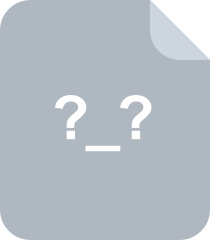
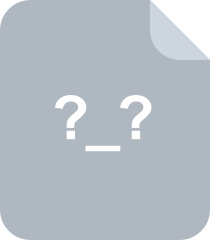
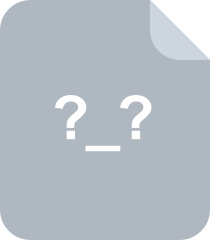
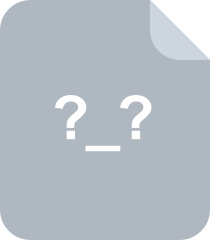
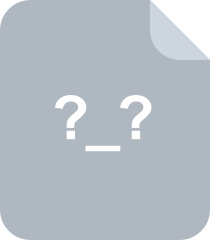
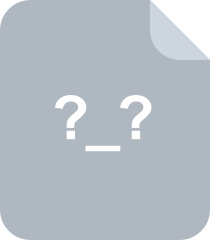
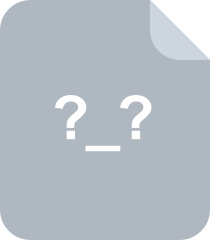
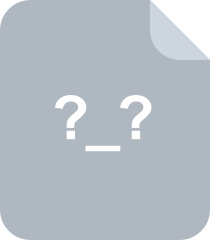
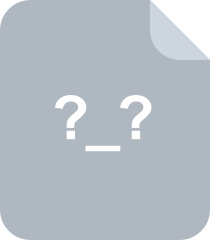
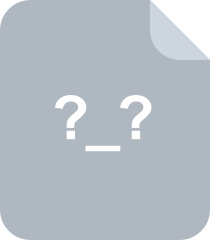
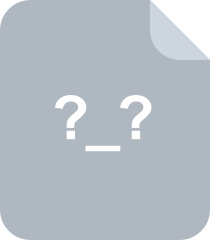
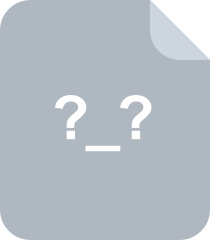
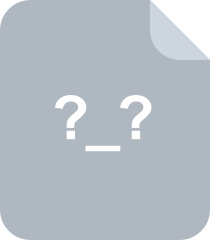
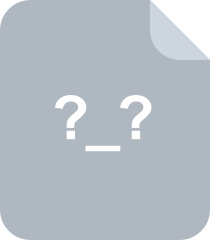
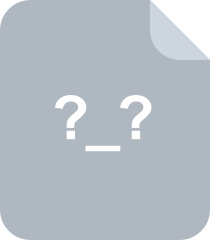
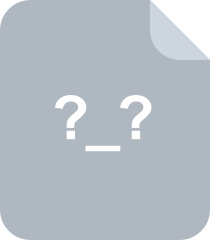
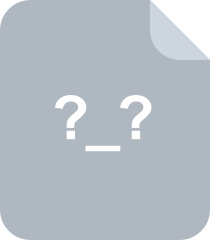
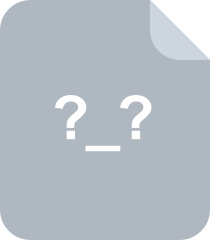
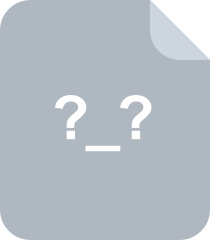
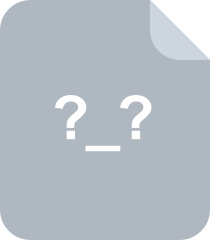
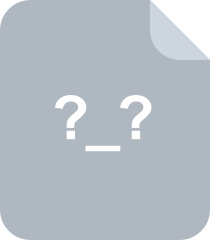
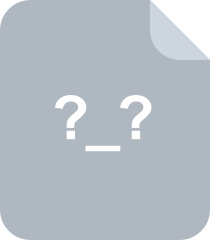
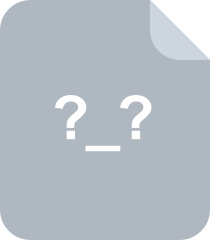
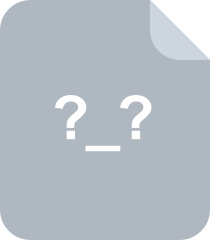
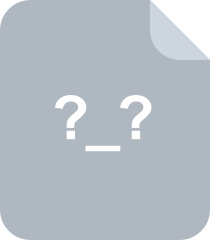
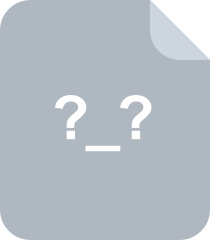
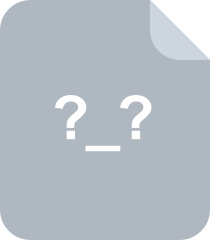
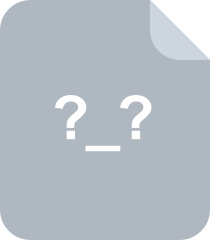
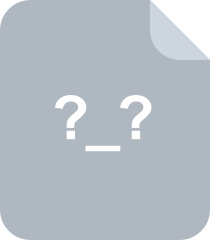
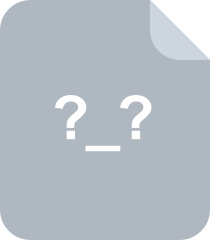
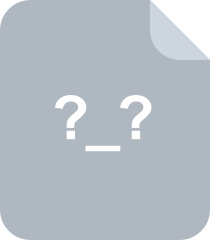
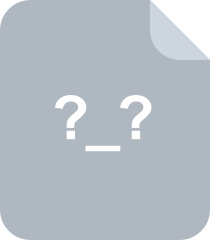
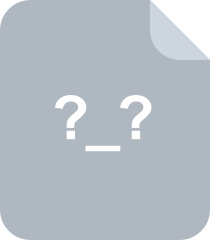
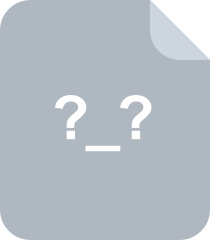
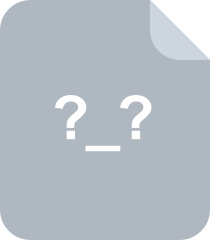
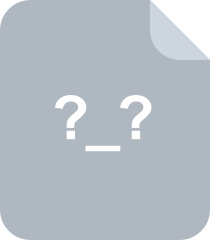
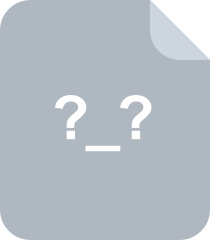
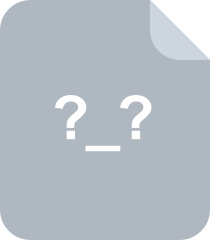
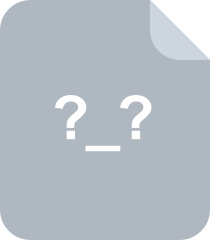
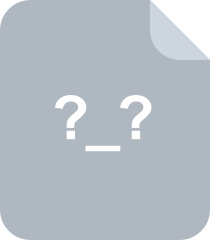
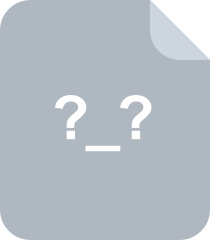
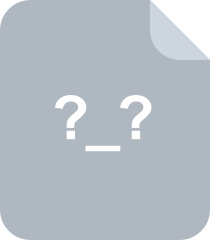
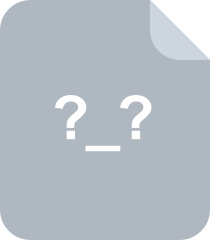
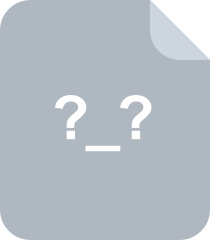
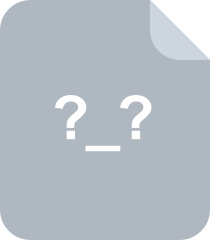
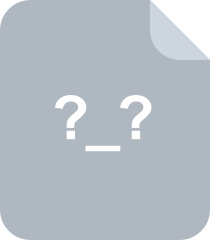
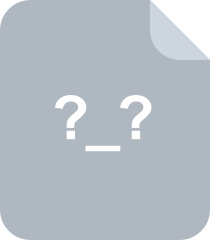
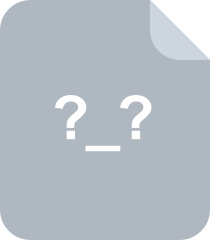
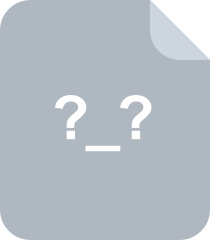
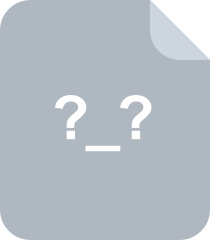
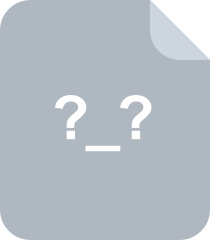
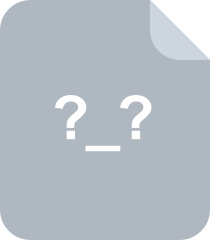
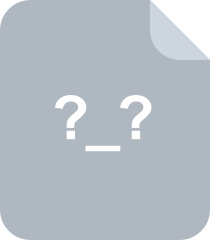
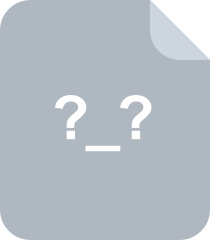
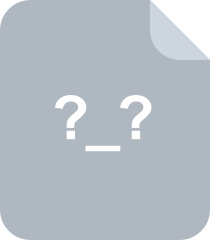
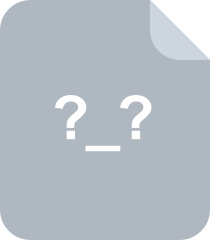
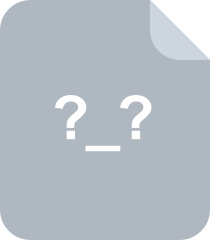
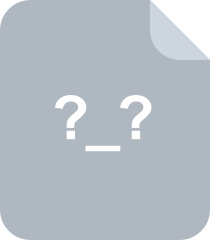
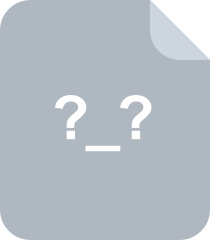
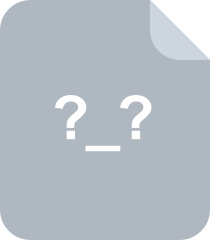
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
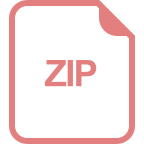
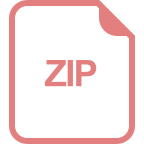
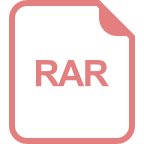
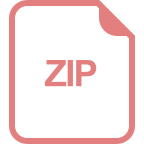
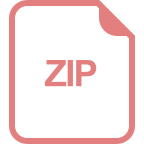
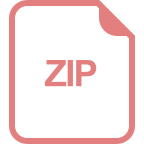
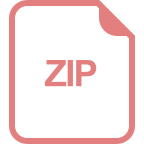
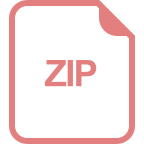
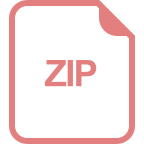
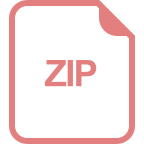
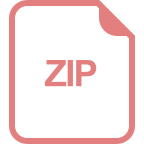
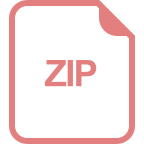
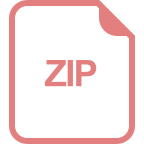
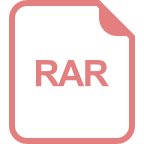
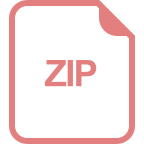
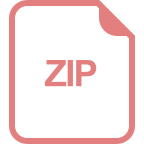
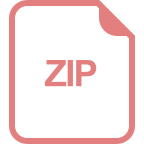
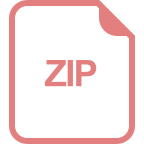
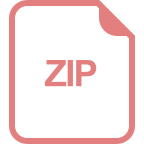
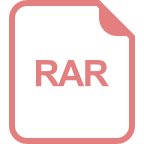
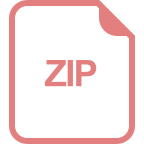
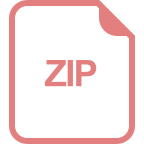
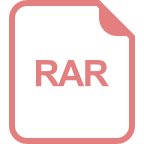

coding部落
- 粉丝: 24
- 资源: 54
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

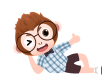
最新资源
- 市建设工程安全生产标准化管理优良工地申报表.docx
- 特殊建设工程消防验收现场评定(其他建设工程消防验收备案现场检查)监督记录表.docx
- 提前报废老旧营运柴油货车补贴标准、新购营运货车补贴标准表.docx
- 基于鸟鸣声识别的鸟类分类系统项目源代码全套技术资料.zip
- 解析XML文件,使用ElementTree模块,并根据流程图设计合适的数据结构保存解析结果-使用Python ElementTree模块解析XML文件并设计数据结构-含源代码及解释
- 膝关节功能丧失程度评定表.docx
- 外出务工就业交通补助申报表.docx
- 腕关节功能丧失程度评定表.docx
- 现场评定检查表—— 防爆.docx
- 现场评定检查表—— 防火分隔、固定窗.docx
- 现场评定检查表——安全疏散.docx
- 现场评定检查表——建筑类别与耐火等级表.docx
- 现场评定检查表——建筑灭火器.docx
- 现场评定检查表--泡沫灭火系统.docx
- 现场评定检查表——平面布置.docx
- 现场评定检查表——建筑内部装修防火.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


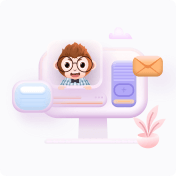
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0