package com.shxt.lesson25.rcp.views;
import java.util.ArrayList;
import org.eclipse.jface.action.IMenuManager;
import org.eclipse.jface.action.IToolBarManager;
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Composite;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
import org.eclipse.ui.part.ViewPart;
import com.shxt.lesson25.rcp.tools.JDBCTools;
import org.eclipse.swt.widgets.Text;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Label;
public class MainView extends ViewPart {
public static final String ID = "com.shxt.lesson25.rcp.views.MainView"; //$NON-NLS-1$
private Table table;
private Text txtCard;
private Text txtPassword;
private Text txtMoney;
private Text txtUser;
private Label lblPage = null;
private int nowPage = 1;
public MainView() {
}
//返回查询到的记录数
private int find() {
//查询记录
JDBCTools tool = new JDBCTools();
String sql = "select * from atm";
ArrayList<String[]> rsList = tool.find(sql, nowPage);
for (int i = 0; i < rsList.size(); i++) {
String[] rows = rsList.get(i);
TableItem tableItem = new TableItem(table, SWT.NONE);
tableItem.setText(rows);
}
return rsList.size();
}
/**
* Create contents of the view part.
* @param parent
*/
@Override
public void createPartControl(Composite parent) {
Composite container = new Composite(parent, SWT.NONE);
table = new Table(container, SWT.BORDER | SWT.FULL_SELECTION);
table.setBounds(10, 10, 421, 242);
table.setHeaderVisible(true);
table.setLinesVisible(true);
TableColumn tblclmnCard = new TableColumn(table, SWT.NONE);
tblclmnCard.setWidth(100);
tblclmnCard.setText("card");
TableColumn tblclmnPassword = new TableColumn(table, SWT.NONE);
tblclmnPassword.setWidth(100);
tblclmnPassword.setText("password");
TableColumn tblclmnMoney = new TableColumn(table, SWT.NONE);
tblclmnMoney.setWidth(100);
tblclmnMoney.setText("money");
TableColumn tblclmnUser = new TableColumn(table, SWT.NONE);
tblclmnUser.setWidth(100);
tblclmnUser.setText("user");
//先查询一次
find();
//添加功能
txtCard = new Text(container, SWT.BORDER);
txtCard.setBounds(500, 10, 73, 23);
txtPassword = new Text(container, SWT.BORDER);
txtPassword.setBounds(500, 49, 73, 23);
txtMoney = new Text(container, SWT.BORDER);
txtMoney.setBounds(500, 91, 73, 23);
txtUser = new Text(container, SWT.BORDER);
txtUser.setBounds(500, 135, 73, 23);
Button btnSave = new Button(container, SWT.NONE);
btnSave.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
//添加到 RCP table
//添加到 数据库中的 table atm
JDBCTools tool = new JDBCTools();
String sql = "insert into atm values ('" + txtCard.getText()
+ "', '" + txtPassword.getText()
+ "', '" + txtMoney.getText()
+ "', '" + txtUser.getText() + "')";
int rows = tool.update(sql);
if (rows > 0) {
TableItem ti = new TableItem(table, SWT.NONE);
ti.setText(new String[]{
txtCard.getText(),
txtPassword.getText(),
txtMoney.getText(),
txtUser.getText()});
} else {
System.out.println("错误");
}
}
});
btnSave.setBounds(500, 179, 73, 27);
btnSave.setText("save");
Button btnPageup = new Button(container, SWT.NONE);
btnPageup.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
if (nowPage > 1) {
nowPage --;
table.removeAll(); //table中原来的数据清空
find();
}
lblPage.setText("" + nowPage);
}
});
btnPageup.setBounds(10, 258, 80, 27);
btnPageup.setText("pageUp");
Button btnPagedown = new Button(container, SWT.NONE);
btnPagedown.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
nowPage ++;
table.removeAll(); //table中原来的数据清空
int result = find();
if (result == 0) {
nowPage--;
find();
}
lblPage.setText("" + nowPage);
}
});
btnPagedown.setBounds(111, 258, 80, 27);
btnPagedown.setText("pageDown");
lblPage= new Label(container, SWT.NONE);
lblPage.setBounds(221, 268, 61, 17);
lblPage.setText("" + nowPage);
createActions();
initializeToolBar();
initializeMenu();
}
/**
* Create the actions.
*/
private void createActions() {
// Create the actions
}
/**
* Initialize the toolbar.
*/
private void initializeToolBar() {
IToolBarManager toolbarManager = getViewSite().getActionBars()
.getToolBarManager();
}
/**
* Initialize the menu.
*/
private void initializeMenu() {
IMenuManager menuManager = getViewSite().getActionBars()
.getMenuManager();
}
@Override
public void setFocus() {
// Set the focus
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
sql-and-rcp-table.zip_Table_rcp
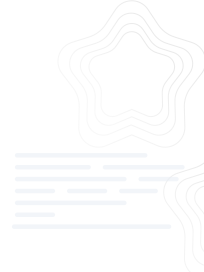
共33个文件
class:12个
java:8个
gif:3个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 86 浏览量
2022-09-24
05:00:18
上传
评论
收藏 541KB ZIP 举报
温馨提示
实现数据库与RCP表格的连接,把数据库上的内容映射到表格中
资源详情
资源评论
资源推荐
收起资源包目录








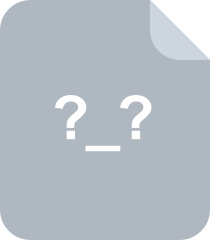

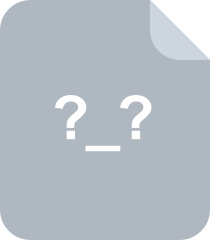
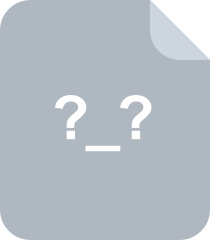
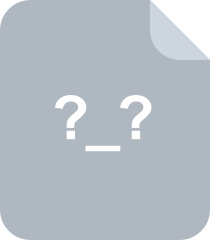
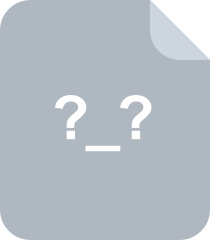

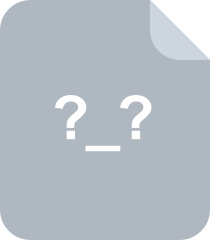
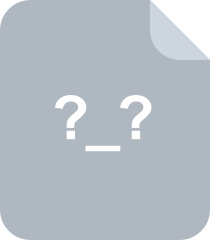
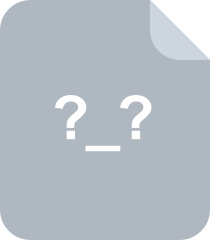
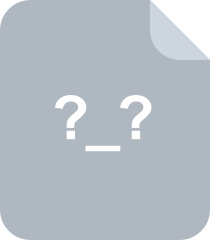
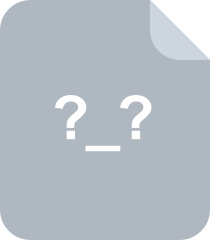
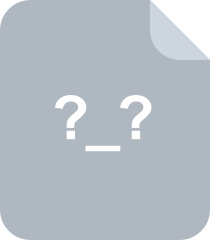
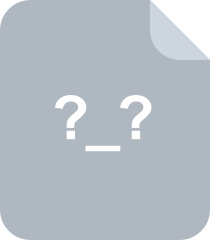

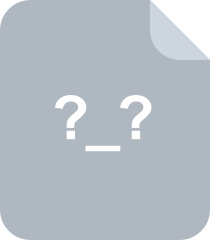

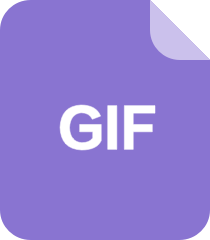
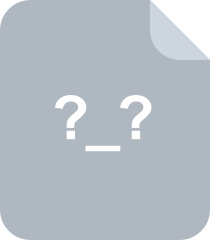
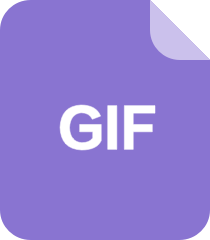
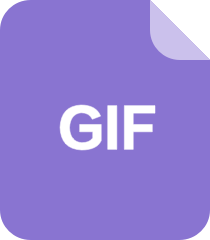
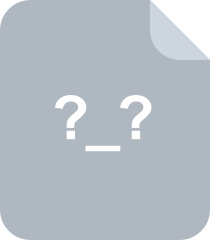
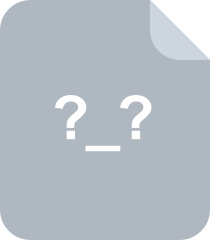

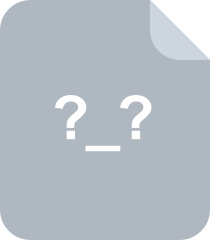






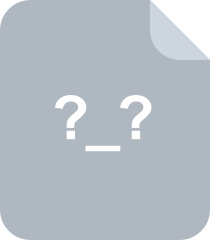

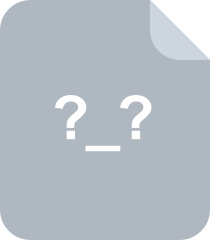

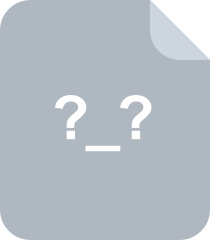
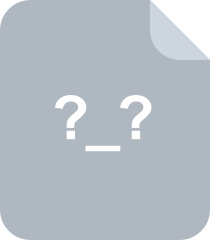
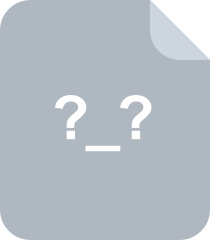
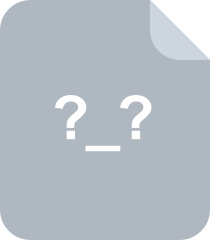
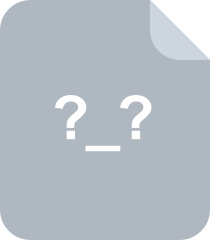
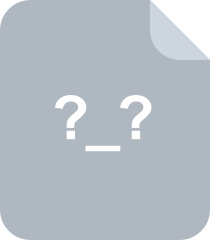
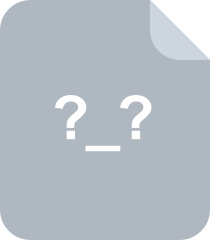
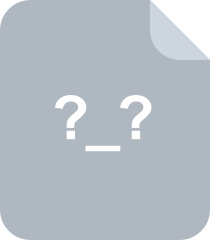
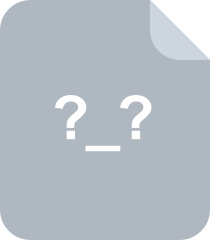
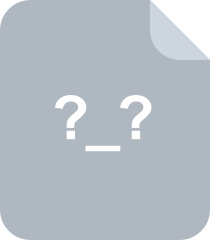

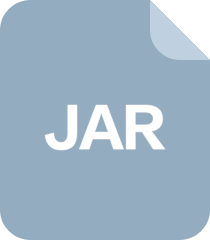
共 33 条
- 1
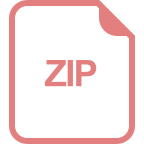
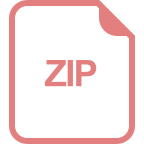
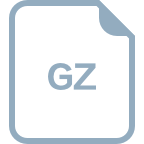
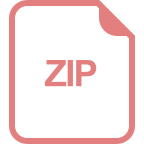
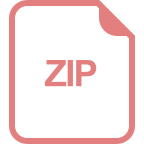
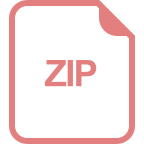
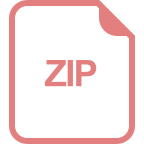
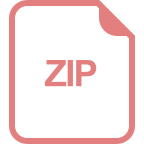
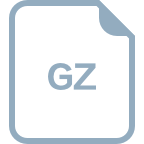
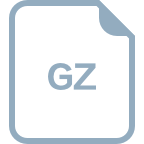
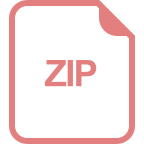

林当时
- 粉丝: 95
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

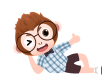
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0