// GDIPlusHelper.cpp: implementation of the CGDIPlusHelper class.
// Download by http://www.codefans.net
//////////////////////////////////////////////////////////////////////
#include "stdafx.h"
#include "ImageEx.h"
#include <process.h>
#ifdef _DEBUG
#undef THIS_FILE
static char THIS_FILE[]=__FILE__;
#define new DEBUG_NEW
#endif
//////////////////////////////////////////////////////////////////////
// Construction/Destruction
//////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: ImageEx::ImageEx
//
// DESCRIPTION: Constructor for constructing images from a resource
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
ImageEx::ImageEx(LPCTSTR sResourceType, LPCTSTR sResource)
{
Initialize();
if (Load(sResourceType, sResource))
{
nativeImage = NULL;
lastResult = DllExports::GdipLoadImageFromStreamICM(m_pStream, &nativeImage);
TestForAnimatedGIF();
}
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: ImageEx::ImageEx
//
// DESCRIPTION: Constructor for constructing images from a file
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
ImageEx::ImageEx(const WCHAR* filename, BOOL useEmbeddedColorManagement) : Image(filename, useEmbeddedColorManagement)
{
Initialize();
m_bIsInitialized = true;
TestForAnimatedGIF();
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: ImageEx::~ImageEx
//
// DESCRIPTION: Free up fresources
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
ImageEx::~ImageEx()
{
Destroy();
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: InitAnimation
//
// DESCRIPTION: Prepare animated GIF
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
bool ImageEx::InitAnimation(HWND hWnd, CPoint pt)
{
m_hWnd = hWnd;
m_pt = pt;
if (!m_bIsInitialized)
{
TRACE(_T("GIF not initialized\n"));
return false;
};
if (IsAnimatedGIF())
{
if (m_hThread == NULL)
{
unsigned int nTID = 0;
m_hThread = (HANDLE) _beginthreadex( NULL, 0, _ThreadAnimationProc, this, CREATE_SUSPENDED,&nTID);
if (!m_hThread)
{
TRACE(_T("Couldn't start a GIF animation thread\n"));
return true;
}
else
ResumeThread(m_hThread);
}
}
return false;
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: LoadFromBuffer
//
// DESCRIPTION: Helper function to copy phyical memory from buffer a IStream
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
bool ImageEx::LoadFromBuffer(BYTE* pBuff, int nSize)
{
bool bResult = false;
HGLOBAL hGlobal = GlobalAlloc(GMEM_MOVEABLE, nSize);
if (hGlobal)
{
void* pData = GlobalLock(hGlobal);
if (pData)
memcpy(pData, pBuff, nSize);
GlobalUnlock(hGlobal);
if (CreateStreamOnHGlobal(hGlobal, TRUE, &m_pStream) == S_OK)
bResult = true;
}
return bResult;
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: GetResource
//
// DESCRIPTION: Helper function to lock down resource
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
bool ImageEx::GetResource(LPCTSTR lpName, LPCTSTR lpType, void* pResource, int& nBufSize)
{
HRSRC hResInfo;
HANDLE hRes;
LPSTR lpRes = NULL;
int nLen = 0;
bool bResult = FALSE;
// Find the resource
hResInfo = FindResource(m_hInst , lpName, lpType);
if (hResInfo == NULL)
{
DWORD dwErr = GetLastError();
return false;
}
// Load the resource
hRes = LoadResource(m_hInst , hResInfo);
if (hRes == NULL)
return false;
// Lock the resource
lpRes = (char*)LockResource(hRes);
if (lpRes != NULL)
{
if (pResource == NULL)
{
nBufSize = SizeofResource(m_hInst , hResInfo);
bResult = true;
}
else
{
if (nBufSize >= (int)SizeofResource(m_hInst , hResInfo))
{
memcpy(pResource, lpRes, nBufSize);
bResult = true;
}
}
UnlockResource(hRes);
}
// Free the resource
FreeResource(hRes);
return bResult;
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: Load
//
// DESCRIPTION: Helper function to load resource from memory
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
bool ImageEx::Load(CString sResourceType, CString sResource)
{
bool bResult = false;
BYTE* pBuff = NULL;
int nSize = 0;
if (GetResource(sResource.GetBuffer(0), sResourceType.GetBuffer(0), pBuff, nSize))
{
if (nSize > 0)
{
pBuff = new BYTE[nSize];
if (GetResource(sResource, sResourceType.GetBuffer(0), pBuff, nSize))
{
if (LoadFromBuffer(pBuff, nSize))
{
bResult = true;
}
}
delete [] pBuff;
}
}
m_bIsInitialized = bResult;
return bResult;
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: GetSize
//
// DESCRIPTION: Returns Width and Height object
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
CSize ImageEx::GetSize()
{
return CSize(GetWidth(), GetHeight());
}
////////////////////////////////////////////////////////////////////////////////
//
// FUNCTION: TestForAnimatedGIF
//
// DESCRIPTION: Check GIF/Image for avialability of animation
//
// RETURNS:
//
// NOTES:
//
// MODIFICATIONS:
//
// Name Date Version Comments
// N T ALMOND 29012002 1.0 Origin
//
////////////////////////////////////////////////////////////////////////////////
bool ImageEx::TestForAnimatedGIF()
{
UINT count = 0;
count = GetFrameDimensionsCount();
GUID* pDimensionIDs = new GUID[count];
// Get the list of frame dimensions from the Image object.
GetFrameDimensionsList(pDimensionIDs, count);
// Get the number of frames in the first dimension.
m_nFrameCount = GetFrameCount(&pDimensionIDs[0]);
// Assume that the image has a property item of type PropertyItemEquipMake.
// Get the size of that property item.
int nSize = GetPropertyItemSize(PropertyTagFrameDelay);
// Allocate a buffer to receive the property item.
m_pPropertyItem = (PropertyItem*) malloc(nSize);
GetPropertyItem(PropertyTagFrameDelay, nSize, m_pPropertyItem);
delete pDimensionIDs;
re

钱亚锋
- 粉丝: 107
- 资源: 1万+
最新资源
- 基于java+springboot+vue+mysql的粮仓管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的美发管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的美术馆管理系统设计与实现.docx
- 2023保健品行业洞察报告
- 基于java+springboot+vue+mysql的民宿管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的培训机构管理系统设计与实现.docx
- 基于java+springboot+vue+mysql的民航网上订票系统设计与实现.docx
- 基于java+springboot+vue+mysql的入校申报审批系统设计与实现.docx
- 基于java+springboot+vue+mysql的汽车租赁系统设计与实现.docx
- 基于java+springboot+vue+mysql的商业辅助决策系统设计与实现.docx
- 基于java+springboot+vue+mysql的水产养殖系统设计与实现.docx
- 基于java+springboot+vue+mysql的社区网格化管理平台设计与实现.docx
- 基于java+springboot+vue+mysql的社区医疗服务系统设计与实现.docx
- 基于java+springboot+vue+mysql的玩具租赁系统设计与实现.docx
- 基于java+springboot+vue+mysql的闲置图书分享平台设计与实现.docx
- 基于java+springboot+vue+mysql的西安旅游系统设计与实现.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


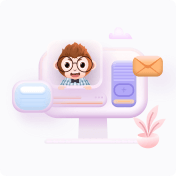