//
// Copyright (c) Microsoft Corporation. All rights reserved.
//
//
// Use of this sample source code is subject to the terms of the Microsoft
// license agreement under which you licensed this sample source code. If
// you did not accept the terms of the license agreement, you are not
// authorized to use this sample source code. For the terms of the license,
// please see the license agreement between you and Microsoft or, if applicable,
// see the LICENSE.RTF on your install media or the root of your tools installation.
// THE SAMPLE SOURCE CODE IS PROVIDED "AS IS", WITH NO WARRANTIES OR INDEMNITIES.
//
//
// (C) Copyright 2006 Marvell International Ltd.
// All Rights Reserved
//
/******************************************************************************
**
** INTEL CONFIDENTIAL
** Copyright 2003-2005 Intel Corporation All Rights Reserved.
**
** The source code contained or described herein and all documents
** related to the source code (Material) are owned by Intel Corporation
** or its suppliers or licensors. Title to the Material remains with
** Intel Corporation or its suppliers and licensors. The Material contains
** trade secrets and proprietary and confidential information of Intel
** or its suppliers and licensors. The Material is protected by worldwide
** copyright and trade secret laws and treaty provisions. No part of the
** Material may be used, copied, reproduced, modified, published, uploaded,
** posted, transmitted, distributed, or disclosed in any way without Intel's
** prior express written permission.
**
** No license under any patent, copyright, trade secret or other intellectual
** property right is granted to or conferred upon you by disclosure or
** delivery of the Materials, either expressly, by implication, inducement,
** estoppel or otherwise. Any license under such intellectual property rights
** must be express and approved by Intel in writing.
**
**
******************************************************************************/
#include <windows.h>
#include <bsp.h>
#include "loader.h"
#include <gpio_soc.h>
#include <i2c_soc.h>
#include <bootpart.h> //for partition table dumping
#include "BLMenu.h"
#include "xllp_lcd_plat.h"
#include "I2C_SOC.h"
#include "PMIC_CHIP.h"
#include "xllp_mfp_plat.h"
//------------------------------------------------------------------------------
// External function prorotypes.
//
extern UINT16 hex_atoi(const char * hexstr);
extern void DumpMFPDomainAuto();
extern void LoadImageDDR(BOOL enableJump);
extern BSP_ARGS *g_pBSPArgs;
extern FlashInfo g_FlashInfo;
extern EBOOT_CFG *g_pEbootCFG ;
#define NUM_READ_WRITES 100000
#define NUM_READ_WRITES_SHORT 10
#define BUFF_SIZE 0x01000000 // 16MB
#define DMC_REG_BASE 0x48100000
#define MDCNFG_REG 0x000
#define MDREFR_REG 0x004
#define MDMRS_REG 0x040
#define DDR_SCAL_REG 0x050
#define DDR_HCAL_REG 0x060
#define DDR_WCAL_REG 0x068
#define DMCIER_REG 0x070
#define DMCISR_REG 0x078
#define EMPI_REG 0x090
#define RCOMP_REG 0x100
#define PAD_MA_REG 0x110
#define PAD_MDMSB_REG 0x114
#define PAD_MDLSB_REG 0x118
#define PAD_SDRAM_REG 0x11C
#define PAD_SDCLK_REG 0x120
#define PAD_SDCS_REG 0x124
#define PAD_SMEM_REG 0x128
#define PAD_SCLK_REG 0x12C
DWORD MenuDumpRegistersDDR(struct MENU_ITEM * pMenu)
{
volatile UINT8* pDFCReg = (volatile UINT8* )OALPAtoVA(DMC_REG_BASE, FALSE);
EdbgOutputDebugString ("DDR Registers Read Expected\r\n\r\n");
EdbgOutputDebugString ("MDCNFG : 0x%x 0x8000072D\r\n", *(UINT32*)(pDFCReg + MDCNFG_REG));
EdbgOutputDebugString ("MDREFR : 0x%x 0x00000019\r\n", *(UINT32*)(pDFCReg + MDREFR_REG));
EdbgOutputDebugString ("MDMRS : 0x%x 0x40000033\r\n", *(UINT32*)(pDFCReg + MDMRS_REG));
EdbgOutputDebugString ("DDR_SCAL : 0x%x 0x00000F0F\r\n", *(UINT32*)(pDFCReg + DDR_SCAL_REG));
EdbgOutputDebugString ("DDR_HCAL : 0x%x 0x90000000\r\n", *(UINT32*)(pDFCReg + DDR_HCAL_REG));
EdbgOutputDebugString ("DDR_WCAL : 0x%x 0x00000100\r\n", *(UINT32*)(pDFCReg + DDR_WCAL_REG));
EdbgOutputDebugString ("DMCIER : 0x%x 0x00000000\r\n", *(UINT32*)(pDFCReg + DMCIER_REG));
EdbgOutputDebugString ("DMCISR : 0x%x 0x00000000\r\n", *(UINT32*)(pDFCReg + DMCISR_REG));
EdbgOutputDebugString ("EMPI : 0x%x 0xC5000000\r\n", *(UINT32*)(pDFCReg + EMPI_REG));
EdbgOutputDebugString ("PAD_MA : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_MA_REG));
EdbgOutputDebugString ("PAD_MDMSB : 0x%x 0x22300808\r\n", *(UINT32*)(pDFCReg + PAD_MDMSB_REG));
EdbgOutputDebugString ("PAD_MDLSB : 0x%x 0x22300808\r\n", *(UINT32*)(pDFCReg + PAD_MDLSB_REG));
EdbgOutputDebugString ("PAD_SDRAM : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_SDRAM_REG));
EdbgOutputDebugString ("PAD_SDCLK : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_SDCLK_REG));
EdbgOutputDebugString ("PAD_SDCS : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_SDCS_REG));
EdbgOutputDebugString ("PAD_SMEM : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_SMEM_REG));
EdbgOutputDebugString ("PAD_SCLK : 0x%x 0x22300404\r\n", *(UINT32*)(pDFCReg + PAD_SCLK_REG));
EdbgOutputDebugString ("RCOMP : 0x%x 0x42000000\r\n", *(UINT32*)(pDFCReg + RCOMP_REG));
return 0;
}
DWORD MenuJumpToImageDDR(struct MENU_ITEM * pMenu)
{
UINT8 *pDataBuffer = (UINT8 *)RAM_LAYOUT_OS_CA_START;
UINT32 PhysAddress;
if (g_pBSPArgs->eBootCFG.dwLaunchAddr)
{
pDataBuffer = (UINT8 *) (g_pBSPArgs->eBootCFG.dwLaunchAddr);
}
#ifdef DEBUG
// Quick hack to find pTOC in image
{
ROMHDR* nk_pTOC=FindTOC((DWORD*) pDataBuffer);
DumpTOC(nk_pTOC);
}
#endif
// Translate the image start address (virtual address) to a physical address.
//
PhysAddress = (UINT32) OALVAtoPA((VOID *)pDataBuffer);
EdbgOutputDebugString("Download successful! Jumping to image at 0x%x (physical 0x%x)...\r\n", pDataBuffer, PhysAddress);
// Jump to the image we just downloaded.
//
EdbgOutputDebugString("\r\n\r\n\r\n");
Launch(PhysAddress);
// Should never get here...
//
SpinForever();
return 0;
}
/// Read each memory cell (as DWORD), compare to its address.
/// It must be cooperating with MenuWriteSimpleDDR().
/// @remarks only for test purpose
DWORD MenuVerifyDDR(struct MENU_ITEM * pMenu)
{
volatile DWORD *pDataBuffer = (volatile DWORD *) RAM_LAYOUT_OS_CA_START;
DWORD count;
DWORD index;
count = (RAM_LAYOUT_BOOT_SERVED_CA_START - RAM_LAYOUT_OS_CA_START) / sizeof(DWORD);
index = 0;
while (index++ < count)
{
if ((DWORD) * pDataBuffer != (DWORD) pDataBuffer)
EdbgOutputDebugString("\r\nAddress 0x%X not the same (Wanted 0x%X, Got 0x%X)\r\n", pDataBuffer, pDataBuffer, *pDataBuffer);
pDataBuffer++;
if (!((DWORD) pDataBuffer & 0xFFFFF))
{
OEMWriteDebugByte('\r');
EdbgOutputDebugString("0x%X", pDataBuffer);
}
}
EdbgOutputDebugString("Read DDR Done!\r\n");
return 0;
}
/// Write the virtual address of each memory cell into it.
///
/// @remarks only for test purpose
DWORD MenuWriteSimpleDDR(struct MENU_ITEM * pMenu)
{
volatile DWORD *pDataBuffer = (volatile DWORD *) RAM_LAYOUT_OS_CA_START;
DWORD count;
DWORD index;
count = (RAM_LAYOUT_BOOT_SERVED_CA_START - RAM_LAYOUT_OS_CA_START) / sizeof(DWORD);
index = 0;
while (index++ < count)
{
*pDataBuffer = (DWORD) pDataBuffer;
pDataBuffer++;
if (!((DWORD) pDataBuffer & 0xFFFFF))
{
OEMWriteDebugByte('\r');
EdbgOutputDebugString("0x%X", pDataBuffer);
}
}
EdbgOutputDebugString("Write DDR Done!\r\n");
return 0;
}
BOOL WriteReadSilent()
{
volatile DWORD
EBOOT.rar_boot_pxa310_pxa310 eboot_windows mobile boot
版权申诉
184 浏览量
2022-09-24
01:34:48
上传
评论
收藏 98KB RAR 举报

寒泊
- 粉丝: 74
- 资源: 1万+
最新资源
- Python爬取淘宝热卖商品并可视化分析
- 5152单片机proteus仿真和源码将按键次数写入AT24C02再读出并用1602LCD显示
- SE-SSD复现过程(Det3D的安装教程)
- 基于Python的在线学习与推荐系统设计与实现(论文+源码)-kaic
- 串口通过 YMODEM 协议进行文件传输
- 蓝桥杯2024年第十五届省赛真题-前缀总分
- com.qihoo.appstore_300101305-1.apk
- tensorflow-gpu-2.7.1-cp37-cp37m-manylinux2010-x86-64.whl
- tensorflow-2.7.2-cp37-cp37m-manylinux2010-x86-64.whl
- tensorflow-2.7.1-cp39-cp39-manylinux2010-x86-64.whl
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


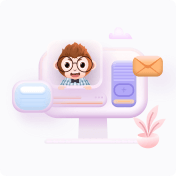
评论0