/******************************************************************************
USB Host Driver Local Header
This file provides local definitions used by the hardware interface for a USB
Host application.
* File Name: usb_host_local.h
* Dependencies: None
* Processor: PIC24/dsPIC30/dsPIC33/PIC32MX
* Compiler: C30 v2.01/C32 v0.00.18
* Company: Microchip Technology, Inc.
Software License Agreement
The software supplied herewith by Microchip Technology Incorporated
(the Company) for its PICmicro® Microcontroller is intended and
supplied to you, the Companys customer, for use solely and
exclusively on Microchip PICmicro Microcontroller products. The
software is owned by the Company and/or its supplier, and is
protected under applicable copyright laws. All rights are reserved.
Any use in violation of the foregoing restrictions may subject the
user to criminal sanctions under applicable laws, as well as to
civil liability for the breach of the terms and conditions of this
license.
THIS SOFTWARE IS PROVIDED IN AN AS IS CONDITION. NO WARRANTIES,
WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING, BUT NOT LIMITED
TO, IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A
PARTICULAR PURPOSE APPLY TO THIS SOFTWARE. THE COMPANY SHALL NOT,
IN ANY CIRCUMSTANCES, BE LIABLE FOR SPECIAL, INCIDENTAL OR
CONSEQUENTIAL DAMAGES, FOR ANY REASON WHATSOEVER.
Change History:
Rev Description
---------- ----------------------------------------------------------
2.6a Removed extraneous definition
2.7 No change
2.7a Removed freez() macro
*******************************************************************************/
#ifndef _USB_HOST_LOCAL_
#define _USB_HOST_LOCAL_
#include "usb_hal_local.h"
// *****************************************************************************
// *****************************************************************************
// Section: Constants
//
// These constants are internal to the stack. All constants required by the
// API are in the header file(s).
// *****************************************************************************
// *****************************************************************************
// *****************************************************************************
// Section: State Machine Constants
// *****************************************************************************
#define STATE_MASK 0x0F00 //
#define SUBSTATE_MASK 0x00F0 //
#define SUBSUBSTATE_MASK 0x000F //
#define NEXT_STATE 0x0100 //
#define NEXT_SUBSTATE 0x0010 //
#define NEXT_SUBSUBSTATE 0x0001 //
#define SUBSUBSTATE_ERROR 0x000F //
#define NO_STATE 0xFFFF //
/*
*******************************************************************************
DETACHED state machine values
This state machine handles the condition when no device is attached.
*/
#define STATE_DETACHED 0x0000 //
#define SUBSTATE_INITIALIZE 0x0000 //
#define SUBSTATE_WAIT_FOR_POWER 0x0010 //
#define SUBSTATE_TURN_ON_POWER 0x0020 //
#define SUBSTATE_WAIT_FOR_DEVICE 0x0030 //
/*
*******************************************************************************
ATTACHED state machine values
This state machine gets the device descriptor of the remote device. We get the
size of the device descriptor, and use that size to get the entire device
descriptor. Then we check the VID and PID and make sure they appear in the TPL.
*/
#define STATE_ATTACHED 0x0100 //
#define SUBSTATE_SETTLE 0x0000 //
#define SUBSUBSTATE_START_SETTLING_DELAY 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_SETTLING 0x0001 //
#define SUBSUBSTATE_SETTLING_DONE 0x0002 //
#define SUBSTATE_RESET_DEVICE 0x0010 //
#define SUBSUBSTATE_SET_RESET 0x0000 //
#define SUBSUBSTATE_RESET_WAIT 0x0001 //
#define SUBSUBSTATE_RESET_RECOVERY 0x0002 //
#define SUBSUBSTATE_RECOVERY_WAIT 0x0003 //
#define SUBSUBSTATE_RESET_COMPLETE 0x0004 //
#define SUBSTATE_GET_DEVICE_DESCRIPTOR_SIZE 0x0020 //
#define SUBSUBSTATE_SEND_GET_DEVICE_DESCRIPTOR_SIZE 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_GET_DEVICE_DESCRIPTOR_SIZE 0x0001 //
#define SUBSUBSTATE_GET_DEVICE_DESCRIPTOR_SIZE_COMPLETE 0x0002 //
#define SUBSTATE_GET_DEVICE_DESCRIPTOR 0x0030 //
#define SUBSUBSTATE_SEND_GET_DEVICE_DESCRIPTOR 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_GET_DEVICE_DESCRIPTOR 0x0001 //
#define SUBSUBSTATE_GET_DEVICE_DESCRIPTOR_COMPLETE 0x0002 //
#define SUBSTATE_VALIDATE_VID_PID 0x0040 //
/*
*******************************************************************************
ADDRESSING state machine values
This state machine sets the address of the remote device.
*/
#define STATE_ADDRESSING 0x0200 //
#define SUBSTATE_SET_DEVICE_ADDRESS 0x0000 //
#define SUBSUBSTATE_SEND_SET_DEVICE_ADDRESS 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_SET_DEVICE_ADDRESS 0x0001 //
#define SUBSUBSTATE_SET_DEVICE_ADDRESS_COMPLETE 0x0002 //
/*
*******************************************************************************
CONFIGURING state machine values
This state machine sets the configuration of the remote device, and sets up
internal variables to support the device.
*/
#define STATE_CONFIGURING 0x0300 //
#define SUBSTATE_INIT_CONFIGURATION 0x0000 //
#define SUBSTATE_GET_CONFIG_DESCRIPTOR_SIZE 0x0010 //
#define SUBSUBSTATE_SEND_GET_CONFIG_DESCRIPTOR_SIZE 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_GET_CONFIG_DESCRIPTOR_SIZE 0x0001 //
#define SUBSUBSTATE_GET_CONFIG_DESCRIPTOR_SIZECOMPLETE 0x0002 //
#define SUBSTATE_GET_CONFIG_DESCRIPTOR 0x0020 //
#define SUBSUBSTATE_SEND_GET_CONFIG_DESCRIPTOR 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_GET_CONFIG_DESCRIPTOR 0x0001 //
#define SUBSUBSTATE_GET_CONFIG_DESCRIPTOR_COMPLETE 0x0002 //
#define SUBSTATE_SELECT_CONFIGURATION 0x0030 //
#define SUBSUBSTATE_SELECT_CONFIGURATION 0x0000 //
#define SUBSUBSTATE_SEND_SET_OTG 0x0001 //
#define SUBSUBSTATE_WAIT_FOR_SET_OTG_DONE 0x0002 //
#define SUBSUBSTATE_SET_OTG_COMPLETE 0x0003 //
#define SUBSTATE_SET_CONFIGURATION 0x0040 //
#define SUBSUBSTATE_SEND_SET_CONFIGURATION 0x0000 //
#define SUBSUBSTATE_WAIT_FOR_SET_CONFIGURATION 0x0001 //
#define SUBSUBSTATE_SET_CONFIGURATION_COMPLETE 0x0002 //
#define SUBSUBSTATE_INIT_CLIENT_DRIVERS 0x0003 //
/*
*******************************************************************************
RUNNING state machine values
*/
#define STATE_RUNNING 0x0400 //
#define SUBSTATE_NORMAL_RUN 0x0000 //
#define SUBSTATE_SUSPEND_AND_RESUME 0x0010 //
#define SUBSUBSTATE_SUSPEND 0x0000 //
#define SUBSUBSTATE_RESUME 0x0001 //
#define SUBSUBSTATE_RESUME_WAIT 0x0002 //
没有合适的资源?快使用搜索试试~ 我知道了~
usb_host_local.zip_keil uvision_purpose_usb
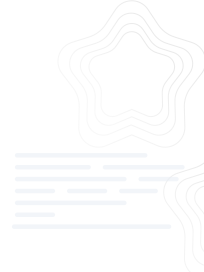
共1个文件
h:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 44 浏览量
2022-09-21
21:26:59
上传
评论
收藏 7KB ZIP 举报
温馨提示
keil uVision 3 with serial key by Sanan Ali,keil uVision 3 cracked, IDE compiler for microcontroller, keil by Sanan Ali. ... And keil uVision is the best software for this purpose. keil uVision with serial key.
资源推荐
资源详情
资源评论
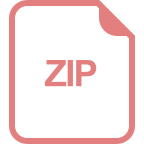
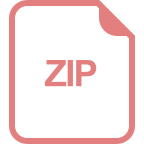
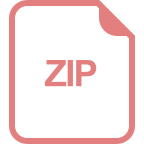
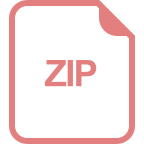
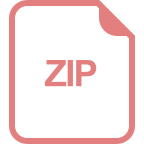
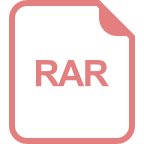
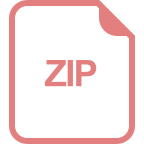
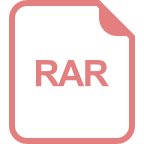
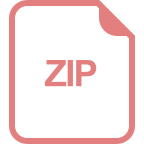
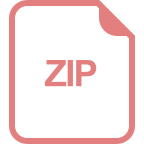
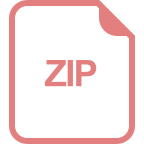
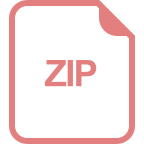
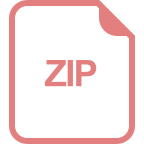
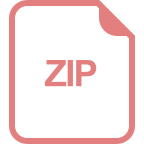
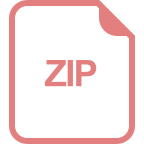
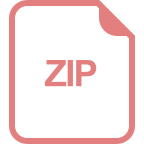
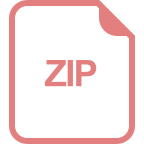
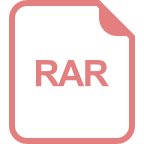
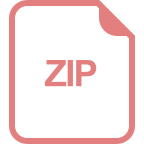
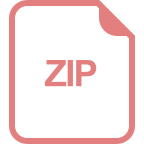
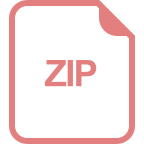
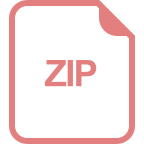
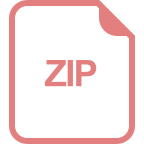
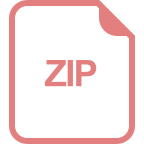
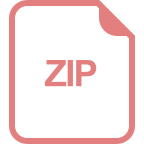
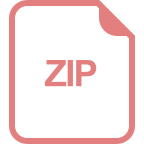
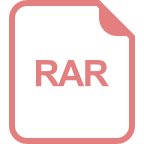
收起资源包目录

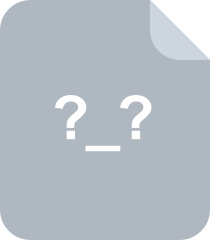
共 1 条
- 1
资源评论
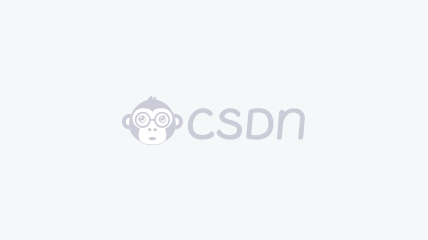

APei
- 粉丝: 83
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

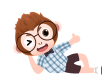
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


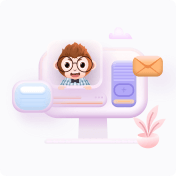
安全验证
文档复制为VIP权益,开通VIP直接复制
