/*************************************************************************
* Name : serial_eeprom.c *
* Author : Rajesh Bhandari 09423207019 *
* Date : 13 / apr / 2009 *
* Summary : this file contains the definitions of all *
* the functions required to store data into, *
* in serial eeprom of 24CXX series and to *
* get the data back from the eeprom *
*************************************************************************/
//---------------------------------Defining Macros---------------------------------------------------------------------------
sbit sda = P3^6; //P3^2;//P1^1;
sbit sclk = P3^7; //P3^3;//P1^0;
//---------------------------------------------------------------------------------------------------------------------------
//---------------------------------FXN prototypes----------------------------------------------------------------------------
void start_s_eeprom();
void send_byte_s_eeprom(char);
char get_byte_s_eeprom();
void stop_s_eeprom();
void send_to_mem(char, char);
unsigned int get_from_mem(char);
void wait();
void acknowledge();
//---------------------------------------------------------------------------------------------------------------------------
//-----------------fxn to send data to serial eeprom-------------------------------------------------------------------------
// give the adress and the data as the parametrs to the fxn
void send_to_mem(char s_address, char s_data)
{
start_s_eeprom(); // sending start condition to eeprom
send_byte_s_eeprom(0XA0); // A0 = 10100000 = sending device address word for write
acknowledge();
send_byte_s_eeprom(s_address); // sending data address
acknowledge();
send_byte_s_eeprom(s_data); // sending data
acknowledge();
stop_s_eeprom(); // sending stop condition to eeprom
acknowledge();
return;
}
//---------------------------------------------------------------------------------------------------------------------------
//------------------fxn to get the data back frm the serial eeprom-----------------------------------------------------------
// just give the adress from where the data is to be retrieved
unsigned int get_from_mem(char s_address)
{
char i = 0;
//-------dummy write seq----+ word address------------------------------------
start_s_eeprom(); // sending start condition to eeprom
send_byte_s_eeprom(0XA0); // sending A0 = 10100000 = device address word for write
acknowledge();
send_byte_s_eeprom(s_address); // sending data address
acknowledge();
//----------------dummy over----------------------------------------------------
start_s_eeprom();
send_byte_s_eeprom(0XA1); // sending A1 =10100001 = device adress word for read
acknowledge();
i = get_byte_s_eeprom(); // sending data
acknowledge();
stop_s_eeprom(); // sending stop condition to eeprom
acknowledge();
return(i);
}
//---------------------------------------------------------------------------------------------------------------------------
//----------------------fxn to transmit a byte to the eeprom ----------------------------------------------------------------
//this fxn just send the 8 bits serialy on the SDA line
//this fnx does not store the data in eeprom but just transmits it, this fxn is used by the storing fxn
//just pass the byte to be transmitted as parameter to this fxn
void send_byte_s_eeprom(char s_byte)
{
char temp = s_byte;
char i ;
for(i = 7 ; i >= 0 ; i--)
{
temp = s_byte;
temp = temp >> i;
temp = temp & 0X01;
if(temp == 0)
sda = 0;
else
sda = 1;
sclk = 1;
wait();
sclk = 0;
}
return;
}
//---------------------------------------------------------------------------------------------------------------------------
//---------------------------fxn to receive 8 bits serialy from sda line-----------------------------------------------------
// this is not a fxn to read from eeprom
// it just receives 8 bits serialy and retuns the byte received to the calling fxn
char get_byte_s_eeprom()
{
char temp, temp_h, i;
temp = 0;
temp_h = 1;
sda = 1; // making SDA as input pin for microcontroller
sclk = 0;
for(i = 7; i >=0 ; i--)
{
sclk = 1;
if(sda == 1)
{
temp = temp | temp_h<<i ;
}
wait();
sclk = 0;
}
sclk = 0;
return(temp);
}
//---------------------------------------------------------------------------------------------------------------------------
//--------------------------fxn to send the start condition -----------------------------------------------------------------
void start_s_eeprom()
{
sda = 1;
sclk = 1;
wait();
sda = 0;
sclk = 0;
return;
}
//---------------------------------------------------------------------------------------------------------------------------
//------------------------------fxn to send stop condition--------------------------------------------------------------------
void stop_s_eeprom()
{
sda = 0;
sclk = 1;
wait();
sda = 1;
sclk = 0;
return;
}
//---------------------------------------------------------------------------------------------------------------------------
//-------------------------------------fxn for acknowledging the eeprom------------------------------------------------------
// this fxn actualy does not read the acknowledge signal
// it just waits for sufficient time and assumes that the eeprom has given tha ack by the time the wait gets over
void acknowledge()
{
sclk = 1;
wait();
sclk = 0;
return;
}
//---------------------------------------------------------------------------------------------------------------------------
//--------------------------a small delay fxn to ensure the line settels down after transition-------------------------------
void wait()
{
char i;
for(i=0;i<=20;i++)
i++;
return;
}
//---------------------------------------------------------------------------------------------------------------------------
new_asm.zip_ADUC847
版权申诉
58 浏览量
2022-09-21
07:37:11
上传
评论
收藏 63KB ZIP 举报

小贝德罗
- 粉丝: 71
- 资源: 1万+
最新资源
- MySQL是一种广泛使用的开源关系型数据库管理系统,它提供了丰富的SQL语句用于数据库的创建、查询、更新和管理 以下是一些常见的
- MySQL是一种广泛使用的开源关系型数据库管理系统,它提供了丰富的SQL语句用于数据库的创建、查询、更新和管理 以下是一些常见
- MySQL是一种广泛使用的开源关系型数据库管理系统,它提供了丰富的SQL语句用于数据库的创建、查询、更新和管理 以下是一些常见的
- 基于Javascript的结婚请帖设计源码 - Invitation
- mysql语句大全及用法
- mysql语句大全及用法
- mysql语句大全及用法
- MySQL是一种广泛使用的开源关系型数据库管理系统
- MySQL是一种广泛使用的开源关系型数据库管理系统
- MySQL是一种广泛使用的开源关系型数据库管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


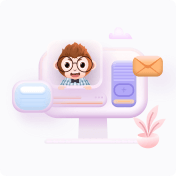