--
-- Copyright (c) 1993,1994 by Exemplar Logic, Inc. All Rights Reserved.
--
-- This source file may be used and distributed without restriction
-- provided that this copyright statement is not removed from the file
-- and that any derivative work contains this copyright notice.
--
-----------
--
-- This is a synthesizable description that implements an emulator
-- of the Mancala game (African beans game).
--
-- Description of the Hardware
-------------------------------
--
-- The hardware for the game includes a number of displays, each with a button and
-- a light, that each represent a 'bin' that can store marbles (beans).
--
-- The display indicates the number of marbles in each bin at any given time.
-- The light indecates that the present bin is not empty and that pushing the
-- button is a valid move in the game.
--
-- The button for each display indicates that a player takes the marbles from
-- the selected bin, and takes them in his hand. The hand is represented by a
-- diplay itself (no button).
--
-- Each player has a home bin, located on opposite sides of the game. The home
-- bin is also represented by a display. There should not be a button on the
-- home bins, since the game does not allow the removal of marbles from the home
-- bins.
--
-- Besides this, the game has a button to start the game, and a reset for power-up
-- purposes.
--
-- Here is a picture that represents the hardware setup of the game :
--
--
-- * == Light for valid move or to indicate the player who is active
-- O == Button to make move
-- _
-- | |
-- - == 7 - segment display
-- |_|
--
-- work bins
-- * O * O * O * O
-- _ _ _ _ _ _ _ _
-- | | | | | | | | | | | | | | | |
-- - - - - - - - -
-- * |_| |_| |_| |_| |_| |_| |_| |_| *
-- _ _ _ _
-- | | | | | | | |
-- - - - -
-- |_| |_| |_| |_|
--
-- home bin LEFT home bin right
-- * O * O * O * O
-- _ _ _ _ _ _ _ _
-- | | | | | | | | | | | | | | | |
-- - - - - - - - -
-- |_| |_| |_| |_| |_| |_| |_| |_|
--
-- work bins
--
-- _ _
-- | | | |
-- - - O Start Game
-- |_| |_|
--
-- Hand bin
--
--
-- The Rules of the game
------------------------
--
-- At the start of the game, the left player is active and can make a move.
-- The left player selects a bin (by pressing the corresponding button).
-- The machine will move the marbles from the bin (display) to the hand (diplay)
-- and drop one marble in each successive bin (clockwise) from the hand,
-- starting with the bin clock-wise adjecent to the selected bin.
-- A marble is never dropped in a opponents home bin (will be skipped).
--
-- If the last marble from the hand is dropped in an empty bin, the players
-- switch turns, and it is the other players turn to make a move.
--
-- If the last marble from the hand is dropped in the players home bin,
-- the player can make another move.
--
-- If the last marble from the hand is dropped in a non-empty work bin,
-- all the marbles from that bin will be moved back to the hand and the
-- game proceeds.
--
-- The game ends if there are no more marbles in any of the work bins.
--
-- The winner of the game is the player who has most marbles in his/her
-- home bin at the end of the game.
--
--
--
-- About the design
--------------------
--
-- The design contains a controller and a data path. The controller contains
-- a state machine that defines the overall state of the game (waiting for a
-- move, end of the game, playing).
-- The controller also has a register that defines which bin is active at any
-- point in time during active playing.
--
-- The controller provides signals for the data path to decrement the hand
-- marble count, or load the hand with the selected work bin count, or indecate
-- that the game is over and a winner should be defined etc.
--
-- The data path contains a register for each bin in the game.
-- The number of bins is easily programmable by setting a integer constant.
-- The data path also contains counters to decrement the hand marble count
-- or increment the bin marble counts.
--
-- The data path provides signals for the controller to indicate that the
-- hand bin is empty, or which of the work bins is empty.
--
-- The work bin registers are loaded with a equal number of marbles at the start
-- of the game. The total number of marbles in the game is programmable by setting
-- a generic in the top entity.
--
-- The data path also includes light drivers for the lights on each button that
-- indicate a valid move, and the lights that indicate which player is active.
-- Two extra signals are generated by the data path that let the home bin
-- display of the winner of the game blink on and off (at the end of the game).
--
-- The design does not include a merry-go-round display driver. This is done
-- outside this design, on the Aptix board.
--
-- The design does also not include a 18 bit clock devider that provides a
-- vary slow ticking clock to let humans follow the moves of the machine
-- cycle by cycle.
--
--
library ieee ;
use ieee.std_logic_1164.all ;
package mancala_pack is
type boolean_array is array (natural range <>) of boolean ;
type player_t is (LEFT, RIGHT, BOTH, NEITHER) ;
-- Define the number of bins in the game here.
-- This include the two home bins
constant nr_of_bins : natural := 10 ;
-- Define the indexes of the two home bins
constant OUTER_LEFT : natural := 0 ;
constant OUTER_RIGHT : natural := nr_of_bins/2 ;
-- Make a 'mask' constant that eliminates the home bins
constant not_home_bins : boolean_array (nr_of_bins-1 downto 0) :=
(OUTER_LEFT=>FALSE, OUTER_RIGHT=>FALSE, OTHERS=>TRUE) ;
-- Component Declaration of the controller of the game
component control
generic (nr_of_bins : natural := 32) ;
port (start_game : in boolean ;
reset, clk : in std_logic ;
buttons : in boolean_array (nr_of_bins-1 downto 0) ;
empty_bins : in boolean_array (nr_of_bins-1 downto 0) ;
hand_is_empty : in boolean ;
active_bin : buffer boolean_array (nr_of_bins-1 downto 0) ;
decrement_hand : out boolean ;
load_hand_with_active_bin : out boolean ;
the_player : out player_t ;
end_of_the_game : out boolean ;
waiting_for_move : out boolean
) ;
end component ;
end mancala_pack ;
library ieee ;
use ieee.std_logic_1164.all ;
use work.mancala_pack.all ;
entity control is
generic (nr_of_bins : natural := 10) ;
port (start_game : in boolean ;
reset, clk : in std_logic ;
buttons : in boolean_array (nr_of_bins-1 downto 0) ;
empty_bins : in boolean_array (nr_of_bins-1 downto 0) ;
hand_is_empty : in boolean ;
active_bin : buffer boolean_array (nr_of_bins-1 downto 0) ;
decrement_hand : out boolean ;
load_hand_with_active_bin : out boolean ;
the_player : out
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
最高优先级编码器 8位相等比较器 三人表决器(三种不同的描述方式) 加法器描述 8位总线收发器:74245 (注2) 地址译码(for m68008) 多路选择器(使用select语句) LED七段译码 多路选择器(使用if-else语句) 双2-4译码器:74139 多路选择器(使用when-else语句) 二进制到BCD码转换 多路选择器 (使用case语句) 二进制到格雷码转换 双向总线(注2) 汉明纠错吗译码器 三态总线(注2) 汉明纠错吗编码器 解复用器
资源详情
资源评论
资源推荐
收起资源包目录



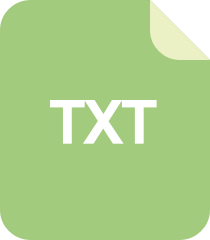
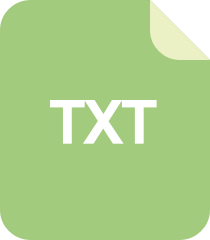
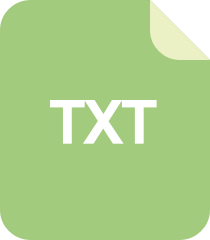
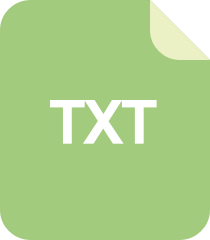
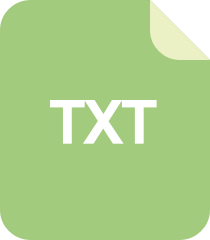
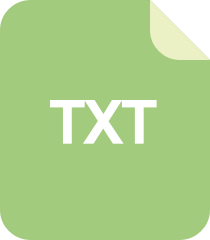
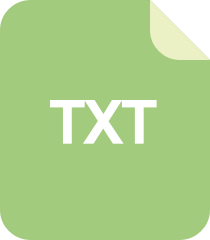

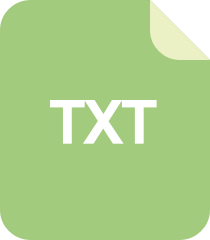
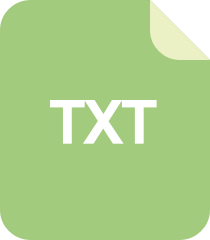
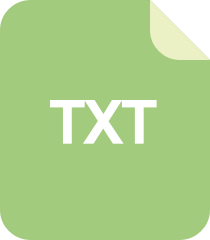
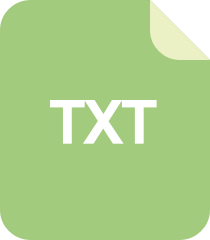

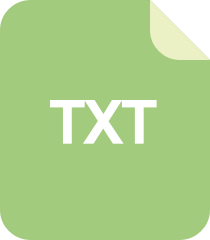

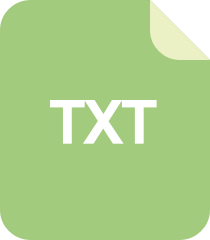
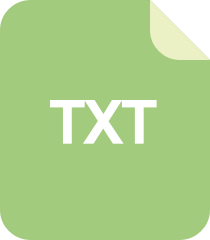
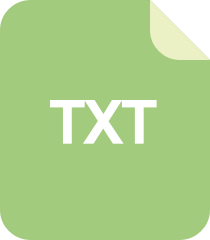

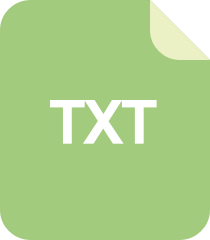
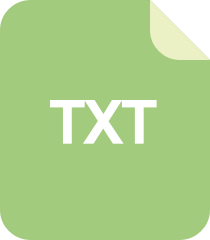
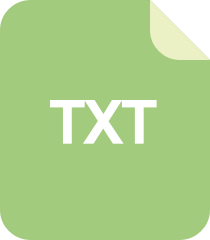
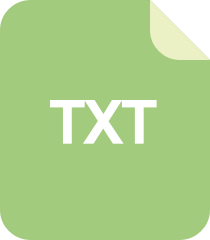
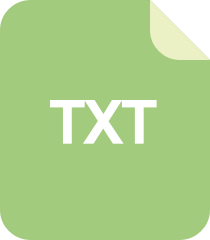
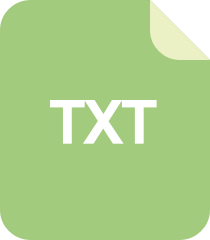
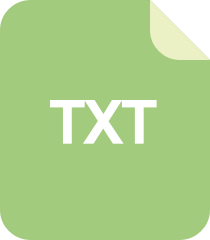
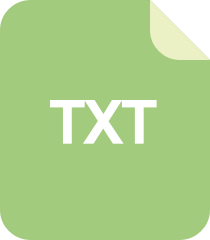

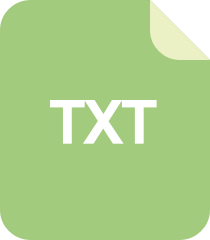
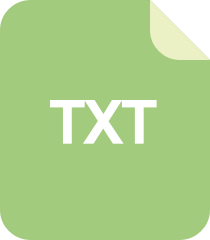
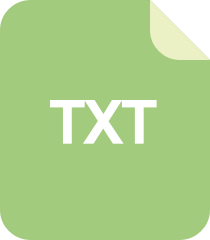
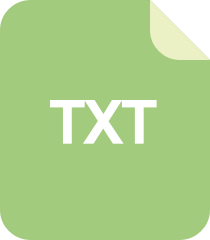
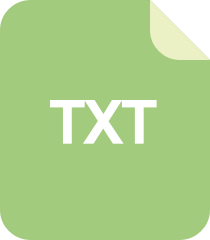
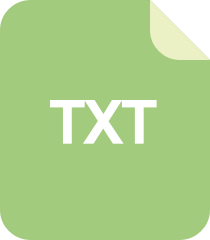
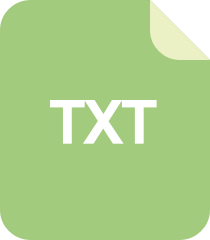

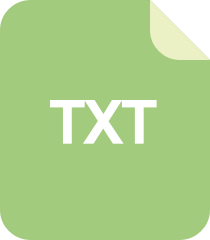
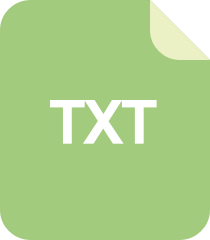
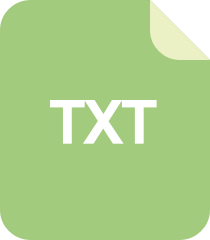
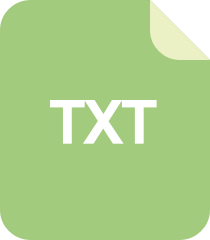
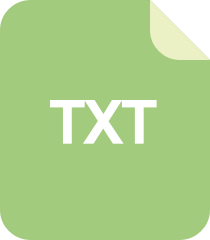
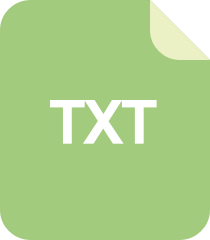
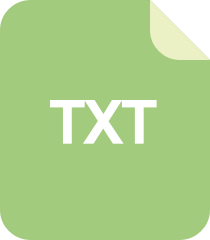
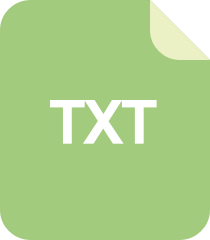
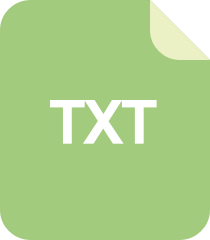
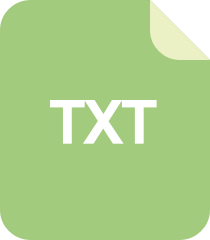
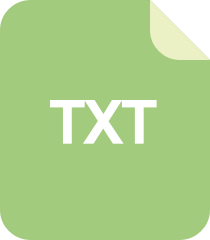
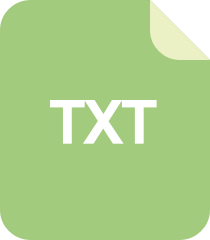
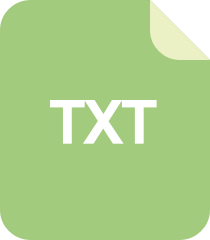
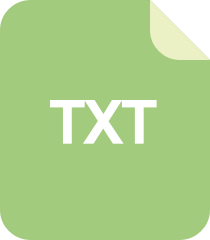
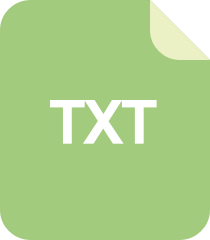
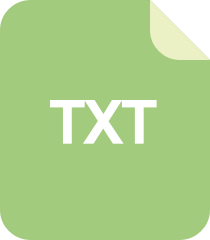
共 46 条
- 1
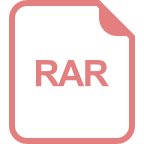
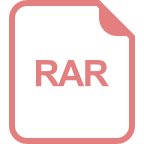
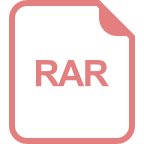
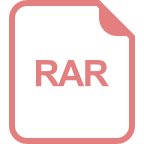
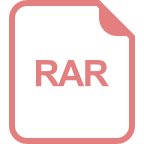
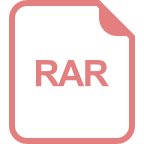
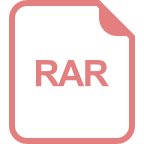
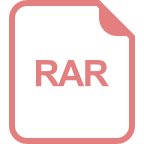
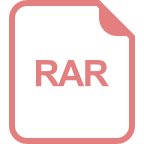
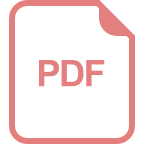
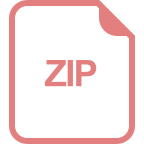
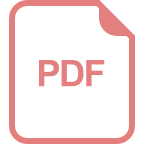
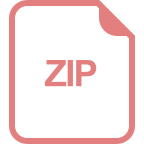

寒泊
- 粉丝: 75
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

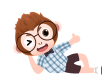
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0