jincheng.rar_jincheng
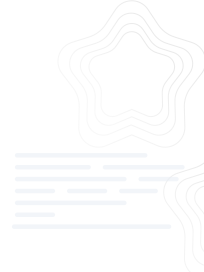

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
标题中的"jincheng.rar_jincheng"似乎是一个压缩文件,可能包含了一个名为"jincheng"的程序或代码库。描述中提到的程序是基于一个特定的调度算法设计的,这个算法遵循“优先权高者优先”(Preemptive Priority Scheduling)原则,并在优先权相同时,考虑进程的运行时间,即“运行时间短的优先”。这是一个典型的操作系统概念,涉及到进程管理和调度策略。 在操作系统中,进程调度是核心任务之一,它的目标是高效地分配CPU资源给等待执行的进程。"优先权高者优先"调度算法是一种抢占式调度,意味着如果一个新到达的进程优先权高于当前执行的进程,那么当前进程会被暂停,新进程立即获得CPU。这种算法常用于实时系统和多任务环境中,以确保关键任务能及时执行。 在优先权相同的情况下,程序考虑了"运行时间短的优先",这可能是短进程优先(Shortest Job First, SJF)策略。SJF算法是一种非抢占式策略,它试图减少平均等待时间,通常会提高系统效率。在描述中提到的情况,即使优先级相同,系统也会倾向于选择预计运行时间较短的进程,这样可以更快地完成更多进程,从而提高系统吞吐量。 压缩包内的文件"jincheng.c"很可能包含了实现这个调度算法的C语言源代码。C语言是一种常用的系统编程语言,适合编写操作系统级别的程序。通过分析和学习这段代码,我们可以深入理解这个调度算法的具体实现,包括如何定义和比较优先级,如何预估进程运行时间,以及如何在运行时进行进程切换等细节。 要深入了解这个程序,我们需要关注以下几个方面: 1. **进程结构**:程序是如何表示和管理进程的,包括进程的状态(如就绪、运行、阻塞)、优先级和预计运行时间。 2. **优先级设置**:如何为进程分配优先级,是否动态调整,以及调整的依据是什么。 3. **调度算法实现**:具体实现优先权高者优先和短进程优先的逻辑,包括何时抢占和如何选择下一个运行的进程。 4. **进程切换**:如何保存和恢复上下文,保证进程切换的正确性和效率。 5. **错误处理和同步机制**:如何处理异常情况,以及在多线程环境下如何保证数据安全。 通过研究这段代码,不仅可以加深对操作系统原理的理解,也可以锻炼实际编程和问题解决能力。对于学习计算机科学特别是操作系统方向的学生来说,这是一个很好的实践案例。
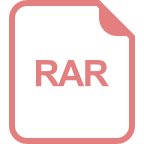
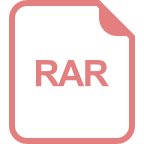
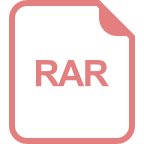
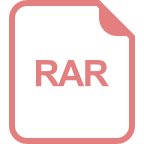
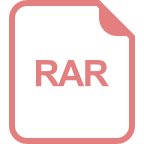
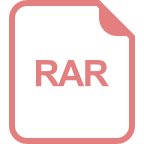
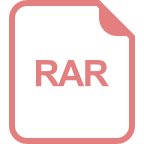
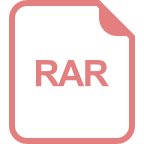
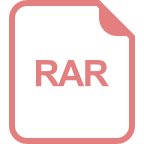
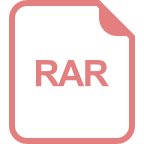
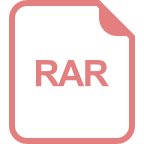
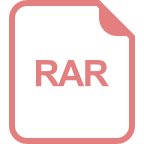
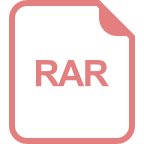
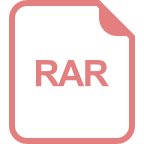

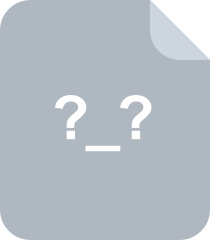
- 1
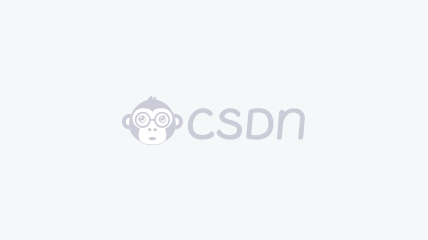

- 粉丝: 86
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

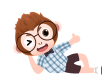
最新资源
- Qu120钢轨的CO2气体保护焊焊接.pdf
- RCC-M2007版与2000版+2002补遗对核级设备焊接过程中热输入要求及差异分析 - .pdf
- QXL锅炉连梁焊接变形的控制与火焰矫正 - .pdf
- RMD焊接工艺隧道管道安装中质量的防控措施.pdf
- RCC-M中的焊接材料评定.pdf
- S31803双相不锈钢球罐制造及焊接技术 - .pdf
- S31803双相不锈钢对接接头焊接工艺参数研究.pdf
- S30408等离子焊接接头组织与性能分析 - .pdf
- S450EW新型耐候钢焊接工艺与低温韧性研究 - .pdf
- S30408不锈钢活性焊接接头微观组织及性能研究.pdf
- S31008(06Cr25Ni20)耐热不锈钢的焊接工艺.pdf
- SA203 Cr.E 的气体容器的焊接工艺评定.pdf
- SA203Gr.D低温钢多道焊焊接性能试验研究.pdf
- SA213-T9合金耐热钢焊接技术.pdf
- SA-204Gr.C的焊接性能与金相组织.pdf
- SA-213T12换热管与SA-387Gr.11CL2管板内孔对接焊接工艺研究.pdf

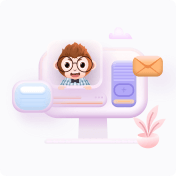
