没有合适的资源?快使用搜索试试~ 我知道了~
TypeScript与前端框架Angular教程.docx
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 128 浏览量
2024-08-28
07:46:30
上传
评论
收藏 35KB DOCX 举报
温馨提示
TypeScript与前端框架Angular教程.docx
资源推荐
资源详情
资源评论
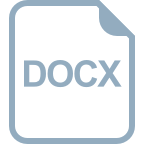
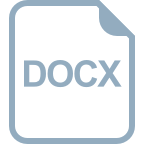
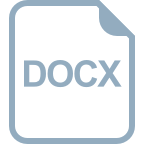
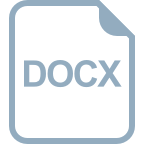
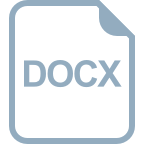
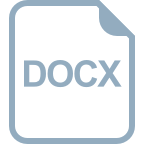
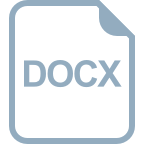
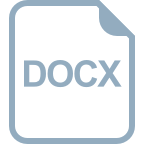
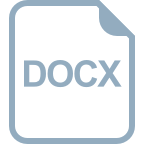
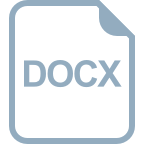
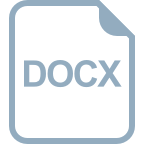
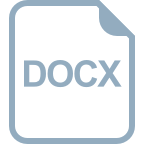
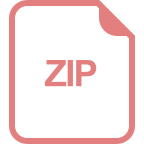
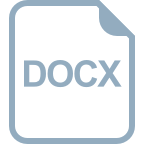
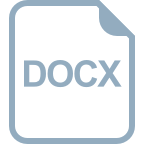
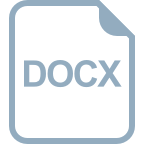
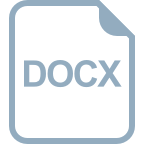
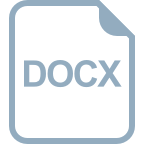
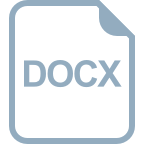
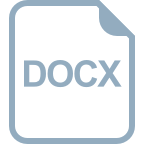
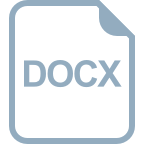
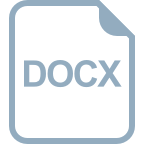
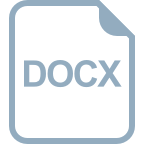
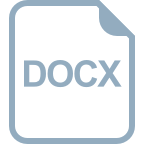
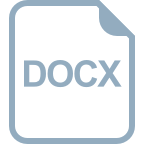
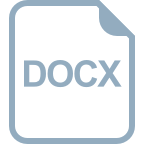
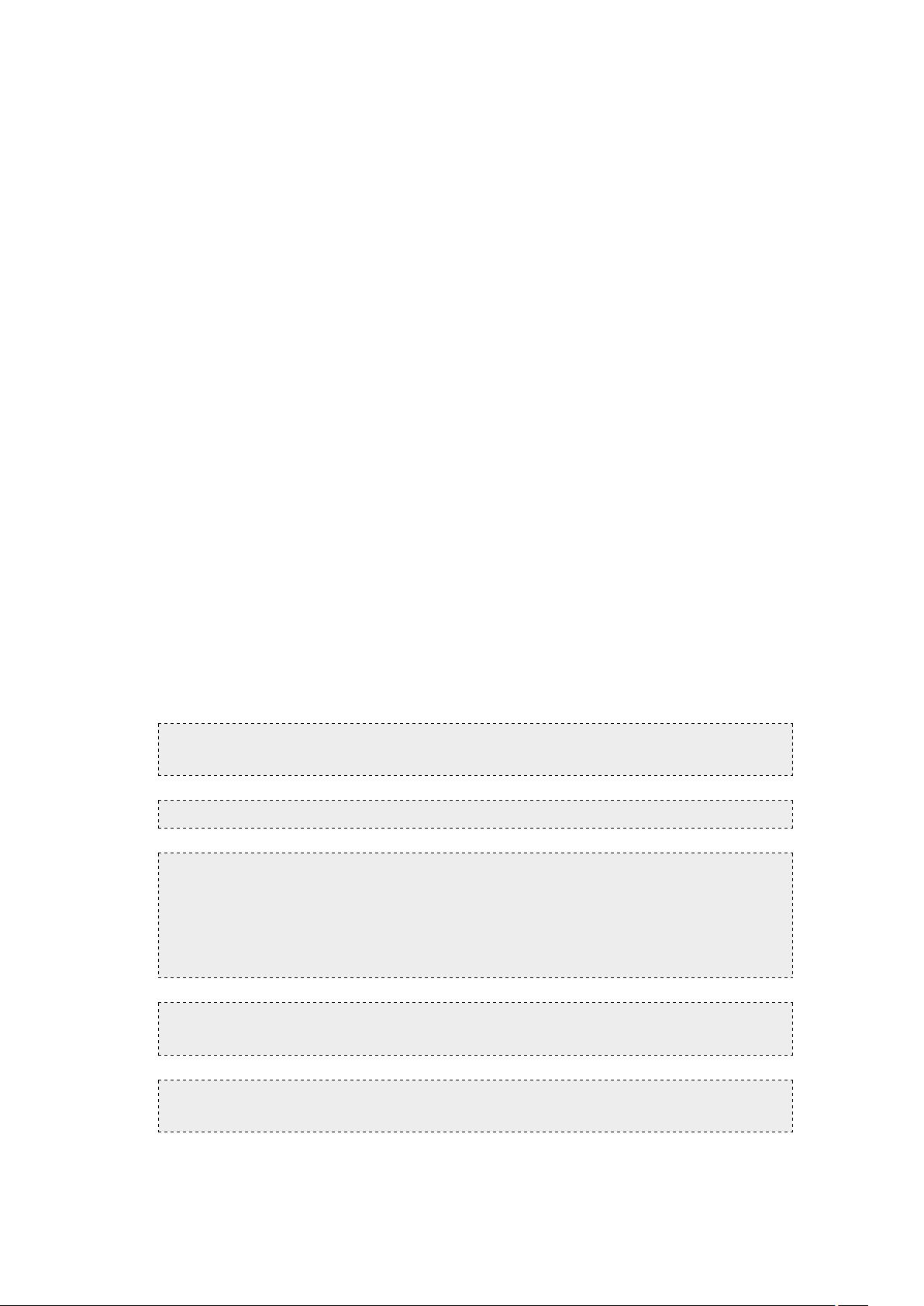
1
TypeScript 与前端框架 Angular 教程
1 TypeScript 基础
1.1 TypeScript 数据类型
TypeScript 是 JavaScript 的超集,它为 JavaScript 添加了静态类型系统。了解
TypeScript 的数据类型是掌握这门语言的基础。
1.1.1 基本数据类型
TypeScript 支持以下基本数据类型:
� number:用于数值。
� string:用于文本。
� boolean:用于布尔值,即 true 或 false。
� null 和 undefined:分别表示空值和未定义的值。
� void:表示没有类型,常用于函数返回值。
� never:表示永远不会出现的值,如抛出异常的函数的返回类型。
� any:表示任何类型,通常在类型未知时使用,但应尽量避免以保
持类型安全性。
1.1.2 复合数据类型
� 数组:可以使用 type[] 或 Array<type> 来定义数组类型。
let numbers: number[] = [1, 2, 3];
let strings: Array<string> = ['a', 'b', 'c'];
� 元组:允许一个数组有固定数量和类型的元素。
let tuple: [number, string] = [1, 'hello'];
� 对象:可以定义具有特定属性的对象类型。
interface Person {
name: string;
age: number;
}
let person: Person = { name: 'John', age: 30 };
� 枚举:用于定义一组命名的常量。
enum Color { Red, Green, Blue }
let c: Color = Color.Green;
� 联合类型:表示多种可能的类型。
let value: string | number = 'hello';
value = 100; //
也是合法的
� 交叉类型:组合多个类型为一个类型。
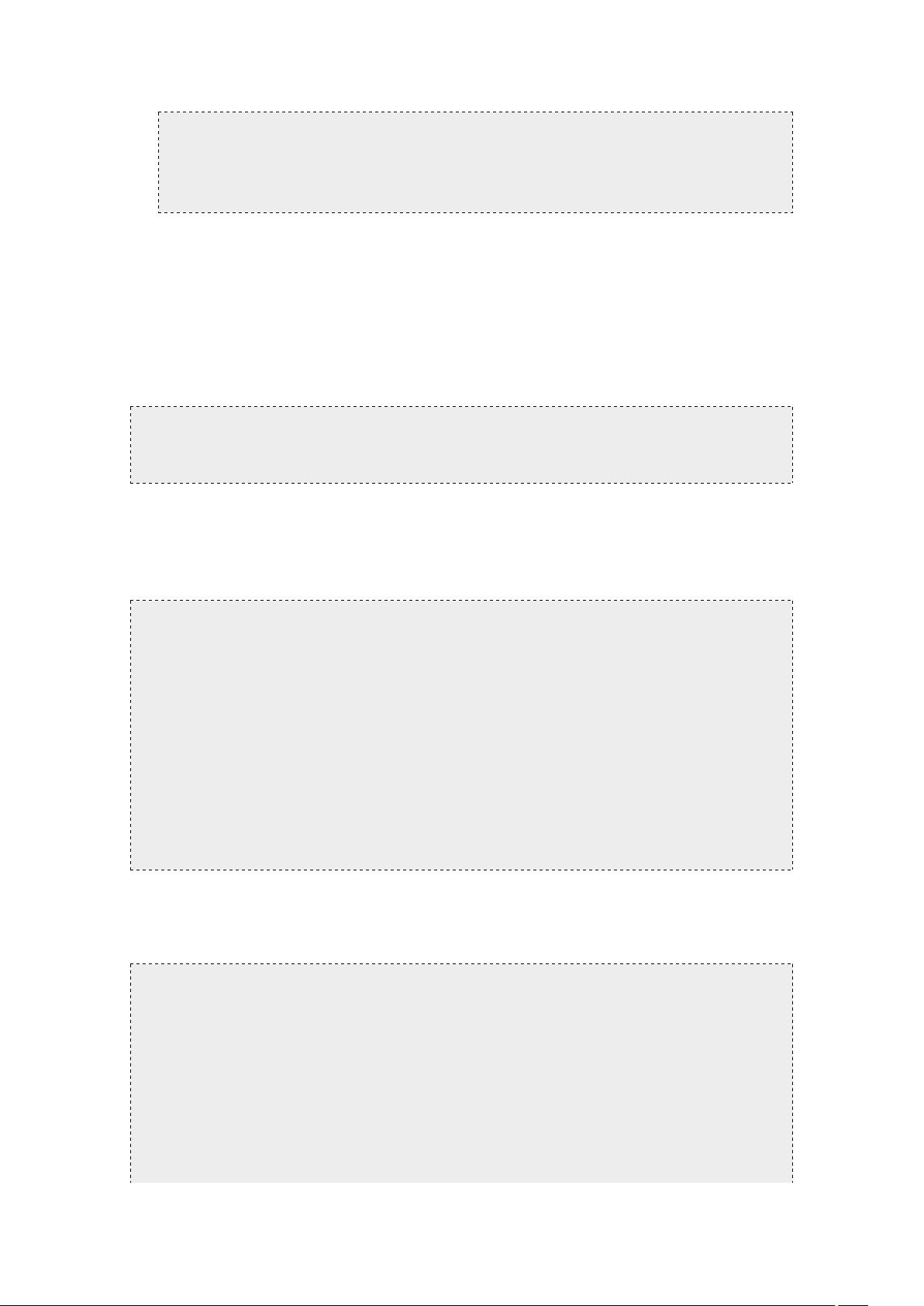
2
interface A { a: string; }
interface B { b: number; }
type AB = A & B;
let ab: AB = { a: 'hello', b: 100 };
1.2 TypeScript 函数与接口
1.2.1 函数类型
TypeScript 允许你定义函数的参数类型和返回类型,这有助于确保函数的正
确使用。
function add(x: number, y: number): number {
return x + y;
}
1.2.2 接口
接口(Interfaces)是 TypeScript 中用于定义对象类型的一种方式。它们可
以描述对象的形状,包括属性、方法和索引签名。
interface LabelledValue {
label: string;
value: number;
}
function printLabel(labelledObj: LabelledValue) {
console.log(labelledObj.label);
}
let myObj = { size: 10, label: 'Size 10 Object' };
printLabel(myObj); //
这将导致错误,因为
myObj
缺少
'value'
属性
1.2.3 泛型接口
泛型接口允许你创建可重用的接口,这些接口可以与任何类型一起工作。
interface Lengthwise {
length: number;
}
function loggingIdentity<T>(arg: T): T {
console.log(arg.length); //
这里
arg
必须是
string
或者
array
return arg;
}
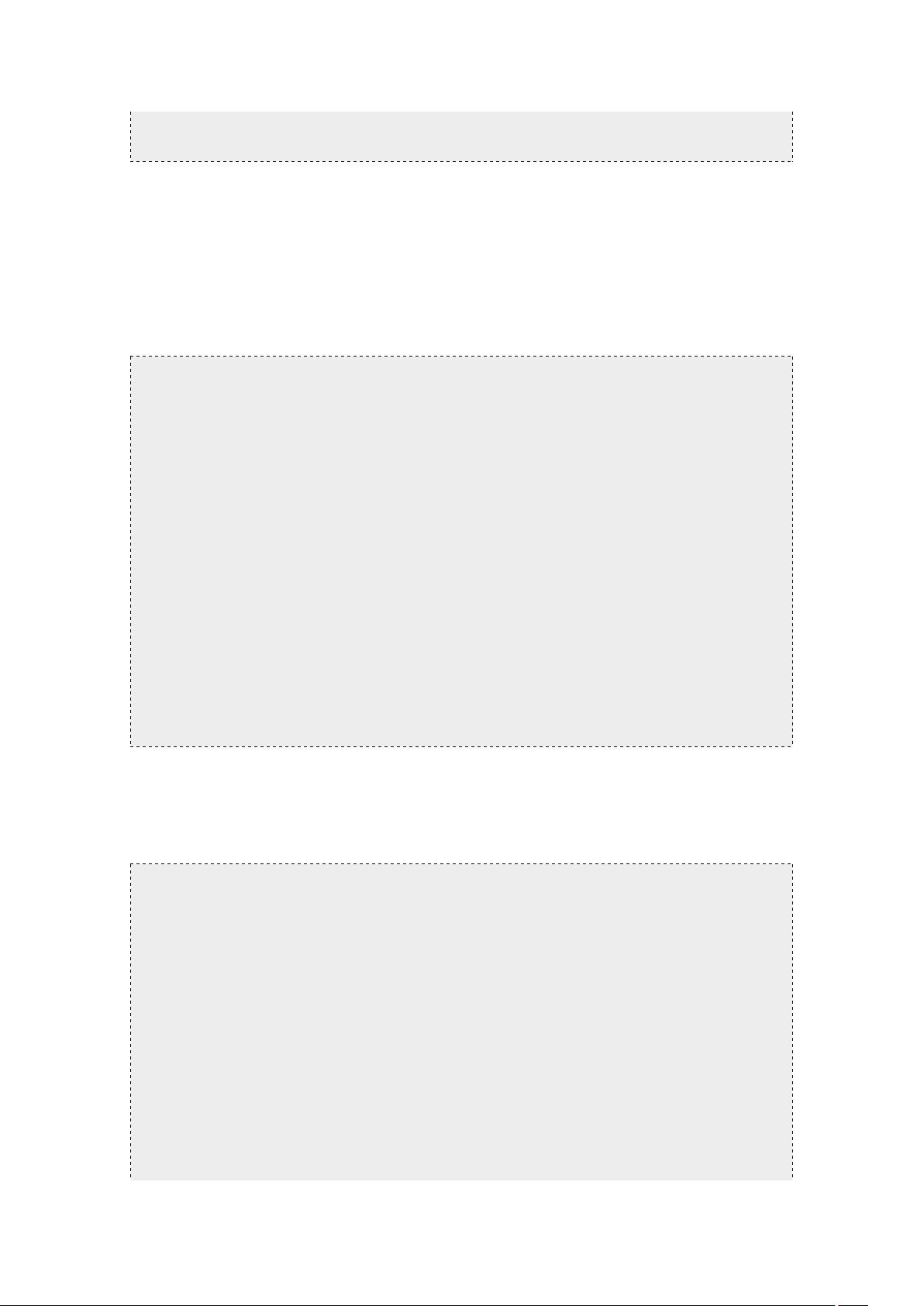
3
loggingIdentity({ length: 10, value: 3 }); //
这将导致错误,因为泛型参数
T
必须是
string
或
arr
ay
1.3 TypeScript 类与模块
1.3.1 类
TypeScript 的类是面向对象编程的基础。它们允许你定义具有属性和方法的
对象模板。
class Car {
brand: string;
model: string;
constructor(brand: string, model: string) {
this.brand = brand;
this.model = model;
}
getCarDetails(): string {
return `${this.brand} ${this.model}`;
}
}
let myCar = new Car('Toyota', 'Corolla');
console.log(myCar.getCarDetails()); //
输出
"Toyota Corolla"
1.3.2 模块
模块是 TypeScript 中用于组织代码的一种方式。它们可以帮助你避免全局
命名空间的污染,并提供代码的重用性。
// car.ts
export class Car {
brand: string;
model: string;
constructor(brand: string, model: string) {
this.brand = brand;
this.model = model;
}
getCarDetails(): string {
return `${this.brand} ${this.model}`;
}
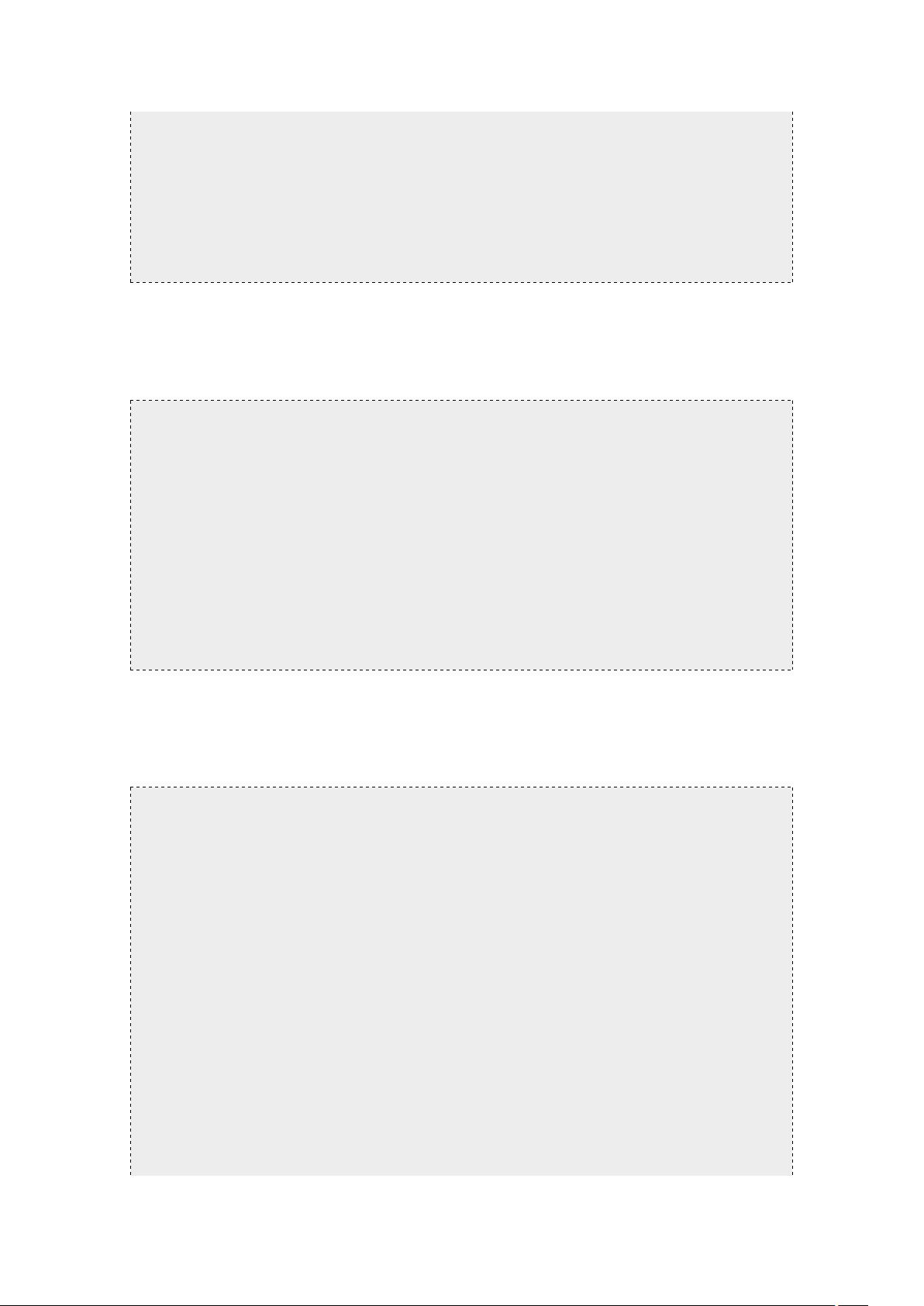
4
}
// main.ts
import { Car } from './car';
let myCar = new Car('Toyota', 'Corolla');
console.log(myCar.getCarDetails()); //
输出
"Toyota Corolla"
1.3.3 模块导入与导出
模块的导入和导出是 TypeScript 中代码组织的关键部分。你可以使用
import 和 export 关键字来导入和导出类、接口、函数等。
// car.ts
export interface Car {
brand: string;
model: string;
}
// main.ts
import { Car } from './car';
let myCar: Car = { brand: 'Toyota', model: 'Corolla' };
console.log(myCar.brand); //
输出
"Toyota"
1.3.4 类的继承
TypeScript 支持类的继承,允许你创建一个类,它继承了另一个类的属性和
方法。
class Vehicle {
brand: string;
constructor(brand: string) {
this.brand = brand;
}
getBrand(): string {
return this.brand;
}
}
class Car extends Vehicle {
model: string;
constructor(brand: string, model: string) {
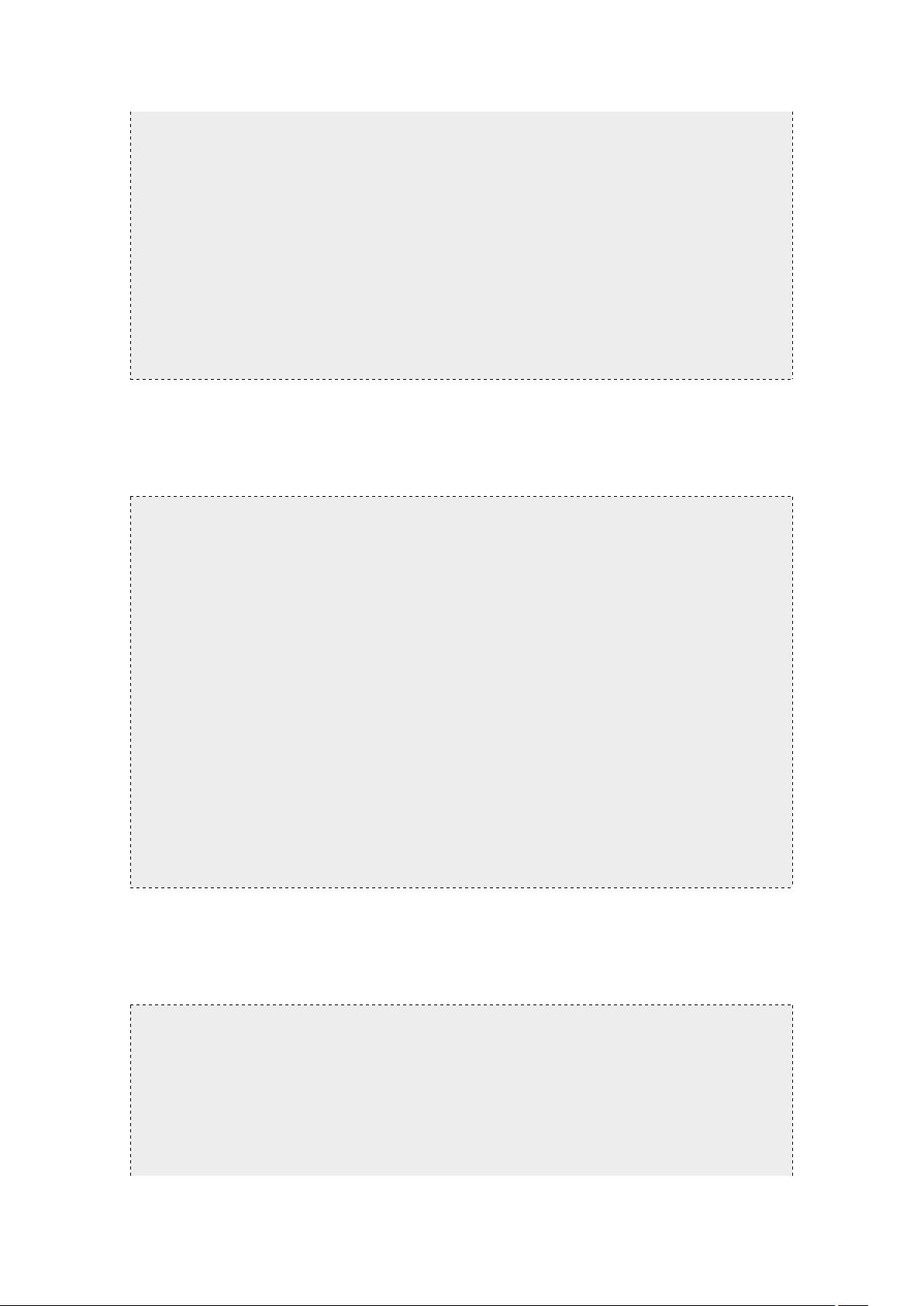
5
super(brand);
this.model = model;
}
getCarDetails(): string {
return `${this.getBrand()} ${this.model}`;
}
}
let myCar = new Car('Toyota', 'Corolla');
console.log(myCar.getCarDetails()); //
输出
"Toyota Corolla"
1.3.5 抽象类
抽象类是不能被实例化的类,它们通常包含一个或多个抽象方法,这些方
法必须在子类中实现。
abstract class Animal {
abstract makeSound(): void;
move(): void {
console.log('roaming the earth...');
}
}
class Dog extends Animal {
makeSound(): void {
console.log('Woof woof!');
}
}
let dog = new Dog();
dog.makeSound(); //
输出
"Woof woof!"
dog.move(); //
输出
"roaming the earth..."
1.3.6 模块的命名空间
命名空间(Namespaces)是 TypeScript 中用于组织大型代码库的一种方式。
它们可以帮助你避免命名冲突,并提供代码的可读性和可维护性。
// car.ts
namespace Car {
export class Toyota {
model: string;
constructor(model: string) {
this.model = model;
剩余24页未读,继续阅读
资源评论
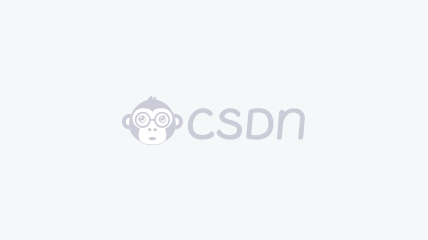


kkchenjj
- 粉丝: 2w+
- 资源: 5479
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

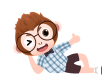
最新资源
- 在不同操作系统下编译Android源码需要更改一些Android源码的配置项,脚本用于自动化更改配置项.zip
- 基于vue3的春节烟花许愿代码.zip学习资料
- YoloV8.2.10的YOLOV8的Segmentation权重文件
- YoloV8.2.10的YOLOV8的Pose权重文件
- 2002 年 Python 周模板 - 4 月 25 日至 29 日 LINUXTips.zip
- 烟花爆炸效果学习代码.zip学习资料开发
- 微信抢红包助手.zip学习资料参考资料程序
- YoloV8.2.10的YOLOV8的Classification权重文件
- 探索Python科学计算:SciPy库的深入指南
- 深入解析栈溢出:原因、影响与解决方案
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


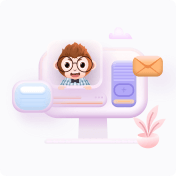
安全验证
文档复制为VIP权益,开通VIP直接复制
