查看DBASE数据库的结构 .zip_dbase
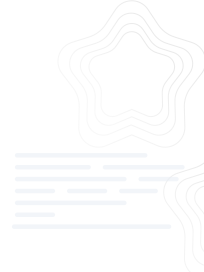

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
DBASE,作为一款早期的数据库管理系统,虽然在现代信息技术领域已不再主流,但它对现代数据库设计的影响深远。DBASE数据库的结构是理解数据库系统发展史的关键部分,它为后来的流行数据库如MySQL、SQL Server、Oracle等奠定了基础。在这个“查看DBASE数据库的结构 .zip_dbase”压缩包中,包含的文件可能提供了关于如何查看和理解DBASE数据库结构的指南。 我们来看看"www.pudn.com.txt"这个文件。这可能是从PUDN(编程开发网络)网站上下载的一个文本文件,其中可能包含了关于DBASE数据库结构的详细解释,包括如何创建数据库、定义字段、设置记录以及如何使用DBF文件格式。DBF文件是DBASE中存储数据的标准文件,每个字段都有其特定的数据类型,如数字、字符、日期等,而记录则由这些字段组成,形成完整的数据行。 另一个文件"DB2_STRU"可能是一个关于如何将DBASE结构映射到更复杂的数据库系统,比如IBM的DB2的文档。DB2是一个功能强大的关系型数据库管理系统,广泛应用于大型企业。DBASE的简单结构可能需要转换为更复杂的关系模型,以适应DB2的表、索引、视图等特性。这个文件可能详细阐述了这种转换过程,包括如何定义表结构、创建索引、设置约束条件等。 DBASE的结构主要包括以下几个核心元素: 1. **数据库文件(DBF)**:这是DBASE存储数据的主要文件,包含表的结构和数据。每个DBF文件都有一对对应的IDX文件,用于存储索引。 2. **字段(Field)**:字段是数据库中的基本单元,每个字段有特定的数据类型,如整数、字符串、日期等,并可以设定长度和小数位数。 3. **记录(Record)**:由一系列字段组成,代表一个完整的数据项。记录按照指定的顺序排列。 4. **表头(Header)**:包含关于数据库的信息,如记录数量、字段名、字段类型等。 5. **索引(Index)**:DBASE允许创建单个或多个索引来加速数据检索。索引文件(IDX)与DBF文件关联,提供快速访问特定记录的能力。 6. **操作(Operations)**:DBASE支持基本的数据库操作,如添加、修改、删除记录,以及查询操作,但不支持复杂的SQL语句。 通过理解DBASE的这些基本概念,我们可以更好地理解现代数据库系统的原理。尽管DBASE已不再主流,但它的设计理念和模式依然影响着当今的数据库技术。学习DBASE可以帮助我们追溯数据库的发展历程,同时也能提高我们处理各种数据库问题的能力。
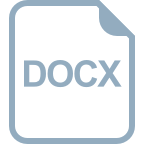
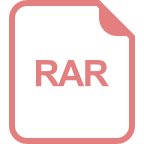
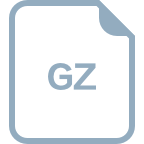
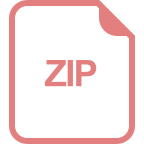
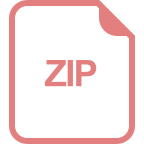
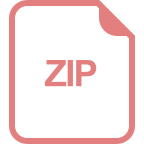
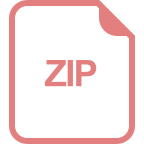
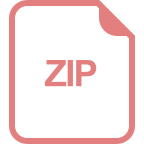
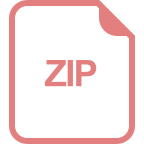
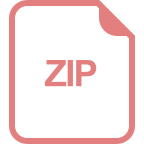
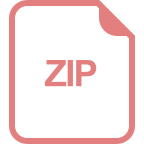
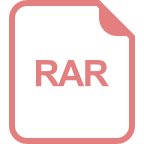
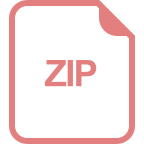
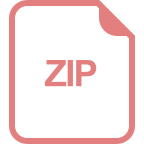
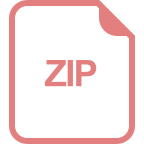
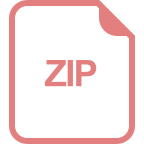
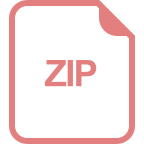
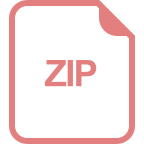
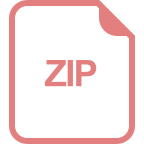
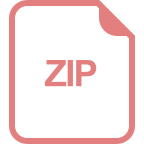
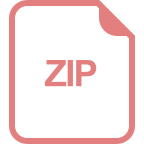
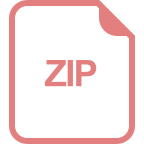
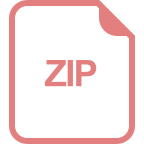
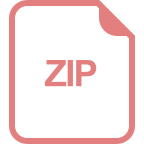
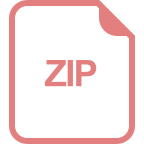
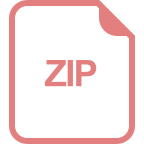
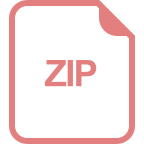
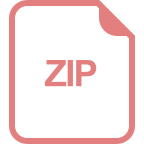


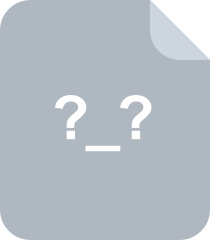
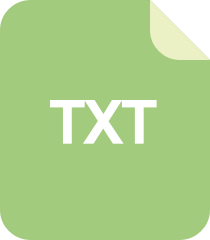
- 1
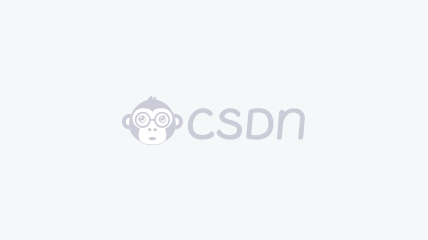

- 粉丝: 85
- 资源: 1万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

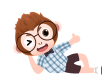
最新资源
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍

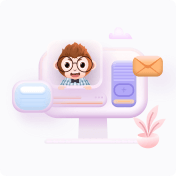
