<?php
namespace app\lib\mail\PHPMailer; class PHPMailer { const CHARSET_ASCII = 'us-ascii'; const CHARSET_ISO88591 = 'iso-8859-1'; const CHARSET_UTF8 = 'utf-8'; const CONTENT_TYPE_PLAINTEXT = 'text/plain'; const CONTENT_TYPE_TEXT_CALENDAR = 'text/calendar'; const CONTENT_TYPE_TEXT_HTML = 'text/html'; const CONTENT_TYPE_MULTIPART_ALTERNATIVE = 'multipart/alternative'; const CONTENT_TYPE_MULTIPART_MIXED = 'multipart/mixed'; const CONTENT_TYPE_MULTIPART_RELATED = 'multipart/related'; const ENCODING_7BIT = '7bit'; const ENCODING_8BIT = '8bit'; const ENCODING_BASE64 = 'base64'; const ENCODING_BINARY = 'binary'; const ENCODING_QUOTED_PRINTABLE = 'quoted-printable'; const ENCRYPTION_STARTTLS = 'tls'; const ENCRYPTION_SMTPS = 'ssl'; const ICAL_METHOD_REQUEST = 'REQUEST'; const ICAL_METHOD_PUBLISH = 'PUBLISH'; const ICAL_METHOD_REPLY = 'REPLY'; const ICAL_METHOD_ADD = 'ADD'; const ICAL_METHOD_CANCEL = 'CANCEL'; const ICAL_METHOD_REFRESH = 'REFRESH'; const ICAL_METHOD_COUNTER = 'COUNTER'; const ICAL_METHOD_DECLINECOUNTER = 'DECLINECOUNTER'; public $Priority; public $CharSet = self::CHARSET_ISO88591; public $ContentType = self::CONTENT_TYPE_PLAINTEXT; public $Encoding = self::ENCODING_8BIT; public $ErrorInfo = ''; public $From = ''; public $FromName = ''; public $Sender = ''; public $Subject = ''; public $Body = ''; public $AltBody = ''; public $Ical = ''; protected static $IcalMethods = [self::ICAL_METHOD_REQUEST, self::ICAL_METHOD_PUBLISH, self::ICAL_METHOD_REPLY, self::ICAL_METHOD_ADD, self::ICAL_METHOD_CANCEL, self::ICAL_METHOD_REFRESH, self::ICAL_METHOD_COUNTER, self::ICAL_METHOD_DECLINECOUNTER,]; protected $MIMEBody = ''; protected $MIMEHeader = ''; protected $mailHeader = ''; public $WordWrap = 0; public $Mailer = 'mail'; public $Sendmail = '/usr/sbin/sendmail'; public $UseSendmailOptions = true; public $ConfirmReadingTo = ''; public $Hostname = ''; public $MessageID = ''; public $MessageDate = ''; public $Host = 'localhost'; public $Port = 25; public $Helo = ''; public $SMTPSecure = ''; public $SMTPAutoTLS = true; public $SMTPAuth = false; public $SMTPOptions = []; public $Username = ''; public $Password = ''; public $AuthType = ''; protected $SMTPXClient = []; protected $oauth; public $Timeout = 300; public $dsn = ''; public $SMTPDebug = 0; public $Debugoutput = 'echo'; public $SMTPKeepAlive = false; public $SingleTo = false; protected $SingleToArray = []; public $do_verp = false; public $AllowEmpty = false; public $DKIM_selector = ''; public $DKIM_identity = ''; public $DKIM_passphrase = ''; public $DKIM_domain = ''; public $DKIM_copyHeaderFields = true; public $DKIM_extraHeaders = []; public $DKIM_private = ''; public $DKIM_private_string = ''; public $action_function = ''; public $XMailer = ''; public static $validator = 'php'; protected $smtp; protected $to = []; protected $cc = []; protected $bcc = []; protected $ReplyTo = []; protected $all_recipients = []; protected $RecipientsQueue = []; protected $ReplyToQueue = []; protected $attachment = []; protected $CustomHeader = []; protected $lastMessageID = ''; protected $message_type = ''; protected $boundary = []; protected $language = []; protected $error_count = 0; protected $sign_cert_file = ''; protected $sign_key_file = ''; protected $sign_extracerts_file = ''; protected $sign_key_pass = ''; protected $exceptions = false; protected $uniqueid = ''; const VERSION = '6.9.1'; const STOP_MESSAGE = 0; const STOP_CONTINUE = 1; const STOP_CRITICAL = 2; const CRLF = "\r\n"; const FWS = ' '; protected static $LE = self::CRLF; const MAIL_MAX_LINE_LENGTH = 63; const MAX_LINE_LENGTH = 998; const STD_LINE_LENGTH = 76; public function __construct($exceptions = null) { if (null !== $exceptions) { $this->exceptions = (bool) $exceptions; } $this->Debugoutput = (strpos(PHP_SAPI, 'cli') !== false ? 'echo' : 'html'); } public function __destruct() { $this->smtpClose(); } private function mailPassthru($to, $subject, $body, $header, $params) { if ((int)ini_get('mbstring.func_overload') & 1) { $subject = $this->secureHeader($subject); } else { $subject = $this->encodeHeader($this->secureHeader($subject)); } $this->edebug('Sending with mail()'); $this->edebug('Sendmail path: ' . ini_get('sendmail_path')); $this->edebug("Envelope sender: {$this->Sender}"); $this->edebug("To: {$to}"); $this->edebug("Subject: {$subject}"); $this->edebug("Headers: {$header}"); if (!$this->UseSendmailOptions || null === $params) { $result = @mail($to, $subject, $body, $header); } else { $this->edebug("Additional params: {$params}"); $result = @mail($to, $subject, $body, $header, $params); } $this->edebug('Result: ' . ($result ? 'true' : 'false')); return $result; } protected function edebug($str) { if ($this->SMTPDebug <= 0) { return; } if ($this->Debugoutput instanceof \Psr\Log\LoggerInterface) { $this->Debugoutput->debug(rtrim($str, "\r\n")); return; } if (is_callable($this->Debugoutput) && !in_array($this->Debugoutput, ['error_log', 'html', 'echo'])) { call_user_func($this->Debugoutput, $str, $this->SMTPDebug); return; } switch ($this->Debugoutput) { case 'error_log': error_log($str); break; case 'html': echo htmlentities(preg_replace('/[\r\n]+/', '', $str), ENT_QUOTES, 'UTF-8'), "<br>\n"; break; case 'echo': default: $str = preg_replace('/\r\n|\r/m', "\n", $str); echo gmdate('Y-m-d H:i:s'), "\t", trim(str_replace("\n", "\n \t ", trim($str))), "\n"; } } public function isHTML($isHtml = true) { if ($isHtml) { $this->ContentType = static::CONTENT_TYPE_TEXT_HTML; } else { $this->ContentType = static::CONTENT_TYPE_PLAINTEXT; } } public function isSMTP() { $this->Mailer = 'smtp'; } public function isMail() { $this->Mailer = 'mail'; } public function isSendmail() { $ini_sendmail_path = ini_get('sendmail_path'); if (false === stripos($ini_sendmail_path, 'sendmail')) { $this->Sendmail = '/usr/sbin/sendmail'; } else { $this->Sendmail = $ini_sendmail_path; } $this->Mailer = 'sendmail'; } public function isQmail() { $ini_sendmail_path = ini_get('sendmail_path'); if (false === stripos($ini_sendmail_path, 'qmail')) { $this->Sendmail = '/var/qmail/bin/qmail-inject'; } else { $this->Sendmail = $ini_sendmail_path; } $this->Mailer = 'qmail'; } public function addAddress($address, $name = '') { return $this->addOrEnqueueAnAddress('to', $address, $name); } public function addCC($address, $name = '') { return $this->addOrEnqueueAnAddress('cc', $address, $name); } public function addBCC($address, $name = '') { return $this->addOrEnqueueAnAddress('bcc', $address, $name); } public function addReplyTo($address, $name = '') { return $this->addOrEnqueueAnAddress('Reply-To', $address, $name); } protected function addOrEnqueueAnAddress($kind, $address, $name) { $pos = false; if ($address !== null) { $address = trim($address); $pos = strrpos($address, '@'); } if (false === $pos) { $error_message = sprintf('%s (%s): %s', $this->lang('invalid_address'), $kind, $address); $this->setError($error_message); $this->edebug($error_message); if ($this->exceptions) { throw new Exception($error_message); } return false; } if ($name !== null && is_string($name)) { $name = trim(preg_replace('/[\r\n]+/', '', $name)); } else { $name = ''; } $params = [$kind, $address, $name]; if (static::idnSupported() && $this->has8bitChars(substr($address, ++$pos))) { if ('Reply-To' !== $kind) { if (!array_key_exists($address, $this->RecipientsQueue)) { $this->RecipientsQueue[$address] = $params; return true; } } elseif (!array_key_exists($address, $this->ReplyToQueue)) { $this->ReplyToQueue[$address] = $params; return true; } return false; } return call_user_func_array([$this, 'addAnAddress'], $params); } public function setBoundaries() { $this->uniqueid = $this->generateId(); $this->boundary[1] = 'b1=_' . $this->uniqueid; $this->boundary[2] = 'b2=_' . $this->uniqueid; $this->boundary[3] = 'b3=_' . $this->uniqueid; } protected function addAnAddress($kind, $address, $name = '') { if (!in_array($kind, ['to', 'cc', 'bcc', 'Reply-To'])) { $error_message = sprintf


梦玄诗
- 粉丝: 3014
- 资源: 97
最新资源
- 基于springboot+Vue的在线互动学习网站设计(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的的信息技术知识竞赛系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于JSP的乡镇自来水收费系统+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于SSM的毕业论文管理系统+vue(Java毕业设计,附源码,数据库,教程).zip
- 基于SSM的安徽新华学院实验中心管理系统的设计与实现+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于SSM的班主任助理系统的设计与实现+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于SpringBoot+Vue的的网络海鲜市场系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于SSM的毕业生就业信息统计系统+vue(Java毕业设计,附源码,数据库,教程).zip
- 基于springboot+Vue的医疗挂号管理系统(Java毕业设计,附源码,部署教程).zip
- 基于SSM的博客系统的设计与实现+vue(Java毕业设计,附源码,数据库,教程).zip
- 基于springboot+Vue的疫情信息管理系统2(Java毕业设计,附源码,部署教程).zip
- 基于springboot+Vue的疫情信息管理系统(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的的农商对接系统的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于SpringBoot+Vue的的失物招领平台的设计与实现(Java毕业设计,附源码,部署教程).zip
- 基于SSM的大学生兼职平台的设计与开发+jsp(Java毕业设计,附源码,数据库,教程).zip
- 基于SpringBoot+Vue的的实习管理系统(Java毕业设计,附源码,部署教程).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


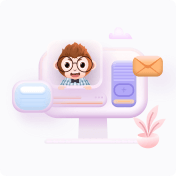