Zoo_java_动物园_zoo类网站_Zoo类_
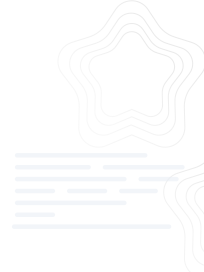

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
在Java编程语言中,设计一个`Zoo`类可以是一个有趣的练习,用于理解和掌握面向对象编程的基本概念。在这个场景中,我们创建一个`Zoo`类来代表一个动物园,而动物园里包含各种不同的动物。这样的设计有助于我们学习如何定义类、对象、继承以及封装等核心概念。 我们需要定义一个`Animal`类作为基础类,它代表所有动物共有的属性和行为。这些属性可能包括动物的名称、年龄和种类。行为可能包括吃、睡和发出声音等。例如: ```java public class Animal { private String name; private int age; private String species; public Animal(String name, int age, String species) { this.name = name; this.age = age; this.species = species; } // getters and setters for the attributes public void eat() { System.out.println("The " + species + " is eating."); } public void sleep() { System.out.println("The " + species + " is sleeping."); } // override toString() method for printing animal details } ``` 接着,我们可以为具体的动物创建子类,如`Lion`、`Elephant`等,它们继承自`Animal`类并添加各自的特有属性和行为。例如,`Lion`类可能有狩猎的能力: ```java public class Lion extends Animal { private boolean canHunt; public Lion(String name, int age, String species, boolean canHunt) { super(name, age, species); this.canHunt = canHunt; } public void hunt() { if (canHunt) { System.out.println("The " + getSpecies() + " is hunting."); } else { System.out.println("The " + getSpecies() + " cannot hunt."); } } } ``` 然后,我们创建`Zoo`类,它将包含一个`Animal`对象的集合,可能是一个`ArrayList`或`LinkedList`。`Zoo`类负责管理动物园中的动物,如添加新动物、展示动物列表、让动物进行特定的行为等。这里是一个简单的`Zoo`类实现: ```java import java.util.ArrayList; import java.util.List; public class Zoo { private List<Animal> animals; public Zoo() { animals = new ArrayList<>(); } public void addAnimal(Animal animal) { animals.add(animal); } public void displayAnimals() { for (Animal animal : animals) { System.out.println(animal); } } public void feedAll() { for (Animal animal : animals) { animal.eat(); } } } ``` 现在,我们可以实例化`Animal`的子类并将其添加到`Zoo`对象中。通过调用`Zoo`对象的方法,我们可以控制动物园中动物的行为: ```java public static void main(String[] args) { Zoo myZoo = new Zoo(); myZoo.addAnimal(new Lion("Simba", 5, "Lion", true)); myZoo.addAnimal(new Elephant("Dumbo", 2, "Elephant", false)); myZoo.displayAnimals(); // displays all animals in the zoo myZoo.feedAll(); // all animals eat myZoo.findByName("Simba").hunt(); // Simba hunts } ``` 在这个示例中,我们展示了如何使用Java进行面向对象编程,创建类和对象,并利用继承和多态性来构建一个复杂的系统。`Zoo`类的设计可以扩展以支持更复杂的功能,例如考虑动物的栖息地、管理员管理、动物之间的交互等。这样的练习有助于提高编程技能,同时加深对面向对象编程核心概念的理解。
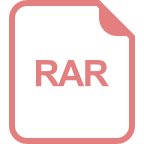
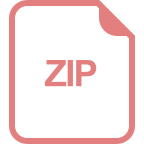
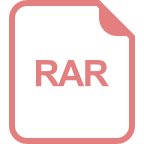
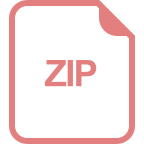
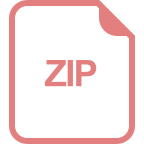
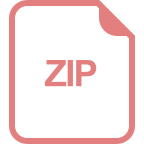
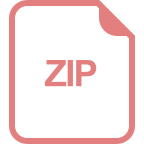
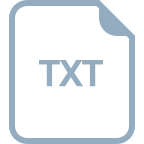
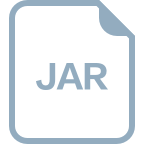
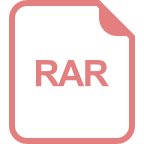
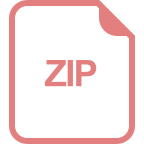
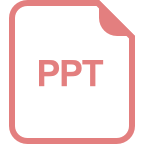
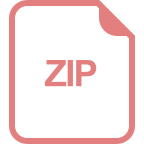
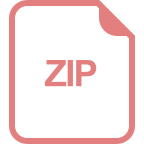
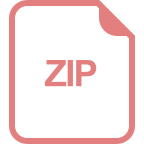
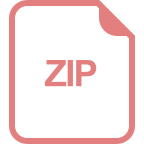
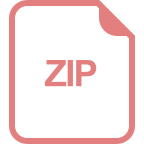



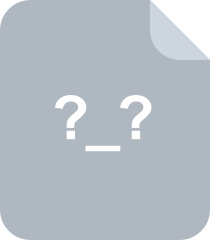
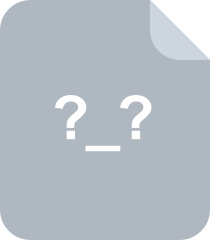
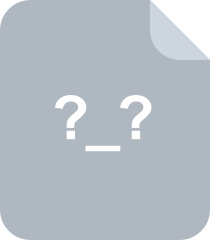
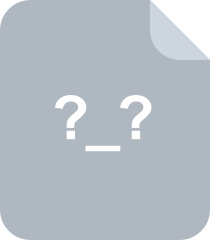
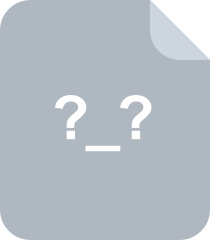
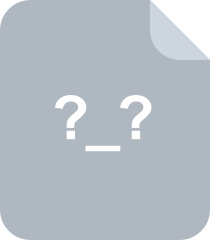
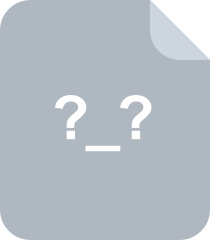
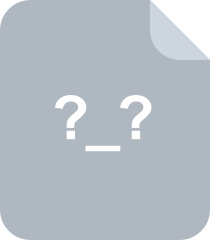
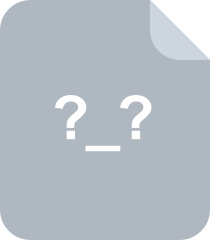
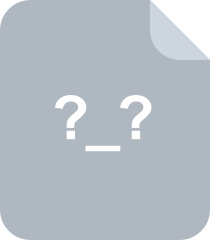
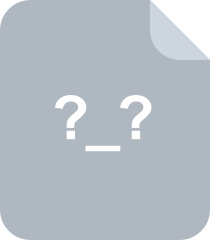
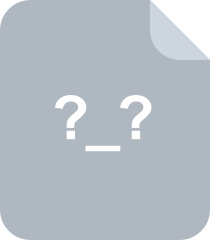
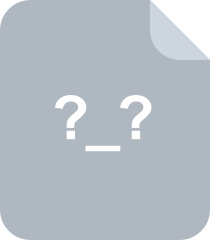
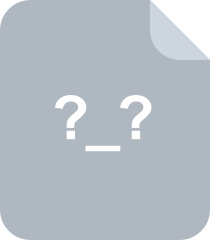
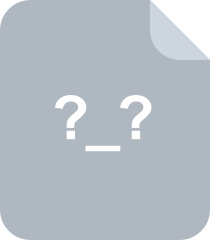
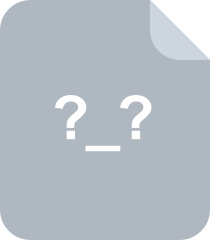

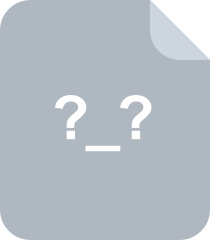
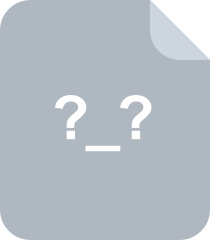
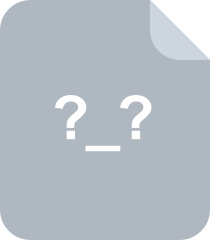
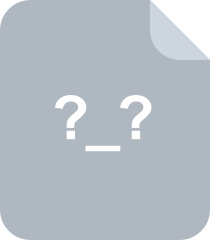
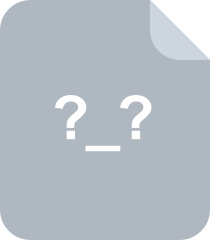



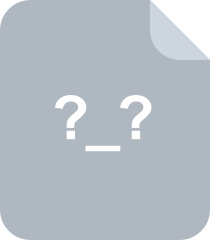
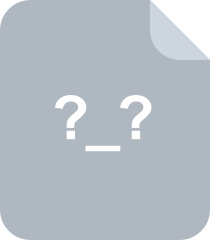
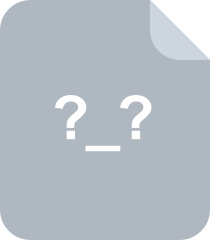
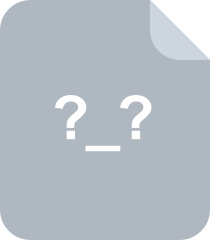
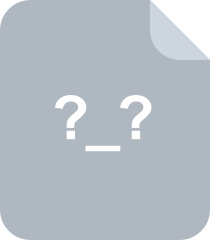
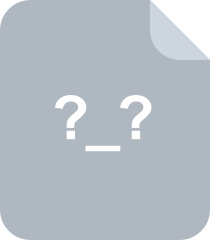
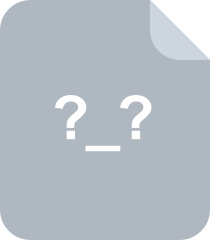
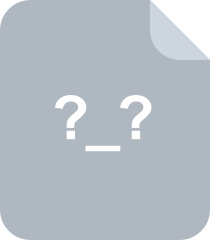
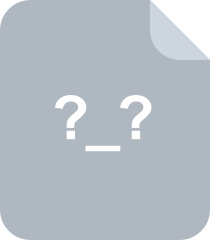
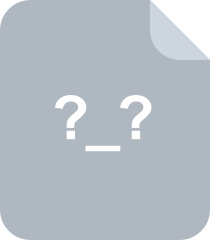

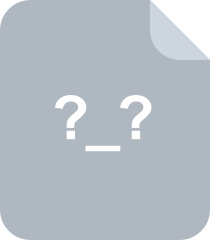
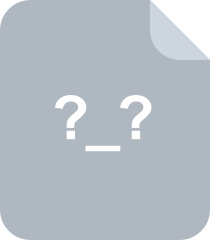
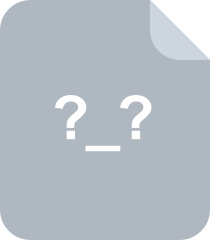
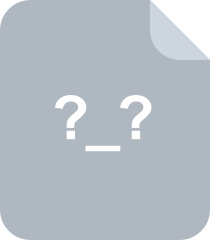
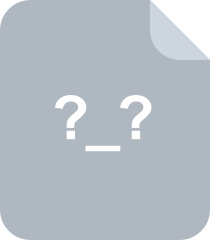
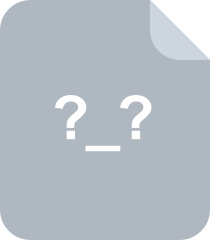
- 1
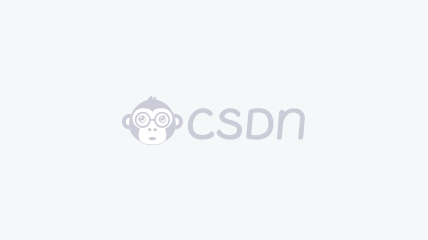

- 粉丝: 66
- 资源: 4738
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

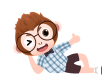
最新资源
- (源码)基于Django和OpenCV的智能车视频处理系统.zip
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip

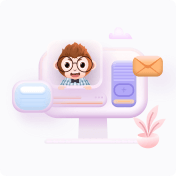
