#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "Windows.h"
#include <QMessageBox>
#include <QDebug>
#include "ControlCAN.h"
#include "QDateTime"
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建线程
thread = new QThread(this);
// 不指定父对象
CanDeal = new MyThread;
CanDeal->moveToThread(thread);
//如果线程没开启就开启线程
if(thread->isRunning() == false)
thread->start();
connect(thread,&QThread::finished,CanDeal,&MyThread::deleteLater);
connect(ui->connectionBtn, &QPushButton::clicked, CanDeal, &MyThread::ReceiveCanData,Qt::QueuedConnection);
connect(CanDeal,&MyThread::ThreadSendCanData,this,&MainWindow::ReceiveThreadDataAndShow,Qt::QueuedConnection);
ui->connectionBtn->setEnabled(true);
ui->interruptBtn->setEnabled(false);
ui->tableWidget->setColumnCount(6);//设置列数,不设置行数,行数动态增加
// ui->tableWidget->horizontalHeader()
ui->tableWidget->setHorizontalHeaderLabels(QStringList()<<"时间"<<"帧ID"<<"帧格式"<<"帧类型"<<"数据长度"<<"数据(HEX)");
// ui->tableWidget->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch);
ui->tableWidget->setEditTriggers(QAbstractItemView::NoEditTriggers);//禁止修改表格中内容
ui->tableWidget->setColumnWidth(0,170);//设置第1列的宽度
ui->tableWidget->setColumnWidth(1,70);//设置第1列的宽度
ui->tableWidget->setColumnWidth(2,70);//设置第3列的宽度
ui->tableWidget->setColumnWidth(3,70);//设置第4列的宽度
ui->tableWidget->setColumnWidth(4,90);//设置第5列的宽度
ui->tableWidget->setColumnWidth(5,530);//设置第6列的宽度
}
MainWindow::~MainWindow()
{
if(thread->isRunning() == true)
{
CanDeal->CloseThread(true);
// thread->terminate();
delete CanDeal;
if(thread->isRunning() == true)
{
qDebug()<<"thread is not quit !!!:";
}
thread->requestInterruption();
thread->quit();
thread->wait();
}
if(thread->isRunning() == true)
{
qDebug()<<"thread is running!!!:";
}
else
{
qDebug()<<"thread is finished normally";
}
qDebug()<<"~MainWindow:";
delete ui;
}
void MainWindow::on_connectionBtn_clicked()
{
if(0==ui->comboBox->currentIndex())
{
nDeviceType = VCI_USBCAN2;//选择设备的类型
}
else if(1==ui->comboBox->currentIndex())
{
nDeviceType = VCI_USBCAN_2E_U;//选择设备的类型
}
else
{
QMessageBox::warning(this,"失败","设备类型有误");
return;
}
qDebug()<<"设备类型:"<<nDeviceType;
if(0==ui->comboBox_4->currentIndex())
{
nDeviceInd = 0;//设备索引号
}
else if(1==ui->comboBox_4->currentIndex())
{
nDeviceInd = 1;//设备索引号
}
else if(2==ui->comboBox_4->currentIndex())
{
nDeviceInd = 2;//设备索引号
}
else if(3==ui->comboBox_4->currentIndex())
{
nDeviceInd = 3;//设备索引号
}
else
{
QMessageBox::warning(this,"失败","错误的设备索引号");
return;
}
qDebug()<<"设备索引号:"<<nDeviceInd;
if(0==ui->comboBox_2->currentIndex())
{
nCANInd = 0;//设备通道号
}
else if(1==ui->comboBox_2->currentIndex())
{
nCANInd = 1;//设备通道号
}
else if(2==ui->comboBox_2->currentIndex())
{
nCANInd = 2;//设备通道号
}
else
{
QMessageBox::warning(this,"失败","通道号有误");
return;
}
qDebug()<<"设备通道号:"<<nCANInd;
dwRel=VCI_OpenDevice(nDeviceType,nDeviceInd,nReserved);//打开CAN设备函数
qDebug()<<"打开CAN设备:"<<dwRel;
if(0==ui->comboBox_3->currentIndex())
{
VCI_INIT_CONFIG initConfig;
memset (&initConfig,0,sizeof(initConfig));
initConfig.AccMask=0xFFFFFFFF;
initConfig.Mode=0;
initConfig.Timing0=0x01;
initConfig.Timing1=0x1c;
dwRel= VCI_InitCAN(nDeviceType,nDeviceInd,nCANInd,&initConfig);//初始化CAN设备
qDebug()<<"设置波特率:250kbps"<<dwRel;
}
else if(1==ui->comboBox_3->currentIndex())
{
VCI_INIT_CONFIG initConfig;
memset (&initConfig,0,sizeof(initConfig));
initConfig.AccMask=0xFFFFFFFF;
initConfig.Mode=0;
initConfig.Timing0=0x00;
initConfig.Timing1=0x1c;
DWORD DATA=0X060007;
dwRel=VCI_SetReference( nDeviceType, nDeviceInd, nCANInd,0, (PVOID)&DATA);
qDebug()<<"0设置波特率:500kbps"<<dwRel;
dwRel= VCI_InitCAN(nDeviceType,nDeviceInd,nCANInd,&initConfig);//初始化CAN设备
qDebug()<<"1设置波特率:500kbps"<<dwRel;
}
else
{
QMessageBox::warning(this,"失败","错误的波特率");
return;
}
dwRel = VCI_StartCAN(nDeviceType,nDeviceInd,nCANInd);//启动CAN设备
qDebug()<<"启动CAN设备:"<<dwRel;
if(dwRel !=STATUS_OK)
{
VCI_CloseDevice(nDeviceType, nDeviceInd);//关闭CAN设备
QMessageBox::warning(this,"失败","启动can失败");
return;
}
ui->connectionBtn->setEnabled(false);
ui->interruptBtn->setEnabled(true);
#if 0// 以下是自己调试测试发送和接收can数据
VCI_CAN_OBJ pSend;
pSend.ID=0x764;
pSend.Data[0]=02;
pSend.Data[1]=0x10;
pSend.Data[2]=01;
pSend.DataLen=8;
pSend.SendType=0;
pSend.ExternFlag=0;
for(int i=3;i<pSend.DataLen;i++)
{
pSend.Data[i]=0XAA;
}
qDebug()<<nDeviceType<<nDeviceInd<<nCANInd;
if( VCI_Transmit(nDeviceType,nDeviceInd, nCANInd, &pSend, 1)!=1)
{
qDebug()<<"VCI_Transmit error";
bRel = VCI_ResetCAN(nDeviceType, nDeviceInd, nCANInd);
bRel =VCI_CloseDevice(nDeviceType,nDeviceInd);
}
else
{
qDebug()<<"VCI_Transmit success";
}
DWORD lenth = VCI_GetReceiveNum(nDeviceType,nDeviceInd,nCANInd);
qDebug()<<"lenth"<<lenth;
if(lenth)
{
VCI_CAN_OBJ frameinfo[lenth];
DWORD len = VCI_Receive(nDeviceType,nDeviceInd,nCANInd,&frameinfo[0],10,5000);
if(len>0)
{
for(DWORD i=0;i<len;i++)
{
qDebug()<<"Received success"<<frameinfo[i].ID<<len;
}
}
else
qDebug()<<"Received error"<<len;
}
#endif
}
void MainWindow::on_interruptBtn_clicked()
{
bRel = VCI_ResetCAN(nDeviceType, nDeviceInd, nCANInd);
bRel =VCI_CloseDevice(nDeviceType,nDeviceInd);
ui->connectionBtn->setEnabled(true);
ui->interruptBtn->setEnabled(false);
}
void MainWindow::ReceiveThreadDataAndShow(QString strCANID, QString strFormat, QString strType, QString strLen, QString strData)
{
//获得系统当前时间
QDateTime time = QDateTime::currentDateTime();
QString strTime = time.toString("yyyy-MM-dd hh:mm:ss");
int row;
row = ui->tableWidget->rowCount();//获取当前表格中行数
ui->tableWidget->insertRow(row);//在当前表格后插入一空行
ui->tableWidget->setItem(row,0,new QTableWidgetItem(strTime));//给表格添加时间戳
ui->tableWidget->setItem(row,1,new QTableWidgetItem(strCANID));//给表格添加ID
ui->tableWidget->item(row,1)->setTextAlignment(Qt::AlignHCenter | Qt::AlignVCenter);//水平竖直居中
ui->tableWidget->setItem(row,2,new QTableWidgetItem(strFormat));//给表格添加帧格式(数据帧还是远程帧)
ui->tableWidget->item(row,2)->setTextAlignment(Qt::AlignHCenter | Qt::AlignVCenter);//水平竖直居中
ui->tableWidget->setItem(row,3,new QTableWidgetItem


的
- 粉丝: 26
- 资源: 2
最新资源
- 微信社团小程序ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 校园综合服务小程序+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 微信平台签到系统的设计与实现springboot-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 微信小程序的高校党费收缴系统ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 学生活动管理系统+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 新闻资讯微信小程序开发后端+php-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 自动驾驶控制器,基于分布式驱动电动汽车的四轮侧偏刚度估计,采用容积卡尔曼(ckf)进行估计,能够很好地估计汽车行驶过程中的侧偏刚度,并与转化的侧向轮胎力进行比较,具有很好的估计效果 模型中第一个模块
- 新闻资讯系统设计+springboot-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 数据结构PTA理论题答案.zip
- 微信小程序的驾校预约管理系统--论文-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 微信小程序的英语学习激励系统--论文-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 微信小程序基于BS模式的学生实习与就业管理系统设计与实现springboot-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 学生知识成果展示与交流+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 学习自律养成小程序+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 医院挂号系统设计与实现+ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 微信小程序评分小程序ssm-微信小程序毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


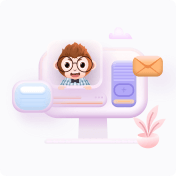