/**************************************************************************************************
Filename: zcl_se.c
Revised: $Date: 2012-03-11 00:25:21 -0800 (Sun, 11 Mar 2012) $
Revision: $Revision: 29708 $
Description: Zigbee Cluster Library - SE (Smart Energy) Profile.
Copyright 2007-2012 Texas Instruments Incorporated. All rights reserved.
IMPORTANT: Your use of this Software is limited to those specific rights
granted under the terms of a software license agreement between the user
who downloaded the software, his/her employer (which must be your employer)
and Texas Instruments Incorporated (the "License"). You may not use this
Software unless you agree to abide by the terms of the License. The License
limits your use, and you acknowledge, that the Software may not be modified,
copied or distributed unless embedded on a Texas Instruments microcontroller
or used solely and exclusively in conjunction with a Texas Instruments radio
frequency transceiver, which is integrated into your product. Other than for
the foregoing purpose, you may not use, reproduce, copy, prepare derivative
works of, modify, distribute, perform, display or sell this Software and/or
its documentation for any purpose.
YOU FURTHER ACKNOWLEDGE AND AGREE THAT THE SOFTWARE AND DOCUMENTATION ARE
PROVIDED �AS IS� WITHOUT WARRANTY OF ANY KIND, EITHER EXPRESS OR IMPLIED,
INCLUDING WITHOUT LIMITATION, ANY WARRANTY OF MERCHANTABILITY, TITLE,
NON-INFRINGEMENT AND FITNESS FOR A PARTICULAR PURPOSE. IN NO EVENT SHALL
TEXAS INSTRUMENTS OR ITS LICENSORS BE LIABLE OR OBLIGATED UNDER CONTRACT,
NEGLIGENCE, STRICT LIABILITY, CONTRIBUTION, BREACH OF WARRANTY, OR OTHER
LEGAL EQUITABLE THEORY ANY DIRECT OR INDIRECT DAMAGES OR EXPENSES
INCLUDING BUT NOT LIMITED TO ANY INCIDENTAL, SPECIAL, INDIRECT, PUNITIVE
OR CONSEQUENTIAL DAMAGES, LOST PROFITS OR LOST DATA, COST OF PROCUREMENT
OF SUBSTITUTE GOODS, TECHNOLOGY, SERVICES, OR ANY CLAIMS BY THIRD PARTIES
(INCLUDING BUT NOT LIMITED TO ANY DEFENSE THEREOF), OR OTHER SIMILAR COSTS.
Should you have any questions regarding your right to use this Software,
contact Texas Instruments Incorporated at www.TI.com.
**************************************************************************************************/
/*********************************************************************
* INCLUDES
*/
#include "ZComDef.h"
#include "OSAL.h"
#include "zcl.h"
#include "zcl_general.h"
#include "zcl_se.h"
#include "DebugTrace.h"
#if defined ( INTER_PAN )
#include "stub_aps.h"
#endif
#include "zcl_key_establish.h"
/*********************************************************************
* MACROS
*/
// Clusters that are supported thru Inter-PAN communication
#define INTER_PAN_CLUSTER( id ) ( (id) == ZCL_CLUSTER_ID_SE_PRICING || \
(id) == ZCL_CLUSTER_ID_SE_MESSAGE )
/*********************************************************************
* CONSTANTS
*/
/*********************************************************************
* TYPEDEFS
*/
typedef struct zclSECBRec
{
struct zclSECBRec *next;
uint8 endpoint; // Used to link it into the endpoint descriptor
zclSE_AppCallbacks_t *CBs; // Pointer to Callback function
} zclSECBRec_t;
/*********************************************************************
* GLOBAL VARIABLES
*/
/*********************************************************************
* GLOBAL FUNCTIONS
*/
/*********************************************************************
* LOCAL VARIABLES
*/
static zclSECBRec_t *zclSECBs = (zclSECBRec_t *)NULL;
static uint8 zclSEPluginRegisted = FALSE;
/*********************************************************************
* LOCAL FUNCTIONS
*/
static ZStatus_t zclSE_HdlIncoming( zclIncoming_t *pInMsg );
static ZStatus_t zclSE_HdlInSpecificCommands( zclIncoming_t *pInMsg );
#ifdef ZCL_SIMPLE_METERING
static ZStatus_t zclSE_ProcessInSimpleMeteringCmds( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_GetProfileCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_GetProfileRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_ReqMirrorCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_ReqMirrorRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_MirrorRemCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_MirrorRemRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_ReqFastPollModeCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_ReqFastPollModeRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
#ifdef SE_UK_EXT
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_GetSnapshotCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_GetSnapshotRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_TakeSnapshotCmd( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_SimpleMeter_MirrorReportAttrRsp( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
#endif // SE_UK_EXT
#endif // ZCL_SIMPLE_METERING
#ifdef ZCL_PRICING
static ZStatus_t zclSE_ProcessInPricingCmds( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_GetCurrentPrice( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_GetScheduledPrice( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishPrice( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PriceAcknowledgement( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_GetBlockPeriod( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishBlockPeriod( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
#ifdef SE_UK_EXT
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishTariffInformation( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishPriceMatrix( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishBlockThreshold( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishConversionFactor( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishCalorificValue( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishCO2Value( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishCPPEvent( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishBillingPeriod( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishConsolidatedBill( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_PublishCreditPaymentInfo( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_GetTariffInformation( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_GetPriceMatrix( zclIncoming_t *pInMsg, zclSE_AppCallbacks_t *pCBs );
static ZStatus_t zclSE_ProcessInCmd_Pricing_
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
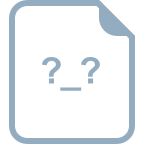
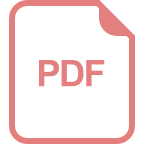
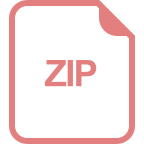
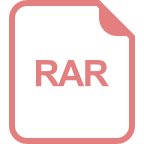
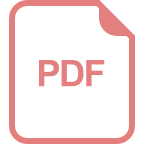
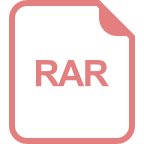
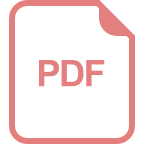
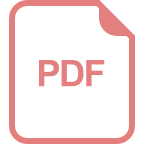
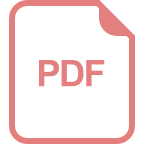
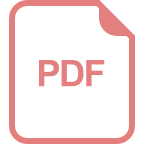
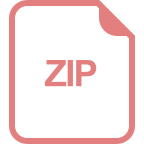
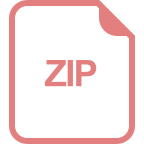
收起资源包目录

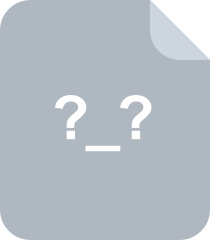
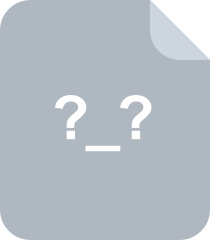
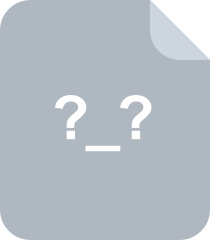
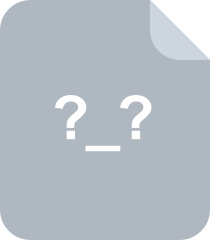
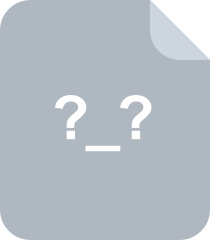
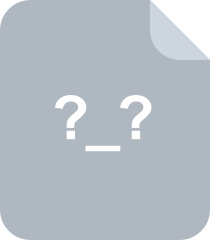
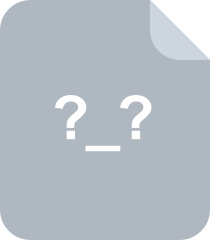
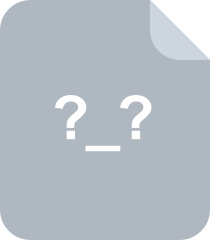
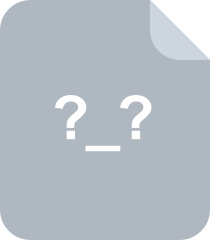
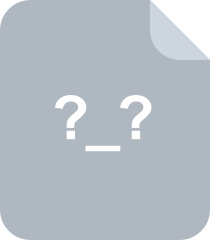
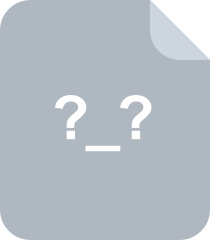
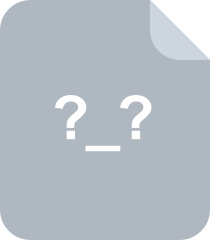
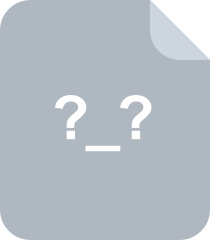
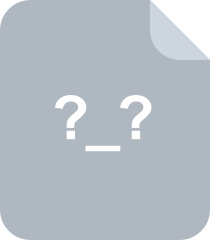
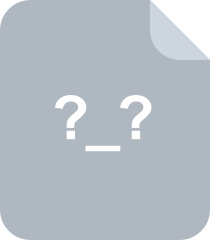
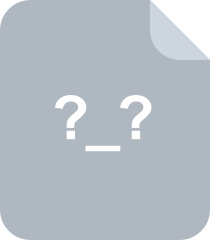
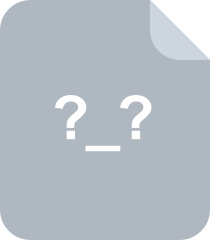
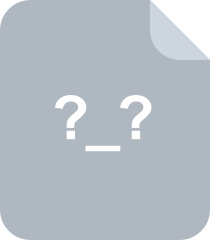
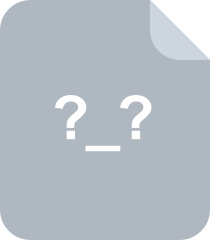
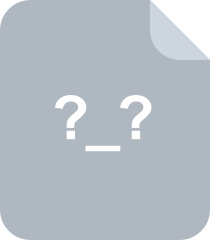
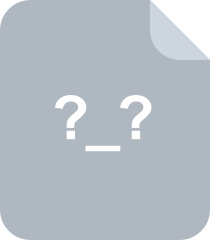
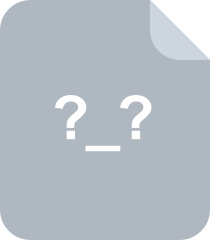
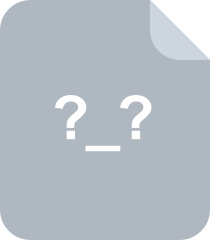
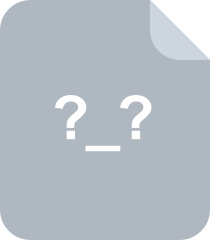
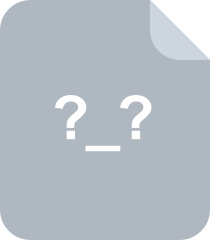
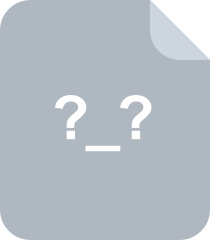
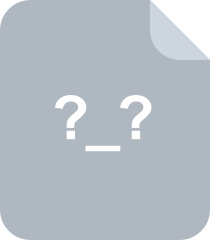
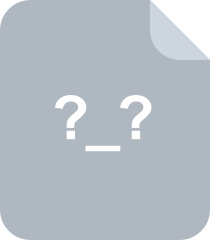
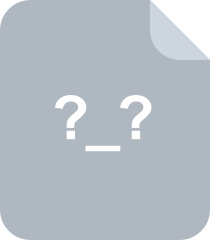
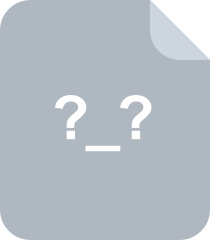
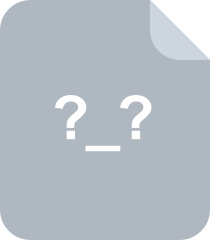
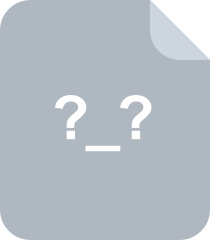
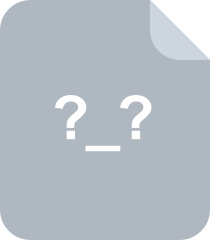
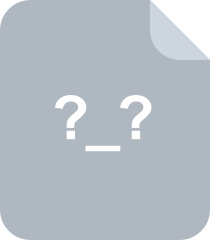
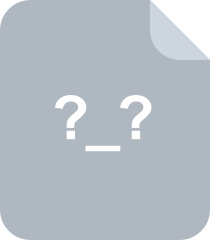
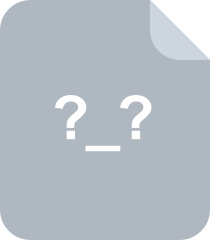
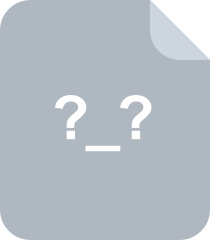
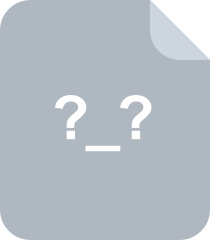
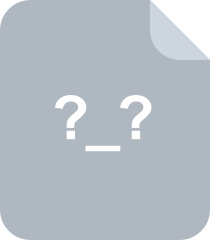
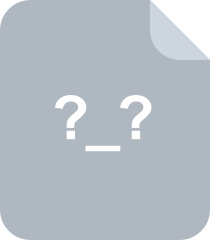
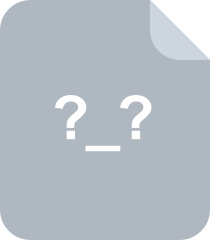
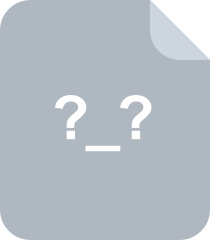
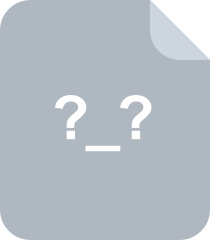
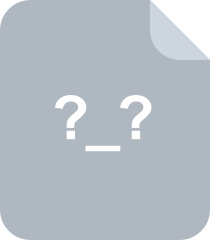
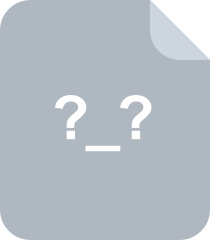
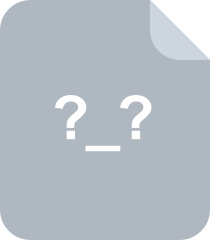
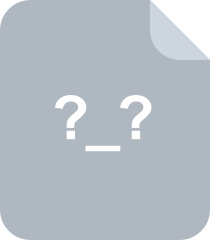
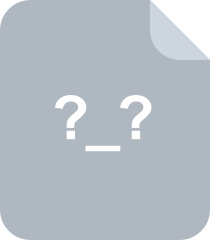
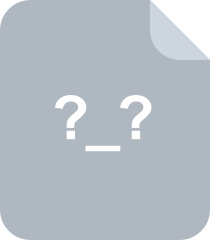
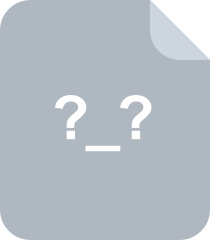
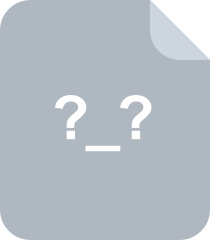
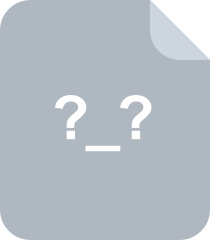
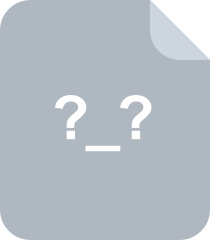
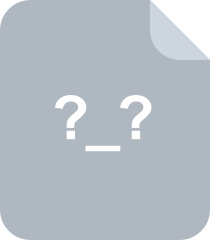
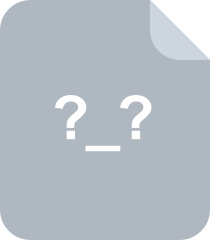
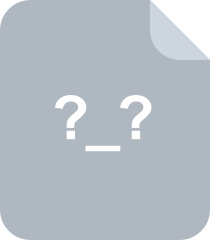
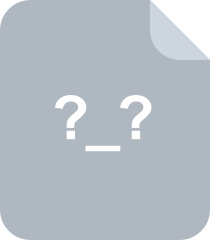
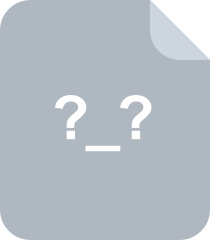
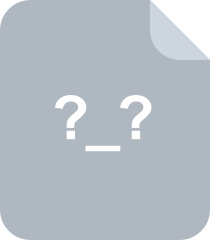
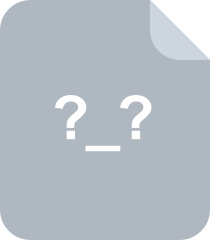
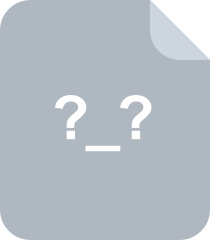
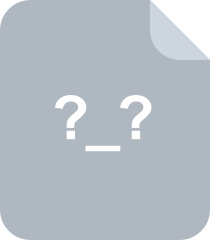
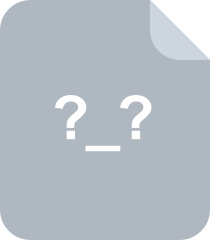
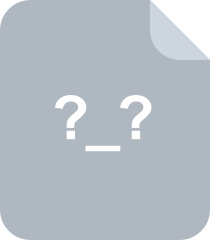
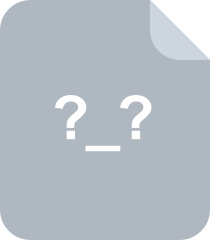
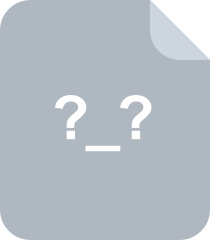
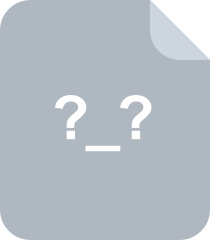
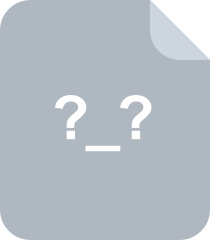
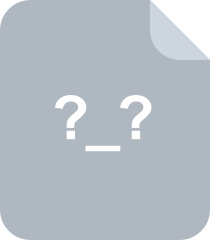
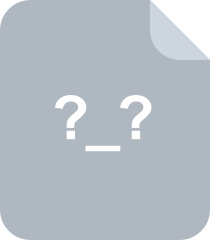
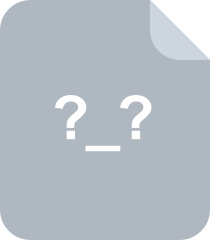
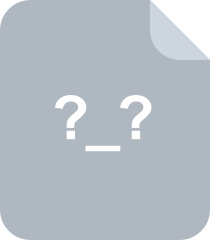
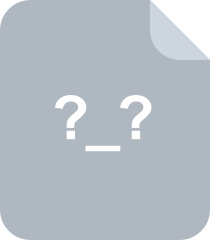
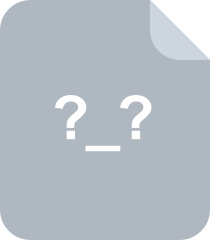
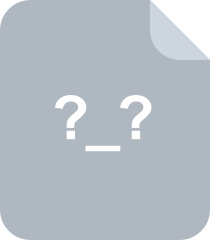
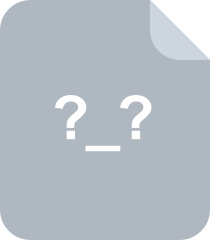
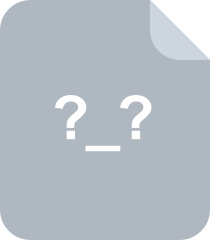
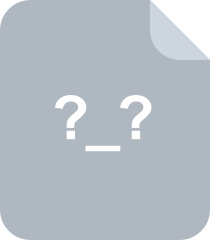
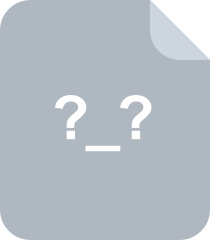
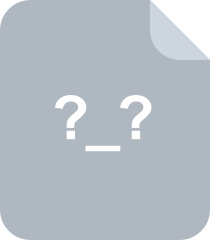
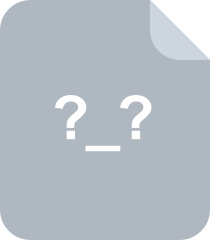
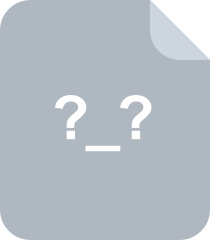
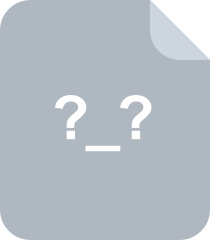
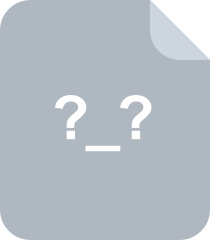
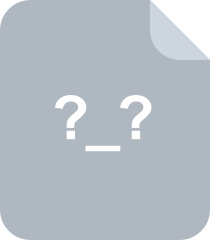
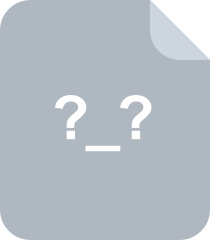
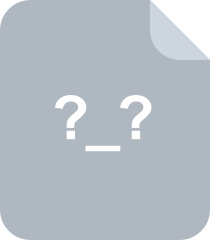
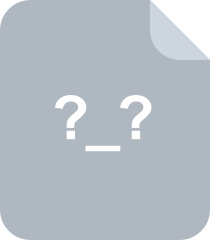
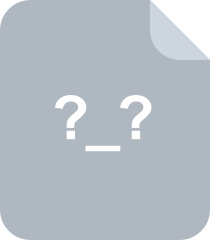
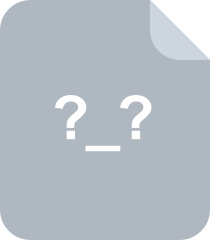
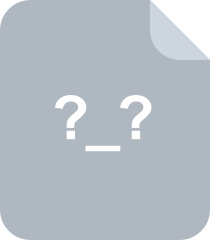
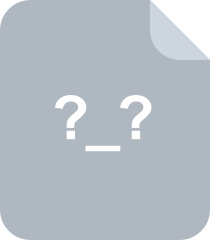
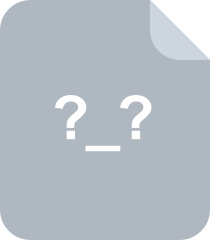
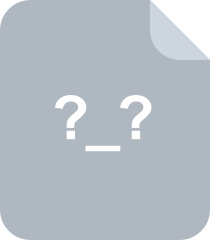
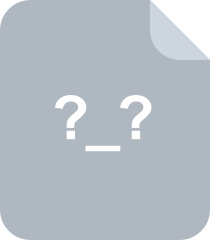
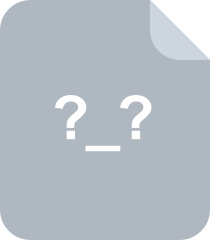
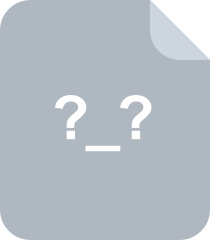
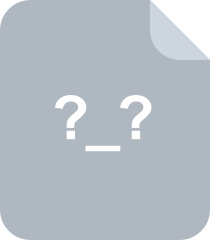
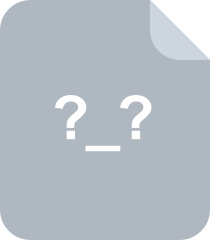
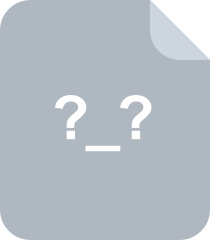
共 1064 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
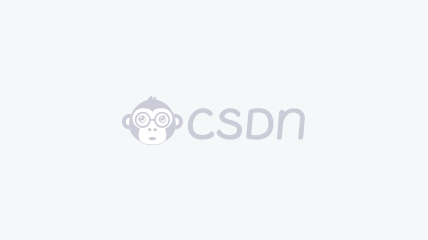

weixin_43692716
- 粉丝: 2
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

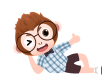
最新资源
- 基于JavaScript和CSS的随寻订购网页设计源码 - web-order
- 基于MATLAB的声纹识别系统设计源码 - VoiceprintRecognition
- 基于Java的微服务插件集合设计源码 - wsy-plugins
- 基于Vue和微信小程序的监理日志系统设计源码 - supervisionLog
- 基于Java和LCN分布式事务框架的设计源码 - tx-lcn
- 基于Java和JavaScript的茶叶评级管理系统设计源码 - tea
- IMG_5680.JPG
- IMG_0437.jpg
- 基于Java的JAVA项目分析工具设计源码 - JAVAProjectAnalysis
- top888.json
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


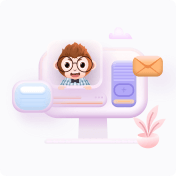
安全验证
文档复制为VIP权益,开通VIP直接复制
