<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>JWChat</title>
<meta http-equiv="content-type" content="text/html; charset=utf-8">
<script type="text/javascript" src="config.js"></script>
<script type="text/javascript" src="shared.js"></script>
<script type="text/javascript" src="browsercheck.js"></script>
<script type="text/javascript" src="sounds.js"></script>
<script type="text/javascript" src="statusLed.js"></script>
<!-- JabberConnection -->
<script type="text/javascript" src="jsjac.js"></script>
<!-- Debugger -->
<script type="text/javascript" src="Debugger.js"></script>
<script type="text/javascript">
<!--
/* ***
* JWChat, a web based jabber client
* Copyright (C) 2003-2007 Stefan Strigler <steve@zeank.in-berlin.de>
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*
* Please visit http://jwchat.sourceforge.net for more information!
*/
/************************************************************************
* ****** GLOBAL WARS(tm) *******
************************************************************************
*/
var jid;
var pass;
var register = false; // whether to register new account
var nick;
var vcard;
var status = '';
var onlstat = '';
var onlmsg = '';
var onlprio = '8';
var autoPopup = true;
var autoPopupAway = false;
var playSounds = true;
var focusWindows = true;
var timestamps = false;
var usersHidden = false;
var enableLog = false;
var loghost;
/* some globals */
var roster;
var fmd; // frames.main.document
var disco; // holds information from service discovery
var statusLed;
var statusMsg;
var onlstatus = new Object();
onlstatus["available"] = "online";
onlstatus["chat"] = "free for chat";
onlstatus["away"] = "away";
onlstatus["xa"] = "not available";
onlstatus["dnd"] = "do not disturb";
onlstatus["invisible"] = "invisible";
onlstatus["unavailable"] = "offline";
/************************************************************************
* ****** Pop-Up's *******
************************************************************************
*/
function openGroupchat(aJid,nick,pass) {
pass = pass || '';
nick = nick || '';
var user = roster.getUserByJID(aJid);
if(!user) {
user = roster.addUser(new RosterUser(aJid,'',["Chat Rooms"],aJid.substring(0,aJid.indexOf('@'))));
user.type = 'groupchat';
roster.print();
}
if (!user.chatW || user.chatW.closed)
user.chatW = open("groupchat.html?jid="+escape(aJid)+"&nick="+escape(nick)+"&pass="+escape(pass),"gchatW"+makeWindowName(aJid+"/"+nick),"width=520,height=390,resizable=yes");
user.chatW.focus();
}
var subw;
function openSubscription(aJid) {
var param = (aJid) ? "?jid="+escape(aJid) : "";
subw = open("subscription.html"+param,"sub","width=320,height=240,resizable=yes");
subw.focus();
return false;
}
function openCustomPresence(aJid) {
var user = roster.getUserByJID(aJid);
if (!user)
return;
if (!user.onlStatW || user.onlStatW.closed)
user.onlStatW = open("changestatus.html?jid="+escape(aJid),"onlStatW","width=330,height=240,resizable=yes");
user.onlStatW.focus();
return false;
}
function sendCustomPresence(aJid,presence,msg) {
var oPresence = new JSJaCPresence();
oPresence.setTo(aJid);
if (roster.getUserByJID(aJid).roster)
oPresence.setXMLNS();
switch (presence) {
case 'offline':
oPresence.setType('unavailable');
case 'unavailable':
oPresence.setType('unavailable');
presence = "invisible";
default:
if (presence != 'available')
oPresence.setShow(presence);
}
if (typeof(msg) != 'undefined' && msg != '')
oPresence.setStatus(msg);
Debug.log(oPresence.getDoc().xml,2);
con.send(oPresence);
}
function openUserProps(aJid) {
open("userprops.html?jid="+escape(aJid),"uProps"+makeWindowName(aJid),"width=480,height=360,resizable=yes");
return false;
}
function removeUser(aJid) {
// get fulljid
var fulljid = roster.getUserByJID(aJid).fulljid;
var iq = new JSJaCIQ();
iq.setType('set');
var query = iq.setQuery('jabber:iq:roster');
var item = query.appendChild(iq.getDoc().createElement('item'));
item.setAttribute('jid',fulljid);
item.setAttribute('subscription','remove');
con.send(iq);
}
var vcardW; // my vcardW;
function openUserInfo(aJid) {
var newin = open("vcard.html?jid="+escape(aJid),"vcardW"+makeWindowName(aJid),"width=400,height=580,scrollbars=yes");
if (cutResource(aJid) == cutResource(jid))
vcardW = newin;
else {
var user = roster.getUserByJID(cutResource(aJid));
if (!user) {
user = new RosterUser(aJid);
roster.addUser(user);
}
if (user.roster) // groupchat(!)
user = user.roster.getUserByJID(aJid);
user.vcardW = newin;
}
return false;
}
function openUserHistory(aJid) {
if (typeof(loghost) == 'undefined' || loghost == '')
return;
var user = roster.getUserByJID(aJid);
if (user == null)
return;
if (!user.histW || user.histW.closed)
user.histW = open("userhist.html?jid="+escape(aJid),"histW"+makeWindowName(aJid),"width=600,height=400,resizable=yes,scrollbars=no");
user.histW.focus();
}
function openUserNote(aJid) { /* store annotations to a user */
var user = roster.getUserByJID(aJid);
if (user == null)
return; // unbelievable
if (!user.noteW || user.noteW.closed)
user.noteW = open("usernote.html?jid="+escape(aJid),"noteW"+makeWindowName(aJid),"width=300,height=200,resizable=yes,scrollbars=no");
user.noteW.focus();
}
var searchW;
function openSearch() {
if (!searchW || searchW.closed)
searchW = open("search.html","searchW"+makeWindowName(jid),"width=480,height=260,resizable=yes,scrollbars=yes");
searchW.focus();
return false;
}
var ebW
function openEditBookmarks() {
if (!ebW || ebW.closed)
ebW = open("editbookmarks.html","ebw"+makeWindowName(jid),"width=330,height=290,resizable=yes");
return false;
}
/************************************************************************
* nifty helpers - always there if you need 'em
************************************************************************
*/
/* command line history */
var messageHistory = new Array();
var historyIndex = 0;
function getHistory(key, message) {
if ((key == "up") && (historyIndex > 0)) historyIndex--;
if ((key == "down") && (historyIndex < messageHistory.length)) historyIndex++;
if (historyIndex >= messageHistory.length) {
if (historyIndex == messageHistory.length) return '';
return message;
} else {
return messageHistory[historyIndex];
}
}
function addtoHistory(message) {
if (is.ie5)
messageHistory = messageHistory.concat(message);
else
messageHistory.push(message);
historyIndex = messageHistory.length;
}
/* system sounds */
var soundPlaying = false;
function soundLoaded() {
soundPlaying = false;
}
function playSound(action) {
if (!playSounds)
return;
if(!SOUNDS[action]) {
Debug.log("no sound for '" + action + "'",1);
return;
}
if (onlstat != '' && onlstat != 'available' && onlstat != 'chat')
return;
if (soundPlaying)
return;
soundPlaying = true;
var frameD = frames["jwc_sound"].document;
var html = "<embed src=\""+SOUNDS[action]+"\" width=\"1\" height=\"1\" quali
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
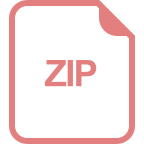
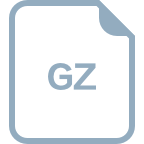
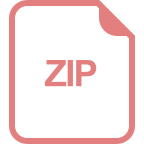
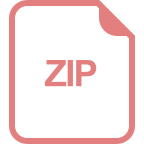
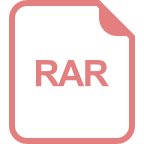
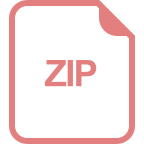
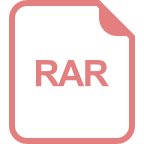
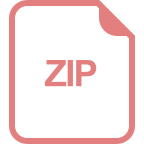
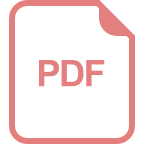
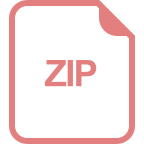
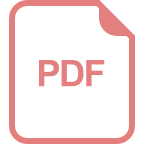
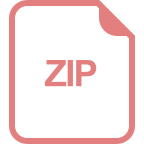
收起资源包目录

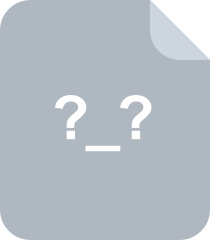
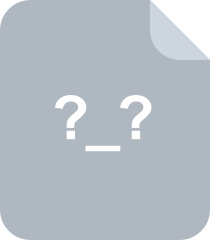
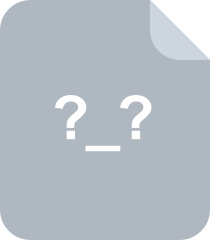
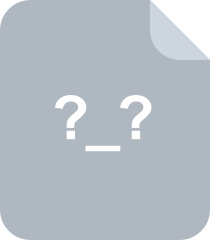
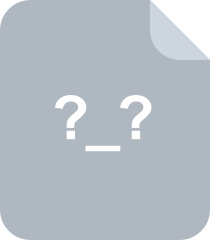
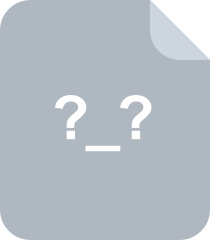
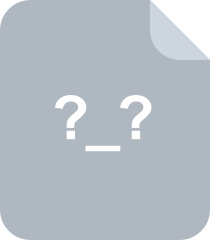
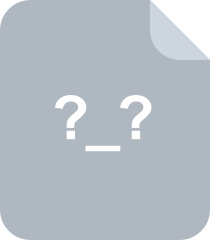
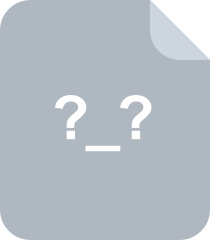
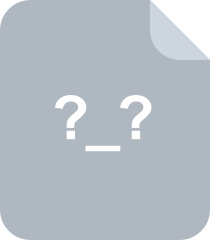
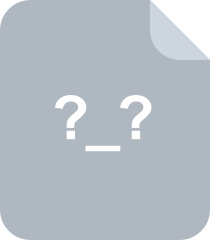
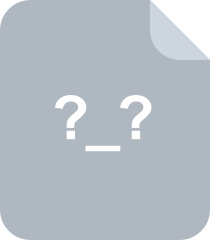
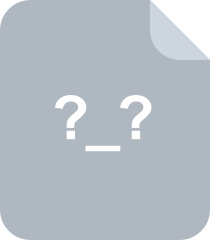
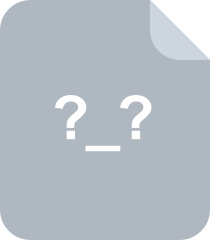
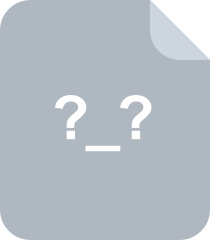
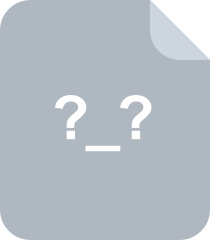
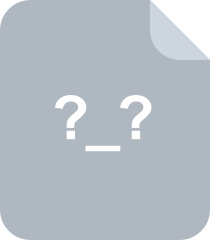
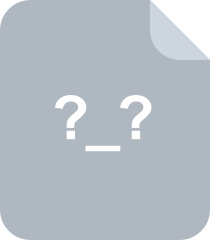
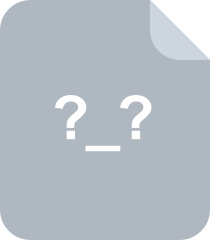
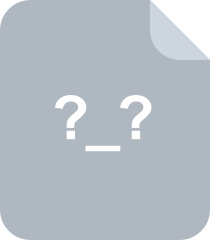
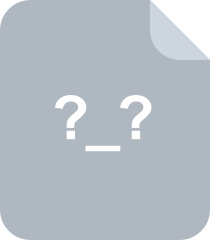
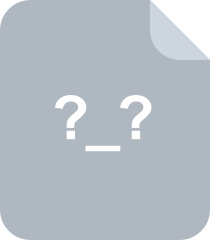
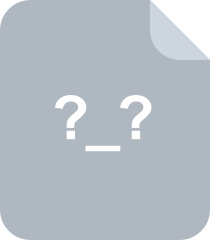
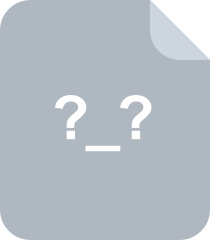
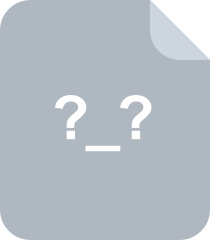
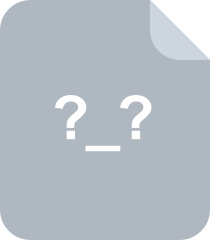
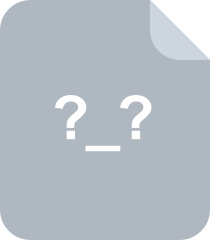
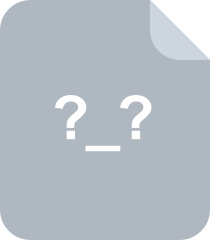
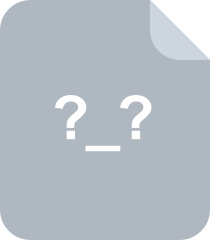
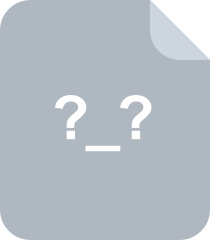
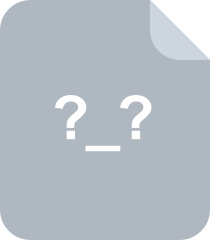
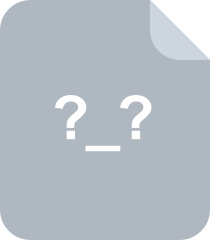
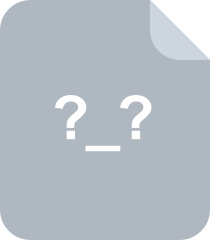
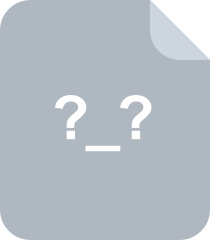
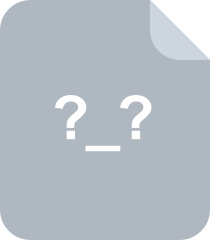
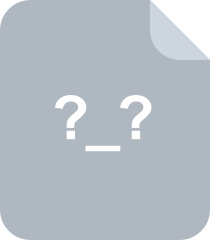
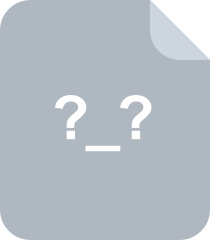
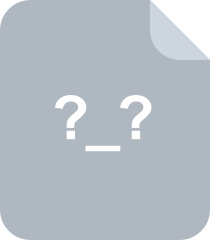
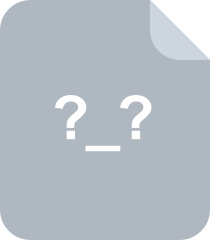
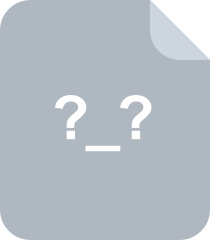
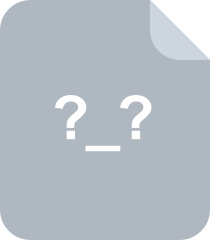
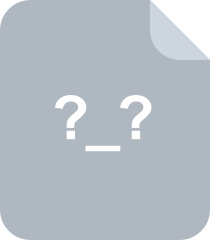
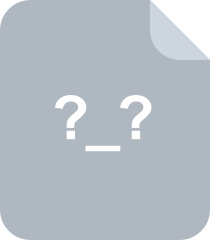
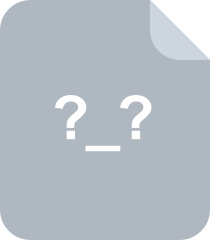
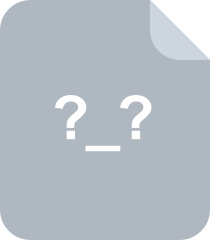
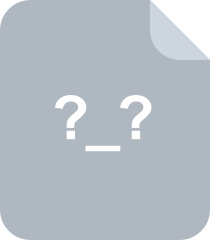
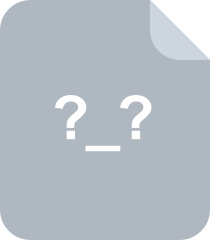
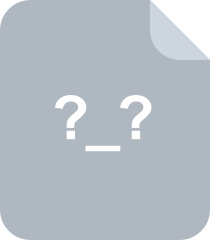
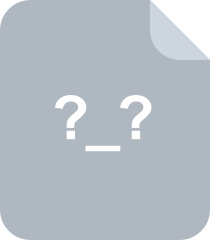
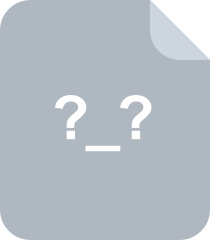
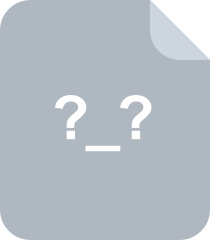
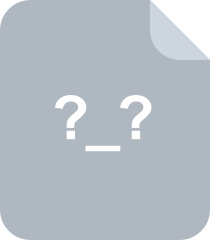
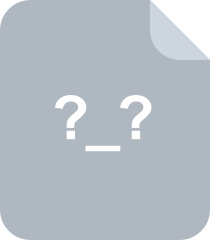
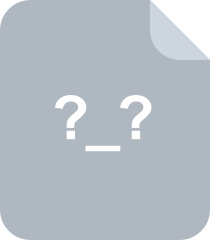
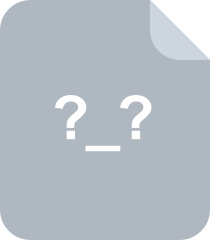
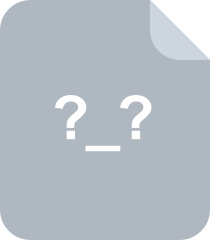
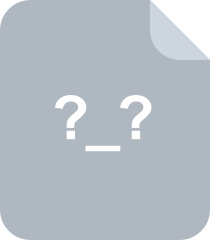
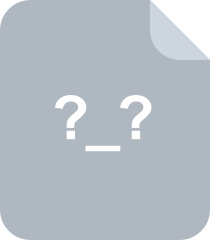
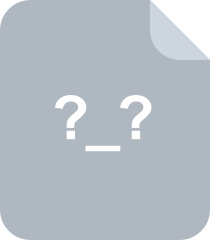
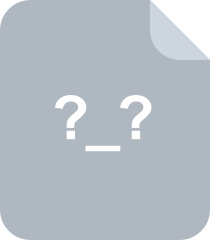
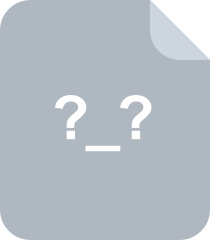
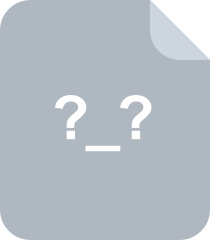
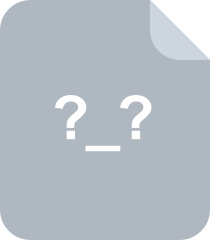
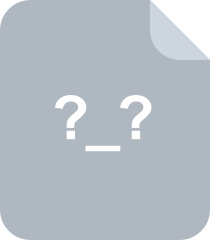
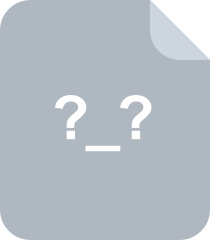
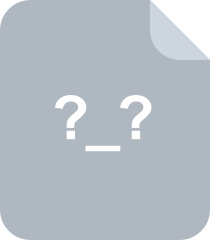
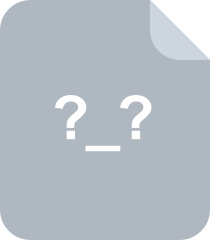
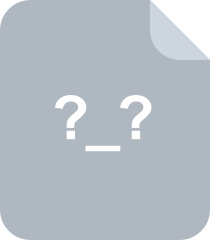
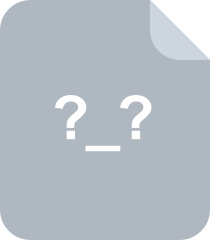
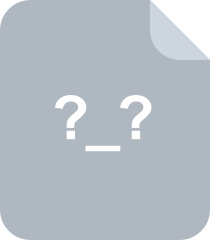
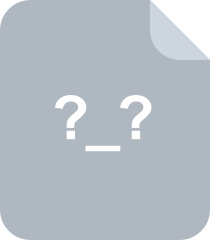
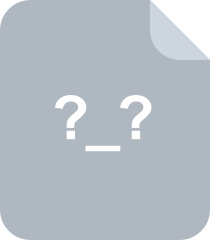
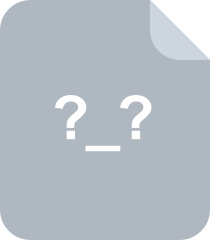
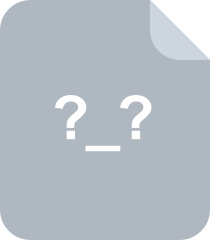
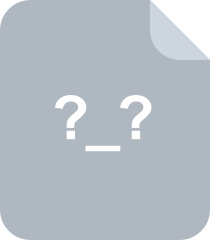
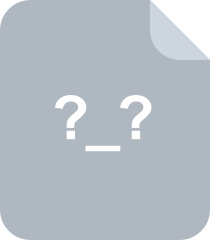
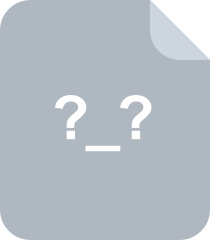
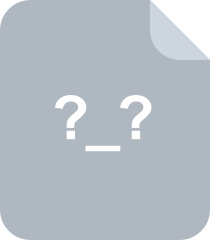
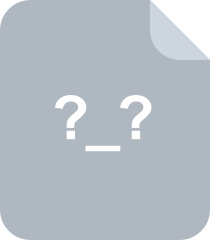
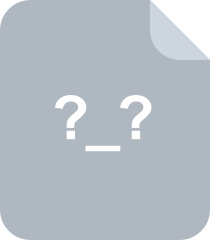
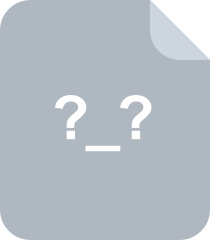
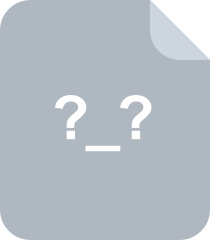
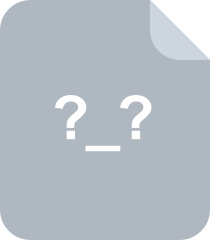
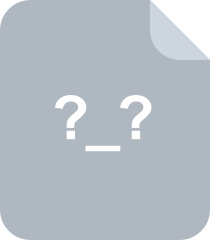
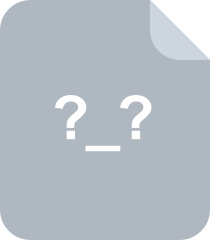
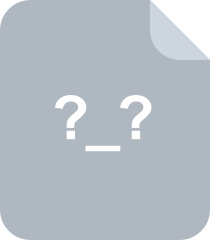
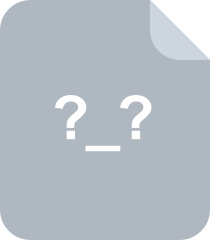
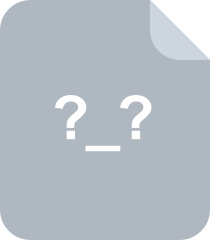
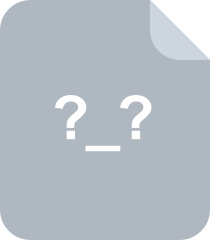
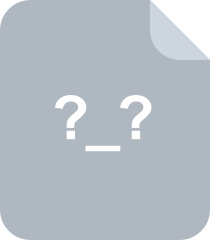
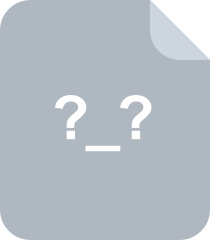
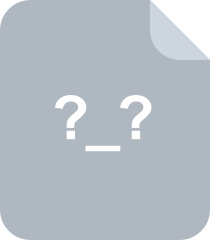
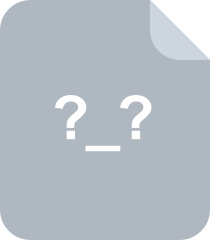
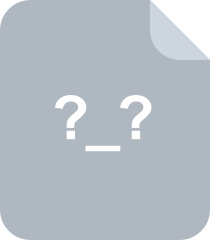
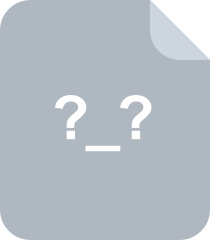
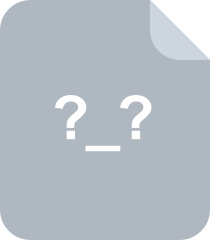
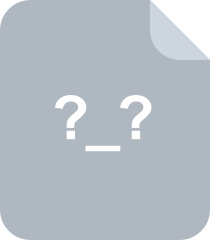
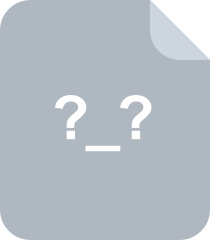
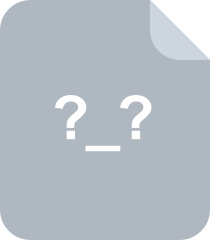
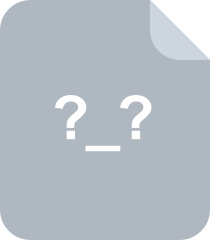
共 856 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
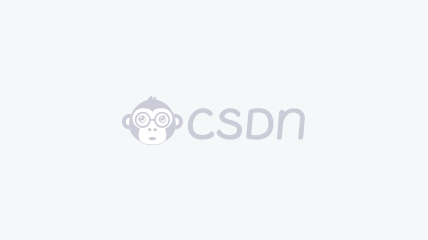

小贝德罗
- 粉丝: 68
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

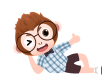
最新资源
- STM8L101F3P6单片机+CC1100模块433M遥控器设计硬件(原理图+PCB)工程文件.zip
- 上传下载铁人下载系统 Liuxing 1.0-liuxing1.0.rar
- 南京邮电大学数学实验实力雄厚,凭借其优秀的师资力量、丰富的实践教学资源和卓越的科研成果,成为国内一流的数学实验教学和科研基地
- 【火爆朋友圈的今天吃什么源码 v1.0】随机的为用户带来每一天的用餐选择和推荐.rar
- MPU6050中文版数据手册
- 上传下载手机电影下载-mobiledy.rar
- 响应式旅游网站源码下载 马尔代夫旅游网站.rar
- CMS小涴熊漫画连载系统漫画网站源码 带采集API.rar
- 福袋点点.apk
- 基于STM32的电子秤采用0.96寸OLED显示UI界面源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


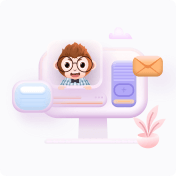
安全验证
文档复制为VIP权益,开通VIP直接复制
