// MainFrm.cpp : implementation of the CMainFrame class
//
#include "stdafx.h"
#include "DbTest.h"
#include "DataSet.h"
#include "MainFrm.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CMainFrame
IMPLEMENT_DYNAMIC(CMainFrame, CFrameWnd)
BEGIN_MESSAGE_MAP(CMainFrame, CFrameWnd)
//{{AFX_MSG_MAP(CMainFrame)
ON_WM_CREATE()
ON_WM_SETFOCUS()
ON_COMMAND(ID_TEST_RECORDSET, OnTestRecordset)
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
static UINT indicators[] =
{
ID_SEPARATOR, // status line indicator
ID_INDICATOR_CAPS,
ID_INDICATOR_NUM,
ID_INDICATOR_SCRL,
};
/////////////////////////////////////////////////////////////////////////////
// CMainFrame construction/destruction
CMainFrame::CMainFrame()
{
// TODO: add member initialization code here
}
CMainFrame::~CMainFrame()
{
}
int CMainFrame::OnCreate(LPCREATESTRUCT lpCreateStruct)
{
if (CFrameWnd::OnCreate(lpCreateStruct) == -1)
return -1;
// create a view to occupy the client area of the frame
if (!m_wndView.Create(NULL, NULL, AFX_WS_DEFAULT_VIEW,
CRect(0, 0, 0, 0), this, AFX_IDW_PANE_FIRST, NULL))
{
TRACE0("Failed to create view window\n");
return -1;
}
if (!m_wndStatusBar.Create(this) ||
!m_wndStatusBar.SetIndicators(indicators,
sizeof(indicators)/sizeof(UINT)))
{
TRACE0("Failed to create status bar\n");
return -1; // fail to create
}
return 0;
}
BOOL CMainFrame::PreCreateWindow(CREATESTRUCT& cs)
{
if( !CFrameWnd::PreCreateWindow(cs) )
return FALSE;
// TODO: Modify the Window class or styles here by modifying
// the CREATESTRUCT cs
cs.dwExStyle &= ~WS_EX_CLIENTEDGE;
cs.lpszClass = AfxRegisterWndClass(0);
return TRUE;
}
/////////////////////////////////////////////////////////////////////////////
// CMainFrame diagnostics
#ifdef _DEBUG
void CMainFrame::AssertValid() const
{
CFrameWnd::AssertValid();
}
void CMainFrame::Dump(CDumpContext& dc) const
{
CFrameWnd::Dump(dc);
}
#endif //_DEBUG
/////////////////////////////////////////////////////////////////////////////
// CMainFrame message handlers
void CMainFrame::OnSetFocus(CWnd* pOldWnd)
{
// forward focus to the view window
m_wndView.SetFocus();
}
BOOL CMainFrame::OnCmdMsg(UINT nID, int nCode, void* pExtra, AFX_CMDHANDLERINFO* pHandlerInfo)
{
// let the view have first crack at the command
if (m_wndView.OnCmdMsg(nID, nCode, pExtra, pHandlerInfo))
return TRUE;
// otherwise, do default handling
return CFrameWnd::OnCmdMsg(nID, nCode, pExtra, pHandlerInfo);
}
struct DataObj {
CString Var1;
CString Var2;
int Var3;
float Var4;
// Etc.
// Member functions
};
void CMainFrame::OnTestRecordset()
{
CDatabase db;
CArray<DataObj, DataObj> A;
TRY {
db.Open("TEST");
// Create recordset object
CDataSet<DataObj, 4> MySet("Table1", &db);
// Set default filter to var1
MySet.m_DefaultFilter = "Var1 = '%s'";
MySet.Open();
// Load all the records
MySet.LoadAll(A);
// Find some record
if ( MySet.Search("Anything") )
{
DataObj B;
// Load, update and store new recoed
MySet.Load(B);
B.Var1 = "Anything else?";
MySet.Store(B);
}
MySet.Close();
AfxMessageBox("Data is loaded!");
}
CATCH_ALL(e) {
e->ReportError();
}
END_CATCH_ALL
}
void CDataSet<DataObj, 4>::DoFieldExchange(CFieldExchange* pFX)
{
pFX->SetFieldType(CFieldExchange::outputColumn);
RFX_Text(pFX, "VAR1", m_Data.Var1);
RFX_Text(pFX, "VAR2", m_Data.Var2);
RFX_Int(pFX, "VAR3", m_Data.Var3);
RFX_Single(pFX, "VAR4", m_Data.Var4);
}
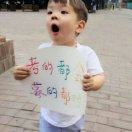
局外狗
- 粉丝: 80
- 资源: 1万+
最新资源
- 基于51单片机LCD1602显示的一个万年历+可调时钟(含原理图)
- Day-06 Vue222222222
- OREO:离线增强型大型语言模型多步推理优化方法
- iClient3D for Cesium 加载shp数据并拉伸为白模
- flowable-demo-master
- 大模型的稀疏激活方法及其高效推理应用研究:基于dReLU激活函数
- au2024_113102-1.zip
- 大规模语言模型在不同NLP任务中的提示工程技术综述
- 廖鹏盛 - 时代进行曲.zip
- 土地利用/土地覆盖数据(蚌埠市)
- Matlab实现VMD-TCN-BiLSTM变分模态分解结合时间卷积双向长短期记忆神经网络多变量光伏功率时间序列预测(含完整的程序,GUI设计和代码详解)
- LLM-Select: Feature Selection with Large Language Models
- Matlab实现RP-LSTM-Attention递归图优化长短期记忆神经网络注意力机制的数据分类预测(含完整的程序,GUI设计和代码详解)
- Another Redis Desktop软件
- 鲸鱼优化算法(WOA)文章复现:《改进鲸鱼优化算法在机械臂时间最优轨迹规划的应用-赵晶》 策略为:Tent混沌初始化种群+非线性权重改进位置更新+非线性概率转-IWOA 复现内容包
- 页岩油四性潜力层判识工具
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


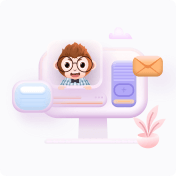