"no use strict";
!(function(window) {
if (typeof window.window != "undefined" && window.document)
return;
if (window.require && window.define)
return;
if (!window.console) {
window.console = function() {
var msgs = Array.prototype.slice.call(arguments, 0);
postMessage({type: "log", data: msgs});
};
window.console.error =
window.console.warn =
window.console.log =
window.console.trace = window.console;
}
window.window = window;
window.ace = window;
window.onerror = function(message, file, line, col, err) {
postMessage({type: "error", data: {
message: message,
data: err && err.data,
file: file,
line: line,
col: col,
stack: err && err.stack
}});
};
window.normalizeModule = function(parentId, moduleName) {
// normalize plugin requires
if (moduleName.indexOf("!") !== -1) {
var chunks = moduleName.split("!");
return window.normalizeModule(parentId, chunks[0]) + "!" + window.normalizeModule(parentId, chunks[1]);
}
// normalize relative requires
if (moduleName.charAt(0) == ".") {
var base = parentId.split("/").slice(0, -1).join("/");
moduleName = (base ? base + "/" : "") + moduleName;
while (moduleName.indexOf(".") !== -1 && previous != moduleName) {
var previous = moduleName;
moduleName = moduleName.replace(/^\.\//, "").replace(/\/\.\//, "/").replace(/[^\/]+\/\.\.\//, "");
}
}
return moduleName;
};
window.require = function require(parentId, id) {
if (!id) {
id = parentId;
parentId = null;
}
if (!id.charAt)
throw new Error("worker.js require() accepts only (parentId, id) as arguments");
id = window.normalizeModule(parentId, id);
var module = window.require.modules[id];
if (module) {
if (!module.initialized) {
module.initialized = true;
module.exports = module.factory().exports;
}
return module.exports;
}
if (!window.require.tlns)
return console.log("unable to load " + id);
var path = resolveModuleId(id, window.require.tlns);
if (path.slice(-3) != ".js") path += ".js";
window.require.id = id;
window.require.modules[id] = {}; // prevent infinite loop on broken modules
importScripts(path);
return window.require(parentId, id);
};
function resolveModuleId(id, paths) {
var testPath = id, tail = "";
while (testPath) {
var alias = paths[testPath];
if (typeof alias == "string") {
return alias + tail;
} else if (alias) {
return alias.location.replace(/\/*$/, "/") + (tail || alias.main || alias.name);
} else if (alias === false) {
return "";
}
var i = testPath.lastIndexOf("/");
if (i === -1) break;
tail = testPath.substr(i) + tail;
testPath = testPath.slice(0, i);
}
return id;
}
window.require.modules = {};
window.require.tlns = {};
window.define = function(id, deps, factory) {
if (arguments.length == 2) {
factory = deps;
if (typeof id != "string") {
deps = id;
id = window.require.id;
}
} else if (arguments.length == 1) {
factory = id;
deps = [];
id = window.require.id;
}
if (typeof factory != "function") {
window.require.modules[id] = {
exports: factory,
initialized: true
};
return;
}
if (!deps.length)
// If there is no dependencies, we inject "require", "exports" and
// "module" as dependencies, to provide CommonJS compatibility.
deps = ["require", "exports", "module"];
var req = function(childId) {
return window.require(id, childId);
};
window.require.modules[id] = {
exports: {},
factory: function() {
var module = this;
var returnExports = factory.apply(this, deps.slice(0, factory.length).map(function(dep) {
switch (dep) {
// Because "require", "exports" and "module" aren't actual
// dependencies, we must handle them seperately.
case "require": return req;
case "exports": return module.exports;
case "module": return module;
// But for all other dependencies, we can just go ahead and
// require them.
default: return req(dep);
}
}));
if (returnExports)
module.exports = returnExports;
return module;
}
};
};
window.define.amd = {};
window.require.tlns = {};
window.initBaseUrls = function initBaseUrls(topLevelNamespaces) {
for (var i in topLevelNamespaces)
this.require.tlns[i] = topLevelNamespaces[i];
};
window.initSender = function initSender() {
var EventEmitter = window.require("ace/lib/event_emitter").EventEmitter;
var oop = window.require("ace/lib/oop");
var Sender = function() {};
(function() {
oop.implement(this, EventEmitter);
this.callback = function(data, callbackId) {
postMessage({
type: "call",
id: callbackId,
data: data
});
};
this.emit = function(name, data) {
postMessage({
type: "event",
name: name,
data: data
});
};
}).call(Sender.prototype);
return new Sender();
};
var main = window.main = null;
var sender = window.sender = null;
window.onmessage = function(e) {
var msg = e.data;
if (msg.event && sender) {
sender._signal(msg.event, msg.data);
}
else if (msg.command) {
if (main[msg.command])
main[msg.command].apply(main, msg.args);
else if (window[msg.command])
window[msg.command].apply(window, msg.args);
else
throw new Error("Unknown command:" + msg.command);
}
else if (msg.init) {
window.initBaseUrls(msg.tlns);
sender = window.sender = window.initSender();
var clazz = this.require(msg.module)[msg.classname];
main = window.main = new clazz(sender);
}
};
})(this);
ace.define("ace/lib/oop",[], function(require, exports, module){"use strict";
exports.inherits = function (ctor, superCtor) {
ctor.super_ = superCtor;
ctor.prototype = Object.create(superCtor.prototype, {
constructor: {
value: ctor,
enumerable: false,
writable: true,
configurable: true
}
});
};
exports.mixin = function (obj, mixin) {
for (var key in mixin) {
obj[key] = mixin[key];
}
return obj;
};
exports.implement = function (proto, mixin) {
exports.mixin(proto, mixin);
};
});
ace.define("ace/apply_delta",[], function(require, exports, module){"use strict";
function throwDeltaError(delta, errorText) {
console.log("Invalid Delta:", delta);
throw "Invalid Delta: " + errorText;
}
function positionInDocument(docLines, position) {
return position.row >= 0 && position.row < docLines.length &&
position.column >= 0 && position.column <= docLines[position.row].length;
}
function validateDelta(docLines, delta) {
if (delta.action != "insert" && delta.action != "remove")
throwDeltaError(delta, "delta.action must be 'insert' or 'remove'");
if (!(delta.lines instanceof Array))
throwDeltaError(delta, "delta.lines must be an Array");
if (!delta.start || !delta.end)
throwDeltaError(delta, "delta.start/end must be an present");
var start = delta.start;
if (!positionInDocument(docLines, delta.start))
throwDeltaError(delta, "delta.start must be contained in
没有合适的资源?快使用搜索试试~ 我知道了~
aceEditor 在线代码编辑器
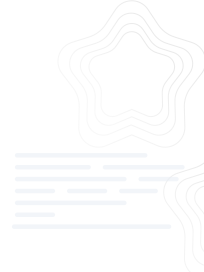
共2000个文件
js:1886个
css:51个
html:24个

需积分: 5 1 下载量 187 浏览量
2024-07-17
15:16:58
上传
评论
收藏 12.02MB ZIP 举报
温馨提示
ACE Editor是一个开源的、独立的、基于浏览器的代码编辑器,它可以嵌入到任何web页面或JavaScript应用程序中。ACE提供了丰富的功能,包括对超过60种语言的语法高亮支持,能够处理多达400万行的大型文档。ACE在性能和功能上可以媲美本地代码编辑器,如Sublime Text、TextMate和Vim等。它是Mozilla Skywriter(以前称为Bespin)项目的继任者,并作为Cloud9的主要在线编辑器。 ACE编辑器的特性包括: 语法着色:支持60多种语言的语法着色,可以导入TextMate/.tmlanguage和Sublime/.tmlanguage文件。 主题支持:提供20多种主题,可以导入TextMate/.tmtheme文件。 自动缩进和减少缩进。 可选的命令行。 处理巨大文件:能够处理4,000,000行代码。 完全自定义的键绑定,包括V正则表达式搜索和替换。 高亮匹配括号。 软标签和真正的标签之间切换。 显示隐藏的字符。 用鼠标拖放文本。 换行。 代码折叠。 多个光标和选择。
资源推荐
资源详情
资源评论
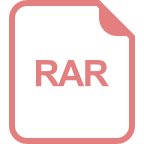
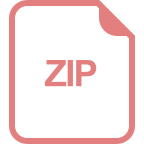
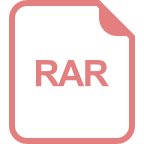
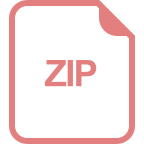
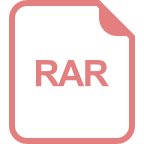
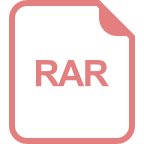
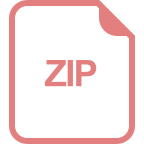
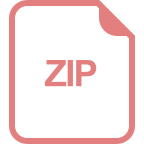
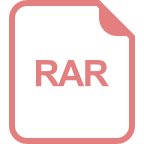
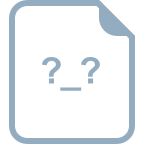
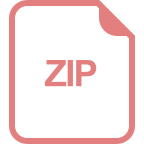
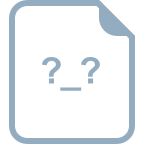
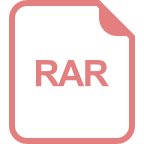
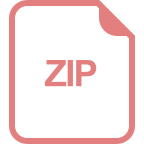
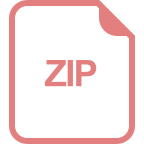
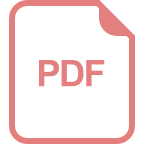
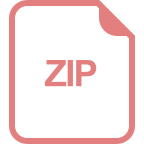
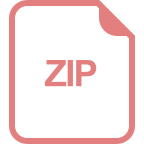
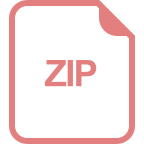
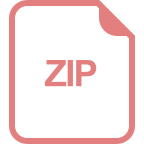
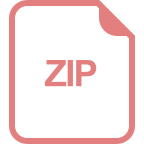
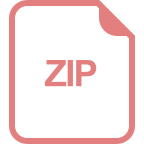
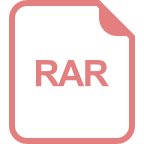
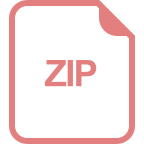
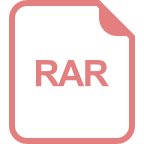
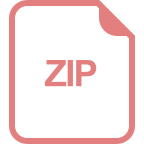
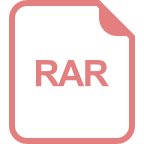
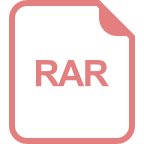
收起资源包目录

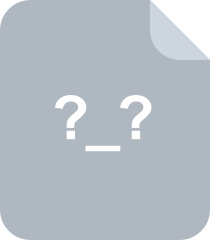
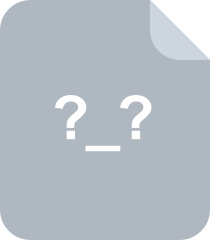
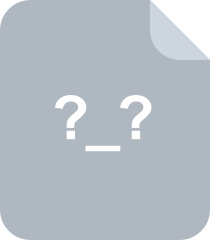
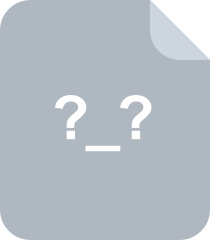
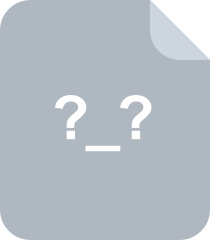
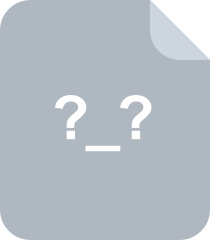
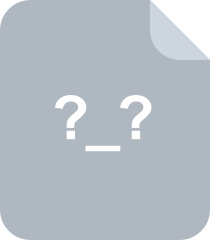
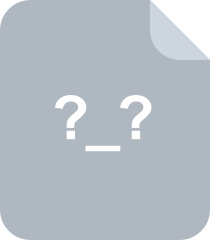
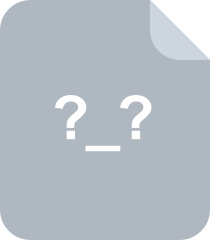
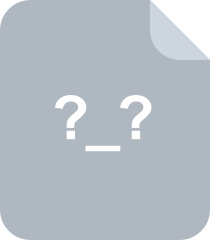
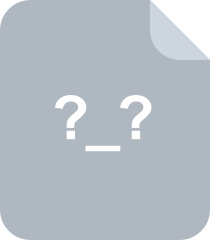
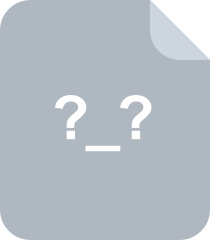
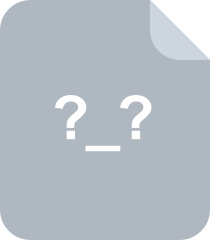
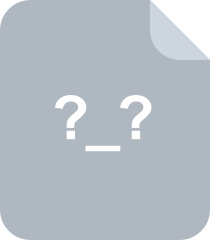
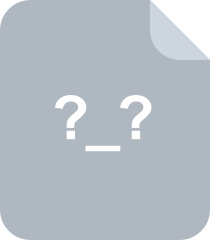
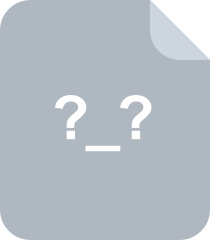
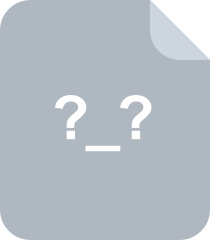
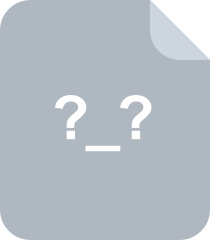
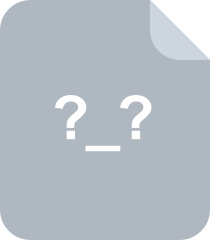
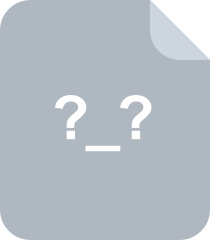
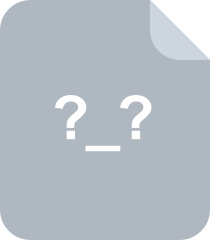
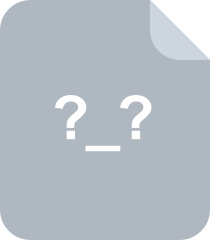
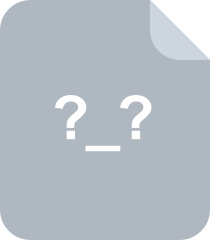
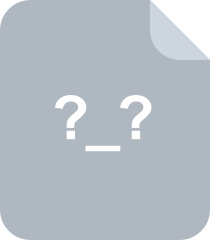
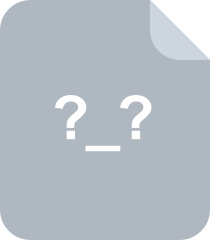
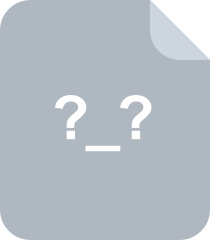
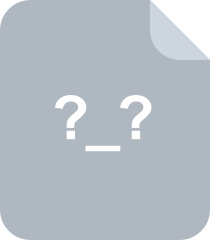
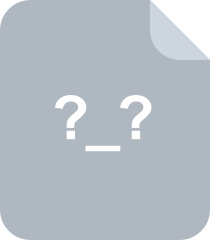
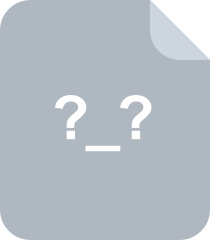
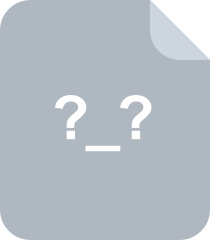
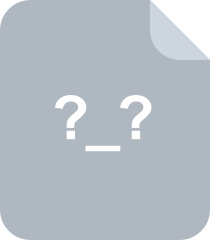
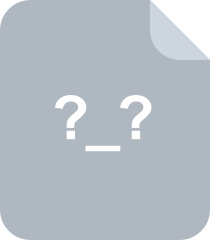
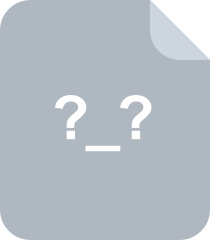
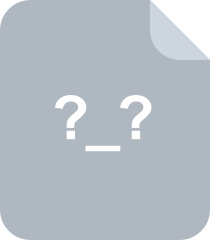
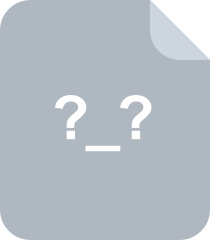
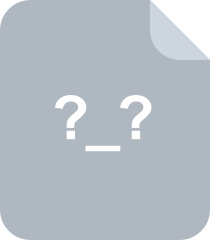
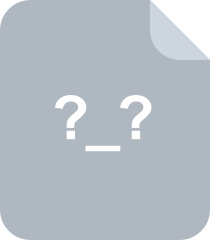
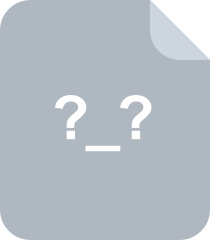
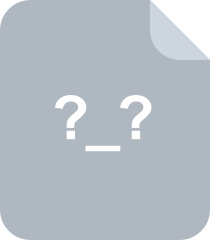
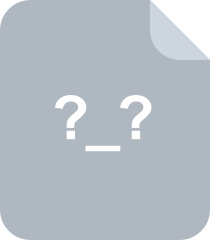
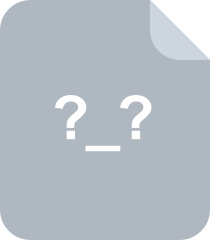
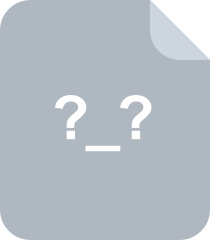
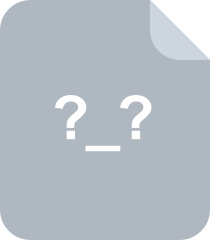
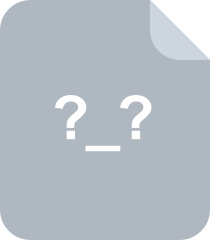
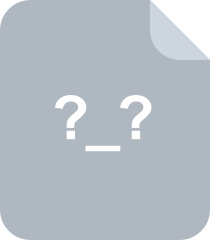
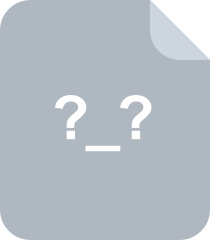
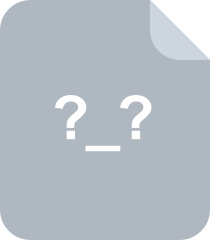
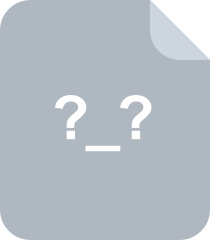
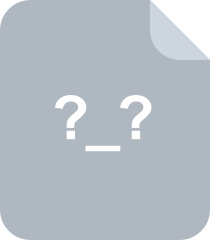
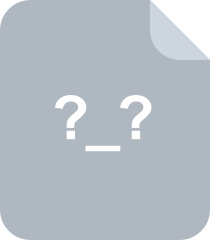
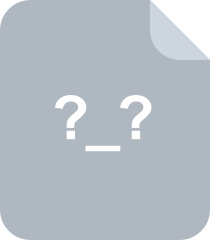
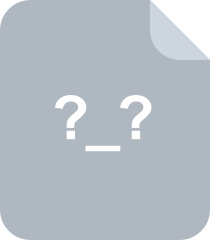
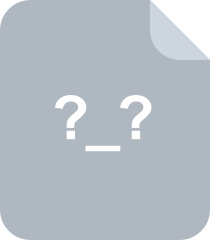
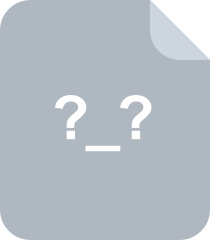
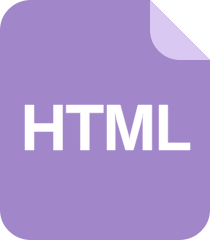
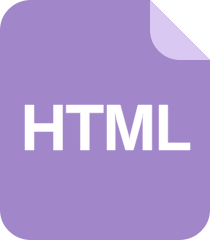
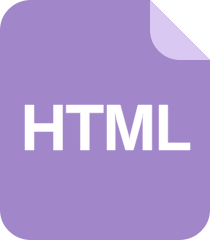
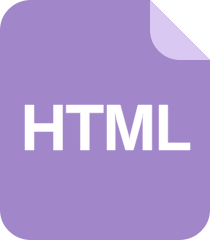
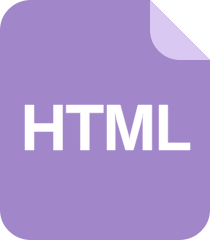
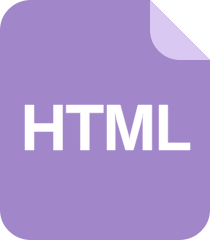
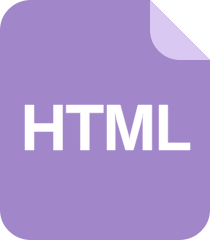
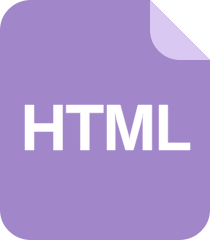
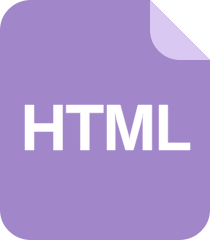
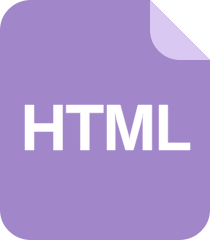
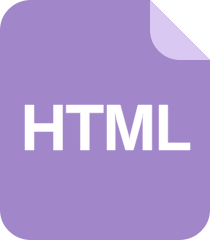
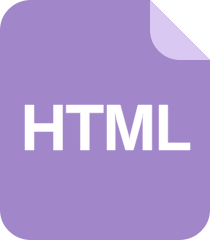
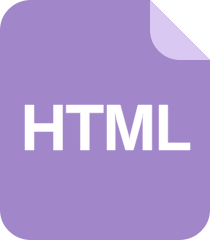
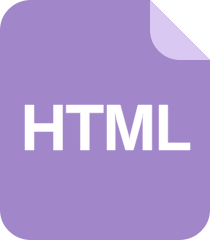
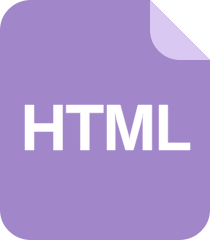
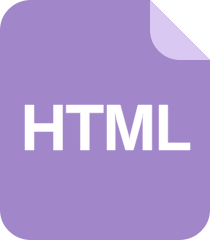
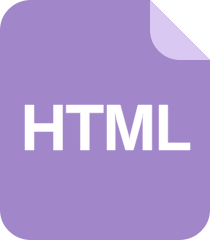
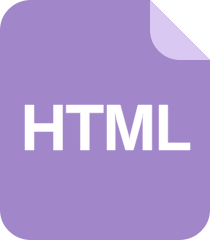
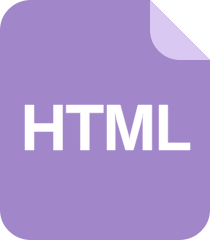
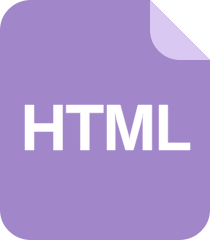
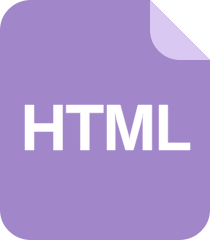
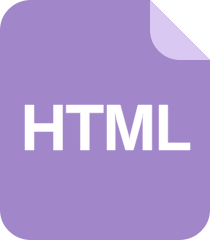
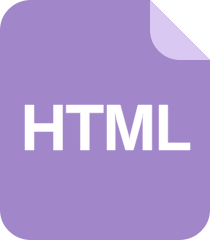
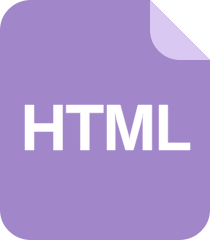
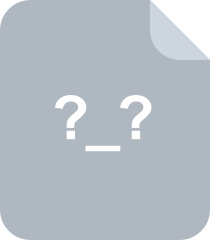
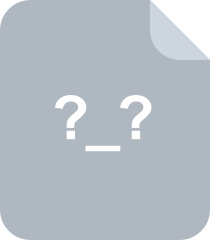
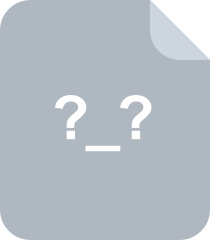
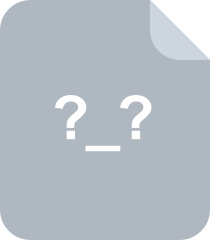
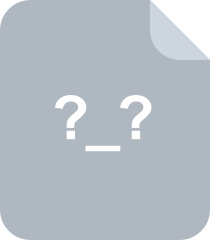
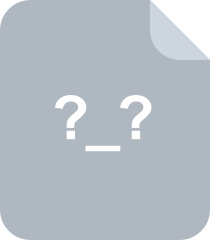
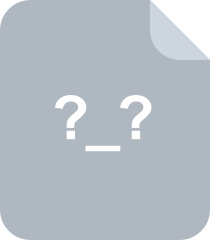
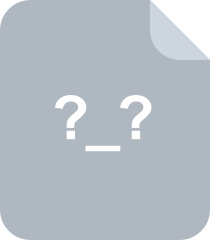
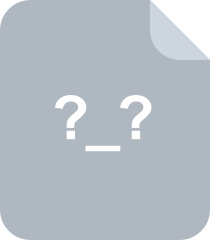
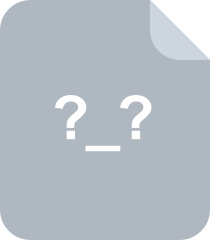
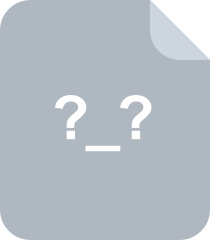
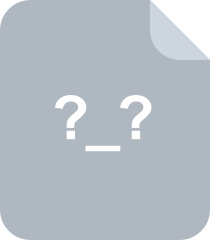
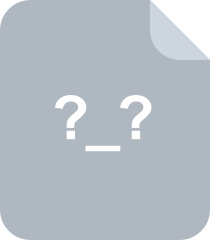
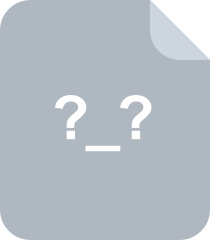
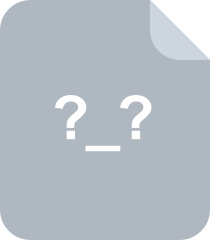
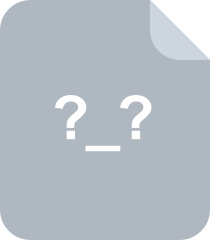
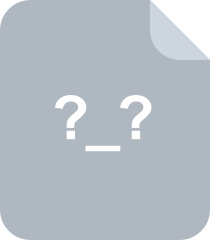
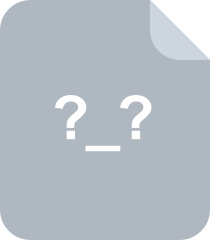
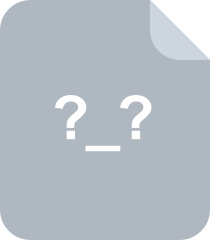
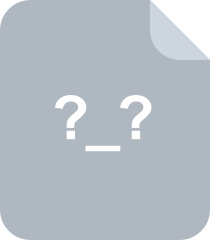
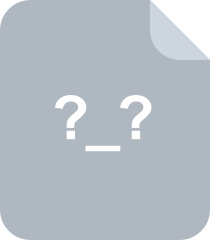
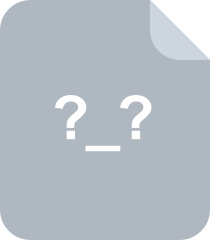
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
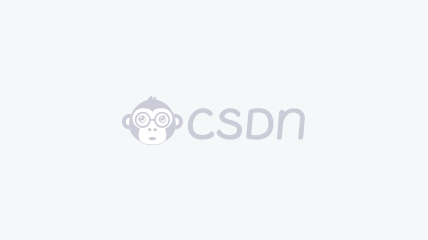


博_泷
- 粉丝: 2
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

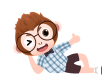
最新资源
- 数据库课程设计-基于的个性化购物平台的建表语句.sql
- 数据库课程设计-基于的图书智能一体化管理系统的建表语句.sql
- Java 代码覆盖率库.zip
- Java 代码和算法的存储库 也为该存储库加注星标 .zip
- 免安装Windows10/Windows11系统截图工具,无需安装第三方截图工具 双击直接使用截图即可 是一款免费可靠的截图小工具哦~
- Libero Soc v11.9的安装以及证书的获取(2021新版).zip
- BouncyCastle.Cryptography.dll
- 5.1 孤立奇点(JD).ppt
- 基于51单片机的智能交通灯控制系统的设计与实现源码+报告(高分项目)
- 什么是 SQL 注入.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


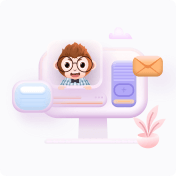
安全验证
文档复制为VIP权益,开通VIP直接复制
