AHE_equalize图片_数字图像处理_
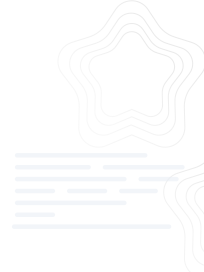

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)

在数字图像处理领域,"AHE_equalize图片_数字图像处理_"这个主题主要涉及的是图像增强技术,特别是自适应直方图均衡化(Adaptive Histogram Equalization,简称AHE)。AHE是一种局部图像处理方法,它通过对图像的不同局部区域进行直方图均衡化来改善图像的对比度和视觉效果。 直方图均衡化是一种广泛使用的图像增强技术,它通过改变像素值的分布来扩大图像的整体动态范围,从而提高图像的对比度。在标准的直方图均衡化中,整个图像被视为一个整体进行处理,可能会导致局部对比度过高或过低的问题。为了解决这个问题,AHE引入了自适应的概念,将图像划分为多个邻域(如8x8的像素块),并对每个邻域单独进行直方图均衡化。 AHE的工作原理如下: 1. **邻域划分**:图像被分割成多个小邻域,每个邻域都有自己的直方图。 2. **直方图计算**:对每个邻域计算其像素值的直方图。 3. **直方图均衡化**:在每个邻域内独立执行直方图均衡化,生成新的像素值映射表。 4. **像素值重映射**:根据生成的映射表,将每个邻域内的像素值更新。 5. **结果拼接**:将所有邻域处理后的图像像素合并成一幅完整的图像。 在实际应用中,AHE特别适用于存在强烈光照不均或阴影的图像,因为它能够增强局部区域的对比度,使得细节更加清晰可见。"two.m"可能是一个MATLAB脚本,用于实现上述过程,而"AHE"可能是一个包含具体算法实现的函数或者类。 AHE虽然能有效提升局部对比度,但也有一些潜在的缺点,比如可能会引入噪声或者边缘模糊。因此,在实际应用中,通常需要根据图像的具体特点和需求来选择是否使用AHE,或者结合其他图像处理技术,如中值滤波等,以达到最佳的增强效果。 AHE是数字图像处理中的一个重要工具,尤其在医学影像分析、遥感图像处理和机器视觉等领域有着广泛应用。通过理解并掌握这种技术,我们可以更好地理解和处理图像数据,从而提取关键信息,提高图像的可读性和分析精度。
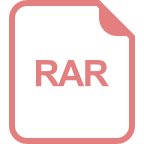
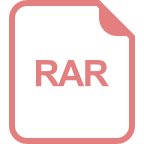
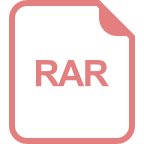
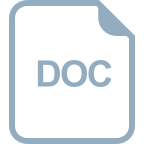
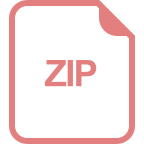
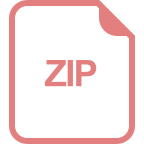
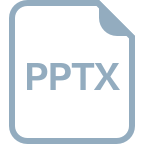
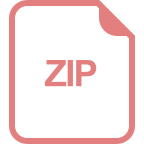
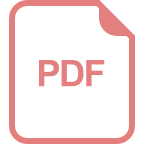
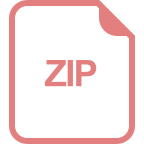


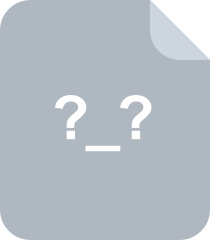
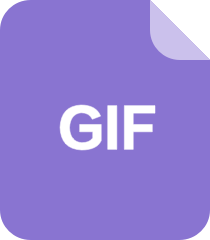
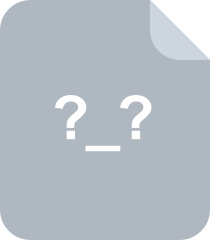
- 1
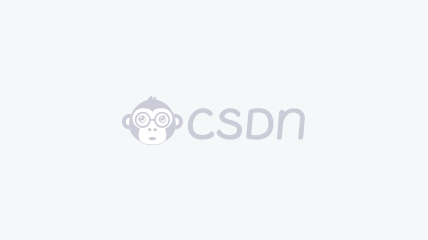
- m0_505521082022-04-17用户下载后在一定时间内未进行评价,系统默认好评。

- 粉丝: 57
- 资源: 4779





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

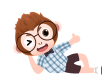
最新资源
- 基于mpc模型预测轨迹跟踪控制,总共包含两套仿真,一套是不加入四轮侧偏角软约束,一套是加入四轮侧偏角的软约束控制,通过carsim与simulink联合仿真发现加入侧偏角软约束在进行轨迹跟踪时,能够通
- 字节跳动人工智能模型DeepSeek:语言理解生成、多模态技术及其广泛应用与未来展望
- 排序算法研究: 快速排序(Quick Sort)原理及其Python实现解析
- java.抽象类与接口(解决方案).md
- 第1章 开始启程-你的第一行Android代码.pdf
- 深度学习中卷积神经网络(CNN)的基本原理及其应用
- 离网型 三相光伏 发电 主电路设计 控制电路设计 以及参数设计 Matlab SIMLINK 仿真 离网 并网 1.主电路设计:光伏boost模块 MPPT 储能双向DC-DC 逆变DC
- FileNotFoundException如何解决.md
- 使用Python正则表达式校验中国大陆手机号格式
- 第2'章 Kotlin语言.pdf
- Java毕业设计基于springboot的物业管理系统源码+数据库(高分项目)
- 第2章 先从看得到的入手,探究活动.pdf
- 第3章 软件也要拼脸蛋,UI开发的点点滴滴.pdf
- 基于javaweb的社区物资交易互助平台.zip
- 文章复现:拉盖尔高斯光束入射石英基底石墨烯涂层的透射光强分布特性研究
- DigitalPlat FreeDomain – Your Free Domain Awaits!

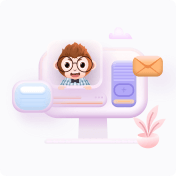
