/*
(c) Copyright 2001-2009 The world wide DirectFB Open Source Community (directfb.org)
(c) Copyright 2000-2004 Convergence (integrated media) GmbH
All rights reserved.
Written by Denis Oliver Kropp <dok@directfb.org>,
Andreas Hundt <andi@fischlustig.de>,
Sven Neumann <neo@directfb.org>,
Ville Syrjälä <syrjala@sci.fi> and
Claudio Ciccani <klan@users.sf.net>.
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the
Free Software Foundation, Inc., 59 Temple Place - Suite 330,
Boston, MA 02111-1307, USA.
*/
#include <config.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <dfb_types.h>
#include <pthread.h>
#include <directfb.h>
#include <core/core.h>
#include <core/coredefs.h>
#include <core/coretypes.h>
#include <core/gfxcard.h>
#include <core/state.h>
#include <core/palette.h>
#include <misc/gfx_util.h>
#include <misc/util.h>
#include <misc/conf.h>
#include <direct/clock.h>
#include <direct/mem.h>
#include <direct/memcpy.h>
#include <direct/messages.h>
#include <direct/util.h>
#include <gfx/convert.h>
#include <gfx/util.h>
#include "generic.h"
#include "duffs_device.h"
/* lookup tables for 2/3bit to 8bit color conversion */
static const u8 lookup3to8[] = { 0x00, 0x24, 0x49, 0x6d, 0x92, 0xb6, 0xdb, 0xff};
static const u8 lookup2to8[] = { 0x00, 0x55, 0xaa, 0xff};
#define EXPAND_1to8(v) ((v) ? 0xff : 0x00)
#define EXPAND_2to8(v) (lookup2to8[v])
#define EXPAND_3to8(v) (lookup3to8[v])
#define EXPAND_4to8(v) (((v) << 4) | ((v) ))
#define EXPAND_5to8(v) (((v) << 3) | ((v) >> 2))
#define EXPAND_6to8(v) (((v) << 2) | ((v) >> 4))
#define EXPAND_7to8(v) (((v) << 1) | ((v) >> 6))
static int use_mmx = 0;
#ifdef USE_MMX
static void gInit_MMX( void );
#endif
#if SIZEOF_LONG == 8
static void gInit_64bit( void );
#endif
#ifdef WORDS_BIGENDIAN
static void gInit_BigEndian();
#endif
/* RGB16 */
#define RGB_MASK 0xffff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_16
#define Bop_PFI_OP_Aop_PFI( op ) Bop_16_##op##_Aop
#include "template_colorkey_16.h"
/* ARGB1555 / RGB555 / BGR555 */
#define RGB_MASK 0x7fff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_15
#define Bop_PFI_OP_Aop_PFI( op ) Bop_15_##op##_Aop
#include "template_colorkey_16.h"
/* ARGB2554 */
#define RGB_MASK 0x3fff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_14
#define Bop_PFI_OP_Aop_PFI( op ) Bop_14_##op##_Aop
#include "template_colorkey_16.h"
/* ARGB4444 / RGB444*/
#define RGB_MASK 0x0fff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_12
#define Bop_PFI_OP_Aop_PFI( op ) Bop_12_##op##_Aop
#include "template_colorkey_16.h"
/* RGBA4444 */
#define RGB_MASK 0xfff0
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_12vv
#define Bop_PFI_OP_Aop_PFI( op ) Bop_12vv_##op##_Aop
#include "template_colorkey_16.h"
/* RGB24/VYU */
#define RGB_MASK 0xffffff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_24_24
#define Bop_PFI_OP_Aop_PFI( op ) Bop_24_24_##op##_Aop
#include "template_colorkey_24.h"
/* ARGB8565 */
#define RGB_MASK 0x00ffff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_24_16
#define Bop_PFI_OP_Aop_PFI( op ) Bop_24_16_##op##_Aop
#include "template_colorkey_24.h"
/* ARGB1666/ARGB6666/RGB18 */
#define RGB_MASK 0x03ffff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_24_18
#define Bop_PFI_OP_Aop_PFI( op ) Bop_24_18_##op##_Aop
#include "template_colorkey_24.h"
/* ARGB/RGB32/AiRGB */
#define RGB_MASK 0x00ffffff
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_32
#define Bop_PFI_OP_Aop_PFI( op ) Bop_32_##op##_Aop
#include "template_colorkey_32.h"
/* RGBAF88871 */
#define RGB_MASK 0xffffff00
#define Cop_OP_Aop_PFI( op ) Cop_##op##_Aop_32_24
#define Bop_PFI_OP_Aop_PFI( op ) Bop_32_24_##op##_Aop
#include "template_colorkey_32.h"
/* RGB16 */
#define EXPAND_Ato8( a ) 0xFF
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_6to8( g )
#define EXPAND_Bto8( b ) EXPAND_5to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_RGB16( r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_rgb16_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_rgb16
#define A_SHIFT 0
#define R_SHIFT 11
#define G_SHIFT 5
#define B_SHIFT 0
#define A_MASK 0
#define R_MASK 0xf800
#define G_MASK 0x07e0
#define B_MASK 0x001f
#include "template_acc_16.h"
/* ARGB1555 */
#define EXPAND_Ato8( a ) EXPAND_1to8( a )
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_5to8( g )
#define EXPAND_Bto8( b ) EXPAND_5to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_ARGB1555( a, r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_argb1555_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_argb1555
#define A_SHIFT 15
#define R_SHIFT 10
#define G_SHIFT 5
#define B_SHIFT 0
#define A_MASK 0x8000
#define R_MASK 0x7c00
#define G_MASK 0x03e0
#define B_MASK 0x001f
#include "template_acc_16.h"
/* RGBA5551 */
#define EXPAND_Ato8( a ) EXPAND_1to8( a )
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_5to8( g )
#define EXPAND_Bto8( b ) EXPAND_5to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_RGBA5551( a, r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_rgba5551_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_rgba5551
#define A_SHIFT 0
#define R_SHIFT 11
#define G_SHIFT 6
#define B_SHIFT 1
#define A_MASK 0x0001
#define R_MASK 0xf800
#define G_MASK 0x07c0
#define B_MASK 0x003e
#include "template_acc_16.h"
/* RGB555 */
#define EXPAND_Ato8( a ) 0xFF
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_5to8( g )
#define EXPAND_Bto8( b ) EXPAND_5to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_RGB555( r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_xrgb1555_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_xrgb1555
#define A_SHIFT 0
#define R_SHIFT 10
#define G_SHIFT 5
#define B_SHIFT 0
#define A_MASK 0
#define R_MASK 0x7c00
#define G_MASK 0x03e0
#define B_MASK 0x001f
#include "template_acc_16.h"
/* BGR555 */
#define EXPAND_Ato8( a ) 0xFF
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_5to8( g )
#define EXPAND_Bto8( b ) EXPAND_5to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_BGR555( r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_xbgr1555_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_xbgr1555
#define A_SHIFT 0
#define B_SHIFT 10
#define G_SHIFT 5
#define R_SHIFT 0
#define A_MASK 0
#define B_MASK 0x7c00
#define G_MASK 0x03e0
#define R_MASK 0x001f
#include "template_acc_16.h"
/* ARGB2554 */
#define EXPAND_Ato8( a ) EXPAND_2to8( a )
#define EXPAND_Rto8( r ) EXPAND_5to8( r )
#define EXPAND_Gto8( g ) EXPAND_5to8( g )
#define EXPAND_Bto8( b ) EXPAND_4to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_ARGB2554( a, r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_argb2554_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_argb2554
#define A_SHIFT 14
#define R_SHIFT 9
#define G_SHIFT 4
#define B_SHIFT 0
#define A_MASK 0xc000
#define R_MASK 0x3e00
#define G_MASK 0x01f0
#define B_MASK 0x000f
#include "template_acc_16.h"
/* ARGB4444 */
#define EXPAND_Ato8( a ) EXPAND_4to8( a )
#define EXPAND_Rto8( r ) EXPAND_4to8( r )
#define EXPAND_Gto8( g ) EXPAND_4to8( g )
#define EXPAND_Bto8( b ) EXPAND_4to8( b )
#define PIXEL_OUT( a, r, g, b ) PIXEL_ARGB4444( a, r, g, b )
#define Sop_PFI_OP_Dacc( op ) Sop_argb4444_##op##_Dacc
#define Sacc_OP_Aop_PFI( op ) Sacc_##op##_Aop_argb4444
#define A_SHIFT 12
#define R_SHIFT 8
#define G_SHIFT 4
#define B_SHIFT 0
#define A_MASK 0xf000
#define R_MASK
没有合适的资源?快使用搜索试试~ 我知道了~
DirectFB-1.6.0.tar.gz_directfb
1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
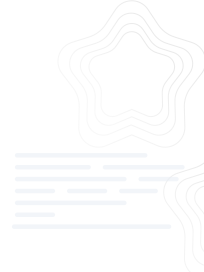
共1256个文件
c:484个
h:430个
in:125个

新版的Direct FB程序,面向对象的Linux图形库。用最小的资源进行图形加速,写屏很快。
资源推荐
资源详情
资源评论
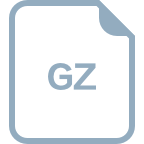
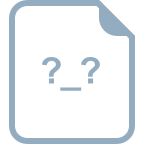
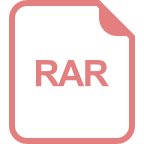
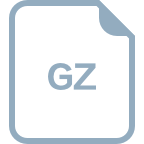
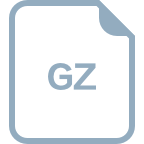
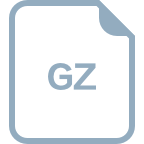
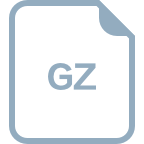
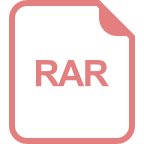
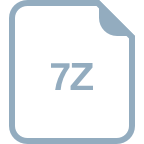
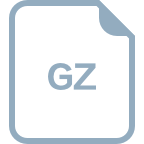
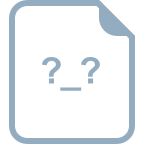
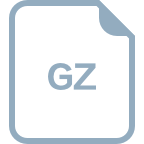
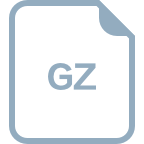
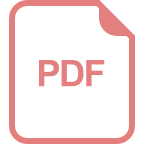
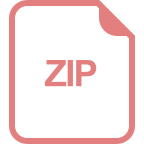
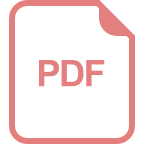
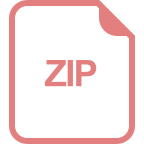
收起资源包目录

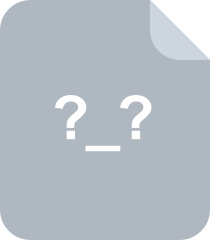
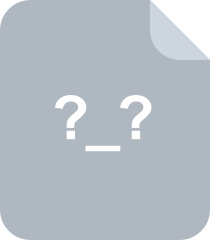
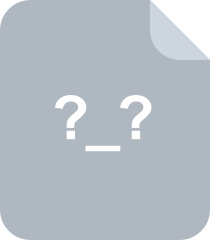
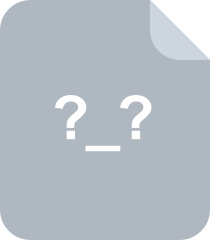
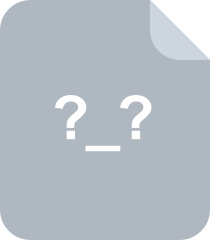
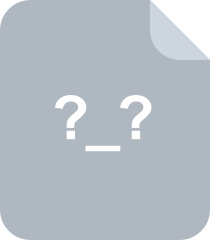
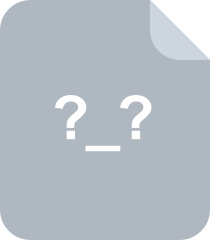
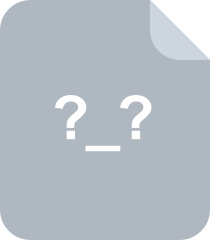
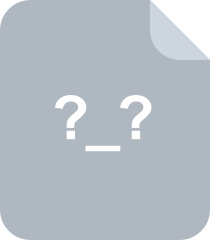
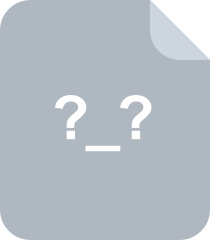
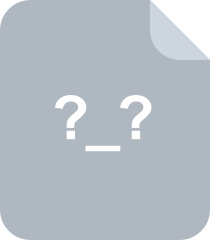
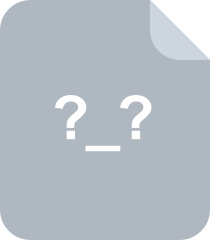
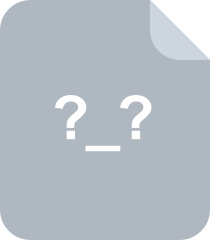
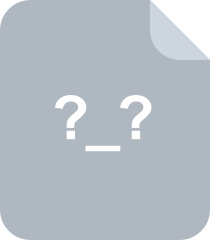
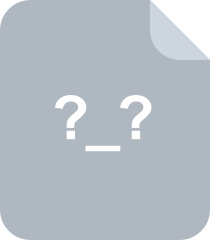
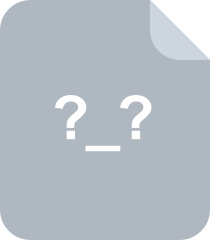
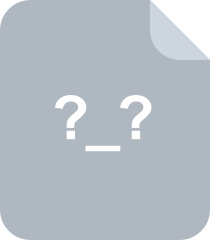
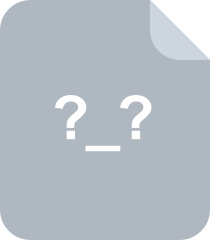
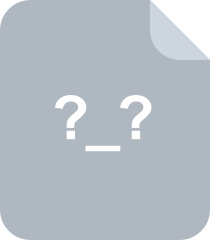
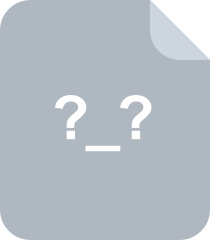
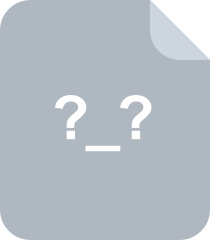
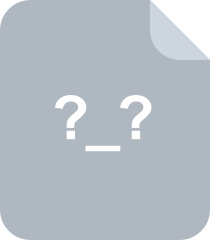
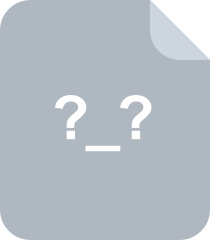
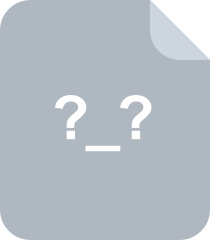
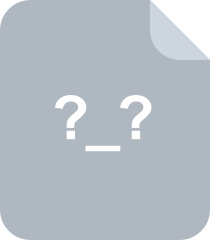
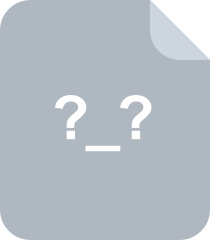
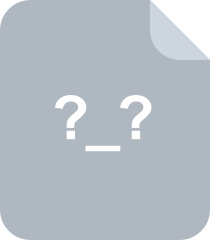
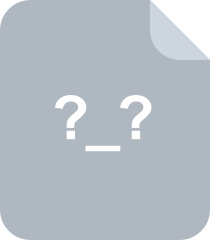
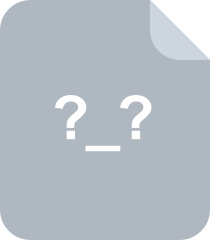
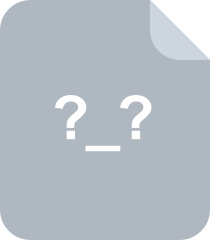
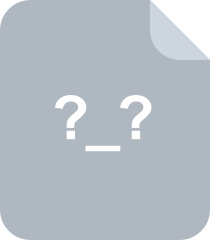
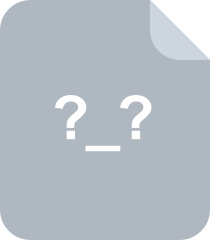
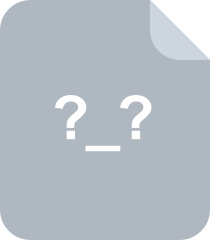
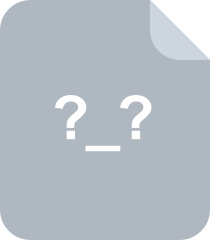
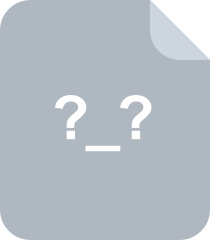
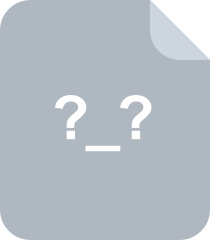
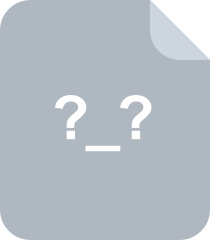
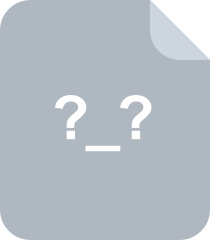
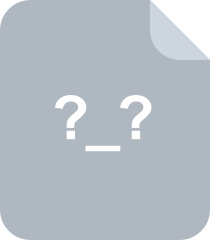
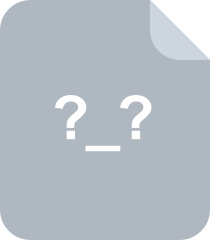
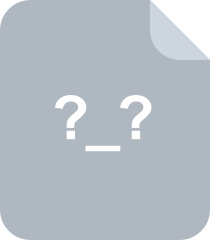
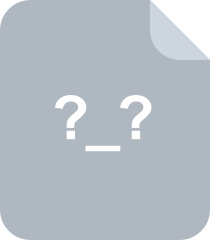
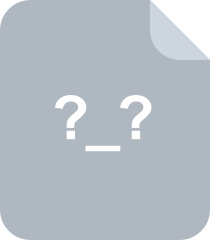
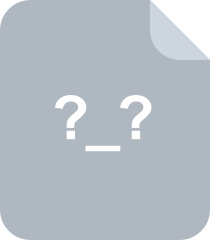
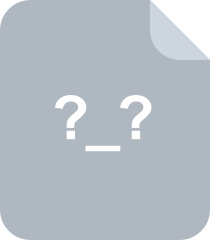
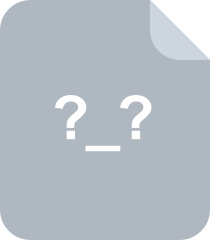
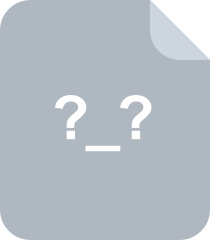
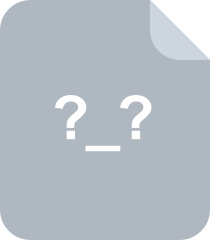
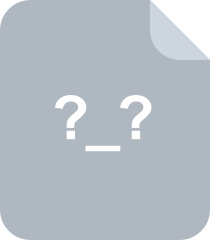
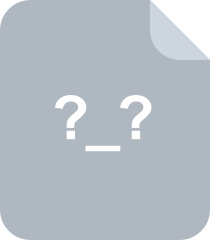
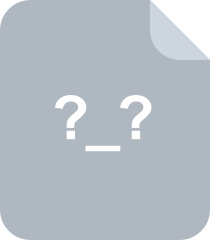
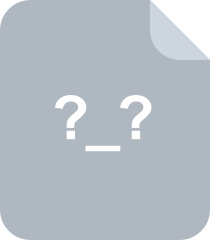
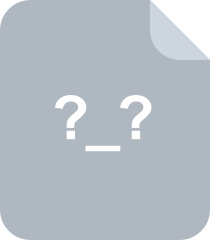
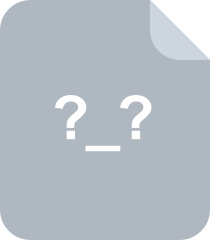
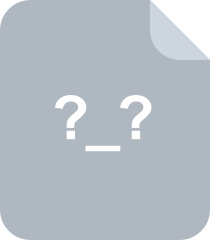
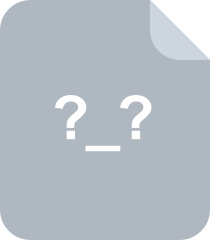
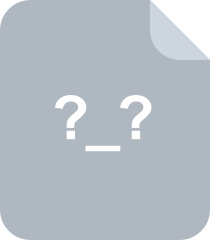
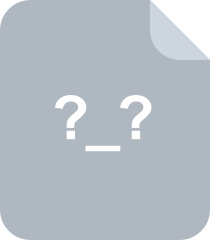
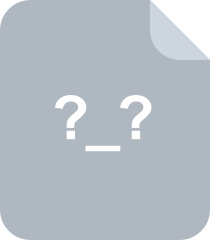
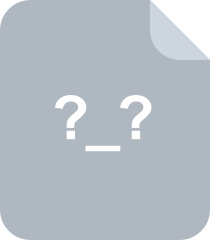
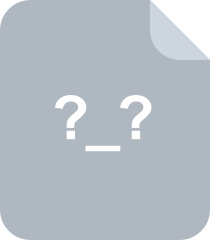
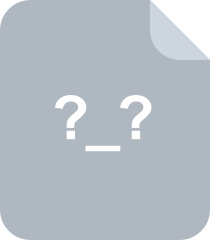
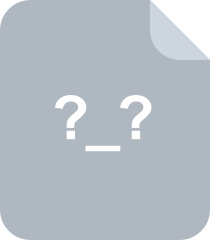
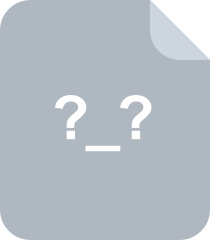
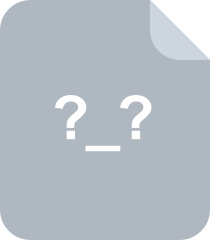
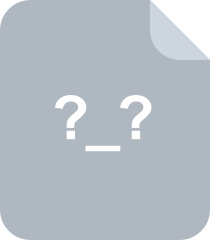
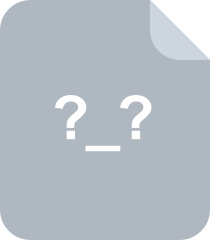
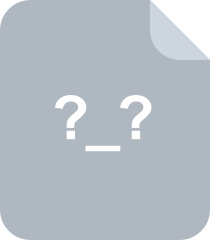
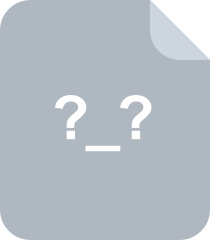
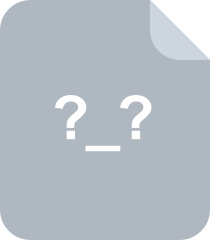
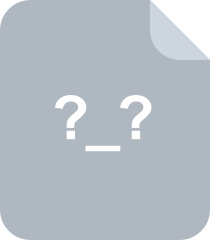
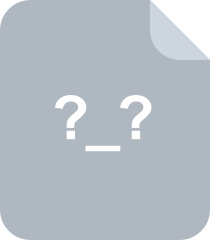
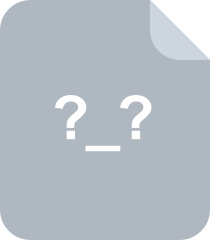
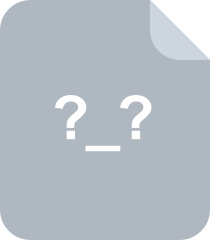
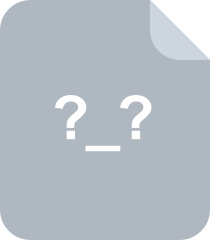
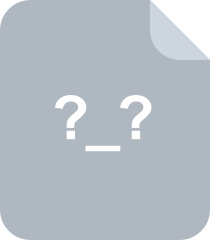
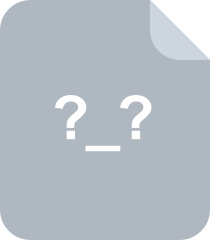
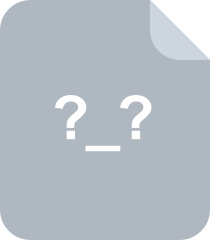
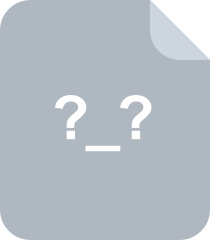
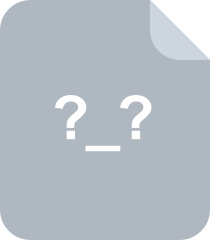
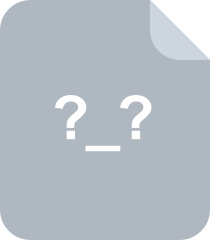
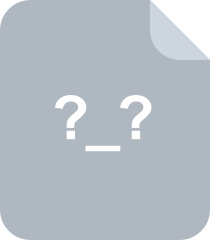
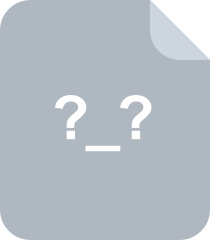
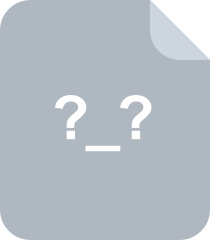
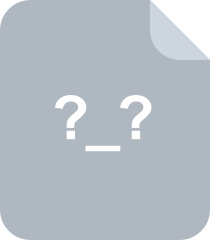
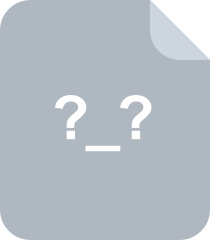
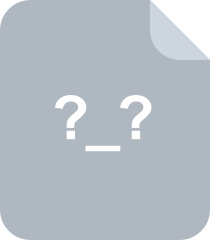
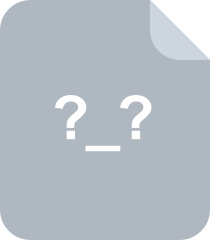
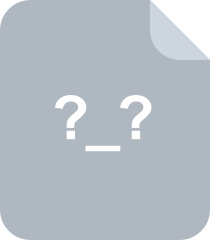
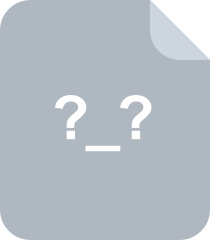
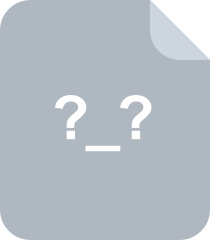
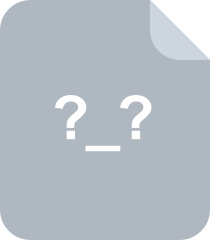
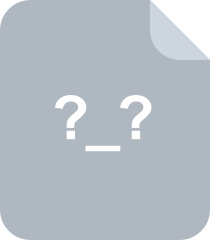
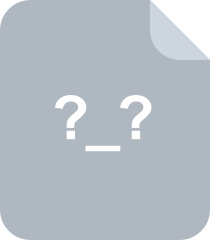
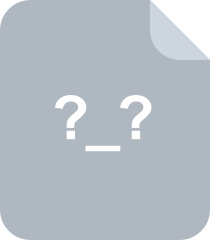
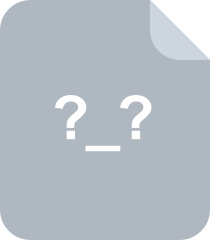
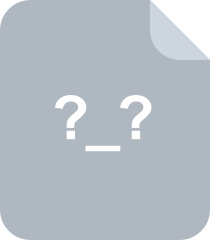
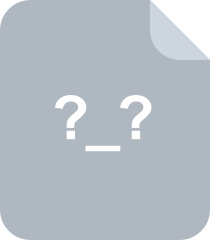
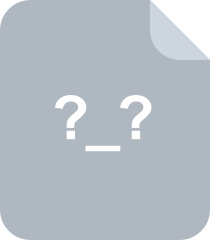
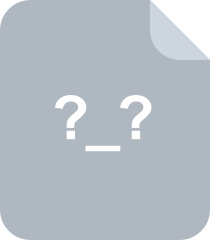
共 1256 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
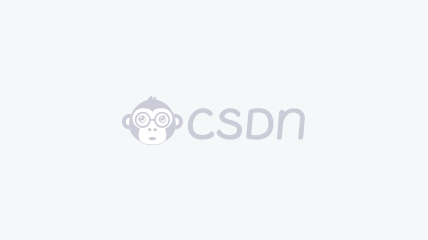
- qqlmx202023-12-08资源有一定的参考价值,与资源描述一致,很实用,能够借鉴的部分挺多的,值得下载。

钱亚锋
- 粉丝: 87
- 资源: 1万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

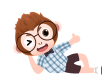
安全验证
文档复制为VIP权益,开通VIP直接复制
