package start;
import database.DBHelper;
import entity.*;
import factory.CircleFactory;
import factory.LineFactory;
import factory.RectFactory;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import java.util.Random;
import java.util.Stack;
/**
* 描述:创建自己的画图面板类,该类还实现了动作监听,鼠标键听的接口;
* 因此,该类不仅可以看作一个面板,还是一个大的监听器
* 注:不考虑只画一个点的情形(即x1=x2,y1=y2)
*/
public class MyPanel extends JPanel implements ActionListener,MouseListener,MouseMotionListener {
private String[] btnName = {"上一步","画圆形","画矩形","画直线","清空","复制","组合","取消组合","装饰","卸装","保存","加载"};
private int flag = -1; // 当前图形对应的标记位
private boolean color_flag = false; // 只能选中一个图形用
// 移动图形所需
private Pattern checkedPattern = null;
private boolean first = false;
private boolean second = false;
private int startPositionX;
private int startPositionY;
private int endPositionX;
private int endPositionY;
// private boolean start = false;
private Random rand = new Random();
private Pattern curPattern = null; //当前做遍历的图形
private ArrayList<Pattern>patternList = new ArrayList<Pattern>(); // 图形对象容器
private Stack<Command>commandStack = new Stack<Command>(); // 命令模式实现撤销
// 具体工厂
private CircleFactory circleFactory = new CircleFactory();
private RectFactory rectFactory = new RectFactory();
private LineFactory lineFactory = new LineFactory();
// 组合模式用
private boolean canComposite = false;
private boolean existedComposite = false;
// 保存使用
private DBHelper db;
public MyPanel(){
for (int i = 0; i < btnName.length; i++) {
JButton button = new JButton(btnName[i]);
button.addActionListener(this::actionPerformed); // 为每个按钮添加点击事件
this.add(button); // 按钮添加至面板
}
}
// 注:在最开始没有图形时 及 当画板上出现新图形时,在最后系统都会自动调用该方法;
@Override
protected void paintComponent(Graphics g) {
// System.out.println("paintComponent");
super.paintComponent(g); // 清除面板上的图像
for (int i = 0; i < patternList.size(); i++) {
g.setColor(Color.black);
curPattern = patternList.get(i);
curPattern.draw(g);
}
}
@Override
public void actionPerformed(ActionEvent e) {
switch (e.getActionCommand()){
case "上一步": // 命令模式
if(! commandStack.empty()){
// patternList = commandStack.pop().execute(patternList);
Command command = commandStack.pop();
if(command instanceof DecorateCommand){
this.checkedPattern = ((DecorateCommand) command).getDeletedPattern();
}
patternList = command.execute(patternList);
}
this.repaint();
break;
case "画圆形":
curPattern = circleFactory.createPattern(); // 工厂方法
flag = 0;
patternList.add(curPattern);
commandStack.push(new AddCommand(curPattern));
break;
case "画矩形":
curPattern = rectFactory.createPattern();
flag = 1;
patternList.add(curPattern);
commandStack.push(new AddCommand(curPattern));
break;
case "画直线":
curPattern = lineFactory.createPattern();
flag = 3; // 2已被占用
patternList.add(curPattern);
commandStack.push(new AddCommand(curPattern));
break;
case "清空":
checkedPattern = null;
curPattern = null;
try {
commandStack.push(new ClearCommand(patternList));
} catch (CloneNotSupportedException e1) {
e1.printStackTrace();
}
patternList.clear();
repaint();
break;
case "复制":
if(checkedPattern != null){
try {
generateSelf();
} catch (CloneNotSupportedException e1) {
e1.printStackTrace();
}
}
break;
case "组合":
if(canComposite){
CompositePattern compositePattern = new CompositePattern();
CompositeCommand compositeCommand = new CompositeCommand(compositePattern);
for(int i=0;i<patternList.size();){
curPattern = patternList.get(i);
if(curPattern.isSelected()){
// System.out.println(i);
compositePattern.add(curPattern);
compositeCommand.addPattern(curPattern);
patternList.remove(curPattern);
}else{
i++;
}
}
patternList.add(compositePattern);
commandStack.push(compositeCommand);
// System.out.println("patternList:"+patternList.size());
// System.out.println(compositePattern.getSize());
compositePattern.setSelected(false);
this.repaint();
}
break;
case "取消组合":
// 当前存在选定的对象
if(checkedPattern != null){
// 当前选定的为组合对象,非叶子节点
if(checkedPattern instanceof CompositePattern){
CompositePattern cur = (CompositePattern)checkedPattern;
for(int i=0; i < cur.getSize(); i++){
patternList.add(cur.getChild(i));
}
patternList.remove(checkedPattern);
}else if(checkedPattern instanceof BorderDecorator){ // 选中的是被装饰的对象
// 保证该被装饰的对象为组合对象
if(((BorderDecorator) checkedPattern).getCompositeRoot() != null){
CompositePattern cur = (CompositePattern)((BorderDecorator) checkedPattern).getCompositeRoot();
for(int i=0; i < cur.getSize(); i++){
patternList.add(new BorderDecorator(cur.getChild(i)));
}
patternList.remove(checkedPattern);
}
}
}
break;
case "装饰":
// System.out.println(checkedPattern);
if(checkedPattern != null){
Pattern decoratorPattern = new BorderDecorator(checkedPattern);
commandStack.push(new DecorateCommand(checkedPattern,decoratorPattern));
patternList.remove(checkedPattern);
patternList.add(decoratorPattern);
checkedPattern = decoratorPattern;
// System.out.println(123);
}
this.repaint();
break;
case "卸装":
// System.out.println(checkedPattern);
if(checkedPattern != null){
// System.out.println(123);
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
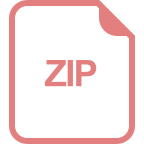
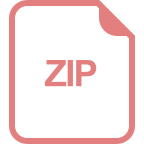
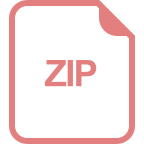
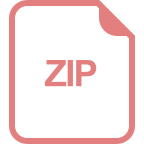
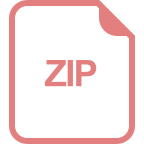
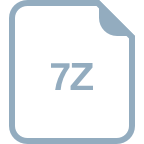
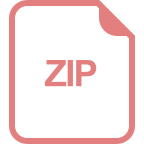
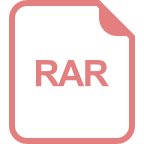
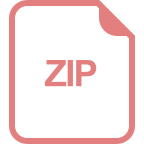
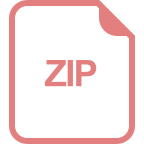
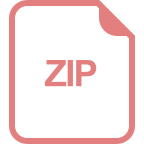
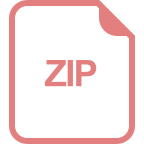
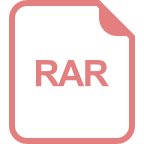
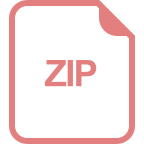
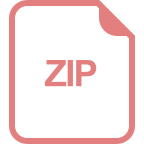
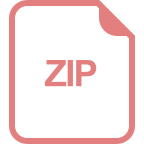
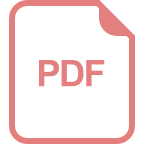
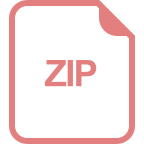
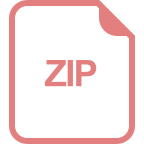
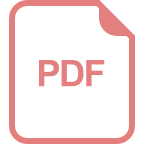
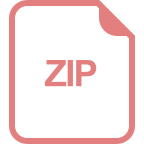
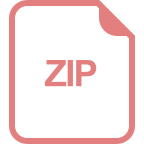
收起资源包目录




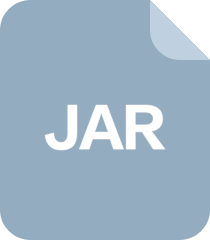


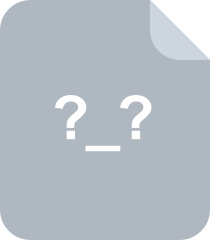

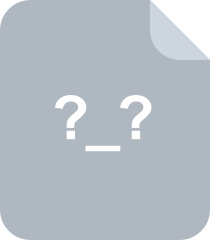
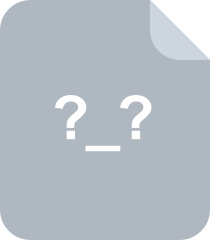
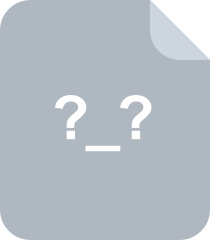
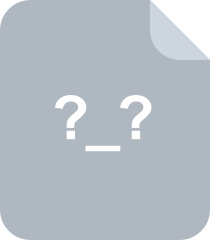

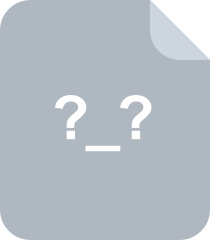

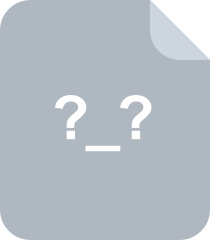
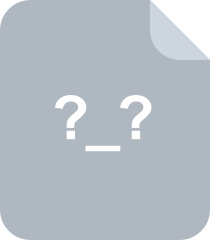

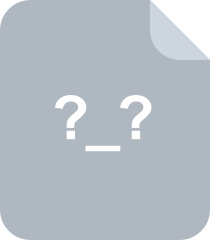
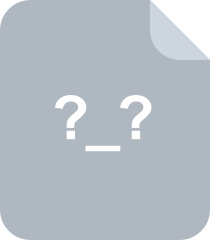
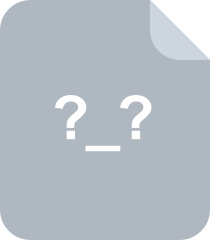
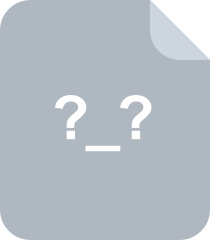
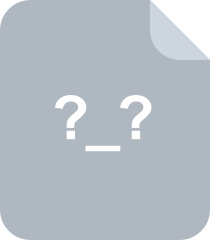
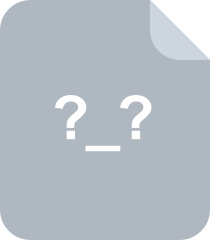
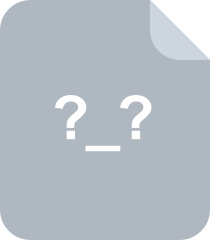
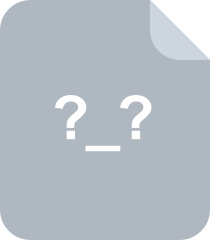
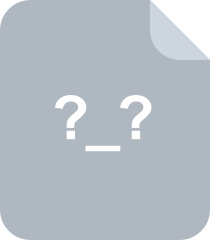
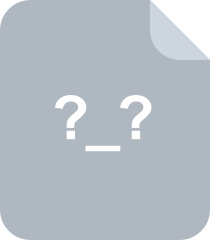
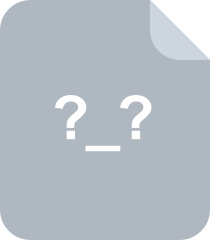
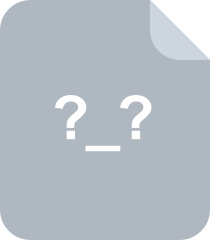
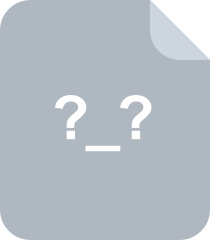




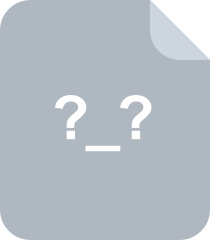

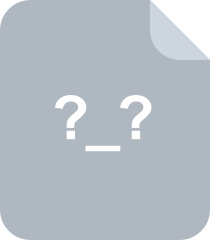
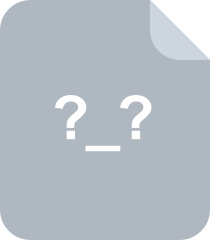
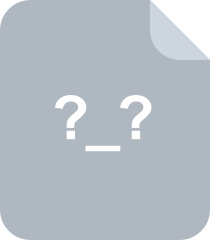
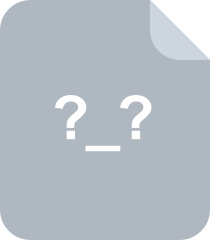

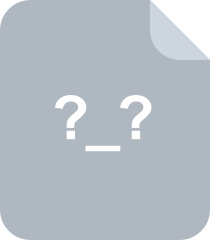

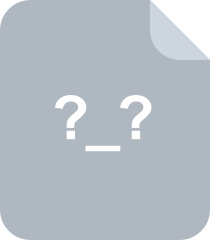
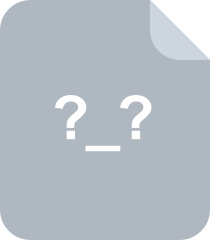

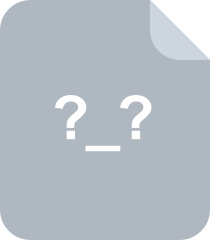
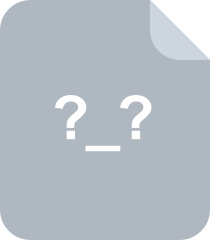
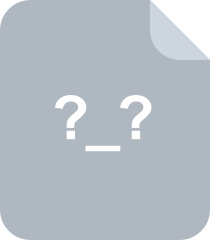
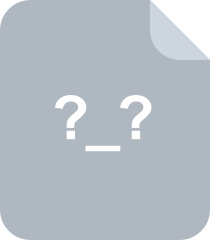
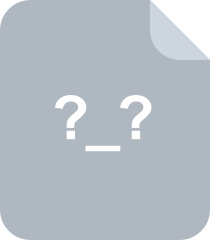
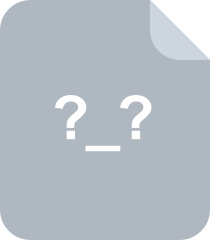
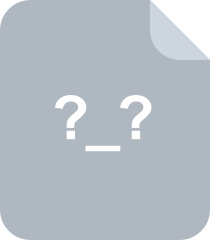
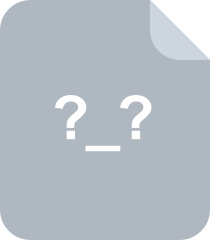
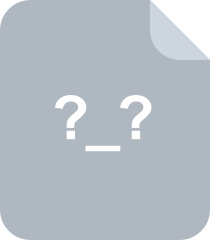
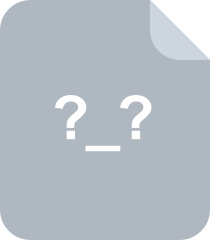
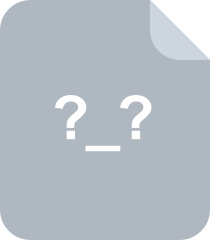
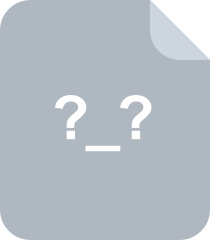

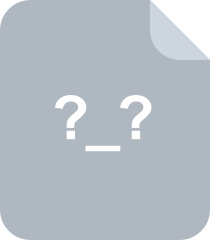
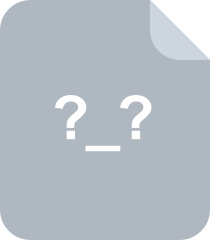
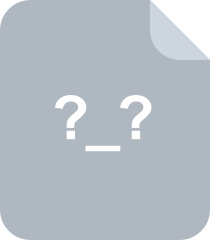
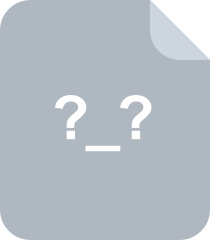
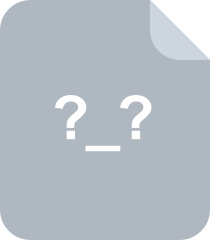
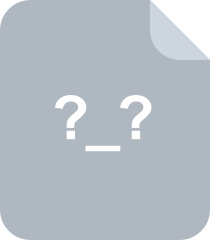
共 48 条
- 1
资源评论
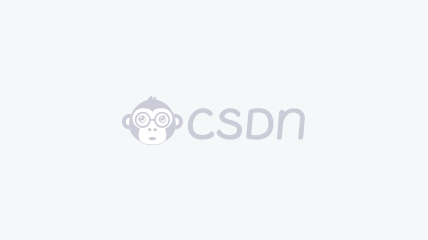

哆啦哆啦S梦
- 粉丝: 156
- 资源: 517
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

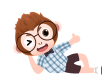
安全验证
文档复制为VIP权益,开通VIP直接复制
