adc.rar_EduKit_adc linux
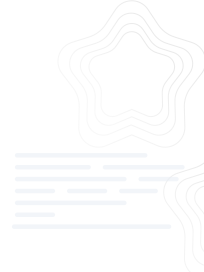

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
在嵌入式Linux系统中,ADC(Analog-to-Digital Converter)驱动是连接硬件与软件的重要桥梁,它允许系统读取模拟信号并将其转化为数字值,以便处理器进行处理。Sumsung S3C2410是一款广泛使用的ARM9微处理器,内置了ADC模块,适用于各种嵌入式应用,如教育套件Embest EduKit III。 标题中的"adc.rar_EduKit_adc linux"表明这是一个关于S3C2410 ADC驱动的资源包,特别针对Embest EduKit III教育平台。这个压缩包可能包含了驱动源代码、编译脚本、配置文件以及相关的教程或说明文档,帮助开发者理解和实现S3C2410上的ADC功能。 描述中的"嵌入式linux Sumsung S3C2410 ADC驱动 embest edukit iii"进一步强调了这个驱动程序是为运行Linux操作系统的嵌入式设备设计的,特别是Embest EduKit III开发板。Embest EduKit III通常用于教学和实验,它提供了一个友好的环境来学习和实践嵌入式系统开发,包括硬件接口和驱动程序编程。 在Linux内核中,驱动程序通常作为一个模块存在,可以通过insmod或modprobe命令动态加载。对于S3C2410的ADC驱动,开发者需要了解以下关键知识点: 1. **硬件接口**:S3C2410的ADC模块包括多个输入通道,每个通道可以连接到不同的模拟信号源。驱动程序需要正确配置这些通道,设置采样率、分辨率和参考电压。 2. **中断处理**:ADC转换完成后,可能会触发中断。驱动程序需要注册中断处理函数,以在转换完成后读取数据并执行相应的操作。 3. **设备树**:在Linux 3.18及以上版本中,设备树是配置硬件的关键。驱动程序需要在设备树中定义ADC节点,描述其属性,如通道数、分辨率等。 4. **字符设备驱动模型**:ADC驱动通常实现为字符设备,通过open、read、write等系统调用来交互。驱动程序需实现对应的设备文件操作结构体。 5. **用户空间接口**:为了使应用程序能够访问ADC,驱动程序可能提供一个/dev节点,或者使用sysfs或procfs暴露数据。用户可以读取这个接口获取ADC的转换结果。 6. **性能优化**:考虑到嵌入式系统的资源限制,驱动程序可能需要优化,例如使用DMA(Direct Memory Access)来减少CPU负载,或者采用异步转换模式。 7. **调试工具**:像`i2c-tools`或`hwmon`这样的工具可以帮助开发者测试和调试ADC驱动。 文件列表中只有一个“adc”,可能是驱动程序的源代码文件,或者是包含编译后的模块。开发者需要解压这个rar文件,然后根据提供的文档或源代码来理解和使用S3C2410的ADC功能。对于初学者来说,这是一次宝贵的实践机会,可以深入理解嵌入式Linux驱动开发的过程。
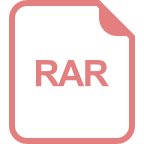
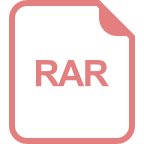
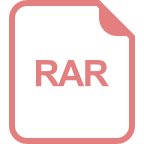
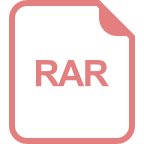
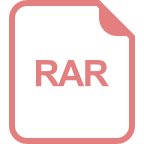
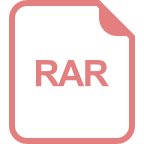
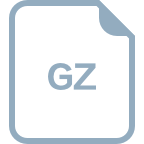
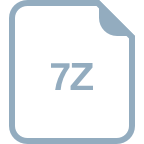
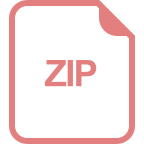
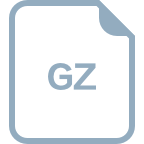
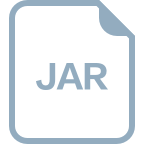
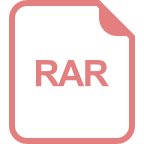
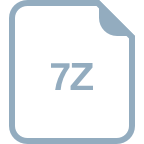
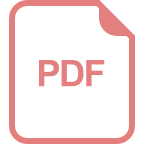
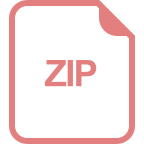


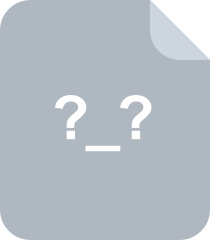
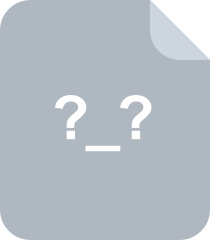
- 1
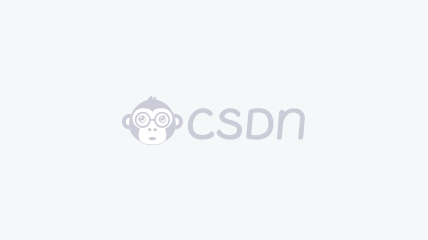

- 粉丝: 86
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

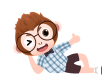
最新资源
- 基于Qt的上海地铁换乘系统详细文档+全部资料+高分项目.zip
- 发那科机器人二次开发 C#读取和写入数据,可以获取点位信息
- 基于QT的人脸识别,定位导航,脑电心率测算,用GPRS传到服务端的疲劳驾驶检测系统详细文档+全部资料+高分项目.zip
- 基于Qt的图书管理系统普通用户操作界面详细文档+全部资料+高分项目.zip
- 基于Qt的文件共享系统,类似百度网盘详细文档+全部资料+高分项目.zip
- 基于QT的网络视频监控系统详细文档+全部资料+高分项目.zip
- 基于QT的图书管理系统详细文档+全部资料+高分项目.zip
- 基于QT的学生成绩管理系统,QSS界面设计,SQL数据库的使用详细文档+全部资料+高分项目.zip
- 基于Qt的物业管理系统详细文档+全部资料+高分项目.zip
- 基于QT的直播管理系统详细文档+全部资料+高分项目.zip
- 基于Qt的学生信息管理系统、教师端:支持增删查改,班级成绩分析。学生端:查看成绩详细文档+全部资料+高分项目.zip
- 基于Qt的智能病房系统详细文档+全部资料+高分项目.zip
- 基于Qt构建的目标检测系统。基于dlib_rear_end_vehicles数据集详细文档+全部资料+高分项目.zip
- 基于QT的智能家居系统详细文档+全部资料+高分项目.zip
- 基于Qt和Mysql的教务管理系统详细文档+全部资料+高分项目.zip
- 基于Qt和mysql的大学生二手管理系统详细文档+全部资料+高分项目.zip

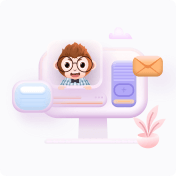
