#include "GUI/battlewindow.h"
#include <random>
#include "ui_battlewindow.h"
battleWindow::battleWindow(int level, int playerType, QWidget *parent)
: QMainWindow(parent),
enemyActionForecastWidget(new QWidget(this)),
ui(new Ui::battleWindow) {
ui->setupUi(this);
this->setWindowTitle("战斗");
this->setAttribute(Qt::WA_TranslucentBackground);
this->level = level; // 添加了一个全局变量level
game = new WholeProgress(playerType, level);
timer.stop();
connect(game, &WholeProgress::showHurtNumber, this,
&battleWindow::showNumber); //
this->showUgly(game->wholeEnemy.size());
enemy_damage = game->wholeEnemy.begin()->getAtack();
cardList = game->battleStart();
this->hideButton();
this->showCard();
this->showHpMp();
enemyAnimation = new QPropertyAnimation;
enemyAnimation->setPropertyName("geometry");
enemyAnimation->setDuration(700);
playerAnimation = new QPropertyAnimation;
playerAnimation->setPropertyName("geometry");
playerAnimation->setDuration(700);
count = new myTime();
// switInEnd = 1;
enter_store = false;
enter_rest = false;
ui->widget->hide();
ui->widget_2->hide();
ui->widget_3->hide();
ui->widget_4->hide();
type = playerType;
if (type == 1) {
ui->label_3->setPixmap(QPixmap(":/WarriorCards.png"));
} else {
ui->label_3->setPixmap(QPixmap(":/green.png"));
}
enemyActionForecastWidget->setGeometry(900, 100, 200,
100); // 你可以根据实际窗口尺寸调整
enemyActionForecastWidget->setStyleSheet(
"background-color: rgba(211, 211, 211, 0.8);" // 浅灰色背景
"border: 1px solid black;" // 边框颜色
"border-radius: 15px;" // 圆角半径
);
QString enemy_action =
QString::asprintf("Preparing to attack! Damage: %d", enemy_damage);
enemyActionLabel = new QLabel(enemy_action, enemyActionForecastWidget);
enemyActionLabel->setAlignment(Qt::AlignCenter); // 居中对齐
enemyActionLabel->setWordWrap(true); // 启用自动换行
enemyActionLabel->setStyleSheet(
"color: black; font-size: 16px;"); // 黑色字体
enemyActionLabel->setGeometry(0, 0, 200, 100); // 设置为和预告框一样大
enemyActionForecastWidget->show();
enemyActionForecastWidget->raise();
// 伤害数字格式设置
for (int i = 0; i < 5; i++) {
hurtNumber[i] = new QLabel("114514", this);
hurtNumber[i]->setStyleSheet(
"color: red; font-weight: bold; font-size: 35px;");
hurtNumber[i]->setAlignment(Qt::AlignCenter);
hurtNumber[i]->resize(250, 50);
opacityEffect[i] = new QGraphicsOpacityEffect(hurtNumber[i]);
hurtNumber[i]->setGraphicsEffect(opacityEffect[i]);
numberMove[i] = new QPropertyAnimation(hurtNumber[i], "pos");
numberMove[i]->setDuration(600); // 动画持续时间
numberMove[i]->setStartValue(QPoint(1040, 200)); // 起始位置
numberMove[i]->setEndValue(QPoint(1040, 120)); // 终点位置
numberMove[i]->setEasingCurve(QEasingCurve::InOutQuad);
numberOpacity[i] = new QPropertyAnimation(opacityEffect[i], "opacity");
numberOpacity[i]->setDuration(600); // 动画持续时间
numberOpacity[i]->setStartValue(1.0); // 起始不透明度
numberOpacity[i]->setEndValue(0.0);
animationGroup[i] = new QParallelAnimationGroup;
animationGroup[i]->addAnimation(numberMove[i]);
animationGroup[i]->addAnimation(numberOpacity[i]);
hurtNumber[i]->hide();
}
// 休息时相关的初始化
// 休息时的背景
rest_back = new QLabel(this);
rest_back->setPixmap(QPixmap(":/chunblack.jpg"));
rest_back->setScaledContents(true);
rest_back->resize(1478, 763);
rest_back->move(-20, 0);
rest_back->hide();
// 休息的文字
rest_text = new QLabel(this);
rest_text->setPixmap(QPixmap(":/resting.png"));
rest_text->setScaledContents(true);
rest_text->resize(480, 366);
int window_width = this->width();
rest_text->move((window_width - rest_text->width()) / 2, -70);
rest_text->hide();
// 休息的动图
rest_gif = new QLabel(this);
gif = new QMovie(":/saber.gif");
gif->start();
rest_gif->setMovie(gif);
rest_gif->setScaledContents(true);
rest_gif->resize(600, 600);
rest_gif->move((this->width() - rest_gif->width()) / 2, 200);
rest_gif->hide();
// 休息的计时器
rest_timer = new QTimer(this);
rest_timer->setInterval(3000);
QObject::connect(rest_timer, &QTimer::timeout, [=]() {
// 超时的处理,利用lambda函数实现
qDebug() << "休息的计时结束";
rest_back->hide();
rest_text->hide();
rest_gif->hide();
rest_timer->stop();
});
// 事件文本自动换行
ui->event_text->setWordWrap(true);
event_num = 1;
}
battleWindow::~battleWindow() { delete ui; }
void battleWindow::usecard(int n) {
// 使用卡牌
qDebug() << "当前人物蓝量为: " << game->playername->getMp();
qDebug() << "使用第" << n + 1 << "张牌";
if (game->ifuse(n, type)) {
// 卡牌音效
// musicPlayer.playMusic(cardList[4].getMusicPath());
this->action(n); // 玩家动作
card0 = game->dataReturn(n);
cardList = game->returnCardInHand();
if (cardList[0].getMp() == 1000) {
vector<card>::iterator k = cardList.begin();
cardList.erase(k);
}
// 玩家动作
this->showHpMp(); // 更新血条蓝条
this->hideButton();
this->showCard(); // 显示当前牌;
qDebug() << game->playername->manager->wastedCard.size()
<< "wastedCard.size";
qDebug() << game->playername->manager->Card2DrugList.size()
<< "Card2DrugList.size";
qDebug() << cardList.size();
this->show_enemy_buff(); // 怪物buff
this->show_player_buff(); // 玩家buff
this->showEnemyAction();
} else {
}
this->whoDeath();
}
void battleWindow::win() {
// 游戏获胜
qDebug() << "游戏获胜";
endwindow *end = new endwindow();
end->show();
this->close();
}
// 战斗按钮
void battleWindow::fight_button(int n) {
qDebug() << "fight" << n << "按下";
this->showUgly(game->wholeEnemy.size());
enemy_damage = game->wholeEnemy.begin()->getAtack();
cardList = game->battleStart();
this->hideButton();
this->showCard(); // 显示当前牌;
this->showHpMp();
this->showEnemyAction();
// switInEnd = 1;
enter_store = false;
enter_rest = false;
ui->widget->hide();
}
// 休息按钮
void battleWindow::rest_button(int n) {
qDebug() << "rest" << n << "按下";
if (enter_rest == false) {
if (this->level != 1) {
// 最低难度允许重复休息
enter_rest = true;
}
game->playername->setHp((11 - level));
// 休息时黑屏3s
rest_back->show();
rest_text->show();
random_gif();
rest_gif->show();
rest_timer->start();
ui->label_6->setGeometry(
60, 30,
game->playername->getHp() * (460 / game->playername->getMaxHp()), 20);
}
// 刷新节点
random_route();
// musicPlayer.playMusic("qrc:/new/prefix1/Music/victory.ogg");
hide_buff();
}
// 商店按钮
void battleWindow::store_button(int n) {
qDebug() << "store" << n << "按下";
if (enter_store == false) {
enter_store = true;
chooseCard = game->playername->manager->shopCard();
ui->label_18->setText("你的金币:" +
QString::number(game->playername->getMoney()));
ui->label_19->setText("花费:" + QString::number(chooseCard[0].getPrice()));
ui->label_20->setText("花费:" + QString::number(chooseCard[1].getPrice()));
ui->label_21->setText("花费:" + QString::number(chooseCard[2].getPrice()));
ui->card1->setIcon(QIcon(chooseCard[0].getPicPath()));
ui->card2->setIcon(QIcon(chooseCard[1].getPicPath()));
ui->card3->setIcon(QIcon(chooseCard[2].getPicPath()));
ui->card1->setVisible(true);
ui->card2->setVisible(true);
ui->card3->setVisible(true);
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
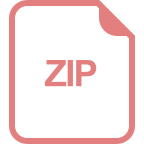
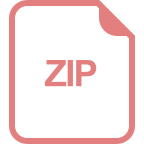
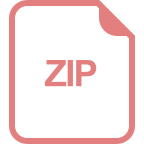
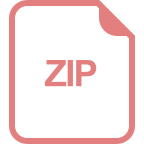
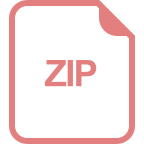
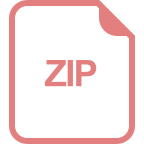
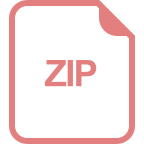
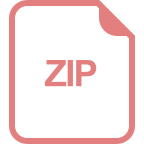
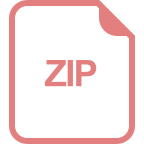
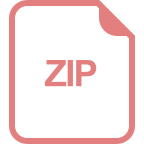
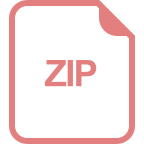
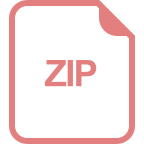
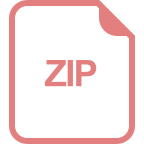
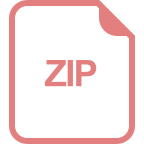
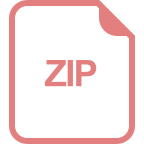
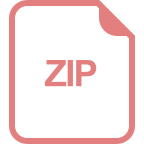
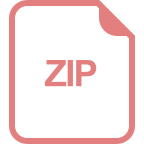
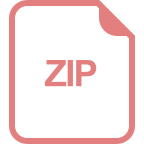
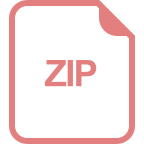
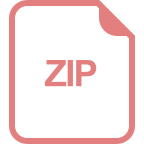
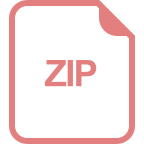
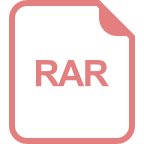
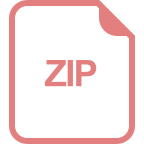
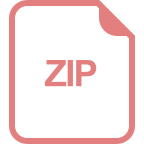
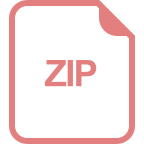
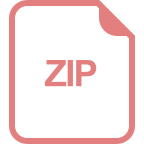
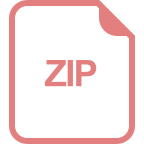
收起资源包目录

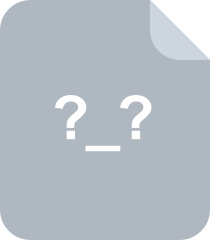
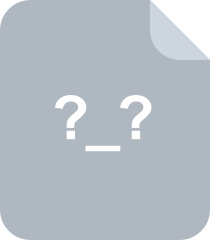
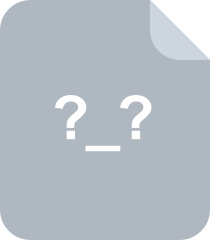
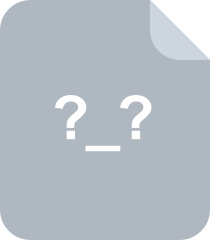
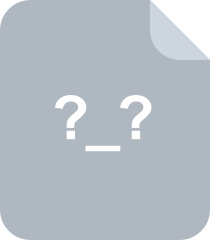
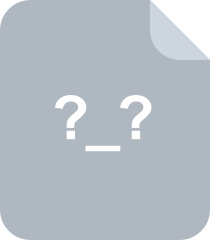
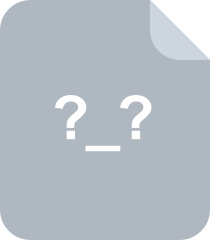
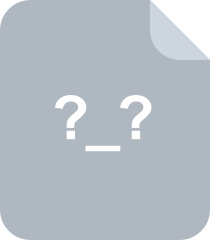
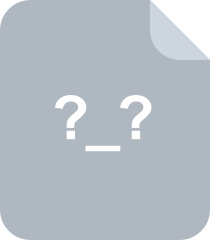
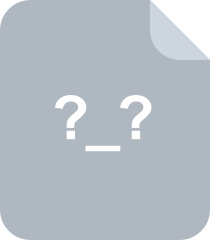
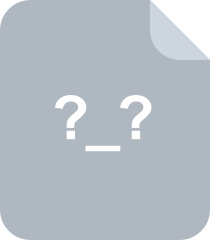
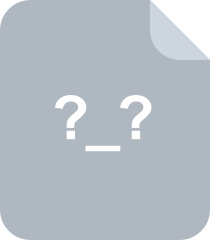
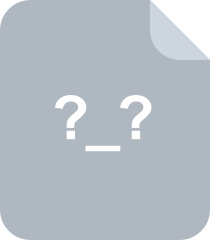
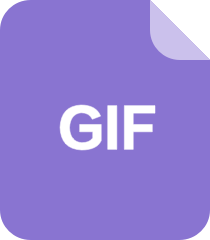
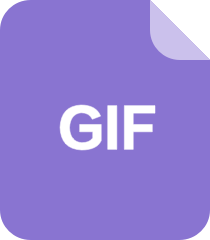
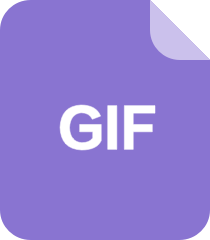
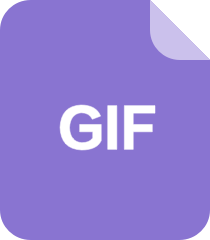
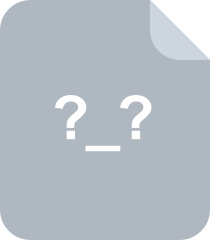
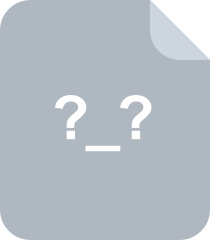
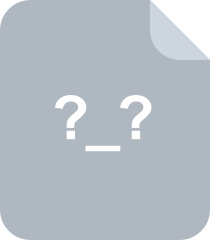
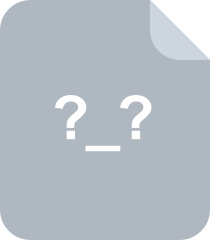
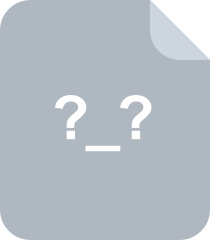
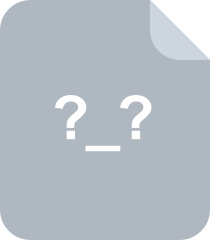
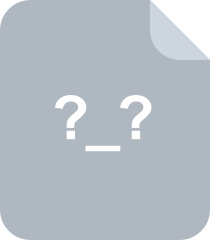
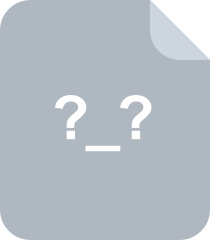
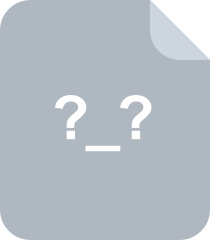
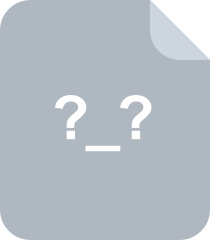
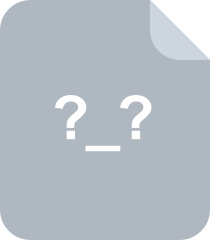
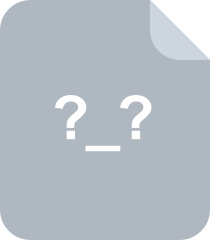
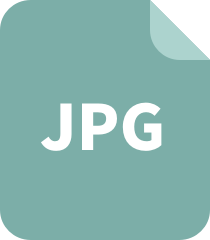
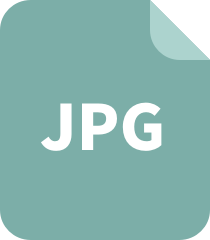
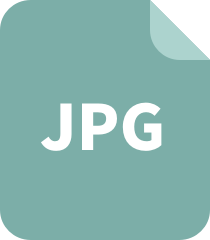
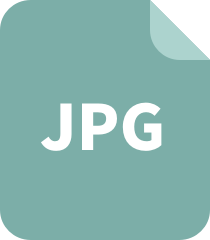
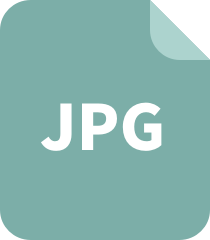
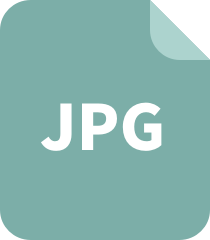
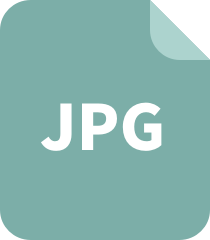
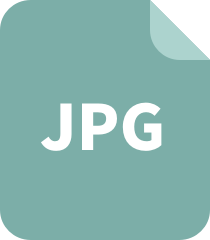
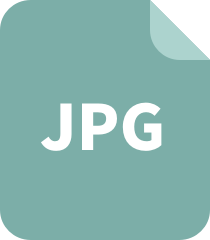
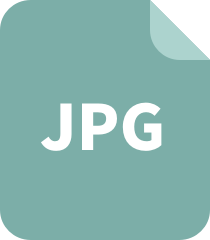
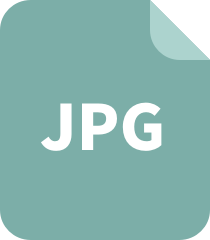
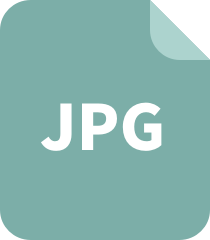
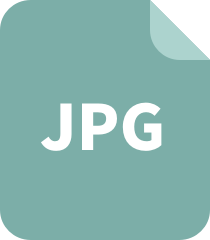
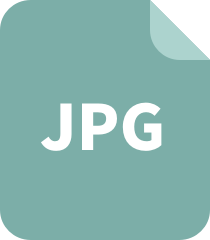
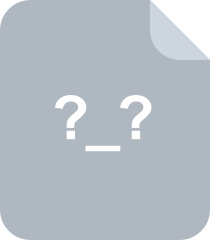
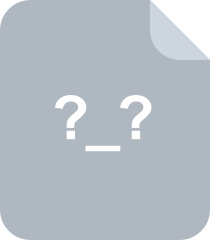
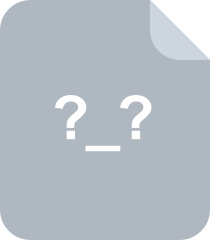
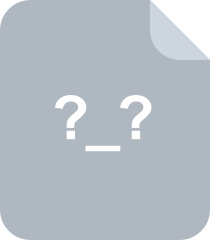
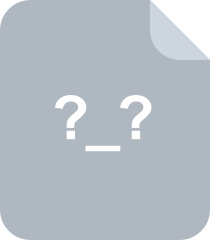
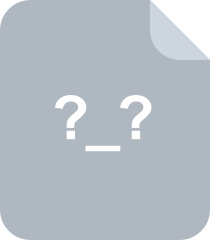
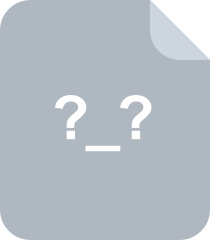
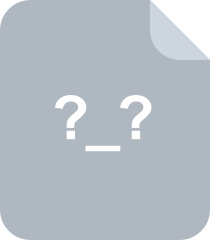
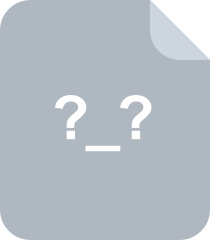
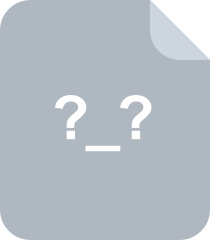
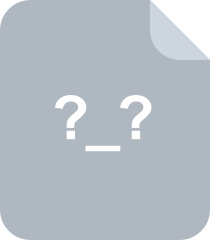
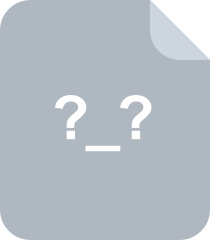
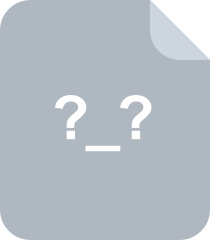
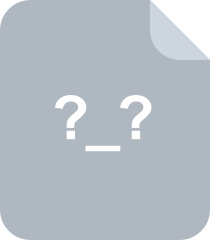
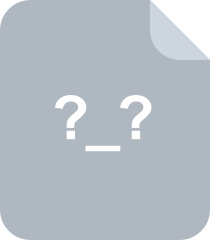
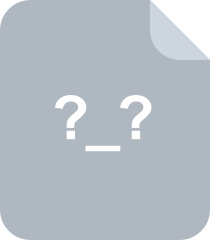
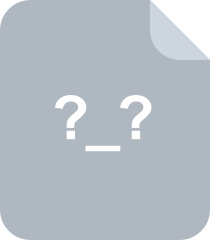
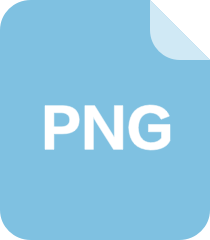
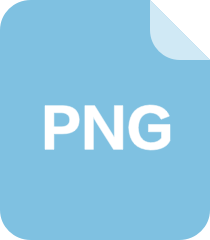
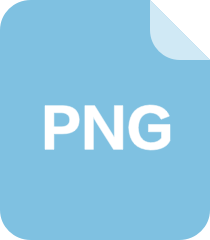
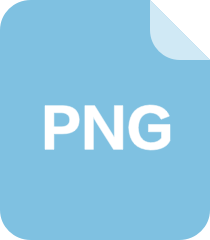
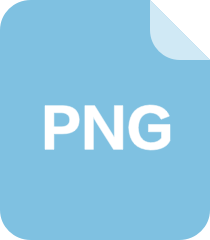
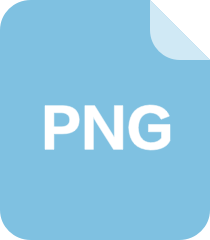
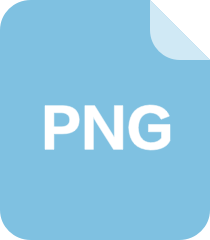
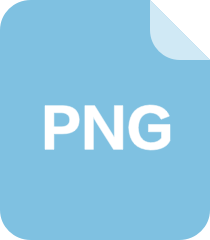
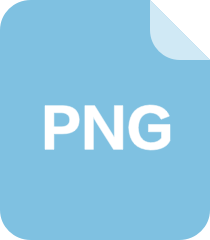
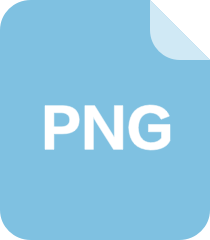
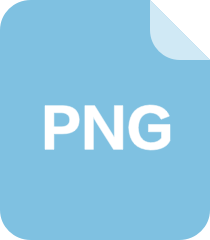
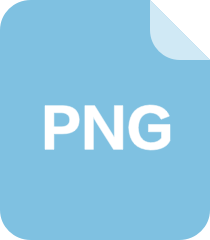
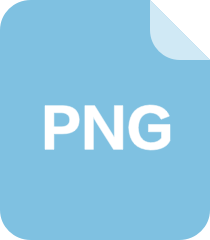
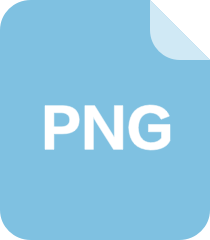
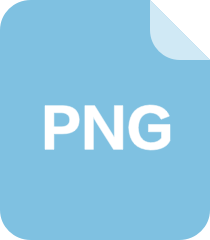
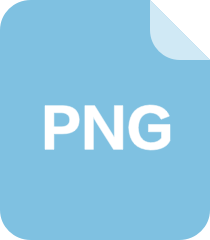
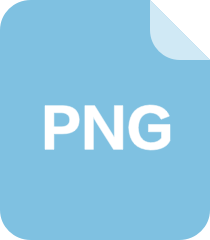
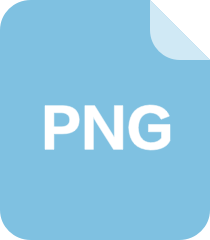
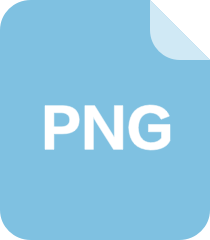
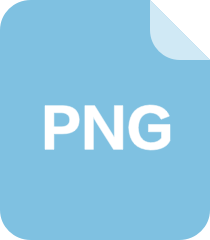
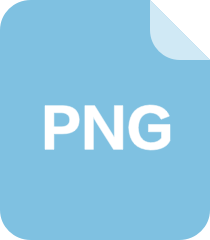
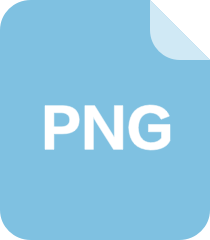
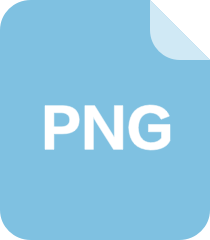
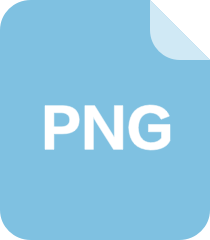
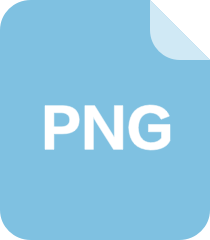
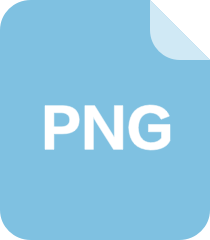
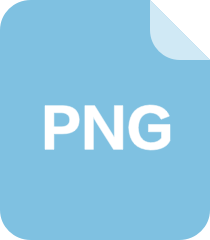
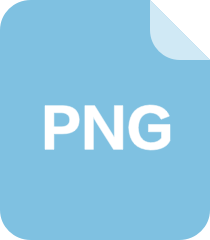
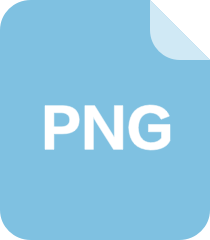
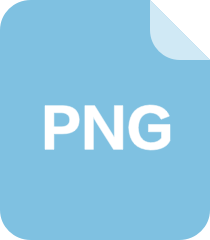
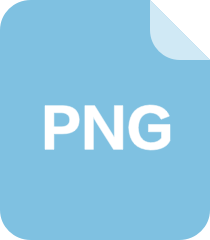
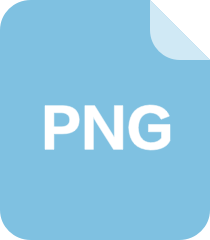
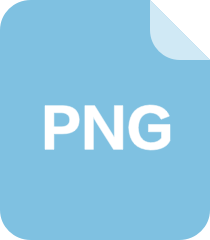
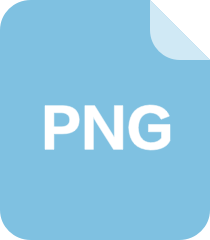
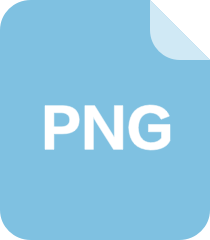
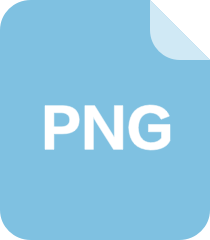
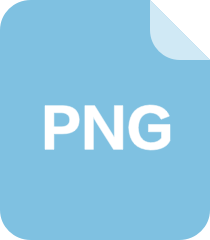
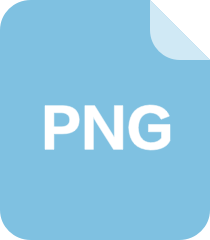
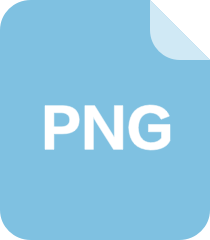
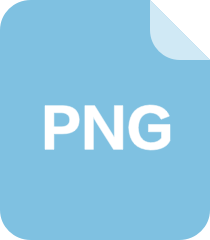
共 193 条
- 1
- 2
资源评论
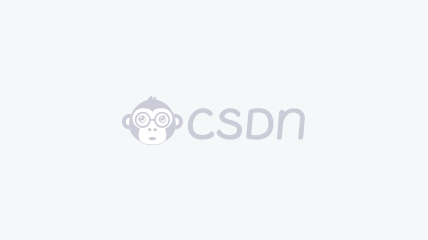

编程ID
- 粉丝: 8w+
- 资源: 766

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

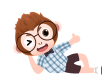
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


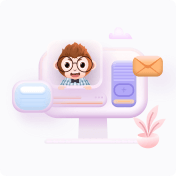
安全验证
文档复制为VIP权益,开通VIP直接复制
