#include<stdio.h>
#include<stdlib.h>
#define TRUE 1
#define FALSE 0
#define OK 1
#define ERROR 0
#define INFEASIBLE -1
#define OVERFLOW -2
#define LIST_INIT_SIZE 100
#define LISTINCREMENT 10
typedef int Status;
typedef int Elemtype;
typedef struct {
Elemtype *elem;
int length;
int listsize;
} SqList;
Status Initlist_Sq(SqList &L)//构造一个空的线性表L
{
L.elem = (Elemtype *)malloc(LIST_INIT_SIZE * sizeof (Elemtype));
if (!L.elem)
return(OVERFLOW);
L.length = 0;
L.listsize = LIST_INIT_SIZE;
return OK;
}
Status ListInsert_Sq(SqList&L, int i, Elemtype e) {
Elemtype *newbase,* p, *q;
if (i < 1 || i > L.length + 1)return ERROR;
if (L.length > L.listsize) {
newbase = (Elemtype*) realloc(L.elem, (L.listsize + LISTINCREMENT) * sizeof (Elemtype));
if (!newbase)return(OVERFLOW);
L.elem = newbase;
L.listsize += LISTINCREMENT;
}
q =& L.elem[i - 1];
for (p =&( L.elem[L.length - 1]); p >= q; --p)
*(p + 1) = *p;
*q = e;
++L.length;
return OK;
}
Status ListDelete_Sq(SqList&L, int i, Elemtype &e) {
int *p, *q;
if (i < 1 || i > L.length + 1)return ERROR;
p = &(L.elem[i - 1]);
e = *p;
q = L.elem + L.length - 1;
for (++p; p <= q; ++p)
*(p - 1) = *p;
--L.length;
return OK;
}
Status ListCreat_Sq(SqList &L) {
int i,x,n;
printf("how many number do you want to scanf?");
scanf("%d",&n);
printf("creat your list now: ");
for (i=1; i<=n; i++)
{
scanf("%d",&x);
ListInsert_Sq(L,i,x);
}
return OK;
}
Status ListLength_sq(SqList &L) {
return( L.length);
}
Status ListPrint_Sq(SqList &L) {
int i;
for (i=0; i <L.length; i++)
printf("%d ", L.elem[i]);
printf("\n");
return OK;
}
int main()
{
SqList L;
int i,j,len,e,f;
if
(!Initlist_Sq(L))printf("init fail.\n") ;
else printf("init success!\n\n");
printf("**************************************\n");
ListCreat_Sq(L);
len=ListLength_sq(L);
printf("your list has %d elem.\n",len);
printf("they are:\n");
ListPrint_Sq(L) ;
printf("**************************************\n");
printf("where do you want to insert?\nputs it:");
scanf("%d",&i);
printf("what item do you want to insert?\nputs it:");
scanf("%d",&e);
ListInsert_Sq(L,i,e);
printf("now your list is:\n");
ListPrint_Sq(L) ;
printf("**************************************\n");
printf("where do you want to delet?\nputs it:");
scanf("%d",&j);
printf("the elem you want to delet is:");
scanf("%d",&f);
ListDelete_Sq(L,j,f);
printf("now your list is:\n");
ListPrint_Sq(L) ;
return 0;
}

钱亚锋
- 粉丝: 107
- 资源: 1万+
最新资源
- 社交网络引流副业的简易实施策略及收益分析
- 西门子消防图层显示软件
- 基于Node.js和Express框架的租房系统房屋出租管理后端设计源码
- VideoSpeed_87621.zip
- 基于Typescript和CSS的八电极指标报告PDF设计源码
- 短视频游戏推广副业快速获利-通过快手小游戏合伙人计划轻松入行
- MATLAB仿真均匀光纤布拉格光栅 传输矩阵法 可以仿真得到其透射谱与反射谱
- 基于Vue框架的快递代取后台管理新版本设计源码
- Linux驱动开发环境Ubuntu,已经制作好网络文件系统和zImage内核,已经交叉编译好Qt5.6.2 1.安装好交叉编译工具链 2.制作好网络文件系统 3.已经编译好Linux内核源码树(版本
- 基于广西忻城红渡初中22班的HTML, JavaScript, CSS同学录设计源码
- MATLAB环境下一种时间序列信号的基线消除算法 算法运行环境为MATLAB r2018a 1.所有代码均经过运行测试,没有问题 2.前请仔细阅读作品简介,这非常重要,因为涉及到不同的编程语言
- 基于Mql5语言的MT5客户端直连期货公司CTP柜台的期货程序化交易软件设计源码
- containerd源码1.7.22 tag
- 基于Java语言的Swing游戏引擎设计源码
- MATLAB环境下一种基于粒子群优化算法神经网络非线性函数拟合方法 算法运行环境为MATLAB R2018a,执行基于粒子群优化算法神经网络非线性函数拟合,并与其他改进的粒子群算法进行对比,结果如下
- 图像处理实验、图像分割 1打开计算机,安装和启动MATLAB程序;程序组中“work”文件夹中应有待处理的图像文件; 2对于血细胞图像 a).对图像进行去噪、增强处理; b)运用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


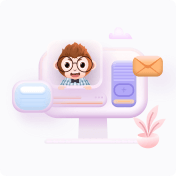
评论0