/*
* asm/tbx.h
*
* Copyright (C) 2000-2012 Imagination Technologies.
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License version 2 as published by the
* Free Software Foundation.
*
* Thread binary interface header
*/
#ifndef _ASM_METAG_TBX_H_
#define _ASM_METAG_TBX_H_
/* for CACHEW_* values */
#include <asm/metag_isa.h>
/* for LINSYSEVENT_* addresses */
#include <asm/metag_mem.h>
#ifdef TBI_1_4
#ifndef TBI_MUTEXES_1_4
#define TBI_MUTEXES_1_4
#endif
#ifndef TBI_SEMAPHORES_1_4
#define TBI_SEMAPHORES_1_4
#endif
#ifndef TBI_ASYNC_SWITCH_1_4
#define TBI_ASYNC_SWITCH_1_4
#endif
#ifndef TBI_FASTINT_1_4
#define TBI_FASTINT_1_4
#endif
#endif
/* Id values in the TBI system describe a segment using an arbitrary
integer value and flags in the bottom 8 bits, the SIGPOLL value is
used in cases where control over blocking or polling behaviour is
needed. */
#define TBID_SIGPOLL_BIT 0x02 /* Set bit in an Id value to poll vs block */
/* Extended segment identifiers use strings in the string table */
#define TBID_IS_SEGSTR( Id ) (((Id) & (TBID_SEGTYPE_BITS>>1)) == 0)
/* Segment identifiers contain the following related bit-fields */
#define TBID_SEGTYPE_BITS 0x0F /* One of the predefined segment types */
#define TBID_SEGTYPE_S 0
#define TBID_SEGSCOPE_BITS 0x30 /* Indicates the scope of the segment */
#define TBID_SEGSCOPE_S 4
#define TBID_SEGGADDR_BITS 0xC0 /* Indicates access possible via pGAddr */
#define TBID_SEGGADDR_S 6
/* Segments of memory can only really contain a few types of data */
#define TBID_SEGTYPE_TEXT 0x02 /* Code segment */
#define TBID_SEGTYPE_DATA 0x04 /* Data segment */
#define TBID_SEGTYPE_STACK 0x06 /* Stack segment */
#define TBID_SEGTYPE_HEAP 0x0A /* Heap segment */
#define TBID_SEGTYPE_ROOT 0x0C /* Root block segments */
#define TBID_SEGTYPE_STRING 0x0E /* String table segment */
/* Segments have one of three possible scopes */
#define TBID_SEGSCOPE_INIT 0 /* Temporary area for initialisation phase */
#define TBID_SEGSCOPE_LOCAL 1 /* Private to this thread */
#define TBID_SEGSCOPE_GLOBAL 2 /* Shared globally throughout the system */
#define TBID_SEGSCOPE_SHARED 3 /* Limited sharing between local/global */
/* For segment specifier a further field in two of the remaining bits
indicates the usefulness of the pGAddr field in the segment descriptor
descriptor. */
#define TBID_SEGGADDR_NULL 0 /* pGAddr is NULL -> SEGSCOPE_(LOCAL|INIT) */
#define TBID_SEGGADDR_READ 1 /* Only read via pGAddr */
#define TBID_SEGGADDR_WRITE 2 /* Full access via pGAddr */
#define TBID_SEGGADDR_EXEC 3 /* Only execute via pGAddr */
/* The following values are common to both segment and signal Id value and
live in the top 8 bits of the Id values. */
/* The ISTAT bit indicates if segments are related to interrupt vs
background level interfaces a thread can still handle all triggers at
either level, but can also split these up if it wants to. */
#define TBID_ISTAT_BIT 0x01000000
#define TBID_ISTAT_S 24
/* Privilege needed to access a segment is indicated by the next bit.
This bit is set to mirror the current privilege level when starting a
search for a segment - setting it yourself toggles the automatically
generated state which is only useful to emulate unprivileged behaviour
or access unprivileged areas of memory while at privileged level. */
#define TBID_PSTAT_BIT 0x02000000
#define TBID_PSTAT_S 25
/* The top six bits of a signal/segment specifier identifies a thread within
the system. This represents a segments owner. */
#define TBID_THREAD_BITS 0xFC000000
#define TBID_THREAD_S 26
/* Special thread id values */
#define TBID_THREAD_NULL (-32) /* Never matches any thread/segment id used */
#define TBID_THREAD_GLOBAL (-31) /* Things global to all threads */
#define TBID_THREAD_HOST ( -1) /* Host interface */
#define TBID_THREAD_EXTIO (TBID_THREAD_HOST) /* Host based ExtIO i/f */
/* Virtual Id's are used for external thread interface structures or the
above special Id's */
#define TBID_IS_VIRTTHREAD( Id ) ((Id) < 0)
/* Real Id's are used for actual hardware threads that are local */
#define TBID_IS_REALTHREAD( Id ) ((Id) >= 0)
/* Generate a segment Id given Thread, Scope, and Type */
#define TBID_SEG( Thread, Scope, Type ) (\
((Thread)<<TBID_THREAD_S) + ((Scope)<<TBID_SEGSCOPE_S) + (Type))
/* Generate a signal Id given Thread and SigNum */
#define TBID_SIG( Thread, SigNum ) (\
((Thread)<<TBID_THREAD_S) + ((SigNum)<<TBID_SIGNUM_S) + TBID_SIGNAL_BIT)
/* Generate an Id that solely represents a thread - useful for cache ops */
#define TBID_THD( Thread ) ((Thread)<<TBID_THREAD_S)
#define TBID_THD_NULL ((TBID_THREAD_NULL) <<TBID_THREAD_S)
#define TBID_THD_GLOBAL ((TBID_THREAD_GLOBAL)<<TBID_THREAD_S)
/* Common exception handler (see TBID_SIGNUM_XXF below) receives hardware
generated fault codes TBIXXF_SIGNUM_xxF in it's SigNum parameter */
#define TBIXXF_SIGNUM_IIF 0x01 /* General instruction fault */
#define TBIXXF_SIGNUM_PGF 0x02 /* Privilege general fault */
#define TBIXXF_SIGNUM_DHF 0x03 /* Data access watchpoint HIT */
#define TBIXXF_SIGNUM_IGF 0x05 /* Code fetch general read failure */
#define TBIXXF_SIGNUM_DGF 0x07 /* Data access general read/write fault */
#define TBIXXF_SIGNUM_IPF 0x09 /* Code fetch page fault */
#define TBIXXF_SIGNUM_DPF 0x0B /* Data access page fault */
#define TBIXXF_SIGNUM_IHF 0x0D /* Instruction breakpoint HIT */
#define TBIXXF_SIGNUM_DWF 0x0F /* Data access read-only fault */
/* Hardware signals communicate events between processing levels within a
single thread all the _xxF cases are exceptions and are routed via a
common exception handler, _SWx are software trap events and kicks including
__TBISignal generated kicks, and finally _TRx are hardware triggers */
#define TBID_SIGNUM_SW0 0x00 /* SWITCH GROUP 0 - Per thread user */
#define TBID_SIGNUM_SW1 0x01 /* SWITCH GROUP 1 - Per thread system */
#define TBID_SIGNUM_SW2 0x02 /* SWITCH GROUP 2 - Internal global request */
#define TBID_SIGNUM_SW3 0x03 /* SWITCH GROUP 3 - External global request */
#ifdef TBI_1_4
#define TBID_SIGNUM_FPE 0x04 /* Deferred exception - Any IEEE 754 exception */
#define TBID_SIGNUM_FPD 0x05 /* Deferred exception - Denormal exception */
/* Reserved 0x6 for a reserved deferred exception */
#define TBID_SIGNUM_BUS 0x07 /* Deferred exception - Bus Error */
/* Reserved 0x08-0x09 */
#else
/* Reserved 0x04-0x09 */
#endif
/* Reserved 0x0A-0x0F */
#define TBID_SIGNUM_TRT 0x10 /* Timer trigger */
#define TBID_SIGNUM_LWK 0x11 /* Low level kick */
#define TBID_SIGNUM_XXF 0x12 /* Fault handler - receives ALL _xxF sigs */
#ifdef TBI_1_4
#define TBID_SIGNUM_DFR 0x13 /* Deferred Exception handler */
#else
#define TBID_SIGNUM_FPE 0x13 /* FPE Exception handler */
#endif
/* External trigger one group 0x14 to 0x17 - per thread */
#define TBID_SIGNUM_TR1(Thread) (0x14+(Thread))
#define TBID_SIGNUM_T10 0x14
#define TBID_SIGNUM_T11 0x15
#define TBID_SIGNUM_T12 0x16
#define TBID_SIGNUM_T13 0x17
/* External trigger two group 0x18 to 0x1b - per thread */
#define TBID_SIGNUM_TR2(Thread) (0x18+(Thread))
#define TBID_SIGNUM_T20 0x18
#define TBID_SIGNUM_T21 0x19
#define TBID_SIGNUM_T22 0x1A
#define TBID_SIGNUM_T23 0x1B
#define TBID_SIGNUM_TR3 0x1C /* External trigger N-4 (global) */
#define TBID_SIGNUM_TR4 0x1D /* External trigger N-3 (global) */
#define TBID_SIGNUM_TR5 0x1E /* External trigger N-2 (global) */
#define TBID_SIGNUM_TR6 0x1F /* External trigger N-1 (global) */
#define TBID_SIGNUM_MAX 0x1F
/* Return the trigger register(TXMASK[I]/TXSTAT[I]) bits related to
each hardware signal, sometimes this is a many-to-one relationship. */
#define TBI_TRIG_B

寒泊
- 粉丝: 86
- 资源: 1万+
最新资源
- java毕设项目之ssm安徽新华学院实验中心管理系统的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm毕业lw管理系统+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm毕业生就业信息统计系统+vue(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm大学生兼职平台的设计与开发+jsp(完整前后端+说明文档+mysql).zip
- java毕设项目之ssm博客系统的设计与实现+vue(完整前后端+说明文档+mysql).zip
- java毕设项目之ssm单位人事管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm电子竞技管理平台的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm房屋租售网站的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm高校专业信息管理系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm会员管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于 Java Web 的校园驿站管理系统+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于JavaEE的龙腾公司员工信息管理系统的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于Java的菜匣子优选系统设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- 大题解题方法等4个文件.zip
- java毕设项目之ssm基于JavaWeb的家居商城系统的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
- java毕设项目之ssm基于Java的汽车客运站管理系统的设计与实现+jsp(完整前后端+说明文档+mysql+lw).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


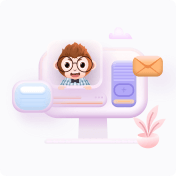