udp.zip_UDP throughput _UDP 延迟_lantency test下载_throughput_udp 延时
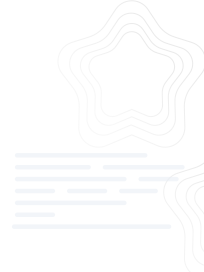

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)

UDP(User Datagram Protocol)是一种无连接的传输层协议,它在Internet协议族中扮演着重要的角色。本项目主要关注UDP的两个关键性能指标:吞吐量(throughput)和延迟(latency)。通过UDPclient.c和UDPserver.c这两个源代码文件,我们可以构建一个简单的UDP通信系统,用于测量数据在两台机器之间传输的效率和速度。 吞吐量(throughput)是指在一定时间内网络能够传输的数据量,通常以比特每秒(bps)或字节每秒(Bps)来衡量。在UDP中,吞吐量测试旨在了解网络的最大数据传输速率。通过发送大量数据包并记录接收端成功接收到的数据量,我们可以计算出实际的吞吐量。这个过程可能涉及到调整数据包的大小、发送速率等因素,以找到网络的最佳性能点。 延迟(latency)是指数据包从发送到接收所需的时间,也称为响应时间。在UDP中,由于其无连接特性,数据包可能会丢失,所以延迟测试不仅关注单个包的传输时间,还需要考虑重传和丢包率对整体延迟的影响。Report part 1.pdf.jpg和Report part 2.jpg.jpg可能包含了关于实验设置、数据收集和分析的详细报告,其中可能包括了如何测量和计算延迟的方法。 Readme file for UDP measure.rtf可能是一个说明文档,提供了如何运行客户端和服务器程序、如何解读结果以及如何分析性能的指导。这通常会包括配置参数的解释,例如端口号、数据包大小、发送速率等,这些都可能影响到吞吐量和延迟的测量结果。 Report part 3.jpg可能是实验结果的可视化展示,如图表或曲线图,展示了吞吐量随时间的变化或延迟分布情况。这些图形有助于直观地理解网络性能,并且可以用来比较不同网络条件下的表现。 这个项目通过编写UDP客户端和服务器程序,执行了一系列的性能测试,以评估UDP在特定环境下的吞吐量和延迟。通过对这些文件进行深入分析,我们可以学习如何设计和实施类似的网络性能测试,以及如何解析和解释测试结果,这对于网络优化和问题诊断具有重要的实践价值。
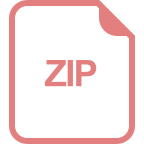
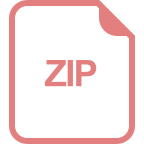
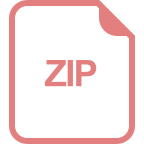
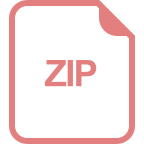
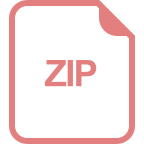
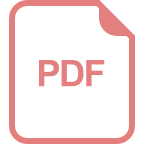
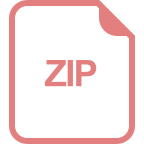
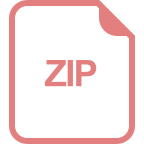
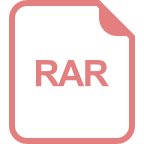
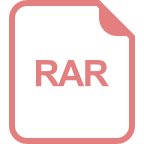
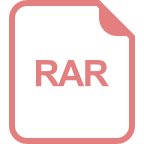
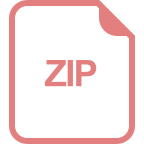
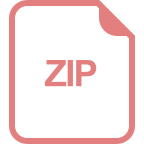
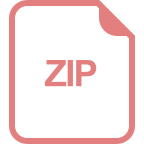

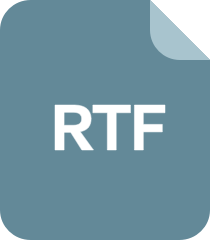
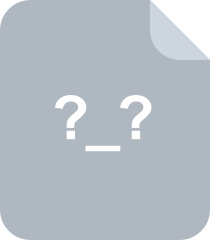
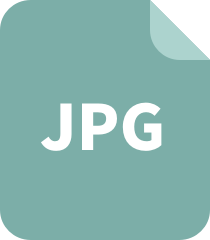
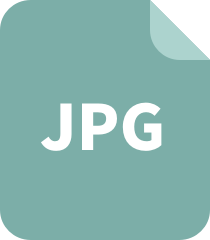
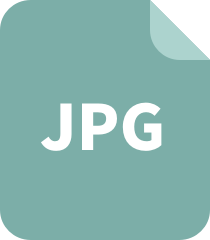
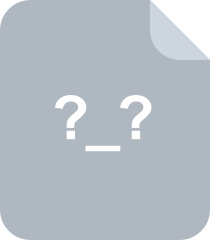
- 1
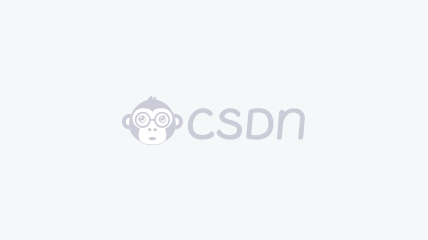
- qq_149368252023-09-17发现一个超赞的资源,赶紧学习起来,大家一起进步,支持!

- 粉丝: 86
- 资源: 1万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

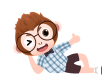
最新资源
- 【岗位说明】供排水制度汇编.docx
- 【岗位说明】自来水公司库房管理岗位职责及工作标准.doc
- Comsol 模拟 仿真 模型 热-流-固四场耦合增透瓦斯抽采,包括动态渗透率、孔隙率变化模型,涉及pde模块等四个物理场
- 【岗位说明】污水处理厂岗位职责和制度02.doc
- 【岗位说明】污水处理厂岗位职责.docx
- 【岗位说明】污水处理厂运行管理部部门职责.doc
- 【岗位说明】污水处理厂岗位职责.doc
- 【岗位说明】污水处理岗位职责及奖惩制度.doc
- 机械设计CNC桌面数控点胶机sw17可编辑全套技术资料100%好用.zip
- 【岗位说明】工会干事工作职责.doc
- 【岗位说明】总公司财务部岗位职责.doc
- 【岗位说明】电话销售经理岗位职责精选6篇.docx
- 【岗位说明】钣金岗位职责20篇.docx
- 【岗位说明】炊事员岗位职责6篇.docx
- 【岗位说明】电话销售岗位职责25篇.docx
- 【岗位说明】生产制造企业采购部的职责.doc

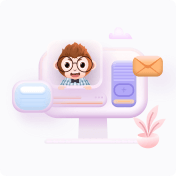
