#include <gtk/gtk.h>
#include "clearlooks_style.h"
#include "clearlooks_rc_style.h"
#include "clearlooks_draw.h"
#include <math.h>
#include <string.h>
#include "bits.c"
#include "support.h"
//#include "config.h"
/* #define DEBUG 1 */
#define SCALE_SIZE 5
#define DETAIL(xx) ((detail) && (!strcmp(xx, detail)))
#define COMPARE_COLORS(a,b) (a.red == b.red && a.green == b.green && a.blue == b.blue)
#define DRAW_ARGS GtkStyle *style, \
GdkWindow *window, \
GtkStateType state_type, \
GtkShadowType shadow_type, \
GdkRectangle *area, \
GtkWidget *widget, \
const gchar *detail, \
gint x, \
gint y, \
gint width, \
gint height
static GdkGC *realize_color (GtkStyle * style, GdkColor * color);
static GtkStyleClass *parent_class;
static GList *progressbars = NULL;
static gint8 pboffset = 10;
static int timer_id = 0;
static void cl_progressbar_remove (gpointer data)
{
if (g_list_find (progressbars, data) == NULL)
return;
progressbars = g_list_remove (progressbars, data);
g_object_unref (data);
if (g_list_first(progressbars) == NULL) {
g_source_remove(timer_id);
timer_id = 0;
}
}
static void update_progressbar (gpointer data, gpointer user_data)
{
gfloat fraction;
if (data == NULL)
return;
fraction = gtk_progress_bar_get_fraction (GTK_PROGRESS_BAR (data));
/* update only if not filled */
if (fraction < 1.0)
gtk_widget_queue_resize ((GtkWidget*)data);
if (fraction >= 1.0 || GTK_PROGRESS (data)->activity_mode)
cl_progressbar_remove (data);
}
static gboolean timer_func (gpointer data)
{
g_list_foreach (progressbars, update_progressbar, NULL);
if (--pboffset < 0) pboffset = 9;
return (g_list_first(progressbars) != NULL);
}
static gboolean cl_progressbar_known(gconstpointer data)
{
return (g_list_find (progressbars, data) != NULL);
}
static void cl_progressbar_add (gpointer data)
{
if (!GTK_IS_PROGRESS_BAR (data))
return;
progressbars = g_list_append (progressbars, data);
g_object_ref (data);
g_signal_connect ((GObject*)data, "unrealize", G_CALLBACK (cl_progressbar_remove), data);
if (timer_id == 0)
timer_id = g_timeout_add (100, timer_func, NULL);
}
static GdkColor *
clearlooks_get_spot_color (ClearlooksRcStyle *clearlooks_rc)
{
GtkRcStyle *rc = GTK_RC_STYLE (clearlooks_rc);
if (clearlooks_rc->has_spot_color)
return &clearlooks_rc->spot_color;
else
return &rc->base[GTK_STATE_SELECTED];
}
/**************************************************************************/
/* used for optionmenus... */
static void
draw_tab (GtkStyle *style,
GdkWindow *window,
GtkStateType state_type,
GtkShadowType shadow_type,
GdkRectangle *area,
GtkWidget *widget,
const gchar *detail,
gint x,
gint y,
gint width,
gint height)
{
#define ARROW_SPACE 2
#define ARROW_LINE_HEIGHT 2
#define ARROW_LINE_WIDTH 5
ClearlooksStyle *clearlooks_style = CLEARLOOKS_STYLE (style);
GtkRequisition indicator_size;
GtkBorder indicator_spacing;
gint arrow_height;
option_menu_get_props (widget, &indicator_size, &indicator_spacing);
indicator_size.width += (indicator_size.width % 2) - 1;
arrow_height = indicator_size.width / 2 + 2;
x += (width - indicator_size.width) / 2;
y += height/2;
if (state_type == GTK_STATE_INSENSITIVE)
{
draw_arrow (window, style->light_gc[state_type], area,
GTK_ARROW_UP, 1+x, 1+y-arrow_height,
indicator_size.width, arrow_height);
draw_arrow (window, style->light_gc[state_type], area,
GTK_ARROW_DOWN, 1+x, 1+y+1,
indicator_size.width, arrow_height);
}
draw_arrow (window, style->fg_gc[state_type], area,
GTK_ARROW_UP, x, y-arrow_height,
indicator_size.width, arrow_height);
draw_arrow (window, style->fg_gc[state_type], area,
GTK_ARROW_DOWN, x, y+1,
indicator_size.width, arrow_height);
}
static void
clearlooks_draw_arrow (GtkStyle *style,
GdkWindow *window,
GtkStateType state,
GtkShadowType shadow,
GdkRectangle *area,
GtkWidget *widget,
const gchar *detail,
GtkArrowType arrow_type,
gboolean fill,
gint x,
gint y,
gint width,
gint height)
{
ClearlooksStyle *clearlooks_style = CLEARLOOKS_STYLE (style);
gint original_width, original_x;
GdkGC *gc;
sanitize_size (window, &width, &height);
if (is_combo_box (widget))
{
width = 7;
height = 5;
x+=2;
y+=4;
if (state == GTK_STATE_INSENSITIVE)
{
draw_arrow (window, style->light_gc[state], area,
GTK_ARROW_UP, 1+x, 1+y-height,
width, height);
draw_arrow (window, style->light_gc[state], area,
GTK_ARROW_DOWN, 1+x, 1+y+1,
width, height);
}
draw_arrow (window, style->fg_gc[state], area,
GTK_ARROW_UP, x, y-height,
width, height);
draw_arrow (window, style->fg_gc[state], area,
GTK_ARROW_DOWN, x, y+1,
width, height);
return;
}
original_width = width;
original_x = x;
/* Make spinbutton arrows and arrows in menus
* slightly larger to get the right pixels drawn */
if (DETAIL ("spinbutton"))
height += 1;
if (DETAIL("menuitem"))
{
width = 6;
height = 7;
}
/* Compensate arrow position for "sunken" look */
if (DETAIL ("spinbutton") && arrow_type == GTK_ARROW_DOWN &&
style->xthickness > 2 && style->ythickness > 2)
y -= 1;
if (widget && widget->parent && GTK_IS_COMBO (widget->parent->parent))
{
width -= 2;
height -=2;
x++;
}
calculate_arrow_geometry (arrow_type, &x, &y, &width, &height);
if (DETAIL ("menuitem"))
x = original_x + original_width - width;
if (DETAIL ("spinbutton") && (arrow_type == GTK_ARROW_DOWN))
y += 1;
if (state == GTK_STATE_INSENSITIVE)
draw_arrow (window, style->light_gc[state], area, arrow_type, x + 1, y + 1, width, height);
gc = style->fg_gc[state];
draw_arrow (window, gc, area, arrow_type, x, y, width, height);
}
static void
draw_flat_box (DRAW_ARGS)
{
ClearlooksStyle *clearlooks_style = CLEARLOOKS_STYLE (style);
g_return_if_fail (GTK_IS_STYLE (style));
g_return_if_fail (window != NULL);
sanitize_size (window, &width, &height);
if (detail &&
clearlooks_style->listviewitemstyle == 1 &&
state_type == GTK_STATE_SELECTED && (
!strncmp ("cell_even", detail, strlen ("cell_even")) ||
!strncmp ("cell_odd", detail, strlen ("cell_odd"))))
{
GdkGC *gc;
GdkColor lower_color;
GdkColor *upper_color;
if (GTK_WIDGET_HAS_FOCUS (widget))
{
gc = style->base_gc[state_type];
upper_color = &style->base[state_type];
}
else
{
gc = style->base_gc[GTK_STATE_ACTIVE];
upper_color = &style->base[GTK_STATE_ACTIVE];
}
if (GTK_IS_TREE_VIEW (widget) && 0)
{
GtkTreeSelection *sel = gtk_tree_view_get_selection (GTK_TREE_VIEW (widget));
if (gtk_tree_selection_count_selected_rows (sel) > 1)
{
parent_class->draw_flat_box (style, window, state_type, shadow_type,
area, widget, detail,
x, y, width, height);
return;
}
}
shade (upper_color, &lower_color, 0.8);
if (area)
gdk_gc_set_clip_rectangle (gc, area);
draw_hgradient (window, gc, style,
x, y, width, height, upper_color, &lower_color);
if (area)
gdk_gc_set_clip_rectangle (gc, NULL);
}
else
{
parent_class->draw_flat_b
没有合适的资源?快使用搜索试试~ 我知道了~
ardour-2.4.1.rar_Ardo_Ardou_Ardour_ardour audio_混音
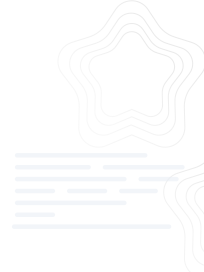
共2000个文件
h:1020个
cc:620个
c:85个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 8 浏览量
2022-09-14
15:11:03
上传
评论
收藏 4.99MB RAR 举报
温馨提示
音频编辑工作站,可以编辑midi等音频文件,进行裁减、混音等操作
资源详情
资源评论
资源推荐
收起资源包目录

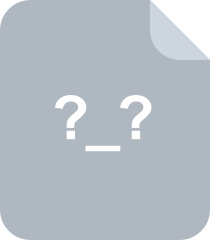
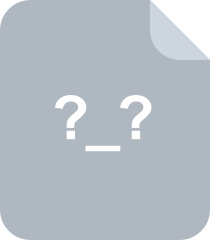
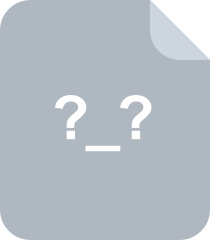
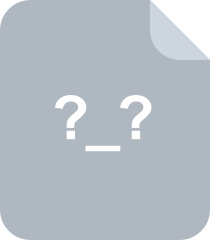
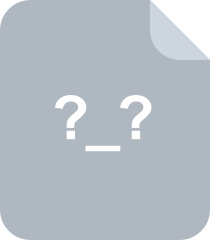
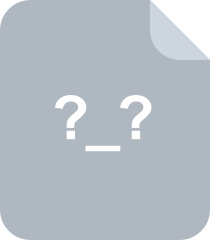
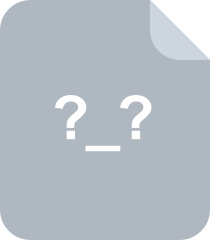
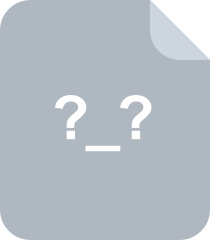
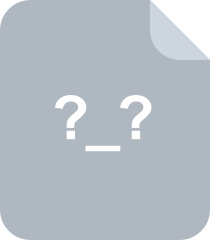
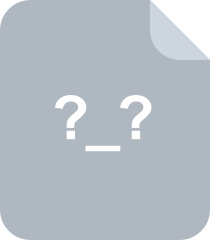
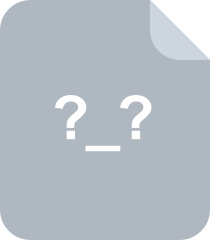
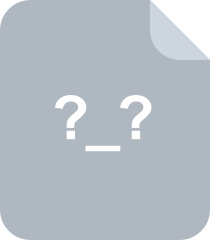
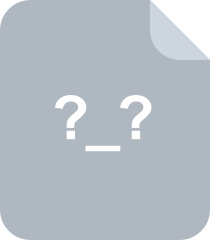
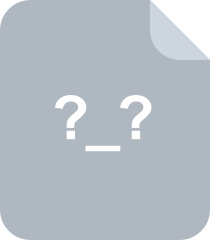
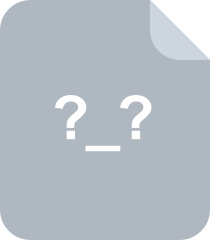
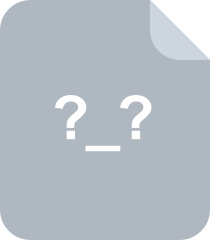
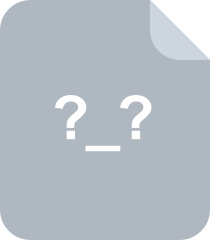
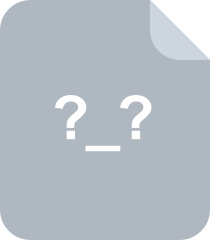
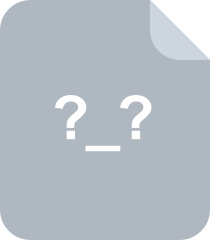
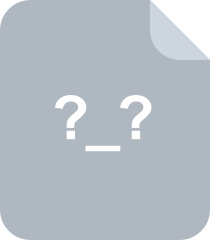
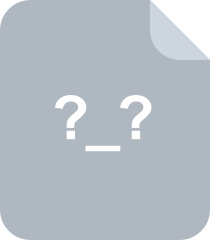
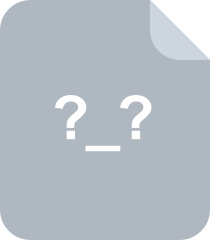
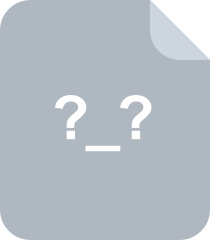
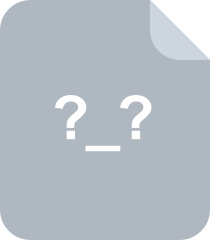
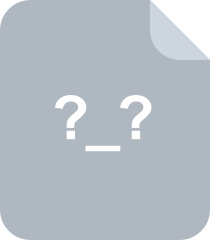
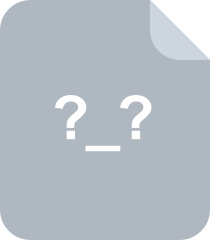
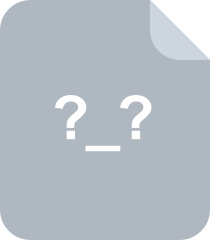
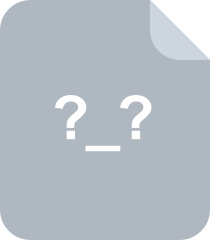
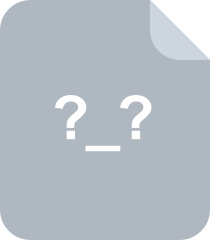
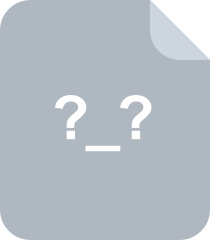
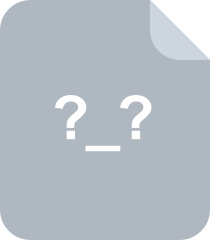
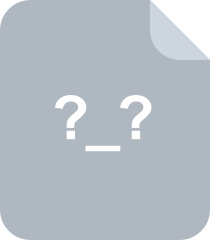
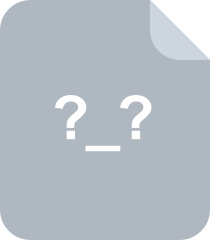
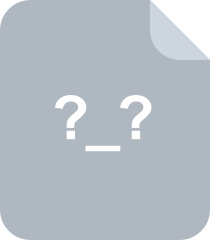
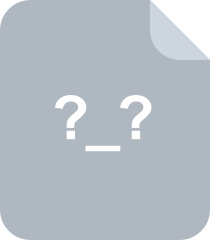
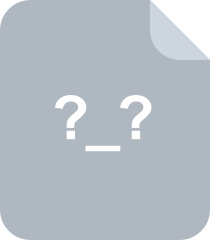
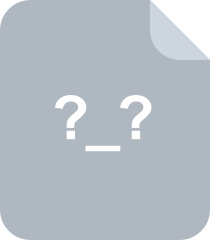
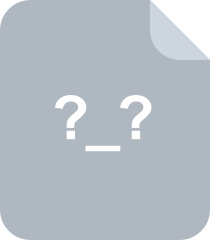
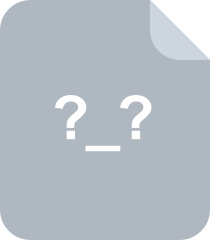
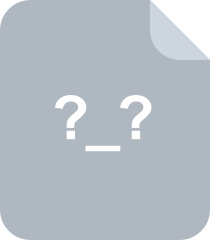
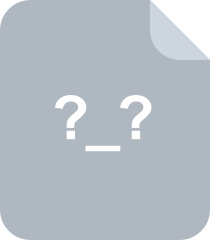
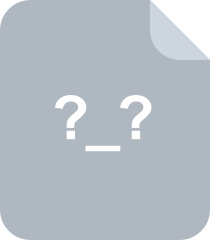
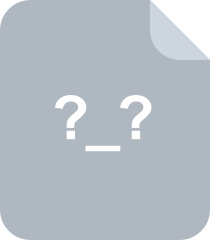
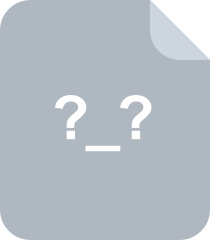
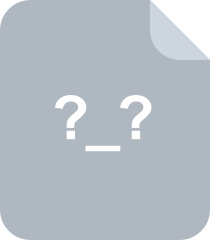
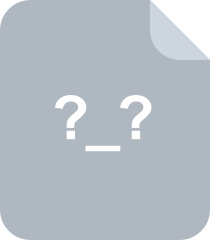
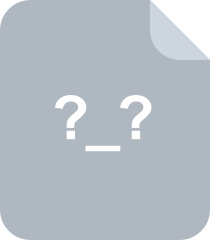
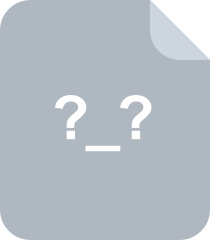
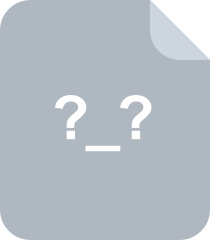
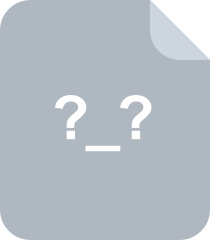
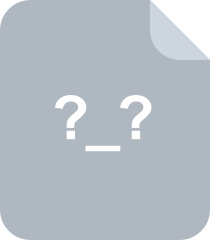
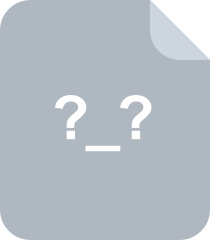
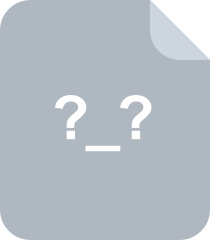
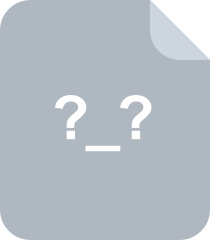
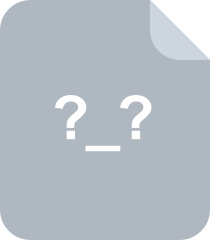
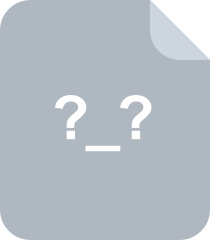
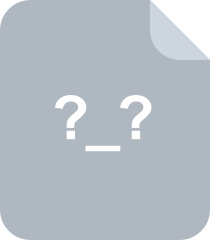
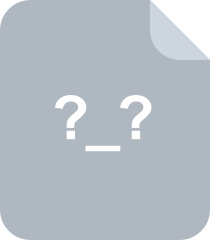
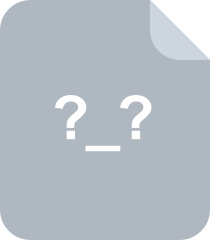
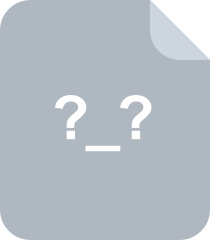
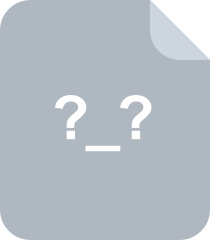
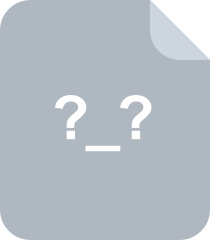
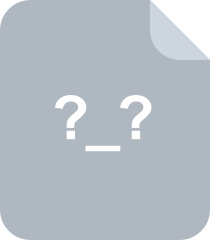
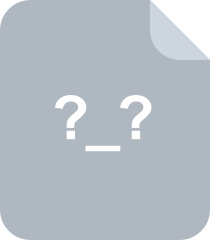
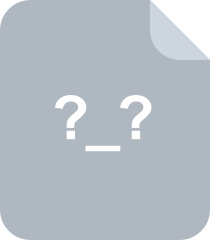
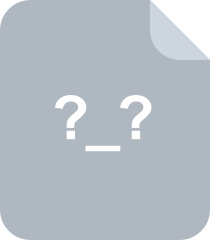
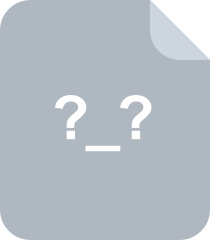
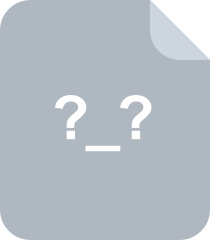
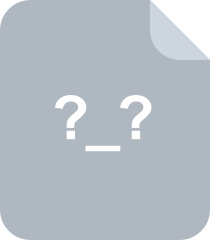
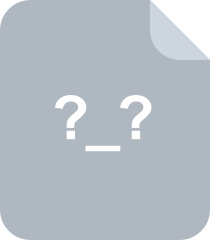
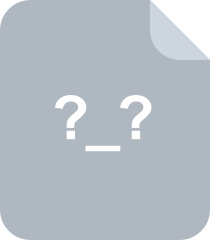
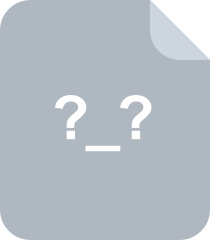
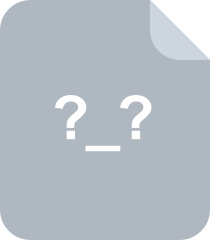
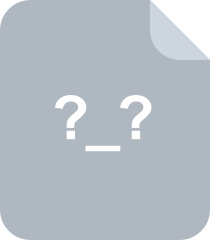
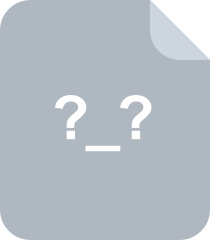
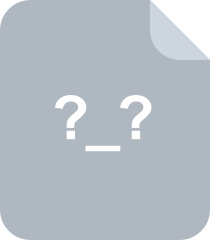
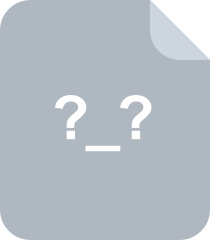
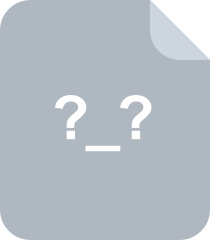
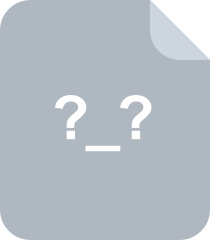
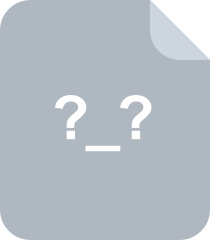
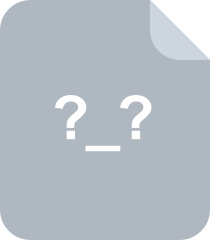
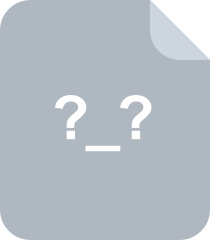
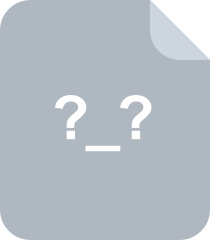
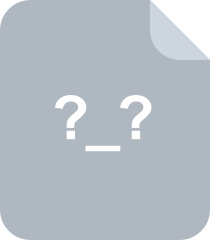
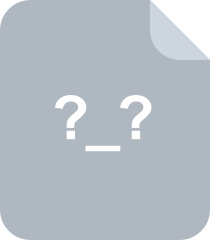
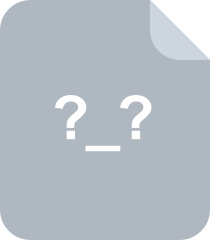
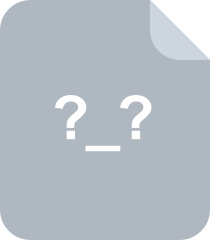
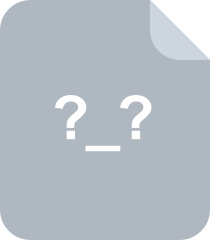
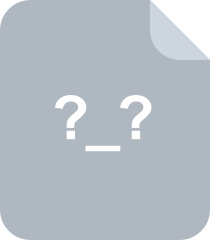
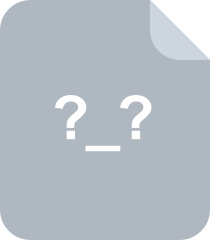
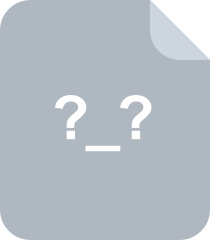
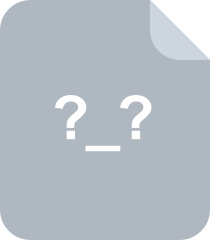
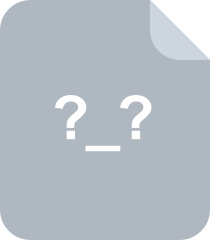
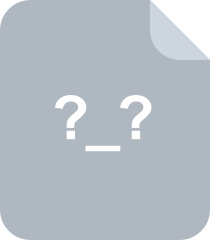
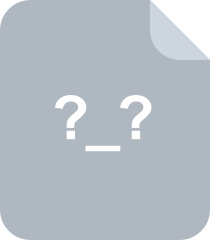
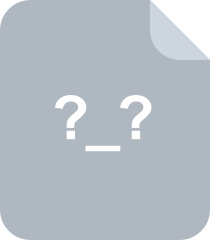
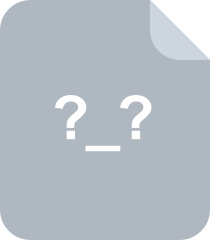
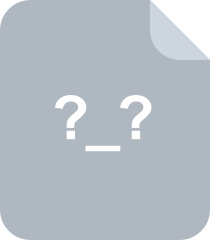
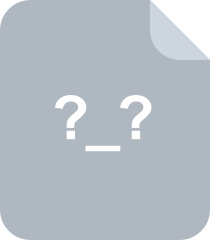
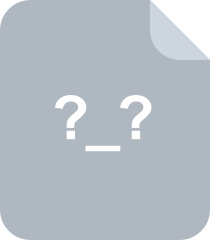
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
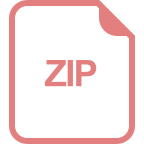
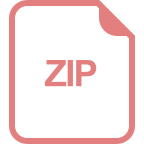
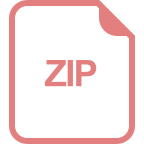
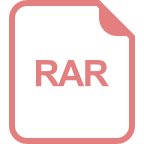
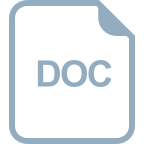
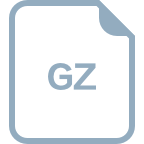
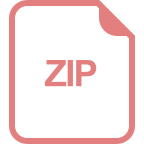
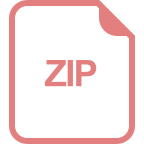
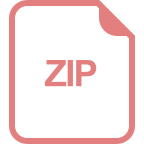
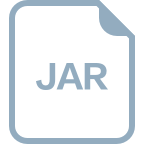
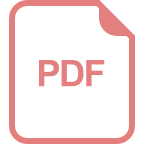
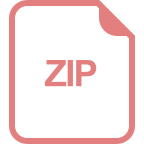
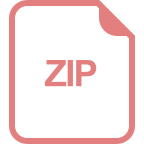
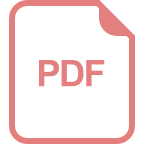

APei
- 粉丝: 65
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

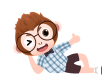
最新资源
- 订单管理系统的示例代码.rar
- python语言+PyQt5实现日历(高分课程设计)
- 计算机视觉技术(CV)详解.pdf
- 圣诞节销售预测和趋势分析 使用历史销售数据和市场趋势预测特定产品或类别的销售量
- 网络通信技术-考试-B卷
- 识别图像中的文字的简单例子.rar
- 计算机网络网络层(Network Layer)**:负责数据在网络中的传输和路由选择,处理逻辑地址和路由表,实现数据包的转发与路
- 计算机网络物理层(Physical Layer)负责传输比特流,定义物理接口和传输介质特性(如电缆、光纤),确保数据的可靠传输
- YOLOv8关键点pose训练自己的数据集教程
- 计算机网络局域网(LAN)、城域网(MAN)、广域网(WAN)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


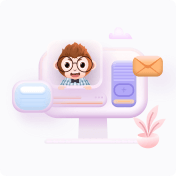
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0