#define GLUT_DISABLE_ATEXIT_HACK
#include <iostream>
#include <gl/glew.h>
//#define GLUT_DISABLE_ATEXIT_HACK
#include <gl/GLUT.H>
#pragma comment(lib, "glew32.lib")
using namespace std;
// cube ///////////////////////////////////////////////////////////////////////
// v6----- v5
// /| /|
// v1------v0|
// | | | |
// | |v7---|-|v4
// |/ |/
// v2------v3
// vertex coords array for glDrawArrays() =====================================
// A cube has 6 sides and each side has 2 triangles, therefore, a cube consists
// of 36 vertices (6 sides * 2 tris * 3 vertices = 36 vertices). And, each
// vertex is 3 components (x,y,z) of floats, therefore, the size of vertex
// array is 108 floats (36 * 3 = 108).
GLfloat vertices[] = {
1, 1, 1, -1, 1, 1, -1,-1, 1, // v0-v1-v2 (front)
-1,-1, 1, 1,-1, 1, 1, 1, 1, // v2-v3-v0
1, 1, 1, 1,-1, 1, 1,-1,-1, // v0-v3-v4 (right)
1,-1,-1, 1, 1,-1, 1, 1, 1, // v4-v5-v0
1, 1, 1, 1, 1,-1, -1, 1,-1, // v0-v5-v6 (top)
-1, 1,-1, -1, 1, 1, 1, 1, 1, // v6-v1-v0
-1, 1, 1, -1, 1,-1, -1,-1,-1, // v1-v6-v7 (left)
-1,-1,-1, -1,-1, 1, -1, 1, 1, // v7-v2-v1
-1,-1,-1, 1,-1,-1, 1,-1, 1, // v7-v4-v3 (bottom)
1,-1, 1, -1,-1, 1, -1,-1,-1, // v3-v2-v7
1,-1,-1, -1,-1,-1, -1, 1,-1, // v4-v7-v6 (back)
-1, 1,-1, 1, 1,-1, 1,-1,-1 }; // v6-v5-v4
// normal array
GLfloat normals[] = {
0, 0, 1, 0, 0, 1, 0, 0, 1, // v0-v1-v2 (front)
0, 0, 1, 0, 0, 1, 0, 0, 1, // v2-v3-v0
1, 0, 0, 1, 0, 0, 1, 0, 0, // v0-v3-v4 (right)
1, 0, 0, 1, 0, 0, 1, 0, 0, // v4-v5-v0
0, 1, 0, 0, 1, 0, 0, 1, 0, // v0-v5-v6 (top)
0, 1, 0, 0, 1, 0, 0, 1, 0, // v6-v1-v0
-1, 0, 0, -1, 0, 0, -1, 0, 0, // v1-v6-v7 (left)
-1, 0, 0, -1, 0, 0, -1, 0, 0, // v7-v2-v1
0,-1, 0, 0,-1, 0, 0,-1, 0, // v7-v4-v3 (bottom)
0,-1, 0, 0,-1, 0, 0,-1, 0, // v3-v2-v7
0, 0,-1, 0, 0,-1, 0, 0,-1, // v4-v7-v6 (back)
0, 0,-1, 0, 0,-1, 0, 0,-1 }; // v6-v5-v4
// color array
GLfloat colors[] = {
1, 1, 1, 1, 1, 0, 1, 0, 0, // v0-v1-v2 (front)
1, 0, 0, 1, 0, 1, 1, 1, 1, // v2-v3-v0
1, 1, 1, 1, 0, 1, 0, 0, 1, // v0-v3-v4 (right)
0, 0, 1, 0, 1, 1, 1, 1, 1, // v4-v5-v0
1, 1, 1, 0, 1, 1, 0, 1, 0, // v0-v5-v6 (top)
0, 1, 0, 1, 1, 0, 1, 1, 1, // v6-v1-v0
1, 1, 0, 0, 1, 0, 0, 0, 0, // v1-v6-v7 (left)
0, 0, 0, 1, 0, 0, 1, 1, 0, // v7-v2-v1
0, 0, 0, 0, 0, 1, 1, 0, 1, // v7-v4-v3 (bottom)
1, 0, 1, 1, 0, 0, 0, 0, 0, // v3-v2-v7
0, 0, 1, 0, 0, 0, 0, 1, 0, // v4-v7-v6 (back)
0, 1, 0, 0, 1, 1, 0, 0, 1 }; // v6-v5-v4
GLuint vboId = 0;
//camera
float cameraAngleX;
float cameraAngleY;
float cameraDistance = -5.0;
//mouse
bool mouseLeftDown;
bool mouseRightDown;
float LastXPos;
float LastYPos;
void initGL()
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glShadeModel(GL_SMOOTH);
glGenBuffers(1, &vboId);
glBindBuffer(GL_ARRAY_BUFFER, vboId);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices)+sizeof(normals)+sizeof(colors), 0, GL_STATIC_DRAW);
glBufferSubData(GL_ARRAY_BUFFER, 0, sizeof(vertices), vertices); //第二个数据是偏移
glBufferSubData(GL_ARRAY_BUFFER, sizeof(vertices), sizeof(normals), normals);
glBufferSubData(GL_ARRAY_BUFFER, sizeof(vertices)+sizeof(normals), sizeof(colors), colors);
}
void display()
{
glClear(GL_COLOR_BUFFER_BIT);
glPushMatrix();
glLoadIdentity();
glTranslatef(0, 0, cameraDistance);
glRotatef(cameraAngleX, 1, 0, 0);
glRotatef(cameraAngleY, 0, 1, 0);
glBindBuffer(GL_ARRAY_BUFFER, vboId);
glEnableClientState(GL_NORMAL_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
glEnableClientState(GL_VERTEX_ARRAY);
glNormalPointer(GL_FLOAT, 0, (void*)sizeof(vertices)); //最后一个值为偏移
glColorPointer(3, GL_FLOAT, 0, (void*)(sizeof(vertices)+sizeof(normals)));
glVertexPointer(3, GL_FLOAT, 0, 0);
glDrawArrays(GL_TRIANGLES, 0, 36);//从0开始
glDisableClientState(GL_VERTEX_ARRAY);
glDisableClientState(GL_COLOR_ARRAY);
glDisableClientState(GL_NORMAL_ARRAY);
glBindBuffer(GL_ARRAY_BUFFER, 0);
glPopMatrix();
glutSwapBuffers();
}
void reshape(int w, int h)
{
glViewport(0, 0, w, h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(60.0f, (GLfloat)w/(GLfloat)h, 0.01f, 100.0f);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
void mouse(int button, int state, int x, int y)
{
if (button == GLUT_LEFT_BUTTON && state == GLUT_DOWN)
{
mouseLeftDown = true;
LastXPos = x;
LastYPos = y;
}
/*if (button == GLUT_RIGHT_BUTTON && state == GLUT_UP)
{
mouseRightDown = true;
}*/
}
void mouseMotion(int x, int y)
{
if (mouseLeftDown)
{
cameraAngleX += GLfloat(y - LastYPos)/GLfloat(20.0);
cameraAngleY += GLfloat(x - LastXPos)/GLfloat(20.0);
LastYPos = y;
LastXPos = x;
}
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGBA);
glutInitWindowSize(480, 640);
glutInitWindowPosition(100, 100);
glutCreateWindow("VBO");
glewInit();
if(!glewIsSupported("GL_VERSION_2_0"))
{
fprintf(stderr, "ERROR: Support for necessary OpengGL extensions missing.");
}
initGL();
glutDisplayFunc(display);
glutIdleFunc(display);
glutReshapeFunc(reshape);
glutMouseFunc(mouse);
glutMotionFunc(mouseMotion);
glutMainLoop();
glDeleteBuffers(GL_ARRAY_BUFFER, &vboId);
return 0;
}

寒泊
- 粉丝: 86
- 资源: 1万+
最新资源
- 电子工程中差动放大电路的性能测试与分析
- 8PSK调制解调通信链路matlab误码率仿真【包括程序,中文注释,程序操作和讲解视频】
- BLDC无刷直流电机电流滞环控制 1.转速环采用pi控制,电流环采用滞环控制 2.提供参考文献和仿真模型;
- 电子工程技术中的电压比较器实验及特性研究
- 4-20mA采集电路,主控为STM32F103,RS485输出 提供原理图和pcb源文件(AD设计),以及源码,包含ADC采样代码,RS485代码等,带隔离功能 备注:精通各种运放的使用,支持其他
- 模拟电子技术中负反馈放大电路的实验研究及其性能优化方法
- 电子工程技术-集成运算放大器的基本运算电路实验研究与仿真实践
- 风光柴储微网优化调度模型(matlb程序),粒子群多目标优化. 程序注释清晰明了,适合研究微网优化调度,微网容量配置方向基础入门的同学
- STM32CubeMX图形化配置与代码生成功能在嵌入式开发的应用
- ouc2024攻防先导作业
- 模拟电子技术-单管共射放大电路实验报告-掌握静态工作点与放大性能的测量方法
- 基于51单片机的直流电机调速仿真 通过调节滑动变阻器控制电机转动速度 没有速度值显示 包括源程序,仿真,proteus软件包 送相关文档资料(不是对应配套的,仅供参考,自行整合取舍使用)
- 模拟电子技术-射极跟随器:特性、原理及其实验方法详解
- ouc2024秋攻防先导作业
- 嵌入式开发中STM32CubeMX图形化配置与代码生成功能详解及其应用
- simulink模块汇总梳理 , 智能座舱域在AUTOSAR 框架中应用层的开发依赖于simulink建模,通过simulink模型设计加上C代码生成来完成繁杂的应用层开发 因此simulink计
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


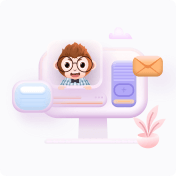