# ecStat
A statistical and data mining tool for [Apache ECharts (incubating)](https://github.com/apache/incubator-echarts). You can use it to analyze data and then visualize the results with ECharts, or just use it to process data.
It works both in node.js and in the browser.
*Read this in other languages: [English](README.md), [简体中文](README.zh-CN.md).*
## Installing
If you use npm, you can install it with:
```sh
npm install echarts-stat
```
Otherwise, download this tool from [dist directory](https://github.com/ecomfe/echarts-stat/tree/master/dist):
```html
<script src='./dist/ecStat.js'></script>
<script>
var result = ecStat.clustering.hierarchicalKMeans(data, clusterNumber, false);
</script>
```
## API Reference
* [Histogram](#histogram)
* [Clustering](#clustering)
* [Regression](#regression)
* [Statistics](#statistic)
### Histogram
A histogram is a graphical representation of the distribution of numerical data. It is an estimate of the probability distribution of a quantitative variable. It is a kind of bar graph. To construct a histogram, the first step is to "bin" the range of values - that is, divide the entire range of values into a series of intervals - and then count how many original sample values fall into each interval. The bins are usually specified as consecutive, non-overlapping intervals of a variable. Here the bins(intervals) must be adjacent, and are of equal size.
#### Syntax
* Used as echarts transform (since echarts 5.0)
```js
echarts.registerTransform(ecStat.transform.histogram);
```
```js
chart.setOption({
dataset: [{
source: data
}, {
type: 'ecStat:histogram',
config: config
}],
...
});
```
* Standalone
```js
var bins = ecStat.histogram(data, config);
// or
var bins = ecStat.histogram(data, method);
```
##### Parameter
* `data` - `number[] | number[][]`. Data samples of numbers.
```js
// One-dimension array
var data = [8.6, 8.8, 10.5, 10.7, 10.8, 11.0, ... ];
```
or
```js
// Two-dimension array
var data = [[8.3, 143], [8.6, 214], ...];
```
* `config` - `object`.
* `config.method` - `'squareRoot' | 'scott' | 'freedmanDiaconis' | 'sturges'`. Optional. Methods to calculate the number of bin. There is no "best" number of bin, and different bin size can reveal different feature of data.
* `squareRoot` - This is the default method, which is also used by Excel histogram. Returns the number of bin according to [Square-root choice](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition):
```js
var bins = ecStat.histogram(data);
```
* `scott` - Returns the number of bin according to [Scott's normal reference Rule](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition):
```js
var bins = ecStat.histogram(data, 'scott');
```
* `freedmanDiaconis` - Returns the number of bin according to [The Freedman-Diaconis rule](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition):
```js
var bins = ecStat.histogram(data, 'freedmanDiaconis');
```
* `sturges` - Returns the number of bin according to [Sturges' formula](https://en.wikipedia.org/wiki/Histogram#Mathematical_definition):
```js
var bins = ecStat.histogram(data, 'sturges');
```
* `config.dimensions` - `(number | string)`. Optional. Specify the dimensions of data that are used to regression calculation. By default `0`, which means the column 0 and 1 is used in the regression calculation. In echarts transform usage, both dimension name (`string`) and dimension index (`number`) can be specified. In standalone usage, only dimension index can be specified (not able to define dimension name).
##### Return Value (only for standalone usage)
* Used as echarts transform (since echarts 5.0)
```js
dataset: [{
source: [...]
}, {
transform: 'ecStat:histogram'
// // The result data of this dataset is like:
// [
// // MeanOfV0V1, VCount, V0, V1, DisplayableName
// [ 10, 212 8, 12, '8 - 12'],
// ...
// ]
// // The rest of the input dimensions that other than
// // config.dimensions specified are kept in the output.
}]
```
* Standalone
* `result` - `object`. Contain detailed messages of each bin and data used for [ECharts](https://github.com/ecomfe/echarts) to draw the histogram.
* `result.bins` - `BinItem[]`. An array of bins, where each bin is an object (`BinItem`), containing three attributes:
* `x0` - `number`. The lower bound of the bin (inclusive).
* `x1` - `number`. The upper bound of the bin (exclusive).
* `sample` - `number[]`. Containing the associated elements from the input data.
* `result.data` - `[MeanOfV0V1, VCount, V0, V1, DisplayableName][]`. Used for bar chart to draw the histogram, where the length of `sample` is the number of sample values in this bin. For example:
```js
var bins.data = [
// MeanOfV0V1, VCount, V0, V1, DisplayableName
[ 10, 212, 8, 12, '8 - 12'],
...
];
// The rest of the input dimensions that other than
// config.dimensions specified are kept in the output.
```
* `result.customData` - `[V0, V1, VCount][]`. Used for custom chart to draw the histogram, where the length of `sample` is the number of sample values in this bin.
#### Examples
[test/transform/histogram_bar.html](https://github.com/ecomfe/echarts-stat/blob/master/test/transform/histogram_bar.html)
[test/standalone/histogram_bar.html](https://github.com/ecomfe/echarts-stat/blob/master/test/standalone/histogram_bar.html)

[Run](http://gallery.echartsjs.com/editor.html?c=xBk5VZddJW)
### Clustering
Clustering can divide the original data set into multiple data clusters with different characteristics. And through [ECharts](https://github.com/ecomfe/echarts), you can visualize the results of clustering, or visualize the process of clustering.
#### Syntax
* Used as echarts transform (since echarts 5.0)
```js
echarts.registerTransform(ecStat.transform.clustering);
```
```js
chart.setOption({
dataset: [{
source: data
}, {
type: 'ecStat:clustering',
config: config
}],
...
});
```
* Standalone
```js
var result = ecStat.clustering.hierarchicalKMeans(data, config);
// or
var result = ecStat.clustering.hierarchicalKMeans(data, clusterCount, stepByStep);
```
##### Parameter
* `data` - `number[][]`. Two-dimensional numeric array, each data point can have more than two numeric attributes in the original data set. In the following example, `data[0]` is called `data point` and `data[0][1]` is one of the numeric attributes of `data[0]`.
```js
var data = [
[232, 4.21, 51, 0.323, 19],
[321, 1.62, 18, 0.139, 10],
[551, 11.21, 13, 0.641, 15],
...
];
```
* `config` - `object`.
* `config.clusterCount` - `number`. Mandatory. The number of clusters generated. **Note that it must be greater than 1.**
* `config.dimensions` - `(number | string)[]`. Optional. Specify which dimensions (columns) of data will be used to clustering calculation. The other columns will also be kept in the output data. By default all of the columns of the data will be used as dimensions. In echarts transform usage, both dimension name (`string`) and dimension index (`number`) can be specified. In standalone usage, only dimension index can be specified (not able to define dimension name).
* `config.stepByStep` - `boolean`. Op
没有合适的资源?快使用搜索试试~ 我知道了~
大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echar
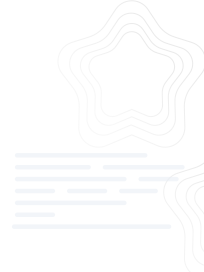
共507个文件
gif:150个
js:76个
xml:66个

需积分: 5 0 下载量 170 浏览量
2024-11-06
11:10:04
上传
评论
收藏 35.84MB 7Z 举报
温馨提示
大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大数据广告echarts图标展示源码大
资源推荐
资源详情
资源评论
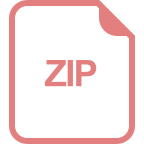
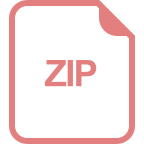
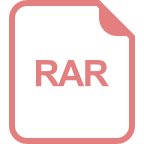
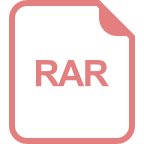
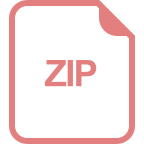
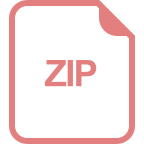
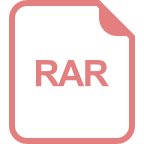
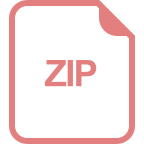
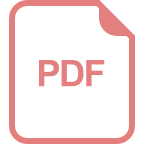
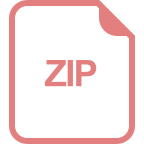
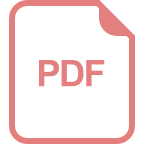
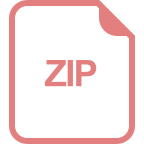
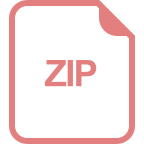
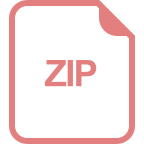
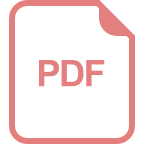
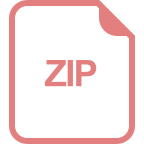
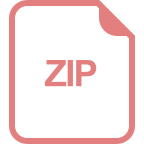
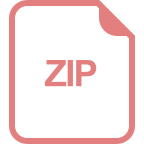
收起资源包目录

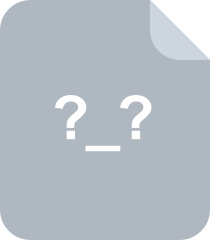
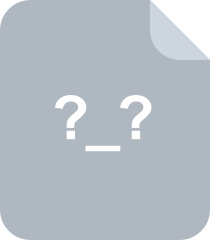
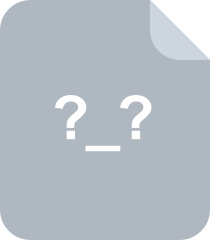
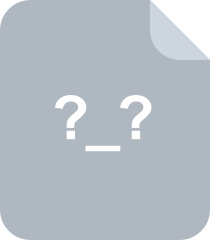
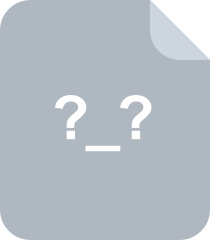
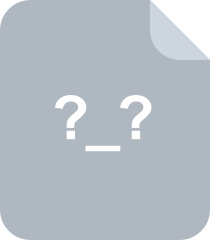
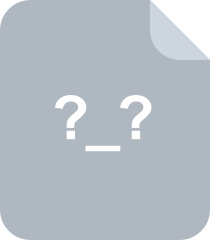
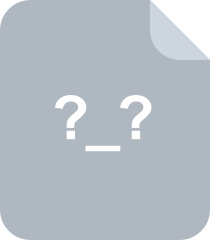
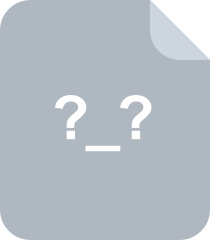
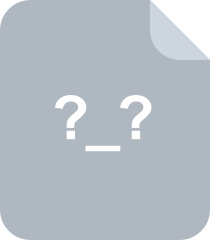
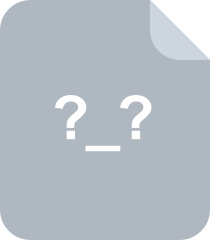
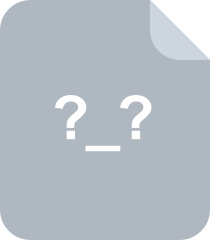
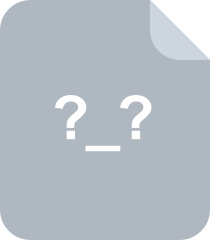
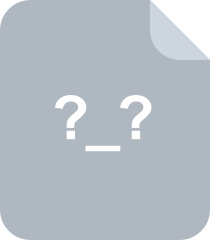
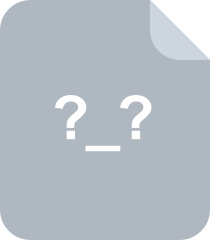
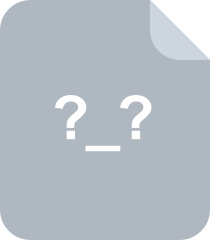
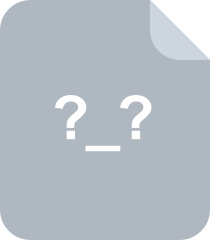
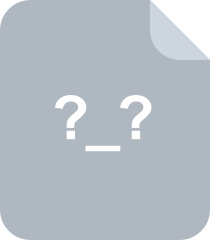
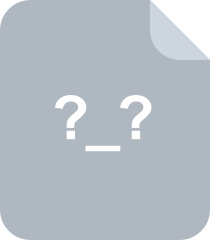
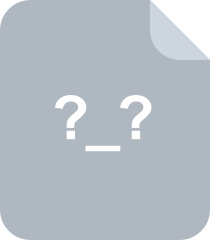
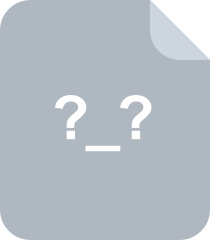
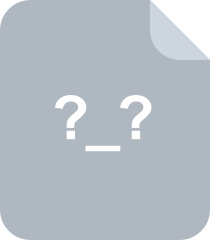
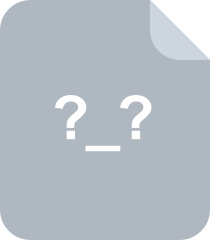
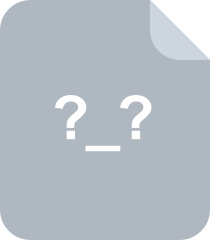
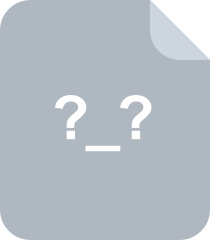
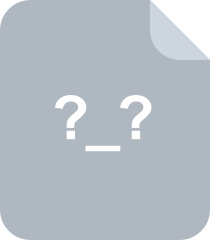
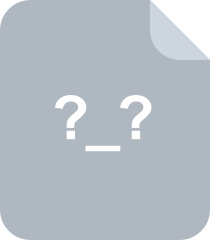
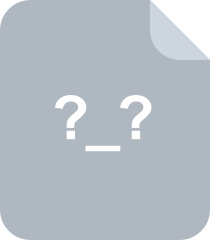
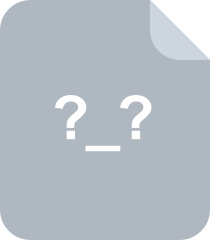
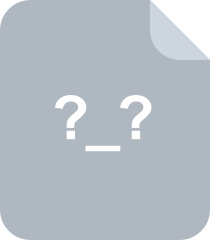
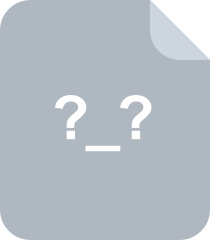
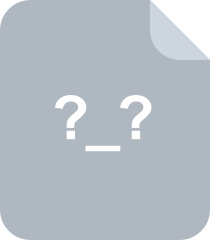
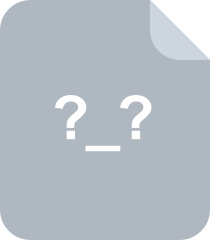
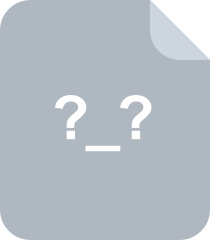
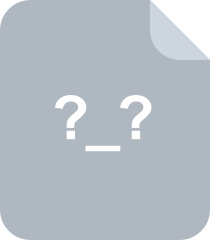
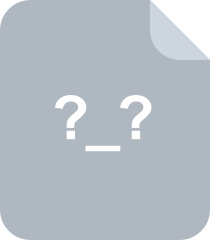
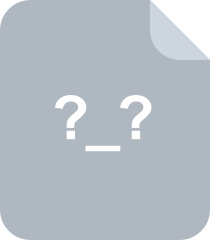
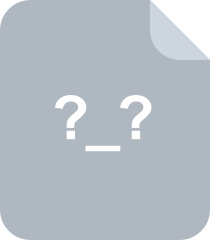
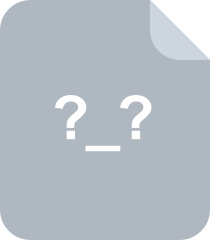
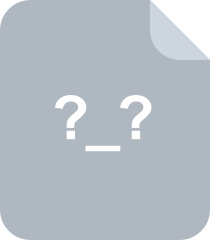
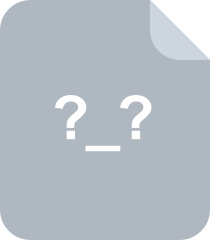
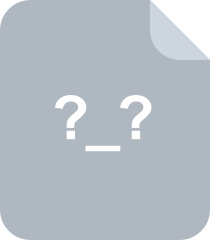
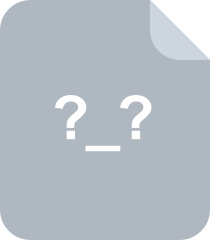
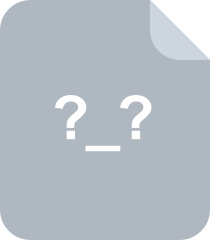
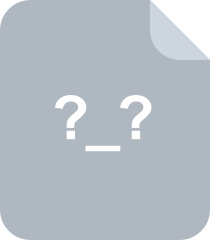
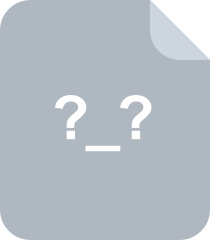
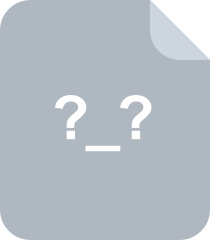
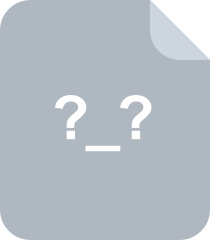
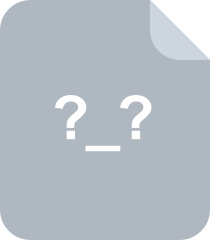
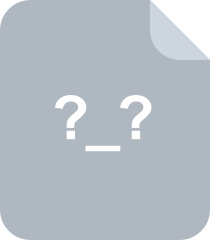
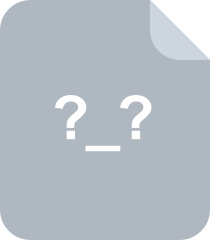
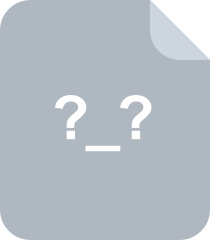
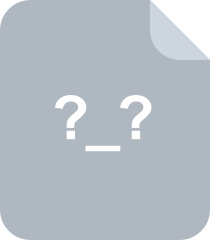
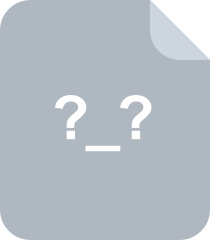
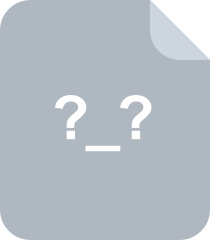
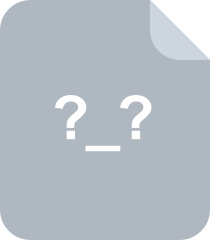
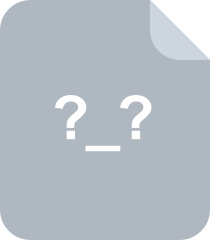
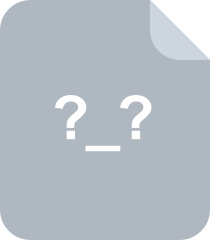
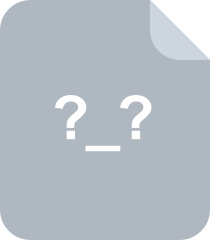
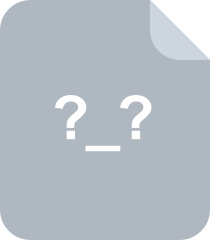
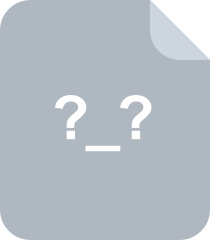
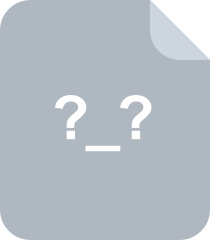
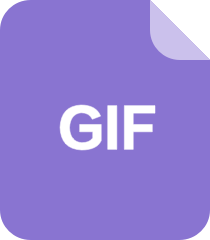
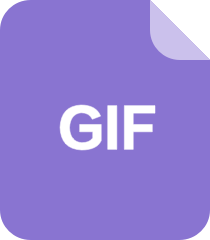
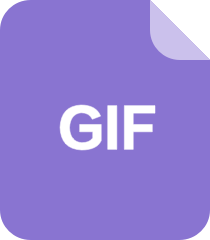
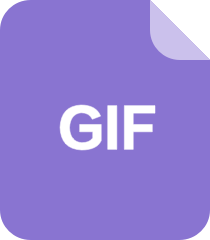
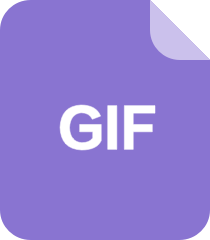
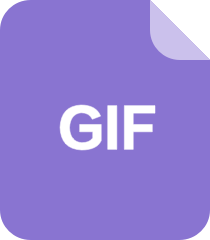
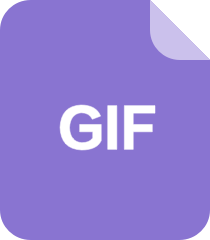
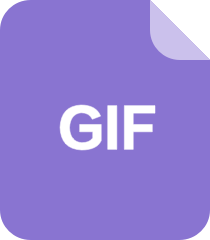
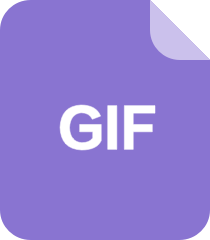
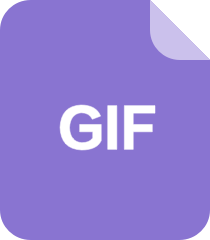
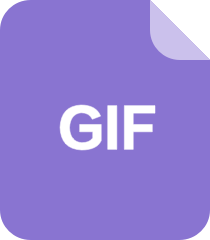
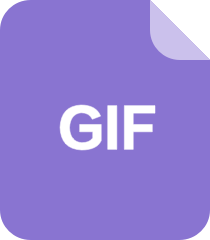
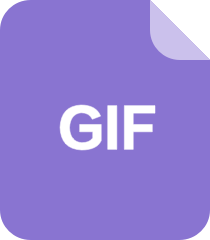
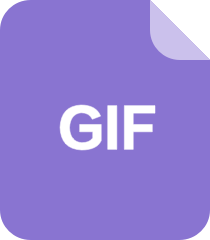
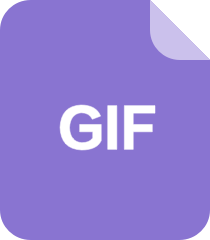
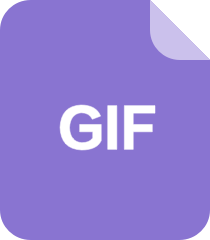
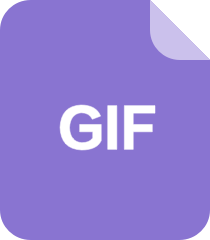
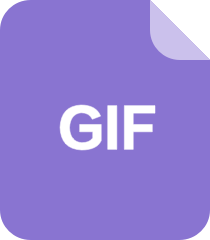
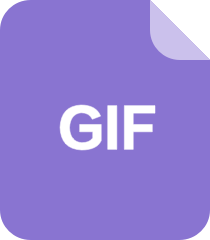
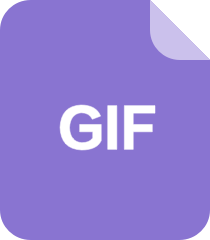
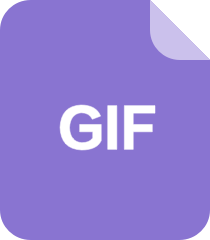
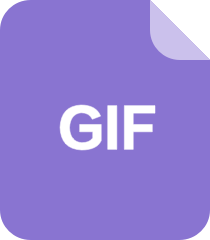
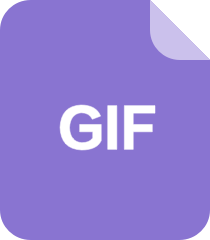
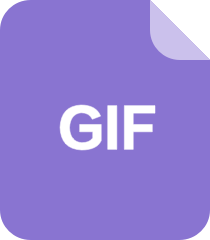
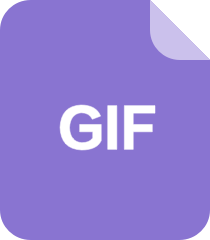
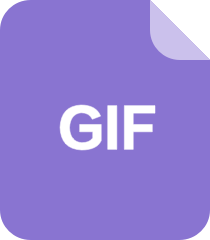
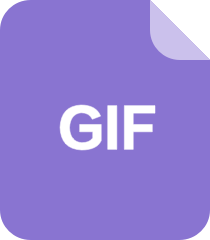
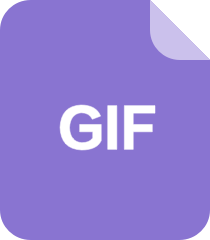
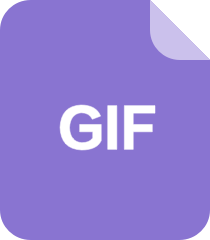
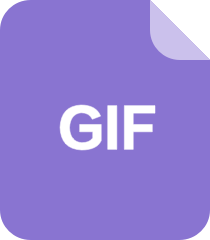
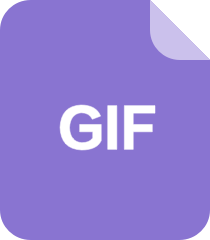
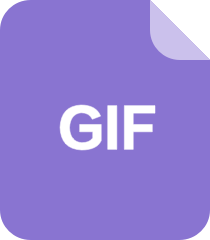
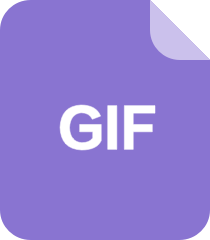
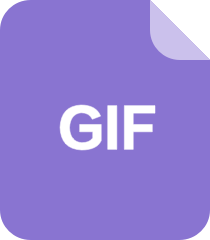
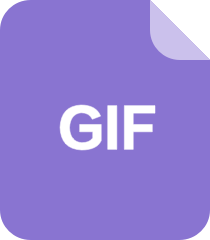
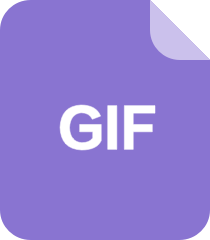
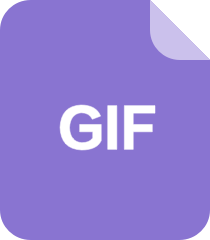
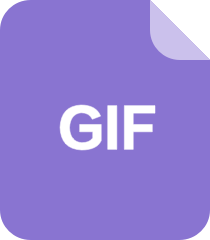
共 507 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
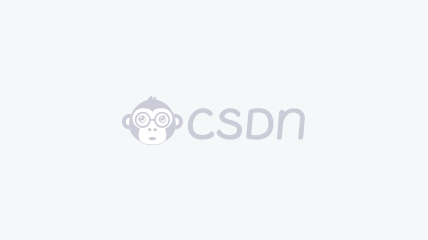

weixin_15624157576
- 粉丝: 52
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

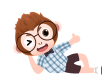
安全验证
文档复制为VIP权益,开通VIP直接复制
